# ECharts Liquid Fill Chart
Liquid Fill Chart plugin for [ECharts](https://github.com/ecomfe/echarts), which is usually used to represent data in percentage.

## Install ECharts
To use ECharts plugins, you need to include the plugin JavaScript file after ECharts file.
```html
<script src='echarts.js'></script>
<script src='echarts-liquidfill.js'></script>
```
ECharts can be downloaded at [GitHub dist directory](https://github.com/ecomfe/echarts/tree/master/dist) or [Download page of Official Website](http://echarts.baidu.com/download.html) (in Chinese).
>#### NOTE
>
> The minimum package of ECharts required by LiquidFill Chart is [simple version](https://github.com/ecomfe/echarts/blob/master/dist/echarts.simple.js) on GitHub, or selecting nothing in [online builder](http://echarts.baidu.com/builder.html) (in Chinese). If you need other chart types or components in your other chart, you should include them accordingly.
## Install echarts-liquidfill with npm
```sh
npm install echarts-liquidfill
```
npm will warn you that you have to install peer dependencies by yourself:
```
npm WARN echarts-liquidfill@2.0.4 requires a peer of echarts@^4.2.1 but none is installed. You must install peer dependencies yourself.
npm WARN echarts-liquidfill@2.0.4 requires a peer of zrender@^4.0.7 but none is installed. You must install peer dependencies yourself.
```
Note that the version number may change in your case. Install will the version it shows.
```
npm i echarts@^4.2.1 zrender@^4.0.7
```
## Download echarts-liquidfill from GitHub
You may download the lastest ECharts files on [ECharts official site](http://echarts.baidu.com/download.html) and download this plugin in [dist directory](https://github.com/ecomfe/echarts-liquidfill/tree/master/dist). Note that if you need tooltip for Liquid Fill Chart, you need the complete ECharts version. Otherwise, simple version will do.
## Notes
### Omitted `normal`
Since [ECharts v4.0.0]((https://github.com/ecomfe/echarts/releases/tag/4.0.0)), `normal` is no longer needed for `itemStyle` or `label`.
### Flatten `textStyle`
Since [ECharts v3.7.0](https://github.com/ecomfe/echarts/releases/tag/3.7.0), `textStyle` option is flatten, so that `series.label[normal|emphasis].textStyle.xxx` is now can be written in `series.label[normal|emphasis].textStyle`. This is supported from [echarts-liquidfill](https://github.com/ecomfe/echarts-liquidfill/releases/tag/v1.0.6) v1.0.6. So if you found examples with `textStyle` in old demo, don't be too surprised.
## Quick Start
- [API](https://github.com/ecomfe/echarts-liquidfill#api)
- [Examples at ECharts Gallery](http://gallery.echartsjs.com/explore.html#tags=liquidFill~sort=rank~timeframe=all~author=all)
Here are some common uses:
- [Use multiple waves in a chart](https://github.com/ecomfe/echarts-liquidfill#multiple-waves)
- [Change waves color and opacity](https://github.com/ecomfe/echarts-liquidfill#color-and-opacity)
- [Make waves static](https://github.com/ecomfe/echarts-liquidfill#static-waves)
- [Water with no waves](https://github.com/ecomfe/echarts-liquidfill#still-water)
- [Set attributes for a single wave](https://github.com/ecomfe/echarts-liquidfill#change-a-single-wave)
- [Change background and border style](https://github.com/ecomfe/echarts-liquidfill#background-style)
- [Hide outline in chart](https://github.com/ecomfe/echarts-liquidfill#outline-style)
- [Change shape with SVG](https://github.com/ecomfe/echarts-liquidfill#shape)
- [Setup animation](https://github.com/ecomfe/echarts-liquidfill#animation)
- [Change text content and style](https://github.com/ecomfe/echarts-liquidfill#change-text)
- [Change shadow style](https://github.com/ecomfe/echarts-liquidfill#shadow)
- [Setup tooltip](https://github.com/ecomfe/echarts-liquidfill#tooltip)
- [Click event](https://github.com/ecomfe/echarts-liquidfill#click-event)
- [Make an element non-interactable](https://github.com/ecomfe/echarts-liquidfill#non-interactable)
To ask a question, you may fork [Liquid Fill Chart Example on Gallery](http://gallery.echartsjs.com/editor.html?c=xr1XplzB4e) and copy your code there. Then you may [open an issue](https://github.com/ecomfe/echarts-liquidfill/issues/new) in this project.
## Examples
### A Simple Example
To create a Liquid Fill Chart, you need to have a series with type of `'liquidFill'`. A basic option may be:
```js
var option = {
series: [{
type: 'liquidFill',
data: [0.6]
}]
};
```

