/*******************************************************************************
* Copyright (c) 2000, 2004 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* IBM Corporation - initial API and implementation
*******************************************************************************/
package org.eclipse.swt.snippets;
/*
* example snippet: embed Swing/AWT in SWT
*
* For a list of all SWT example snippets see
* http://www.eclipse.org/swt/snippets/
*
* @since 3.0
*/
import java.awt.EventQueue;
import java.io.*;
import java.util.*;
import javax.swing.*;
import javax.swing.table.*;
import org.eclipse.swt.*;
import org.eclipse.swt.widgets.*;
import org.eclipse.swt.graphics.*;
import org.eclipse.swt.layout.*;
import org.eclipse.swt.awt.SWT_AWT;
public class Snippet135 {
static class FileTableModel extends AbstractTableModel {
File[] files;
String[] columnsName = {"Name", "Size", "Date Modified"};
public FileTableModel (File[] files) {
this.files = files;
}
public int getColumnCount () {
return columnsName.length;
}
public Class getColumnClass (int col) {
if (col == 1) return Long.class;
if (col == 2) return Date.class;
return String.class;
}
public int getRowCount () {
return files == null ? 0 : files.length;
}
public Object getValueAt (int row, int col) {
if (col == 0) return files[row].getName();
if (col == 1) return new Long(files[row].length());
if (col == 2) return new Date(files[row].lastModified());
return "";
}
public String getColumnName (int col) {
return columnsName[col];
}
}
public static void main(String[] args) {
final Display display = new Display();
final Shell shell = new Shell(display);
shell.setText("SWT and Swing/AWT Example");
Listener exitListener = new Listener() {
public void handleEvent(Event e) {
MessageBox dialog = new MessageBox(shell, SWT.OK | SWT.CANCEL | SWT.ICON_QUESTION);
dialog.setText("Question");
dialog.setMessage("Exit?");
if (e.type == SWT.Close) e.doit = false;
if (dialog.open() != SWT.OK) return;
shell.dispose();
}
};
Listener aboutListener = new Listener() {
public void handleEvent(Event e) {
final Shell s = new Shell(shell, SWT.DIALOG_TRIM | SWT.APPLICATION_MODAL);
s.setText("About");
GridLayout layout = new GridLayout(1, false);
layout.verticalSpacing = 20;
layout.marginHeight = layout.marginWidth = 10;
s.setLayout(layout);
Label label = new Label(s, SWT.NONE);
label.setText("SWT and AWT Example.");
Button button = new Button(s, SWT.PUSH);
button.setText("OK");
GridData data = new GridData();
data.horizontalAlignment = GridData.CENTER;
button.setLayoutData(data);
button.addListener(SWT.Selection, new Listener() {
public void handleEvent(Event event) {
s.dispose();
}
});
s.pack();
Rectangle parentBounds = shell.getBounds();
Rectangle bounds = s.getBounds();
int x = parentBounds.x + (parentBounds.width - bounds.width) / 2;
int y = parentBounds.y + (parentBounds.height - bounds.height) / 2;
s.setLocation(x, y);
s.open();
while (!s.isDisposed()) {
if (!display.readAndDispatch()) display.sleep();
}
}
};
shell.addListener(SWT.Close, exitListener);
Menu mb = new Menu(shell, SWT.BAR);
MenuItem fileItem = new MenuItem(mb, SWT.CASCADE);
fileItem.setText("&File");
Menu fileMenu = new Menu(shell, SWT.DROP_DOWN);
fileItem.setMenu(fileMenu);
MenuItem exitItem = new MenuItem(fileMenu, SWT.PUSH);
exitItem.setText("&Exit\tCtrl+X");
exitItem.setAccelerator(SWT.CONTROL + 'X');
exitItem.addListener(SWT.Selection, exitListener);
MenuItem aboutItem = new MenuItem(fileMenu, SWT.PUSH);
aboutItem.setText("&About\tCtrl+A");
