package com.linkwechat.service.impl;
import cn.hutool.core.collection.CollectionUtil;
import cn.hutool.core.collection.ListUtil;
import cn.hutool.core.util.ArrayUtil;
import cn.hutool.core.util.ObjectUtil;
import com.alibaba.fastjson.JSONObject;
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.google.common.collect.Lists;
import com.linkwechat.common.annotation.SynchRecord;
import com.linkwechat.common.constant.Constants;
import com.linkwechat.common.constant.HttpStatus;
import com.linkwechat.common.constant.SynchRecordConstants;
import com.linkwechat.common.constant.WeConstans;
import com.linkwechat.common.context.SecurityContextHolder;
import com.linkwechat.common.core.domain.AjaxResult;
import com.linkwechat.common.core.domain.entity.SysUser;
import com.linkwechat.common.core.domain.model.LoginUser;
import com.linkwechat.common.core.page.PageDomain;
import com.linkwechat.common.core.page.TableDataInfo;
import com.linkwechat.common.enums.CustomerAddWay;
import com.linkwechat.common.enums.MessageNoticeType;
import com.linkwechat.common.enums.TrajectorySceneType;
import com.linkwechat.common.enums.WeErrorCodeEnum;
import com.linkwechat.common.exception.wecom.WeComException;
import com.linkwechat.common.utils.DateUtils;
import com.linkwechat.common.utils.SecurityUtils;
import com.linkwechat.common.utils.SnowFlakeUtil;
import com.linkwechat.common.utils.StringUtils;
import com.linkwechat.common.utils.bean.BeanUtils;
import com.linkwechat.config.rabbitmq.RabbitMQSettingConfig;
import com.linkwechat.domain.*;
import com.linkwechat.domain.customer.WeBacthMakeCustomerTag;
