package com.travel.controller;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.travel.bean.*;
import com.travel.bean.vo.CartVO;
import com.travel.bean.vo.EvaluateVO;
import com.travel.bean.vo.OrderVO;
import com.travel.bean.vo.WishVO;
import com.travel.service.*;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import javax.servlet.http.HttpSession;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Date;
import java.util.List;
import java.util.UUID;
@Controller
public class IndexController {
@Autowired
private ScenicSpotService scenicSpotService;
@Autowired
private WishService wishService;
@Autowired
private UserService userService;
@Autowired
private CartService cartService;
@Autowired
private OrderService orderService;
@Autowired
private EvaluateService evaluateService;
@GetMapping("/")
public String index(Model model) {
List<ScenicSpot> scenicSpotList = scenicSpotService.list();
model.addAttribute("index_list", scenicSpotList);
return "index/index";
}
@GetMapping("/see/{id}")
public String see(@PathVariable Long id, Model model) {
ScenicSpot one = scenicSpotService.getById(id);
model.addAttribute("one", one);
QueryWrapper<Evaluate> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("spot_id", id);
List<Evaluate> list = evaluateService.list(queryWrapper);
List<EvaluateVO> evaluateVOList = new ArrayList<>();
for (Evaluate evaluate : list) {
EvaluateVO evaluateVO = new EvaluateVO();
User user = userService.getById(evaluate.getUserId());
if (user != null) {
BeanUtils.copyProperties(user, evaluateVO);
}
ScenicSpot scenicSpot = scenicSpotService.getById(evaluate.getSpotId());
if (scenicSpot != null) {
BeanUtils.copyProperties(scenicSpot, evaluateVO);
}
