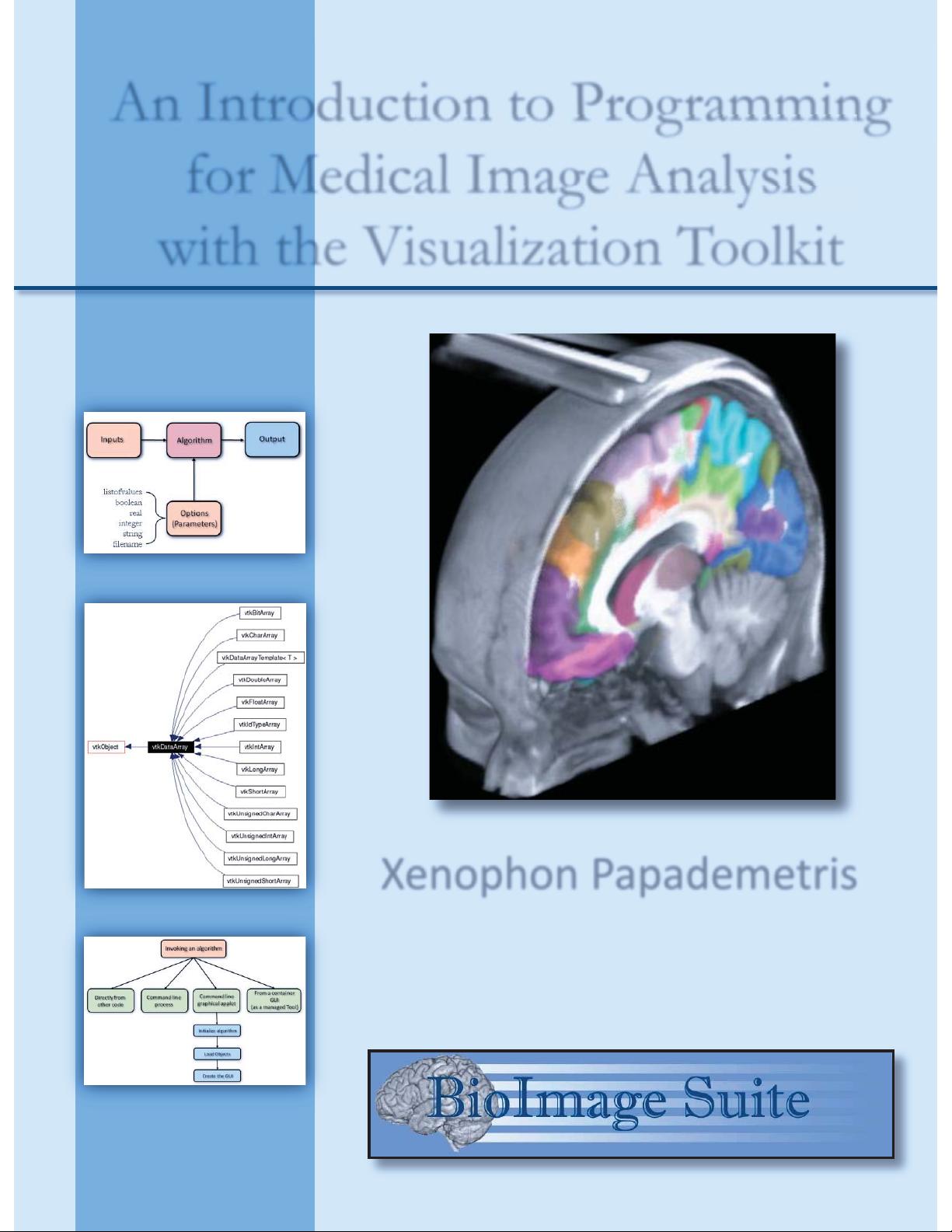
Xenophon Papademetris
An Introduction to Programming
for Medical Image Analysis
with the Visualization Toolkit
A Programming Guide for
the BioImage Suite Project
www.bioimagesuite.org
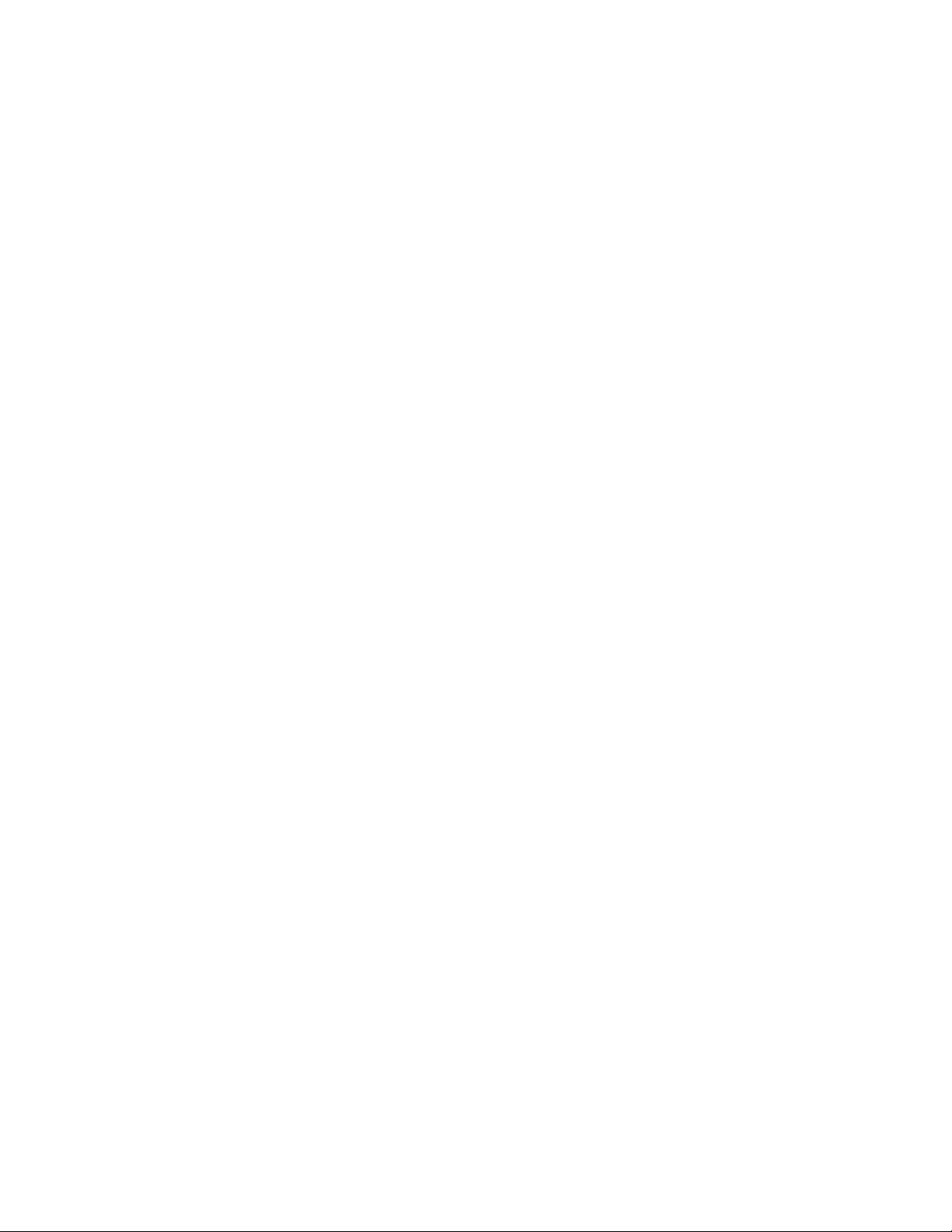
c
Copyright 2006 by Xenophon Papademetris
All Rights Reserved
This book is an edited collection of class handouts that was written for the graduate seminar
“Programming for Medical Image Analysis” (E NAS 920a). This class was taught at Yale University,
Department of Biomedical Engineering, in the Fall of 2006. Some the comments in this draft version
of the book reflect this fact. For example, see comments beginning “at Yale”. Also the word “session”
is often used interchangeably with the work “chapter”, this is also a leftover from the class handouts.
