#############################################################################
##
# Copyright (C) 2007 Trolltech ASA. All rights reserved.
##
# This file is part of the Qt Concurrent project on Trolltech Labs.
##
# This file may be used under the terms of the GNU General Public
# License version 2.0 as published by the Free Software Foundation
# and appearing in the file LICENSE.GPL included in the packaging of
# this file. Please review the following information to ensure GNU
# General Public Licensing requirements will be met:
# http://www.trolltech.com/products/qt/opensource.html
##
# If you are unsure which license is appropriate for your use, please
# review the following information:
# http://www.trolltech.com/products/qt/licensing.html or contact the
# sales department at sales@trolltech.com.
##
# This file is provided AS IS with NO WARRANTY OF ANY KIND, INCLUDING THE
# WARRANTY OF DESIGN, MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE.
##
#############################################################################
from PyQt5 import QtCore, QtGui
# This was originally a Trolltech file. The QBzr folks did some work to
# bring it up to date, and I've done some work on top of theirs to fix a few
# minor bugs.
#
# To test a model, instantiate this class with a reference to the model and
# its parent
# from modeltest import ModelTest
# model = MyFancyModel(self)
# self.modeltest = ModelTest(model, self)
class ModelTest(QtCore.QObject):
def __init__(self, _model, verbose=True, parent=None):
"""
Connect to all of the models signals, Whenever anything happens check everything.
"""
QtCore.QObject.__init__(self, parent)
self._model = _model
self.model = _model
self.insert = []
self.remove = []
self.fetchingMore = False
self._verbose = verbose
assert self.model
self.model.columnsAboutToBeInserted.connect(self.runAllTests)
self.model.columnsAboutToBeRemoved.connect(self.runAllTests)
self.model.columnsInserted.connect(self.runAllTests)
self.model.columnsRemoved.connect(self.runAllTests)
self.model.dataChanged.connect(self.runAllTests)
self.model.headerDataChanged.connect(self.runAllTests)
self.model.layoutAboutToBeChanged.connect(self.runAllTests)
self.model.layoutChanged.connect(self.runAllTests)
self.model.modelReset.connect(self.runAllTests)
self.model.rowsAboutToBeInserted.connect(self.runAllTests)
self.model.rowsAboutToBeRemoved.connect(self.runAllTests)
self.model.rowsInserted.connect(self.runAllTests)
self.model.rowsRemoved.connect(self.runAllTests)
# Special checks for inserting/removing
self.model.rowsAboutToBeInserted.connect(self.rowsAboutToBeInserted)
self.model.rowsAboutToBeRemoved.connect(self.rowsAboutToBeRemoved)
self.model.rowsInserted.connect(self.rowsInserted)
self.model.rowsRemoved.connect(self.rowsRemoved)
self.runAllTests()
def nonDestructiveBasicTest(self):
"""
nonDestructiveBasicTest tries to call a number of the basic functions (not all)
to make sure the model doesn't outright segfault,
testing the functions that makes sense.
"""
assert self.model.buddy(QtCore.QModelIndex()) == QtCore.QModelIndex()
self.model.canFetchMore(QtCore.QModelIndex())
assert self.model.columnCount(QtCore.QModelIndex()) >= 0
assert (
self.model.data(QtCore.QModelIndex(), QtCore.Qt.DisplayRole)
== QtCore.QVariant()
)
self.fetchingMore = True
self.model.fetchMore(QtCore.QModelIndex())
self.fetchingMore = False
flags = self.model.flags(QtCore.QModelIndex())
assert (
int(flags & QtCore.Qt.ItemIsEnabled) == QtCore.Qt.ItemIsEnabled
or int(flags & QtCore.Qt.ItemIsEnabled) == 0
)
self.model.hasChildren(QtCore.QModelIndex())
self.model.hasIndex(0, 0)
self.model.headerData(0, QtCore.Qt.Horizontal, QtCore.Qt.DisplayRole)
self.model.index(0, 0, QtCore.QModelIndex())
self.model.itemData(QtCore.QModelIndex())
cache = QtCore.QVariant()
self.model.match(QtCore.QModelIndex(), -1, cache)
self.model.mimeTypes()
assert self.model.parent(QtCore.QModelIndex()) == QtCore.QModelIndex()
assert self.model.rowCount(QtCore.QModelIndex()) >= 0
variant = QtCore.QVariant()
self.model.setData(QtCore.QModelIndex(), variant, -1)
self.model.setHeaderData(-1, QtCore.Qt.Horizontal, QtCore.QVariant())
self.model.setHeaderData(0, QtCore.Qt.Horizontal, QtCore.QVariant())
self.model.setHeaderData(999999, QtCore.Qt.Horizontal, QtCore.QVariant())
self.model.sibling(0, 0, QtCore.QModelIndex())
self.model.span(QtCore.QModelIndex())
self.model.supportedDropActions()
def rowCount(self):
"""
Tests self.model's implementation of QtCore.QAbstractItemModel::rowCount()
and hasChildren()
self.models that are dynamically populated are not as fully tested here.
