#include "client.h"
#include "ui_client.h"
#include <QGridLayout>
#include <QCloseEvent>
#include <QMessageBox>
#include <QDebug>
#include <QtNetwork>
#include <QFileDialog>
#include <QFile>
Client::Client(QWidget *parent) :
QWidget(parent),
ui(new Ui::Client)
{
ui->setupUi(this);
//添加样式
qssFile = new QFile(":/qss/myStyleSheetB.qss",this);
qssFile->open(QFile::ReadOnly);
QString styleSheet = tr(qssFile->readAll());
qApp->setStyleSheet(styleSheet);
qssFile->close();
//栅格化初始布局
setMyLayout();
//进入登录注册界面
loginView();
tcpClient = new QTcpSocket(this);
tcpClient->connectToHost(QHostAddress::LocalHost,6666);
//先不进行文件传输
isUploadingFile = false;
//接收文件初始化
isDownloadingFile = false;
downTotalBytes = 0;
downBytesReceived = 0;
downFileNameSize = 0;
//当有可读数据时,发射readyread信号
connect(tcpClient, &QTcpSocket::readyRead, this, &Client::readMessage);
//连接错误
connect(tcpClient, SIGNAL(error(QAbstractSocket::SocketError)), this, SLOT(disPlayError(QAbstractSocket::SocketError)));//发生错误时error信号
//发射开始上传信息好后开始上传
connect(this, SIGNAL(startUploadSignal()), this, SLOT(startUpload()));
//上传过程中更新上传进度
connect(tcpClient, SIGNAL(bytesWritten(qint64)), this, SLOT(updateUploadProgress(qint64)));
qDebug() <<"ok";
}
Client::~Client()
{
delete tcpClient;
delete ui;
}
/*-------------------------页面布局模块--------------------------*/
/*栅格化初始布局*/
void Client::setMyLayout()
{
QGridLayout *layout = new QGridLayout;
layout->addWidget(ui->idLabel,1,0,1,2);
layout->addWidget(ui->idLineEdit,1,2,1,4);
layout->addWidget(ui->passLabel,2,0,1,2);
layout->addWidget(ui->passLineEdit,2,2,1,4);
layout->addWidget(ui->regiButton,3,0,1,3);
layout->addWidget(ui->loginButton,3,3,1,3);
layout->addWidget(ui->stateLabel,4,0,1,2);
layout->addWidget(ui->contentLabel,4,2,1,4);
layout->addWidget(ui->loginStatusLabel,5,0,1,4);
layout->addWidget(ui->exitButton,5,4,1,2);
layout->addWidget(ui->actLine,6,0,1,6);
layout->addWidget(ui->upWarnLabel,7,2,1,2);
layout->addWidget(ui->openButton,8,0,1,3);
layout->addWidget(ui->upButton,8,3,1,3);
layout->addWidget(ui->filenameLabel,9,0,1,6);
layout->addWidget(ui->upBar,10,0,1,6);
layout->addWidget(ui->sepLine,11,0,1,6);
layout->addWidget(ui->downWarnLabel,12,2,1,2);
layout->addWidget(ui->searchEdit,13,0,1,4);
layout->addWidget(ui->searchButton,13,4,1,2);
