<?
include_once("myconnect.php");
include_once("logincheck.php");
include_once("check_msg_function.php");
function RTESafe($strText) {
//returns safe code for preloading in the RTE
$tmpString = trim($strText);
//convert all types of single quotes
$tmpString = str_replace(chr(145), chr(39), $tmpString);
$tmpString = str_replace(chr(146), chr(39), $tmpString);
$tmpString = str_replace("'", "'", $tmpString);
//convert all types of double quotes
$tmpString = str_replace(chr(147), chr(34), $tmpString);
$tmpString = str_replace(chr(148), chr(34), $tmpString);
// $tmpString = str_replace("\"", "\"", $tmpString);
//replace carriage returns & line feeds
$tmpString = str_replace(chr(10), " ", $tmpString);
$tmpString = str_replace(chr(13), " ", $tmpString);
return $tmpString;
}
$errcnt=0;
if(count($_POST)<>0) //IF SOME FORM WAS POSTED DO VALIDATION
{
$config=mysql_fetch_array(mysql_query("select * from b2b_config"));
$sql="Select * from b2b_groups where sb_memtype=".$_SESSION["b2b_memtype"];
$rs0_query=mysql_query($sql);
$rs0=mysql_fetch_array($rs0_query);
$cats=$rs0["sb_profilecat_cnt"];
$allowed= $rs0["sb_profile"];
$posturl= $rs0["sb_posturl"];
if(!get_magic_quotes_gpc())
{
$companyname=str_replace("$","\$",addslashes($_REQUEST["companyname"]));
$logo=str_replace("$","\$",addslashes($_REQUEST["list1"]));
$services=str_replace("$","\$",addslashes($_REQUEST["services"]));
$yearestablished=str_replace("$","\$",addslashes($_REQUEST["yearestablished"]));
$othermarkets=str_replace("$","\$",addslashes($_REQUEST["othermarkets"]));
$companyprofile=str_replace("$","\$",addslashes($_REQUEST["companyprofile"]));
$ceo=str_replace("$","\$",addslashes($_REQUEST["ceo"]));
$phone=str_replace("$","\$",addslashes($_REQUEST["phone"]));