"""
# check top row
topindex = self.model.index(0, 0, QtCore.QModelIndex())
rows = self.model.rowCount(topindex)
assert rows >= 0
if rows > 0:
hasChildren = self.model.hasChildren(topindex)
assert hasChildren is True
secondlvl = self.model.index(0, 0, topindex)
if secondlvl.isValid():
# check a row count where parent is valid
rows = self.model.rowCount(secondlvl)
assert rows >= 0
if rows > 0:
assert self.model.hasChildren(secondlvl) is True
# The self.models rowCount() is tested more extensively in checkChildren,
# but this catches the big mistakes
def columnCount(self):
"""
Tests self.model's implementation of QtCore.QAbstractItemModel::columnCount()
and hasChildren()
"""
# check top row
topidx = self.model.index(0, 0, QtCore.QModelIndex())
assert self.model.columnCount(topidx) >= 0
# check a column count where parent is valid
childidx = self.model.index(0, 0, topidx)
if childidx.isValid():
assert self.model.columnCount(childidx) >= 0
# columnCount() is tested more extensively in checkChildren,
# but this catches the big mistakes
def hasIndex(self):
"""
Tests self.model's implementation of QtCore.QAbstractItemModel::hasIndex()
"""
# Make sure that invalid values returns an invalid index
assert self.model.hasIndex(-2, -2) is False
assert self.model.hasIndex(-2, 0) is False
assert self.model.hasIndex(0, -2) is False