layout->addWidget(ui->downLabel,14,0,1,6);
layout->addWidget(ui->downButton,15,0,1,3);
layout->addWidget(ui->unDownButton,15,3,1,3);
layout->addWidget(ui->downBar,16,0,1,6);
layout->setHorizontalSpacing(20); //控件间横向距离
layout->setVerticalSpacing(30); //控件间纵向距离
layout->setMargin(30); //设置内边距
setLayout(layout);
}
/*登录界面*/
void Client::loginView()
{
//要隐藏的控件
ui->loginStatusLabel->hide();
ui->exitButton->hide();
ui->actLine->hide();
ui->openButton->hide();
ui->upButton->hide();
ui->filenameLabel->hide();
ui->upBar->hide();
ui->sepLine->hide();
ui->searchEdit->hide();
ui->searchButton->hide();
ui->downLabel->hide();
ui->downButton->hide();
ui->downBar->hide();
ui->upWarnLabel->hide();
ui->downWarnLabel->hide();
ui->unDownButton->hide();
//要显示的控件
ui->idLabel->show();
ui->idLineEdit->show();
ui->passLabel->show();
ui->passLineEdit->show();
ui->loginButton->show();
ui->regiButton->show();
ui->stateLabel->show();
ui->contentLabel->show();
//设置大小
this->resize(350,230);
}
/*客户端操作界面*/
void Client::actView()
{
//要隐藏的控件
ui->idLabel->hide();
ui->idLineEdit->hide();
ui->passLabel->hide();
ui->passLineEdit->hide();
ui->loginButton->hide();
ui->regiButton->hide();
ui->stateLabel->hide();
ui->contentLabel->hide();
//要显示的控件
ui->loginStatusLabel->show();
ui->actLine->show();
ui->openButton->show();
ui->upButton->show();
ui->filenameLabel->show();
ui->upBar->show();
ui->sepLine->show();
ui->searchEdit->show();
ui->searchButton->show();
ui->downLabel->show();
ui->downButton->show();
ui->downBar->show();
ui->exitButton->show();
ui->upWarnLabel->show();
ui->downWarnLabel->show();
ui->unDownButton->show();
//控件初始化
ui->downBar->reset();
ui->upBar->reset();
ui->filenameLabel->setText(tr("当前未选择文件"));
ui->downLabel->setText(tr("当前未搜索文件"));
ui->searchEdit->setText(tr(""));
//上传下载按钮设置无效
ui->upButton->setEnabled(false);
ui->downButton->setEnabled(false);
ui->unDownButton->setEnabled(false);
//设置大小
this->resize(350,420);
}
/*-------------------------页面布局模块结束--------------------------*/
/*-------------------------操作模块--------------------------------*/
/*发送消息的函数
* Mess -- 消息指令
* str1 -- 第一内容字符串
* str2 -- 第二内容字符串
* */
void Client::sendMessToServer(QString Mess, QString str1, QString str2){
QByteArray requestBlock; //用来存放请求信息