$phone1=str_replace("$","\$",addslashes($_REQUEST["phone1"]));
$phone2=str_replace("$","\$",addslashes($_REQUEST["phone2"]));
$fax=str_replace("$","\$",addslashes($_REQUEST["fax"]));
$fax1=str_replace("$","\$",addslashes($_REQUEST["fax1"]));
$fax2=str_replace("$","\$",addslashes($_REQUEST["fax2"]));
$website=str_replace("$","\$",addslashes($_REQUEST["website"]));
}
else
{
$companyname=str_replace("$","\$",$_REQUEST["companyname"]);
$logo=str_replace("$","\$",$_REQUEST["list1"]);
$services=str_replace("$","\$",$_REQUEST["services"]);
$yearestablished=str_replace("$","\$",$_REQUEST["yearestablished"]);
$othermarkets=str_replace("$","\$",$_REQUEST["othermarkets"]);
$companyprofile=str_replace("$","\$",$_REQUEST["companyprofile"]);
$ceo=str_replace("$","\$",$_REQUEST["ceo"]);
$phone=str_replace("$","\$",$_REQUEST["phone"]);
$phone1=str_replace("$","\$",$_REQUEST["phone1"]);
$phone2=str_replace("$","\$",$_REQUEST["phone2"]);
$fax=str_replace("$","\$",$_REQUEST["fax"]);
$fax1=str_replace("$","\$",$_REQUEST["fax1"]);
$fax2=str_replace("$","\$",$_REQUEST["fax2"]);
$website=str_replace("$","\$",$_REQUEST["website"]);
}
$phone_no="";
if(strlen(trim($phone))<>0)
{$phone_no.=$phone;}
$phone_no.="-";
if(strlen(trim($phone1))<>0)
{$phone_no.=$phone1;}
$phone_no.="-";
if(strlen(trim($phone2))<>0)
{$phone_no.=$phone2;}
$fax_no="";
if(strlen(trim($fax))<>0)
{$fax_no.=$fax;}
$fax_no.="-";
if(strlen(trim($fax1))<>0)
{$fax_no.=$fax1;}
$fax_no.="-";
if(strlen(trim($fax2))<>0)
{$fax_no.=$fax2;}
$markets="0";
$rs_query_t=mysql_query("select * from b2b_markets order by sb_market");
$cnt=1;
while( ( $rs_t=mysql_fetch_array($rs_query_t) ))
{
$indx="market".$cnt;
$cnt++;