BeanUtils.copyProperties(evaluate, evaluateVO);
evaluateVOList.add(evaluateVO);
}
model.addAttribute("list", evaluateVOList);
return "index/blog-details-fullwidth";
}
@GetMapping("/index/wish/list")
public String wishList(Model model, HttpSession session) {
User currentUser = (User) session.getAttribute("currentUser");
if (currentUser == null) {
return "redirect:/index/toLogin";
} else {
QueryWrapper<Wish> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("user_id", currentUser.getId());
List<Wish> list = wishService.list(queryWrapper);
List<WishVO> wishVOList = new ArrayList<>();
for (Wish wish : list) {
WishVO wishVO = new WishVO();
User user = userService.getById(wish.getUserId());
if (user != null) {
BeanUtils.copyProperties(user, wishVO);
}
ScenicSpot scenicSpot = scenicSpotService.getById(wish.getSpotId());
if (scenicSpot != null) {
BeanUtils.copyProperties(scenicSpot, wishVO);
}
BeanUtils.copyProperties(wish, wishVO);
wishVOList.add(wishVO);
}
model.addAttribute("list", wishVOList);
return "index/wishlist";
}
}
@GetMapping("/wish/add/{id}")
public String wishAdd(@PathVariable Long id, HttpSession session) {
User currentUser = (User) session.getAttribute("currentUser");
if (currentUser == null) {
return "redirect:/index/toLogin";
} else {
Wish wish = new Wish();
wish.setUserId(currentUser.getId());
wish.setSpotId(id);
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date date = new Date();
String time = dateFormat.format(date);
wish.setCreateTime(time);
boolean save = wishService.save(wish);
return "redirect:/index/wish/list";
}
}
@GetMapping("/index/wish/delete/{id}")
public String wishDelete(@PathVariable Long id) {
boolean b = wishService.removeById(id);
