# Cropper
> A simple jQuery image cropping plugin.
- [Website](https://fengyuanchen.github.io/cropper)
- [Cropper without jQuery](https://github.com/fengyuanchen/cropperjs)
[](https://travis-ci.org/fengyuanchen/cropper)
## Table of contents
- [Features](#features)
- [Main](#main)
- [Getting started](#getting-started)
- [Options](#options)
- [Methods](#methods)
- [Events](#events)
- [No conflict](#no-conflict)
- [Browser support](#browser-support)
- [Contributing](#contributing)
- [Versioning](#versioning)
- [License](#license)
## Features
- Supports 39 [options](#options)
- Supports 27 [methods](#methods)
- Supports 7 [events](#events)
- Supports touch (mobile)
- Supports zooming
- Supports rotating
- Supports scaling (flipping)
- Supports multiple croppers
- Supports to crop on a canvas
- Supports to crop image in the browser-side by canvas
- Supports to translate Exif Orientation information
- Cross-browser support
## Main
```
dist/
├── cropper.css ( 5 KB)
├── cropper.min.css ( 4 KB)
├── cropper.js (78 KB)
└── cropper.min.js (28 KB)
```
## Getting started
### Quick start
Four quick start options are available:
- [Download the latest release](https://github.com/fengyuanchen/cropper/archive/master.zip).
- Clone the repository: `git clone https://github.com/fengyuanchen/cropper.git`.
- Install with [NPM](https://npmjs.com): `npm install cropper`.
- Install with [Bower](https://bower.io): `bower install cropper`.
### Installation
Include files:
```html
<script src="/path/to/jquery.js"></script><!-- jQuery is required -->
<link href="/path/to/cropper.css" rel="stylesheet">
<script src="/path/to/cropper.js"></script>
```
The [cdnjs](https://github.com/cdnjs/cdnjs) provides CDN support for Cropper's CSS and JavaScript. You can find the links [here](https://cdnjs.com/libraries/cropper).
### Usage
Initialize with `$.fn.cropper` method.
```html
<!-- Wrap the image or canvas element with a block element (container) -->
<div>
<img id="image" src="picture.jpg">
</div>
```
```css
/* Limit image width to avoid overflow the container */
img {
max-width: 100%; /* This rule is very important, please do not ignore this! */
}
```
```js
$('#image').cropper({
aspectRatio: 16 / 9,
crop: function(e) {
// Output the result data for cropping image.
console.log(e.x);
console.log(e.y);
console.log(e.width);
console.log(e.height);
console.log(e.rotate);
console.log(e.scaleX);
console.log(e.scaleY);
}
});
```
#### FAQ
##### How to crop a new area after zoom in or zoom out?
> Just double click your mouse to enter crop mode.
##### How to move the image after crop an area?
> Just double click your mouse to enter move mode.
##### How to fix aspect ratio in free ratio mode?
> Just hold the `shift` key when you resize the crop box.
##### How to crop a square area in free ratio mode?
> Just hold the `shift` key when you crop on the image.
#### Notes
- The size of the cropper inherits from the size of the image's parent element (wrapper), so be sure to wrap the image with a **visible block element**.
> If you are using cropper in a modal, you should initialize the cropper after the modal shown completely. Otherwise, you will not get a correct cropper.
- The outputted cropped data bases on the original image size, so you can use them to crop the image directly.
- If you try to start cropper on a cross-origin image, please make sure that your browser supports HTML5 [CORS settings attributes](https://developer.mozilla.org/en-US/docs/Web/HTML/CORS_settings_attributes), and your image server supports the `Access-Control-Allow-Origin` option (see the [HTTP access control (CORS)](https://developer.mozilla.org/en-US/docs/Web/HTTP/Access_control_CORS)).
#### Known issues
- [Known iOS resource limits](https://developer.apple.com/library/mac/documentation/AppleApplications/Reference/SafariWebContent/CreatingContentforSafarioniPhone/CreatingContentforSafarioniPhone.html): As iOS devices limit memory, the browser may crash when you are cropping a large image (iPhone camera resolution). To avoid this, you may resize the image first (below 1024px) before start a cropper.
- Known image size increase: When export the cropped image on browser-side with the `HTMLCanvasElement.toDataURL` method, the size of the exported image may be greater than the original image's. This is because the type of the exported image is not the same as the original image's. So just pass the type the original image's as the first parameter to `toDataURL` to fix this. For example, if the original type is JPEG, then use `$().cropper('getCroppedCanvas').toDataURL('image/jpeg')` to export image.