import com.linkwechat.domain.customer.WeMakeCustomerTag;
import com.linkwechat.domain.customer.query.WeCustomersQuery;
import com.linkwechat.domain.customer.query.WeOnTheJobCustomerQuery;
import com.linkwechat.domain.customer.vo.*;
import com.linkwechat.domain.groupmsg.vo.WeGroupMessageExecuteUsertipVo;
import com.linkwechat.domain.tag.vo.WeTagVo;
import com.linkwechat.domain.wecom.entity.customer.WeCustomerFollowInfoEntity;
import com.linkwechat.domain.wecom.entity.customer.WeCustomerFollowUserEntity;
import com.linkwechat.domain.wecom.query.WeBaseQuery;
import com.linkwechat.domain.wecom.query.customer.UnionidToExternalUserIdQuery;
import com.linkwechat.domain.wecom.query.customer.WeBatchCustomerQuery;
import com.linkwechat.domain.wecom.query.customer.WeCustomerQuery;
import com.linkwechat.domain.wecom.query.customer.tag.WeMarkTagQuery;
import com.linkwechat.domain.wecom.query.customer.transfer.WeTransferCustomerQuery;
import com.linkwechat.domain.wecom.vo.customer.UnionidToExternalUserIdVo;
import com.linkwechat.domain.wecom.vo.customer.WeBatchCustomerDetailVo;
import com.linkwechat.domain.wecom.vo.customer.WeCustomerDetailVo;
import com.linkwechat.domain.wecom.vo.customer.WeFollowUserListVo;
import com.linkwechat.domain.wecom.vo.customer.transfer.WeTransferCustomerVo;
import com.linkwechat.fegin.QwCustomerClient;
import com.linkwechat.mapper.WeCustomerMapper;
import com.linkwechat.service.*;
import org.springframework.amqp.rabbit.core.RabbitTemplate;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Lazy;
import org.springframework.scheduling.annotation.Async;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import java.util.*;
