package com.gt.utils;
import java.util.ArrayList;
import java.util.List;
public class Des {
public Des() {
}
public static void main(String[] args) {
Des desObj = new Des();
String key1 = "1";
String key2 = "2";
String key3 = "3";
String data = "admin";
String str = desObj.strEnc(data, key1, key2, key3);
System.out.println(str);
String dec = desObj.strDec(str, key1, key2, key3);
System.out.println(dec);
}
/**
* DES加密/解密
*
* @Copyright Copyright (c) 2006
* @author Guapo
* @see DESCore
*/
/*
* encrypt the string to string made up of hex return the encrypted string
*/
public String strEnc(String data, String firstKey, String secondKey,
String thirdKey) {
int leng = data.length();
String encData = "";
List firstKeyBt = null, secondKeyBt = null, thirdKeyBt = null;
int firstLength = 0, secondLength = 0, thirdLength = 0;
if (firstKey != null && firstKey != "") {
firstKeyBt = getKeyBytes(firstKey);
firstLength = firstKeyBt.size();
}
if (secondKey != null && secondKey != "") {
secondKeyBt = getKeyBytes(secondKey);
secondLength = secondKeyBt.size();
}
if (thirdKey != null && thirdKey != "") {
thirdKeyBt = getKeyBytes(thirdKey);
thirdLength = thirdKeyBt.size();
}
if (leng > 0) {
if (leng < 4) {
int[] bt = strToBt(data);
int[] encByte = null;
if (firstKey != null && firstKey != "" && secondKey != null
&& secondKey != "" && thirdKey != null
&& thirdKey != "") {
int[] tempBt;
int x, y, z;
tempBt = bt;
for (x = 0; x < firstLength; x++) {
tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));
}
for (y = 0; y < secondLength; y++) {