[⬆ back to top](#table-of-contents)
## Options
You may set cropper options with `$().cropper(options)`.
If you want to change the global default options, You may use `$.fn.cropper.setDefaults(options)`.
### viewMode
- Type: `Number`
- Default: `0`
- Options:
- `0`: the crop box is just within the container
- `1`: the crop box should be within the canvas
- `2`: the canvas should not be within the container
- `3`: the container should be within the canvas
Define the view mode of the cropper.
### dragMode
- Type: `String`
- Default: `'crop'`
- Options:
- `'crop'`: create a new crop box
- `'move'`: move the canvas
- `'none'`: do nothing
Define the dragging mode of the cropper.
### aspectRatio
- Type: `Number`
- Default: `NaN`
Set the aspect ratio of the crop box. By default, the crop box is free ratio.
### data
- Type: `Object`
- Default: `null`
The previous cropped data if you had stored, will be passed to `setData` method automatically.
### preview
- Type: `String` (**jQuery selector**)
- Default: `''`
Add extra elements (containers) for previewing.
**Notes:**
- The maximum width is the initial width of preview container.
- The maximum height is the initial height of preview container.
- If you set an `aspectRatio` option, be sure to set the preview container with the same aspect ratio.
- If preview is not getting properly displayed, set `overflow:hidden` to the preview container.
### responsive
- Type: `Boolean`
- Default: `true`
Re-render the cropper when resize the window.
### restore
- Type: `Boolean`
- Default: `true`
Restore the cropped area after resize the window.
### checkCrossOrigin
- Type: `Boolean`
- Default: `true`
Check if the current image is a cross-origin image.
If it is, when clone the image, a `crossOrigin` attribute will be added to the cloned image element and a timestamp will be added to the `src` attribute to reload the source image to avoid browser cache error.
By adding `crossOrigin` attribute to image will stop adding timestamp to image url, and stop reload of image.
### checkOrientation
- Type: `Boolean`
- Default: `true`
Check the current image's Exif Orientation information.
More exactly, read the Orientation value for rotating or flipping the image, and then override the Orientation value with `1` (the default value) to avoid some issues (#120, #509) on iOS devices.
Requires to set both the `rotatable` and `scalable` options to `true` at the same time.
**Note:** Don't trust this all the time as some JPG images have incorrect (not standard) Orientation values.
> Requires [Typed Arrays](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/TypedArray) support ([IE 10+](http://caniuse.com/typedarrays)).
### modal
- Type: `Boolean`
- Default: `true`
Show the black modal above the image and under the crop box.
### guides
- Type: `Boolean`
- Default: `true`
Show the dashed lines above the crop box.
### center
- Type: `Boolean`
- Default: `true`
Show the center indicator above the crop box.
### highlight
- Type: `Boolean`
- Default: `true`
Show the white modal above the crop box (highlight the crop box).
### b
没有合适的资源?快使用搜索试试~ 我知道了~
java毕业设计 基于springCloud+Vue微服务架构前后端分离的B2C电子商务平台源码+详细文档+全部资料(优秀项目)
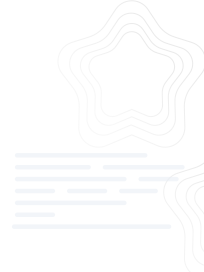
共2003个文件
js:647个
html:574个
txt:423个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 183 浏览量
2024-04-16
14:07:47
上传
评论 2
收藏 35.86MB ZIP 举报
温馨提示
【资源说明】 java毕业设计 基于springCloud+Vue微服务架构前后端分离的B2C电子商务平台源码+详细文档+全部资料(优秀项目)java毕业设计 基于springCloud+Vue微服务架构前后端分离的B2C电子商务平台源码+详细文档+全部资料(优秀项目) 【备注】 1、该资源内项目代码都经过测试运行成功,功能ok的情况下才上传的,请放心下载使用! 2、本项目适合计算机相关专业(如软件工程、计科、人工智能、通信工程、自动化、电子信息等)的在校学生、老师或者企业员工下载使用,也可作为毕设项目、课程设计、作业、项目初期立项演示等,当然也适合小白学习进阶。 3、如果基础还行,可以在此代码基础上进行修改,以实现其他功能,也可直接用于毕设、课设、作业等。 欢迎下载,沟通交流,互相学习,共同进步!