import java.util.function.Function;
import java.util.stream.Collectors;
@Service
public class WeCustomerServiceImpl extends ServiceImpl<WeCustomerMapper, WeCustomer> implements IWeCustomerService {
@Autowired
private QwCustomerClient qwCustomerClient;
@Autowired
private IWeFlowerCustomerTagRelService iWeFlowerCustomerTagRelService;
@Autowired
private IWeTagService iWeTagService;
@Autowired
private IWeCustomerTrajectoryService iWeCustomerTrajectoryService;
@Autowired
private IWeAllocateCustomerService iWeAllocateCustomerService;
@Autowired
@Lazy
private IWeGroupService iWeGroupService;
@Autowired
private RabbitMQSettingConfig rabbitMQSettingConfig;
@Autowired
private RabbitTemplate rabbitTemplate;
@Autowired
private IWeMessagePushService iWeMessagePushService;
@Autowired
private IWeCustomerSeasService iWeCustomerSeasService;
@Autowired
private IWeCustomerInfoExpandService iWeCustomerInfoExpandService;
@Autowired
@Lazy
private IWeFissionService iWeFissionService;
@Override
public List<WeCustomersVo> findWeCustomerList(WeCustomersQuery weCustomersQuery, PageDomain pageDomain) {
List<WeCustomersVo> weCustomersVos = new ArrayList<>();
List<String> ids = this.baseMapper.findWeCustomerListIds(weCustomersQuery, pageDomain);
if (CollectionUtil.isNotEmpty(ids)) {
weCustomersVos = this.baseMapper.findWeCustomerList(ids);
}
return weCustomersVos;
}
@Override
public TableDataInfo<List<WeCustomersVo>> findWeCustomerListByApp(WeCustomersQuery weCustomersQuery, PageDomain pageDomain) {
TableDataInfo<List<WeCustomersVo>> tableDataInfo = new TableDataInfo<>();
tableDataInfo.setCode(HttpStatus.SUCCESS);
