## `dnn_cool`: Deep Neural Networks for Conditional objective oriented learning
To install, just do:
```bash
pip install dnn_cool
```
* [Introduction](#introduction): What is `dnn_cool` in a nutshell?
* [Examples](#examples): a simple step-by-step example.
* [Features](#features): a list of the utilities that `dnn_cool` provides for you
* [Customization](#customization): Learn how to add new tasks, modify them, etc.
* [Inspiration](#inspiration): list of papers and videos which inspired this library
To see the predefined tasks for this release, see [list of predefined tasks](#list-of-predefined-tasks)
### Introduction
A framework for multi-task learning in Pytorch, where you may precondition tasks and compose them into bigger tasks.
Many complex neural networks can be trivially implemented with `dnn_cool`.
For example, creating a neural network that does classification and localization is as simple as:
```python
@project.add_flow
def localize_flow(flow, x, out):
out += flow.obj_exists(x.features)
out += flow.obj_x(x.features) | out.obj_exists
out += flow.obj_y(x.features) | out.obj_exists
out += flow.obj_w(x.features) | out.obj_exists
out += flow.obj_h(x.features) | out.obj_exists
out += flow.obj_class(x.features) | out.obj_exists
return out
```
If for example you want to classify first if the camera is blocked and then do localization **given that the camera
is not blocked**, you could do:
```python
@project.add_flow
def full_flow(flow, x, out):
out += flow.camera_blocked(x.cam_features)
out += flow.localize_flow(x.localization_features) | (~out.camera_blocked)
return out
```
Based on these "task flows" as we call them, `dnn_cool` provides a bunch of [features](#features).
Currently, this is the list of the predefined tasks (they are all located in `dnn_cool.task_flow`):
##### List of predefined tasks
In the current release (0.1.0), the following tasks are availble out of the box:
* `BinaryClassificationTask` - sigmoid activation, thresholding decoder, binary cross entropy loss function. In the
examples above, `camera_blocked` and `obj_exists` are `BinaryClassificationTask`s.
* `ClassificationTask` - softmax activation, sorting classes decoder, categorical cross entropy loss. In the example
above, `obj_class` is a `ClassificationTask`
* `MultilabelClassificationTask` - sigmoid activation, thresholding decoder, binary cross entropy loss function.
* `BoundedRegressionTask` - sigmoid activation, rescaling decoder, mean squared error loss function. In the examples
above, `obj_x`, `obj_y`, `obj_w`, `obj_h` are bounded regression tasks.
* `TaskFlow` - a composite task, that contains a list of children tasks. We saw 2 task flows above.
### Examples
#### Quick Imagenet example
We just have to add a `ClassificationTask` named `classifier` and add the flow below:
```python
@project.add_flow()
def imagenet_model(flow, x, out):
out += flow.classifier(x.features)
return out
```
That's great! But what if there is not an object always? Then we have to first check if an object exists. Let's
add a `BinaryClassificationTask` and use it as a precondition to classifier.
```python
@project.add_flow()
def imagenet_model(flow, x, out):
out += flow.object_exists(x.features)
out += flow.classifier(x.features) | out.object_exists
return out
```
But what if we also want to localize the object? Then we have to add new tasks that regress the bounding box. Let's
call them `object_x`, `object_y`, `object_w`, `object_h` and make them a `BoundedRegressionTask`. To avoid
preconditioning all tasks on `object_exists`, let's group them first. Then we modify the
flow:
```python
@project.add_flow()
def object_flow(flow, x, out):
out += flow.classifier(x.features)
out += flow.object_x(x.features)
out += flow.object_y(x.features)
out += flow.object_w(x.features)
out += flow.object_h(x.features)
return out
@project.add_flow()
def imagenet_flow(flow, x, out):
out += flow.object_exists(x.features)
out += flow.object_flow(x.features) | out.object_exists
return out
```
But what if the camera is blocked? Then there is no need to do anything, so let's create a new flow
that executes our `imagenet_flow` only when the camera is not blocked.
```python
def full_flow(flow, x, out):
out += flow.camera_blocked(x.features)
out += flow.imagenet_flow(x.features) | (~out.camera_blocked)
return out
```
But what if for example we want to check if the object is a kite, and if it is, to classify its color?