资源推荐
资源详情
资源评论
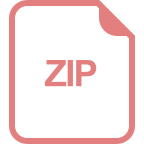
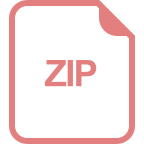
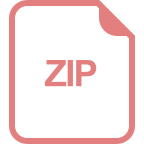
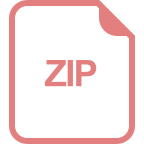
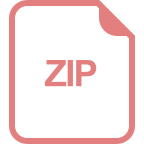
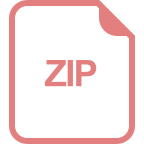
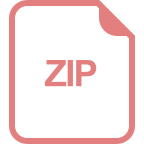
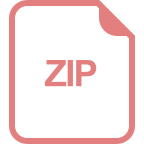
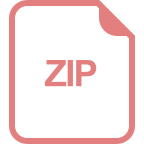
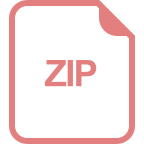
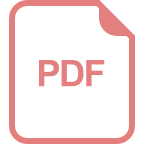
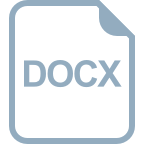
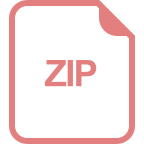
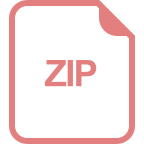
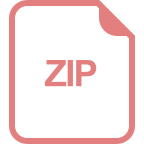
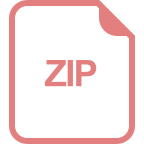
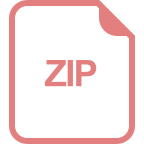
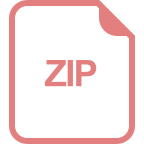
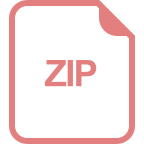
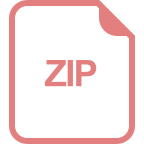
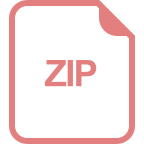
收起资源包目录

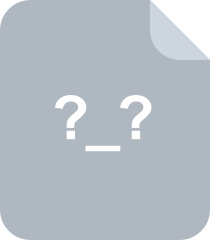
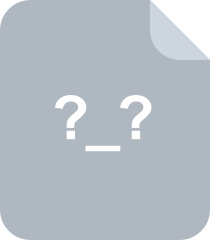
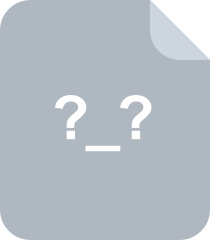
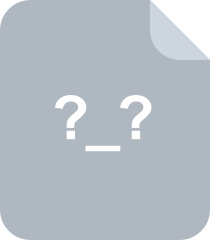
