SSH学生信息管理系统
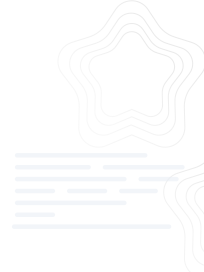


SSH学生信息管理系统是一款基于Struts、Hibernate和Spring框架的Java Web应用,旨在高效地管理和处理学生数据。这个系统为教育机构提供了便捷的方式来存储、检索和更新学生的个人信息、学术记录和其他相关资料。下面将详细介绍SSH框架及其在学生信息管理系统中的应用。 1. **Struts框架**: Struts是Apache组织提供的一个开源MVC(Model-View-Controller)框架,用于构建Web应用程序。在本系统中,Struts负责控制应用程序的流程,处理HTTP请求,将请求分发到相应的业务逻辑组件,并将结果呈现给用户。它通过Action类和配置文件(struts-config.xml)定义了请求和响应的映射,实现了业务逻辑与表现层的解耦。 2. **Hibernate框架**: Hibernate是一个强大的对象关系映射(ORM)工具,简化了Java应用与数据库之间的交互。在SSH系统中,Hibernate负责持久化学生信息,将数据库表与Java对象关联起来,使得开发者可以使用面向对象的方式来操作数据库。它通过实体类(Entity)和映射文件(hbm.xml)定义了数据模型,提供了透明的CRUD(创建、读取、更新、删除)操作。 3. **Spring框架**: Spring是企业级Java应用的核心框架,提供了依赖注入(DI)和面向切面编程(AOP)等特性。在SSH系统中,Spring作为整个应用的“胶水”,整合了Struts和Hibernate,管理了应用的生命周期和事务。此外,Spring还提供了数据访问抽象,如JDBC模板,使得数据库操作更简洁。通过配置文件(applicationContext.xml),Spring可以配置和管理所有bean的实例。 4. **学生信息管理功能**: - **用户登录与权限管理**:系统应包含用户登录验证机制,确保只有授权的人员能访问学生信息。同时,根据不同的角色(如管理员、教师、学生),提供不同的操作权限。 - **学生信息录入**:允许管理员或教师输入新学生的基本信息,如姓名、学号、性别、出生日期、专业等。 - **信息查询**:支持按多种条件搜索学生信息,如学号、姓名、班级等。 - **信息修改与删除**:允许对错误或过时的学生信息进行修改,必要时可以删除某个学生的信息。 - **成绩管理**:记录并管理学生的课程成绩,包括学期成绩、总评成绩等,支持批量导入和导出功能。 - **报表生成**:可以生成各类统计报表,如班级成绩排名、学生出勤率等,以辅助教学决策。 