package cardclient;
import javax.microedition.io.SocketConnection;
import java.io.InputStream;
import javax.microedition.io.Connector;
import java.io.OutputStream;
/**
* <p>Title: CardClient</p>
*
* <p>Description: lizhenpeng</p>
*
* <p>Copyright: Copyright (c) 2005</p>
*
* <p>Company: LP&P</p>
*
* @author lipeng
* @version 1.0
*/
import lipeng.*;
import javax.microedition.lcdui.*;
public class GameManager
implements Runnable
{
public GameManager()
{
inst=this;
gameState=0;
for(int i=0;i<30;i++)
{
userCard[i]=new Card();
oppoCard[i]=new Card();
userPostCard[i]=new Card();
oppoPostCard[i]=new Card();
}
}
public void run()
{
while(networkEnable)
{
try
{
in.read(recvHeadBuffer,0,4);
recvDataHead.FillData(recvHeadBuffer,0);
if(recvDataHead.size!=0)
{
recvBuffer=new byte[recvDataHead.size];
in.read(recvBuffer,0,recvDataHead.size);
//处理数据
ProcessData();
} else
{
//处理信息
ProcessData();
}
} catch(Exception e)
{
System.out.println(e);
}
}
}
public void openSocket()
{
try
{
conn=(SocketConnection)Connector.open("socket://localhost:8070");
in=conn.openInputStream();
out=conn.openOutputStream();
networkEnable=true;
sendDataHead.command=NetProtocol.LOGIN;
//16X2
sendDataHead.size=32;
byte[] buffer=userName.getBytes();
byte[] buffer2=new byte[32];
System.arraycopy(buffer,0,buffer2,0,buffer.length);
this.sendBuffer=sendDataHead.getBytes();
out.write(sendBuffer,0,sendBuffer.length);
out.write(buffer2,0,buffer2.length);
gameState=2;
} catch(Exception e)
{
System.out.print(e);
}
networkThread=new Thread(this);
networkThread.start();
}
private void hasControl()
{
this.keyAction=0;
this.iControl=true;
cursorX=0;
cursorY=MainGame.inst.h-22*3;
cardSelect=0;
currentCard=0;
isSelect=false;
}
public void ProcessData()
{
switch(recvDataHead.command)
{
case NetProtocol.HASLOGIN:
{
userList=new String[recvDataHead.size/32];
int i;
int place=0;
for(i=0;i<userList.length;i++)
{
userList[i]=new String(this.recvBuffer,place,32);
place+=32;
}
MainGame.inst.userList=new UserList();
Display.getDisplay(CardClientMIDlet.instance).setCurrent(MainGame.inst.
userList);
}
break;
case NetProtocol.STARTGAME_FIRST:
{
Display.getDisplay(CardClientMIDlet.instance).setCurrent(MainGame.inst);
int place = 0;
this.userCardNum = 54/2;
this.oppoCardNum = 54/2;
for(int i=0;i<27;i++)
{
this.userCard[i].fillData(this.recvBuffer,place);
place += 4;
}
for(int i=0;i<27;i++)
{
this.oppoCard[i].fillData(this.recvBuffer,place);
place +=4;
}
gameState = 3;
hasControl();
}
break;
case NetProtocol.STARTGAME_LAST:
{
Display.getDisplay(CardClientMIDlet.instance).setCurrent(MainGame.inst);
int place=0;
this.iControl=false;
this.userCardNum=54/2;
this.oppoCardNum=54/2;
for(int i=0;i<27;i++)
{
this.userCard[i].fillData(this.recvBuffer,place);
place+=4;
}
for(int i=0;i<27;i++)
{
this.oppoCard[i].fillData(this.recvBuffer,place);
place+=4;
}
