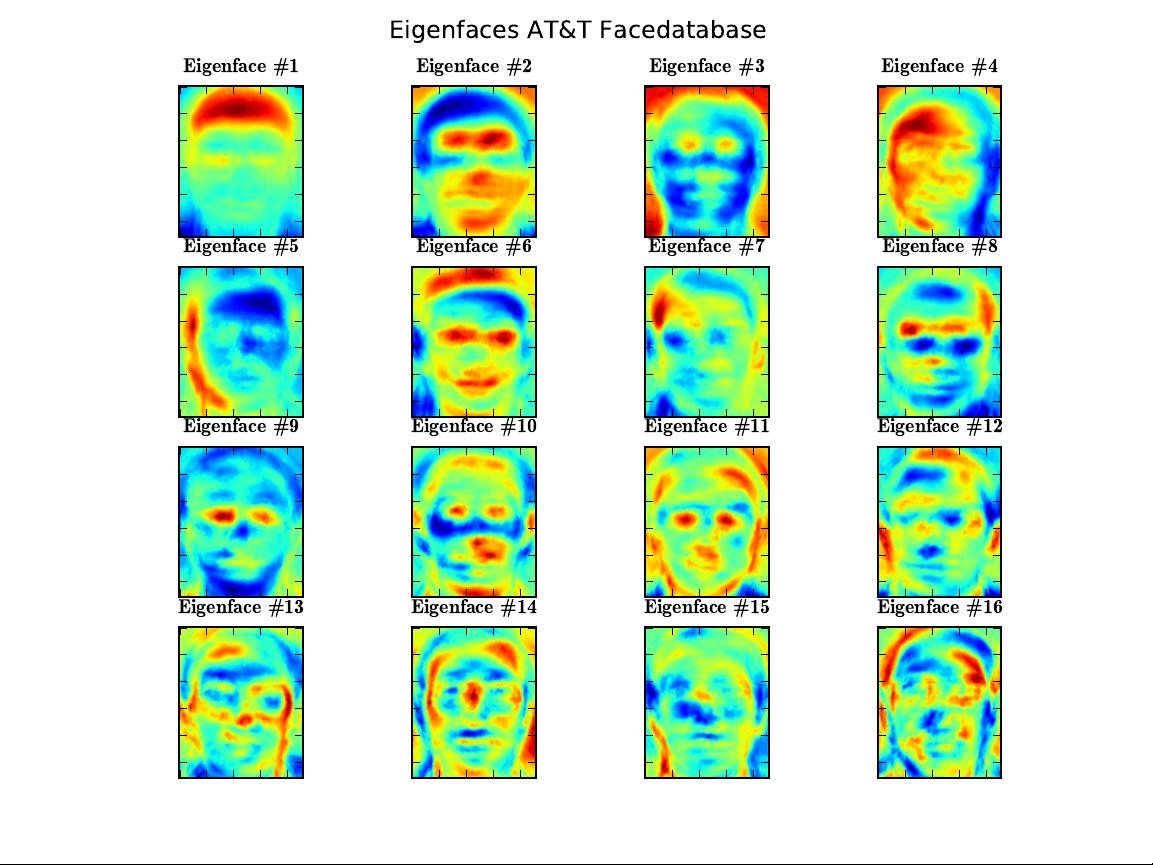
Face Recognition with Python
Philipp Wagner
http://www.bytefish.de
July 18, 2012
Contents
1 Introduction 1
2 Face Recognition 2
2.1 Face Database . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 2
2.1.1 Reading the images with Python . . . . . . . . . . . . . . . . . . . . . . . . . . 3
2.2 Eigenfaces . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
2.2.1 Algorithmic Description . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 4
2.2.2 Eigenfaces in Python . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 5
2.3 Fisherfaces . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
2.3.1 Algorithmic Description . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 10
2.3.2 Fisherfaces in Python . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . 12
3 Conclusion 15
1 Introduction
In this document I’ll show you how to implement the Eigenfaces [13] and Fisherfaces [3] method with
Python, so you’ll understand the basics of Face Recognition. All concepts are explained in detail, but a
basic knowledge of Python is assumed. Originally this document was a Guide to Face Recognition with
OpenCV. Since OpenCV now comes with the cv::FaceRecognizer, this document has been reworked
into the official OpenCV documentation at:
• http://docs.opencv.org/trunk/modules/contrib/doc/facerec/index.html
I am doing all this in my spare time and I simply can’t maintain two separate documents on the
same topic any more. So I have decided to turn this document into a guide on Face Recognition with
Python only. You’ll find the very detailed documentation on the OpenCV cv::FaceRecognizer at:
• FaceRecognizer - Face Recognition with OpenCV
– FaceRecognizer API
– Guide to Face Recognition with OpenCV
– Tutorial on Gender Classification
– Tutorial on Face Recognition in Videos
– Tutorial On Saving & Loading a FaceRecognizer
By the way you don’t need to copy and paste the code snippets, all code has been pushed into my
github repository:
• github.com/bytefish
• github.com/bytefish/facerecognition guide
Everything in here is released under a BSD license, so feel free to use it for your projects. You
are currently reading the Python version of the Face Recognition Guide, you can compile the GNU
Octave/MATLAB version with make octave.
1