# python-validity
Validity fingerprint sensor driver.
Table of Contents
=================
* [python-validity](#python-validity)
* [Setting up](#setting-up)
* [Error situations](#error-situations)
* [list devices failed ](#list-devices-failed)
* [Errors on startup](#errors-on-startup)
* [Fingerprint not working after waking up from suspend](#fingerprint-not-working-after-waking-up-from-suspend)
* [Enabling fingerprint for system authentication](#enabling-fingerprint-for-system-authentication)
* [The actual change from pam-auth-update](#the-actual-change-from-pam-auth-update)
* [Windows interoperability](#windows-interoperability)
* [Playground](#playground)
* [Initialize a session](#initialize-a-session)
* [Enroll a new user](#enroll-a-new-user)
* [Delete database record (user/finger/whatever)](#delete-database-record-userfingerwhatever)
* [Identify a finger (scan)](#identify-a-finger-scan)
* [DBus service](#dbus-service)
* [Debugging](#debugging)
## Setting up
On Ubuntu system:
```
$ sudo apt remove fprintd
$ sudo add-apt-repository ppa:uunicorn/open-fprintd
$ sudo apt-get update
$ sudo apt install open-fprintd fprintd-clients python3-validity
...wait a bit...
$ fprintd-enroll
```
On Arch Linux
(Or Arch Linux based system, not including Artix)
```
$ yay -S python-validity
(Press Enter twice when prompted)
$ fprintd-enroll
```
On Fedora Linux
```
$ sudo dnf copr enable tigro/python-validity
$ sudo dnf install open-fprintd fprintd-clients fprintd-clients-pam python3-validity
...wait a bit...
$ fprintd-enroll
```
### Error situations
#### List devices failed
If `fprintd-enroll` returns with `list_devices failed:`, you can check
the logs of the `python3-validity` daemon using `$ sudo systemctl status python3-validity`.
If it's not running, you can enable and/or start it by substituting `status` with `enable` or `start`.
#### Errors on startup
It `systemctl status python3-validity` complains about errors on startup, you may need to factory-reset the fingerprint chip. Do that like so:
```
$ sudo systemctl stop python3-validity
$ sudo validity-sensors-firmware
$ sudo python3 /usr/share/python-validity/playground/factory-reset.py
# At some of the above points you may get a 'device busy' error,
# depending on how systemctl plays along. Kill offending processes if
# necessary, or re-run the systemctl stop python3-validity command,
# in case it has automatically been restarted, or or kill other
# offending processes.
$ sudo systemctl start python3-validity
$ fprintd-enroll
```
#### Fingerprint not working after waking up from suspend
Enable *open-fprintd-resume* and *open-fprintd-suspend* services:
```
$ sudo systemctl enable open-fprintd-resume open-fprintd-suspend
```
For even more error procedures, check [this Arch comment thread](https://aur.archlinux.org/packages/python-validity/#comment-755904) or [this python-validity bug comment thread](https://github.com/uunicorn/python-validity/issues/3).
## Enabling fingerprint for system authentication
To enable fingerprint login, if it doesn't come automatically, run
```
$ sudo pam-auth-update
```
and use the space-bar to enable fingerprint authentication.
The change will take effect immediately. At this point, the fingerprint
will be tried first, and only if that fails or times out will you see
a password prompt. Take note of the led-stripe above the fingerprint
sensor to see whether it is active.
### The actual change from pam-auth-update
The above mentioned command `$ sudo pam-auth-update` simply makes a small modification to /etc/pam.d/common-auth:
```
# In /etc/pam.d/common-auth, the following line is added, and the next line changed.
# The end result (apart from other things that may be in the file) is this:
auth [success=2 default=ignore] pam_fprintd.so max_tries=1 timeout=10 # debug
auth [success=1 default=ignore] pam_unix.so nullok_secure try_first_pass
```
## Windows interoperability
Note: This section is likely only relevant if you will be dual booting.
To be able to use the same set of fingerprints for Windows and Linux, you first
need to extract the Windows user IDs (known as SIDs). To do this, start Windows,
start `cmd.exe` and run `wmic useraccount get name,sid`. This will provide a
list of all users and the corresponding SIDs.
You can then create a mapping from the Linux user names (as written in the
first `:`-separated field of `/etc/passwd`). This mapping is defined in
`/etc/python-validity/dbus-service.yaml`. For example:
```yaml
user_to_sid:
"myusername": "S-1-5-21-1234567890-1234567890-1234567890-1001"
"someotheruser": "S-1-5-21-1234567890-1234567890-1234567890-1003"
```
Note the indentation; each entry has to be preceded by at least one space.