5. **系统架构与设计模式**: SSH系统通常采用三层架构:表现层(Struts)、业务逻辑层(Spring)和数据访问层(Hibernate)。此外,系统可能还应用了单例、工厂、代理等设计模式,以提高代码的可维护性和可扩展性。 6. **开发与部署**: 开发过程中,开发者需使用IDE(如Eclipse或IntelliJ IDEA)进行编码,使用Maven或Gradle管理依赖,使用Tomcat或Jetty等应用服务器运行和部署应用。同时,还需要进行单元测试、集成测试以及性能测试,确保系统的稳定性和效率。 通过SSH框架实现的学生信息管理系统,不仅提高了开发效率,也保证了代码的高质量和可维护性。它为教育机构提供了一个强大而灵活的工具,帮助他们更好地管理学生数据,提升教育管理效率。
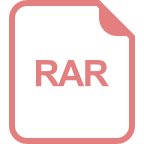
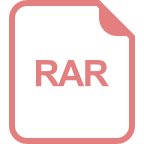
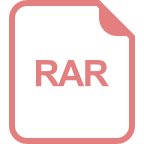
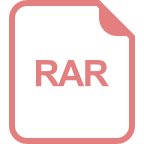
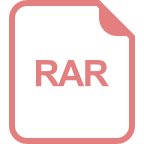
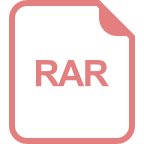
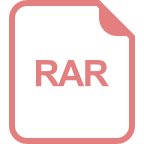
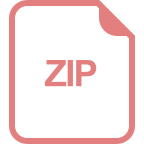
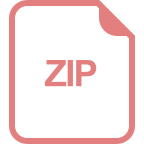
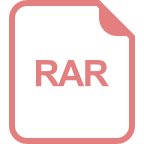
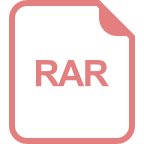
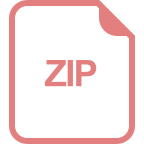
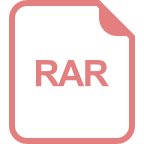
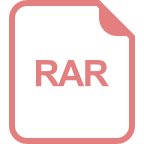
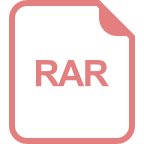

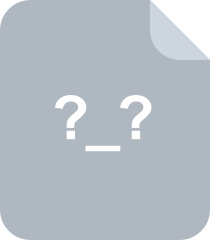
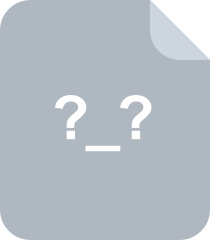
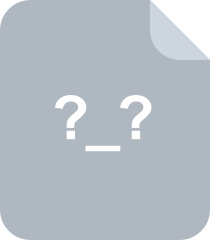
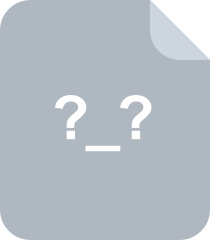