QDataStream out(&requestBlock, QIODevice::WriteOnly);
//设置数据流的版本,客户端和服务器使用的版本要相同
out.setVersion(QDataStream::Qt_5_6);//版本
out << (quint64)0;//因为在写入数据以前可能不知道实际数据的大小,所以要先在数据块的最前面留四个字节位置,以便以后填写数据大小
out << Mess << str1 << str2; //输入实际数据
out.device()->seek(0);//跳转到数据块头部
out << (quint64)(requestBlock.size() - sizeof(quint64));//填写信息大小
tcpClient->write(requestBlock); //发送
qDebug() <<"send" << Mess << str1 << str2;
requestBlock.resize(0);
}
/*点击x按钮*/
void Client::closeEvent(QCloseEvent *event)
{
QMessageBox::StandardButton button;
if(isUploadingFile){ //如果正在传输文件,提示有文件正在上传
button = QMessageBox::warning(this, tr("警告"), QString(tr("有文件正在上传,无法退出程序")),
QMessageBox::Cancel);
event->ignore(); //忽略退出信号,程序继续运行
}
else if(isDownloadingFile){ //如果正在下载文件,提示有文件正在下载
button = QMessageBox::warning(this, tr("警告"), QString(tr("有文件正在下载,无法退出程序")),
QMessageBox::Cancel);
event->ignore(); //忽略退出信号,程序继续运行
}
else{
button = QMessageBox::question(this, tr("退出程序"), QString(tr("确认退出程序?")),
QMessageBox::Yes | QMessageBox::No);
if (button == QMessageBox::No) {
event->ignore(); //忽略退出信号,程序继续运行
}
else if (button == QMessageBox::Yes) {
sendMessToServer(EXITAPP, MESSNULL, MESSNULL); //向服务器发送退出客户端信息
tcpClient->close();
event->accept(); //接受退出信号,程序退出
qDebug() <<"exit";
}
}
}
/*点击登录按钮,向服务器发送请求*/
void Client::on_loginButton_clicked()
{
QString id = ui->idLineEdit->text(); //获取账户
QString pass = ui->passLineEdit->t
没有合适的资源?快使用搜索试试~ 我知道了~
毕业设计:基于C++和QT的文件传输系统(含客户端和服务端).zip
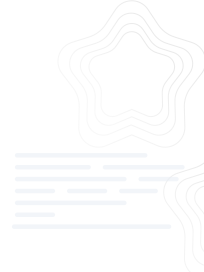
共27个文件
cpp:7个
h:7个
ui:3个

0 下载量 119 浏览量
2023-08-27
16:23:37
上传
评论
收藏 31KB ZIP 举报
温馨提示
本资源中的源码都是经过本地编译过可运行的,下载后按照文档配置好环境就可以运行。资源项目源码系统完整,内容都是经过专业老师审定过的,能够满足基本的学习、使用参考需求,如果有需要的话可以放心下载使用。
资源推荐
资源详情
资源评论
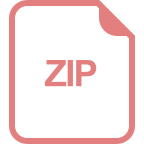
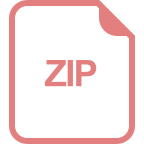
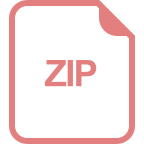
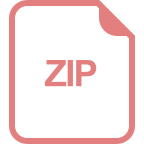
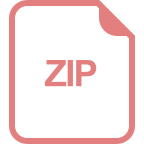
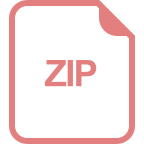
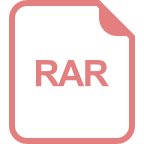
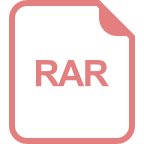
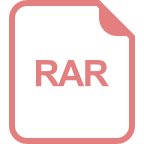
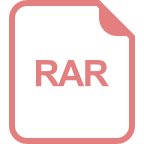
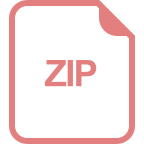
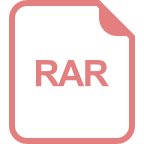
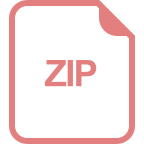
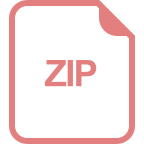
