<?php
/*
* phpMyEdit - instant MySQL table editor and code generator
*
* phpMyEdit.class.php - main table editor class definition file
* ____________________________________________________________
*
* Copyright (c) 1999-2002 John McCreesh <jpmcc@users.sourceforge.net>
* Copyright (c) 2001-2002 Jim Kraai <jkraai@users.sourceforge.net>
* Versions 5.0 and higher developed by Ondrej Jombik <nepto@php.net>
* Copyright (c) 2002-2006 Platon Group, http://platon.sk/
* All rights reserved.
*
* See README file for more information about this software.
* See COPYING file for license information.
*
* Download the latest version from
* http://platon.sk/projects/phpMyEdit/
*/
/* $Platon: phpMyEdit/phpMyEdit.class.php,v 1.199 2007-09-02 21:48:32 nepto Exp $ */
/* This is a generic table editing program. The table and fields to be
edited are defined in the calling program.
This program works in three passes.
* Pass 1 (the last part of the program) displays the selected SQL
table in a scrolling table on the screen. Radio buttons are used to
select a record for editing or deletion. If the user chooses Add,
Change, Copy, View or Delete buttons.
* Pass 2 starts, displaying the selected record. If the user chooses
the Save button from this screen.
* Pass 3 processes the update and the display returns to the
original table view (Pass 1).
*/
class phpMyEdit_timer /* {{{ */
{
var $startTime;
var $started;
function phpMyEdit_timer($start = true)
{
$this->started = false;
if ($start) {
$this->start();
}
}
function start()
{
$startMtime = explode(' ', microtime());
$this->startTime = (double) $startMtime[0] + (double) $startMtime[1];
$this->started = true;
}
function end($iterations = 1)
{
// get the time, check whether the timer was started later
$endMtime = explode(' ', microtime());
if ($this->started) {
$endTime = (double)($endMtime[0])+(double)($endMtime[1]);
$dur = $endTime - $this->startTime;
$avg = 1000 * $dur / $iterations;
$avg = round(1000 * $avg) / 1000;
return $avg;
} else {
return 'phpMyEdit_timer ERROR: timer not started';
}
}
} /* }}} */
if (! function_exists('array_search')) { /* {{{ */
function array_search($needle, $haystack)
{
foreach ($haystack as $key => $value) {
if ($needle == $value)
return $key;
}
return false;
}
} /* }}} */