rows = self.model.rowCount(QtCore.QModelIndex())
cols = self.model.columnCount(QtCore.QModelIndex())
# check out of bounds
assert self.model.hasIndex(rows, cols) is False
assert self.model.hasIndex(rows + 1, cols + 1) is False
if rows > 0:
assert self.model.hasIndex(0, 0) is True
# hasIndex() is tested more extensively in checkChildren()
# but this catches the big mistakes
def index(self):
"""
Tests self.model's implementation of QtCore.QAbstractItemModel::index()
"""
# Make sure that invalid values returns an invalid index
assert self.model.index(-2, -2, QtCore.QModelIndex()) == QtCore.QModelIndex()
assert self.model.index(-2, 0, QtCore.QModelIndex()) == QtCore.QModelIndex()
assert self.model.index(0, -2, QtCore.QModelIndex()) == QtCore.QModelIndex()
rows = self.model.rowCount(QtCore.QModelIndex())
cols = self.model.columnCount(QtCore.QModelIndex())
if rows == 0:
return
# Catch off by on
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源分类:Python库 所属语言:Python 资源全名:PrettyQt-0.87.0.tar.gz 资源来源:官方 安装方法:https://lanzao.blog.csdn.net/article/details/101784059
资源推荐
资源详情
资源评论
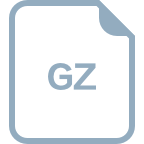
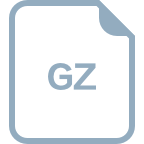
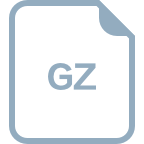
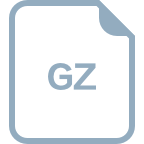
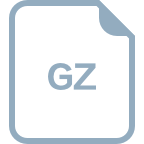
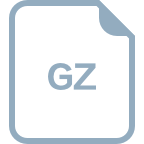