this.gameState=3;
}
break;
case NetProtocol.OPPO_REJECT:
{
this.userPostCardNum = 0;
hasControl();
}
break;
case NetProtocol.OPPO_POSTCARD:
{
this.oppoPostCard[0].fillData(recvBuffer,0);
userPostCardNum = 0;
oppoPostCardNum=1;
hasControl();
}
break;
}
}
public void keyPressd(int keyCode,int gameKeyCode)
{
switch(gameKeyCode)
{
case Canvas.UP:
keyAction|=LPKeyMask.MASK_KEY_UP;
break;
case Canvas.DOWN:
keyAction|=LPKeyMask.MASK_KEY_DOWN;
break;
case Canvas.FIRE:
keyAction|=LPKeyMask.MASK_KEY_OK;
break;
case Canvas.LEFT:
keyAction|=LPKeyMask.MASK_KEY_LEFT;
break;
case Canvas.RIGHT:
keyAction|=LPKeyMask.MASK_KEY_RIGHT;
break;
}
}
public void keyReleased(int keyCode,int gameKeyCode)
{
switch(gameKeyCode)
{
case Canvas.FIRE:
keyAction&=~LPKeyMask.MASK_KEY_OK;
break;
case Canvas.UP:
keyAction&=~LPKeyMask.MASK_KEY_UP;
break;
case Canvas.DOWN:
keyAction&=~LPKeyMask.MASK_KEY_DOWN;
break;
case Canvas.LEFT:
keyAction&=~LPKeyMask.MASK_KEY_LEFT;
break;
case Canvas.RIGHT:
keyAction&=~LPKeyMask.MASK_KEY_RIGHT;
break;
}
}
public void menuAction()
{
if((keyAction&LPKeyMask.MASK_KEY_UP)!=0)
{
keyAction&=~LPKeyMask.MASK_KEY_UP;
if(this.menuWhichActive>0)
{
--menuWhichActive;
} else
{
menuWhichActive=2;
}
} else if((keyAction&LPKeyMask.MASK_KEY_DOWN)!=0)
{
keyAction&=~LPKeyMask.MASK_KEY_DOWN;
if(this.menuWhichActive<2)
{
++menuWhichActive;
} else
{
menuWhichActive=0;
}
} else if((keyAction&LPKeyMask.MASK_KEY_OK)!=0)
{
keyAction&=~LPKeyMask.MASK_KEY_OK;
switch(menuWhichActive)
{
case 0:
//登陆游戏
//openSocket();
gameState = 1;
Display.getDisplay(CardClientMIDlet.instance).setCurrent(MainGame.inst.input);
break;
case 1:
//帮助
break;
case 2:
//退出
break;
}
}
}
public void gameAction()
{
if(iControl)
{
int intern=MainGame.inst.w/14;
if((keyAction&LPKeyMask.MASK_KEY_OK)!=0)
{
keyAction&=~LPKeyMask.MASK_KEY_OK;
if(this.isSelect)
{
try
{
byte[] buffer=this.userCard[this.cardSelect].getBytes();
this.sendDataHead.command=NetProtocol.POST_CARD;
this.sendDataHead.size=(char)buffer.length;
this.sendBuffer=this.sendDataHead.getBytes();
this.out.write(sendBuffer,0,sendBuffer.length);
this.out.write(buffer,0,buffer.length);
this.iControl = false;
//出牌
this.userPostCard[0]= this.userCard[cardSelect];
this.userPostCardNum = 1;
//整理牌
for(int i=cardSelect;i<this.userCardNum-1;i++)
{
userCard[i] = userCard[i+1];
}
isSelect = false;
--userCardNum;
}
catch(Exception e)
{
System.out.print(e);
}
}
else
{
try
{
this.sendDataHead.command=NetProtocol.REJECT_CARD;
this.sendDataHead.size=0;
this.sendBuffer=this.sendDataHead.getBytes();
this.out.write(sendBuffer,0,sendBuffer.length);
}
catch(Exception e)
{
System.out.print(e);
}
}
} else if((keyAction&LPKeyMask.MASK_KEY_UP)!=0)
{
keyAction&=~LPKeyMask.MASK_KEY_UP;
this.isSelect = true;
this.cardSelect = currentCard;
} else if((keyAction&L
没有合适的资源?快使用搜索试试~ 我知道了~
资源详情
资源评论
资源推荐
收起资源包目录