if (! function_exists('realpath')) { /* {{{ */
function realpath($path)
{
return $path;
}
} /* }}} */
class phpMyEdit
{
// Class variables {{{
// Database handling
var $hn; // hostname
var $un; // user name
var $pw; // password
var $tb; // table
var $db; // database
var $dbp; // database with point and delimiters
var $dbh; // database handle
var $close_dbh; // if database handle should be closed
// Record manipulation
var $key; // name of field which is the unique key
var $key_num; // number of field which is the unique key
var $key_type; // type of key field (int/real/string/date etc.)
var $key_delim; // character used for key value quoting
var $rec; // number of record selected for editing
var $inc; // number of records to display
var $fm; // first record to display
var $fl; // is the filter row displayed (boolean)
var $fds; // sql field names
var $fdn; // sql field names => $k
var $num_fds; // number of fields
var $options; // options for users: ACDFVPI
var $fdd; // field definitions
var $qfn; // value of all filters used during the last pass
var $sfn; // sort field number (- = descending sort order)
var $cur_tab; // current selected tab
// Operation
var $navop; // navigation buttons/operations
var $sw; // filter display/hide/clear button
var $operation; // operation to do: Add, Change, Delete
var $saveadd;
var $moreadd;
var $canceladd;
var $savechange;
var $morechange;
var $cancelchange;
var $savecopy;
var $cancelcopy;
var $savedelete;
var $canceldelete;
var $cancelview;
// Additional features
var $labels; // multilingual labels
var $cgi; // CGI variable features array
var $js; // JS configuration array
var $dhtml; // DHTML configuration array
var $url; // URL array
var $message; // informational message to print
var $notify; // change notification e-mail adresses
var $logtable; // name of optional logtable
var $navigation; // navigation style
var $tabs; // TAB names
var $timer = null; // phpMyEdit_timer object
var $sd; var $ed; // sql start and end delimiters '`' in case of MySQL
// Predefined variables
var $comp_ops = array('<'=>'<','<='=>'<=','='=>'=','>='=>'>=','>'=>'>');
var $sql_aggrs = array(
'sum' => 'Total',
'avg' => 'Average',
'min' => 'Minimum',
'max' => 'Maximum',
'count' => 'Count');
var $page_types = array(
'L' => 'list',
'F' => 'filter',
