package com.swtdesigner;
import java.io.BufferedInputStream;
import java.io.FileInputStream;
import java.io.InputStream;
import java.util.HashMap;
import java.util.Iterator;
import org.eclipse.swt.SWT;
import org.eclipse.swt.graphics.Color;
import org.eclipse.swt.graphics.Cursor;
import org.eclipse.swt.graphics.Font;
import org.eclipse.swt.graphics.FontData;
import org.eclipse.swt.graphics.GC;
import org.eclipse.swt.graphics.Image;
import org.eclipse.swt.graphics.ImageData;
import org.eclipse.swt.graphics.Point;
import org.eclipse.swt.graphics.RGB;
import org.eclipse.swt.graphics.Rectangle;
import org.eclipse.swt.widgets.Canvas;
import org.eclipse.swt.widgets.Control;
import org.eclipse.swt.widgets.CoolBar;
import org.eclipse.swt.widgets.CoolItem;
import org.eclipse.swt.widgets.Display;
/**
* Utility class for managing OS resources associated with SWT controls such as
* colors, fonts, images, etc.
*
* !!! IMPORTANT !!! Application code must explicitly invoke the <code>dispose()</code>
* method to release the operating system resources managed by cached objects
* when those objects and OS resources are no longer needed (e.g. on
* application shutdown)
*
* This class may be freely distributed as part of any application or plugin.
* <p>
* Copyright (c) 2003 - 2005, Instantiations, Inc. <br>All Rights Reserved
*
* @author scheglov_ke
* @author Dan Rubel
*/
public class SWTResourceManager {
/**
* Dispose of cached objects and their underlying OS resources. This should
* only be called when the cached objects are no longer needed (e.g. on
* application shutdown)
*/
public static void dispose() {
disposeColors();
disposeFonts();
disposeImages();
disposeCursors();
}
//////////////////////////////
// Color support
//////////////////////////////
/**
* Maps RGB values to colors
*/
private static HashMap<RGB, Color> m_ColorMap = new HashMap<RGB, Color>();
/**
* Returns the system color matching the specific ID
* @param systemColorID int The ID value for the color
* @return Color The system color matching the specific ID
*/
public static Color getColor(int systemColorID) {
Display display = Display.getCurrent();
return display.getSystemColor(systemColorID);
}
/**
* Returns a color given its red, green and blue component values
* @param r int The red component of the color
* @param g int The green component of the color
* @param b int The blue component of the color
* @return Color The color matching the given red, green and blue componet values
*/
public static Color getColor(int r, int g, int b) {
return getColor(new RGB(r, g, b));
}
/**
* Returns a color given its RGB value
* @param rgb RGB The RGB value of the color
* @return Color The color matching the RGB value
*/
public static Color getColor(RGB rgb) {
Color color = m_ColorMap.get(rgb);
if (color == null) {
Display display = Display.getCurrent();
color = new Color(display, rgb);
m_ColorMap.put(rgb, color);
}
return color;
}
/**
* Dispose of all the cached colors
*/
public static void disposeColors() {
for (Iterator<Color> iter = m_ColorMap.values().iterator(); iter.hasNext();)
iter.next().dispose();
m_ColorMap.clear();
}
//////////////////////////////
// Image support
//////////////////////////////
/**
* Maps image names to images
*/
private static HashMap<String, Image> m_ClassImageMap = new HashMap<String, Image>();
/**
* Maps images to image decorators
*/
private static HashMap<Image, HashMap<Image, Image>> m_ImageToDecoratorMap = new HashMap<Image, HashMap<Image, Image>>();
/**
* Returns an image encoded by the specified input stream
* @param is InputStream The input stream encoding the image data
* @return Image The image encoded by the specified input stream
*/
protected static Image getImage(InputStream is) {
Display display = Display.getCurrent();
ImageData data = new ImageData(is);
if (data.transparentPixel > 0)
return new Image(display, data, data.getTransparencyMask());
return new Image(display, data);
}
/**
* Returns an image stored in the file at the specified path
* @param path String The path to the image file
* @return Image The image stored in the file at the specified path
*/
public static Image getImage(String path) {
return getImage("default", path); //$NON-NLS-1$
}
/**
* Returns an image stored in the file at the specified path
* @param section The section to which belongs specified image
* @param path String The path to the image file
* @return Image The image stored in the file at the specified path
*/
public static Image getImage(String section, String path) {
String key = section + '|' + SWTResourceManager.class.getName() + '|' + path;
Image image = m_ClassImageMap.get(key);
if (image == null) {
try {
FileInputStream fis = new FileInputStream(path);
image = getImage(fis);
m_ClassImageMap.put(key, image);
fis.close();
} catch (Exception e) {
image = getMissingImage();
m_ClassImageMap.put(key, image);
}
}
return image;
}
/**
* Returns an image stored in the file at the specified path relative to the specified class
* @param clazz Class The class relative to which to find the image
* @param path String The path to the image file
* @return Image The image stored in the file at the specified path
*/
public static Image getImage(Class<?> clazz, String path) {
String key = clazz.getName() + '|' + path;
Image image = m_ClassImageMap.get(key);
if (image == null) {
try {
if (path.length() > 0 && path.charAt(0) == '/') {
String newPath = path.substring(1, path.length());
image = getImage(new BufferedInputStream(clazz.getClassLoader().getResourceAsStream(newPath)));
} else {
image = getImage(clazz.getResourceAsStream(path));
}
m_ClassImageMap.put(key, image);
} catch (Exception e) {
image = getMissingImage();
m_ClassImageMap.put(key, image);
}
}
return image;
}
private static final int MISSING_IMAGE_SIZE = 10;
private static Image getMissingImage() {
Image image = new Image(Display.getCurrent(), MISSING_IMAGE_SIZE, MISSING_IMAGE_SIZE);
//
GC gc = new GC(image);
gc.setBackground(getColor(SWT.COLOR_RED));
gc.fillRectangle(0, 0, MISSING_IMAGE_SIZE, MISSING_IMAGE_SIZE);
gc.dispose();
//
return image;
}
/**
* Style constant for placing decorator image in top left corner of base image.
*/
public static final int TOP_LEFT = 1;
/**
* Style constant for placing decorator image in top right corner of base image.
*/
public static final int TOP_RIGHT = 2;
/**
* Style constant for placing decorator image in bottom left corner of base image.
*/
public static final int BOTTOM_LEFT = 3;
/**
* Style constant for placing decorator image in bottom right corner of base image.
*/
public static final int BOTTOM_RIGHT = 4;
/**
* Returns an image composed of a base image decorated by another image
* @param baseImage Image The base image that should be decorated
* @param decorator Image The image to decorate the base image
* @return Image The resulting decorated image
*/
public static Image decorateImage(Image baseImage, Image decorator) {
return decorateImage(baseImage, decorat
没有合适的资源?快使用搜索试试~ 我知道了~
java swt 浏览器
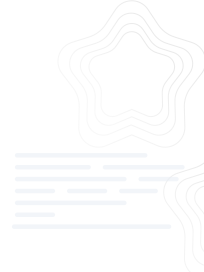
共70个文件
class:45个
java:16个
jar:4个


