java发http请求(post&get)
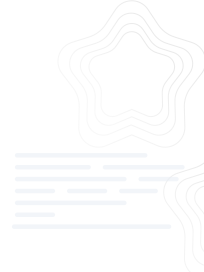

在Java编程中,发送HTTP请求是常见的任务,无论是获取网页数据、调用API接口还是进行自动化测试,都可能需要这个功能。本篇文章将详细介绍如何使用Java实现POST和GET两种HTTP请求方法。 GET请求是最基础的HTTP请求方式,通常用于获取资源。在Java中,我们可以使用`java.net.HttpURLConnection`类来实现GET请求。以下是一个简单的示例: ```java import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; public class GetRequestExample { public static void main(String[] args) throws Exception { String url = "http://example.com"; URL obj = new URL(url); HttpURLConnection con = (HttpURLConnection) obj.openConnection(); // 设置请求方法为GET con.setRequestMethod("GET"); int responseCode = con.getResponseCode(); System.out.println("Response Code : " + responseCode); BufferedReader in = new BufferedReader( new InputStreamReader(con.getInputStream())); String inputLine; StringBuffer response = new StringBuffer(); while ((inputLine = in.readLine()) != null) { response.append(inputLine); } in.close(); // 打印结果 System.out.println(response.toString()); } } ``` 接下来,我们看POST请求,它常用于向服务器提交数据。POST请求同样使用`HttpURLConnection`,但需要设置请求头并写入请求体。下面是一个POST请求的例子: ```java import java.io.OutputStream; import java.net.HttpURLConnection; import java.net.URL; import java.nio.charset.StandardCharsets; public class PostRequestExample { public static void main(String[] args) throws Exception { String url = "http://example.com"; URL obj = new URL(url); HttpURLConnection con = (HttpURLConnection) obj.openConnection(); // 设置请求方法为POST con.setRequestMethod("POST"); // 允许输出,因为POST请求需要写入数据 con.setDoOutput(true); // 设置请求头,例如Content-Type String urlParameters = "param1=value1¶m2=value2"; con.setRequestProperty("Content-Type", "application/x-www-form-urlencoded;charset=UTF-8"); // 写入请求体 try(OutputStream os = con.getOutputStream()) { byte[] input = urlParameters.getBytes(StandardCharsets.UTF_8); os.write(input, 0, input.length); } int responseCode = con.getResponseCode(); System.out.println("Response Code : " + responseCode); if (responseCode == HttpURLConnection.HTTP_OK) { // success BufferedReader in = new BufferedReader(new InputStreamReader(con.getInputStream())); String inputLine; StringBuffer response = new StringBuffer(); while ((inputLine = in.readLine()) != null) { response.append(inputLine); } in.close(); // 打印结果 System.out.println(response.toString()); } else { System.out.println("POST request not worked"); } } } ``` 以上代码展示了Java发送HTTP GET和POST请求的基本步骤。然而,在实际开发中,为了提高代码的可读性和可维护性,我们通常会使用HTTP客户端库,如Apache HttpClient或OkHttp。这些库提供了更高级的功能,如自动处理重定向、超时、连接池等。 Apache HttpClient使用示例: ```java import org.apache.http.HttpEntity; import org.apache.http.client.methods.CloseableHttpResponse; import org.apache.http.client.methods.HttpGet; import org.apache.http.client.methods.HttpPost; import org.apache.http.entity.StringEntity; import org.apache.http.impl.client.CloseableHttpClient; import org.apache.http.impl.client.HttpClients; import org.apache.http.util.EntityUtils; public class HttpClientExample { public static void main(String[] args) throws Exception { CloseableHttpClient httpclient = HttpClients.createDefault(); // GET请求 HttpGet httpGet = new HttpGet("http://example.com"); CloseableHttpResponse responseGet = httpclient.execute(httpGet); try { System.out.println(responseGet.getStatusLine()); HttpEntity entityGet = responseGet.getEntity(); EntityUtils.consume(entityGet); } finally { responseGet.close(); } // POST请求 HttpPost httpPost = new HttpPost("http://example.com"); StringEntity postingString = new StringEntity("param1=value1¶m2=value2"); httpPost.setEntity(postingString); httpPost.setHeader("Content-type", "application/x-www-form-urlencoded"); CloseableHttpResponse responsePost = httpclient.execute(httpPost); try { System.out.println(responsePost.getStatusLine()); HttpEntity entityPost = responsePost.getEntity(); EntityUtils.consume(entityPost); } finally { responsePost.close(); } httpclient.close(); } } ``` OkHttp是一个现代、高效的HTTP客户端,其API简洁且易于使用: ```java import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.RequestBody; import okhttp3.Response; public class OkHttpExample { OkHttpClient client = new OkHttpClient(); // GET请求 public void sendGetRequest() throws IOException { Request request = new Request.Builder() .url("http://example.com") .build(); Response response = client.newCall(request).execute(); response.body().close(); } // POST请求 public void sendPostRequest() throws IOException { RequestBody body = RequestBody.create(MediaType.parse("application/x-www-form-urlencoded"), "param1=value1¶m2=value2"); Request request = new Request.Builder() .url("http://example.com") .post(body) .build(); Response response = client.newCall(request).execute(); response.body().close(); } } ``` 总结,Java中发送HTTP请求的方法多种多样,可以选择标准库的`HttpURLConnection`,也可以选择第三方库如Apache HttpClient和OkHttp。根据项目需求和团队习惯,选择合适的方式即可。在处理网络请求时,务必注意异常处理、连接管理和响应处理,以确保程序的健壮性。
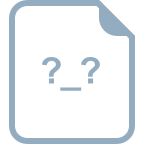
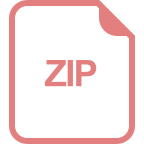
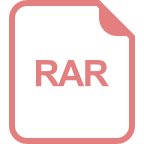
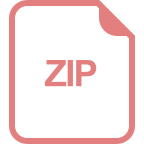
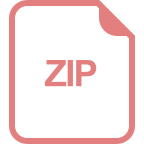
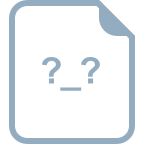
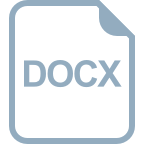
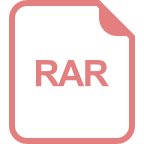
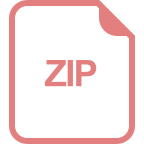
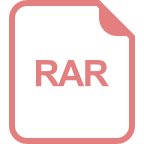
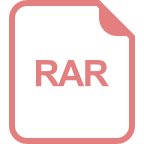
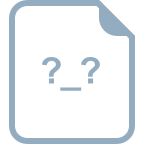
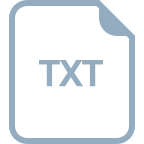
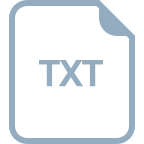
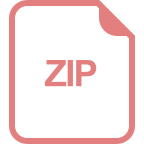