tempBt = enc(tempBt, (int[]) secondKeyBt.get(y));
}
for (z = 0; z < thirdLength; z++) {
tempBt = enc(tempBt, (int[]) thirdKeyBt.get(z));
}
encByte = tempBt;
} else {
if (firstKey != null && firstKey != "" && secondKey != null
&& secondKey != "") {
int[] tempBt;
int x, y;
tempBt = bt;
for (x = 0; x < firstLength; x++) {
tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));
}
for (y = 0; y < secondLength; y++) {
tempBt = enc(tempBt, (int[]) secondKeyBt.get(y));
}
encByte = tempBt;
} else {
if (firstKey != null && firstKey != "") {
int[] tempBt;
int x = 0;
tempBt = bt;
for (x = 0; x < firstLength; x++) {
tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));
}
encByte = tempBt;
}
}
}
encData = bt64ToHex(encByte);
} else {
int iterator = (leng / 4);
int remainder = leng % 4;
int i = 0;
for (i = 0; i < iterator; i++) {
String tempData = data.substring(i * 4 + 0, i * 4 + 4);
int[] tempByte = strToBt(tempData);
int[] encByte = null;
if (firstKey != null && firstKey != "" && secondKey != null
&& secondKey != "" && thirdKey != null
&& thirdKey != "") {
int[] tempBt;
int x, y, z;
tempBt = tempByte;
for (x = 0; x < firstLength; x++) {
tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));
}
for (y = 0; y < secondLength; y++) {
tempBt = enc(tempBt, (int[]) secondKeyBt.get(y));
}
for (z = 0; z < thirdLength; z++) {
tempBt = enc(tempBt, (int[]) thirdKeyBt.get(z));
}
encByte = tempBt;
} else {
if (firstKey != null && firstKey != ""
&& secondKey != null && secondKey != "") {
int[] tempBt;
int x, y;