温馨提示
自己写的java浏览器的源代码 使用的是swt做得界面 实现了浏览器的基本功能 重点是新窗口打开的处理 鉴于有人说我是骗子,特此申明 下载里面只有源代码和引用的SWT包, classpath包括进去的,可以直接用Eclipse导入 如果需要运行,请自己编译 (导出个fatjar包要4M左右,就没放进来了)
资源推荐
资源详情
资源评论
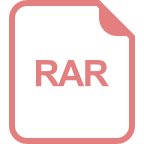
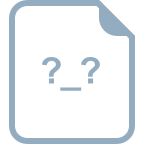
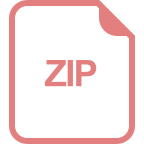
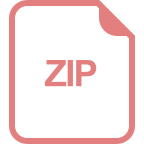
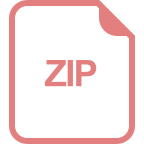
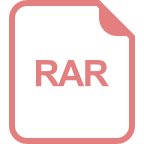
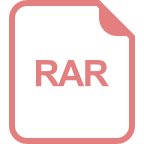
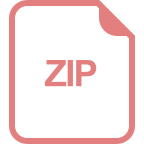
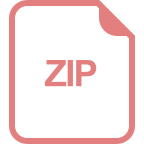
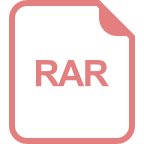
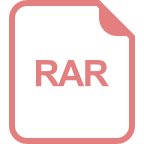
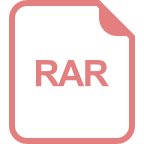
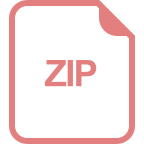
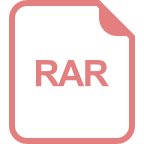
收起资源包目录


