没有合适的资源?快使用搜索试试~ 我知道了~
Beej's Guide to Network Programming
需积分: 9 4 下载量 21 浏览量
2015-04-03
16:31:14
上传
评论
收藏 727KB PDF 举报
温馨提示
网络编程经典书,学C++的必看,英文原版
资源推荐
资源详情
资源评论
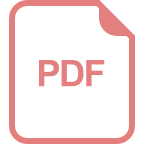
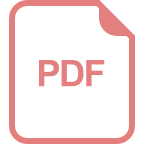
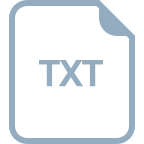
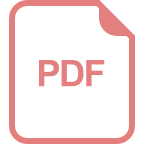
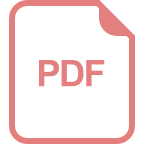
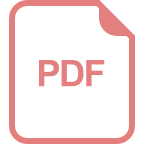
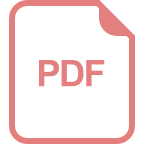
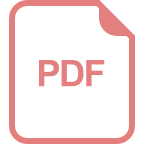
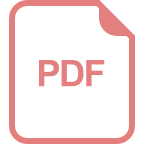
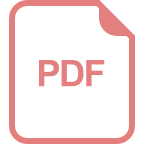
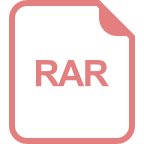
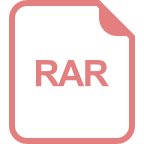
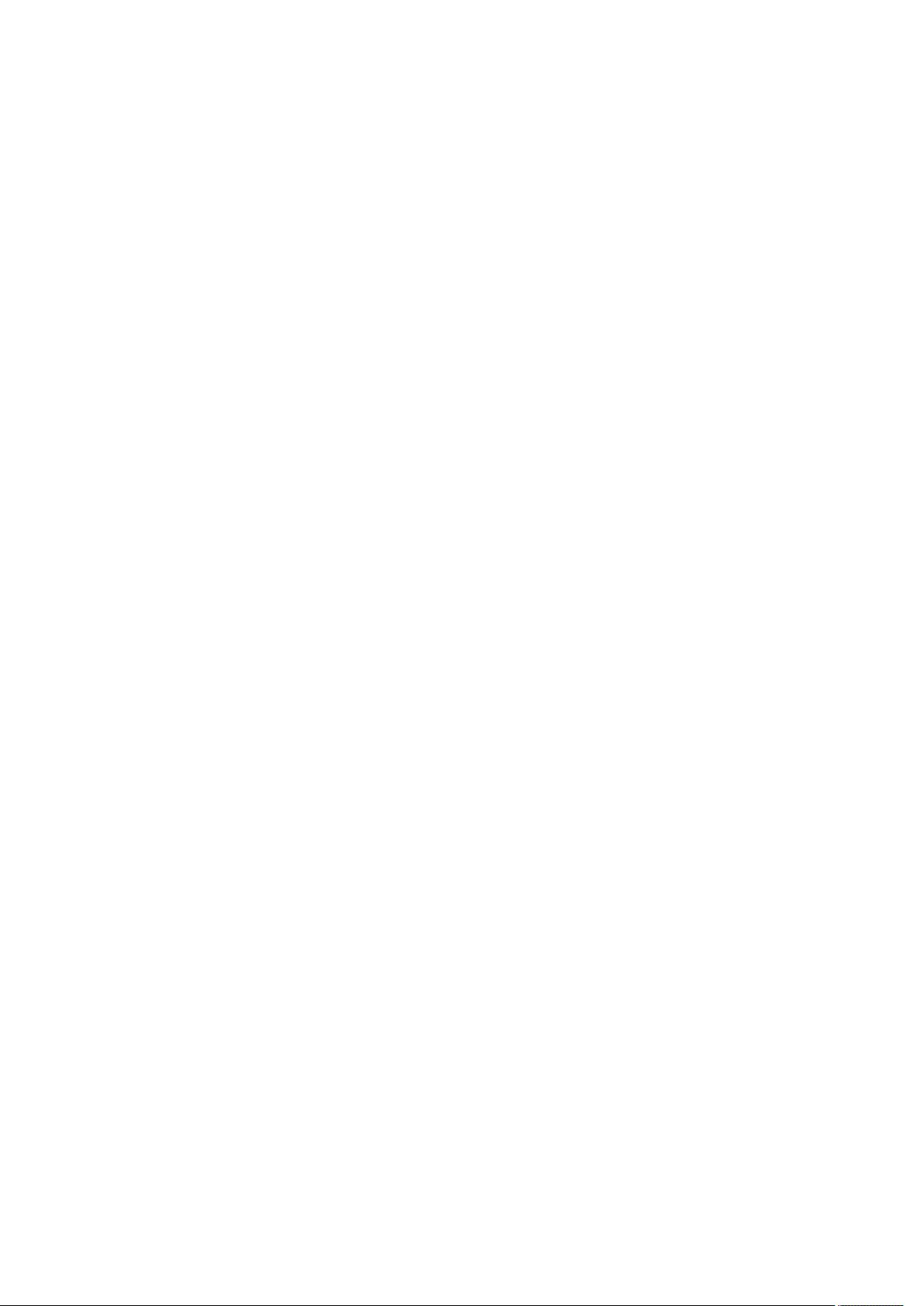
Beej's Guide to Network Programming
Using Internet Sockets
Brian “Beej Jorgensen” Hall
beej@beej.us
Version 3.0.15
July 3, 2012
Copyright © 2012 Brian “Beej Jorgensen” Hall
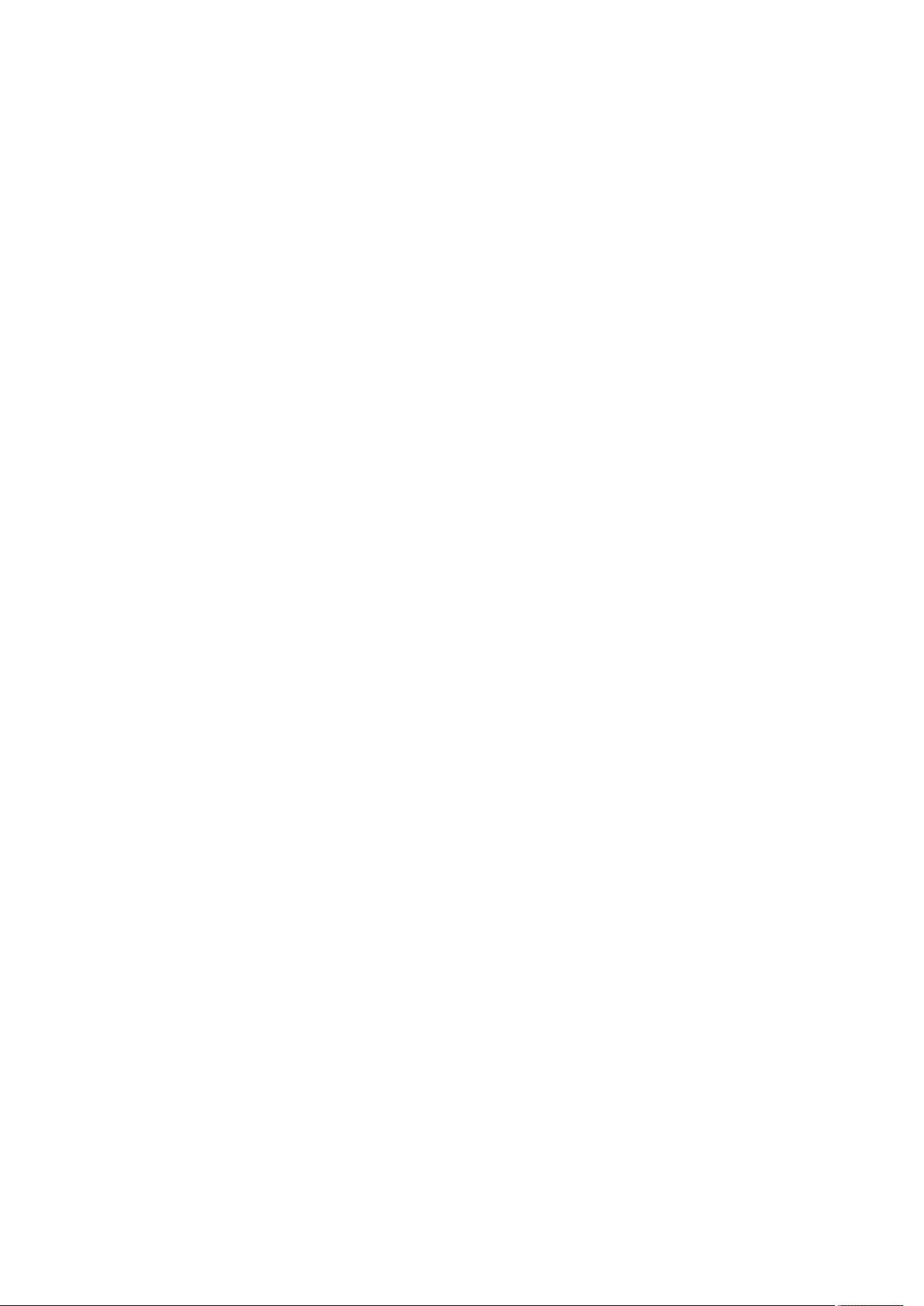
Thanks to everyone who has helped in the past and future with me getting this guide written. Thanks to Ashley for
helping me coax the cover design into the best programmer art I could. Thank you to all the people who produce the