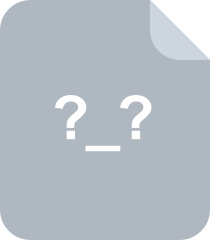
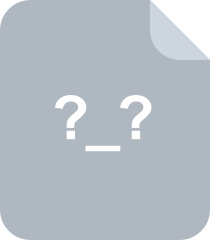
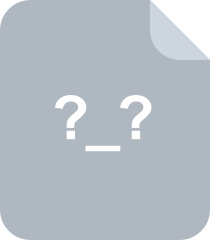
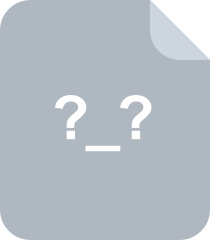
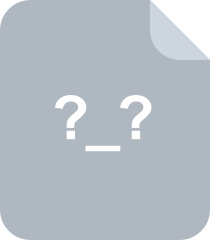
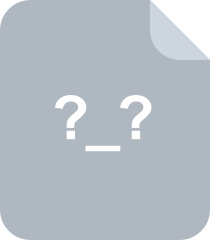
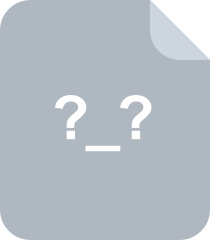
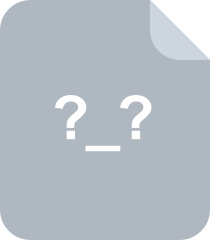
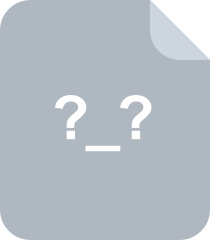
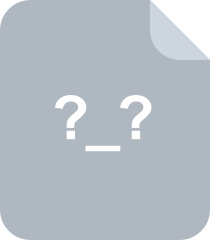
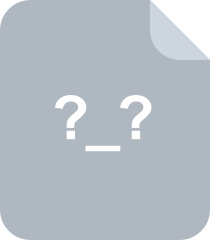
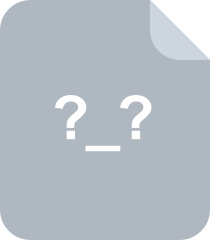
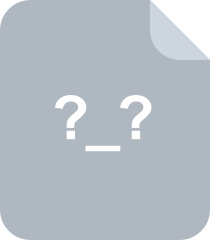
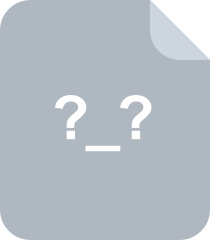
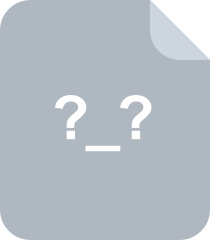
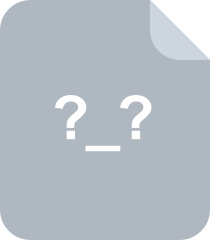
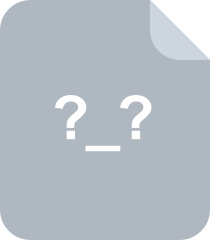
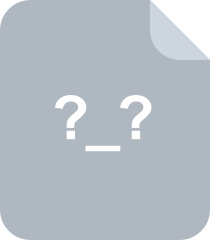
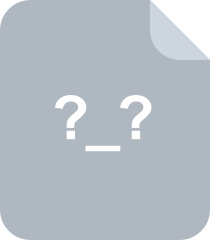
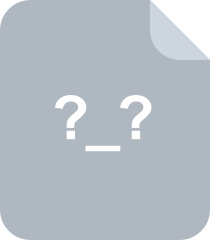
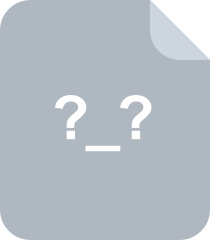
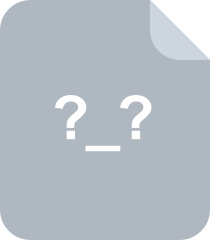
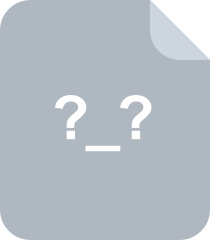
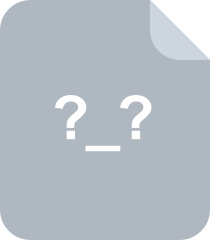
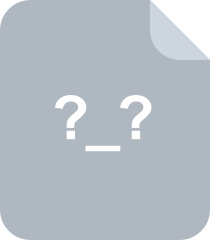
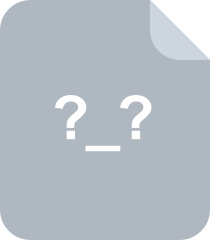
