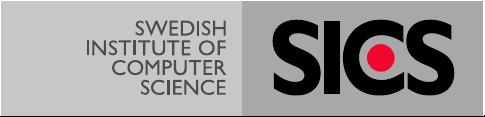
The uIP Embedded TCP/IP Stack
The uIP 1.0 Reference Manual
June 2006
Adam Dunkels
adam@sics.se
Swedish Institute of Computer Science
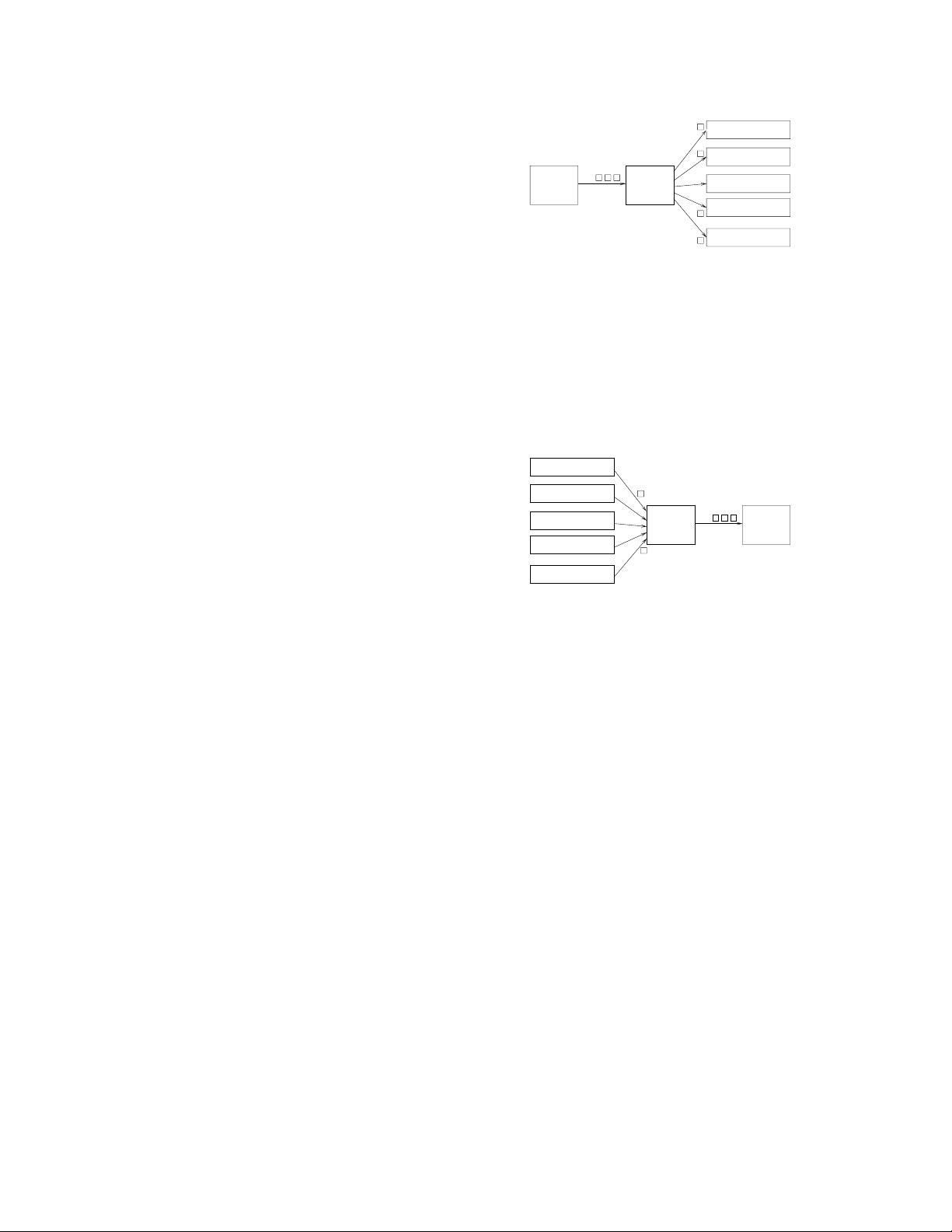
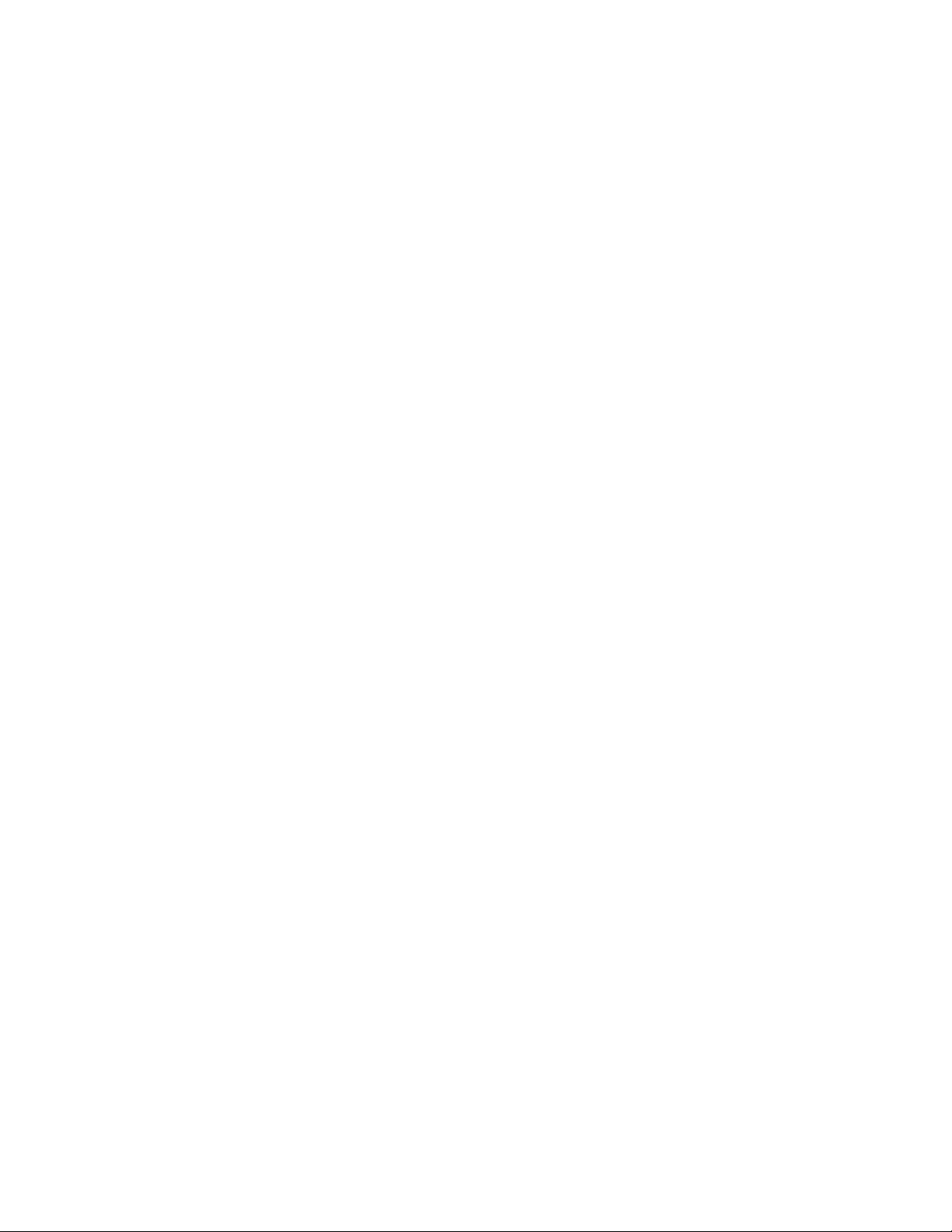
Contents
1 The uIP TCP/IP stack 1
1.1 Introduction . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 1
1.2 TCP/IP Communication . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2
1.3 Main Control Loop . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2
1.4 Architecture Specific Functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
1.5 Memory Management . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 3
1.6 Application Program Interface (API) . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
1.7 Examples . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 7
1.8 Protocol Implementations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 14
1.9 Performance . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 16
2 uIP 1.0 Module Index 19
2.1 uIP 1.0 Modules . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 19
3 uIP 1.0 Hierarchical Index 21
3.1 uIP 1.0 Class Hierarchy . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 21
4 uIP 1.0 Data Structure Index 23
4.1 uIP 1.0 Data Structures . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 23
5 uIP 1.0 File Index 25
5.1 uIP 1.0 File List . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 25
6 uIP 1.0 Module Documentation 27
6.1 Protothreads . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 27