if (weCustomersQuery.getDataScope()) {//这里指的全部数据,是角色的那些数据
tableDataInfo.setRows(
this.findWeCustomerList(weCustomersQuery, pageDomain)
);
tableDataInfo.setTotal(
this.countWeCustomerList(weCustomersQuery)
);
} else {//个人数据
weCustomersQuery.setFirstUserId(
SecurityUtils.getLoginUser().getSysUser().getWeUserId()
);
List<String> customerIds = this.baseMapper.findWeCustomerListIdsByApp(weCustomersQuery, pageDomain);
if (CollectionUtil.isNotEmpty(customerIds)) {
tableDataInfo.setRows(
this.baseMapper.findWeCustomerList(customerIds)
);
tableDataInfo.setTotal(
this.countWeCustomerListByApp(weCustomersQuery)
);
}
}
List<WeCustomersVo> rows = tableDataInfo.getRows();
if (CollectionUtil.isEmpty(rows)) {
tableDataInfo.setRows(new ArrayList<>());
}
return tableDataInfo;
}
@Override
public long countWeCustomerList(WeCustomersQuery weCustomersQuery) {
return this.baseMapper.countWeCustomerList(weCustomersQuery);
}
@Override
public long countWeCustomerListByApp(WeCustomersQuery weCustomersQuery) {
return this.baseMapper.countWeCustomerListByApp(weCustomersQuery);
}
@Override
public long noRepeatCountCustomer(WeCustomersQuery weCustomersQuery) {
return this.baseMapper.noRepeatCountCustomer(weCustomersQuery);
}
@Override
@SynchRecord(synchType = SynchRecordConstants.SYNCH_CUSTOMER)
public void synchWeCustomer() {
LoginUser loginUser = SecurityUtils.getLoginUser();
rabbitTemplate.convertAndSend(rabbitMQSettingConfig.getWeSyncEx(), rabbitMQSettingConfig.getWeCustomerRk(), JSONObject.toJSONString(loginUser));
}
@Override
@Async
public void synchWeCustomerHandler(String msg) {