tempBt = tempByte;
for (x = 0; x < firstLength; x++) {
tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));
}
for (y = 0; y < secondLength; y++) {
tempBt = enc(tempBt, (int[]) secondKeyBt.get(y));
}
encByte = tempBt;
} else {
if (firstKey != null && firstKey != "") {
int[] tempBt;
int x;
tempBt = tempByte;
for (x = 0; x < firstLength; x++) {
tempBt = enc(tempBt, (int[]) firstKeyBt
.get(x));
}
encByte = tempBt;
}
}
}
encData += bt64ToHex(encByte);
}
if (remainder > 0) {
String remainderData = data.substring(iterator * 4 + 0,
leng);
int[] tempByte = strToBt(remainderData);
int[] encByte = null;
if (firstKey != null && firstKey != "" && secondKey != null
&& secondKey != "" && thirdKey != null
&& thirdKey != "") {
int[] tempBt;
int x, y, z;
tempBt = tempByte;
for (x = 0; x < firstLength; x++) {
tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));
}
for (y = 0; y < secondLength; y++) {
tempBt = enc(tempBt, (int[]) secondKeyBt.get(y));
}
for (z = 0; z < thirdLength; z++) {
tempBt = enc(tempBt, (int[]) thirdKeyBt.get(z));
}
encByte = tempBt;
} else {
if (firstKey != null && firstKey != ""
&& secondKey != null && secondKey != "") {
int[] tempBt;
int x, y;
tempBt = tempByte;
for (x = 0; x < firstLength; x++) {
tempBt = enc(tempBt, (int[]) firstKeyBt.get(x));
}
for (y = 0; y < secondLength; y++) {
tempBt = enc(tempBt, (int[]) secondKeyBt.get(y));
}
encByte = tempBt;
} else {
if (
没有合适的资源?快使用搜索试试~ 我知道了~
layui+java ssh快速开发框架系统源码.zip
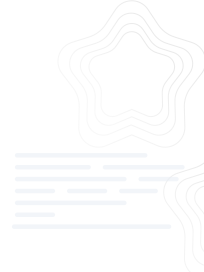
共589个文件
java:120个
class:120个
gif:79个

