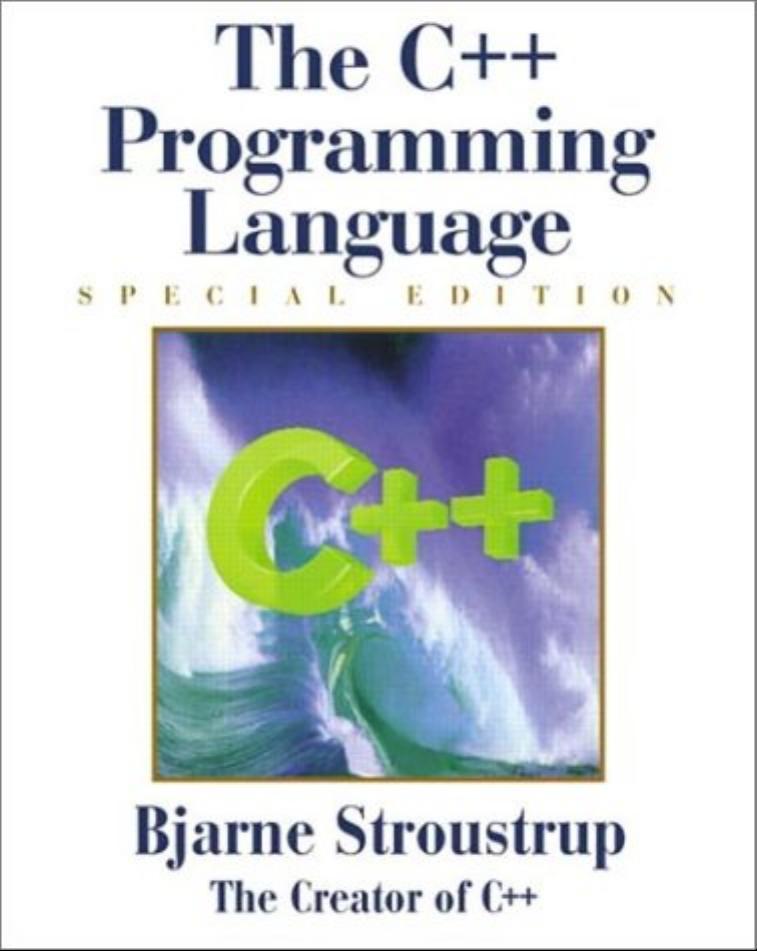
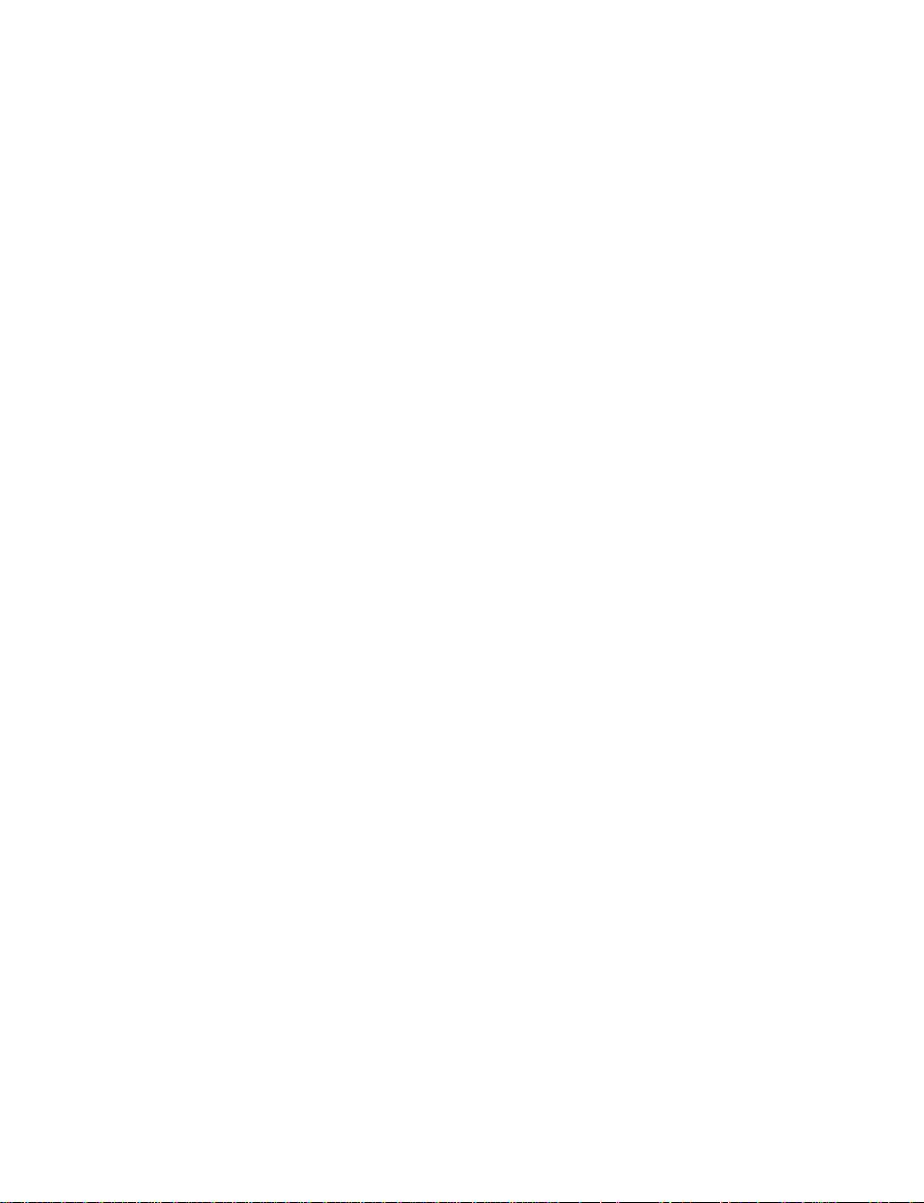
The
C
+ +
Programming
Language
Third Edition
Bjarne Stroustrup
AT&T Labs
Murray Hill, New Jersey
Addison-Wesley
An Imprint of Addison Wesley Longman, Inc.
Reading, Massachusetts
•
Harlow, England
•
Menlo Park, California
Berkeley, California
•
Don Mills, Ontario
•
Sydney
Bonn
•
Amsterdam
•
Tokyo
•
Mexico City
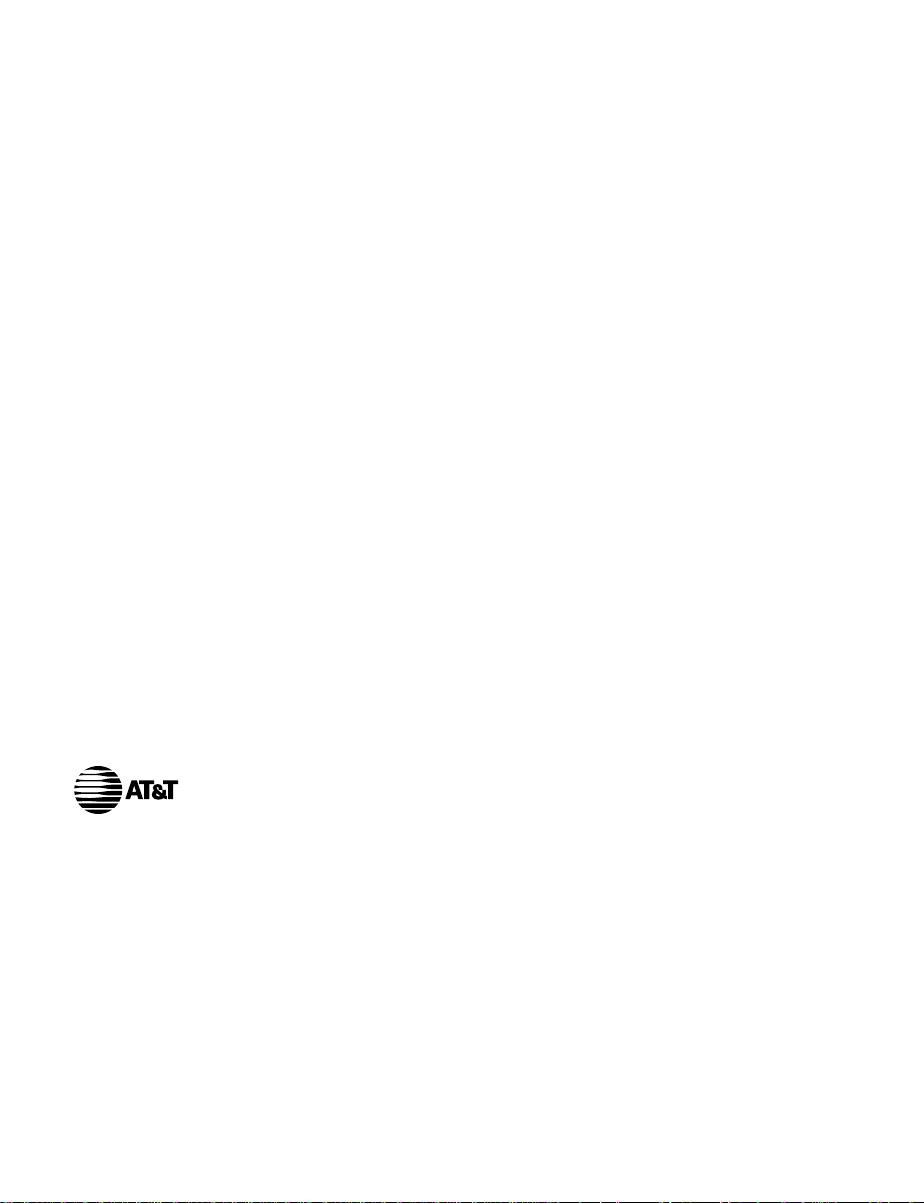
ii
Many of the designations used by manufacturers and sellers to distinguish their products are claimed as trademarks. Where
those designations appear in this book, and Addison-Wesley was aware of a trademark claim, the designations have been
printed in initial capital letters or all capital letters
The author and publisher have taken care in the preparation of this book, but make no expressed or implied warranty of any
kind and assume no responsibility for errors or omissions. No liability is assumed for incidental or consequential damages in
connection with or arising out of the use of the information contained herein.
The publisher offers discounts on this book when ordered in quantity for special sales. For more information please contact:
Corporate & Professional Publishing Group
Addison-Wesley Publishing Company
One Jacob Way
Reading, Massachusetts 01867
Library of Congress Cataloging-in-Publication Data
Stroustrup, Bjarne
The C
++
Programming Language / Bjarne Stroustrup. — 3rd. ed.
p. cm.
Includes index.
ISBN 0-201-88954-4
1. C
++
(Computer Programming Language) I. Title
QA76.73.C153S77 1997 97-20239
005.13’3—dc21 CIP
Copyright © 1997 by AT&T
All rights reserved. No part of this publication may be reproduced, stored in a retrieval system, or transmitted, in any form or
by any means, electronic, mechanical, photocopying, recording, or otherwise, without the prior written permission of the
publisher. Printed in the United States of America.
This book was typeset in Times and Courier by the author.
