/**
* Copyright (c) 2013, 2016 ControlsFX
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of ControlsFX, any associated website, nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL CONTROLSFX BE LIABLE FOR ANY
* DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES
* (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES;
* LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND
* ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.only.fx;
import static java.util.Objects.requireNonNull;
import static javafx.scene.input.MouseEvent.MOUSE_CLICKED;
import com.only.fx.skin.OnlyPopupOverSkin;
import javafx.animation.FadeTransition;
import javafx.beans.InvalidationListener;
import javafx.beans.Observable;
import javafx.beans.WeakInvalidationListener;
import javafx.beans.property.BooleanProperty;
import javafx.beans.property.DoubleProperty;
import javafx.beans.property.ObjectProperty;
import javafx.beans.property.SimpleBooleanProperty;
import javafx.beans.property.SimpleDoubleProperty;
import javafx.beans.property.SimpleObjectProperty;
import javafx.beans.property.SimpleStringProperty;
import javafx.beans.property.StringProperty;
import javafx.beans.value.ChangeListener;
import javafx.beans.value.ObservableValue;
import javafx.beans.value.WeakChangeListener;
import javafx.event.EventHandler;
import javafx.event.WeakEventHandler;
import javafx.geometry.Bounds;
import javafx.geometry.Insets;
import javafx.scene.Node;
import javafx.scene.control.Label;
import javafx.scene.control.PopupControl;
import javafx.scene.control.Skin;
import javafx.scene.layout.StackPane;
import javafx.stage.Window;
import javafx.stage.WindowEvent;
import javafx.util.Duration;
/**
* The PopOver control provides detailed information about an owning node in a
* popup window. The popup window has a very lightweight appearance (no default
* window decorations) and an arrow pointing at the owner. Due to the nature of
* popup windows the PopOver will move around with the parent window when the
* user drags it. <br>
* <center> <img src="popover.png" alt="Screenshot of PopOver"> </center> <br>
* The PopOver can be detached from the owning node by dragging it away from the
* owner. It stops displaying an arrow and starts displaying a title and a close
* icon. <br>
* <br>
* <center> <img src="popover-detached.png" alt=
* "Screenshot of a detached PopOver"> </center> <br>
* The following image shows a popover with an accordion content node. PopOver
* controls are automatically resizing themselves when the content node changes
* its size.<br>
* <br>
* <center> <img src="popover-accordion.png" alt=
* "Screenshot of PopOver containing an Accordion"> </center> <br>
* For styling apply stylesheets to the root pane of the PopOver.
*
* <h3>Example:</h3>
*
* <pre>
* PopOver popOver = new PopOver();
* popOver.getRoot().getStylesheets().add(...);
* </pre>
*
*/
public class OnlyPopupOver extends PopupControl {
private static final String DEFAULT_STYLE_CLASS = "only-popup-over"; //$NON-NLS-1$
private static final Duration DEFAULT_FADE_DURATION = Duration.seconds(.2);
private double targetX;
private double targetY;
private final SimpleBooleanProperty animated = new SimpleBooleanProperty(true);
private final ObjectProperty<Duration> fadeInDuration = new SimpleObjectProperty<>(DEFAULT_FADE_DURATION);
private final ObjectProperty<Duration> fadeOutDuration = new SimpleObjectProperty<>(DEFAULT_FADE_DURATION);
/**
* Creates a pop over with a label as the content node.
*/
public OnlyPopupOver() {
super();
getStyleClass().add(DEFAULT_STYLE_CLASS);
//getRoot().getStylesheets().add(OnlyPopupOver.class.getResource("/com/only/fx/OnlyPopupOver.css").toExternalForm()); //$NON-NLS-1$
getRoot().getStylesheets().add(this.getClass().getResource("/com/only/fx/OnlyPopupOver.css").toString());
setAnchorLocation(AnchorLocation.WINDOW_TOP_LEFT);
setOnHiding(new EventHandler<WindowEvent>() {
@Override
public void handle(WindowEvent evt) {
setDetached(false);
}
});
/*
* Create some initial content.
