import java.io.*;
import java.util.Random;
import javax.microedition.lcdui.*;
import javax.microedition.media.*;
public class C extends com.nokia.mid.ui.FullCanvas implements Runnable
{
//声音相关
boolean hasSound;
boolean loadMusic=false;
boolean hasPlayer=false;
public static Player rotateMusic;
private InputStream is1;
private M app;
private Thread thread;
private Random god;
private Boss temp;
private Boss boss;
private boolean gameRunning = true;
private int mainFlag = 0;
private int bossFlag = 0;
private int gameFlag = 0;//全局的游戏计数器,只在这里被赋值
private int stageClearFlag = 0;
private final int SCREEN_W = 176; //屏幕宽
private final int SCREEN_H = 208; //屏幕高
//状态量
private final int MAIN_LOGO = 1; //LOGO
private final int MAIN_TITLE = 2; //标题
private final int MAIN_ABOUT = 3; //主菜单
private final int MAIN_GAME = 8; //游戏
private final int MAIN_HELP = 10; //帮助
private final int MAIN_RESULT = 12; //
private final int MAIN_END = 13; //结局
private final int MAIN_MAKING = 14; //读取关卡
private int hiScore = 0;
private boolean flagBossDie;
private int flagBossDieCount;
//圆弧的偏移路径
private final int CIRCLEX[] = {
10, 10, 9, 8, 7, 6, 3, 2, 0,
-2, -3, -6, -7, -8, -9, -10, -10,
-10, -9, -8, -7, -6, -3, -2, 0,
2, 3, 6, 7, 8, 9, 10, 10,
10, 9, 8, 7, 6, 3, 2 //40个
};
private final int CIRCLEY[] = {
0, -2, -3, -6, -7, -8, -9, -10, -10,
-10, -9, -8, -7, -6, -3, -2, 0,
2, 3, 6, 7, 8, 9, 10, 10,
10, 9, 8, 7, 6, 3, 2, 0,
-2, -3, -6, -7, -8, -9, -10 //40个
};
//每个boss的size
private final int BOSS_SIZEX[] =
{
0,88,179,97,247
};
private final int BOSS_SIZEY[] = {
0,71,60,126,127
};
//boss的有效被攻击size
private final int BOSS_D_SIZEX[] = {
0,27,89,16,123
};
private final int BOSS_D_SIZEY[] = {
0,27,13,30,3
};
private static Image title;
private static Image arrow;
private static Image menu;
private int drawPoint = 1;
private static Image imgMyShip; //玩家飞机图片