if ( isset($_REQUEST[$indx]) )
{
$markets =($markets==0)?$rs_t["sb_id"]:$markets.",".$rs_t["sb_id"];
}
}
$markets_arr=explode(",",$markets);
$cat_name=str_replace(";",",",$_REQUEST["category"]);
$sbcid_list=str_replace(";",",",$_REQUEST["cid"]);
$cat=explode(",",$sbcid_list);
if ( strlen(trim($companyname)) == 0 )
{
$errs[$errcnt]="Company Name must be provided";
$errcnt++;
}
elseif(preg_match ("/[;<>&]/", $_REQUEST["companyname"]))
{
$errs[$errcnt]="Company Name can not have any special character (e.g. & ; < >)";
$errcnt++;
}
if ( $_REQUEST["businesstype"]=="" )
{
$errs[$errcnt]="Business Type must be choosen";
$errcnt++;
}
if ( $_REQUEST["cid"]=="")
{
$errs[$errcnt]="Atleast one Category must be provided";
$errcnt++;
}
if ( count($cat)>$cats)
{
$errs[$errcnt]="Too many Categories provided";
$errcnt++;
}
if ( strlen(trim($services)) == 0 )
{
$errs[$errcnt]="Product/Services must be specified.";
$errcnt++;
}
elseif( $config["sb_profile_approval"] == 'auto')
{
if ( check_msg($services,0) == 'yes' )
{
$errs[$errcnt]="Product/Services must not contain bad words";
$errcnt++;
}
}
/*elseif(preg_match ("/[;<>&]/", $_REQUEST["services"]))
{
$errs[$errcnt]="Product/Services can not have any special character (e.g. & ; < >)";
$errcnt++;
}*/
if ( $markets=="-1" && (strlen(trim($othermarkets)) == 0))
{
$errs[$errcnt]="At least one market must be choosen";
$errcnt++;
}
if ( $_REQUEST["productfocus"]=="" )
{
$errs[$errcnt]="Product Focus must be choosen";
$errcnt++;
}
if ( $_REQUEST["employees"]=="" )
{
$errs[$errcnt]="Employees must be choosen";
$errcnt++;
}
if ( strlen(trim($companyprofile)) == 0 )
{
$errs[$errcnt]="Company Profile must be given";
$errcnt++;
}
elseif( $config["sb_profile_approval"] == 'auto')