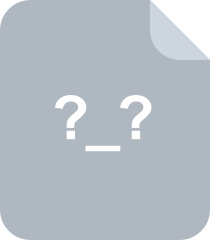
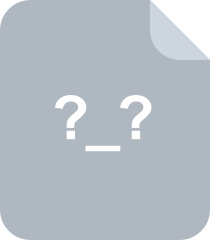



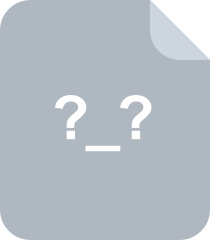
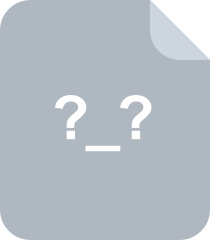


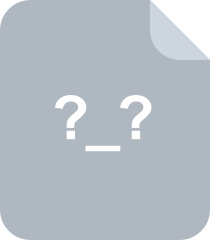
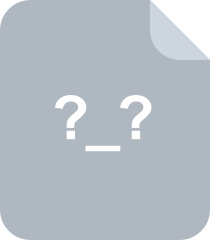


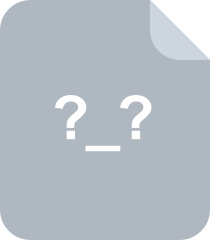
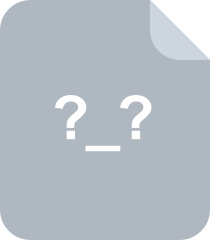
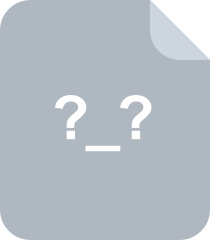
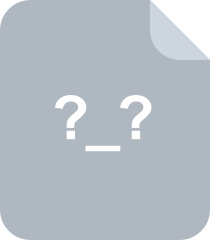
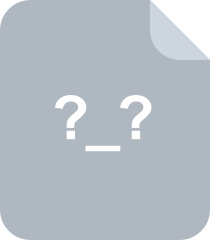
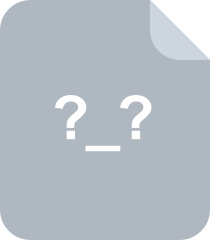
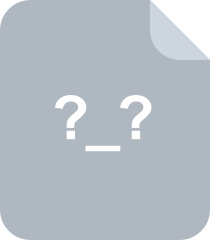
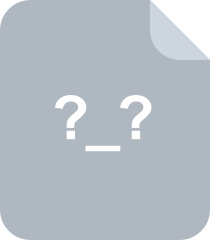