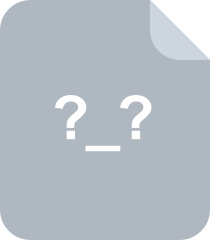
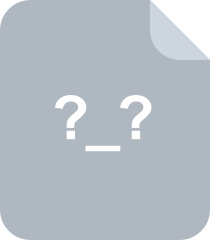
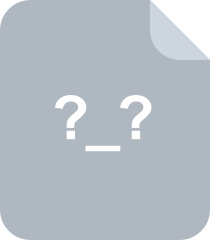
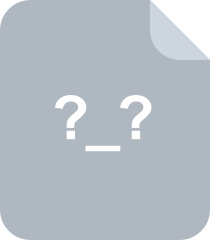
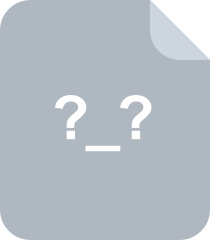
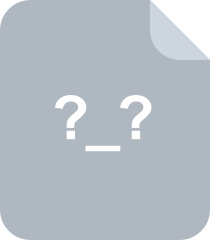
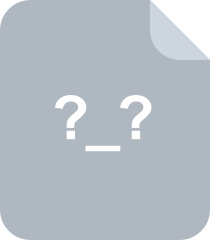
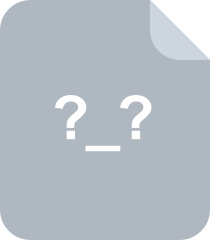
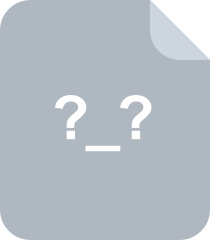
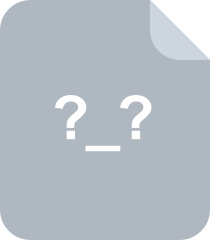
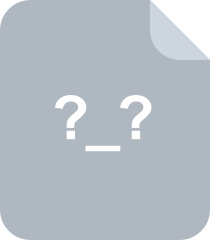
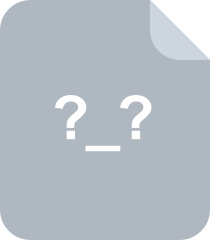
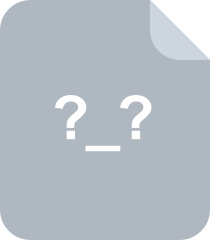
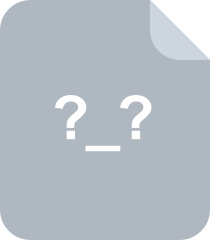
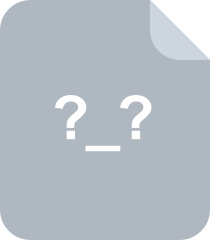
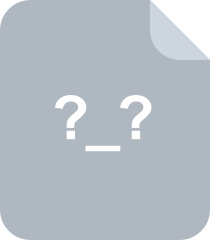
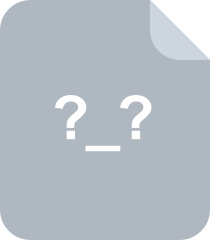
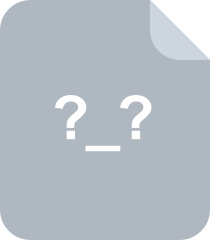
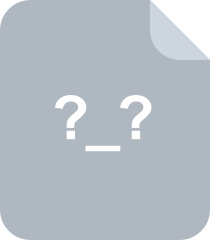
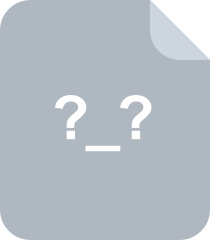
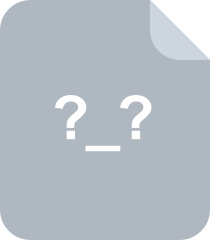
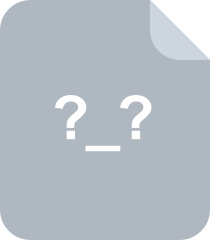
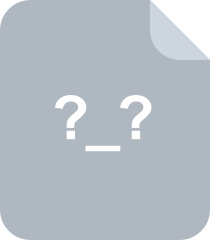
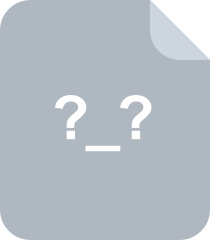
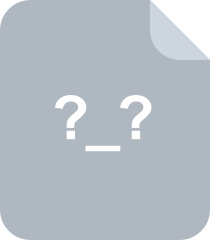
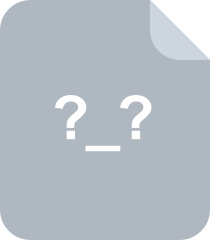