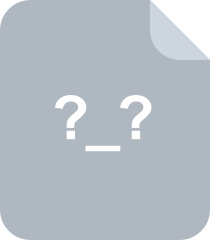
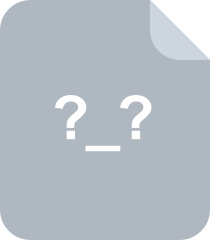
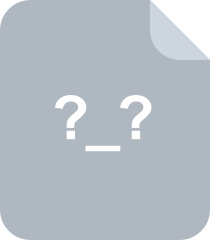
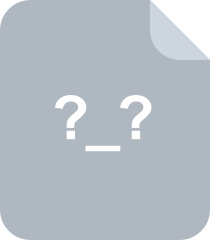
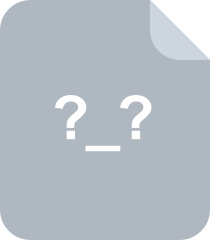
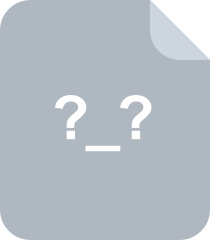
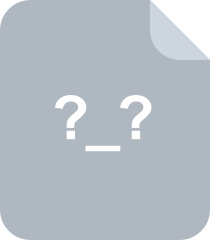
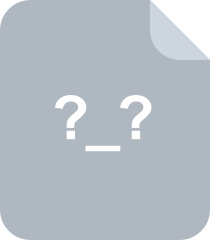
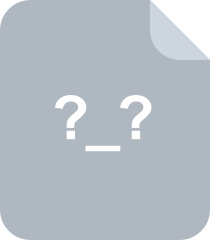
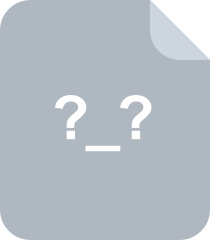
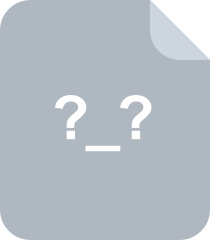
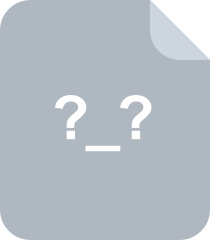
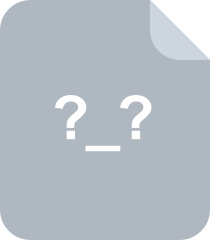
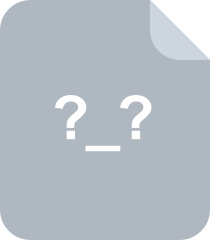
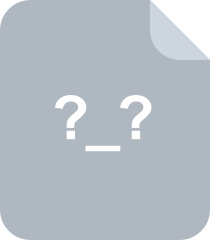
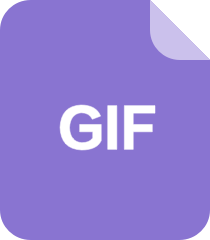
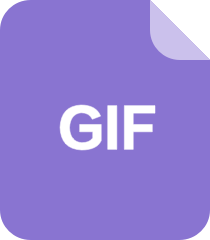
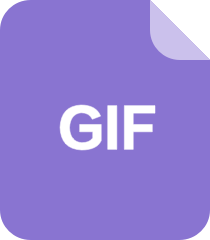
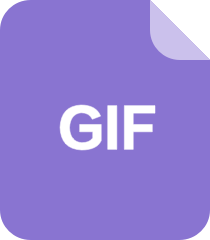
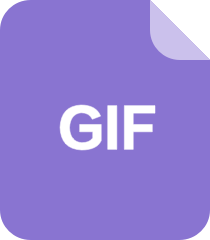
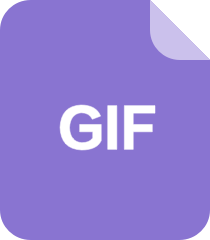
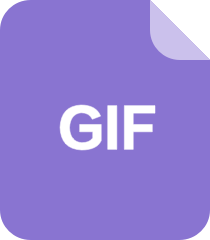
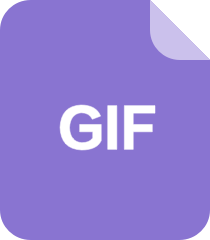
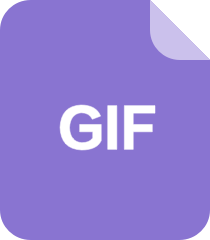
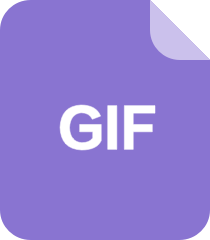
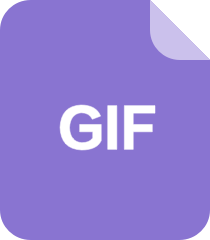
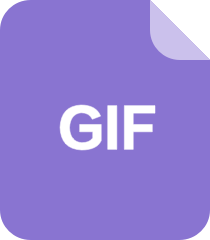
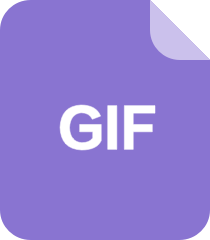