## Playground
This package contains a set of scripts you can use to do a low-level debugging of the sensor protocol.
Here is a couple of examples of how you can use them.
Before using the scripts, make sure you've disabled the dbus service shipped with this package.
All examples assume that you are in `/usr/share/python-validity/playground/` directory and your device is already paired.
### Initialize a session
Before talking to a device you will need to open it and start a new TLS session
```
$ python3
Python 3.6.7 (default, Oct 22 2018, 11:32:17)
[GCC 8.2.0] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>> from prototype import *
>>> open9x()
>>>
```
### Enroll a new user
Note: 0xf5 == WINBIO_FINGER_UNSPECIFIED_POS_01 (see [ms docs](https://docs.microsoft.com/en-us/windows/desktop/SecBioMet/winbio-ansi-381-pos-fingerprint-constants))
```
>>> db.dump_all()
8: User S-1-5-21-111111111-1111111111-1111111111-1000 with 1 fingers:
9: f5 (WINBIO_FINGER_UNSPECIFIED_POS_01)
>>> enroll(sid_from_string('S-1-5-21-394619333-3876782012-1672975908-3333'), 0xf5)
Waiting for a finger...
Progress: 14 % done
Progress: 28 % done
Progress: 42 % done
Progress: 57 % done
Progress: 71 % done
Progress: 85 % done
Progress: 100 % done
All done
11
>>> db.dump_all()
8: User S-1-5-21-111111111-1111111111-1111111111-1000 with 1 fingers:
9: f5 (WINBIO_FINGER_UNSPECIFIED_POS_01)
10: User S-1-5-21-394619333-3876782012-1672975908-3333 with 1 fingers:
11: f5 (WINBIO_FINGER_UNSPECIFIED_POS_01)
>>>
```
### Delete database record (user/finger/whatever)
```
>>> db.dump_all()
8: User S-1-5-21-111111111-1111111111-1111111111-1000 with 1 fingers:
9: f5 (WINBIO_FINGER_UNSPECIFIED_POS_01)
10: User S-1-5-21-394619333-3876782012-1672975908-3333 with 1 fingers:
11: f5 (WINBIO_FINGER_UNSPECIFIED_POS_01)
>>> db.del_record(11)
>>> db.dump_all()
8: User S-1-5-21-111111111-1111111111-1111111111-1000 with 1 fingers:
9: f5 (WINBIO_FINGER_UNSPECIFIED_POS_01)
10: User S-1-5-21-394619333-3876782012-1672975908-3333 with 0 fingers:
>>>
```
### Identify a finger (scan)
```
>>> identify()
Recognised finger f5 (WINBIO_FINGER_UNSPECIFIED_POS_01) from user S-1-5-21-111111111-1111111111-1111111111-1000
Template hash: 36bc1fe077e59a3090c816fcf2798c30a85d8a8fbe000ead5c6a946c3bacef7b
```
## DBus service
When started, DBus service will first try to initialize the device, then it will try to register itself with the
[open-fprintd](https://github.com/uunicorn/open-fprintd) service. If `open-fprintd` is not available it will wait for it
to come up.