需积分: 5 0 下载量 113 浏览量
2023-08-30
14:34:53
上传
评论
收藏 25.14MB ZIP 举报
温馨提示
基于layui+SpringMVC+Spring+Hibernate+Mysql搭建而成,内置代码生成器,能够快速生成增删改查代码,节省开发时间,快速构建企业级的web应用系统。 该框架具备一整套完整的权限管理系统,代码简洁,容易入门,适合开发者研究学习。 系统功能:菜单管理角色管理系统功能数据字典机构信息URL拦截用户管理日志管理代码生成器定时任务
资源推荐
资源详情
资源评论
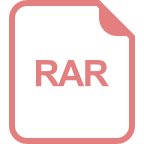
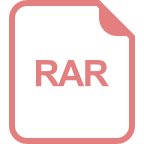
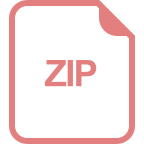
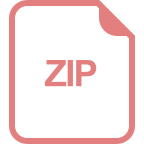
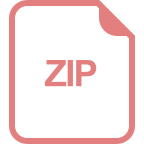
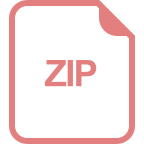
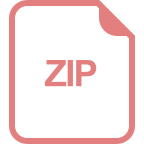
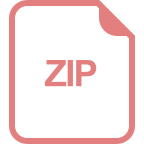
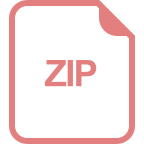
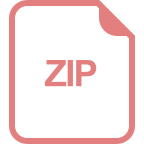
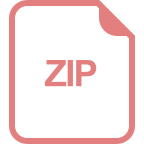
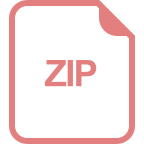
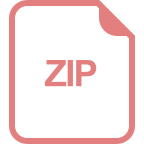
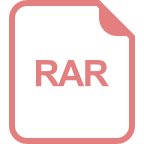
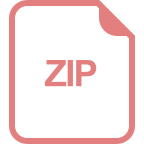
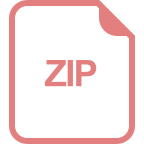
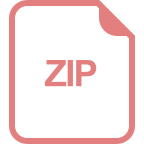
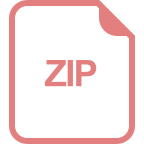
收起资源包目录

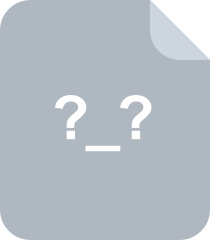
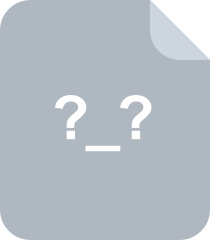
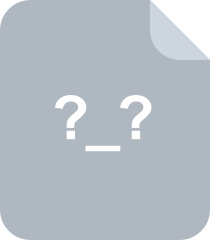
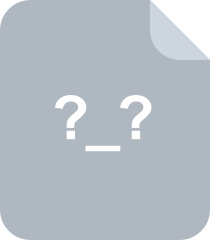
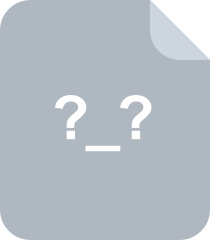
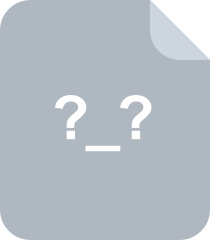
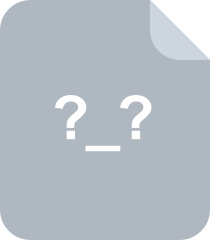
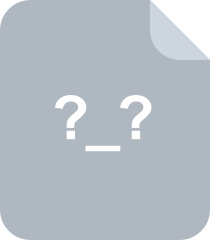
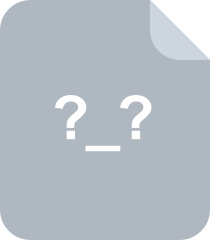
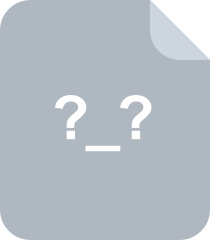
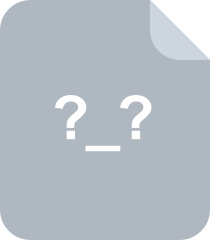
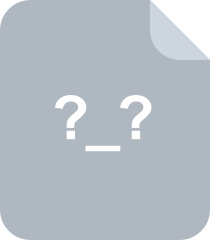
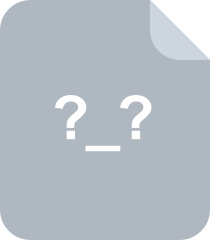