6.2 Applications . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 36
6.3 uIP configuration functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 37
6.4 uIP initialization functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 40
6.5 uIP device driver functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 41
6.6 uIP application functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 46
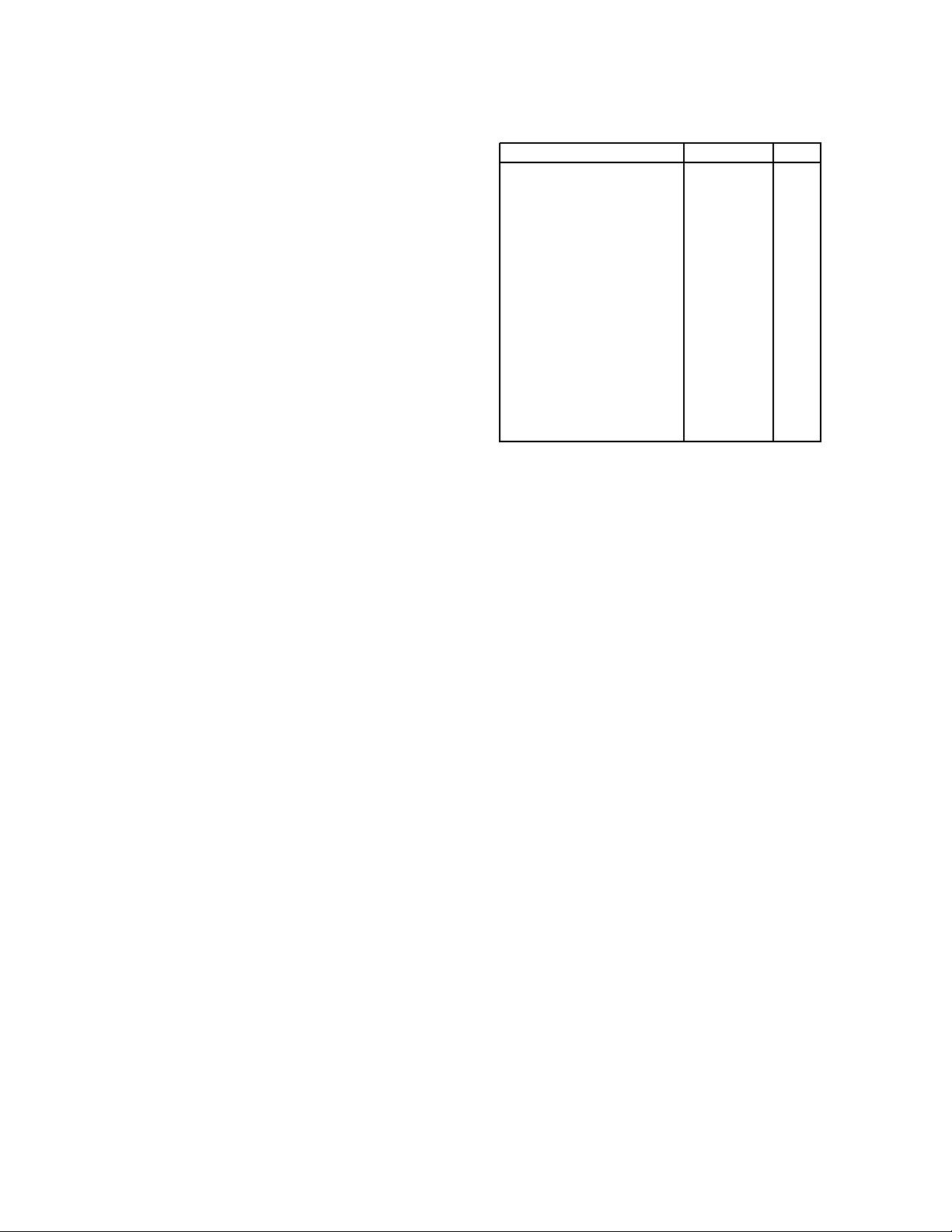
ii CONTENTS
6.7 uIP conversion functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 55
6.8 Variables used in uIP device drivers . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 61
6.9 The uIP TCP/IP stack . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 62
6.10 Architecture specific uIP functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 74
6.11 uIP Address Resolution Protocol . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 76
6.12 Configuration options for uIP . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 79
6.13 uIP TCP throughput booster hack . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 89
6.14 Local continuations . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 90
6.15 Timer library . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 91
6.16 Clock interface . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 94
6.17 Protosockets library . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 95
6.18 Memory block management functions . . . . . . . . . . . . . . . . . . . . . . . . . . . . 102
6.19 DNS resolver . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 105