LoginUser loginUser = JSONObject.parseObject(msg, LoginUser.class);
SecurityContextHolder.setCorpId(loginUser.getCorpId());
SecurityContextHolder.setUserName(loginUser.getUserName());
SecurityContextHolder.setUserId(String.valueOf(loginUser.getSysUser().getUserId()));
SecurityContextHolder.setUserType(loginUser.getUserType());
WeFollowUserListVo followUserList = qwCustomerClient.getFollowUserList(new WeBaseQuery()).getData();
if (null != followUserList && CollectionUtil.isNotEmpty(followUserList.getFollowUser())) {
this.synchWe
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
LinkWeChat 是基于企业微信的开源 SCRM 系统,采用主流的 Java 微服务架构,是企业私域流量管理与营销的综合解决方案,助力企业提高客户运营效率,强化营销能力,拓展盈利空间。
资源推荐
资源详情
资源评论
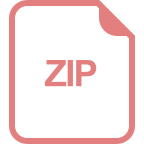
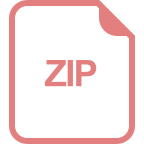
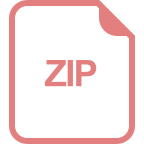
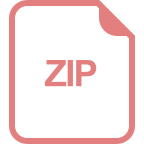
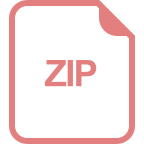
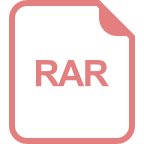
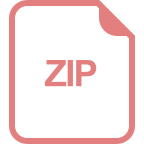
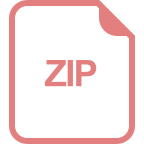
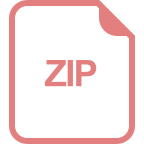
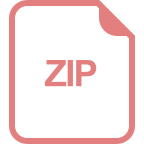
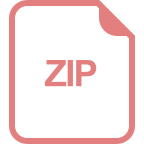
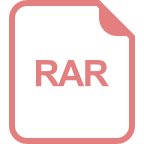
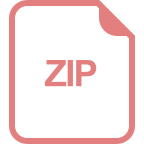
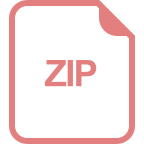
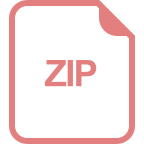
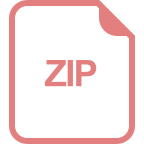
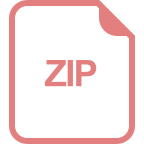
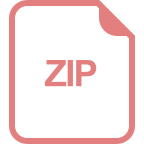
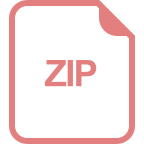
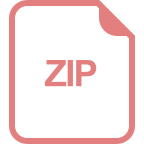
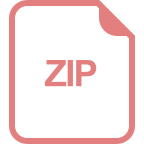
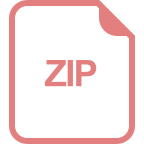
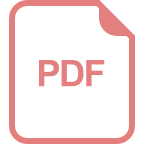
收起资源包目录

