# eth-signer
A Python library for transection signing using AWS Key Management Service.
## Dependencies
- Python 3.6+
## QuickStart
This Package is available on [PyPI](https://pypi.org/project/eth-signer/). Install via pip as:
```sh
pip install eth-signer
```
## Usage
`eth-signer` needs AWS-KMS Client Object from `boto3` to sign Ethereum transaction or message.
In the following example, the Basic use of `eth-signer` with `boto3` has been given.
Step - 1 : Install required dependencies
```bash
$ pip3 install boto3 eth-signer web3
```
Step - 2 : Create a new key pair using boto3 ( Optional )
```python
import boto3
from eth_signer.signer import AWSKMSKey
from web3 import Web3
# Get a kms_client Object From boto3
kms_client = boto3.client('kms', 'us-east-1')
# Generate new Key Pair using kms_client
new_key = kms.create_key(
Description='New Eth Key',
KeyUsage='SIGN_VERIFY',
KeySpec='ECC_SECG_P256K1',
Origin='AWS_KMS',
MultiRegion=False
)
# Use KekId of newly generated key pair for AWSKMSKey Object
key_id = new_key['KeyMetadata']['KeyId']
kms_signer = AWSKMSKey(kms_client, key_id)
web3 = Web3(Web3.HTTPProvider('https://ropsten.infura.io/v3/<PROJECT_ID>'))
# Print Key ID and Eth address
print("KeyId: ", key_id)
print("Eth Address: ", kms_signer.address)
```
Step - 3 : Send a new transaction using web3, boto3 and eth-signer
```python
import boto3
from eth_signer.signer import AWSKMSKey
from web3 import Web3
# Get a kms_client Object From boto3
kms_client = boto3.client('kms', 'us-east-1')
# User a KeyId of the AWS KMS Key
key_id = 'af8929db-010c-4476-00X0-0X00000X00X0'
kms_signer = AWSKMSKey(kms_client, key_id)
web3 = Web3(Web3.HTTPProvider('https://ropsten.infura.io/v3/<PROJECT_ID>'))
nonce = web3.eth.get_transaction_count(kms_signer.address)
print(web3.eth.getBalance(kms_signer.address))
# build a transaction in a dictionary
tx_obj = {
"nonce": nonce,
"from": kms_signer.address,
"to": '0xBe0745cF5b82aB1de6fB1CEd849081BE06d9b3be',
"value": web3.toWei(0.01, "ether"),
"gas": 200000,
"gasPrice": web3.toWei("50", "gwei"),
}
signed_tx = AWSKMSKey.sign_transaction(kms_signer, transaction_dict = tx_obj)
tx_hash = signed_tx.hash
web3.eth.send_raw_transaction(signed_tx.rawTransaction)
```
Output:
`HexBytes('0x826a52e59431a4be8780807cdd09da01d0dbbb00848fd7c9dff8383869c7372c')`
Transaction on [etherscan.io](https://ropsten.etherscan.io/tx/0x826a52e59431a4be8780807cdd09da01d0dbbb00848fd7c9dff8383869c7372c)
### Features
- Support for Ethereum Transaction and Message Signing using AWS Key Management Service
### Contributors
* [Kalpesh Sejpal](https://github.com/sejpalkalpesh/)
* [Medium Article from Lucas Henning](https://luhenning.medium.com/the-dark-side-of-the-elliptic-curve-signing-ethereum-transactions-with-aws-kms-in-javascript-83610d9a6f81)
### Runtime dependencies
The distributed eth-signer contains software from the following projects from PyPi:
* eth-utils
* eth-typing
* hexbytes
* eth-rlp
* pycryptodome
* boto3
* flake8
* isort
* mypy
* Sphinx
* sphinx_rtd_theme
* towncrier
* bumpversion
* setuptools
* tox
* twine

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
最新资源
- python图像识别程序源代码.zip
- ESP32S3驱动AP3216C多功能传感器模块实验源码
- 使用 Go 语言实现电商交易系统,该系统聚集类似淘宝、京东、、当当、小米、携程等子系统 希望该项目对你熟悉Java技术和毕业设计一定有很多的帮助 .zip
- 使用 C++ (VC6.0) 开发的易语言枚举文件子目录支持库(原“辅助调试支持库”).zip
- leetcode 2. 两数相加
- 临时起意使用Java语言编写的简易邮件服务器,包含客户端和服务端,功能基础但够用(无前端).zip
- k8s1.28.2 docker镜像 包含网络插件 calico 3.28.1
- 数据结构-顺序表的实现代码
- 一款基于`kotlin`语言开发的简单易用的依赖注入框架,拥有较强的扩展性,可以自定义注入器、拦截器 .zip
- LiuShuaiDong:springboot跨域解决方案
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


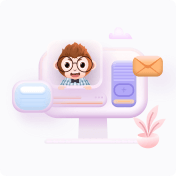