Then we would have to modify our `object_flow` as follows:
```python
@project.add_flow()
def object_flow(flow, x, out):
out += flow.classifier(x.features)
out += flow.object_x(x.features)
out += flow.object_y(x.features)
out += flow.object_w(x.features)
out += flow.object_h(x.features)
out += flow.is_kite(x.features)
out += flow.color(x.features) | out.is_kite
return out
```
I think you can see what `dnn_cool` is meant to do! :)
To see a full walkthrough on a synthetic dataset, check out the [Colab notebook](https://colab.research.google.com/drive/1fEidcOszTI9JXptbuU5GGC-O_yxb6hxO?usp=sharing)
or the [markdown write-up](./story.md).
### Features
Main features are:
* [Task precondition](#task-preconditioning)
* [Missing values handling](#missing-values)
* [Task composition](#task-composition)
* [Tensorboard metrics logging](#tensorboard-logging)
* [Task interpretations](#task-interpretation)
* [Task evaluation](#task-evaluation)
* [Task threshold tuning](#task-threshold-tuning)
* [Dataset generation](#dataset-generation)
* [Tree explanations](#tree-explanations)
##### Task preconditioning
Use the `|` for task preconditioning (think of `P(A|B)` notation). Preconditioning - ` A | B` means that:
* Include the ground truth for `B` in the input batch when training
* When training, update the weights of the `A` only when `B` is satisfied in the ground truth.
* When training, compute the loss function for `A` only when `B` is satisfied in the ground truth
* When training, compute the metrics for `A` only when `B` is satisfied in the ground truth.
* When tuning threshold for `A`, optimize only on values for which `B` is satisfied in the ground truth.
* When doing inference, compute the metrics for `A` only when the precondition is satisfied according to the decoded
result of the `B` task
* When generating tree explanation in inference mode, do not show the branch for `A` if `B` is not
satisfied.
* When computing results interpretation, include only loss terms when the precondition is satisfied.
Usually, you have to keep track of all this stuff manually, which makes adding new preconditions very difficult.