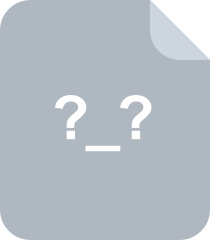
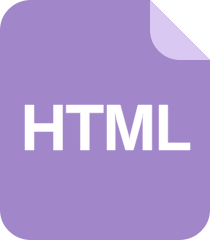
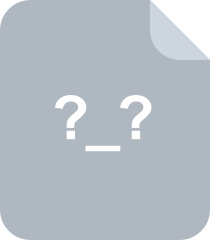
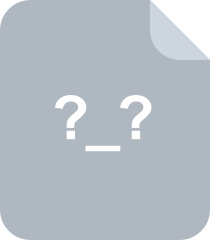
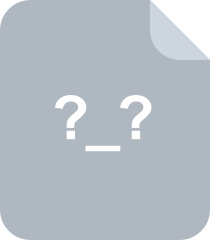
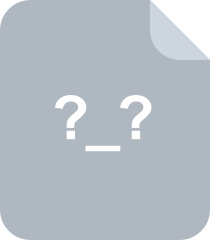
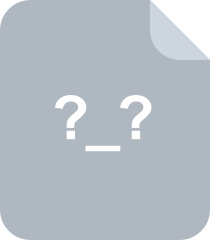
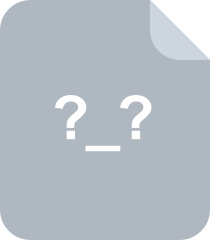
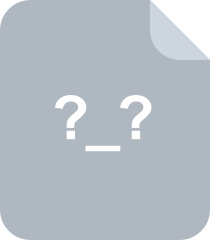
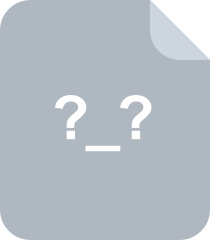
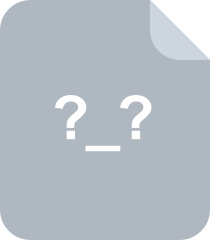
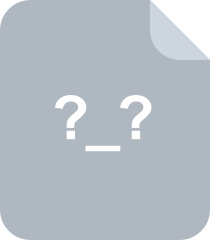
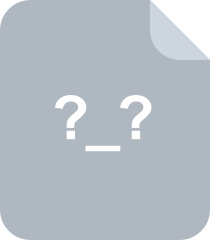
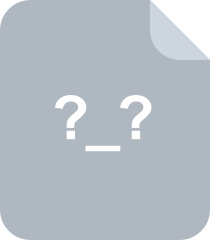
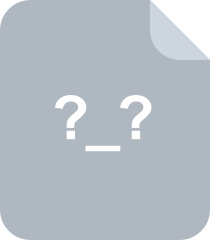
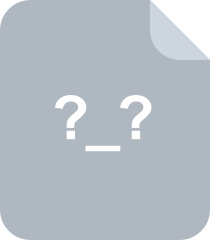
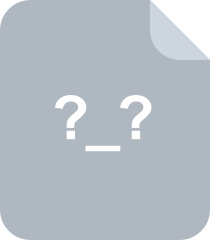
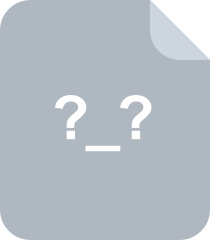
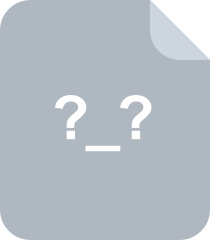
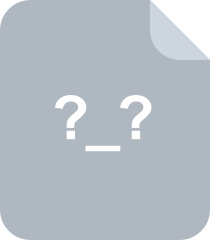
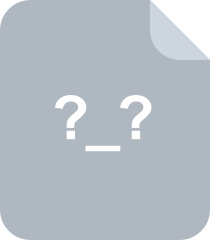
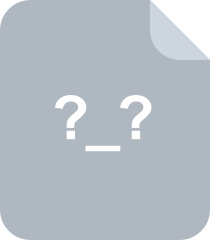
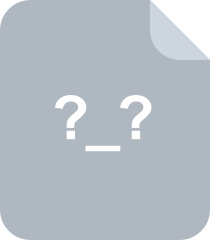
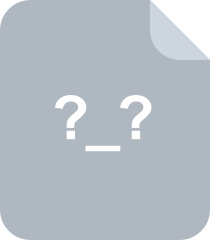
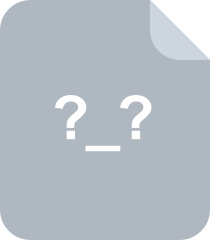
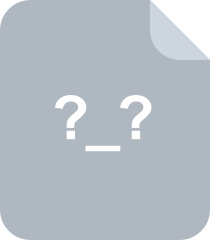
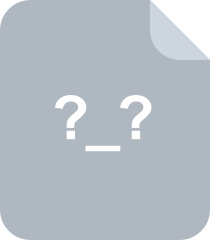
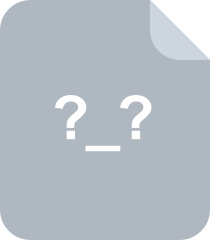
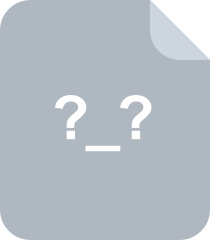
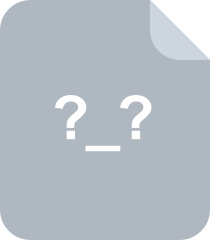
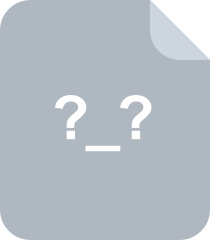
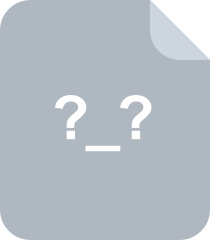
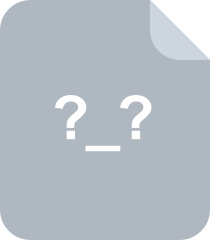
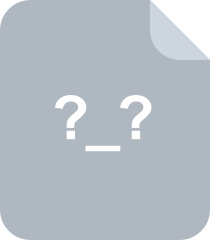
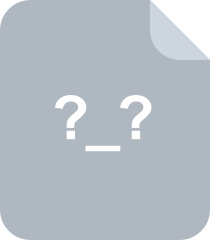
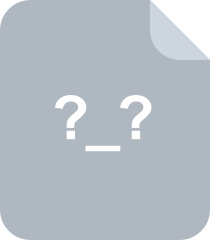
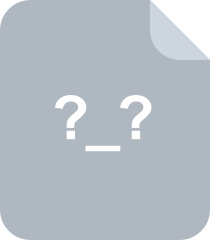
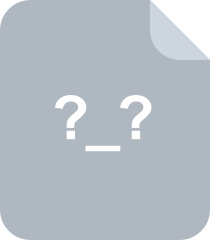
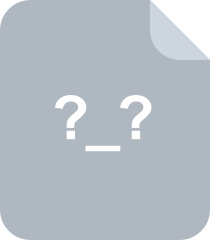