{
if ( check_msg($companyprofile,0) == 'yes' )
{
$errs[$errcnt]="Company Profile must not contain bad words";
$errcnt++;
}
}
/*elseif(preg_match ("/[;<>&]/", $_REQUEST["companyprofile"]))
{
$errs[$errcnt]="Company Profile can not have any special character (e.g. & ; < >)";
$errcnt++;
}*/
if ( strlen(trim($ceo)) == 0 )
{
$errs[$errcnt]="CEO/Owner's Name Must be provided";
$errcnt++;
}
elseif(preg_match ("/[;<>&]/", $_REQUEST["ceo"]))
{
$errs[$errcnt]="CEO/Owner's Name can not have any special character (e.g. & ; < >)";
$errcnt++;
}
if(preg_match ("/[;<>&]/", $phone_no))
{
$errs[$errcnt]="Phone No. can not have any special character (e.g. & ; < >)";
$errcnt++;
}
if(preg_match ("/[;<>&]/", $fax_no))
{
$errs[$errcnt]="Fax can not have any special character (e.g. & ; < >)";
$errcnt++;
}
if($errcnt==0)
{
$approved="yes";
$sb_msg='Company Profile has been updated.';
if($config["sb_profile_approval"]=="admin")
{
$approved="no";
$sb_msg='Company Profile has been sent for admin approval.';
}
$sb_profile_id=$_POST["profile_id"];
if($_POST["profile_id"]==0)
{
if($approved=="no")
{ $approved="new";}
$insert_query="insert into b2b_companyprofiles (sb_companyname,sb_logo,sb_businesstype,sb_services,sb_yearestablished,sb_othermarkets,sb_productfocus,sb_companyprofile,sb_employees,sb_ceo,sb_website,sb_uid,sb_type,sb_phone,sb_fax,sb_approved,sb_viewed,sb_postedon) values ('$companyname','$logo',".$_REQUEST["businesstype"].",'$services',$yearestablished,'$othermarkets',".$_REQUEST["productfocus"].",'$companyprofile',".$_REQUEST["employees"].",'$ceo','$website',".$_SESSION["b2b_userid"].",0,'$phone_no','$fax_no','$approved',0,'".date("YmdHis",time())."')";
mysql_query($insert_query);
if(mysql_affected_rows()>0)