*/
Label label = new Label(""); //$NON-NLS-1$
label.setPrefSize(30, 10);
label.setPadding(new Insets(4));
setContentNode(label);
InvalidationListener repositionListener = observable -> {
if (isShowing() && !isDetached()) {
show(getOwnerNode(), targetX, targetY);
adjustWindowLocation();
}
};
arrowSize.addListener(repositionListener);
cornerRadius.addListener(repositionListener);
arrowLocation.addListener(repositionListener);
arrowIndent.addListener(repositionListener);
headerAlwaysVisible.addListener(repositionListener);
/*
* A detached popover should of course not automatically hide itself.
*/
detached.addListener(it -> {
if (isDetached()) {
setAutoHide(false);
} else {
setAutoHide(true);
}
});
setAutoHide(true);
}
/**
* Creates a pop over with the given node as the content node.
*
* @param content
* The content shown by the pop over
*/
public OnlyPopupOver(Node content) {
this();
setContentNode(content);
}
private final StackPane root = new StackPane();
/**
* The root pane stores the content node of the popover. It is accessible
* via this method in order to support proper styling.
*
* <h3>Example:</h3>
*
* <pre>
* PopOver popOver = new PopOver();
* popOver.getRoot().getStylesheets().add(...);
* </pre>
*
* @return the root pane
*/
public final StackPane getRoot() {
return root;
}
// Content support.