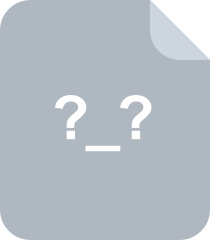
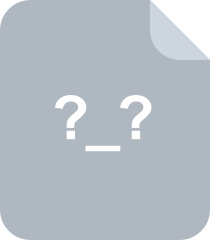
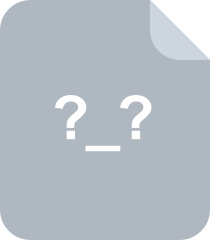
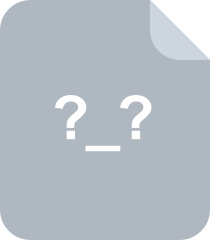
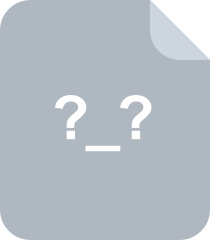
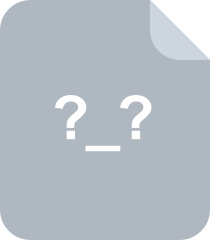
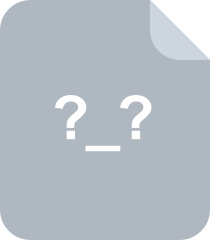
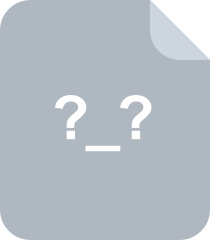
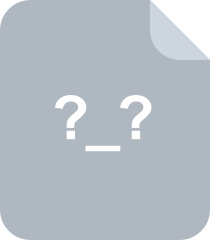
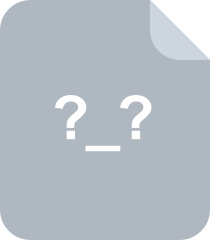
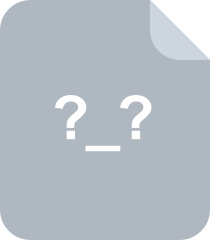
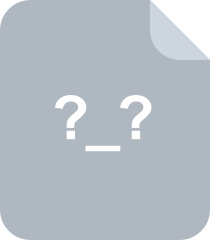
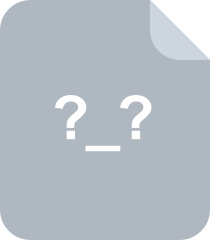
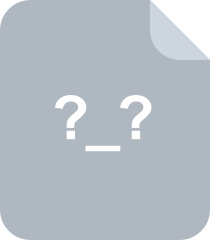
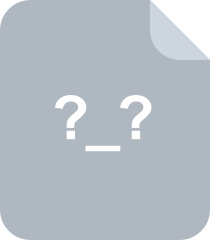
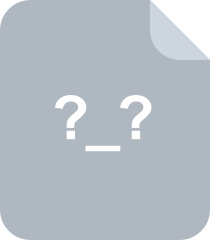
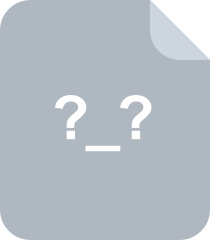
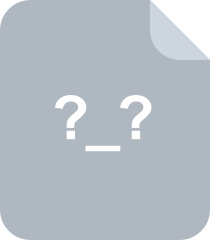
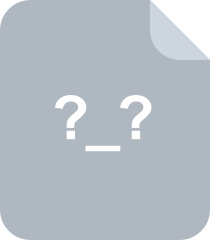
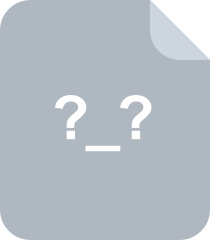
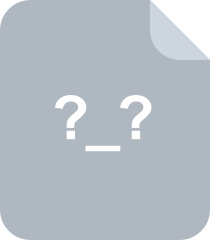
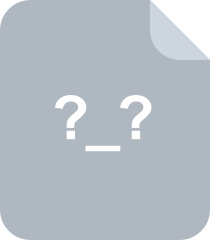
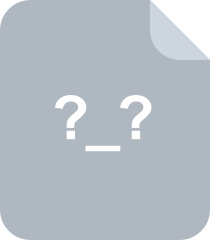
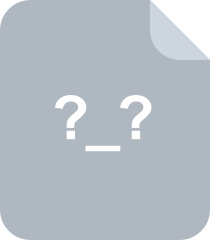
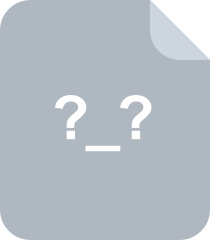
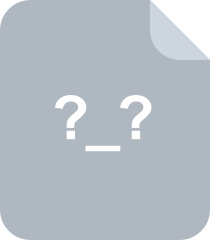
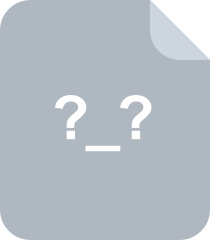
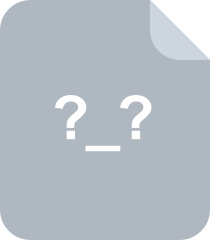
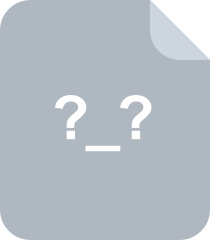
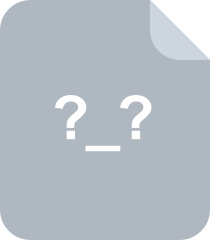
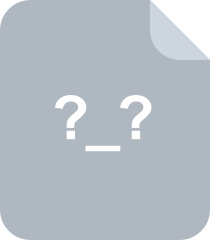
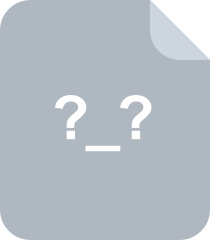
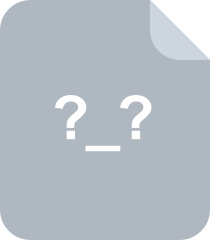