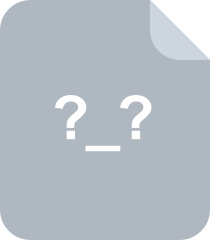
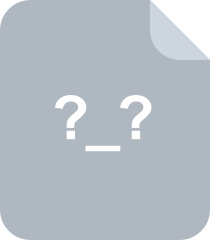
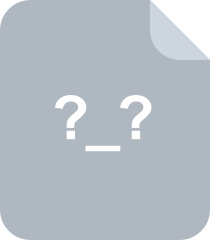
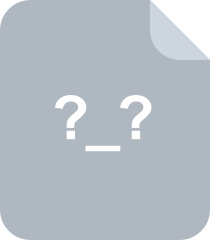
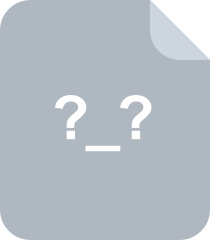
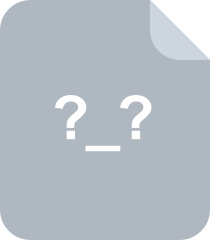
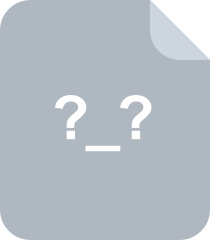
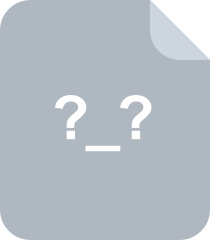
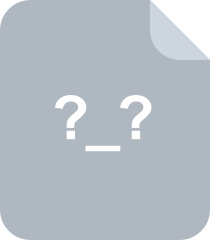
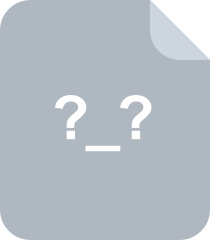
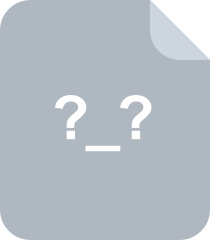
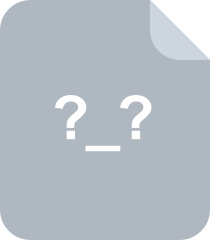
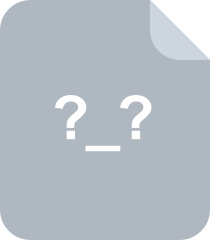
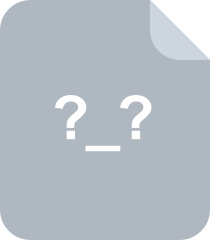
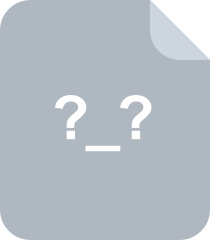
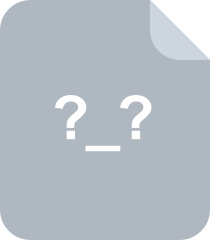
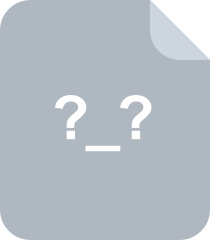
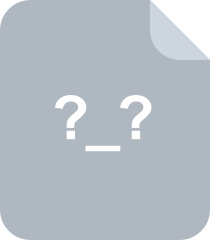
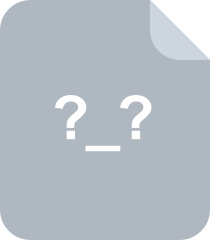
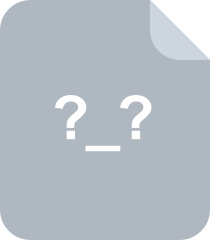
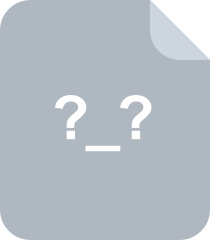
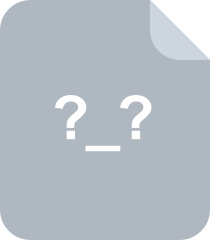
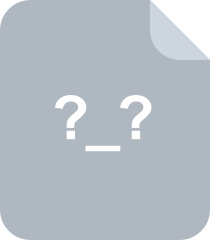
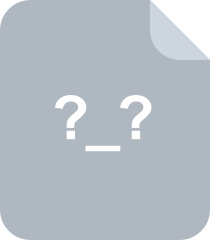
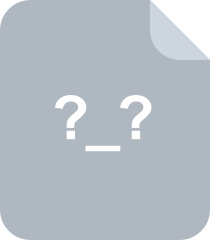
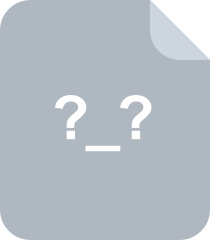
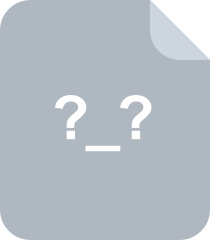
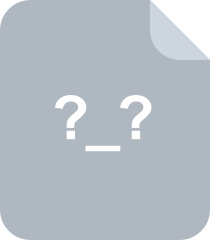
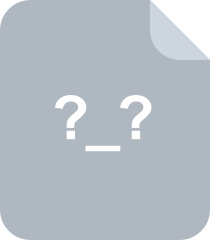
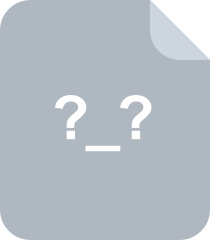
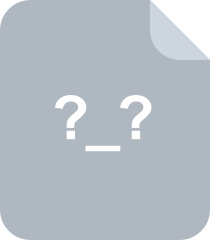
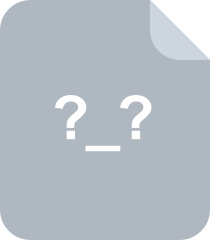
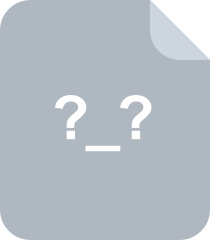
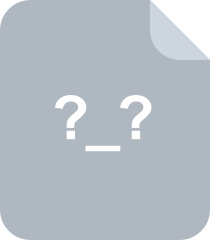
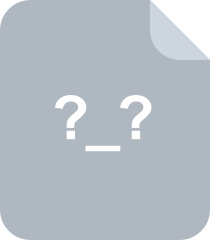
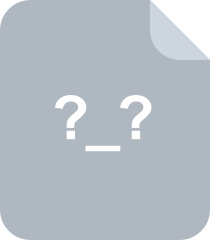
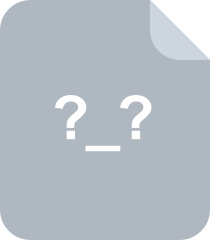