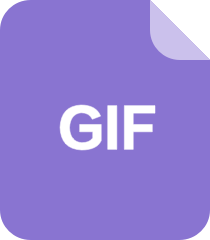
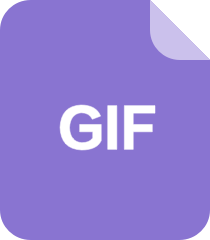
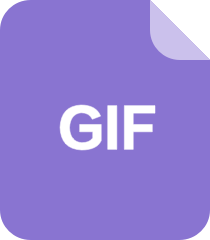
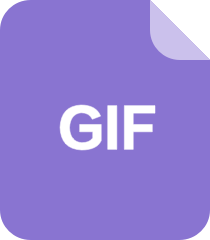
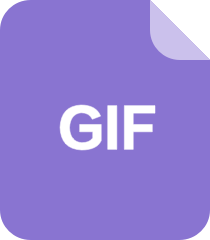
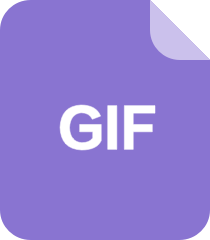
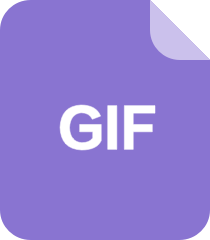
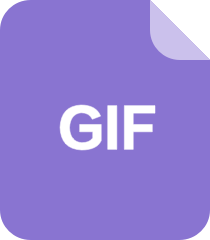
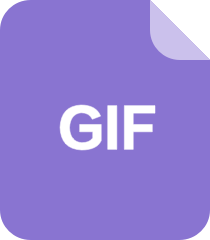
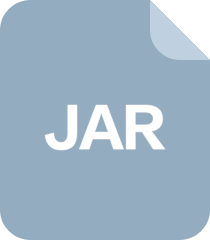
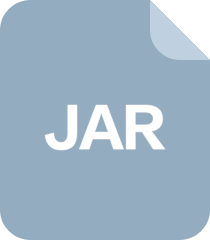
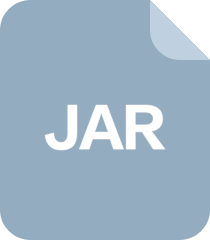
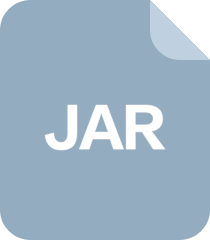
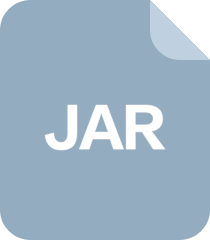
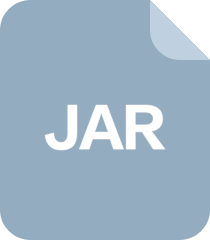
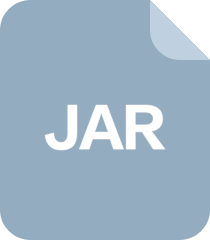
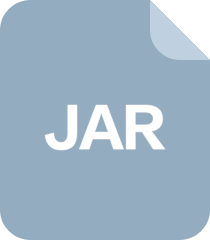
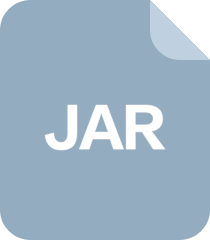
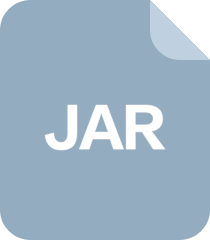
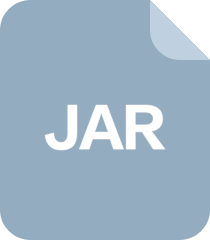
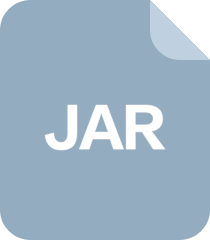
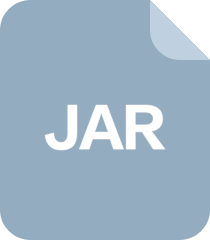
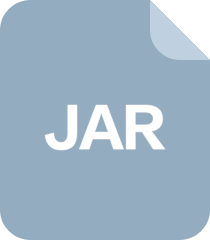
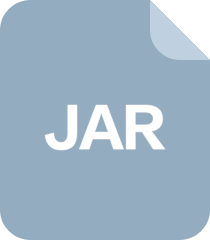
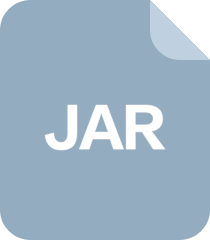
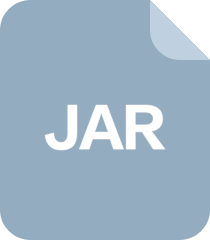
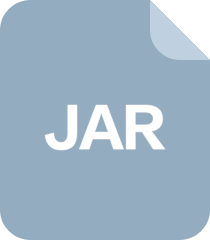
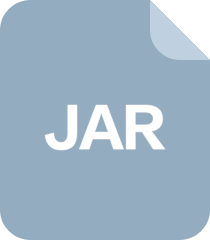