To start DBus service from the sources (useful for debugging):
```
PYTHONPATH=. ./dbus_service/dbus-service
```
## Debugging
If you are curious you can enable tracing to see what flows in and out of device before and after encryption
```
>>> tls.trace_enabled=True
>>> usb.trace_enabled=True
>>> logging.basicConfig(level=logging.DEBUG)
>>> db.dump_all()
>tls> 17: 4b00000b0053746757696e64736f7200
>cmd> 1703030050c00a7ff1cf76e90f168141b4bc519ca9598eacb575ff01b7552a3707be8506b246d5272cb119e7b8b3eccd991cb7d8387245953ff1da62cebfb07fae7e47b9b5
没有合适的资源?快使用搜索试试~ 我知道了~
Validity 有效性指纹传感器原型_Python_代码_相关文件_下载
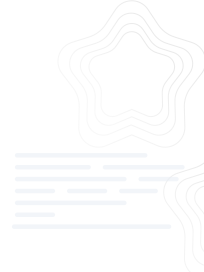
共51个文件
py:28个
spec:1个
compat:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 99 浏览量
2022-07-13
01:05:22
上传
评论
收藏 147KB ZIP 举报
温馨提示
有效性指纹传感器驱动程序。 目录 python-有效性 配置 错误情况 列出设备失败 启动时出错 从挂起唤醒后指纹不工作 启用指纹以进行系统身份验证 pam-auth-update 的实际变化 Windows 互操作性 操场 初始化会话 注册新用户 删除数据库记录(用户/手指/其他) 识别手指(扫描) DBus 服务 调试 更多详情、使用方法,请下载后细读README.md文件
资源推荐
资源详情
资源评论
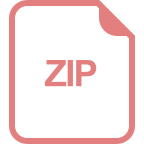
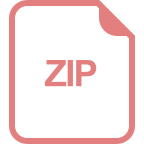
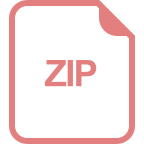
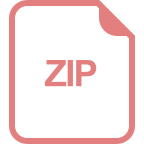
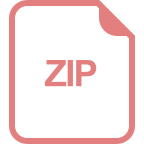
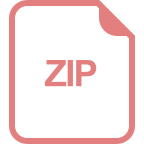
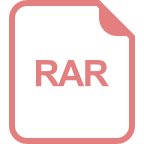
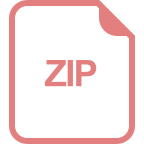
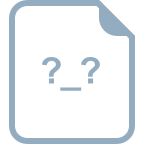
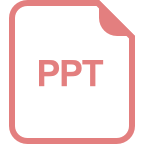
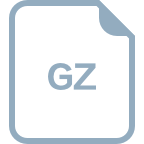
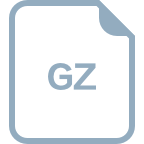