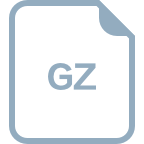
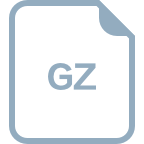
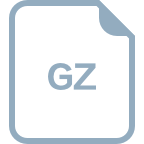
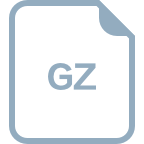
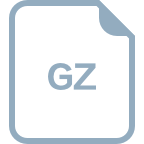
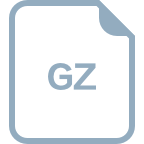
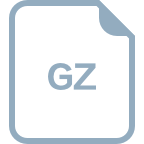
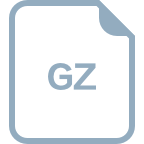
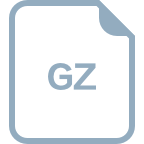
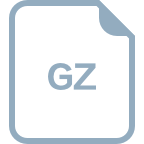
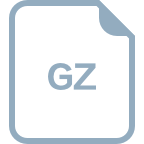
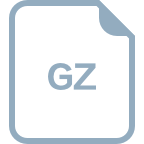
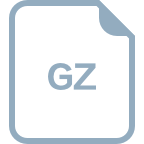
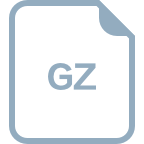
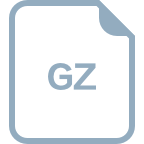
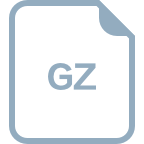
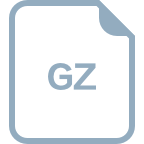
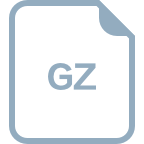
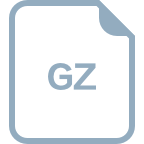
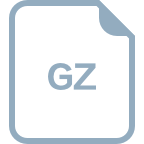
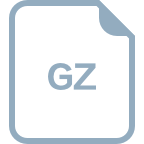
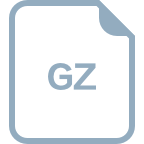
收起资源包目录

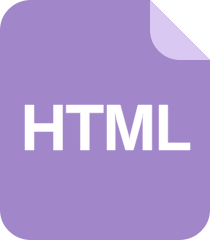
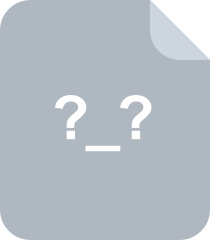
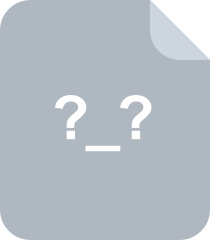
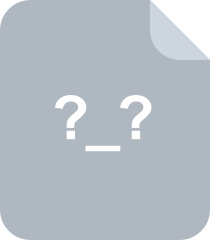
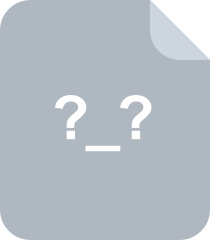
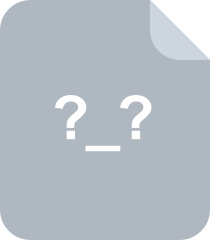
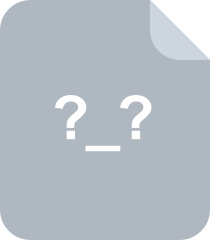
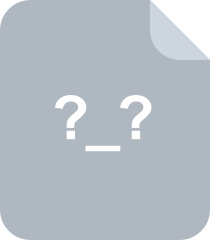
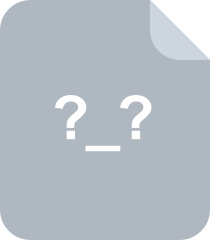
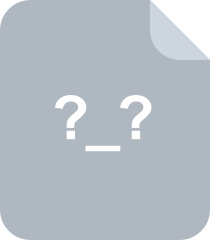