private final ObjectProperty<Node> contentNode = new SimpleObjectProperty<Node>(this, "contentNode") { //$NON-NLS-1$
@Override
public void setValue(Node node) {
if (node == null) {
throw new IllegalArgumentException("content node can not be null"); //$NON-NLS-1$
}
};
};
/**
* Returns the content shown by the pop over.
*
* @return the content node property
*/
public final ObjectProperty<Node> contentNodeProperty() {
return contentNode;
}
/**
* Returns the value of the content property
*
* @return the content node
*
* @see #contentNodeProperty()
*/
public final Node getContentNode() {
return contentNodeProperty().get();
}
/**
* Sets the value of the content property.
*
* @param content
* the new content node value
*
* @see #contentNodeProperty()
*/
public final void setContentNode(Node content) {
contentNodeProperty().set(content);
}
private InvalidationListener hideListener = new InvalidationListener() {
@Override
public void invalidated(Observable observable) {
if (!isDetached()) {
hide(D
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
oim-fx-ui 是我开发的oim聊天软件的界面,使用JavaFX开发。oim是已经实现聊天功能的完整程序,有兴趣的朋友可以去开源中国的git库下载,地址:https://git.oschina.net/onlysoftware/oim-fx,而这里就仅仅只是界面的源码,有些朋友可能只对界面感兴趣,所有我把界面提取出来了,还要当时开发的时候做了一定的解耦设计,业务代码和界面代码没多少依赖。为了让不熟悉maven的朋友能直接使用,这里我放了2个目录,一个eclipse和maven,两份源码都是一样的,只是按2种不同的项目格式分开。 项目的test目录下有可运行类,这是我自己开发的时候写的测试类,也就相当于demo了。
资源推荐
资源详情
资源评论