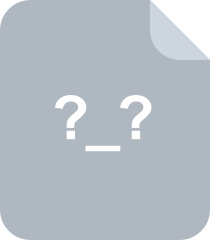


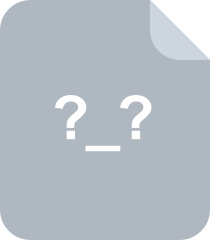
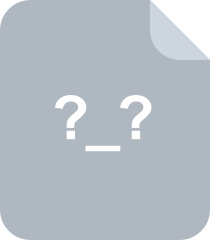


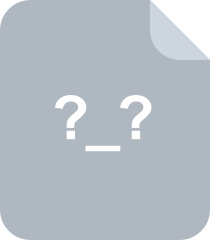
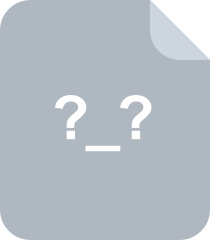
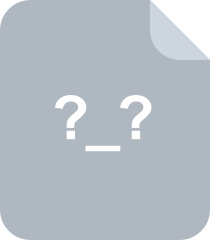
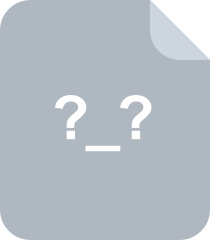
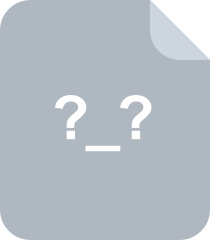
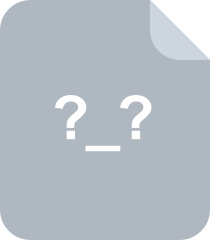
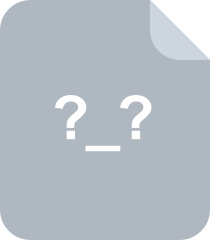
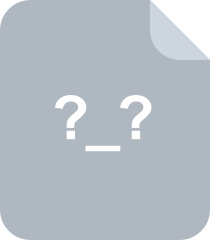