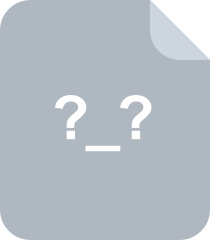
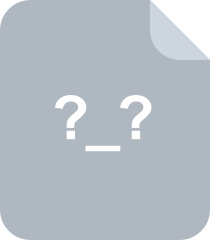
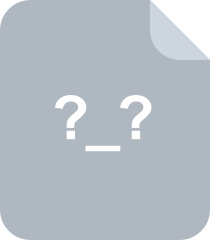
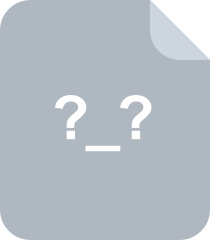
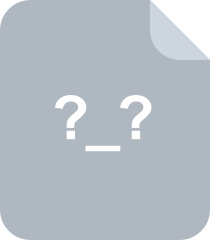
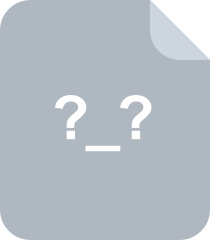
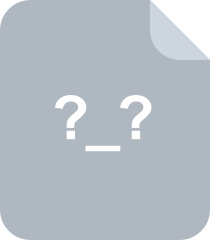
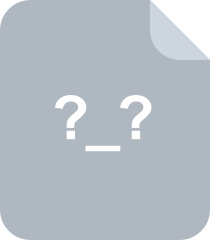
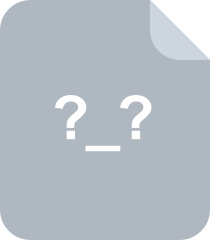
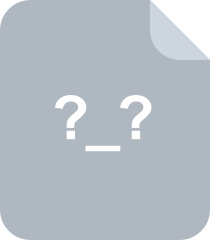
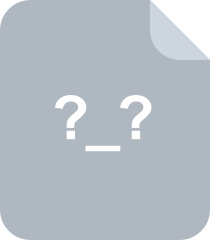
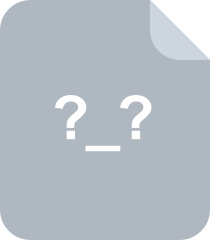
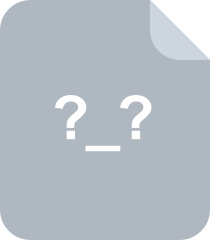
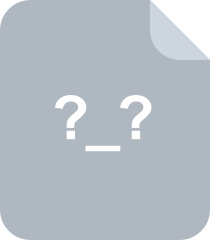
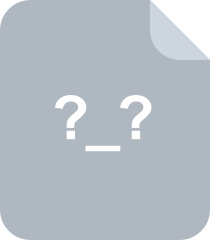
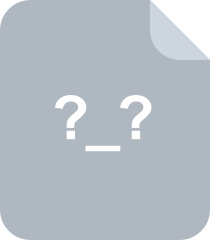
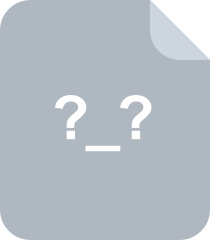
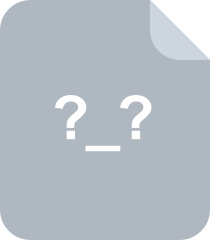
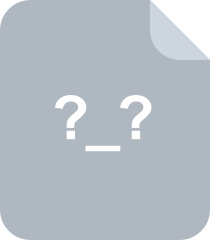
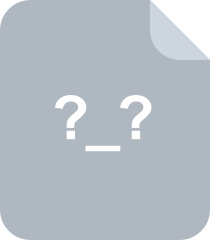
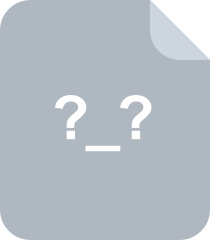
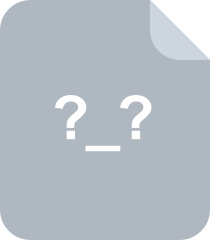
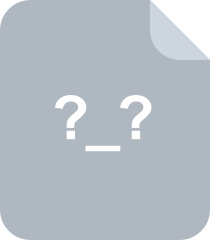
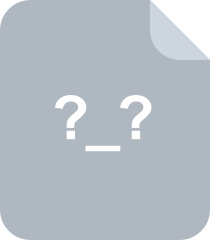
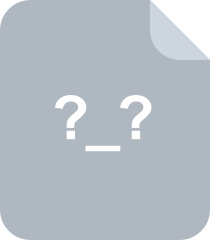
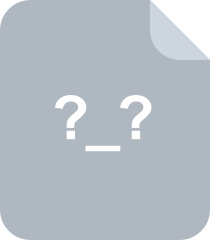
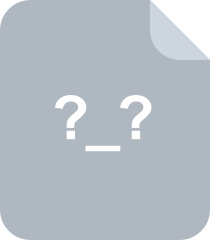
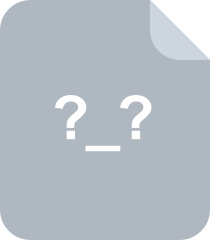
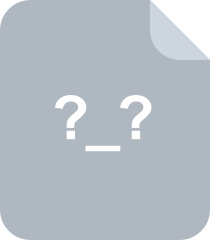
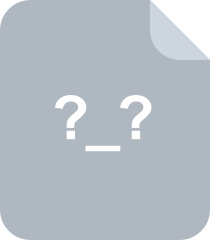
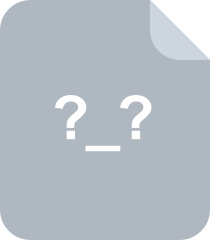
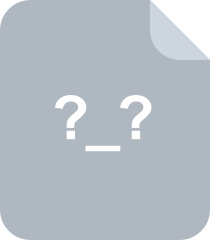