Free software and packages that I use to make the Guide: GNU, Linux, Slackware, vim, Python, Inkscape, Apache
FOP, Firefox, Red Hat, and many others. And finally a big thank-you to the literally thousands of you who have
written in with suggestions for improvements and words of encouragement.
I dedicate this guide to some of my biggest heroes and inpirators in the world of computers: Donald Knuth, Bruce
Schneier, W. Richard Stevens, and The Woz, my Readership, and the entire Free and Open Source Software
Community.
This book is written in XML using the vim editor on a Slackware Linux box loaded with GNU tools. The cover
“art” and diagrams are produced with Inkscape. The XML is converted into HTML and XSL-FO by custom Python
scripts. The XSL-FO output is then munged by Apache FOP to produce PDF documents, using Liberation fonts.
The toolchain is composed of 100% Free and Open Source Software.
Unless otherwise mutually agreed by the parties in writing, the author offers the work as-is and makes no
representations or warranties of any kind concerning the work, express, implied, statutory or otherwise, including,
without limitation, warranties of title, merchantibility, fitness for a particular purpose, noninfringement, or the absence
of latent or other defects, accuracy, or the presence of absence of errors, whether or not discoverable.
Except to the extent required by applicable law, in no event will the author be liable to you on any legal theory for
any special, incidental, consequential, punitive or exemplary damages arising out of the use of the work, even if the
author has been advised of the possibility of such damages.
This document is freely distributable under the terms of the Creative Commons Attribution-Noncommercial-No
Derivative Works 3.0 License. See the Copyright and Distribution section for details.
Copyright © 2012 Brian “Beej Jorgensen” Hall
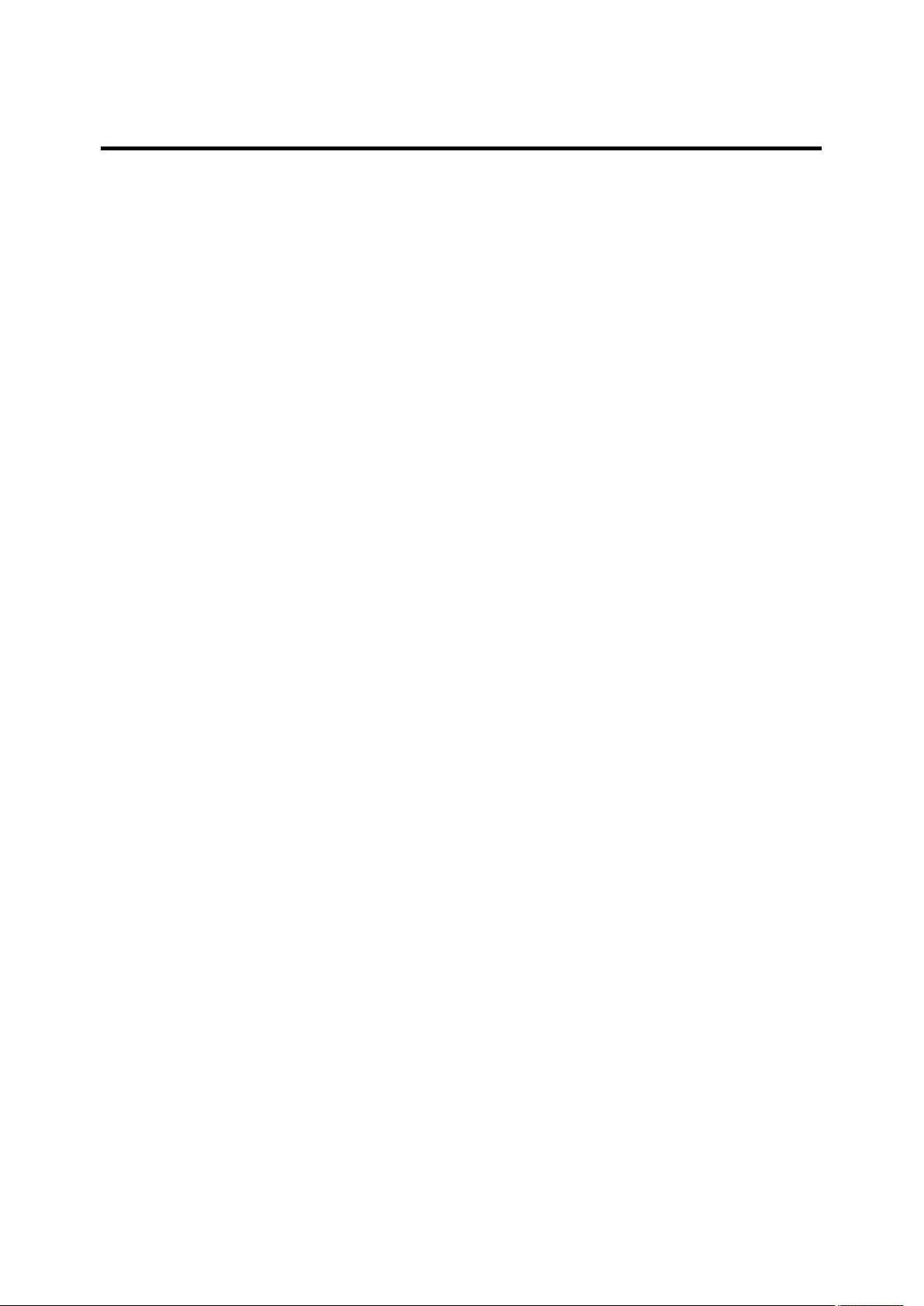
iii
Contents
1. Intro......................................................................................................................................................... 1
1.1. Audience 1
1.2. Platform and Compiler 1
1.3. Official Homepage and Books For Sale 1
1.4. Note for Solaris/SunOS Programmers 1
1.5. Note for Windows Programmers 1
1.6. Email Policy 2