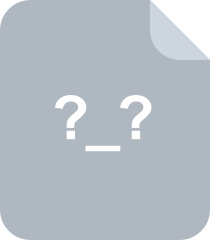
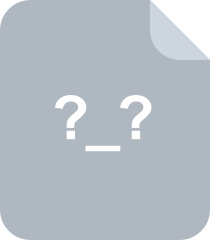
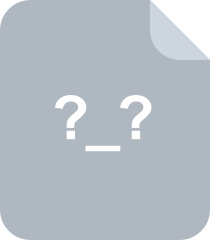


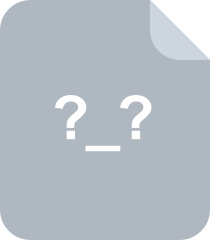
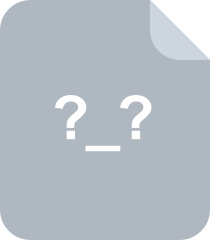
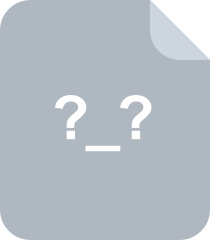
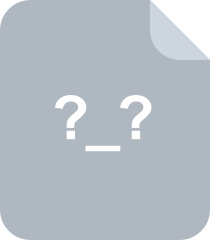
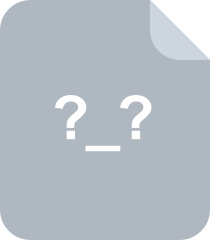
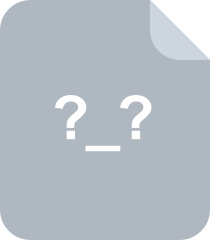
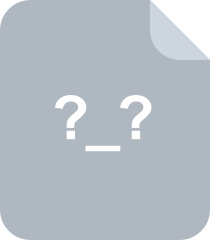



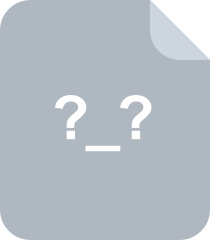

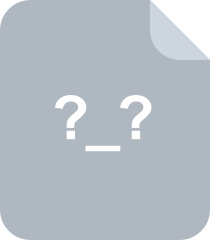
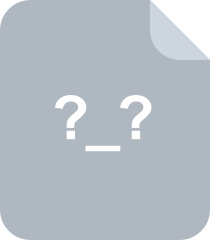
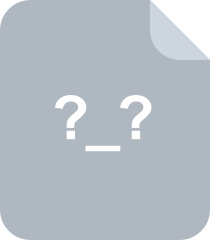


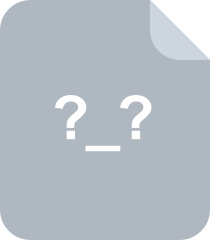

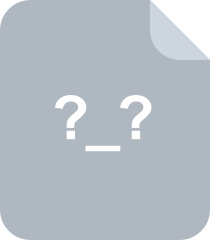
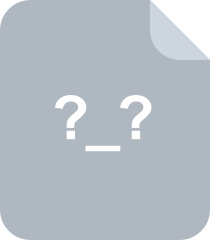
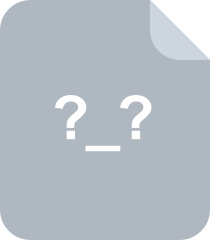
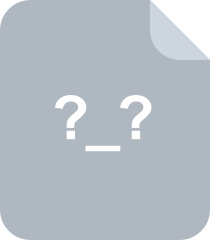
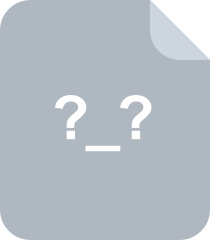
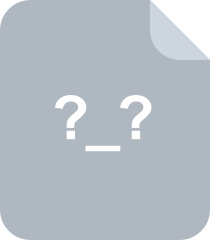
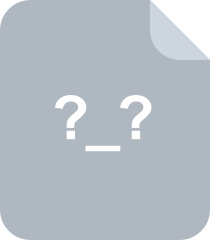