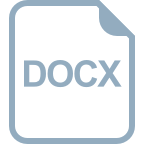
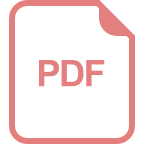
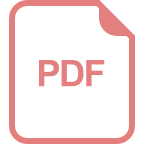
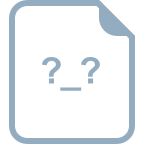
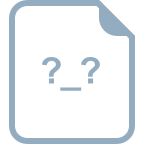
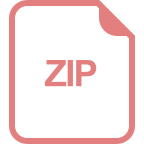
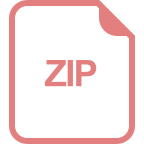
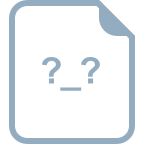
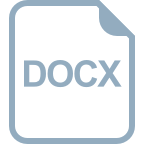
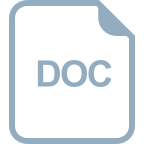
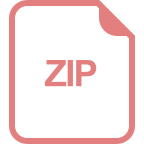
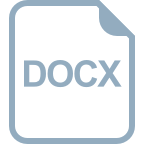
收起资源包目录


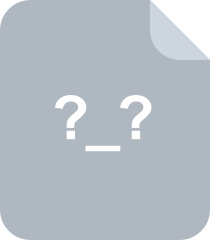

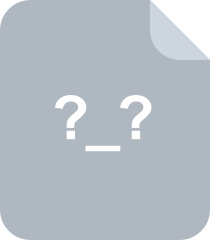

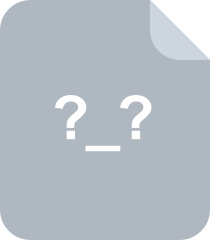
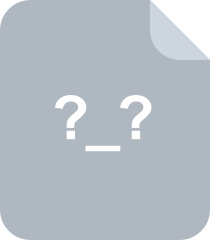
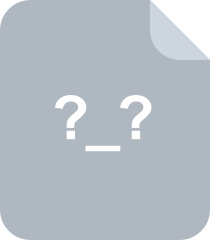

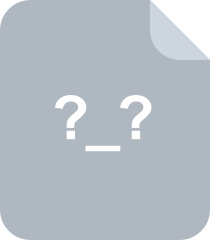
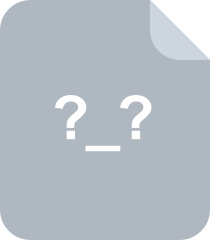
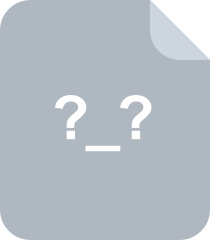
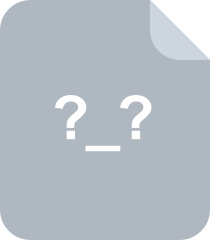
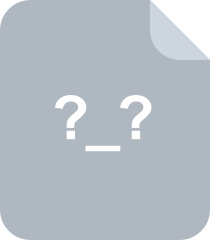
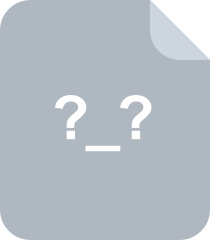
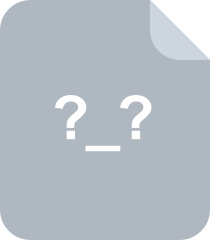
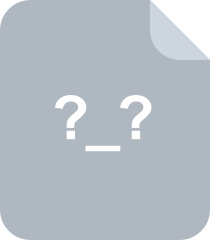
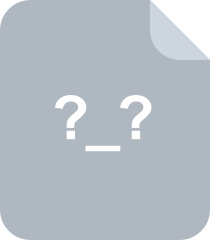
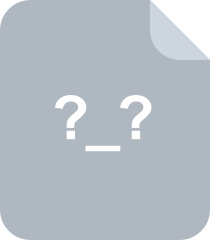
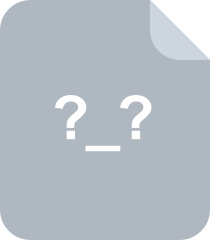

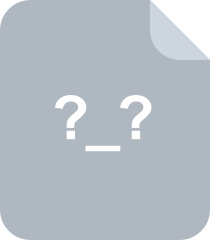

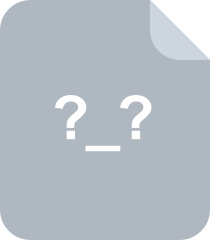
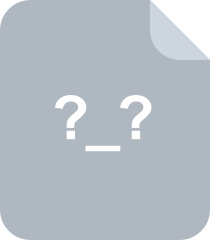
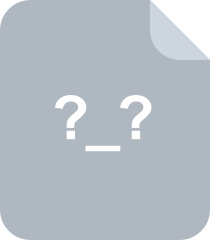
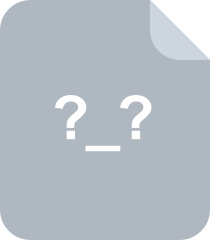

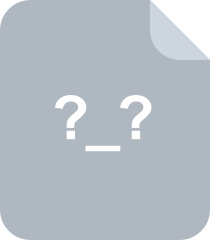
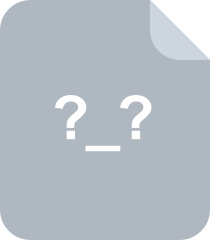