1.7. Mirroring 3
1.8. Note for Translators 3
1.9. Copyright and Distribution 3
2. What is a socket?................................................................................................................................... 4
2.1. Two Types of Internet Sockets 4
2.2. Low level Nonsense and Network Theory 5
3. IP Addresses, structs, and Data Munging........................................................................................ 7
3.1. IP Addresses, versions 4 and 6 7
3.2. Byte Order 9
3.3. structs 10
3.4. IP Addresses, Part Deux 12
4. Jumping from IPv4 to IPv6................................................................................................................ 14
5. System Calls or Bust............................................................................................................................15
5.1. getaddrinfo()—Prepare to launch! 15
5.2. socket()—Get the File Descriptor! 18
5.3. bind()—What port am I on? 18
5.4. connect()—Hey, you! 20
5.5. listen()—Will somebody please call me? 20
5.6. accept()—“Thank you for calling port 3490.” 21
5.7. send() and recv()—Talk to me, baby! 22
5.8. sendto() and recvfrom()—Talk to me, DGRAM-style 23
5.9. close() and shutdown()—Get outta my face! 23
5.10. getpeername()—Who are you? 24
5.11. gethostname()—Who am I? 24
6. Client-Server Background...................................................................................................................25
6.1. A Simple Stream Server 25
6.2. A Simple Stream Client 27
6.3. Datagram Sockets 29
7. Slightly Advanced Techniques............................................................................................................ 33
7.1. Blocking 33
7.2. select()—Synchronous I/O Multiplexing 33
7.3. Handling Partial send()s 38
7.4. Serialization—How to Pack Data 39
7.5. Son of Data Encapsulation 46
7.6. Broadcast Packets—Hello, World! 48
8. Common Questions.............................................................................................................................. 51
9. Man Pages.............................................................................................................................................56
9.1. accept() 57
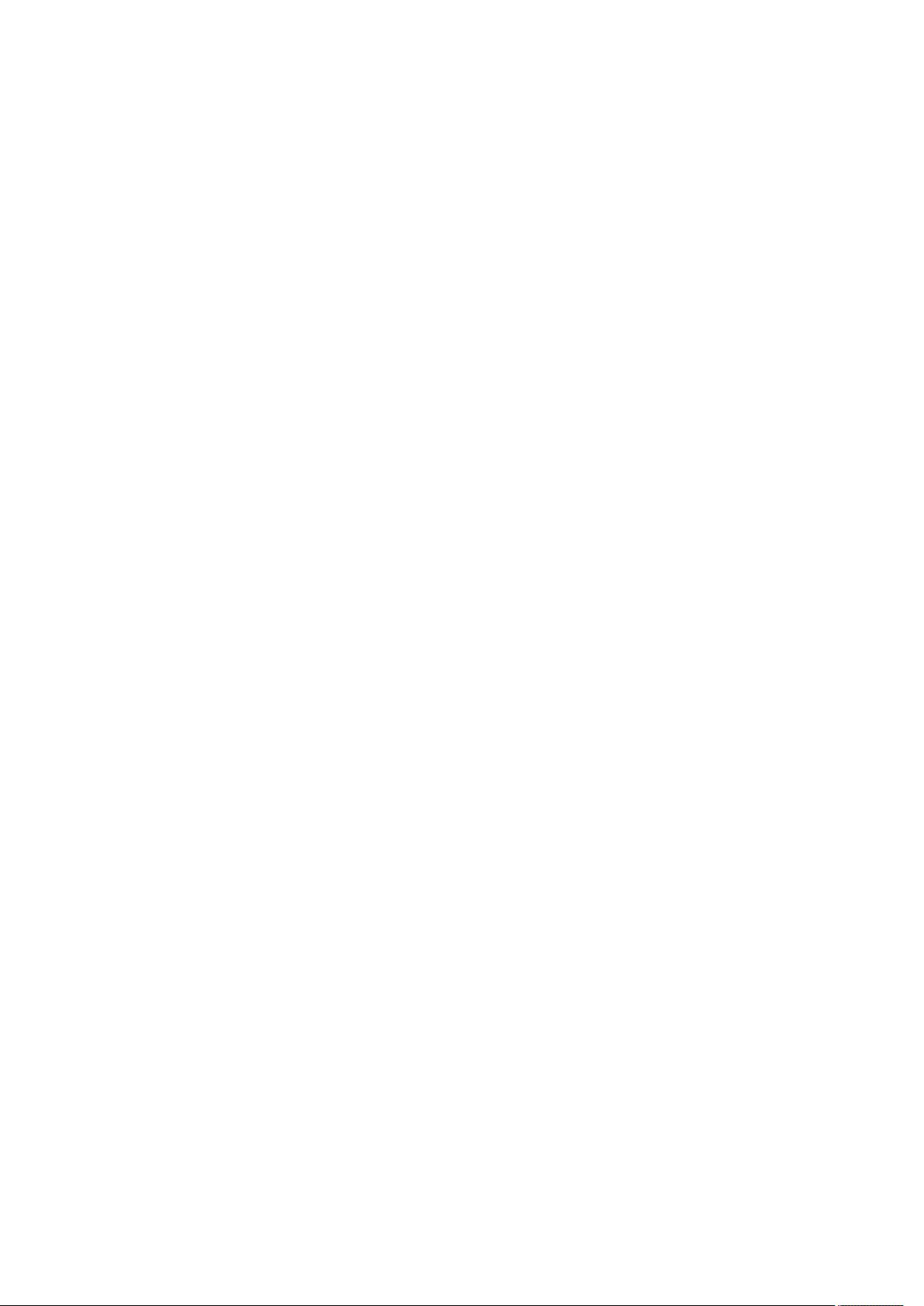
Contents
iv
9.2. bind() 59