{
//---------------IMAGE MAGIK CODE--------------------------------------------------------
if($config["sb_image_magik"]=="enable")
{
$size_str=$config["sb_th_width"] . "x" . $config["sb_th_width"];
$path1="uploadedimages" . '/' . $logo;
$path2="thumbs1" . '/' . $logo;
if($config["sb_water_marking"]=="enable")
{
exec("composite -dissolve 20 images/watermark.gif $path1 $path1");
}
exec("convert $path1 -resize $size_str $path
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【项目资源】:包含前端、后端、移动开发、操作系统、人工智能、物联网、信息化管理、数据库、硬件开发、大数据、课程资源、音视频、网站开发等各种技术项目的源码。包括STM32、ESP8266、PHP、QT、Linux、iOS、C++、Java、python、web、C#、EDA、proteus、RTOS等项目的源码。【项目质量】:所有源码都经过严格测试,可以直接运行。功能在确认正常工作后才上传。【适用人群】:适用于希望学习不同技术领域的小白或进阶学习者。可作为毕设项目、课程设计、大作业、工程实训或初期项目立项。【附加价值】:项目具有较高的学习借鉴价值,也可直接拿来修改复刻。对于有一定基础或热衷于研究的人来说,可以在这些基础代码上进行修改和扩展,实现其他功能。【沟通交流】:有任何使用上的问题,欢迎随时与博主沟通,博主会及时解答。鼓励下载和使用,并欢迎大家互相学习,共同进步。
资源推荐
资源详情
资源评论
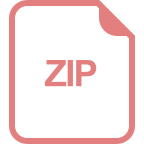
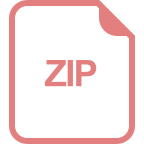
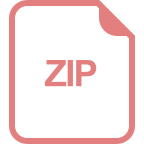
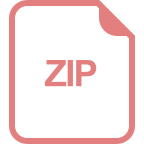
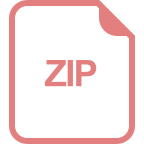
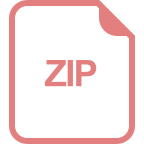
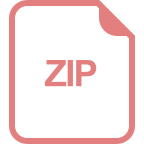
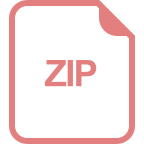
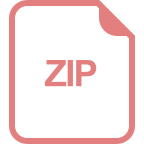
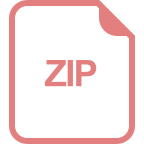
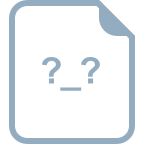
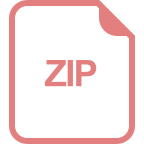
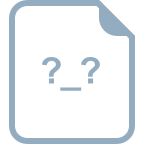
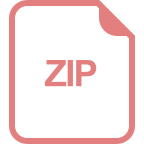
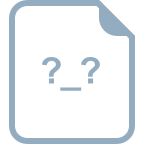
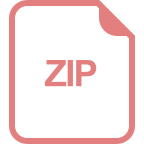
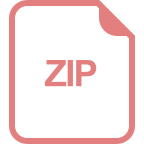
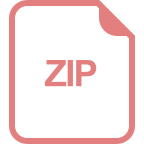
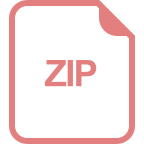
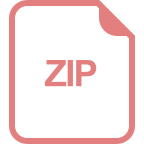
