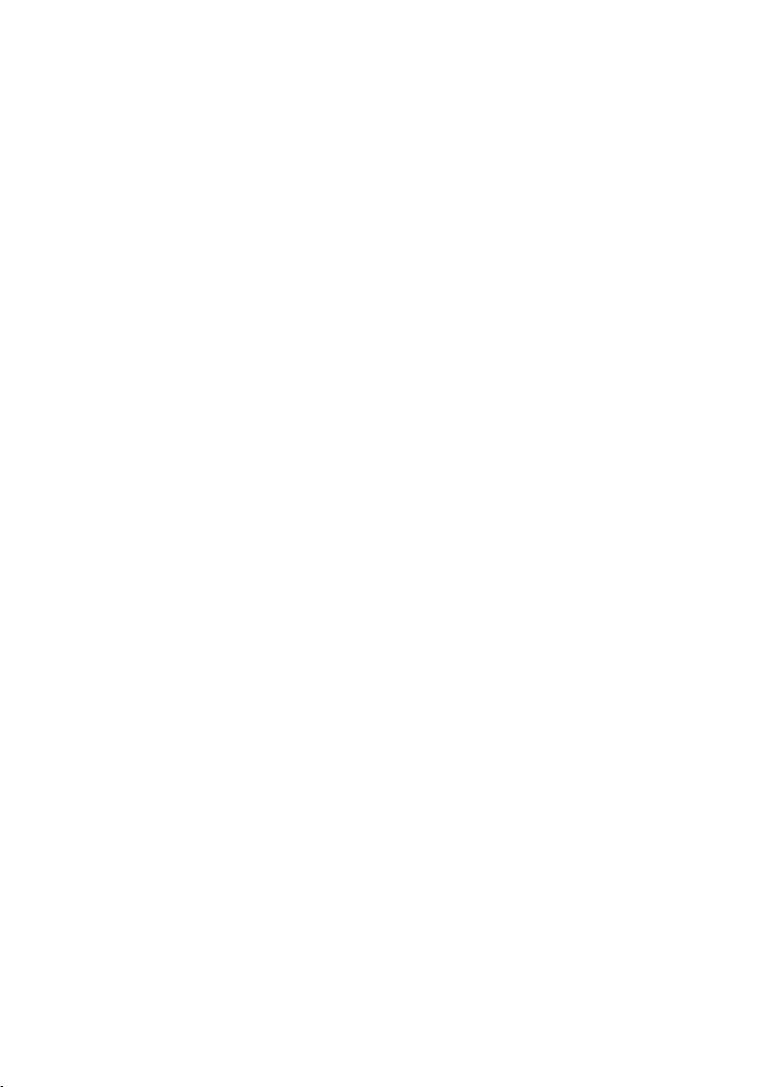
ptg18655082
Dynamic Arrays
...................................................................................................74
C-style Character Strings
.......................................................................................76
C++ Strings: Using
std::string ...........................................................................79
LESSON 5: Working with Expressions, Statements, and Operators 85
Statements ..........................................................................................................86
Compound Statements or Blocks
............................................................................87
Using Operators
...................................................................................................87
The Assignment Operator (
=) ........................................................................87
Understanding L-values and R-values
.............................................................87
Operators to Add (
+), Subtract (-), Multiply (*), Divide (/),
and Modulo Divide (
%) .................................................................................88
Operators to Increment (
++) and Decrement (--) ..............................................89
To Postfix or to Prefix?
................................................................................90
Equality Operators (
==) and (!=) ....................................................................92
Relational Operators
....................................................................................92
Logical Operations NOT, AND, OR, and XOR
.................................................95
Using C++ Logical Operators NOT (
!), AND (&&), and OR (||) .........................96
Bitwise NOT (
~), AND (&), OR (|), and XOR (^) Operators ............................. 100
Bitwise Right Shift (
>>) and Left Shift (<<) Operators ..................................... 102
Compound Assignment Operators
................................................................ 104
Using Operator
sizeof to Determine the Memory Occupied by a Variable .......... 106
Operator Precedence
.................................................................................. 108
LESSON 6: Controlling Program Flow 113
Conditional Execution Using if … else ............................................................... 114
Conditional Programming Using
if … else .................................................. 115
Executing Multiple Statements Conditionally
................................................. 117
Nested
if Statements ................................................................................. 118
Conditional Processing Using
switch-case................................................... 122
Conditional Execution Using Operator (
?:).................................................... 126
Getting Code to Execute in Loops
......................................................................... 128
A Rudimentary Loop Using
goto ................................................................. 128
The
while Loop ....................................................................................... 130
The
do…while Loop .................................................................................. 132
The
for Loop ........................................................................................... 133
The Range-Based
for Loop ........................................................................ 137