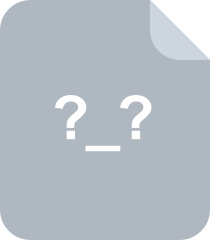
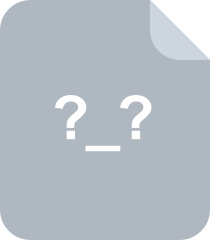
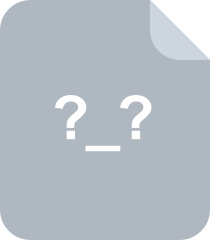
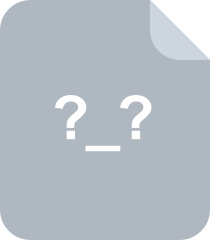
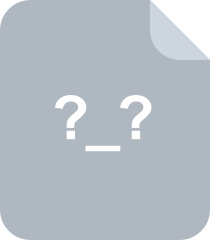
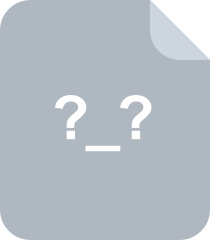
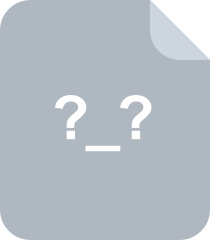
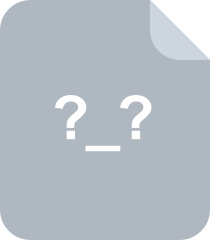
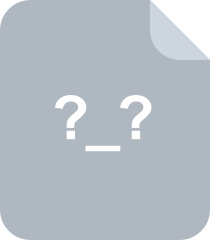
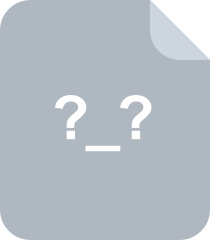
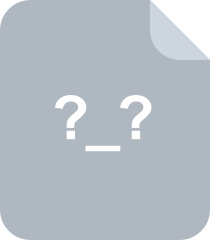
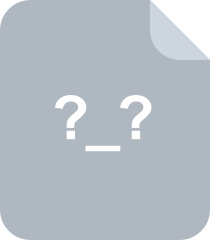
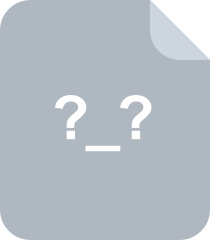
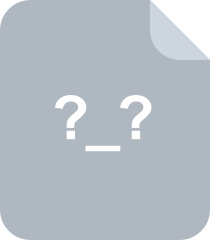
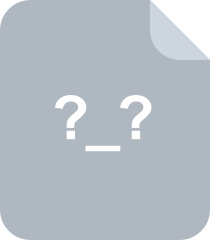
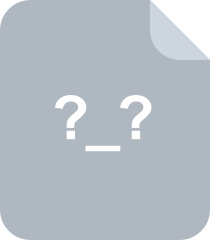
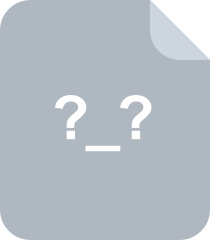
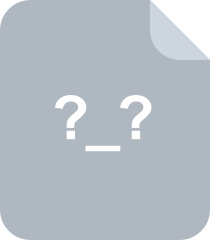
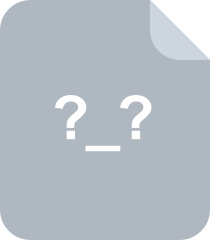
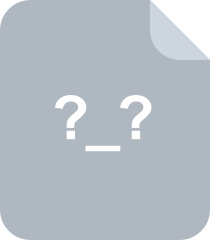
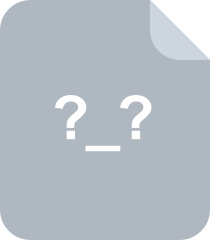
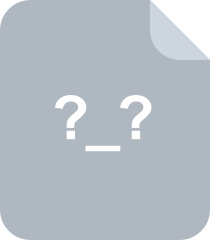
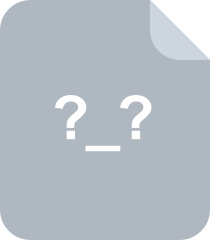
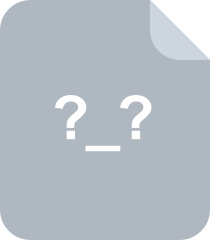
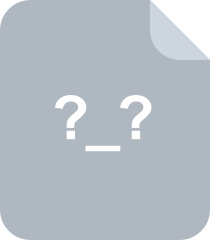
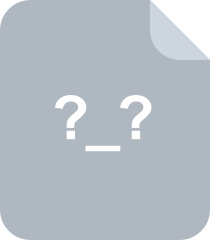