9.3. connect() 61
9.4. close() 62
9.5. getaddrinfo(), freeaddrinfo(), gai_strerror() 63
9.6. gethostname() 66
9.7. gethostbyname(), gethostbyaddr() 67
9.8. getnameinfo() 69
9.9. getpeername() 70
9.10. errno 71
9.11. fcntl() 72
9.12. htons(), htonl(), ntohs(), ntohl() 73
9.13. inet_ntoa(), inet_aton(), inet_addr 74
9.14. inet_ntop(), inet_pton() 75
9.15. listen() 77
9.16. perror(), strerror() 78
9.17. poll() 79
9.18. recv(), recvfrom() 81
9.19. select() 83
9.20. setsockopt(), getsockopt() 85
9.21. send(), sendto() 87
9.22. shutdown() 89
9.23. socket() 90
9.24. struct sockaddr and pals 91
10. More References................................................................................................................................. 93
10.1. Books 93
10.2. Web References 93
10.3. RFCs 94
Index 96
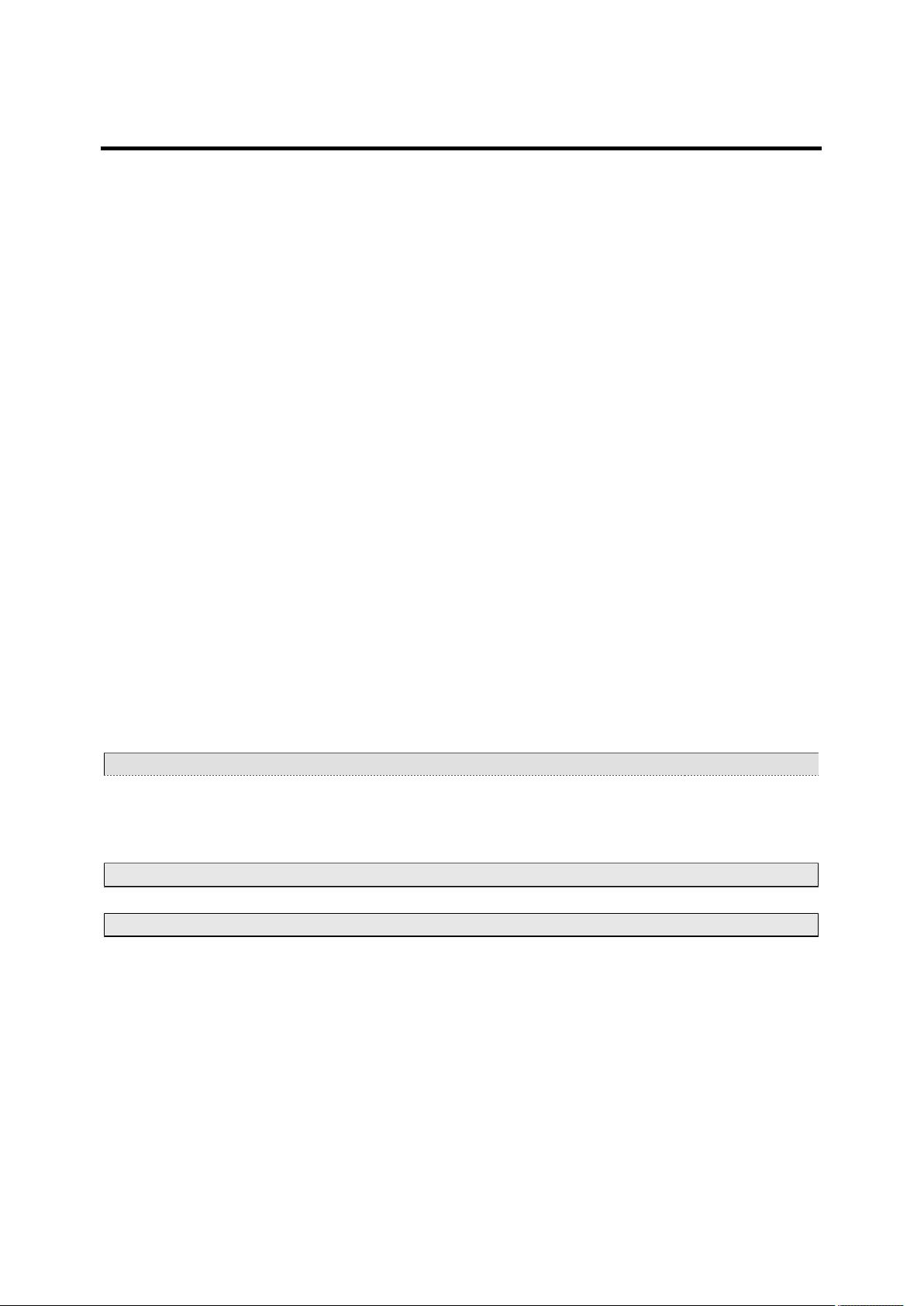
1
1. Intro
Hey! Socket programming got you down? Is this stuff just a little too difficult to figure out from the
man pages? You want to do cool Internet programming, but you don't have time to wade through a gob
of structs trying to figure out if you have to call bind() before you connect(), etc., etc.