private static Image background;
private static Image lifeicon;
private static Image bombicon;
private static Image playerFront;//座机前的小飞机
private static Image playerShot;//座机1、2钟普通子弹
private static Image subShot;//座机导弹
private static Image[] jiGuang = new Image[4];
private static Image imgLazerHit;//被激光击中时的图片
private static Image superAttack_1;
private static Image superAttack_2;
private static Image superAttack_3;
private int superAttackFlag = 0;//何种超级武器
private int playerX; //玩家坐标
private int playerY;
private int playerV = 3;//座机偏移
//两颗导弹
private boolean hasSub = true;
private int [] subX = new int[2];
private int [] subY = new int[2];
private boolean playerAlive = true;
private int playerBombFlag = 0;//爆炸计时器
private int playerShow = 0;//出新座机的计数器max is 20
private int life = 3;
private int bomb = 3;
private int bombRecover = 0;//加炸弹的气
private int score = 0;
private int mudeki = 0;//无敌计时器
private int playerWeapon;//当前的武器
private int playerFireLV = 1;//活力级别、伤害级别
//子弹
private int[] playerShots = new int[30];//id
private int[] playerShotX = new int[30];//坐标
private int[] playerShotY = new int[30];
private int[] playerShotVX = new int[30];//偏移
private int[] playerShotVY = new int[30];
private boolean[] playerShotAlive = new boolean[30];//生存标志
private int playerShotType[] = new int[30];//类型
private int shotWait,subShotWait;
private int lazerLength;
private int backY;//控制背景的Y
//按键是否被按下
private boolean P_UP = false;
private boolean P_DOWN = false;
private boolean P_LEFT = false;
private boolean P_RIGHT = false;
//3种爆炸效果图片
private static Image playerDieBomb;
private static Image enemyDieBomb;
private static Image bossDieBomb;
//敌人
private static Image[] enemy = new Image[9];
private boolean enemyAlive[] = new boolean[20];//敌人是否生存
private int enemyType[] = new int[20];//类型
private int enemyX[] = new int[20]; //坐标