'A' => 'add',
'V' => 'view',
'C' => 'change',
'P' => 'copy',
'D' => 'delete'
);
var $default_buttons = array(
'L' => array('<<','<','add','view','change','copy','delete','>','>>','goto','goto_combo'),
'F' => array('<<','<','add','view','change','copy','delete','>','>>','goto','goto_combo'),
'A' => array('save','more','cancel'),
'C' => array('save','more','cancel'),
'P' => array('save', 'cancel'),
'D' => array('save','cancel'),
'V' => array('change','cancel')
);
// }}}
/*
* column specific functions
*/
function col_has_sql($k) { return isset($this->fdd[$k]['sql']); }
function col_has_sqlw($k) { return isset($this->fdd[$k]['sqlw']) && !$this->virtual($k); }
function col_has_values($k) { return isset($this->fdd[$k]['values']) || isset($this->fdd[$k]['values2']); }
function col_has_php($k) { return isset($this->fdd[$k]['php']); }
function col_has_URL($k) { return isset($this->fdd[$k]['URL'])
|| isset($this->fdd[$k]['URLprefix']) || isset($this->fdd[$k]['URLpostfix']); }
function col_has_multiple($k)
{ return $this->col_has_multiple_select($k) || $this->col_has_checkboxes($k); }
function col_has_multiple_select($k)
{ return $this->fdd[$k]['select'] == 'M' && ! $this->fdd[$k]['values']['table']; }
function col_has_checkboxes($k)
{ return $this->fdd[$k]['select'] == 'C' && ! $this->fdd[$k]['values']['table']; }
function col_has_radio_buttons($k)
{ return $this->fdd[$k]['select'] == 'O' && ! $this->fdd[$k]['values']['table']; }
function col_has_datemask($k)
{ return isset($this->fdd[$k]['datemask']) || isset($this->fdd[$k]['strftimemask']); }
/*
* functions for indicating whether navigation style is enabled
*/
function nav_buttons() { return stristr($this->navigation, 'B'); }
function nav_text_links() { return stristr($this->navigation, 'T'); }
function nav_graphic_links() { return stristr($this->navigation, 'G'); }
function nav_up() { return (stristr($this->navigation, 'U') && !($this->buttons[$this->page_type]['up'] === false)); }
function nav_down() { return (stristr($this->navigation, 'D') && !($this->buttons[$this->page_type]['down'] === false)); }
/*
* functions for indicating whether operations are enabled
*/
function add_enabled() { return stristr($this->options, 'A'); }
function change_enabled() { return stristr($this->options, 'C'); }