`dnn_cool` makes this stuff easy, so that you can chain a long list of preconditions without worrying you forgot
something.
##### Missing values
Sometimes for an input you don't have labels for all tasks. With `dnn_cool`, you can just mark the missing label and
`dnn_cool` will update only the weights of the tasks for which labels are available.
This feature has the awesome property that you don't need a single dataset with all tasks labeled, you can
have different datasets for different tasks and it will work. For example, you can train a single object detection
neural network that trains its classifier head on ImageNet, and its detection head on COCO.
##### Task composition
You can group tasks in a task flow (we already saw 2 above - `localize_flow` and `full_flow`). You can use this to
organize things better, for example when you want to precondition a whole task flow. For example:
```python
@project.add_flow
def face_regression(flow, x, out):
out += flow.face_x1(x.face_localization)
out += flow.face_y1(x.face_localization)
out += flow.face_w(x.face_localization)
out += flow.face_h(x.face_localization)
out += flow.facial_characteristics(x.features)
return out
```
##### Tensorboard logging
`dnn_co
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
资源分类:Python库 所属语言:Python 资源全名:dnn_cool-0.1.2.1.tar.gz 资源来源:官方 安装方法:https://lanzao.blog.csdn.net/article/details/101784059
资源推荐
资源详情
资源评论
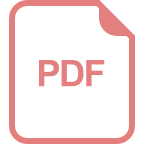
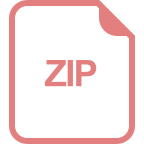
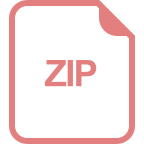
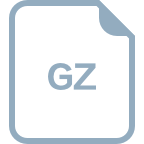
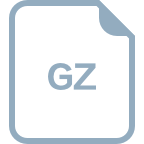
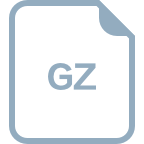
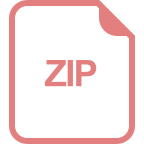
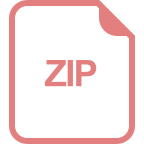
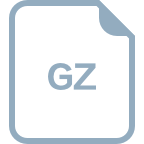
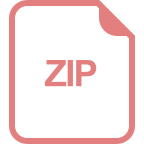
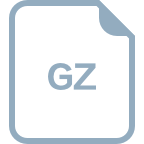
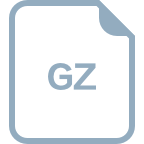
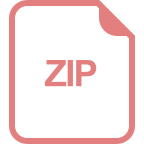
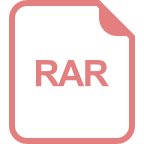
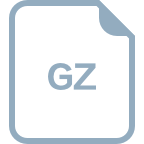
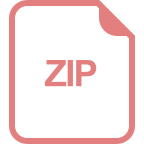