private int enemyY[] = new int[20];
private int enemyDirection[] = new int[20];//方向
private int enemyVX[] = new int[20];//偏移
private int enemyVY[] = new int[20];
private int enemyXSize[] = new int[20];//size
private int enemyYSize[] = new int[20];
private int enemyLife[] = new int[20];//生命值
private int enemyPoint[] = new int[20];//分值
private int hasItem[] = new int[20];
private int enemyBombFlag[] = new int[20];
private int enemyAttackFlag[] = new int[20];
//敌人子弹
private Image imgEShot[] = new Image[8];//8种子弹pic
//一屏内100个子弹的属性
private boolean EShotAlive[] = new boolean[100];//是否存活
private int EShotType[] = new int[100];//类型
private int EShotX[] = new int[100];//当前坐标
private int EShotY[] = new int[100];
private int EShotVX[] = new int[100];//偏移
private int EShotVY[] = new int[100];
private int path[] = new int[2];//路径点
//物品
private static Image imgItem;
private boolean itemAlive[] = new boolean[10];
private int itemType[] = new int[10];
private int itemX[] = new int[10];
private int itemY[] = new int[10];
private int[] itemDirection = new int[10];
private int[] itemVX = new int[10];
private int[] itemVY = new int[10];
private int[] itemMoveFlag = new int[10];
private int readCount = 0;
private int logoCount = 0;
private int makingCount = 0;
private int titleCount = 0;
private static Image net;
private static Image sp;
private static Image cp;
private int stage = 1;
private boolean stageInited = false;//当前地图场景是否已初始化
private int createEnemy[] = null;
private int mapScroll;
private boolean bossBattle = false;
private boolean bossCreated = false;
private int gameOverFlag = 0;
private boolean stageClear = false;
// private boolean soundON = false;
private boolean gameAlive = false;
boolean alowRun = true;
private boolean alowSound = true;
private int soundPause = 0;
private final int[] superMode = {0,50,50,56,56,52,54,52,54,53,0};