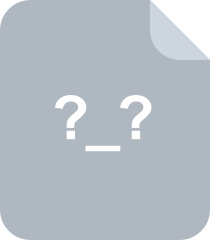
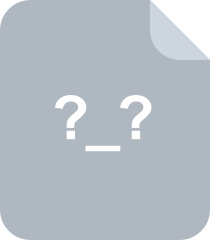
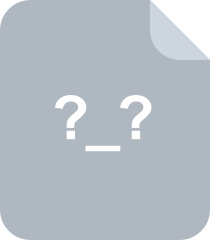
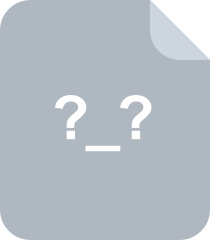
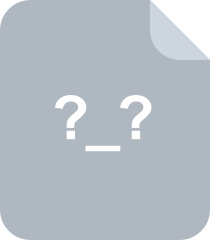
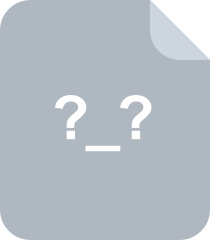
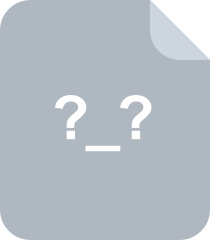
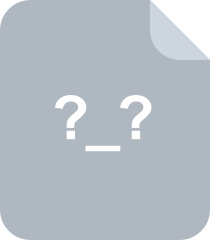
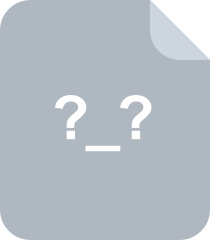
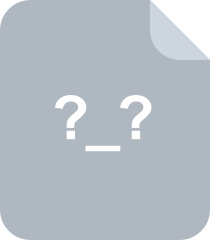
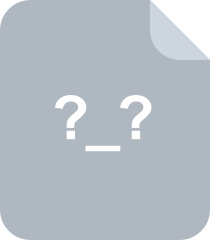
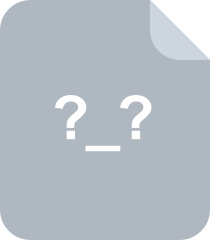
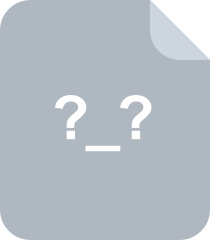
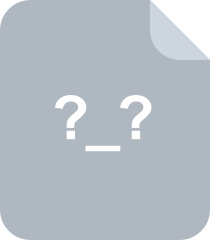
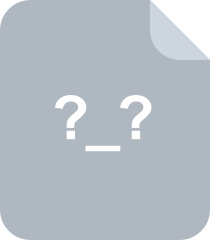
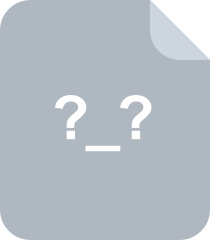
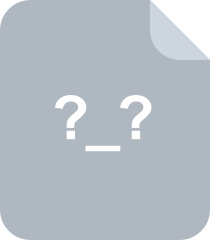
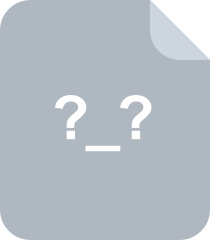
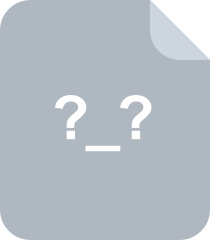
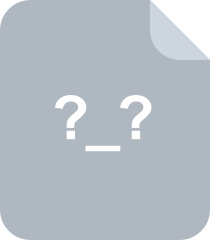
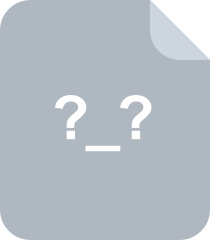
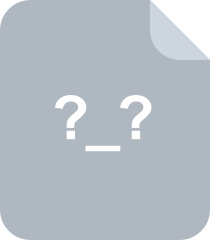
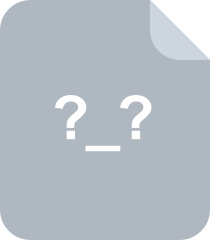
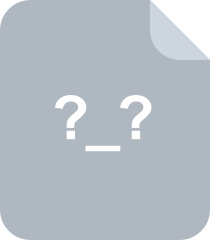
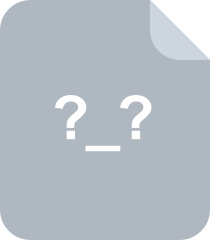
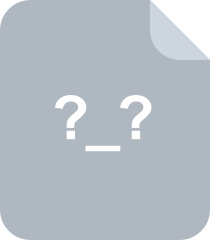
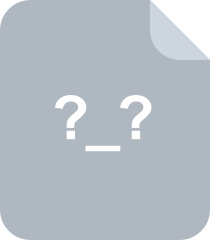
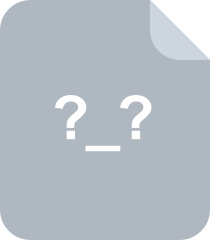
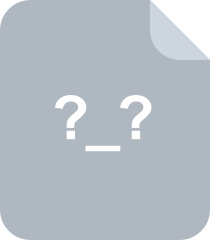
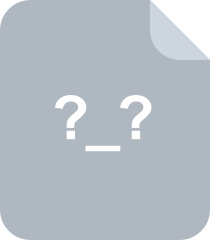
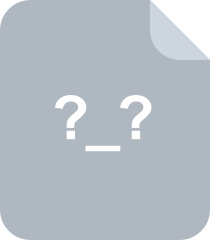
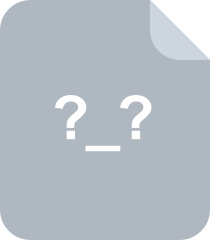
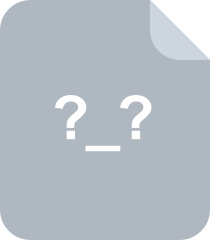