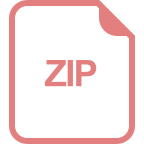
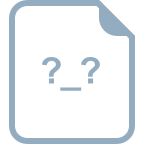
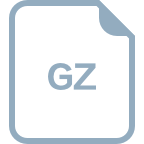
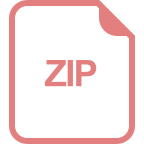
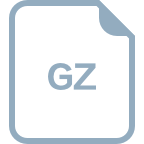
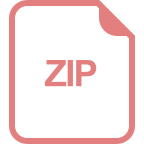
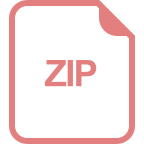
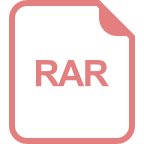
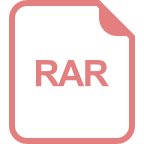
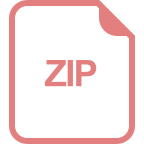
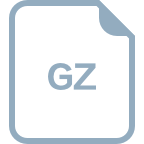
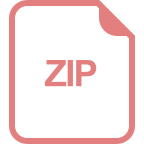
收起资源包目录


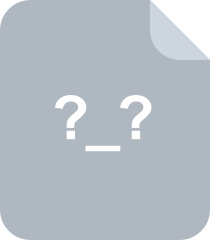

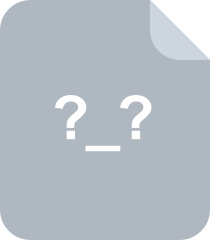
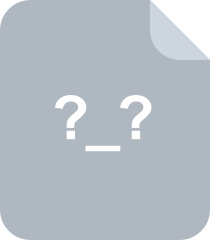
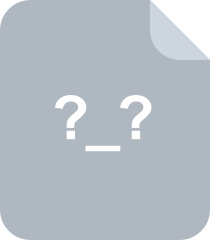
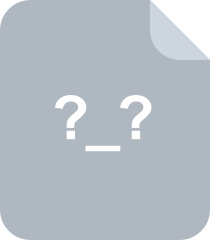
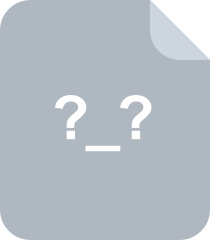
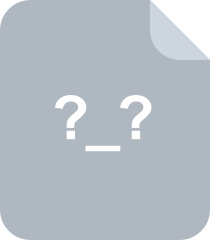
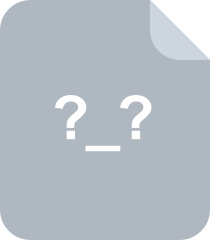
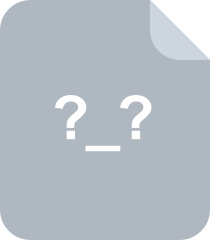
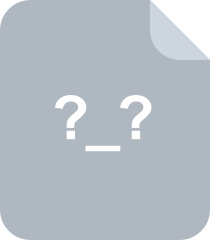
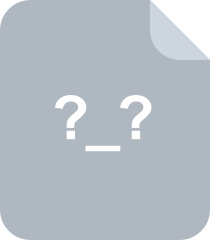
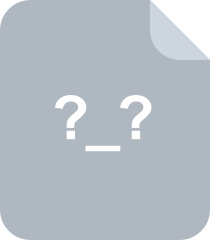
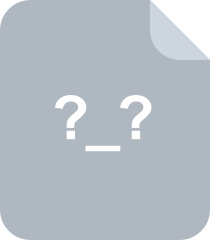
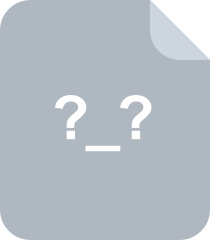
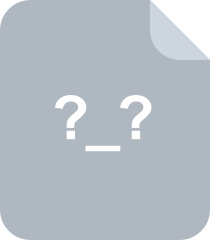
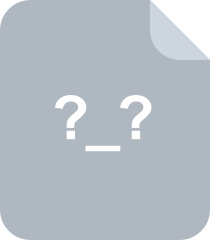
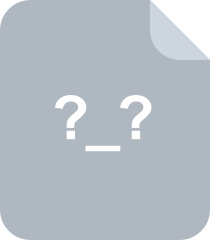
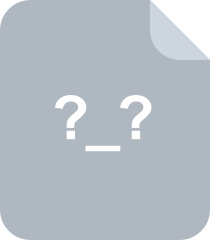
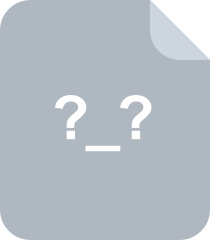
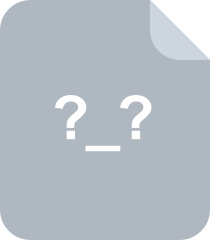
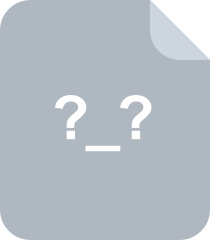
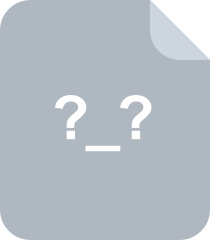
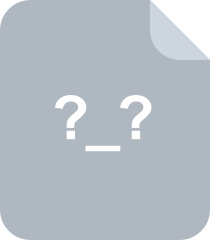
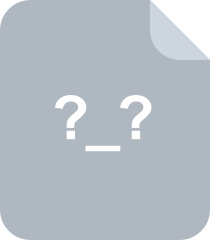
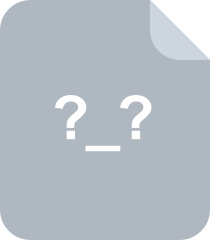
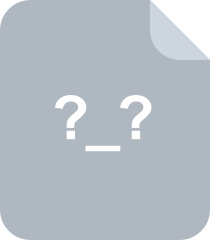
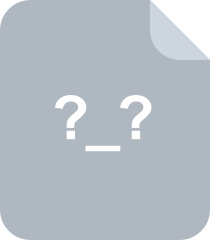

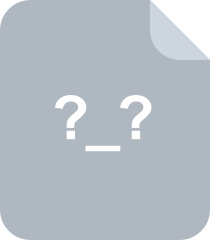
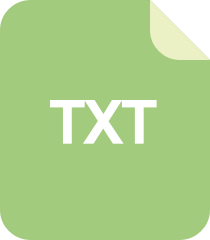
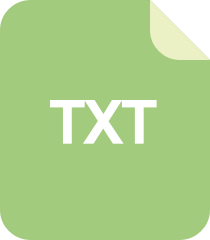
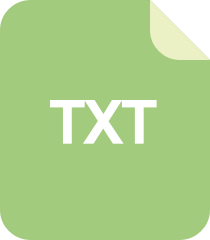
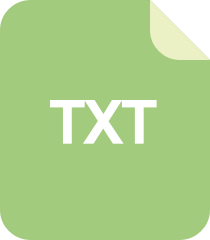
共 32 条
- 1
资源评论
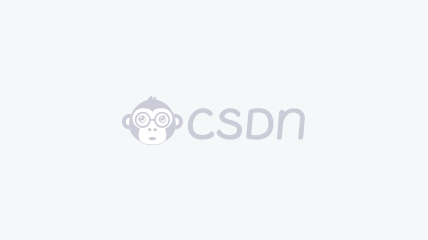

挣扎的蓝藻
- 粉丝: 14w+
- 资源: 15万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

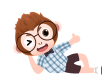
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


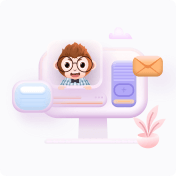
安全验证
文档复制为VIP权益,开通VIP直接复制