return "redirect:/index/wish/list";
}
@GetMapping("/index/cart/list")
public String cartList(Model model, HttpSession session) {
User currentUser = (User) session.getAttribute("currentUser");
if (currentUser == null) {
return "redirect:/index/toLogin";
} else {
QueryWrapper<Cart> queryWrapper = new QueryWrapper<>();
queryWrapper.eq("user_id", currentUser.getId());
List<Cart> list = cartService.list(queryWrapper);
List<CartVO> cartVOList = new ArrayList<>();
for (Cart cart : list) {
CartVO cartVO = new CartVO();
User user = userService.getById(cart.getUserId());
if (user != null) {
BeanUtils.copyProperties(user, cartVO);
}
ScenicSpot scenicSpot = scenicSpotService.getById(cart.getSpotId());
if (scenicSpot != null) {
BeanUtils.copyProperties(scenicSpot, cartVO);
}
BeanUtils.copyProperties(cart, cartVO);
cartVOList.add(cartVO);
}
model.addAttribute("list", cartVOList);
return "index/cart";
}
}
@GetMapping("/cart/add/{id}")
public String cartAdd(@PathVariable Long id, HttpSession session) {
User currentUser = (User) session.getAttribute("currentUser");
if (currentUser == null) {
return "redirect:/index/toLogin";
} else {
Cart cart = new Cart();
cart.setUserId(currentUser.getId());
cart.setSpotId(id);
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date date = new Date();
String time = dateFormat.format(date);
cart.setCreateTime(time);
boolean save = cartService.save(cart);
return "redirect:/index/cart/list";
}
}
@GetMapping("/cart/add2/{id}")
public String cartAdd2(@PathVariable Long id, HttpSession session) {
User currentUser = (User) session.getAttribute("currentUser");
if (currentUser == null) {
return "redirect:/index/toLogin";
} else {
Cart cart = new Cart();