function delete_enabled() { return stristr($this->options, 'D'); }
function filter_enabled() { return stristr($this->options, 'F'); }
function view_enabled() { return stristr($this->options, 'V'); }
function copy_enabled() { return stristr($this->options, 'P') && $this->add_enabled(); }
function tabs_enabled() { return $this->display['tabs'] && count($this->tabs) > 0; }
function hidden($k) { return stristr($this->fdd[$k]['input'],'H'); }
function password($k) { return stristr($this->fdd[$k]['input'],'W'); }
function readonly($k) { return stristr($this->fdd[$k]['input'],'R') || $this->virtual($k); }
function virtual($k) { return stristr($this->fdd[$k]['input'],'V') && $this->col_has_sql($k); }
function add_operation()
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【项目资源】:包含前端、后端、移动开发、操作系统、人工智能、物联网、信息化管理、数据库、硬件开发、大数据、课程资源、音视频、网站开发等各种技术项目的源码。包括STM32、ESP8266、PHP、QT、Linux、iOS、C++、Java、python、web、C#、EDA、proteus、RTOS等项目的源码。【项目质量】:所有源码都经过严格测试,可以直接运行。功能在确认正常工作后才上传。【适用人群】:适用于希望学习不同技术领域的小白或进阶学习者。可作为毕设项目、课程设计、大作业、工程实训或初期项目立项。【附加价值】:项目具有较高的学习借鉴价值,也可直接拿来修改复刻。对于有一定基础或热衷于研究的人来说,可以在这些基础代码上进行修改和扩展,实现其他功能。【沟通交流】:有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。鼓励下载和使用,并欢迎大家互相学习,共同进步。
资源推荐
资源详情
资源评论
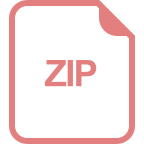
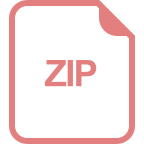
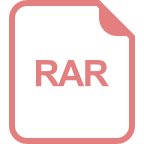
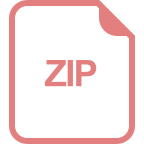
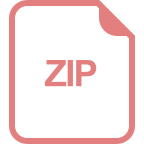
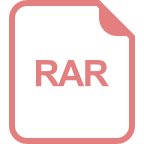
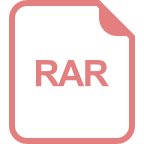
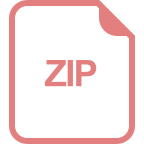
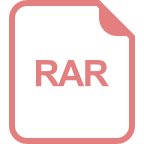
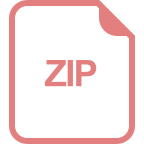
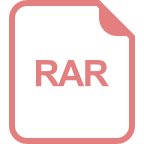
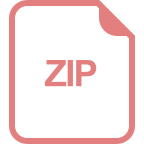
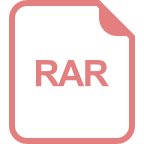
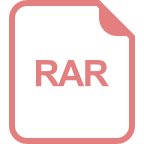
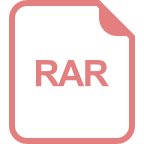
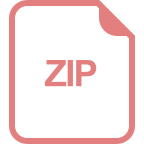
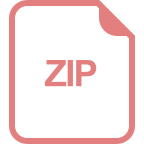
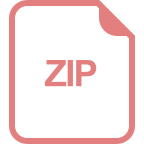
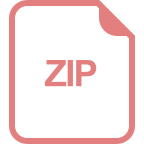
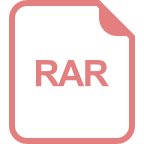
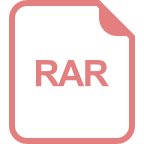
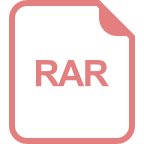
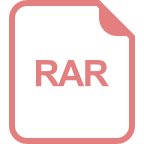
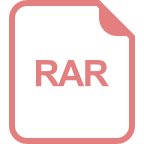
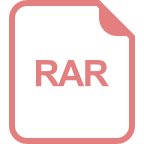
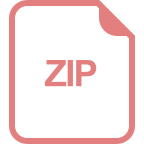
收起资源包目录