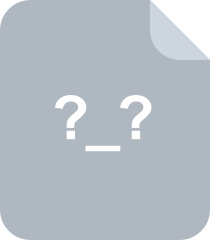
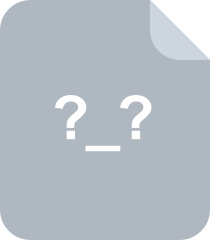
- 1
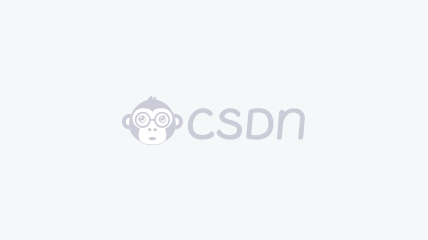

- 粉丝: 0
- 资源: 4
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

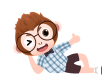
最新资源
- (源码)基于C语言的系统服务框架.zip
- (源码)基于Spring MVC和MyBatis的选课管理系统.zip
- (源码)基于ArcEngine的GIS数据处理系统.zip
- (源码)基于JavaFX和MySQL的医院挂号管理系统.zip
- (源码)基于IdentityServer4和Finbuckle.MultiTenant的多租户身份认证系统.zip
- (源码)基于Spring Boot和Vue3+ElementPlus的后台管理系统.zip
- (源码)基于C++和Qt框架的dearoot配置管理系统.zip
- (源码)基于 .NET 和 EasyHook 的虚拟文件系统.zip
- (源码)基于Python的金融文档智能分析系统.zip
- (源码)基于Java的医药管理系统.zip

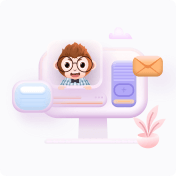