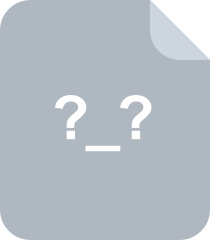
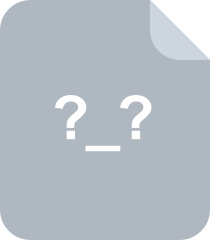
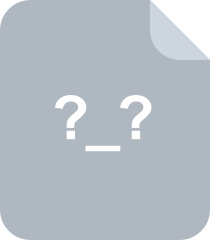
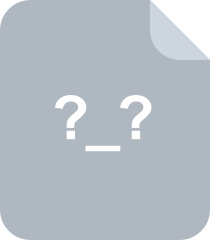
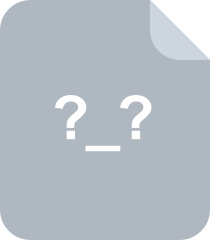
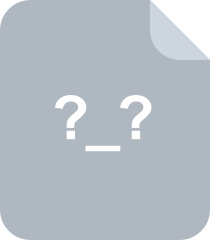
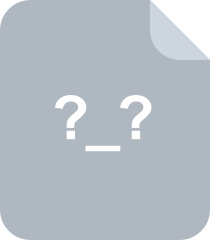
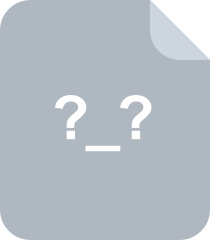
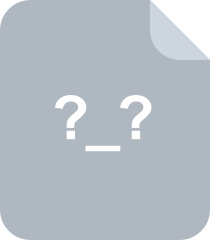
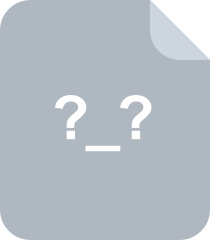
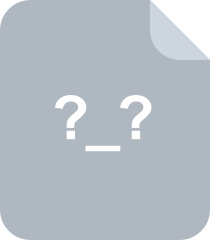
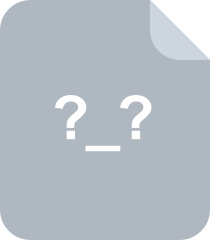
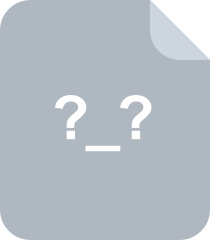
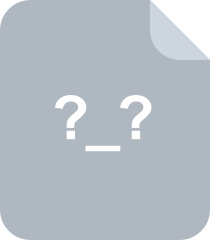
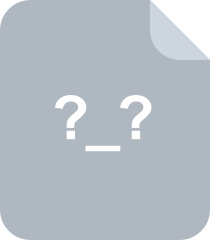
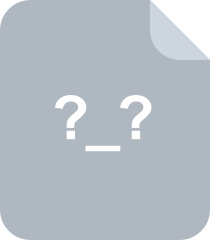
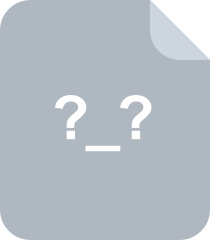
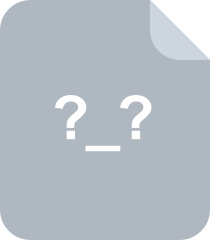
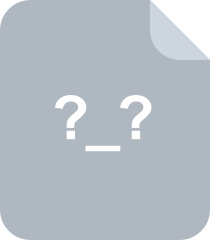
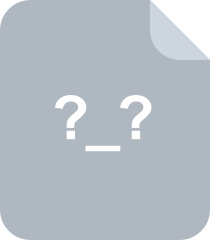
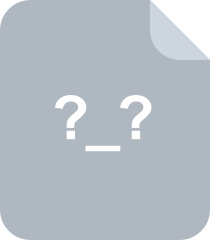
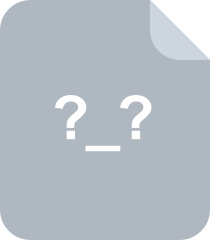
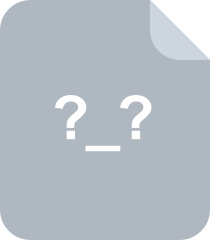
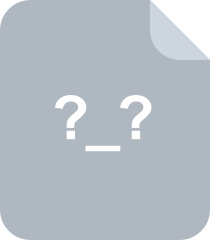
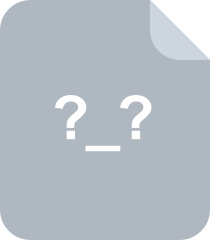
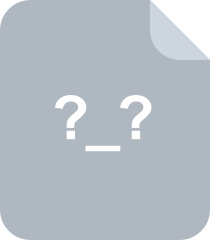
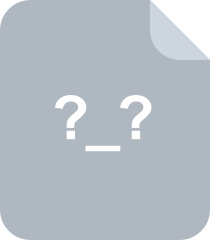
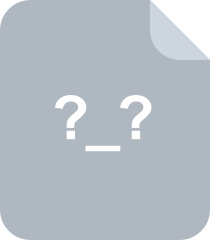
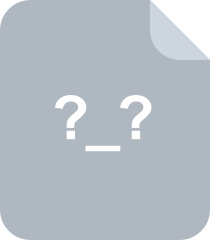
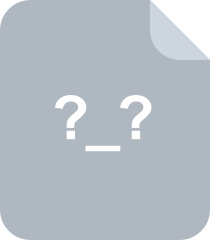
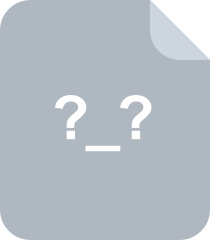
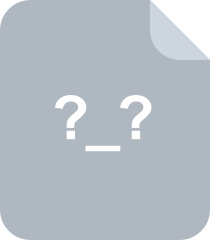
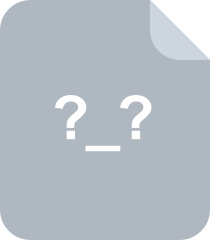
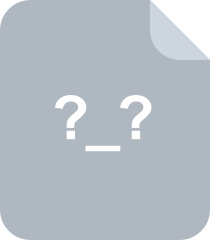
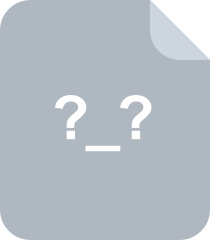
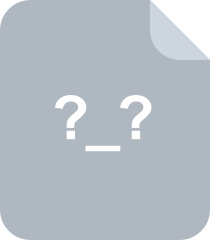
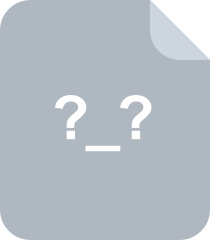