private int superKey = 0;
private boolean manyLife = false;//是否为无限生命
private int helpCount = 0;
public C(M m)
{
setFullScreenMode(true);
app = m; //Midlet Object
thread = null;
god = new Random(); //新的随机数
playerX = SCREEN_W/2;
playerY = SCREEN_H*4/5;
init();
try
{
is1 = getClass().getResourceAsStream("/1.mid");
rotateMusic = Manager.createPlayer(is1, "audio/midi");
}catch (MediaException ex)
{
System.out.println(ex.toString());
}catch (IOException ex)
{
System.out.println("2");
}
loadMusic=true;
thread = new Thread(this); //当前类的线程
thread.start();
}
public void init()
{
mainFlag = MAIN_LOGO;
for (int i=0; i<playerShots.length; i++)
{
playerShotVX[i] = 0;
playerShotVY[i] = -24;
}
for (int i=0; i<20; i++)
{
enemyBombFlag[i] = 99;
}
try
{
if (net == null)
net = Image.createImage("/logo1.png");
if (sp == null)
sp = Image.createImage("/logo2.png");
if (cp == null)
cp = Image.createImage("/cp.png");
}catch(Exception
《J2ME飞机游戏源码解析与学习指南》 J2ME,全称为Java 2 Micro Edition,是Java平台的一个子集,主要用于嵌入式设备和移动设备的开发,如手机游戏、应用等。本篇将围绕“j2me飞机游戏源码”这一主题,深入探讨J2ME游戏开发中的关键知识点,帮助读者理解游戏开发流程,以及如何通过源码学习编程技巧。 一、J2ME基础架构 1. MIDP(Mobile Information Device Profile):J2ME的核心组件,定义了移动设备上的应用程序接口,包括用户界面、网络通信等功能。 2. CLDC(Connected Limited Device Configuration):负责定义内存有限设备的基础运行环境,提供了Java虚拟机和基本类库。 二、游戏画面与渲染 在J2ME中,游戏画面通常由Canvas类来实现。Canvas是可绘制区域,开发者可以通过重写其`paint()`方法来绘制游戏场景。源码中的画面更新可能涉及到以下技术: 1. 更新循环:游戏的主循环,用于处理游戏逻辑、更新屏幕显示等。 2. 图形API:使用`Graphics`对象进行图形绘制,包括线条、填充、文字等。 3. 图片加载与动画:通过`Image`对象加载图片资源,结合帧动画实现飞机动态效果。 三、游戏逻辑与碰撞检测 1. 游戏对象:飞机、敌人、子弹等,通过类表示,存储位置、速度等属性。 2. 运动逻辑:通过更新每个游戏对象的位置,实现它们在屏幕上的移动。 3. 碰撞检测:检测飞机与其他物体的碰撞,如子弹击中敌人或飞机碰到障碍物,通常采用矩形碰撞检测算法。 四、用户交互与控制 1. 键盘事件:监听键盘输入,根据按键动作控制飞机的移动和射击。 2. 用户界面:创建菜单、按钮等,提供游戏开始、暂停、重新开始等功能。 五、资源管理 1. 资源加载:音频、图像等静态资源的加载和缓存。 2. 内存优化:限制内存占用,避免内存泄露,确保游戏在低内存设备上流畅运行。 六、网络通信与数据存储 1. 数据存储:可能包含用户进度、高分记录等,可以使用RecordStore进行本地数据存储。 2. 