在图像处理领域,FBP(Filtered Back Projection,滤波反投影)是一种常见的计算机断层扫描(CT)图像重建算法。该算法通过应用傅立叶变换和逆变换来恢复物体内部结构的二维图像。本主题主要关注FBP算法中的两个关键环节:滤波器和插值方法,以及它们对重建图像质量的影响。我们将深入探讨这两个概念,并结合MATLAB环境进行分析。 滤波器在FBP中起着至关重要的作用。在傅立叶域中,滤波器可以消除噪声并改善图像的分辨率。常见的滤波器有Ram-Lak滤波器、Hann滤波器、Shepp-Logan滤波器等。Ram-Lak滤波器是最简单的形式,它对应于理想的低通滤波器,适用于初步理解FBP算法。Hann滤波器和Shepp-Logan滤波器则考虑了更复杂的频率特性,能够提供更好的图像质量,但计算上更为复杂。在MATLAB中,可以使用`ifftshift`和`fft2`函数实现傅立叶变换和逆变换,同时利用自定义函数或内置滤波函数进行滤波操作。 插值方法是FBP中用于提高空间分辨率的关键步骤。原始的投影数据通常是离散的,而反投影过程需要连续的数据。因此,需要通过插值来估计缺失的像素值。常见的插值方法有最近邻插值、双线性插值和立方插值等。最近邻插值简单快速,但可能导致图像锯齿状边缘;双线性插值平滑效果更好,但可能引入轻微的模糊;立方插值则提供了更高的精度,但计算量相对较大。在MATLAB中,可以使用`imresize`函数进行不同类型的插值操作。 进行有无滤波器及插值方式的比较时,通常会观察重建图像的对比度、噪声水平和边缘清晰度。没有滤波器的FBP可能会导致噪声增强和分辨率下降,而合适的滤波器能有效抑制噪声并提高细节可见性。同样,不同的插值方法将影响图像的整体质量和细节保真度。通过MATLAB编程,可以对比不同组合下的重建结果,进一步理解滤波器和插值方式的选择对图像重建质量的影响。 为了实现这个比较,你可以编写MATLAB代码,先读取原始投影数据,然后分别应用不同的滤波器和插值方法进行重建。使用`imshow`或`imagesc`函数展示重建图像,通过肉眼观察或量化指标(如均方误差、峰值信噪比PSNR)来评估结果。这样,你不仅可以直观地看到不同选择的效果,还可以定量地比较它们的优劣。 滤波器和插值方式是FBP算法中决定重建图像质量的重要因素。在MATLAB环境下,通过实际操作和比较,我们可以深入理解这些概念,并优化图像重建过程,以获得最佳的图像质量。这不仅有助于理论学习,也为实际的CT成像技术提供了有价值的参考。
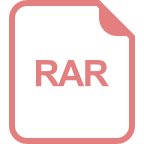
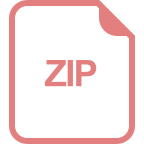
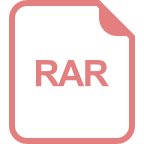
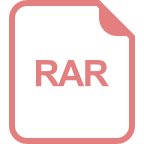
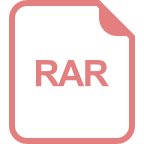
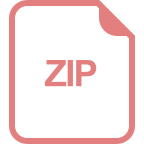
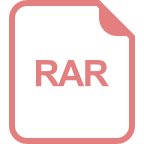
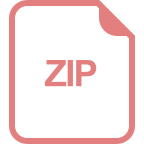
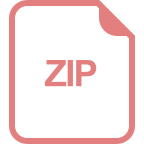
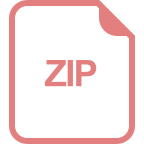
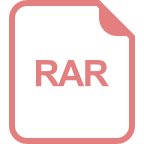


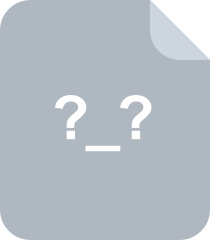
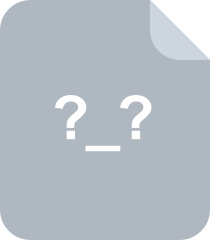
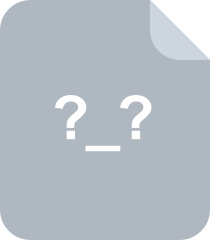
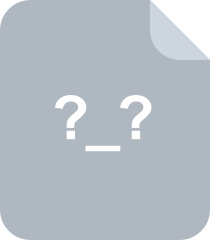
- 1
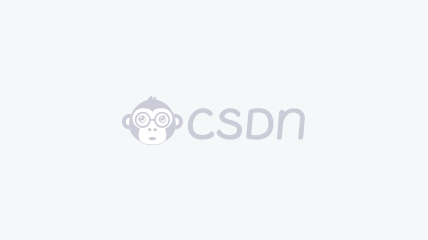
- qq_427574322019-08-20不错。。。
- qq_407756512018-04-21很有用谢谢

- 粉丝: 4w+
- 资源: 3
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

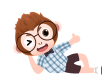
最新资源
- 学校课程软件工程常见10道题目以及答案demo
- javaweb新手开发中常见的目录结构讲解
- 新手小白的git使用的手册入门学习demo
- 基于Java观察者模式的info-express多对多广播通信框架设计源码
- 利用python爬取豆瓣电影评分简单案例demo
- 机器人开发中常见的几道问题以及答案demo
- 基于SpringBoot和layuimini的简洁美观后台权限管理系统设计源码
- 实验报告五六代码.zip
- hdw-dubbo-ui基于vue、element-ui构建开发,实现后台管理前端功能.zip
- (Grafana + Zabbix + ASP.NET Core 2.1 + ECharts + Dapper + Swagger + layuiAdmin)基于角色授权的权限体系.zip

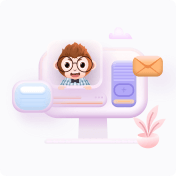