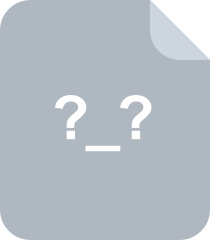
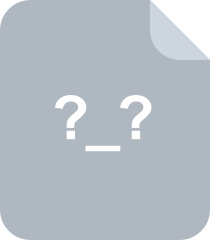
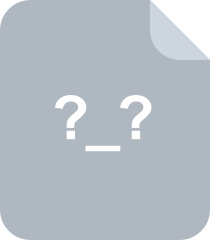
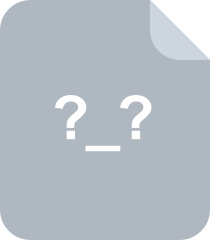
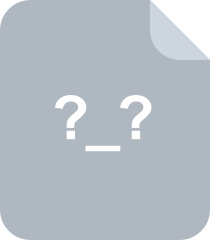
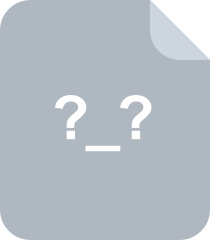
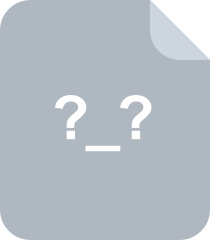
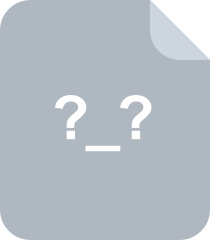
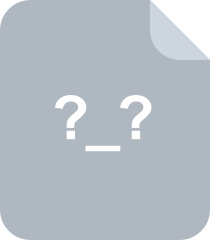
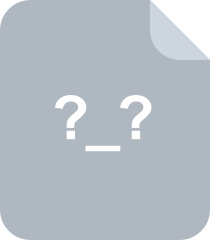
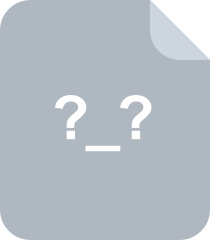
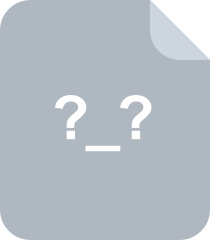
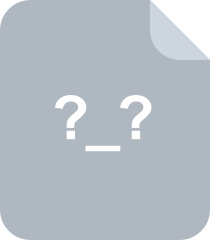
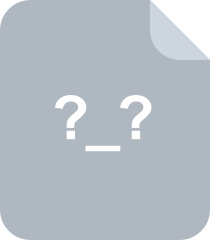
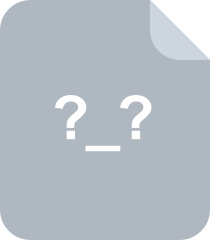
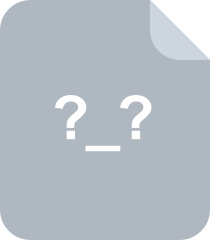
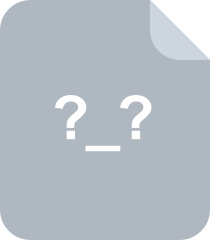
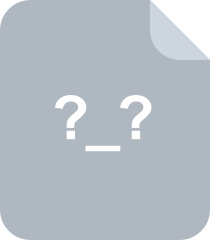
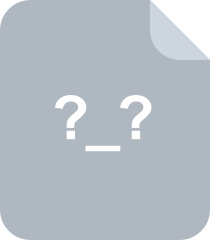
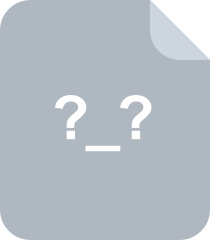
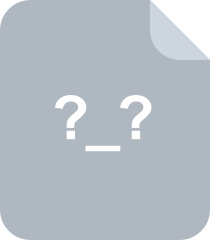
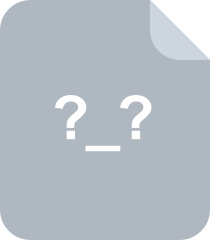
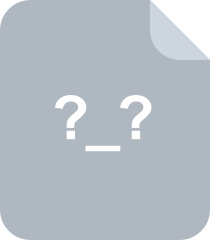
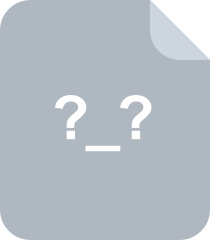
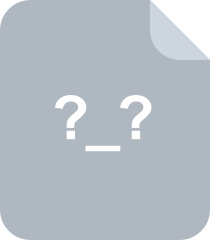
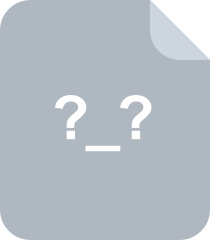
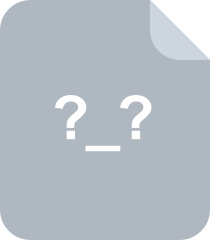

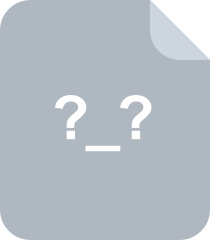
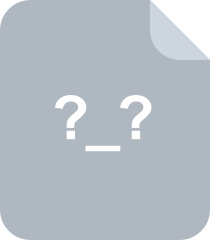
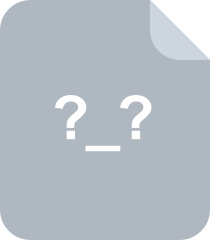
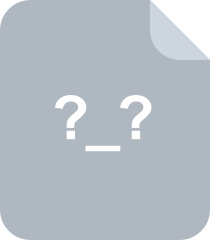
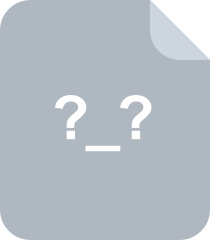
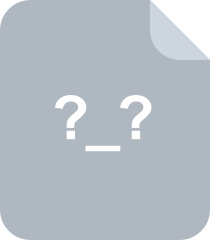
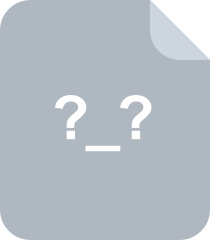
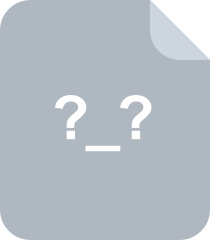
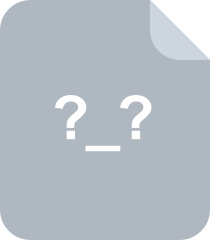

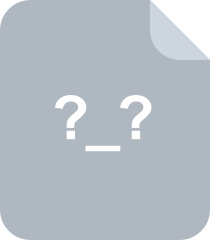
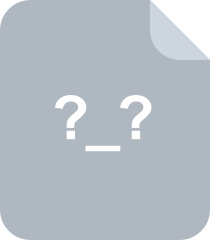
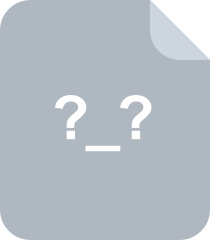


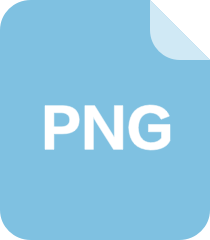
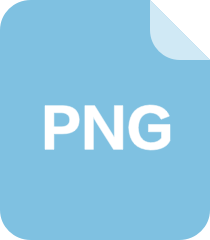
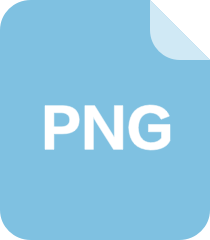
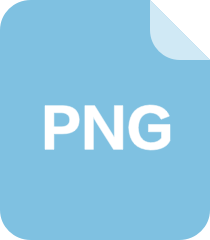
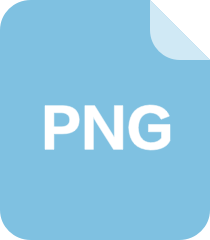
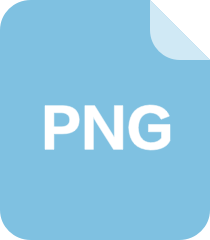
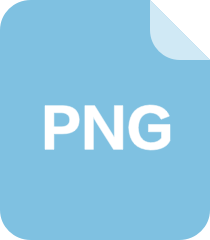
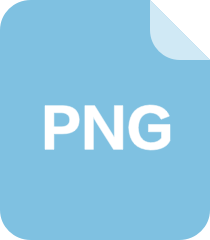
共 47 条
- 1
资源评论
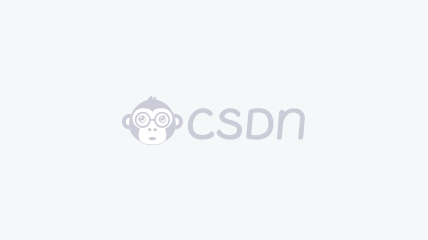
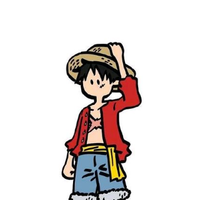
CrMylive.
- 粉丝: 1w+
- 资源: 4万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

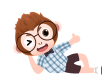
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


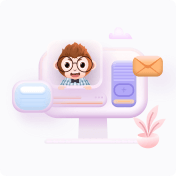
安全验证
文档复制为VIP权益,开通VIP直接复制