网络功能:J2ME支持HTTP、WAP等协议,实现在线排行榜、更新游戏版本等功能。 七、源码学习方法 1. 结构分析:了解项目结构,如bin目录存放编译后的类文件,src目录存放源代码,res目录存放资源文件。 2. 类分析:逐个阅读关键类,如GameCanvas、Player、Bullet等,理解它们的职责和相互关系。 3. 逻辑追踪:通过断点调试,观察游戏运行时的状态变化,理解游戏逻辑的执行过程。 4. 实践改进:尝试修改源码,增加新功能或优化现有行为,加深对J2ME游戏开发的理解。 通过以上分析,我们可以看到,"j2me飞机游戏源码"是一个极好的学习材料,涵盖了J2ME平台上的游戏开发基础,包括图形渲染、游戏逻辑、用户交互等多个方面。通过深入研究和实践,不仅能够掌握J2ME游戏开发的基本技能,也能培养解决问题和创新设计的能力。
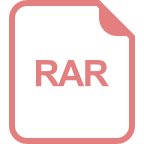
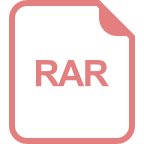
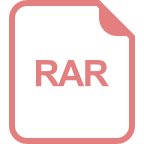
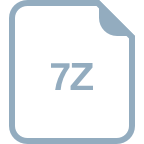
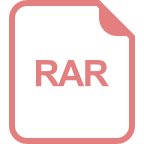
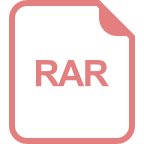
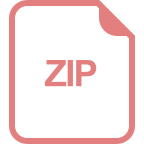
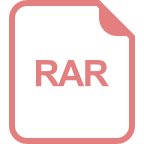
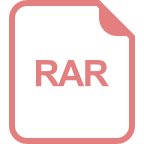
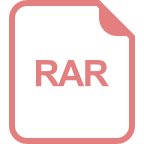
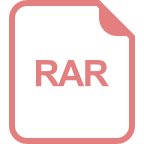
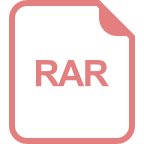
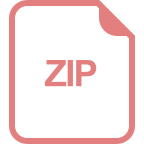
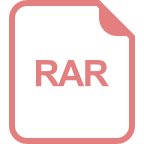
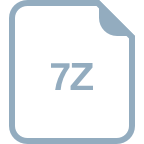


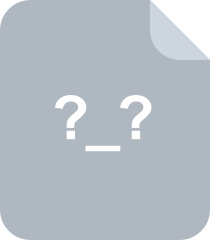
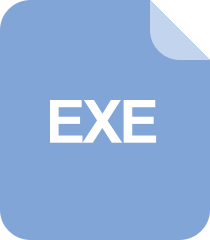
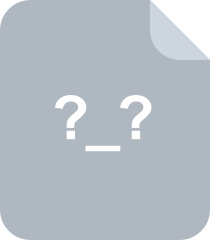

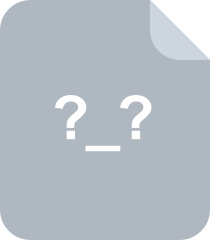
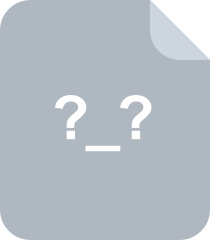

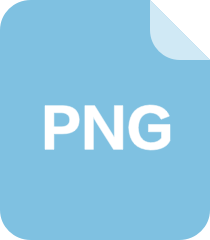
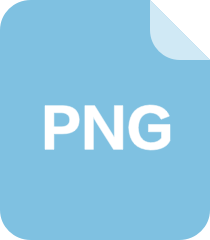
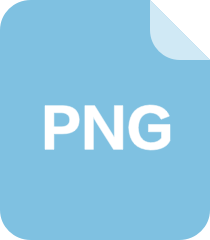
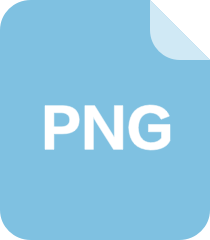
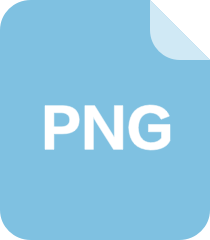
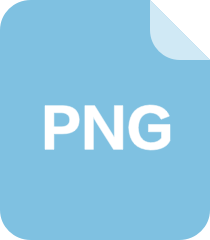
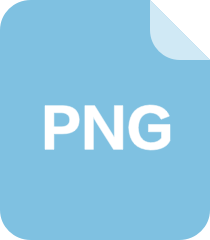
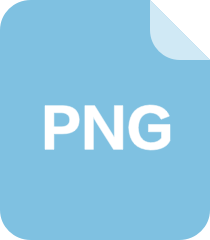
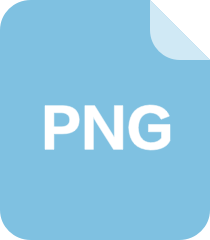
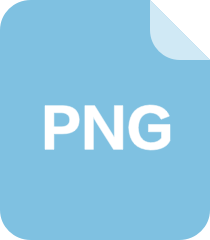
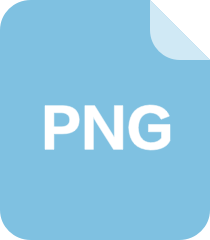
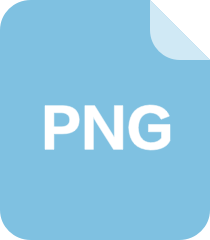
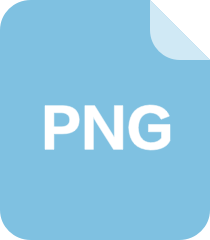
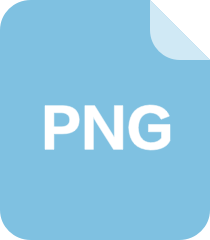
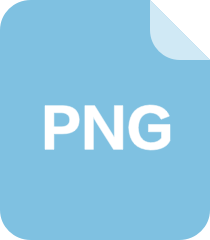
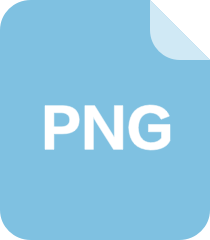