ISBN 0-201-88954-4
Printed on recycled paper
1 2 3 4 5 6 7 8 9—CRW—0100999897
First printing, June 1997
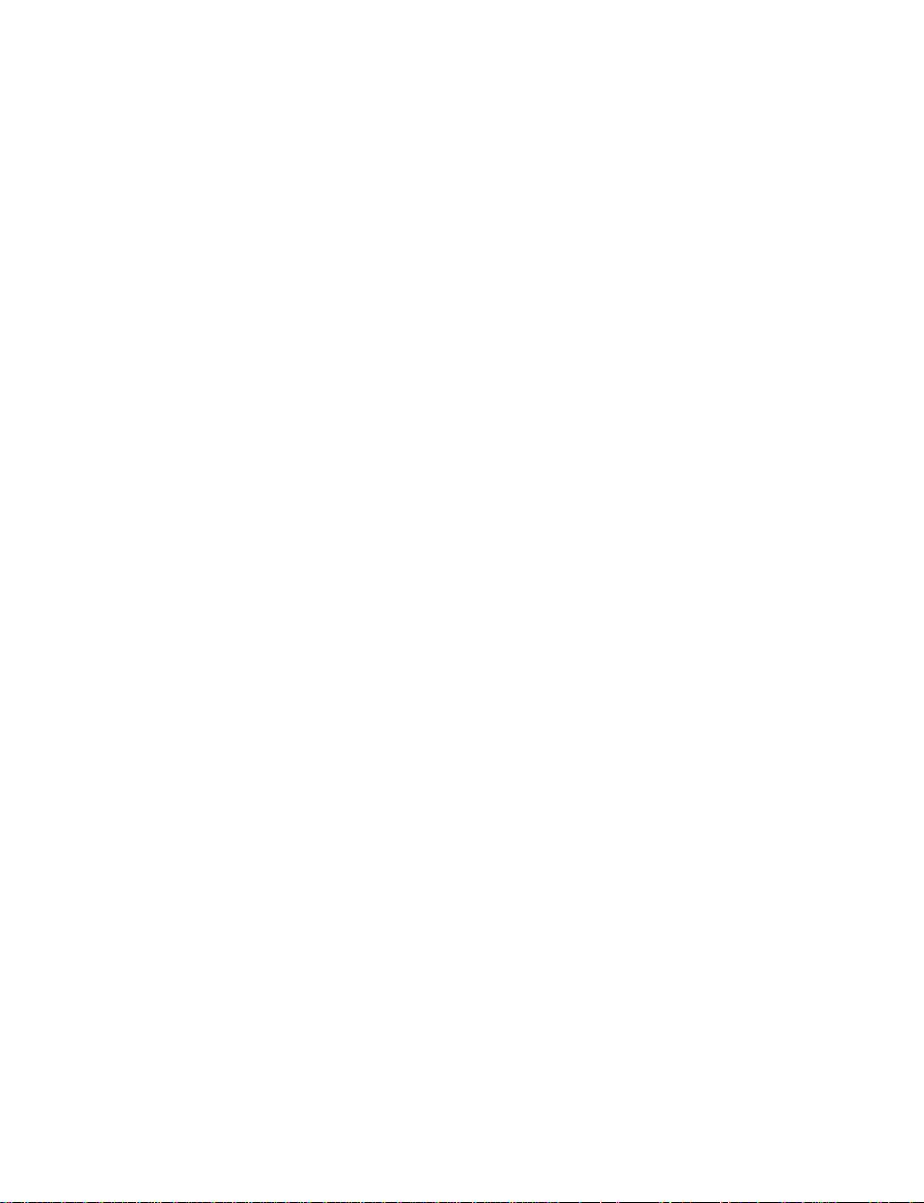
Contents
Contents iii
Preface v
Preface to Second Edition vii
Preface to First Edition ix
Introductory Material 1
1 Notes to the Reader ..................................................................... 3
2 A Tour of C
++
............................................................................. 21
3 A Tour of the Standard Library .................................................. 45
Part I: Basic Facilities 67
4 Types and Declarations ............................................................... 69
5 Pointers, Arrays, and Structures .................................................. 87
6 Expressions and Statements ........................................................ 107
7 Functions ..................................................................................... 143
8 Namespaces and Exceptions ....................................................... 165
9 Source Files and Programs .......................................................... 197
The C++ Programming Language, Third Edition by Bjarne Stroustrup. Copyright ©1997 by AT&T.
Published by Addison Wesley Longman, Inc. ISBN 0-201-88954-4. All rights reserved.