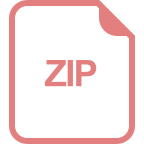
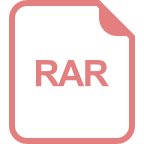
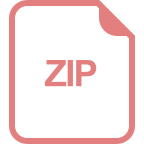
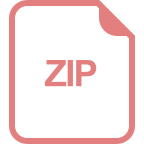
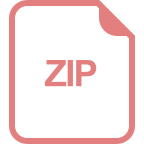
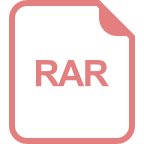
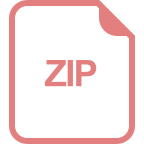
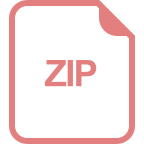
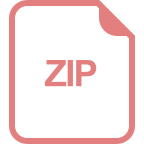
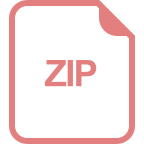
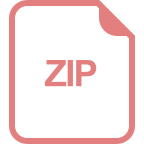
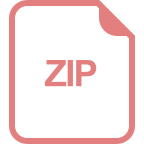
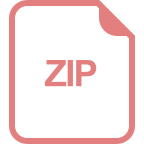
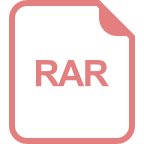
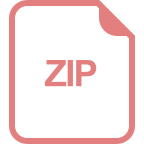
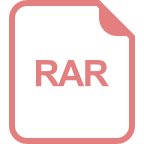
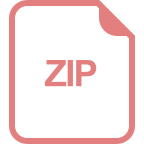
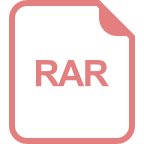
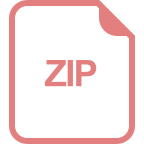
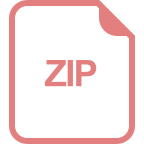
收起资源包目录

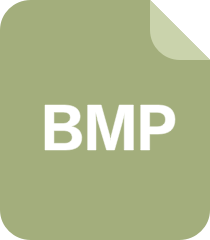
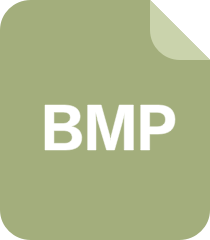
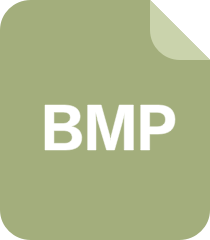
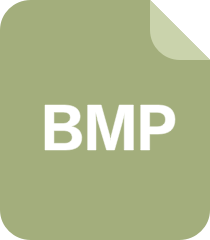
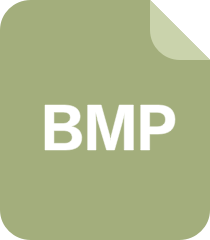
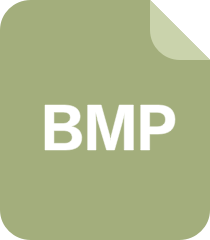
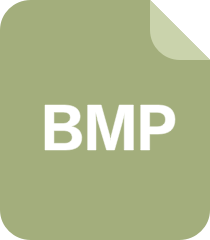
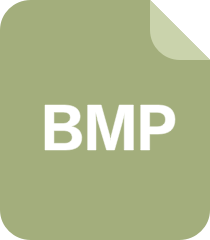
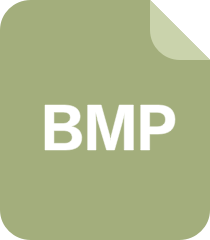
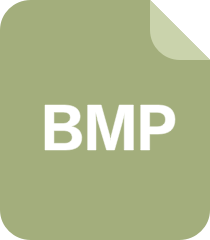
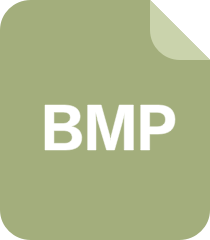
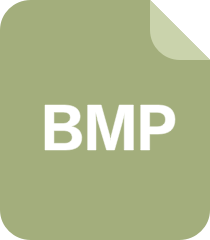
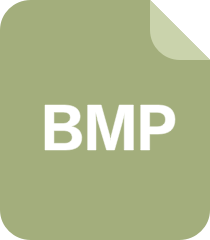