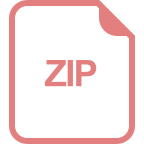
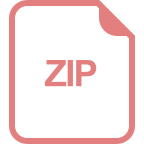
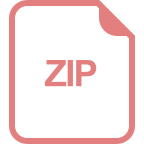
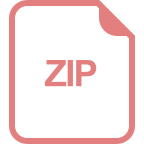
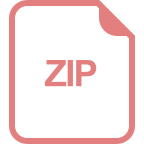
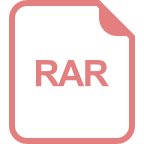
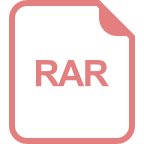
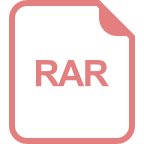
收起资源包目录

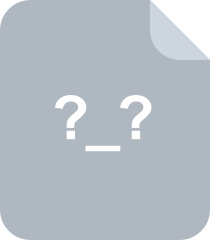
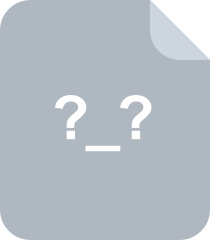
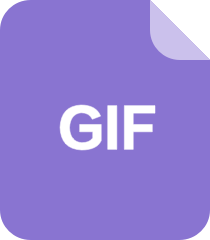
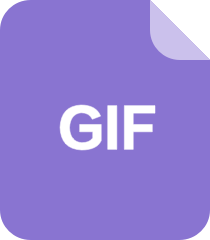
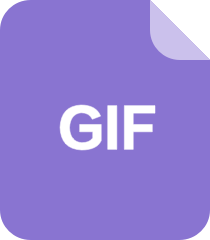
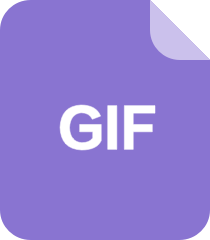
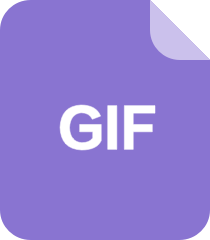
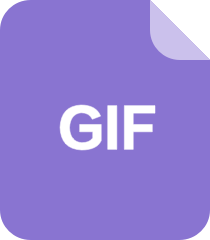
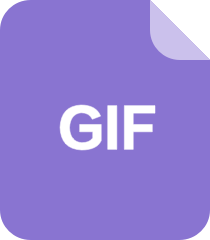
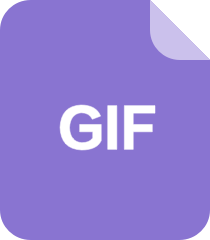
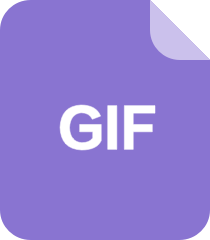
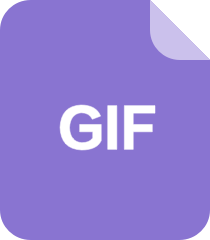
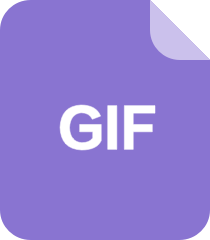
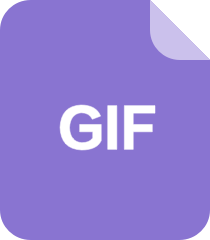
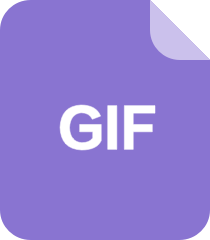
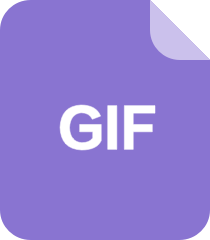
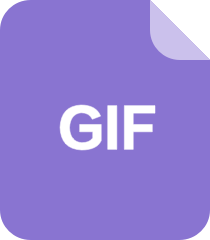
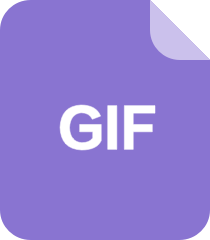
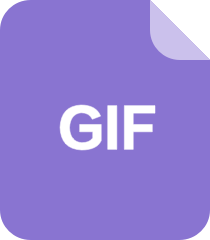
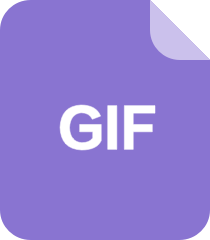
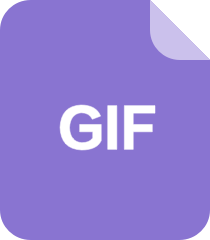
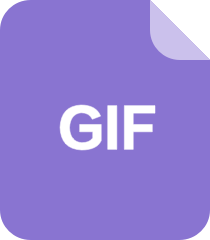
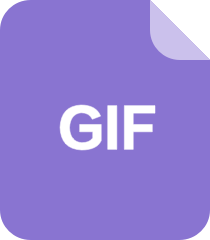
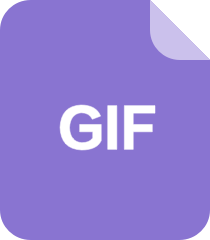
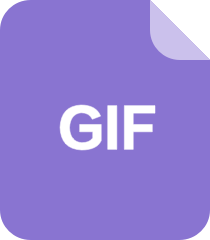
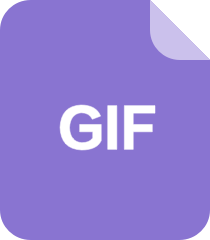
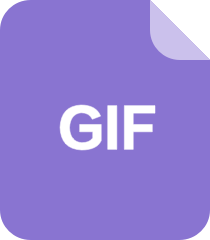
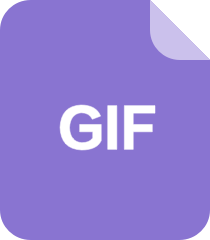
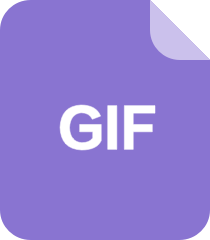
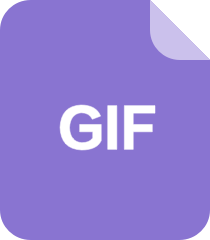
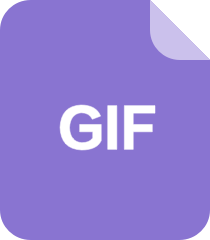
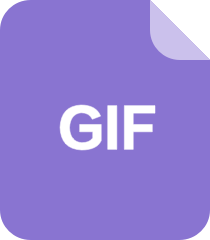
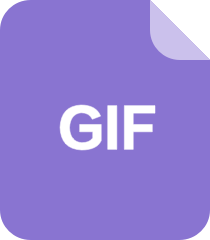