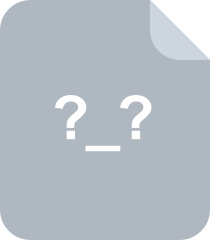
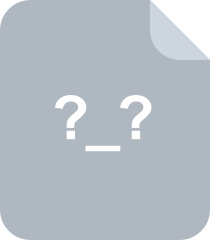
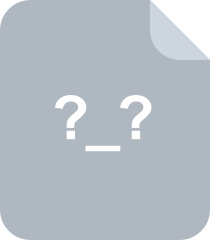
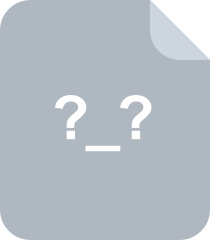
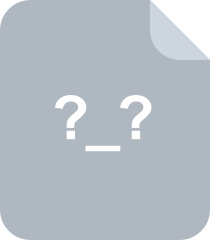
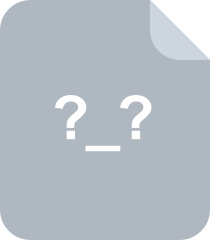
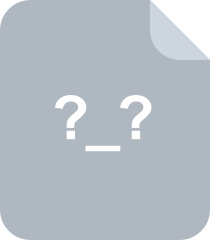
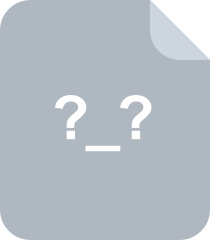
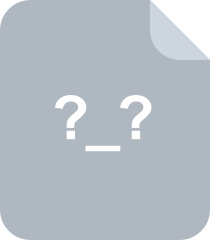
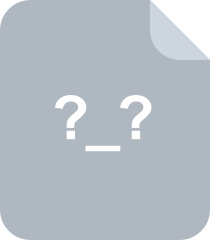
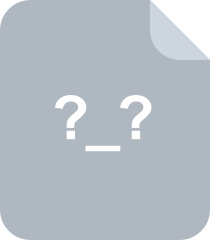
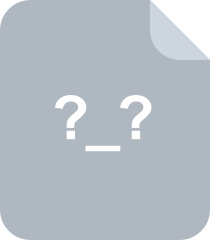
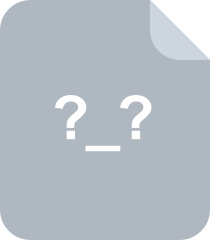
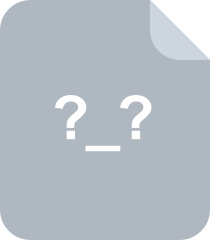
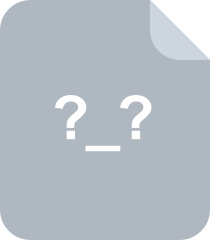
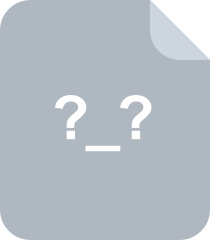
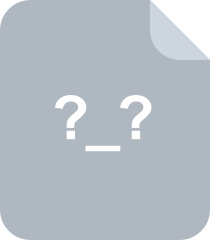
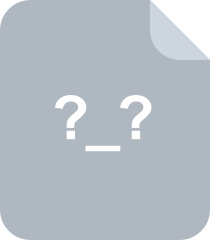
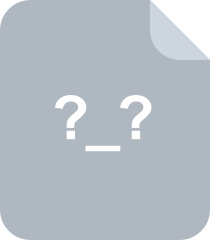
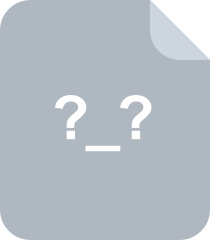
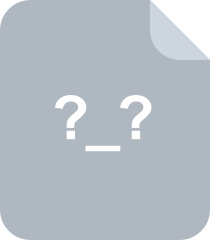
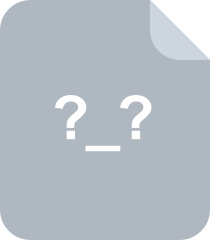
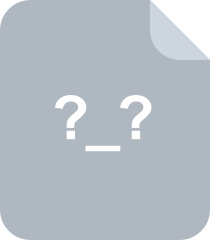
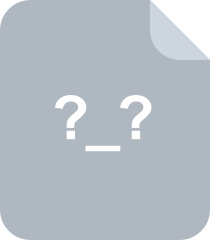
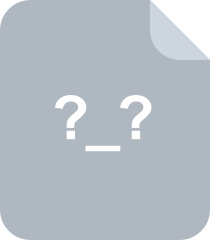
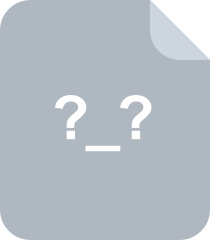
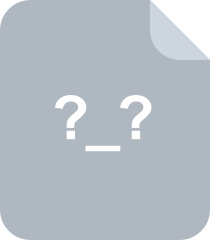
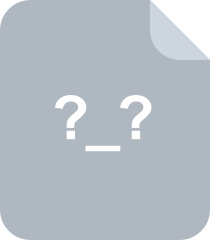
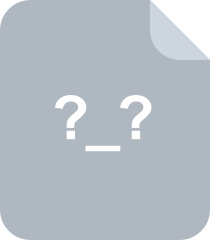
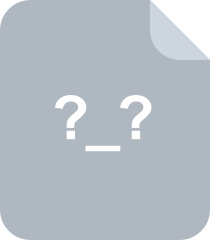
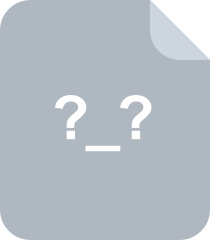
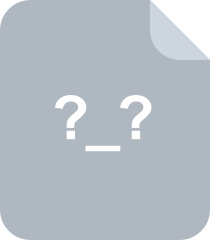
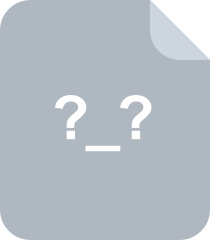
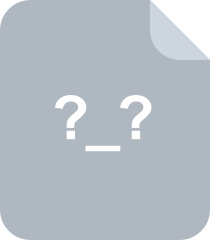
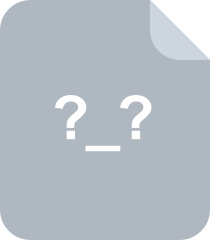
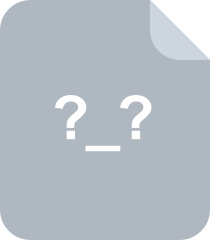
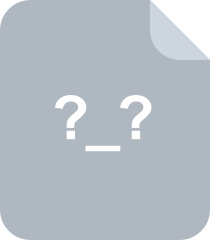
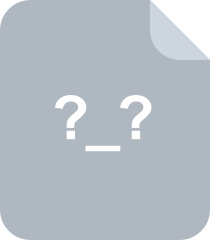
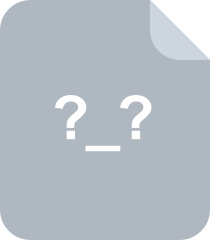
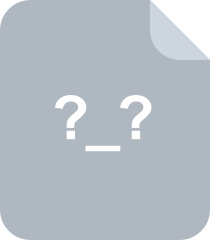