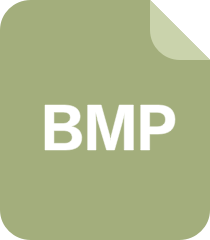
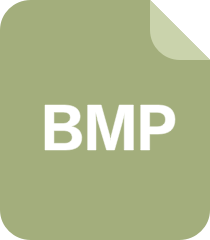
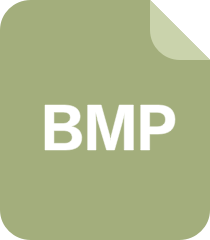
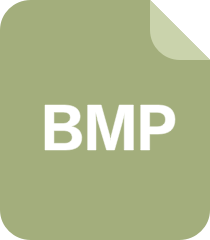
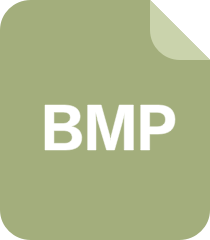
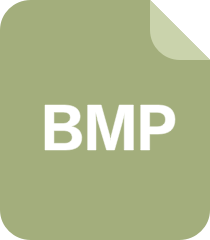
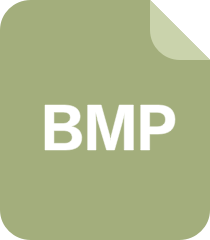
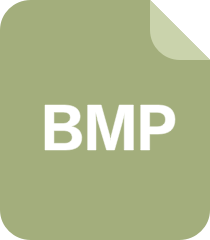
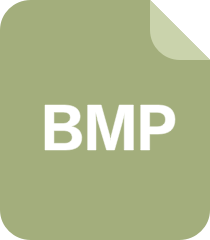
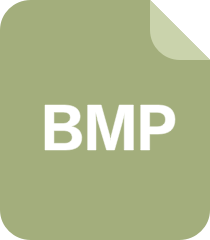
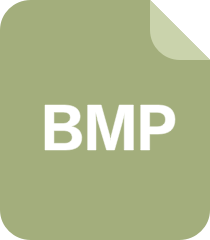
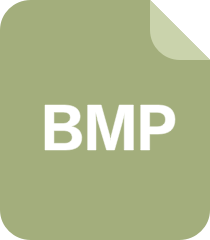
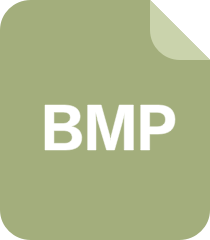
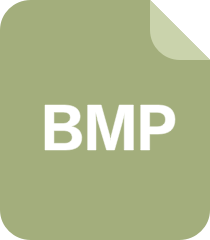
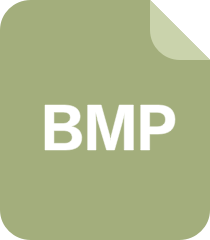
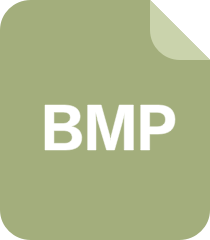
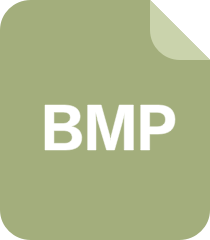
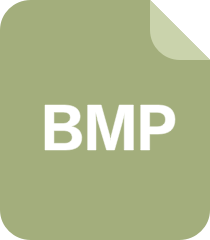
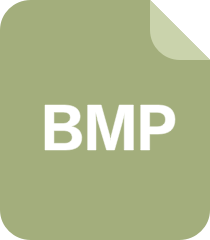
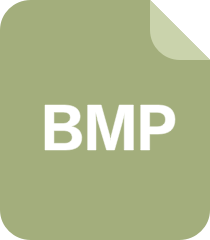
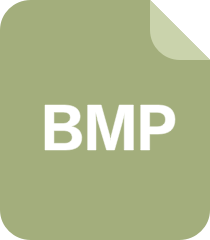
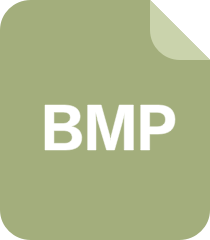
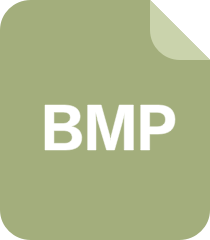
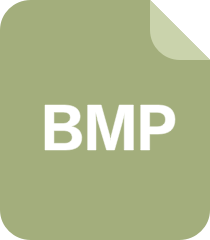
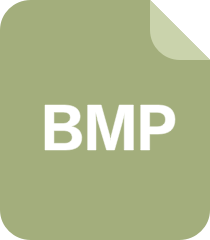
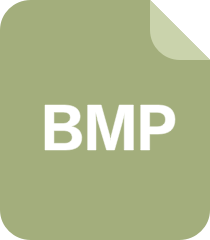
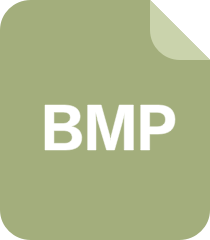
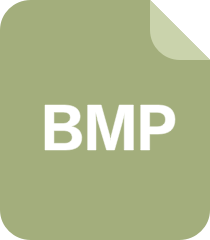
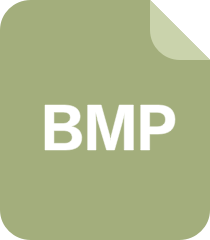
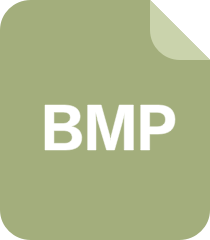
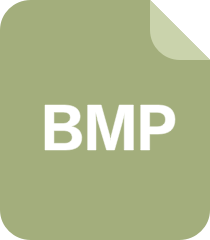
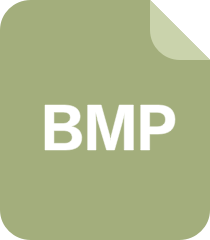
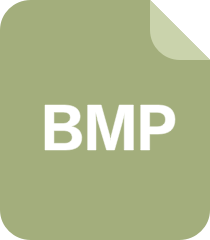