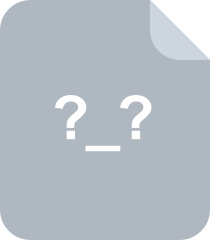
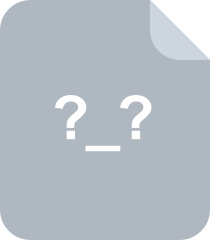
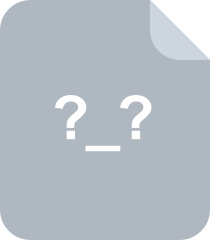
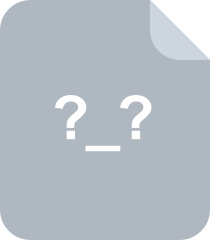
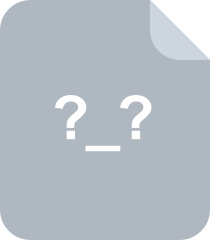
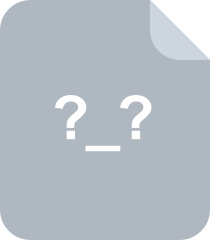
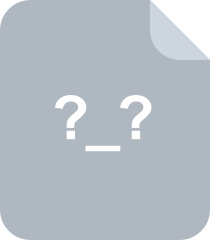
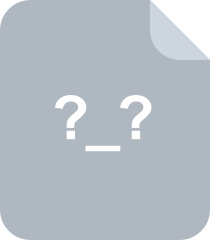
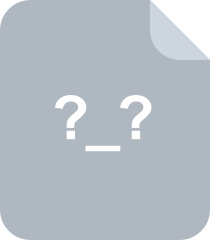
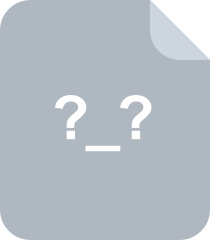
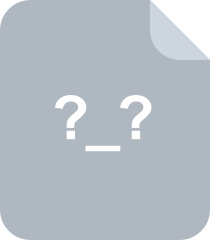
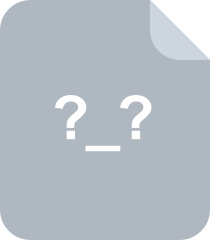
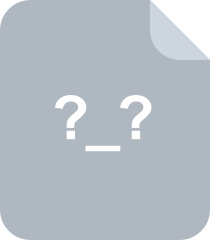
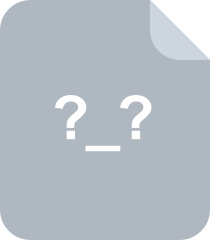
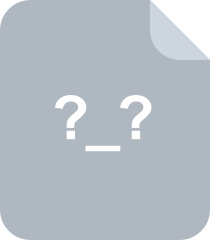
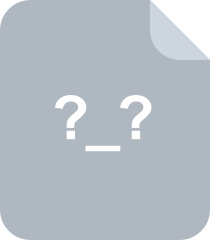
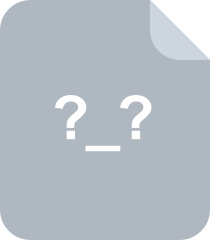
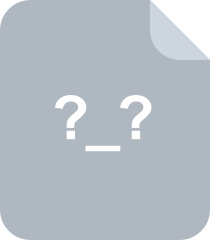
共 2003 条
- 1
- 2
- 3
- 4
- 5
- 6
- 21
资源评论
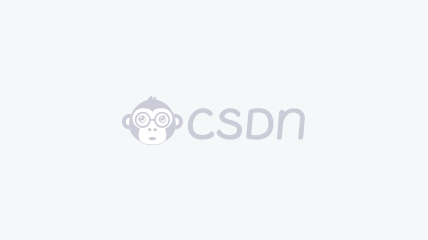

不走小道
- 粉丝: 3338
- 资源: 5059
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

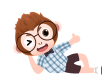
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


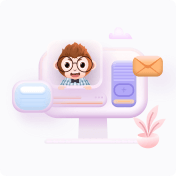
安全验证
文档复制为VIP权益,开通VIP直接复制