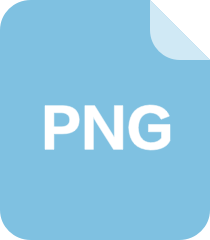
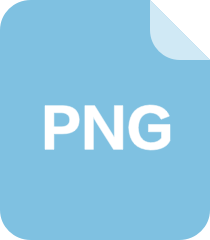


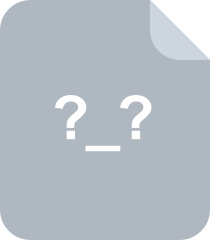
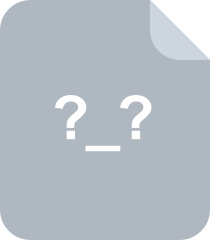
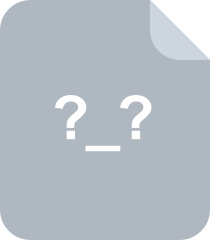
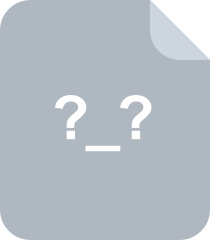
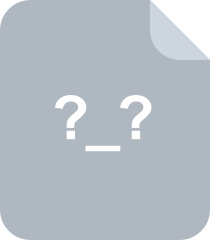
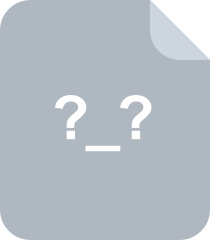
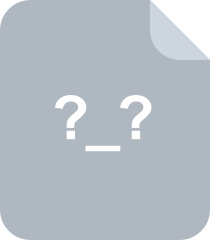
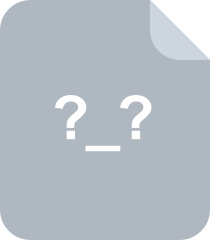
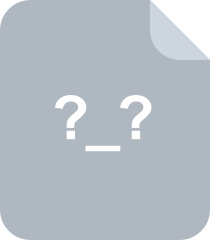
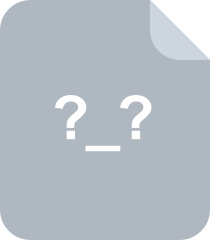
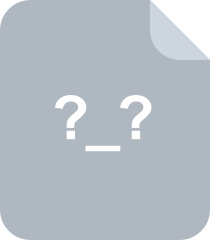
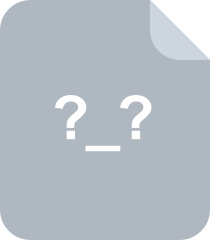
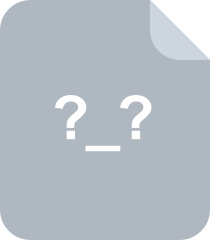
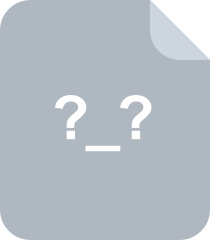
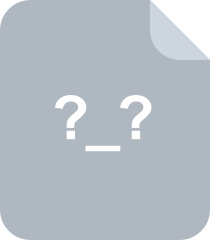
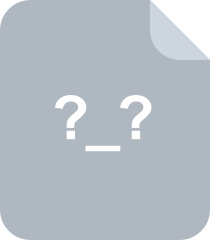
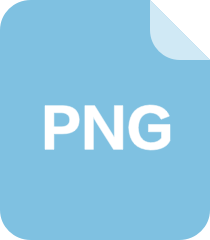