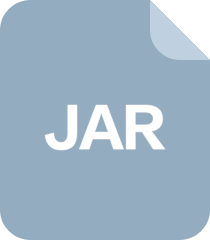
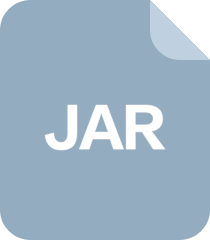
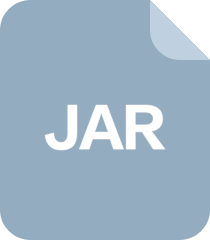
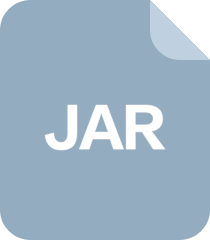
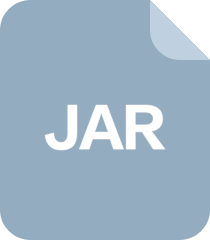
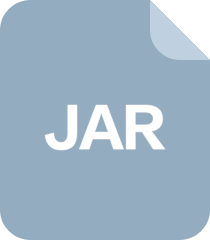
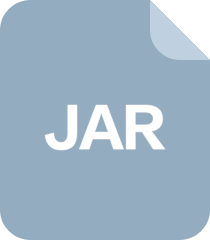
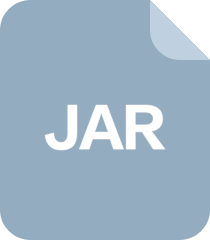
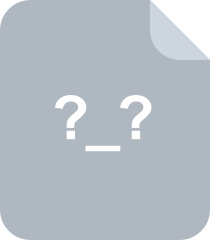
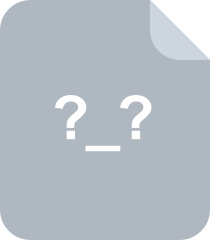
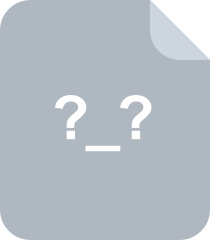
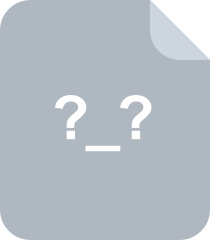
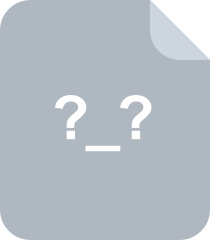
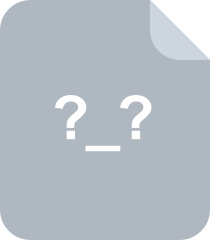
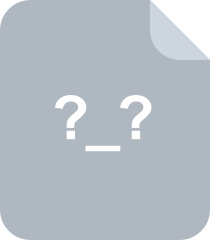
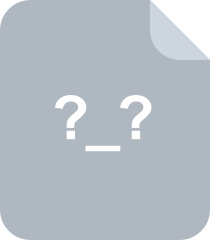
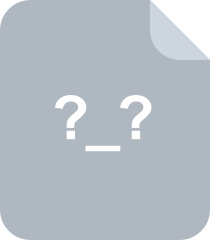
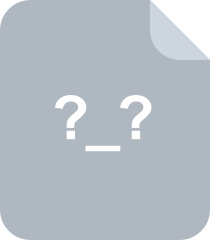
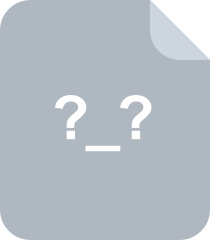
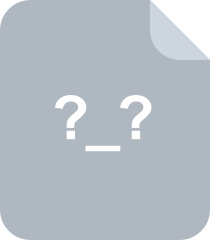
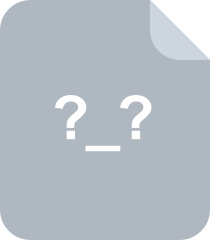
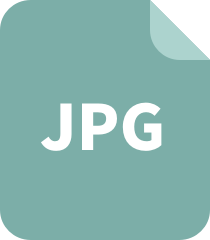
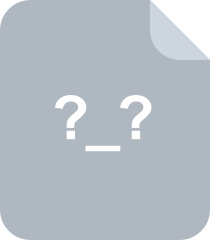
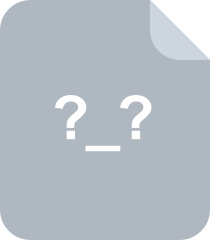
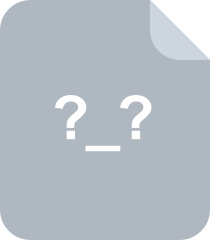
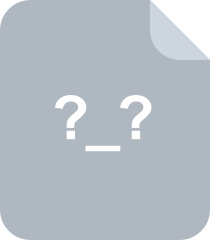
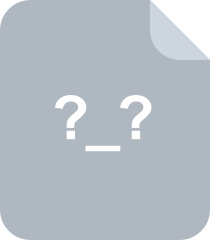