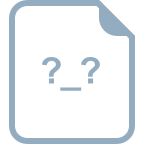
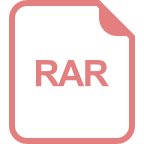
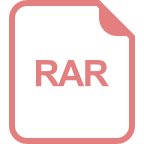
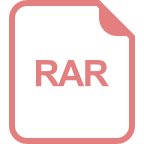
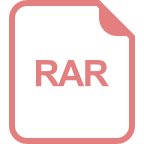
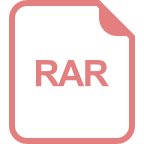
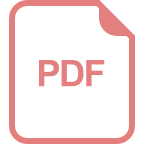
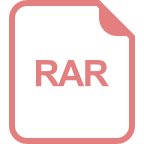
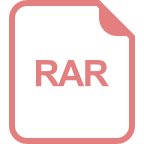
收起资源包目录



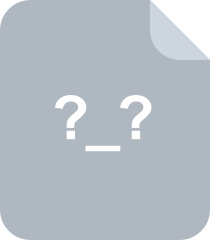
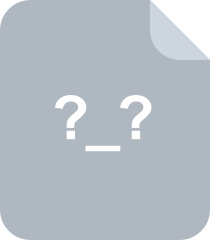
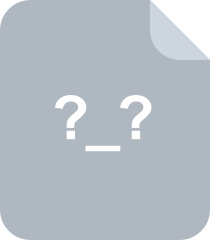
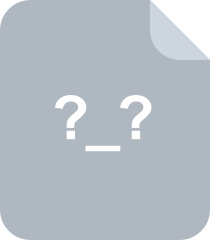
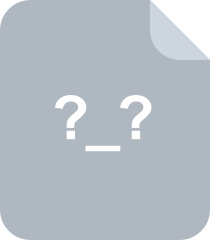
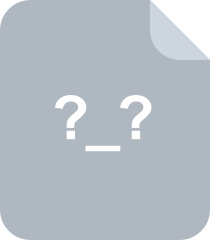
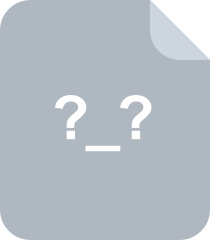
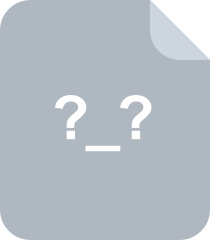
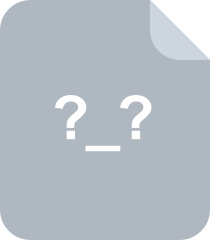

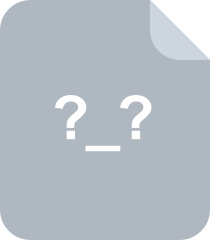
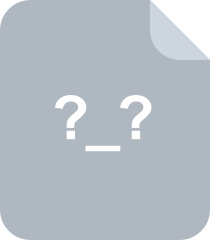
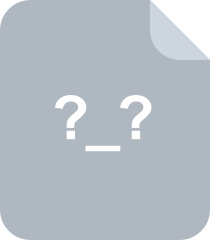
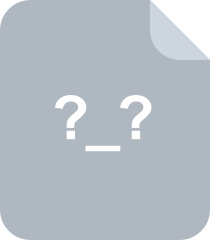
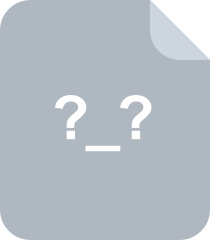
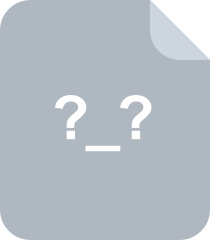
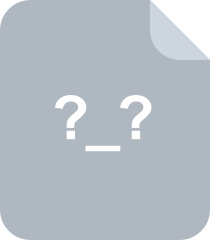
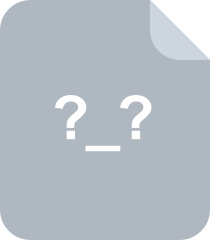
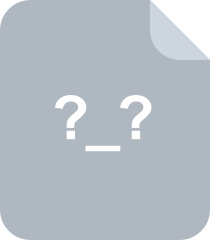
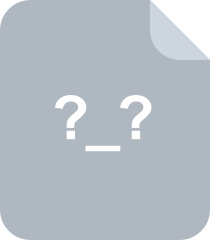
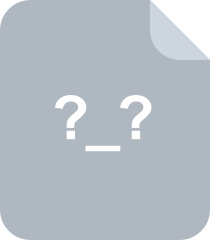
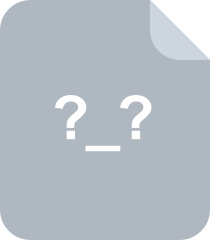
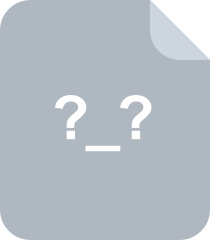
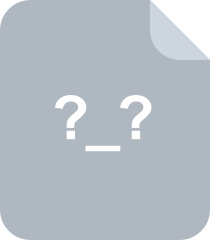
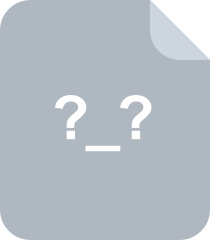
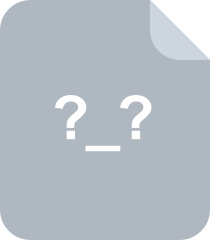
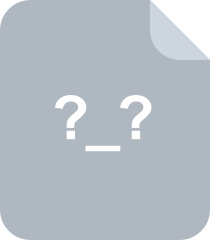
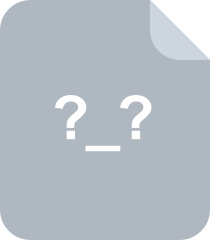
共 27 条
- 1
资源评论
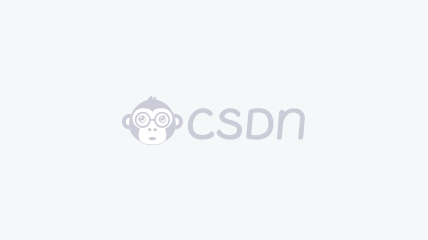

LeapMay
- 粉丝: 3w+
- 资源: 2305
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

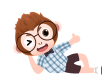
最新资源
- 4_家乡宣传海报.psd
- 基于 SpringCloud 开发的酒店管理系统(PPT+ 开发文档 + 数据库文件 + 源码)
- SM4-CTR代码实现及基本补位示例代码
- 基于SpringBoot的超市订单和收银管理系统源码+数据库脚本+说明文档(毕业设计).zip
- Links Challenging Puzzle Game Template v1.2
- 基于 Unet++ 对人体完整脊柱二分割实战【包含数据集、完整代码、训练好的结果】
- 基于javaweb的超市订单和收银管理系统源码+数据库脚本+说明文档(毕业设计).zip
- Scratch 街机游戏:极限街机.sb3
- Java项目-基于Springboot+Vue的心灵治愈交流平台的设计与实现(源码+万字LW+部署视频+代码讲解视频+全套软件)
- Java项目-基于Springboot+Vue的滴答拍摄影项目的设计与实现(源码+万字LW+部署视频+代码讲解视频+全套软件)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


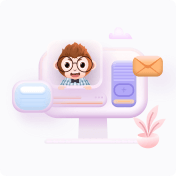
安全验证
文档复制为VIP权益,开通VIP直接复制