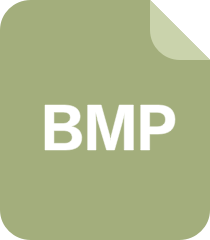
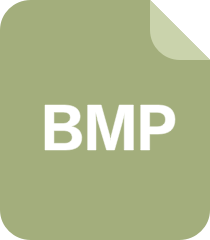
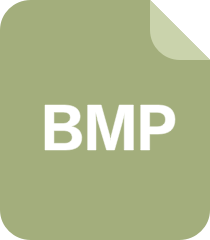
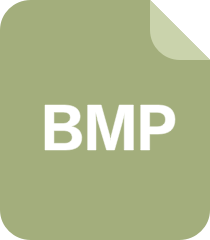
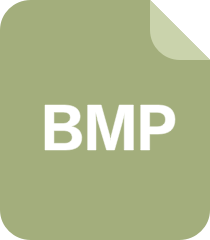
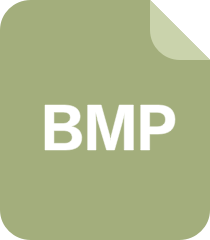
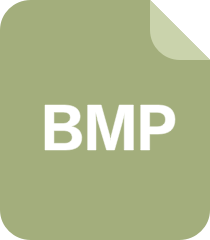
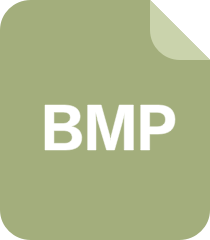
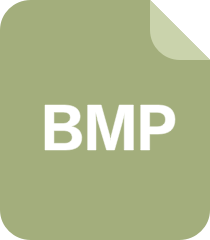
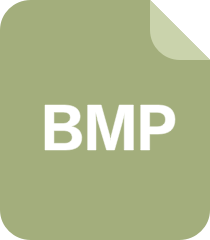
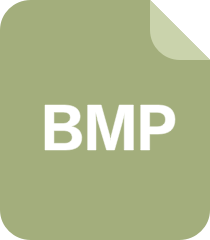
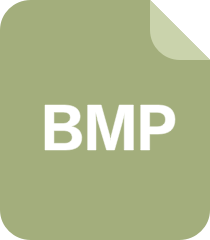
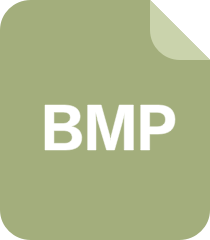
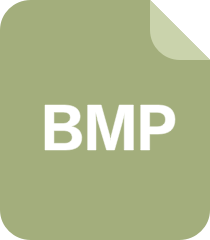
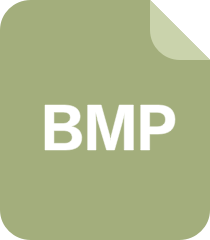
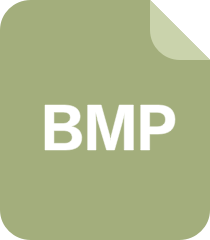
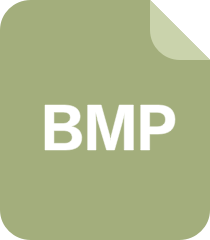
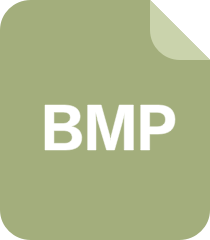
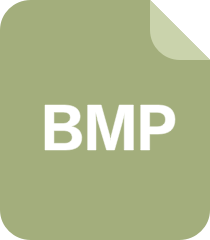
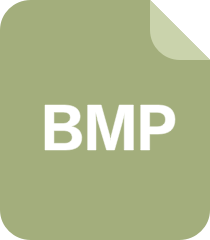
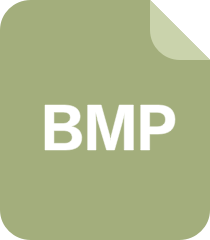
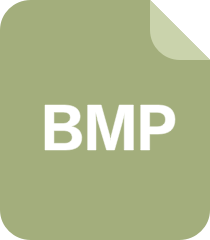
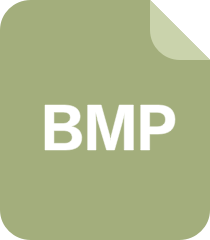
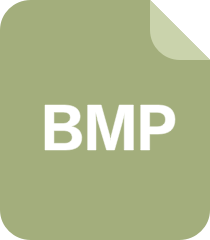
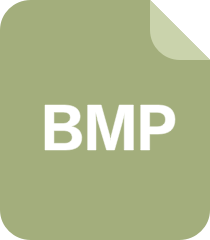
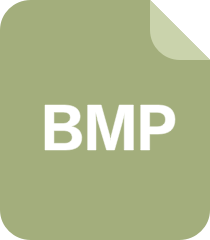
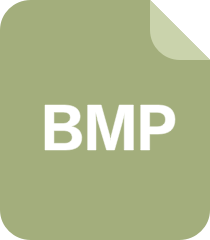
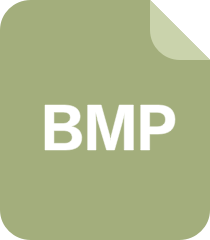
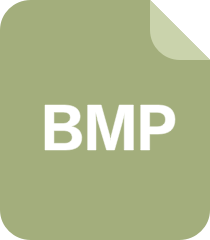
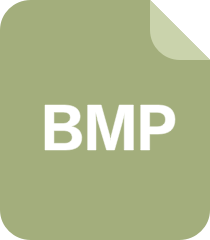
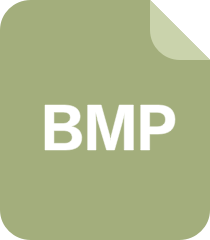
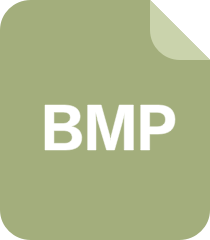
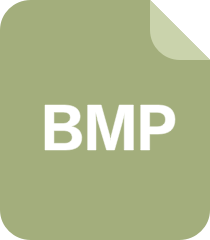
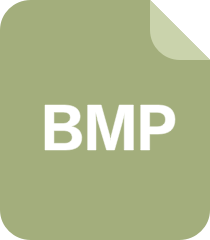