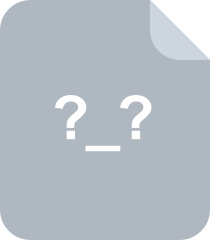
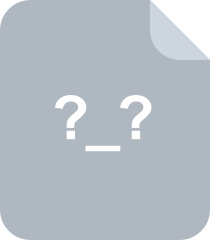
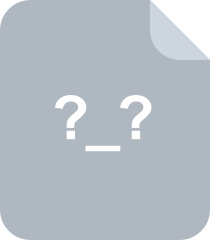
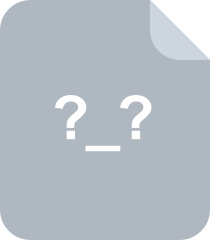
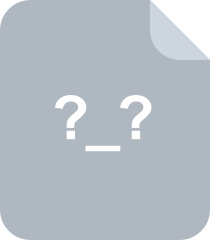
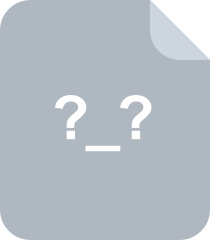
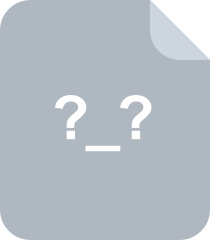

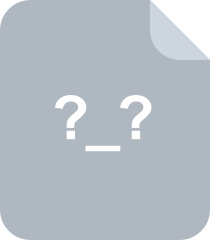
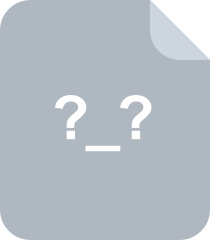
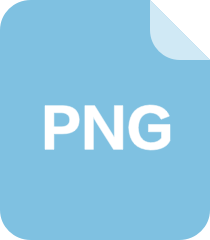
共 51 条
- 1
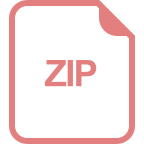
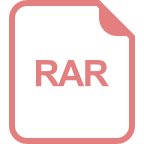
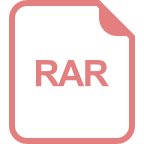
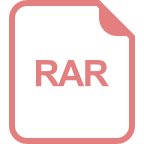
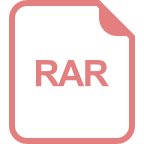
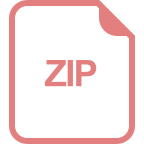
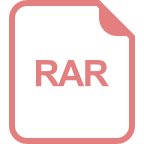
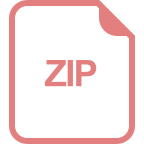
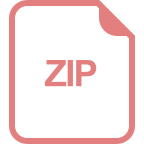
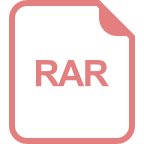
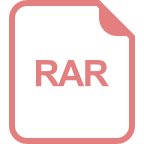
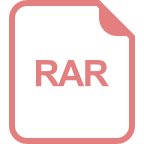
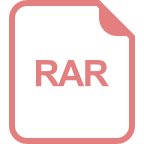
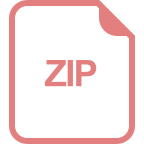
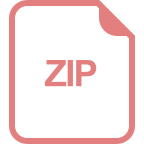
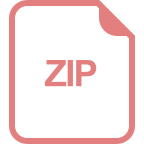
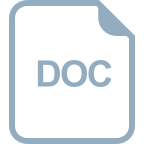
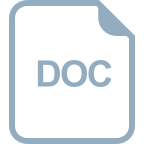
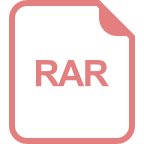
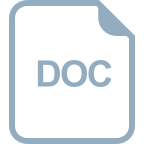

pengzhen295
- 粉丝: 4
- 资源: 10
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

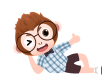
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


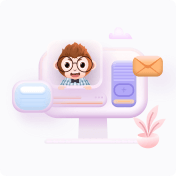
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0