Eventually, such comments will be corrected or removed from the text. Furthermore, many of the
references that will appear in the final version are still omitted. It is made available at this stage in the
hope that it will be useful.
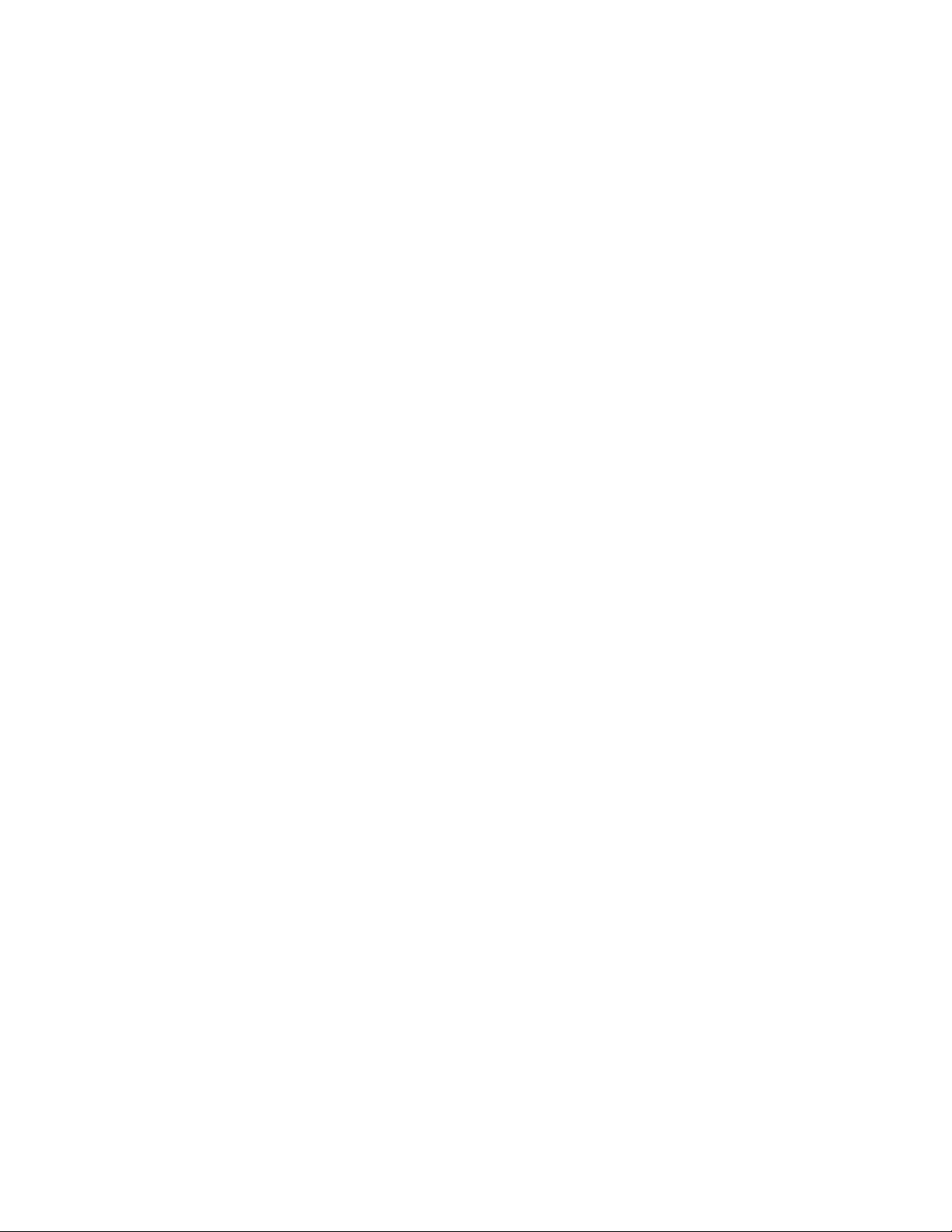
Draft December 13, 2006
Contents
Preface 5
I Introduction 7
1. Introduction 8
2. Revision Control With Subversion 13
II Programming with Tcl/Tk 18
3. Introduction to Tcl 19
4. Advanced Topics in Tcl 27
5. An Introduction To Tk 35
6. Tk Part II 43
7. Object Oriented Programming with [ Incr ] Tcl 53
8. Iwidgets: Object Oriented GUIs 63
III The Visualization Toolkit I – Using Tcl 70
9. An Introduction to the Visualization Toolkit 71
10.Curves and Surfaces in VTK 76
11.Images in VTK 86
12.Displaying Images in VTK 93
13.Transformations 105
14.Some Additional VTK Classes 114
3
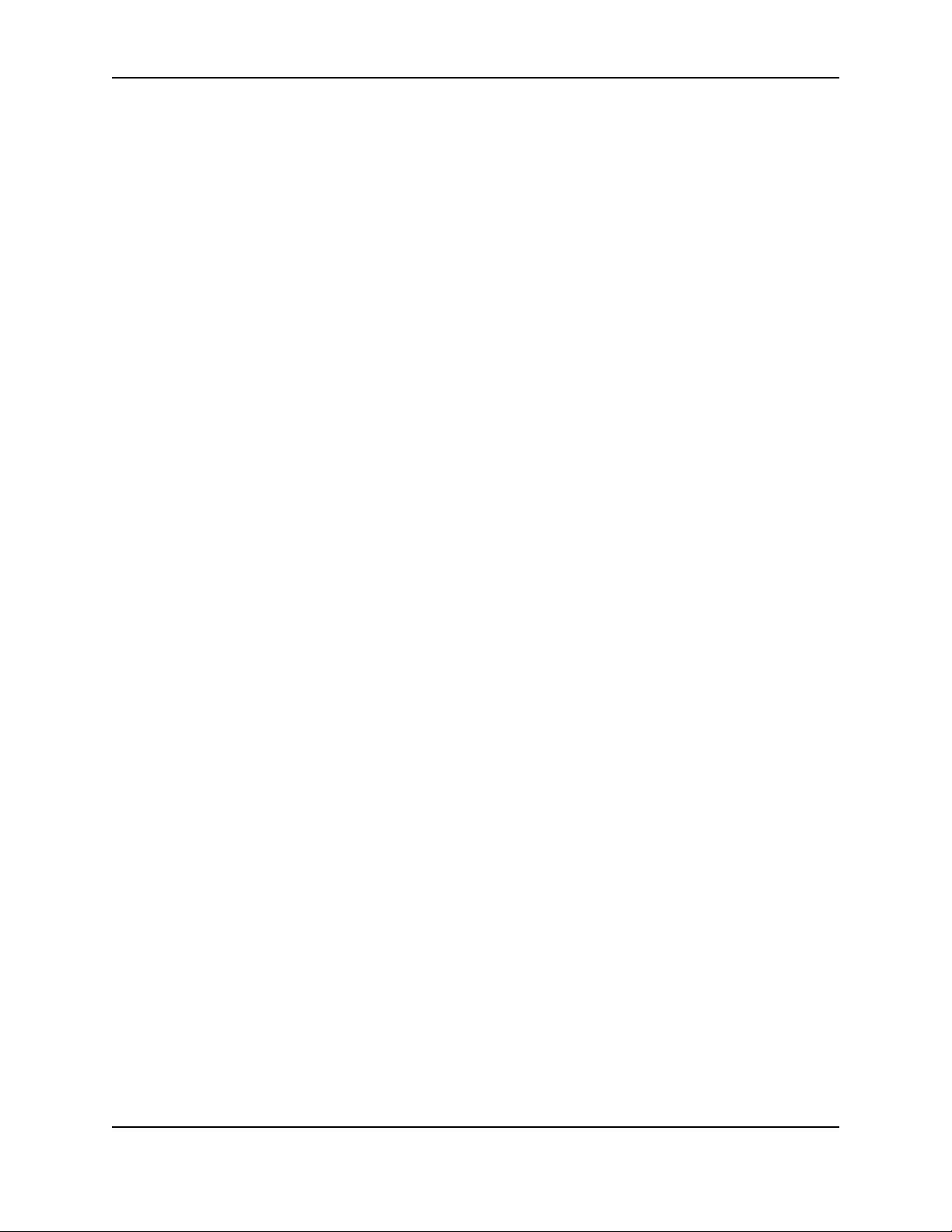
CONTENTS Draft December 13, 2006
IV Interfacing To BioImage Suite using Tcl 121
15.Leveraging BioImage Suite Components 122
16.Writing your own BioImage Suite Application 132
V C++ Techniques 146
17.Cross-Platform Compiling with CMAKE 147
18.C++ Techniques and VTK 154
VI VTK Programming with C++ and Tcl 166