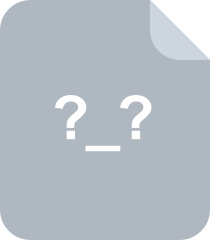
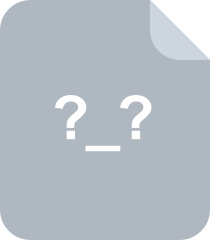
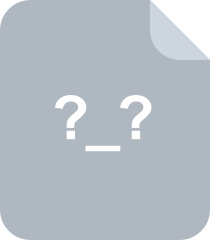
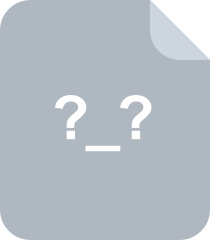
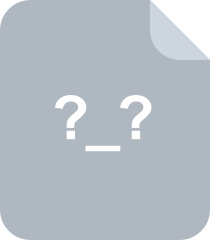
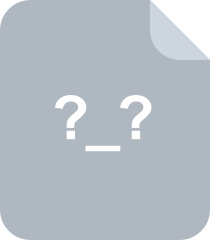
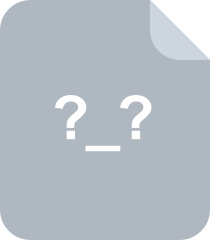
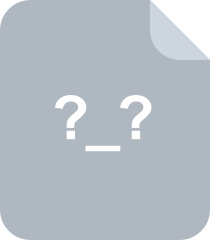
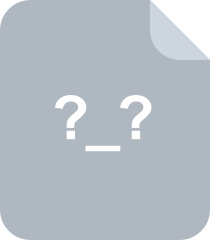
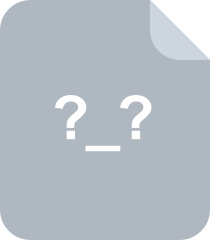
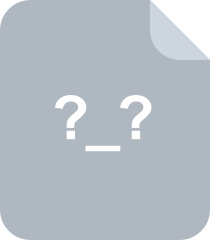
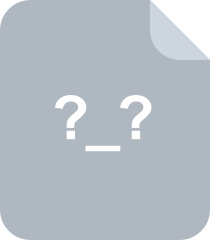
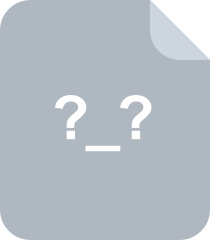
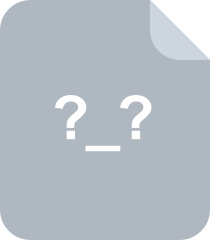
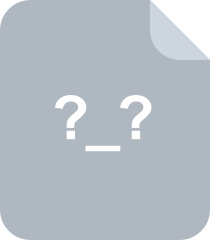
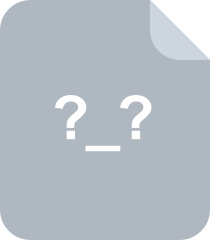
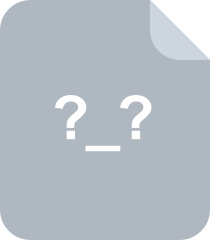
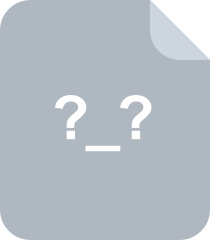
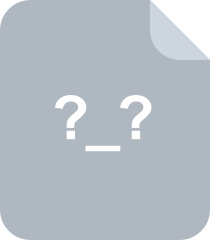
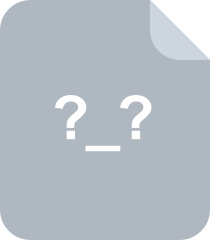
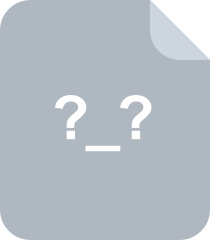
共 1835 条
- 1
- 2
- 3
- 4
- 5
- 6
- 19
资源评论
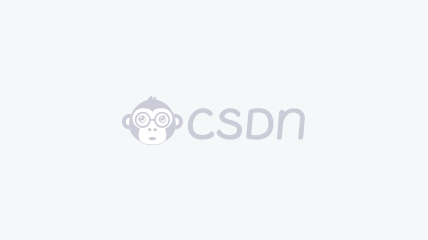
- weixin_453956132023-08-07资源太好了,解决了我当下遇到的难题,抱紧大佬的大腿~

Java程序员-张凯
- 粉丝: 1w+
- 资源: 7361

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

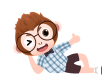
最新资源
- (源码)基于JavaFX和MySQL的医院挂号管理系统.zip
- (源码)基于IdentityServer4和Finbuckle.MultiTenant的多租户身份认证系统.zip
- (源码)基于Spring Boot和Vue3+ElementPlus的后台管理系统.zip
- (源码)基于C++和Qt框架的dearoot配置管理系统.zip
- (源码)基于 .NET 和 EasyHook 的虚拟文件系统.zip
- (源码)基于Python的金融文档智能分析系统.zip
- (源码)基于Java的医药管理系统.zip
- (源码)基于Java和MySQL的学生信息管理系统.zip
- (源码)基于ASP.NET Core的零售供应链管理系统.zip
- (源码)基于PythonSpleeter的戏曲音频处理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


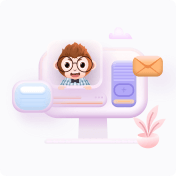
安全验证
文档复制为VIP权益,开通VIP直接复制