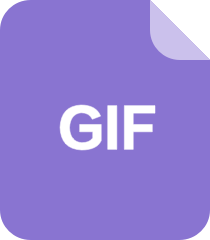
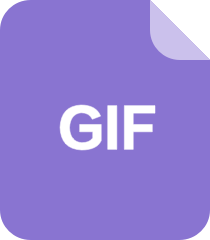
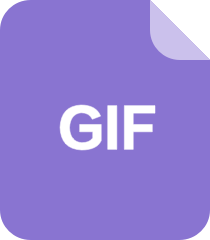
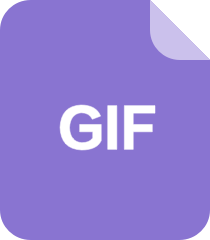
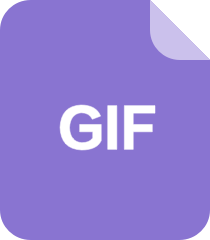
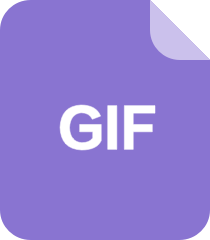
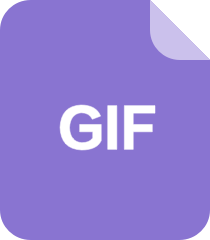
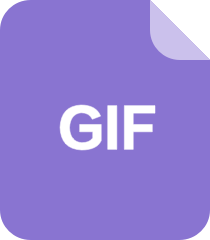
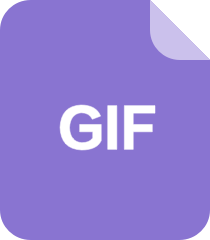
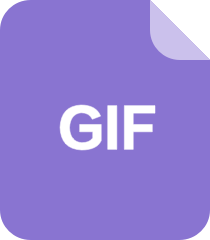
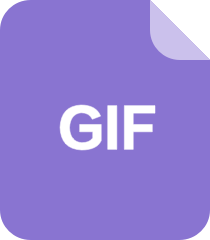
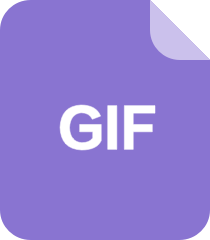
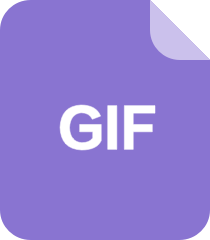
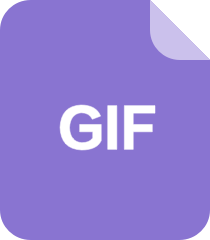
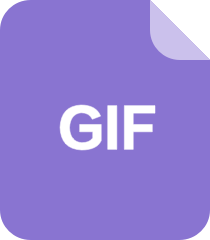
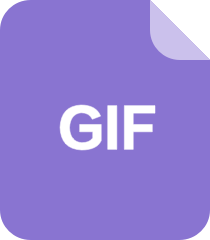
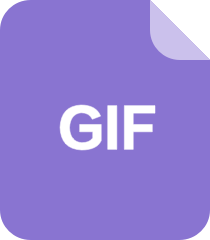
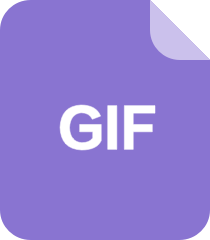
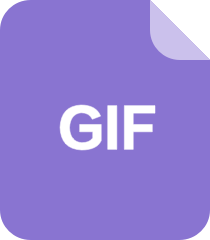
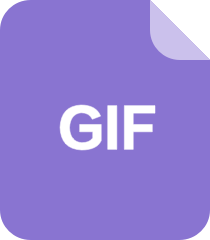
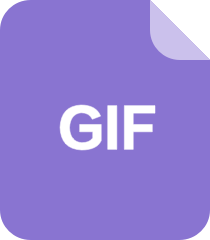
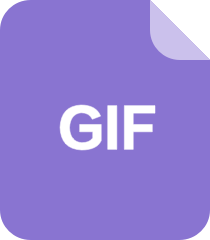
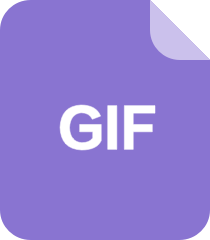
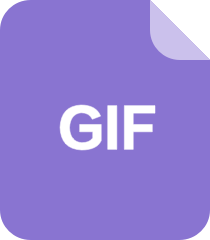
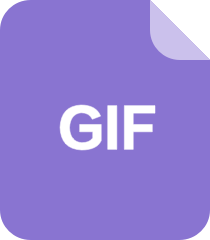
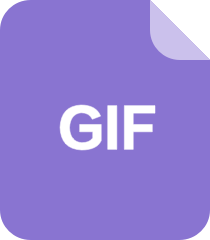
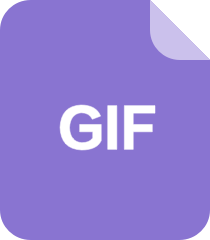
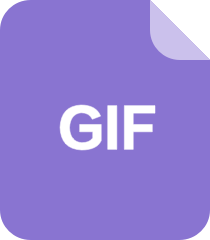
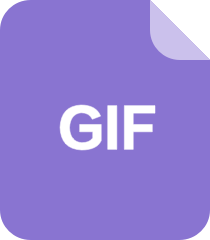
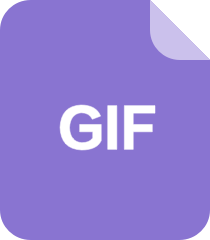
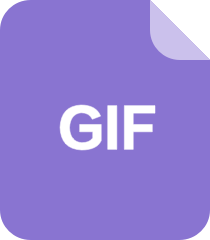
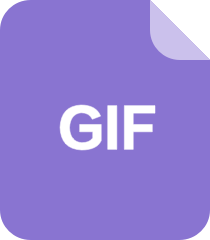
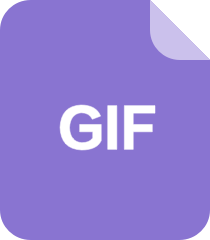
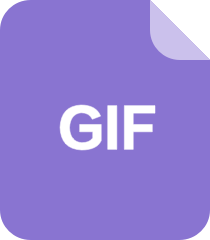
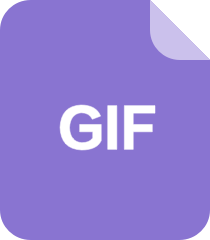
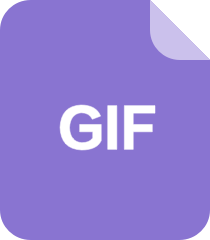
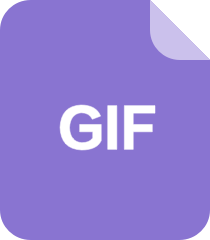
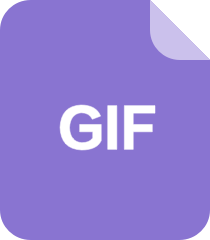
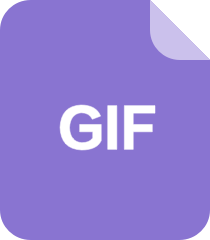
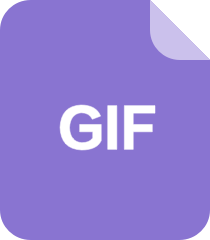
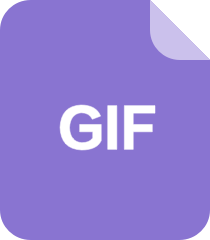
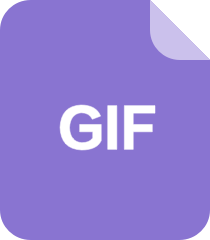