19.Extending VTK using C++ 167
20.Point-based Registration with ICP 179
21.Intensity Based Segmentation 197
22.A Templated Image to Image Filter 215
23.Copying Data Objects 222
24.The Insight Toolkit 226
VII Appendices 234
A. Final Exam 235
B. Code License 236
References 237
4
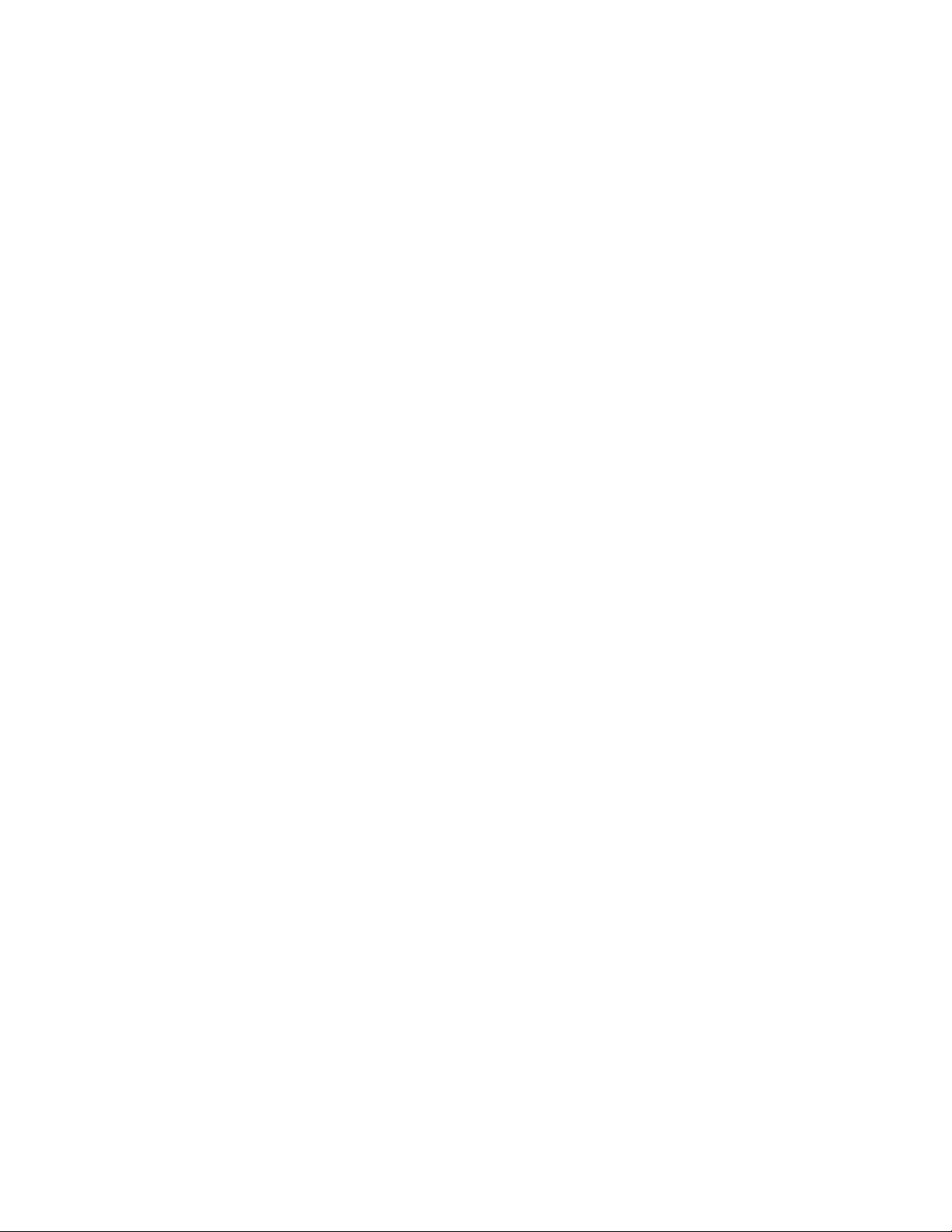
Draft December 13, 2006
Preface
This book is an edited collection of c lass handouts that I wrote for the graduate seminar “Programming
for Medical Image Analysis” (ENAS 920a) that was t aught at Yale University, Department of Biomedical
Engineering, in the Fall of 2006. My goal for the class was to provide sufficient introductory material for
a typical 1st year engineering graduate student with some background in programming in C and C++
to acquire the skills to leverage modern open source t oolkits in medical image analysis and visualization
such as the Visualization Toolkit (VTK)[24] and, to a lesser extent, the Insight Toolkit (ITK)[12].
One obviously has to acknowledge that there are many programming books out there that cover the
material discussed in this book in more depth (and more correctly perhaps); I list many of my sources