cart.setUserId(currentUser.getId());
Wish wish = wishService.getById(id);
cart.setSpotId(wish.getSpotId());
SimpleDateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
Date date = new Date();
String time = dateFormat.format(date);
cart.setCreateTime(time);
boolean save = cartService.save(cart);
if (save) {
boolean b = wishService.removeById(id);
}
return "redirect:/index/cart/list";
}
}
@GetMapping("/index/order/list")
public String orderList(Model model, HttpSession session) {
User currentUser = (User) session.getAttribute("currentUser");
if (currentUser == null) {
return "redirect:/index/toLogin";
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
功能丰富的旅游景点管理系统包括以下几个主要功能: 1. 景点浏览:用户可以浏览系统中提供的各个旅游景点的相关信息,包括景点介绍、图片、位置等。用户可以通过搜索功能或分类导航快速找到感兴趣的景点。 2. 收藏景点:用户可以将自己喜爱的景点添加到收藏夹中,方便日后查看和管理。用户可以创建多个收藏夹,并根据个人喜好对景点进行分类。 3. 加购功能:对于一些需要预订门票或购买旅游产品的景点,用户可以将其加入购物车进行集中管理。用户可以选择不同的日期、票种和数量等细节,并实时显示价格计算。 4. 提交订单:用户可以通过系统提交自己的预订订单,包括所选景点、日期、人数、联系信息等。系统会进行订单确认,并生成订单号和支付信息。用户可以选择不同的支付方式完成订单支付。 5. 后台管理:管理员可以对景点信息、订单信息、用户信息等进行管理和维护。管理员拥有对系统的权限控制,可以添加、编辑和删除景点信息,审核订单和用户注册等。 6. 用户管理:系统提供用户注册和登录功能,用户可以创建个人账号,并管理个人信息和订单记录。用户可以查看自己的收藏景点、历史订单等,并进行评价和反馈。 7. 评论和评价
资源推荐
资源详情
资源评论
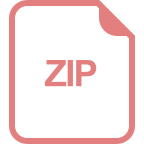
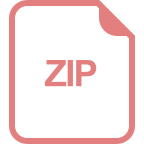
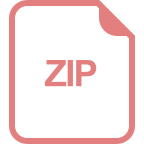
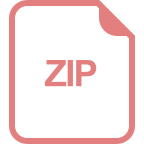
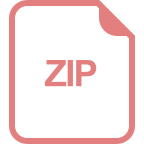
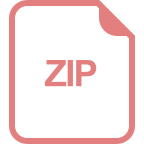
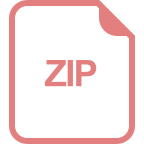
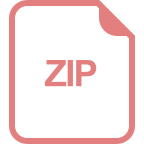
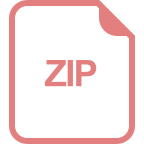
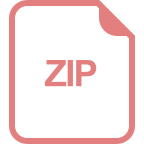
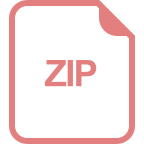
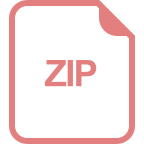
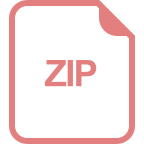