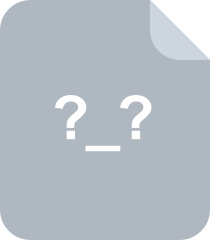
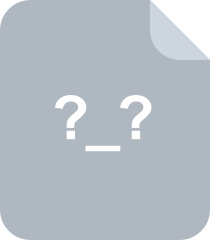
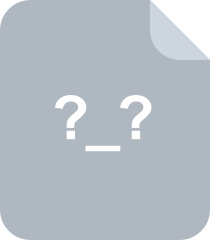
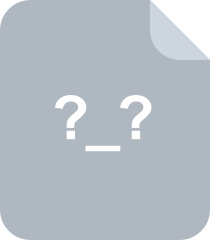
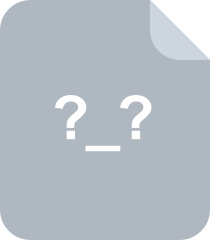
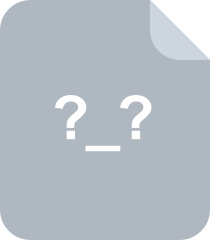
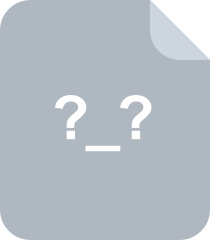
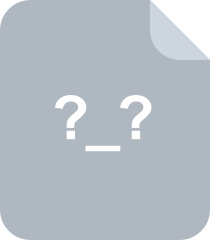
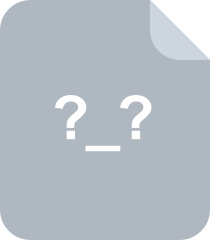
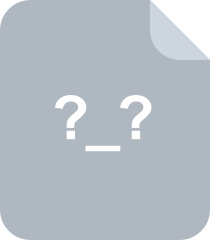
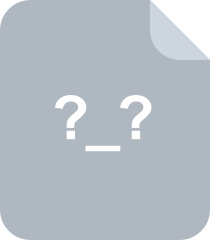
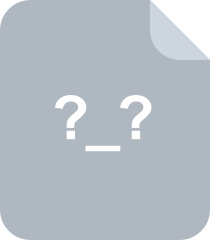
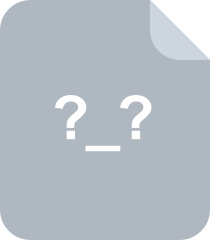
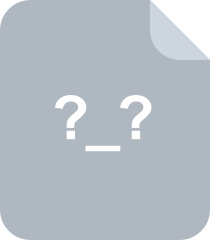
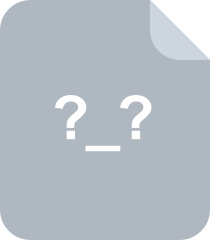
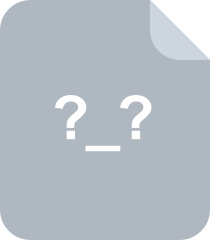
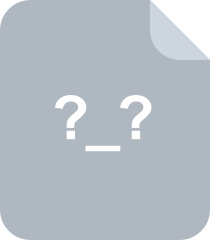
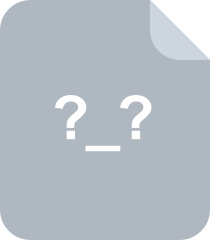
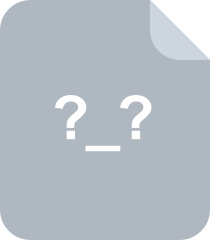
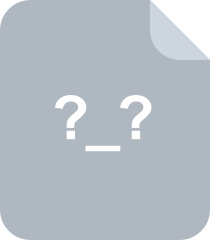
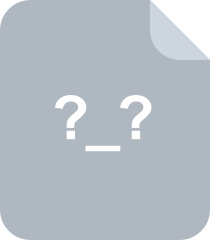
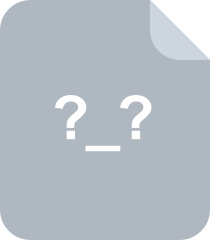

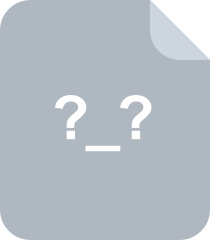
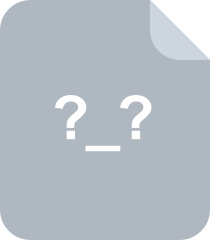
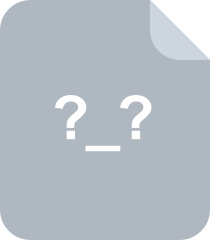
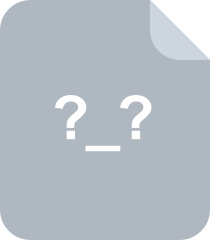


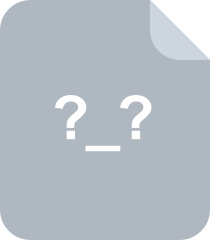
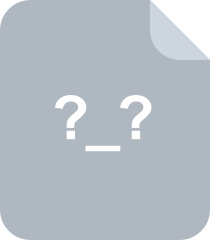
共 51 条
- 1
资源评论
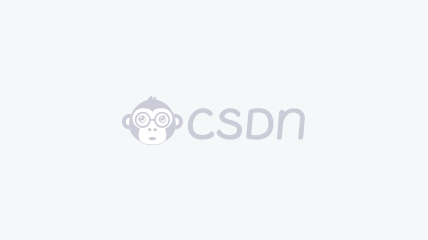

快撑死的鱼
- 粉丝: 1w+
- 资源: 9149
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

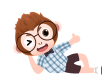
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


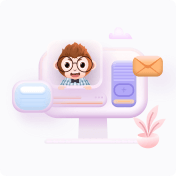
安全验证
文档复制为VIP权益,开通VIP直接复制