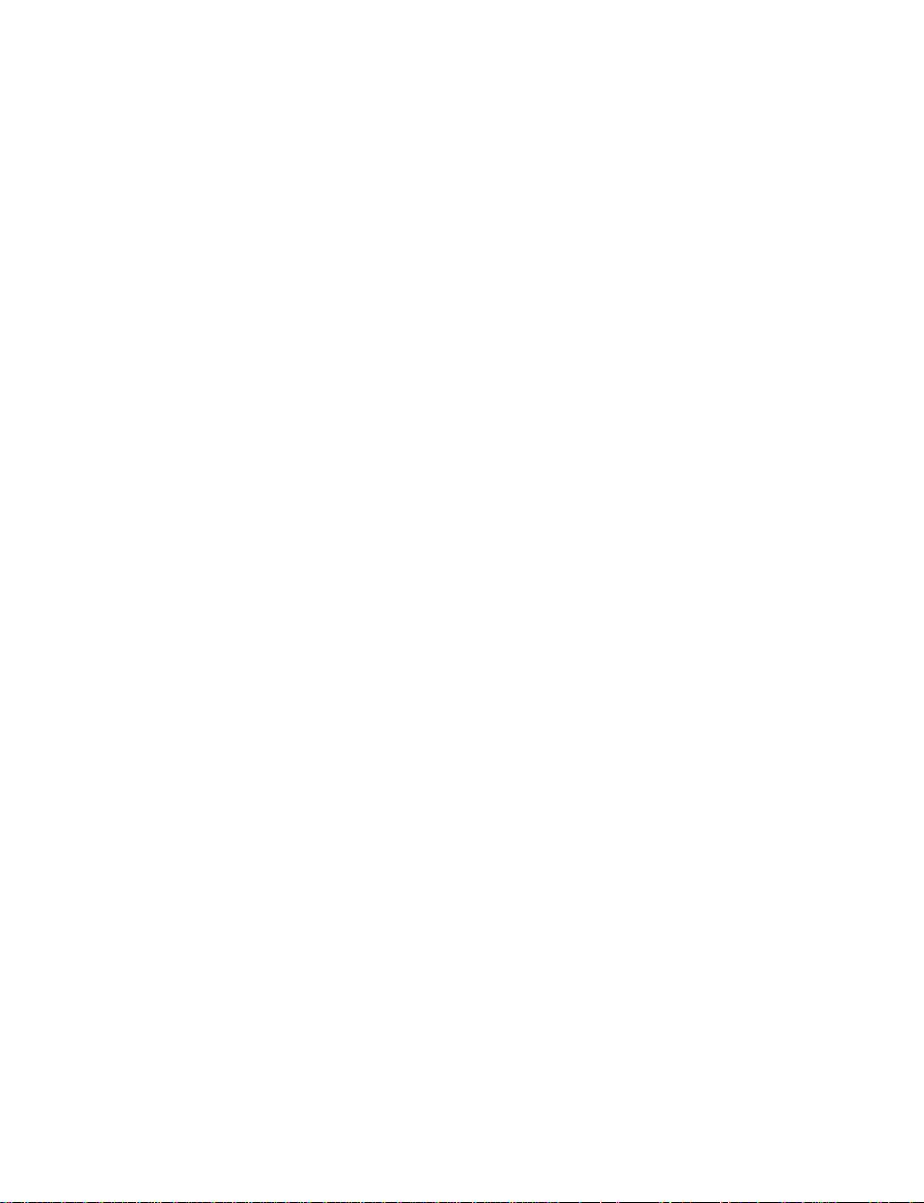
iv Contents
Part II: Abstraction Mechanisms 221
10 Classes ........................................................................................ 223
11 Operator Overloading ................................................................. 261
12 Derived Classes ........................................................................... 301
13 Templates .................................................................................... 327
14 Exception Handling .................................................................... 355
15 Class Hierarchies ........................................................................ 389
Part III: The Standard Library 427
16 Library Organization and Containers .......................................... 429
17 Standard Containers .................................................................... 461
18 Algorithms and Function Objects ............................................... 507
19 Iterators and Allocators ............................................................... 549
20 Strings ......................................................................................... 579
21 Streams ........................................................................................ 605
22 Numerics ..................................................................................... 657
Part IV: Design Using C
++
689
23 Development and Design ............................................................ 691
24 Design and Programming ........................................................... 723
25 Roles of Classes .......................................................................... 765
Appendices 791
A The C
++
Grammar ...................................................................... 793
B Compatibility .............................................................................. 815
C Technicalities .............................................................................. 827
Index 869
The C++ Programming Language, Third Edition by Bjarne Stroustrup. Copyright ©1997 by AT&T.
Published by Addison Wesley Longman, Inc. ISBN 0-201-88954-4. All rights reserved.