6.20 SMTP E-mail sender . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 108
6.21 Telnet server . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 110
6.22 Hello, world . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 113
6.23 Web client . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 114
6.24 Web server . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 120
7 uIP 1.0 Data Structure Documentation 123
7.1 dhcpc_state Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 123
7.2 hello_world_state Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 124
7.3 httpd_cgi_call Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 125
7.4 httpd_state Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 126
7.5 memb_blocks Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 127
7.6 psock Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 128
7.7 psock_buf Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 129
7.8 pt Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 130
7.9 smtp_state Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 131
7.10 telnetd_state Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 132
7.11 timer Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 133
7.12 uip_conn Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 134
7.13 uip_eth_addr Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 136
7.14 uip_eth_hdr Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 137
7.15 uip_icmpip_hdr Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 138
7.16 uip_neighbor_addr Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 139
7.17 uip_stats Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 140
Generated on Mon Jun 12 10:23:20 2006 for uIP 1.0 by Doxygen
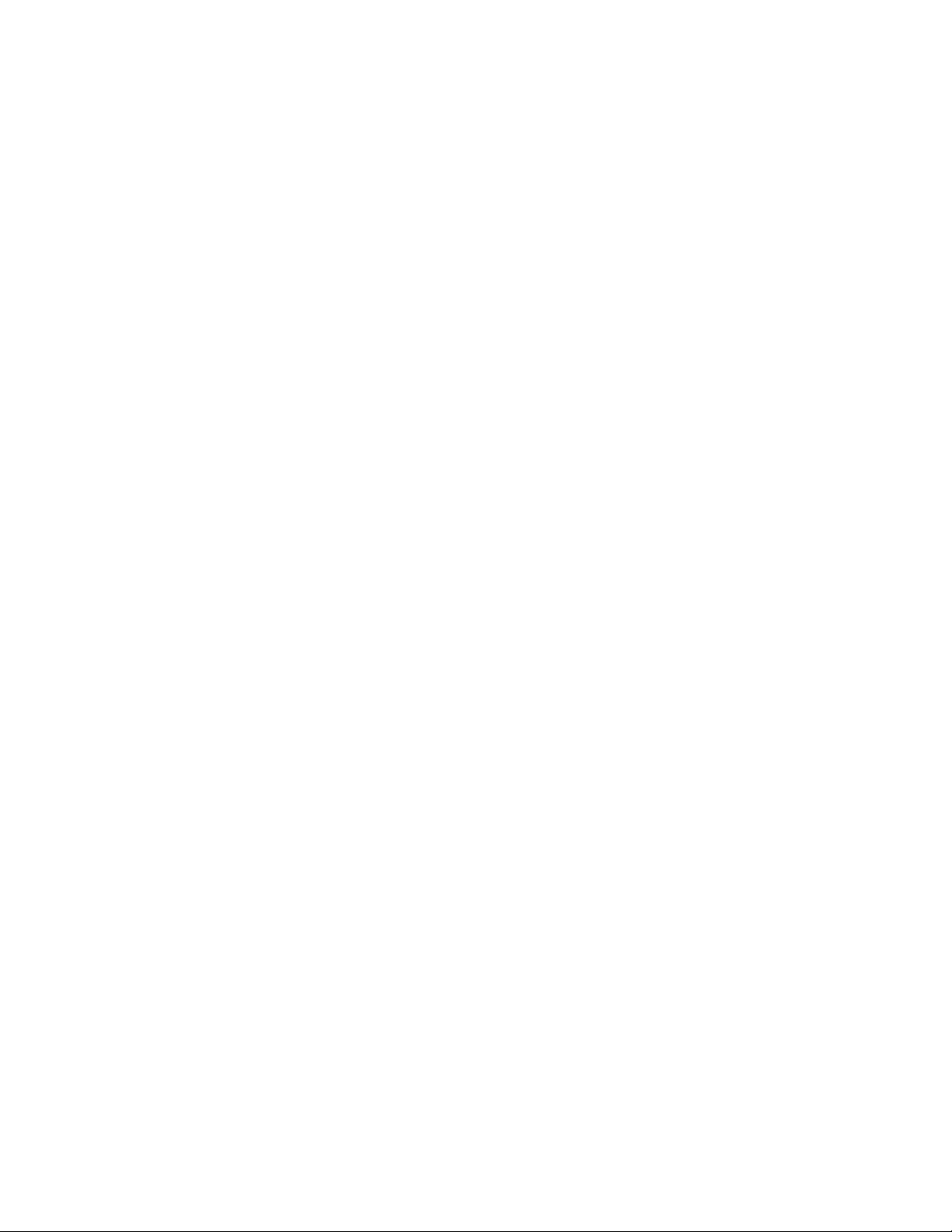
CONTENTS iii
7.18 uip_tcpip_hdr Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 142
7.19 uip_udp_conn Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 143
7.20 uip_udpip_hdr Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 144
7.21 webclient_state Struct Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 145
8 uIP 1.0 File Documentation 147
8.1 apps/hello-world/hello-world.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . 147
8.2 apps/hello-world/hello-world.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . 148
8.3 apps/resolv/resolv.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 149
8.4 apps/resolv/resolv.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 151
8.5 apps/smtp/smtp.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 153
8.6 apps/smtp/smtp.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 154
8.7 apps/telnetd/shell.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 156
8.8 apps/telnetd/shell.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 157
8.9 apps/telnetd/telnetd.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 158
8.10 apps/telnetd/telnetd.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 159
8.11 apps/webclient/webclient.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . 160
8.12 apps/webclient/webclient.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . 162
8.13 apps/webserver/httpd-cgi.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . 164
8.14 apps/webserver/httpd-cgi.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . 165
8.15 apps/webserver/httpd.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . 166
8.16 lib/memb.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 167
8.17 lib/memb.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 168
8.18 uip/lc-addrlabels.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 169
8.19 uip/lc-switch.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 170
8.20 uip/lc.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 171
8.21 uip/psock.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 172
8.22 uip/pt.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 174
8.23 uip/timer.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 176
8.24 uip/timer.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 177
8.25 uip/uip-neighbor.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 178
8.26 uip/uip-neighbor.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 179
8.27 uip/uip-split.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 180
8.28 uip/uip.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 181
8.29 uip/uip.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 184
8.30 uip/uip_arch.h File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 190
8.31 uip/uip_arp.c File Reference . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 191
Generated on Mon Jun 12 10:23:20 2006 for uIP 1.0 by Doxygen