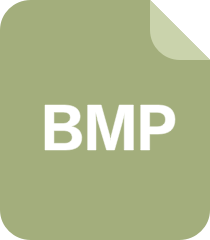
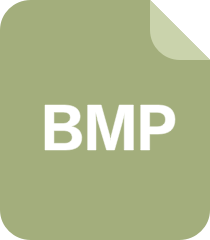
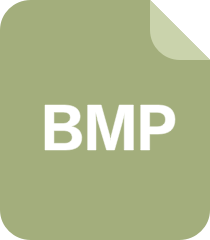
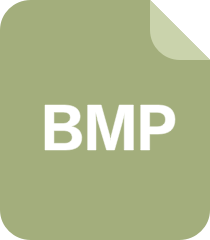
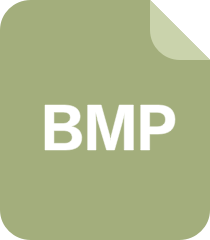
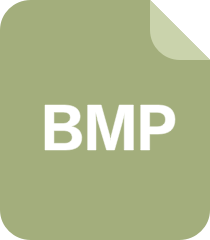
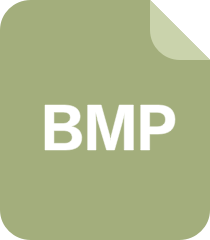
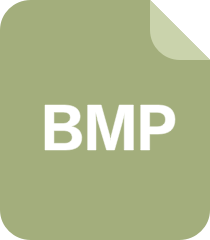
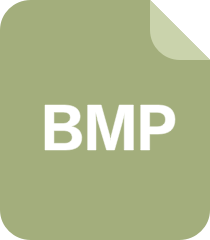
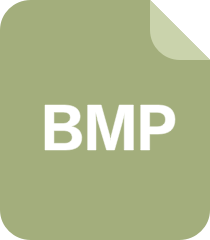
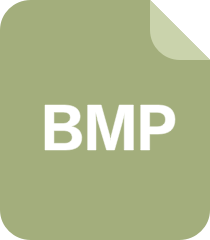
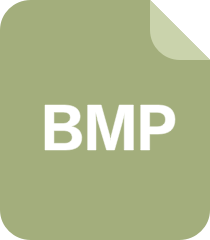
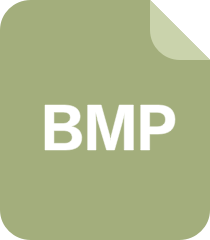
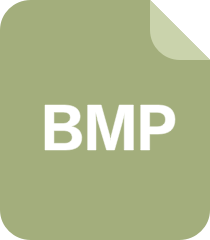
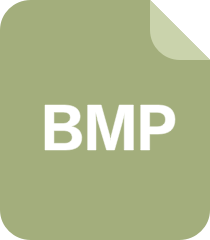
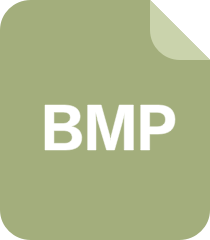
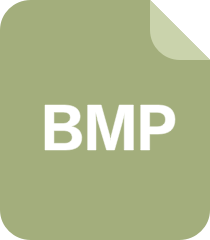
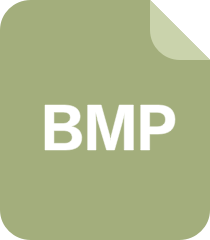
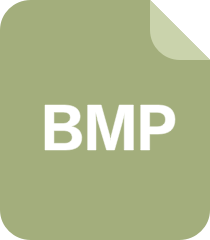
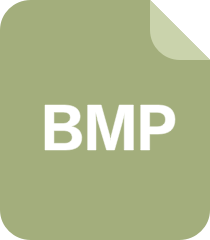
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
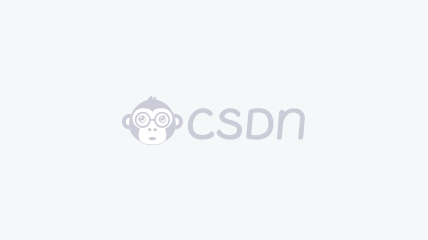
- weixin_384988132018-09-17谢谢,正在学javafx,很值得参考
- weixin_353098292018-06-19谢谢你的资源,最近课设在写IM正想美化
- 立个flag坚持写博客2018-04-14谢谢你的资源,最近课设在写IM正想美化
- MJBOBO2017-07-24资料很棒,
- h寒霜s2019-03-23挺好的,帮助很大,感谢!

烙灵
- 粉丝: 51
- 资源: 10
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

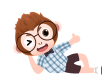
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


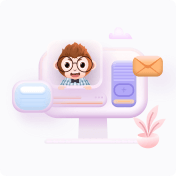
安全验证
文档复制为VIP权益,开通VIP直接复制