[Run](http://gallery.echartsjs.com/editor.html?c=xr1XplzB4e)
### Multiple Waves
It is easy to create a liquid fill chart will multiple waves, either to represent multiple data, or to improve the visual effect of the chart.
```js
var option = {
series: [{
type: 'liquidFill',
data: [0.6, 0.5, 0.4, 0.3]
}]
};
```
This creates a chart wit waves at position of 60%, 50%, 40%, and 30%.

[Run](http://gallery.echartsjs.com/editor.html?c=xSyIEWMBNl)
### Color and Opacity
To set colors for liquid fill chart series, set `color` to be an array of colors. To set opacity, use `itemStyle.opacity` and `itemStyle.emphasis.opacity` for normal style and hover style.
```js
var option = {
series: [{
type: 'liquidFill',
data: [0.5, 0.4, 0.3],
color: ['red', '#0f0', 'rgb(0, 0, 255)'],
itemStyle: {
opacity: 0.6
},
emphasis: {
itemStyle: {
opacity: 0.9
}
}
}]
};
```

[Run](http://gallery.echartsjs.com/editor.html?c=xrJpDC704l)
You may also set the color and opacity of a single data item by:
```js
var option = {
series: [{
type: 'liquidFill',
data: [0.5, 0.4, {
value: 0.3,
itemStyle: {
color: 'red',
opacity: 0.6
},
emphasis: {
itemStyle: {
opacity: 0.9
}
}
}]
}]
};
```

[Run](http://gallery.echartsjs.com/editor.html?c=xBJPCRXR4l)
### Static Waves
To provent the waves from moving left or right, you may simply set `waveAnimation` to be `false`. To disable the animation of waves raising, set `animationDuration` and `animationDurationUpdate` to be 0.
```js
var option = {
series: [{
type: 'liquidFill',
waveAnimation: false,
animationDuration: 0,
animationDurationUpdate: 0,
data: [0.6, 0.5, 0.4, 0.3]
}]
};
```

[Run](http://gallery.echartsjs.com/editor.html?c=xH1VfVVREx)
### Still Water
You may set the `amplitude` to be 0 to make still waves.
```js
var option = {
series: [{
type: 'liquidFill',
data: [0.6, 0.5, 0.4, 0.3],
amplitude: 0,
waveAnimation: 0
}]
};
```
It is recommended to set `waveAnimation` to be false in this case to disable animation for performance consideration.

[Run](http://gallery.echartsjs.com/editor.html?c=xHy1NHVCNx)
### Change A Single Wave
To change a single wave, overwrite the options in data item.
```js
var option = {
series: [{
type: 'liquidFill',
data: [0.6, {
value: 0.5,
direction: 'left',
itemStyle: {
color: 'red'
}
}, 0.4, 0.3]
}]
};
```

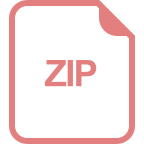
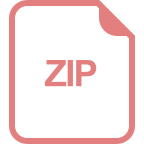
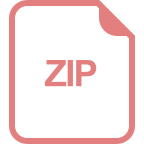
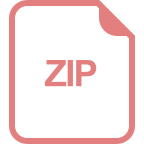
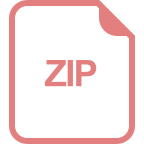
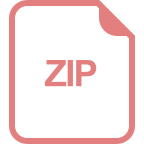
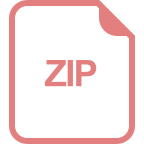
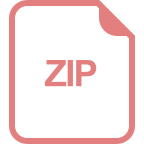
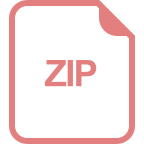
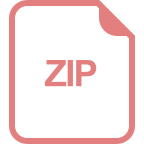
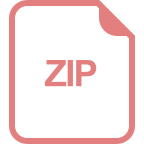
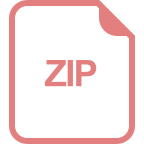
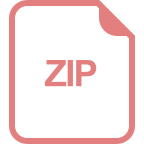
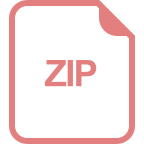
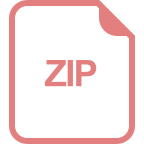
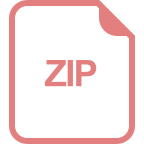
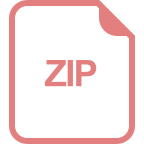
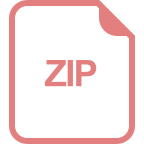
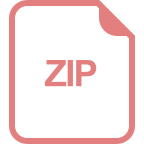
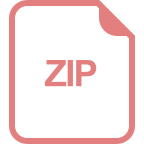
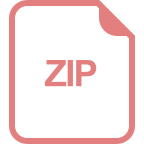
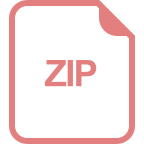
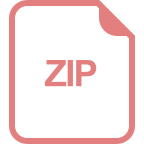
收起资源包目录