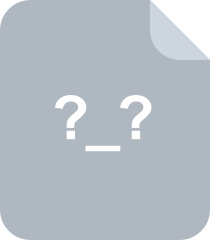
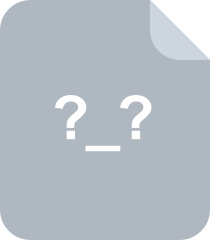
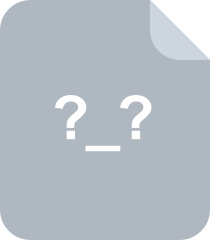
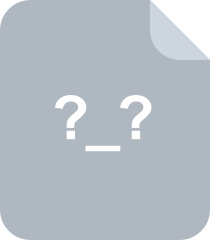
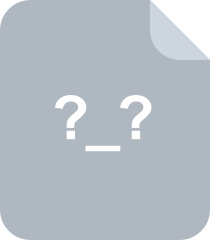
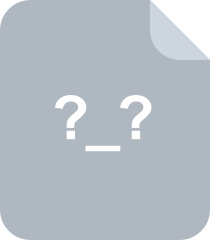
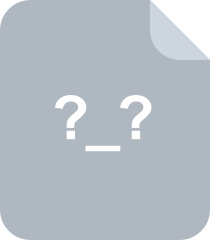
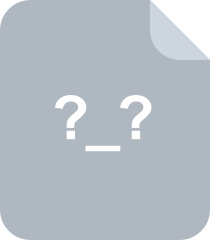
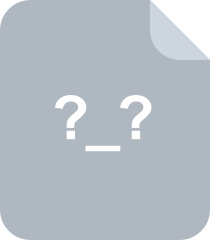
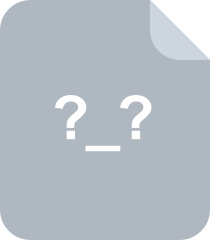
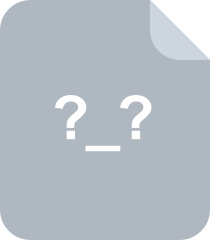
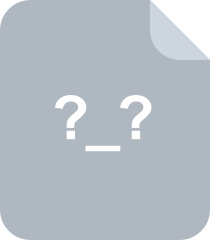
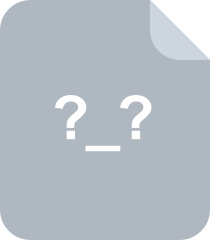
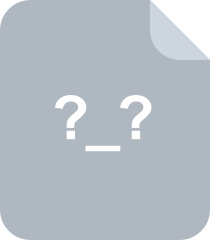
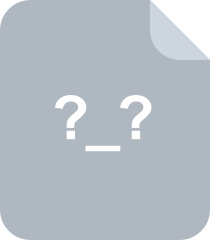
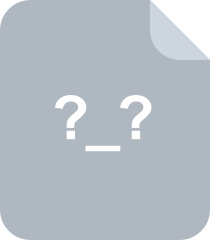
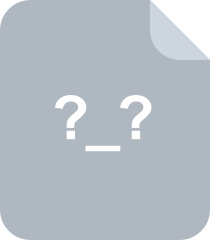
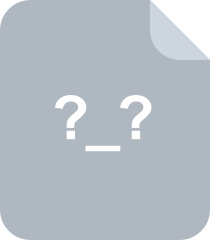
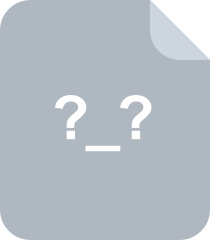
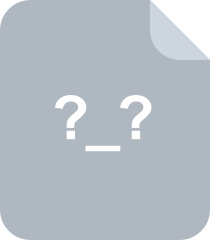
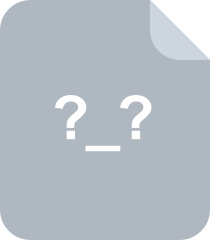
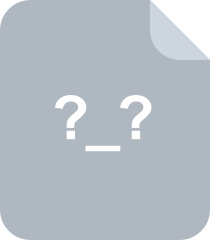
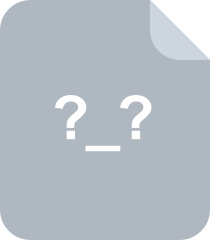
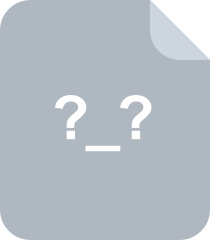
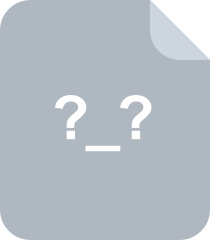
共 242 条
- 1
- 2
- 3
资源评论
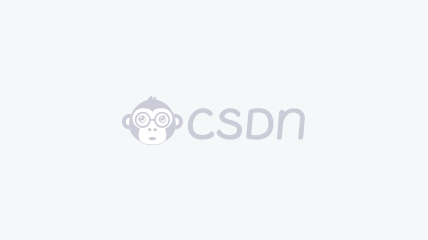

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

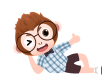
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


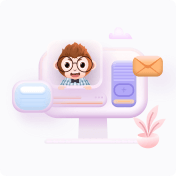
安全验证
文档复制为VIP权益,开通VIP直接复制