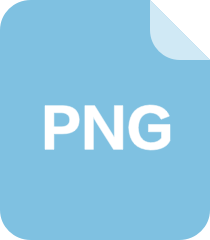
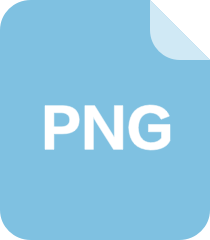
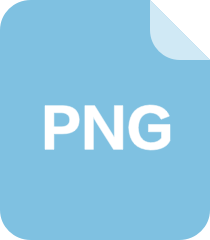
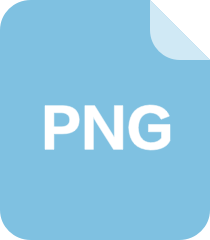
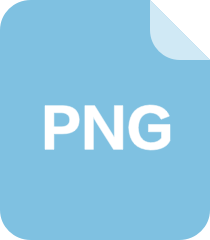
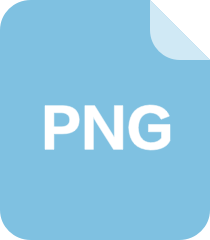
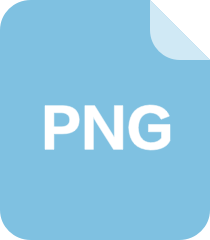
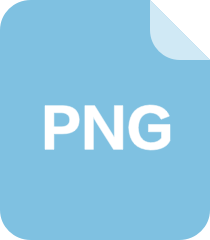
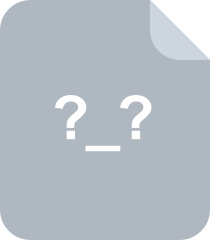
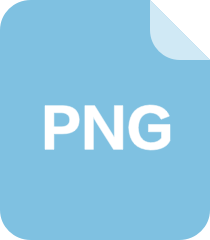
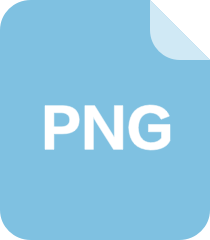
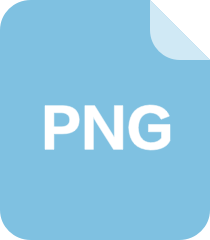
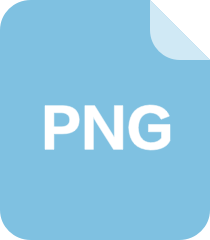
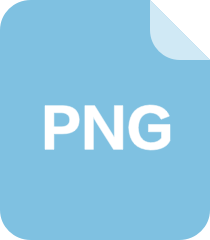
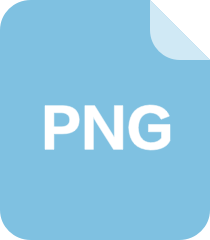
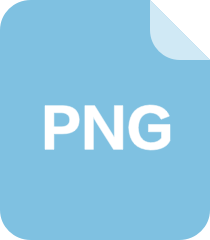
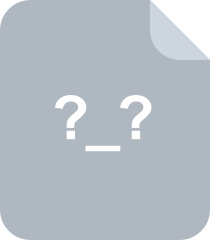
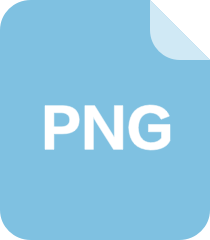
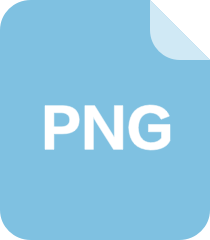
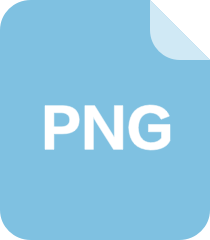
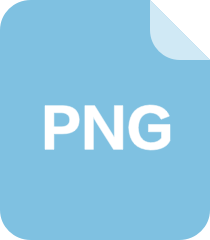
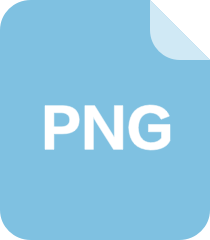
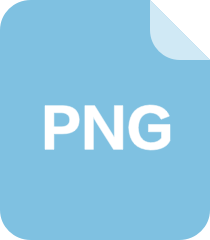
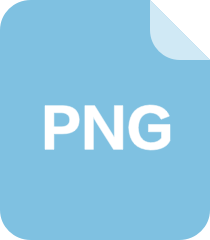
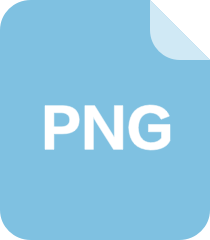
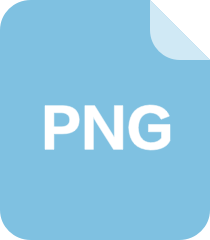
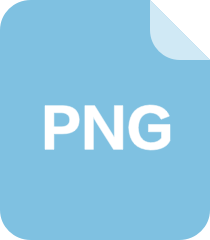
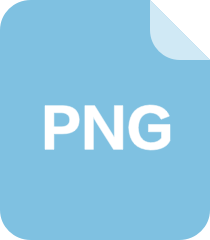
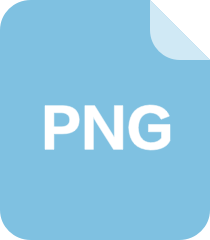
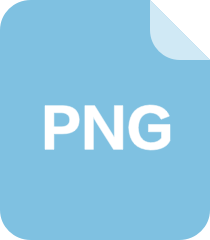
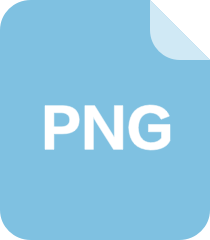
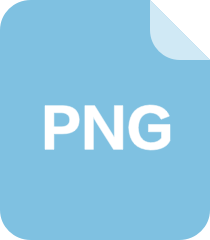
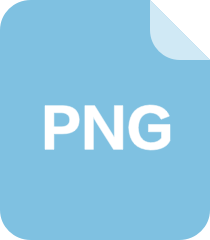
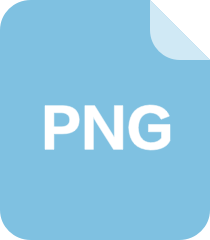
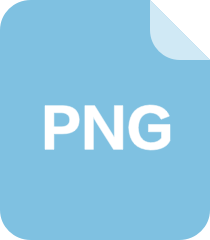
- 1
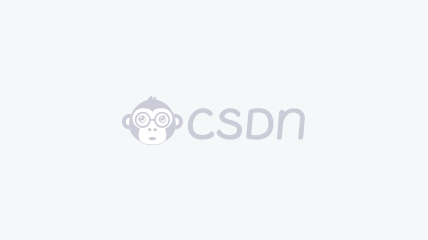
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整
- ytsmtxxi2016-04-26值得参考借鉴,共享学习
- tsh912011-12-25eclipse好像没法运行出来,找不到com.nokia.mid.ui.FullCanvas
- Mr_Xu2012-07-20没跑起来,我想改成android的,可以借鉴

- 粉丝: 44
- 资源: 47
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

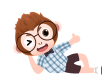
最新资源

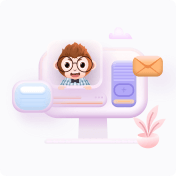