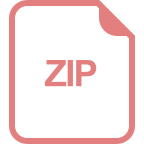
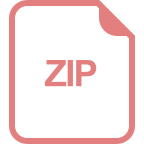
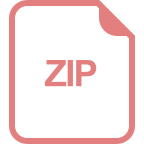
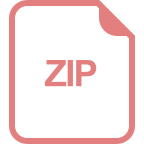
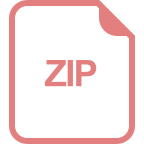
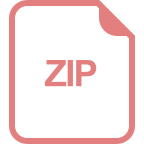
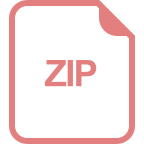
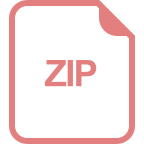
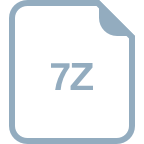
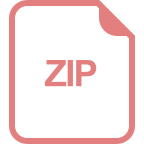
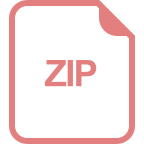
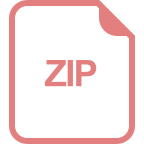
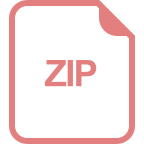
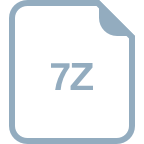
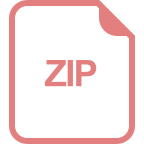
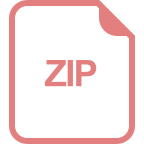
收起资源包目录

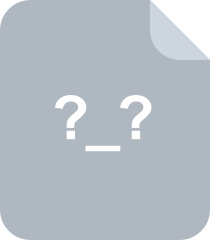
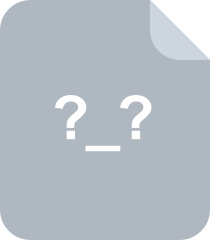
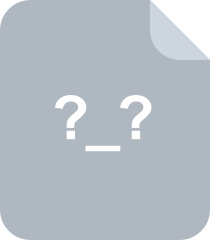
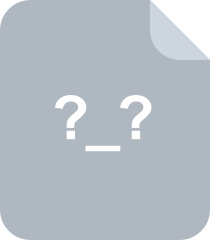
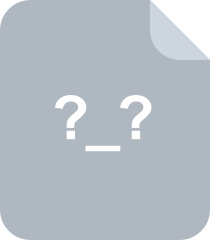
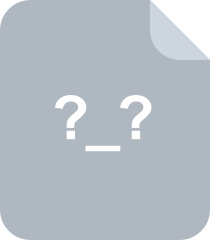
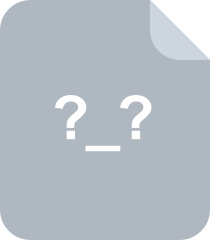
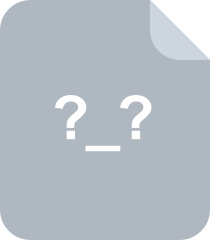
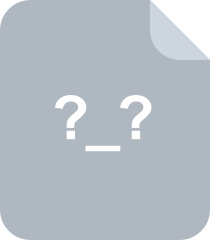
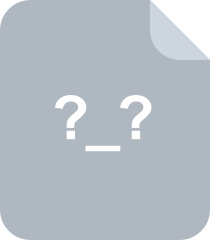
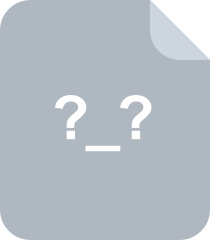
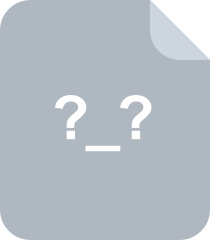
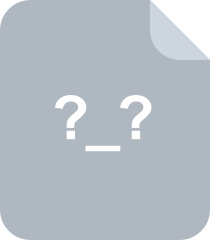
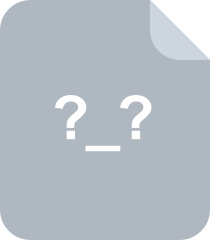
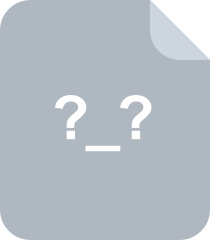
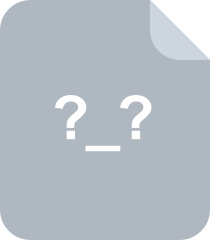
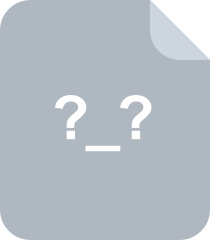
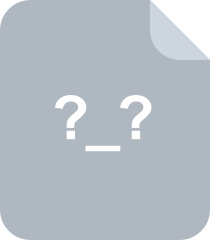
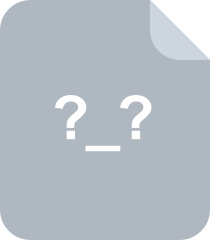
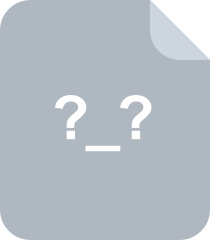
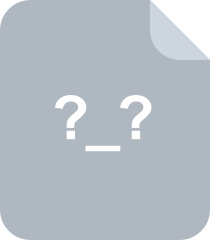
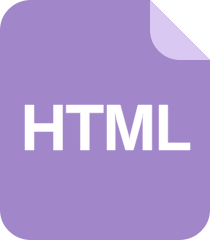
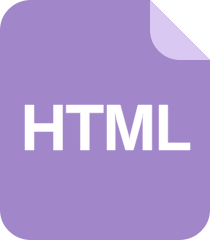
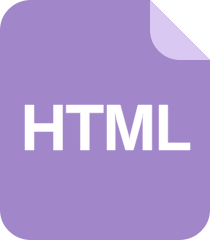
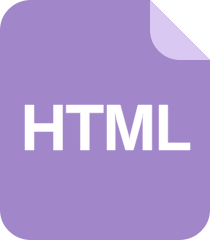