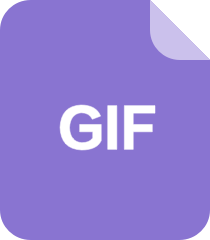
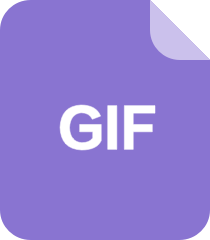
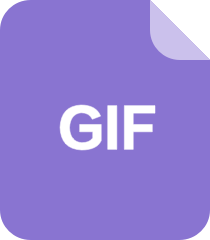
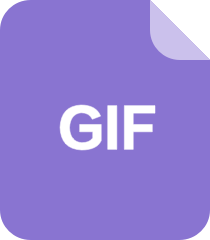
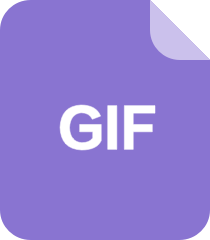
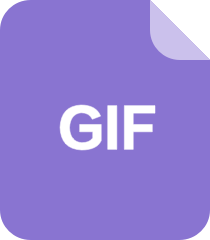
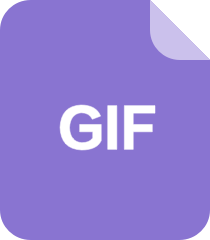
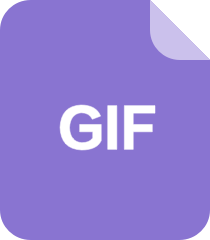
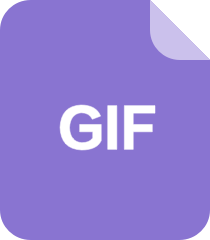
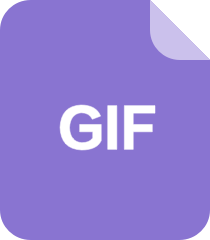
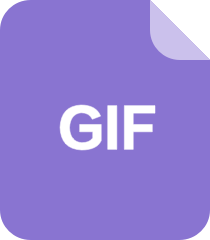
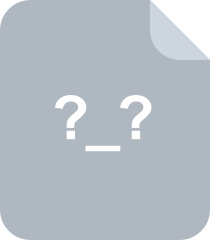
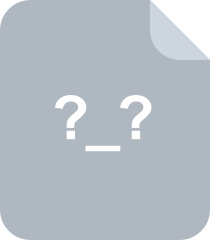
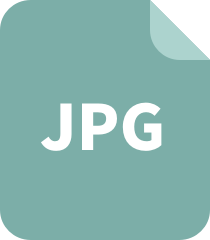
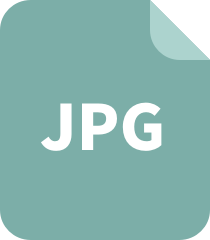
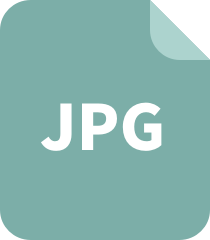
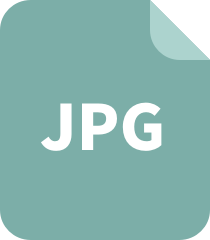
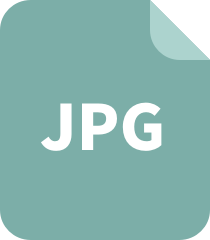
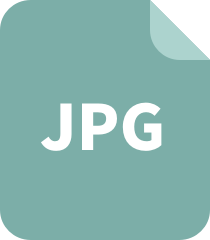
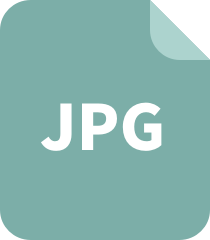
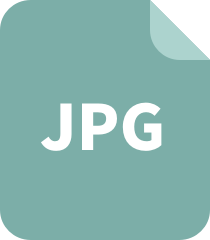
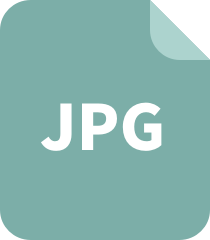
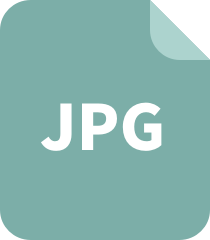
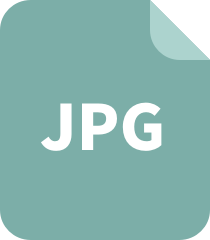
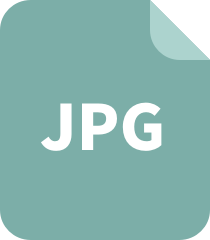
共 373 条
- 1
- 2
- 3
- 4
资源评论
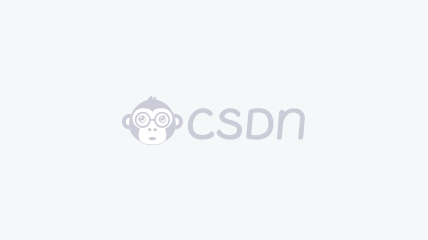
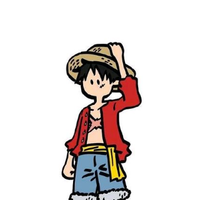
CrMylive.
- 粉丝: 1w+
- 资源: 4万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

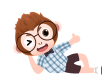
最新资源
- C#ASP.NET大型制造业进销存管理系统源码数据库 SQL2008源码类型 WebForm
- liunx project 2
- (源码)基于ROS框架的智能无人机作业系统.zip
- 网页打包封装器V1.0支持苹果IOS/安卓/分发打包
- (源码)基于BERT的KBQA问答系统.zip
- (源码)基于Java和Python的笔声语音识别系统.zip
- 网络安全基础实验1-使用python中cryptography库进行对称加密和解密实验
- Python数电的课程设计网络同步时钟.zip
- (源码)基于Arduino的天文数据库管理系统.zip
- C#餐饮管理系统源码 触摸屏餐饮管理系统源码数据库 SQL2008源码类型 WinForm
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


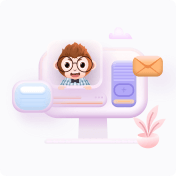
安全验证
文档复制为VIP权益,开通VIP直接复制