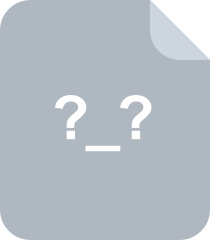
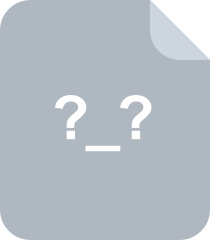
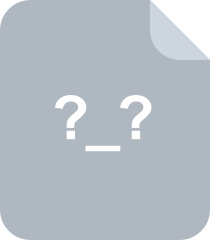
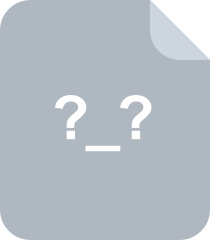
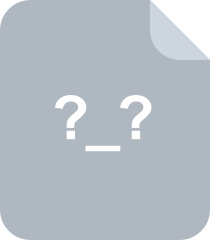
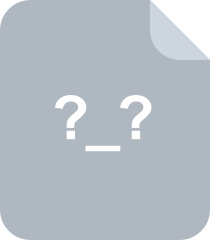
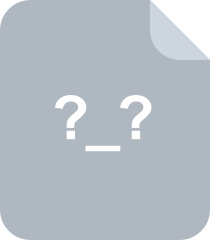
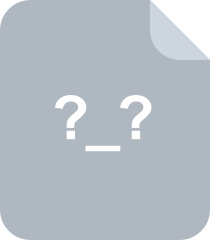

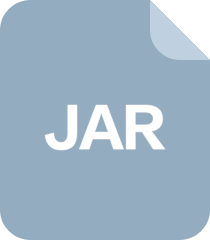
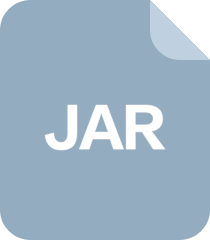
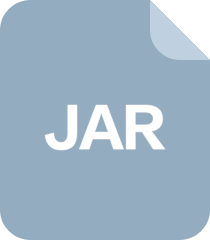
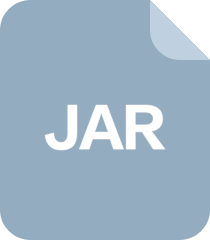
共 70 条
- 1

nevergiveup23
- 粉丝: 8
- 资源: 16
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

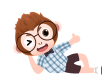
最新资源
- Java 多线程课程的代码及少量注释.zip
- 数据库课程设计-基于的个性化购物平台的建表语句.sql
- 数据库课程设计-基于的图书智能一体化管理系统的建表语句.sql
- Java 代码覆盖率库.zip
- Java 代码和算法的存储库 也为该存储库加注星标 .zip
- 免安装Windows10/Windows11系统截图工具,无需安装第三方截图工具 双击直接使用截图即可 是一款免费可靠的截图小工具哦~
- Libero Soc v11.9的安装以及证书的获取(2021新版).zip
- BouncyCastle.Cryptography.dll
- 5.1 孤立奇点(JD).ppt
- 基于51单片机的智能交通灯控制系统的设计与实现源码+报告(高分项目)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


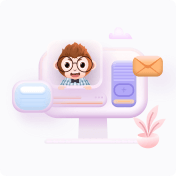
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
前往页