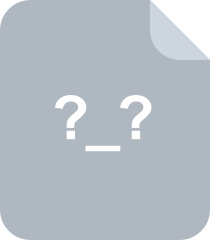

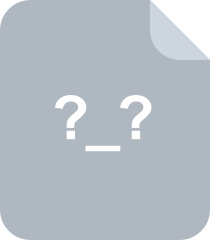
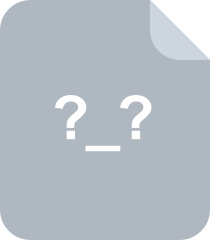
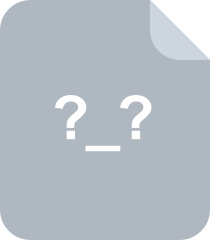

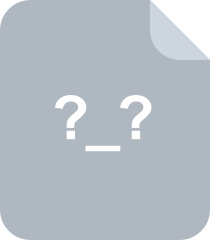
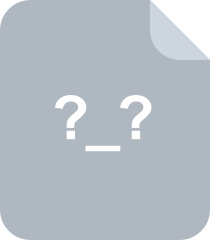
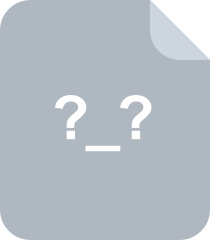
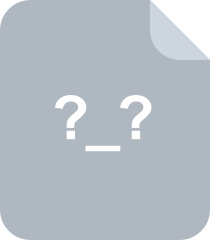
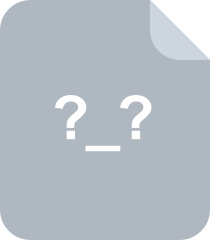
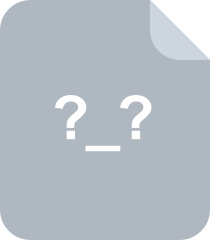

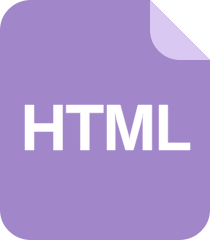
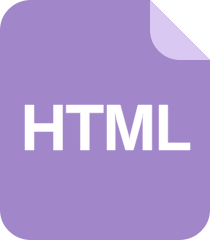
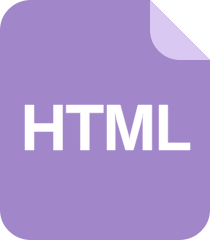
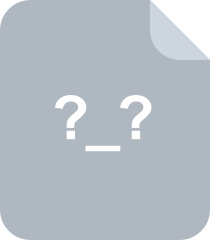

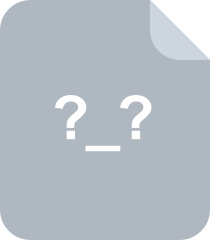
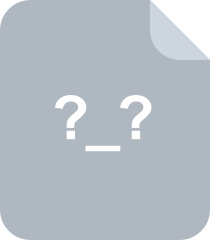
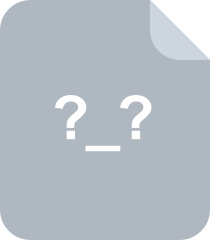
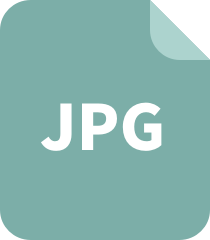
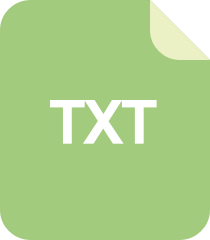