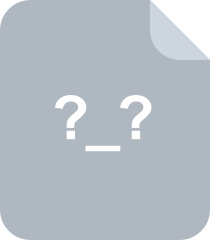
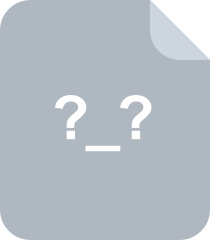
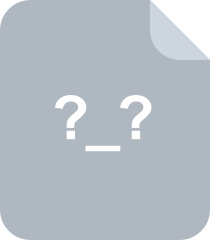
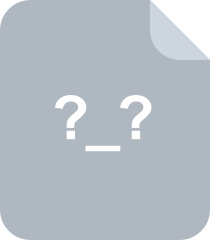
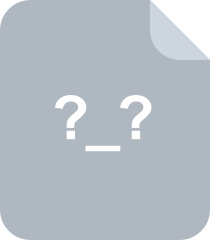
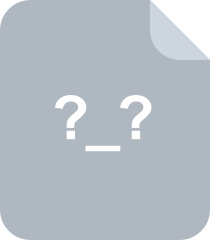
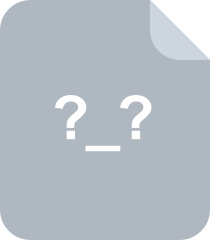
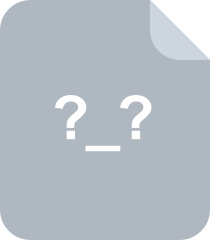
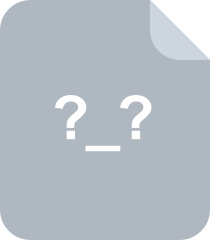
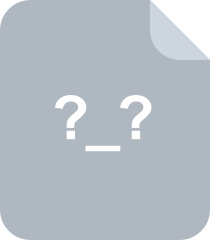
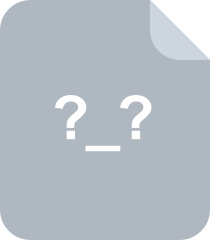
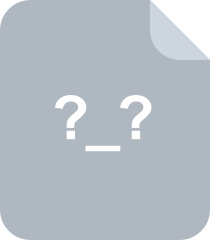
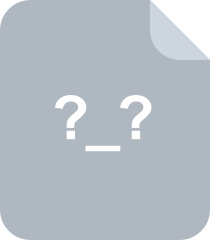
共 589 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
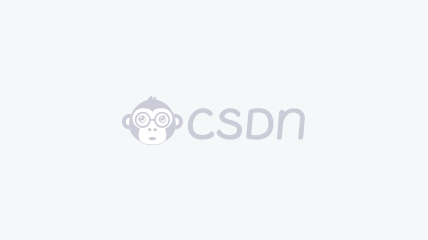

码云笔记
- 粉丝: 3w+
- 资源: 5852
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

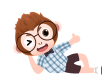
最新资源
- 云计算,搭建分布式,然后实现Titantic数据集训练、分类的的代码
- 同城宠物照看-JAVA-基于Spring Boot的同城宠物照看系统的设计与实现(毕业论文)
- 云计算,实现中文字频统计代码,课程设计
- weixin138社区互助养老+ssm(论文+源码)-kaic.zip
- 扶贫助农系统-JAVA-基于spring boot扶贫助农系统设计与实现(毕业论文)
- 母婴护理知识共享-JAVA-基于SpringBoot+vue 的母婴护理知识共享系统(毕业论文)
- 番茄叶片图像病害多标签分类,约5600张数据
- 影音互动科普网站-JAVA-基于SpringBoot的哈利波特书影音互动科普网站设计与实现(毕业论文)
- 航空散货调度-JAVA-基于SpringBoot的航空散货调度系统设计与实现(毕业论文)
- 基于Python Scrapy的贝壳找房爬虫程序
- zigbee CC2530无线自组网协议栈实现一个协调器+多个终端的通讯及控制.zip
- 校园二手物品交易-JAVA-基于springBoot的校园二手物品交易系统的设计与实现(毕业论文)
- 计算机视觉项目:Swin-Transformer 【tiny、small、base】模型实现的图像识别项目:番茄病害图像分类
- 功能完善的电商数据智能爬虫采集系统项目全套技术资料.zip
- 青少年心理健康教育网-JAVA-基于springboot的青少年心理健康教育网站的设计与实现(毕业论文)
- 密评流程及商密应用方案解析
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


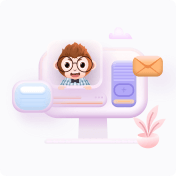
安全验证
文档复制为VIP权益,开通VIP直接复制