in Chapter 1. However, placed one on top of the other, these books form a pile ab out 1- 2 feet high,
which can be discouraging to a beginner. This book is meant to be an introductory guide. Many of the
definitions are informal, much like one teaches math at 1st grade. As the student progresses and begins
to understand the introductory material, then he/she should look at more specialized books which offer
a deeper insight into what is going on. The manual pages for many of these toolkits also bec ome useful
at this point.
Most of our graduate students – the intended audience for this book and class – while having a strong
applied mathematics/signal processing background, are not expert programmers. Frequently, they would
have had some programming classes at the undergraduate level and would have been, most likely, exposed
to C/C++ at some point. However, with rare exceptions, a dive into the combination of object-oriented
and generic programming model used in ITK, for instance, would leave most of them befuddled.
Such students begin their graduate research in semester long projects called “special investigations”.
This is part of the proce ss of identifying a topic for their research as well as a lab in which they will
pursue their dissertation work. In our own research in medical image analysis, the typical product of a
doctoral dissertation is a mathematical framework for attacking an image analysis problem which has
to be translated into computer code for te sting and validation.
Most of the students, in these special investigations, prototype ideas in MATLAB [15]. While MATLAB
is a wonderful prototyping tool, it leaves much to be desired in terms of the developme nt of the
programming habits needed to write a large, sustainable, and reusable body of code. Unfortunately,
many students ending up in the trap of developing the best algorithms that can be implem ente d in
MATLAB as opposed to focusing on what on optimal algorithmic strategy would be. This is especially
apparent once large 3D and 4D datasets enter into the picture, and their algorithms end up taking hours
and days to run.
At this point in the game, a helpful professor suggests that they should probably look to move to a
more efficient language such as C++. However, one lo ok at straight C++ without any of the additional
toolkits, makes them realize that switching to C++ is easier said than done. There are very few default
5