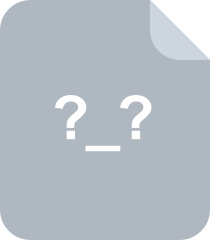
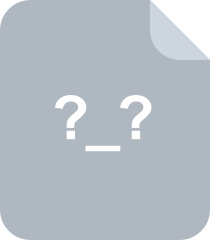
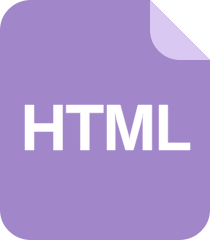

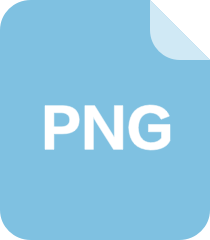
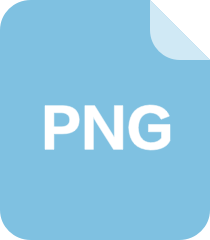
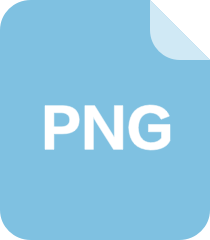
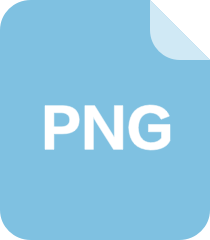
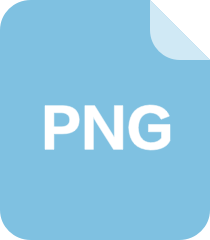
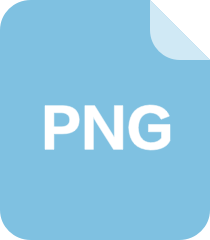
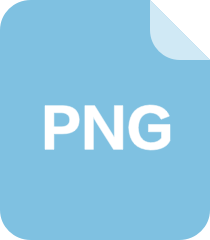
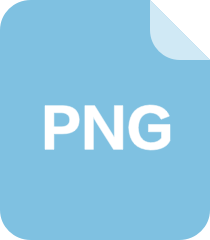
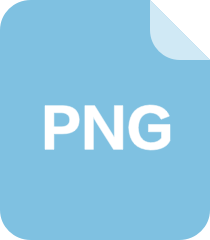
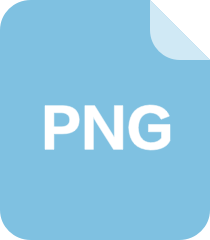
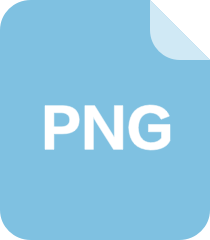
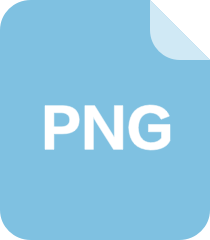
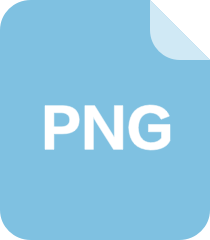
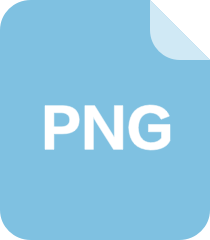
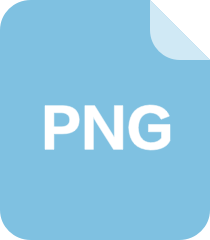
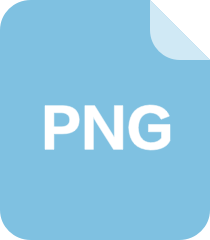
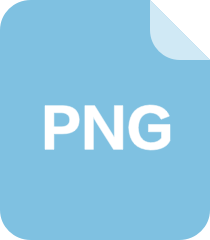
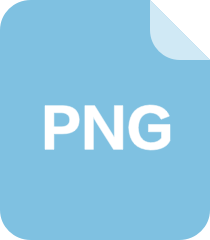
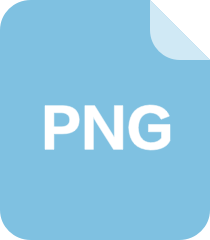
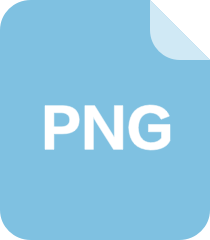
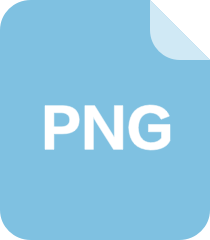
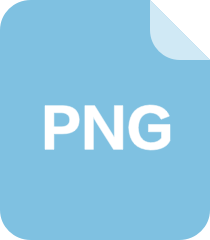
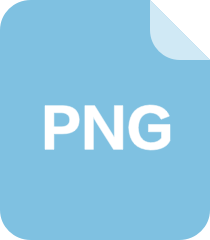
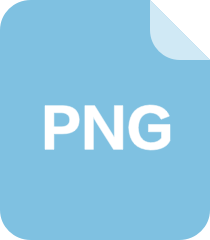
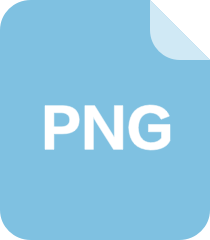
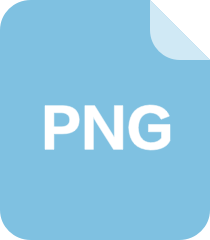
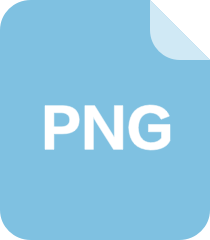
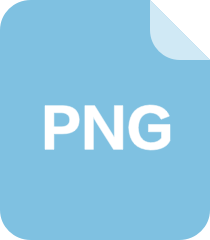
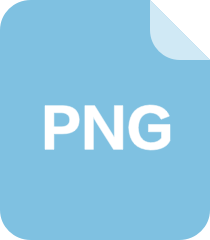
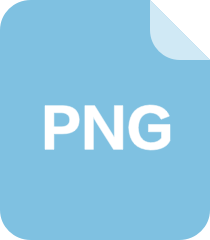
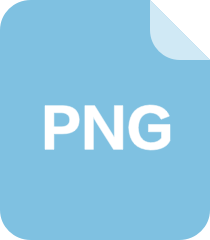
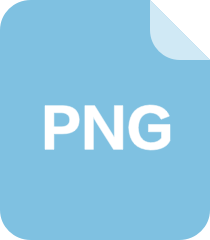
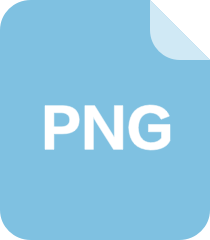