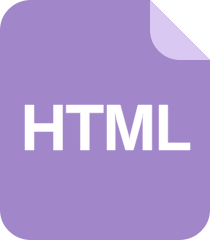
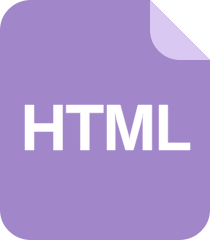
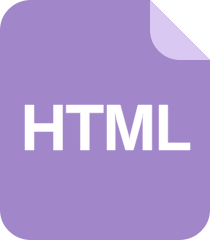
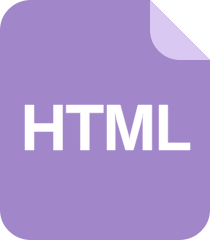
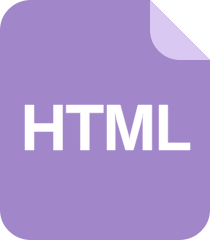
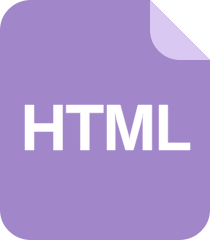
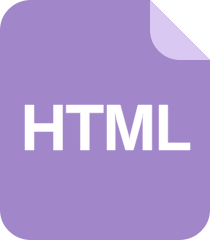
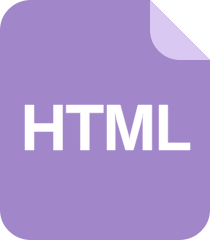
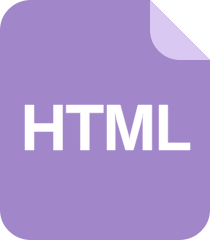
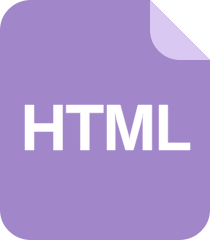
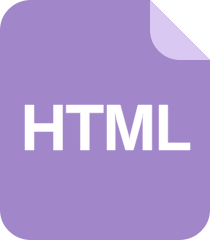
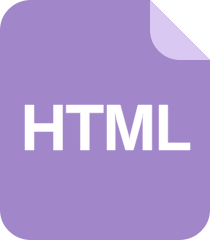
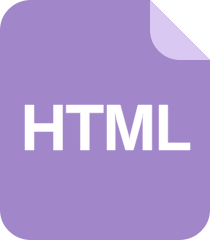
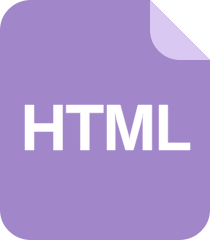
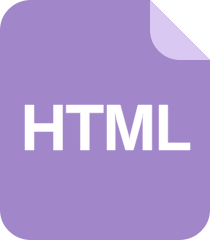
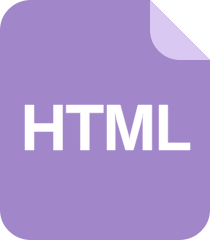
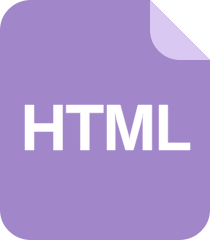
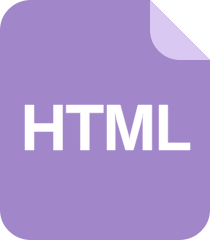
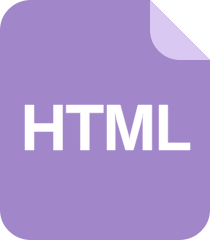
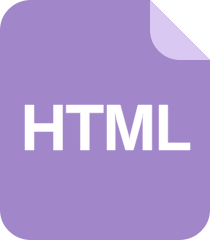
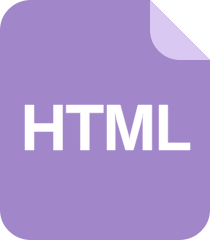
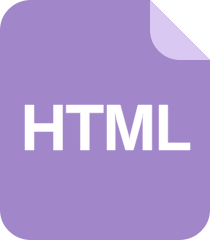
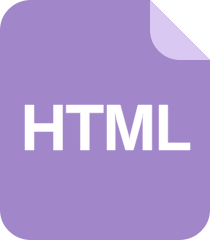
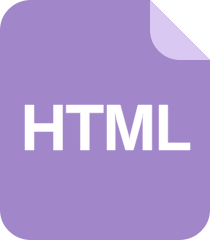
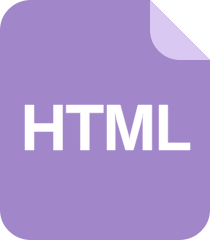
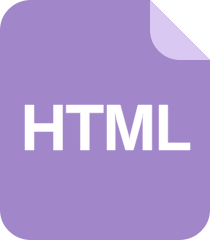
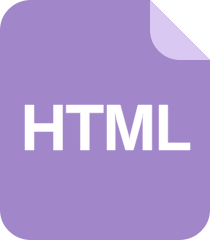
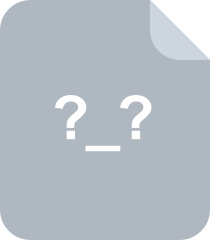
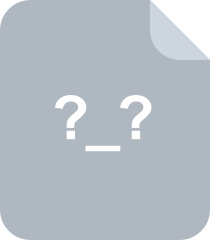
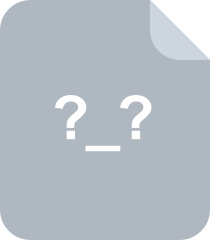
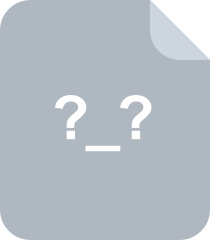
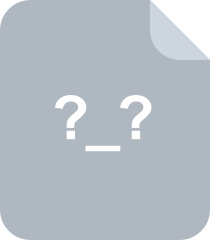
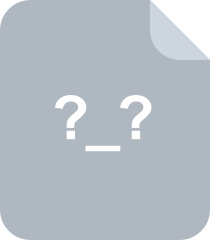
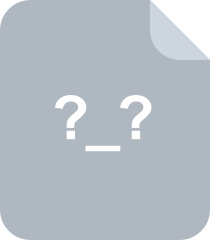
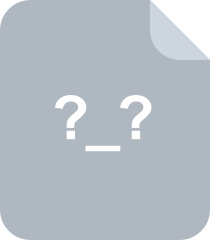
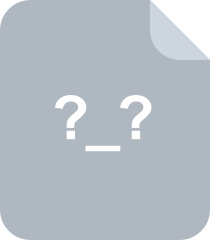
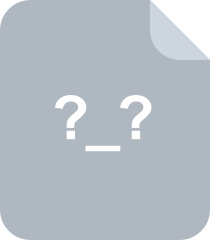
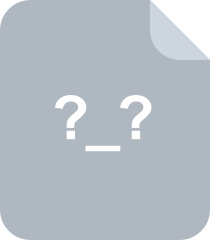
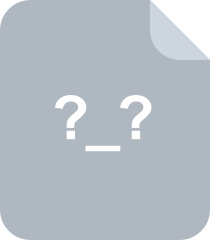
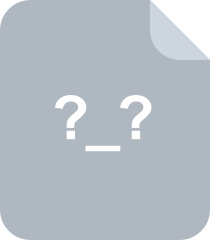
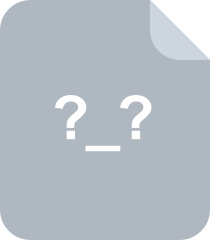