Well, guess what! I've already done this nasty business, and I'm dying to share the information
with everyone! You've come to the right place. This document should give the average competent C
programmer the edge s/he needs to get a grip on this networking noise.
And check it out: I've finally caught up with the future (just in the nick of time, too!) and have
updated the Guide for IPv6! Enjoy!
1.1. Audience
This document has been written as a tutorial, not a complete reference. It is probably at its best
when read by individuals who are just starting out with socket programming and are looking for a
foothold. It is certainly not the complete and total guide to sockets programming, by any means.
Hopefully, though, it'll be just enough for those man pages to start making sense... :-)
1.2. Platform and Compiler
The code contained within this document was compiled on a Linux PC using Gnu's gcc compiler.
It should, however, build on just about any platform that uses gcc. Naturally, this doesn't apply if you're
programming for Windows—see the section on Windows programming, below.
1.3. Official Homepage and Books For Sale
This official location of this document is http://beej.us/guide/bgnet/. There you will also
find example code and translations of the guide into various languages.
To buy nicely bound print copies (some call them “books”), visit http://beej.us/guide/url/
bgbuy. I'll appreciate the purchase because it helps sustain my document-writing lifestyle!
1.4. Note for Solaris/SunOS Programmers
When compiling for Solaris or SunOS, you need to specify some extra command-line switches for
linking in the proper libraries. In order to do this, simply add “-lnsl -lsocket -lresolv” to the end
of the compile command, like so:
$ cc -o server server.c -lnsl -lsocket -lresolv
If you still get errors, you could try further adding a “-lxnet” to the end of that command line. I
don't know what that does, exactly, but some people seem to need it.
Another place that you might find problems is in the call to setsockopt(). The prototype differs
from that on my Linux box, so instead of:
int yes=1;
enter this:
char yes='1';
As I don't have a Sun box, I haven't tested any of the above information—it's just what people have
told me through email.
1.5. Note for Windows Programmers
At this point in the guide, historically, I've done a bit of bagging on Windows, simply due to the fact
that I don't like it very much. But I should really be fair and tell you that Windows has a huge install base
and is obviously a perfectly fine operating system.
They say absence makes the heart grow fonder, and in this case, I believe it to be true. (Or maybe
it's age.) But what I can say is that after a decade-plus of not using Microsoft OSes for my personal work,
I'm much happier! As such, I can sit back and safely say, “Sure, feel free to use Windows!” ...Ok yes, it
does make me grit my teeth to say that.
剩余100页未读,继续阅读
资源评论
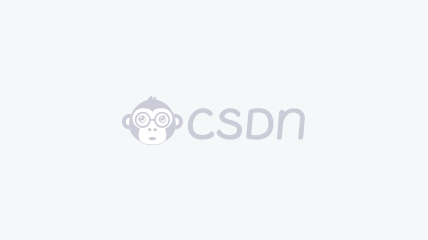

MQ_H
- 粉丝: 0
- 资源: 12
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

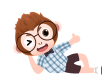
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


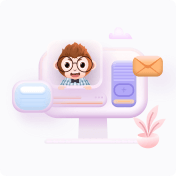
安全验证
文档复制为VIP权益,开通VIP直接复制