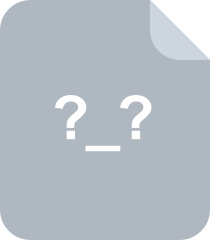
共 56 条
- 1
资源评论
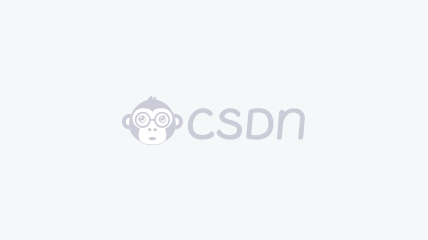
- 誏語錄2023-11-02资源有很好的参考价值,总算找到了自己需要的资源啦。
- 2301_801753712024-05-26非常有用的资源,可以直接使用,对我很有用,果断支持!

柯晓楠
- 粉丝: 2w+
- 资源: 2847
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

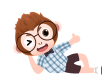
最新资源
- (源码)基于Java和MySQL的学生信息管理系统.zip
- (源码)基于ASP.NET Core的零售供应链管理系统.zip
- (源码)基于PythonSpleeter的戏曲音频处理系统.zip
- (源码)基于Spring Boot的监控与日志管理系统.zip
- (源码)基于C++的Unix V6++二级文件系统.zip
- (源码)基于Spring Boot和JPA的皮皮虾图片收集系统.zip
- (源码)基于Arduino和Python的实时歌曲信息液晶显示屏展示系统.zip
- (源码)基于C++和C混合模式的操作系统开发项目.zip
- (源码)基于Arduino的全球天气监控系统.zip
- OpenCVForUnity2.6.0.unitypackage
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


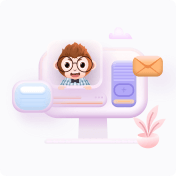
安全验证
文档复制为VIP权益,开通VIP直接复制