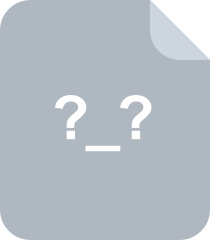
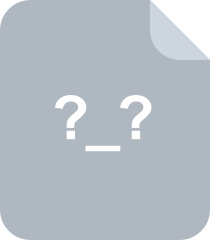
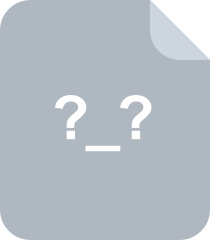
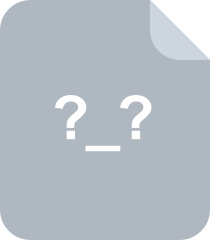
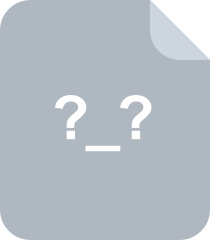
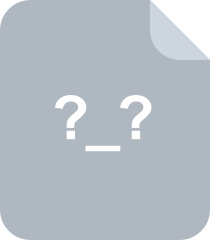
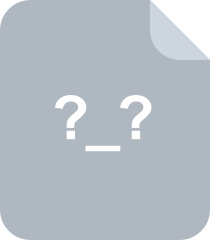
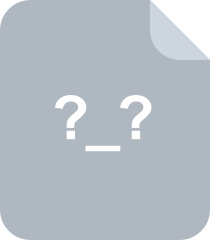
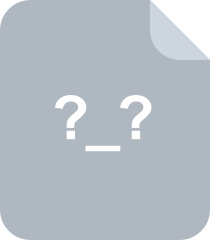
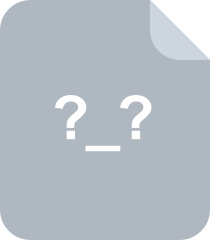
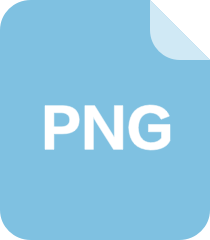
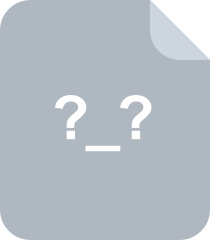
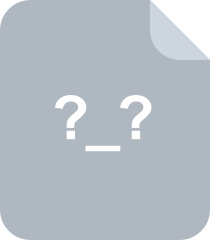
- 1
- 2
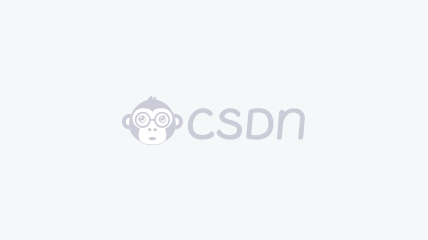
- 可乐奶昔2017-04-02兄弟 数据库呢

- 粉丝: 1
- 资源: 5
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

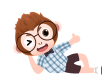
最新资源
- 基于Java开发的日程管理FlexTime应用设计源码
- SM2258XT-BGA144-4BGA180-6L-R1019 三星KLUCG4J1CB B0B1颗粒开盘工具 , EC, 3A, 94, 43, A4, CA 七彩虹SL300这个固件有用
- GJB 5236-2004 军用软件质量度量
- 30天开发操作系统 第 8 天 - 鼠标控制与切换32模式
- spice vd interface接口
- 安装Git时遇到找不到`/dev/null`的问题
- 标量(scalar)、向量(vector)、矩阵(matrix)、数组(array)等概念的深入理解与运用
- 数值计算复习内容,涵盖多种方法,内容为gpt生成
- 标量(scalar)、向量(vector)、矩阵(matrix)、数组(array)等概念的深入理解与运用
- 网络综合项目实验12.19

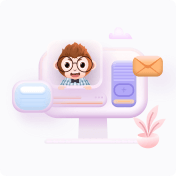