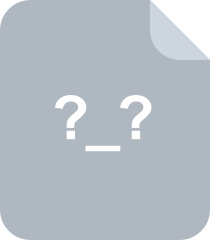
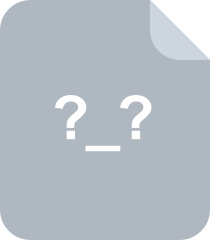
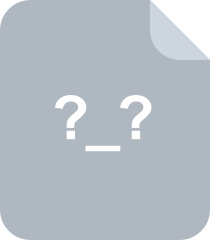
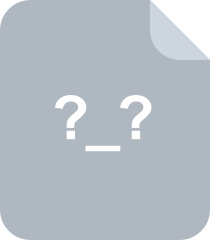
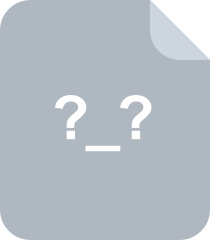
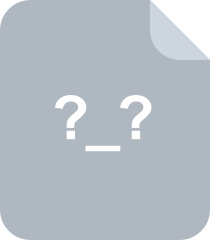
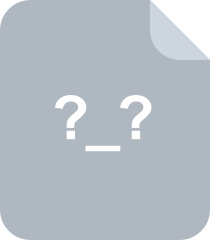
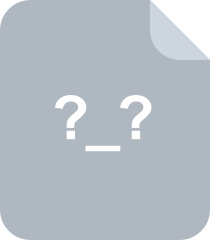
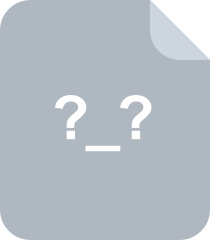
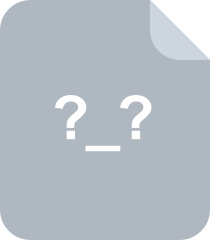
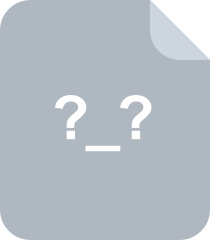
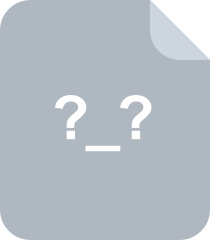
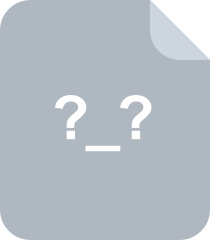
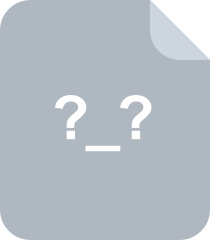
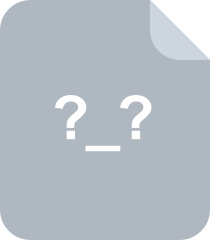
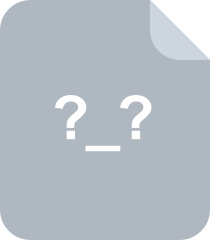
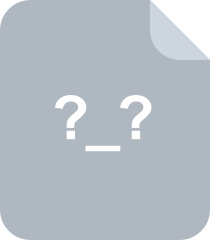
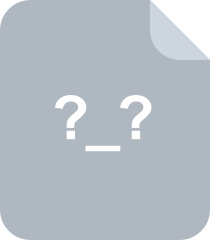
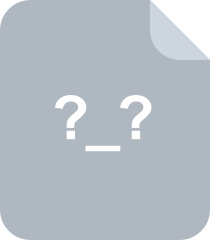
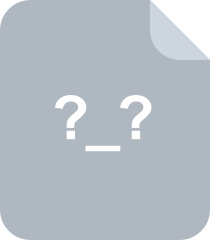
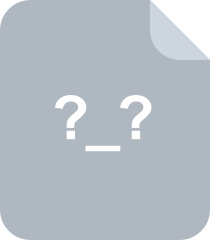
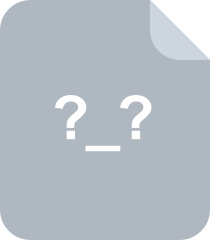
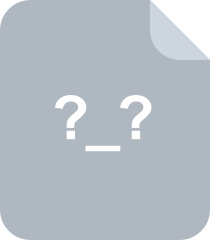
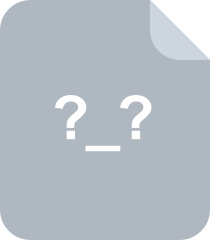
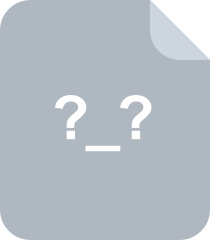
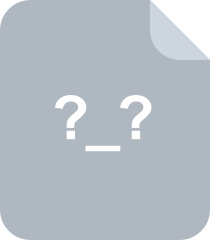
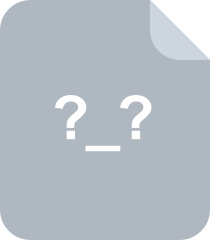
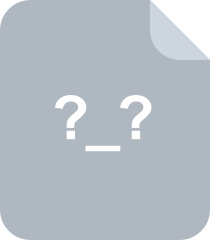
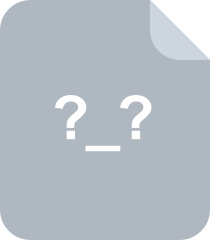
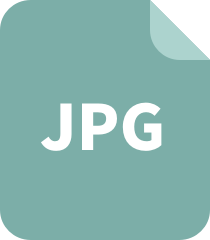
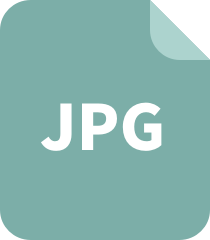
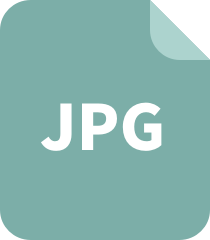
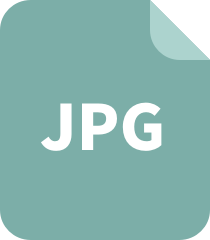
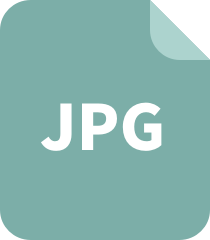
共 223 条
- 1
- 2
- 3
资源评论
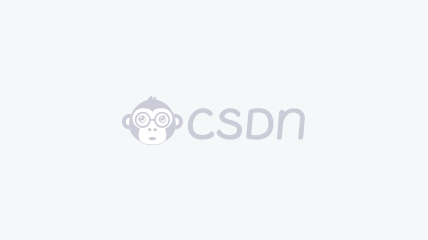

竹山全栈
- 粉丝: 2369
- 资源: 261
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

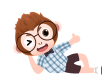
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


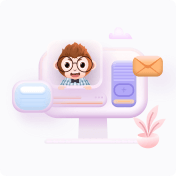
安全验证
文档复制为VIP权益,开通VIP直接复制