aboutItem.setAccelerator(SWT.CONTROL + 'A');
aboutItem.addListener(SWT.Selection, aboutListener);
shell.setMenuBar(mb);
RGB color = shell.getBackground().getRGB();
Label separator1 = new Label(shell, SWT.SEPARATOR | SWT.HORIZONTAL);
Label locationLb = new Label(shell, SWT.NONE);
locationLb.setText("Location:");
Composite locationComp = new Composite(shell, SWT.EMBEDDED);
ToolBar toolBar = new ToolBar(shell, SWT.FLAT);
ToolItem exitToolItem = new ToolItem(toolBar, SWT.PUSH);
exitToolItem.setText("&Exit");
exitToolItem.addListener(SWT.Selection, exitListener);
ToolItem aboutToolItem = new ToolItem(toolBar, SWT.PUSH);
aboutToolItem.setText("&About");
aboutToolItem.addListener(SWT.Selection, aboutListener);
Label separator2 = new Label(shell, SWT.SEPARATOR | SWT.HORIZONTAL);
final Composite comp = new Composite(shell, SWT.NONE);
final Tree fileTree = new Tree(comp, SWT.SINGLE | SWT.BORDER);
Sash sash = new Sash(comp, SWT.VERTICAL);
Composite tableComp = new Composite(comp, SWT.EMBEDDED);
Label separator3 = new Label(shell, SWT.SEPARATOR | SWT.HORIZONTAL);
Composite statusComp = new Composite(shell, SWT.EMBEDDED);
java.awt.Frame locationFrame = SWT_AWT.new_Frame(locationComp);
final java.awt.TextField locationText = new java.awt.TextField();
locationFrame.add(locationText);
java.awt.Frame fileTableFrame = SWT_AWT.new_Frame(tableComp);
java.awt.Panel panel = new java.awt.Panel(new java.awt.BorderLayout());
fileTableFrame.add(panel);
final JTable fileTable = new JTable(new FileTableModel(null));
fileTable.setDoubleBuffered(true);
fileTable.setShowGrid(false);
fileTable.createDefaultColumnsFromModel();
JScrollPane scrollPane = new JScrollPane(fileTable);
panel.add(scrollPane);
java.awt.Frame statusFrame = SWT_AWT.new_Frame(statusComp);
statusFrame.setBackground(new java.awt.Color(color.red, color.green, color.blue));
final java.awt.Label statusLabel = new java.awt.Label();
statusFrame.add(statusLabel);
statusLabel.setText("Select a file");
sash.addListener(SWT.Selection, new Listener() {
public void handleEvent(Event e) {
if (e.detail == SWT.DRAG) return;
GridData data = (GridData)fileTree.getLayoutData();
Rectangle trim = fileTree.computeTrim(0, 0, 0, 0);
data.widthHint = e.x - trim.width;
comp.layout();
}
});
File[] roots = File.listRoots();
for (int i = 0; i < roots.length; i++) {
File file = roots[i];
TreeItem treeItem = new TreeItem(fileTree, SWT.NONE);
treeItem.setText(file.getAbsolutePath());
treeItem.setData(file);
new TreeItem(treeItem, SWT.NONE);
}
fileTree.addListener(SWT.Expand, new Listener() {
public void handleEvent(Event e) {
TreeItem item = (TreeItem)e.item;
if (item == null) return;
if (item.getItemCount() == 1) {
TreeItem firstItem = item.getItems()[0];
if (firstItem.getData() != null) return;
firstItem.dispose();
} else {
return;
}
File root = (File)item.getData();
File[] files = root.listFiles();
if (files == null) return;
for (int i = 0; i < files.length; i++) {
File file = files[i];
if (file.isDirectory()) {
TreeItem treeItem = new TreeItem(item, SWT.NONE);
treeItem.setText(file.getName());
treeItem.setData(file);
new TreeItem(treeItem, SWT.NONE);
}
}
}
});
fileTree.addListener(SWT.Selection, new Listener() {
public void handleEvent(Event e) {
TreeItem item = (TreeItem)e.item;
if (item == null) return;
final File root = (File)item.getData();
EventQueue.invokeLater(new Runnable() {
public void run() {
statusLabel.setText(root.getAbsolutePath());
locationText.setText(root.getAbsolutePath(
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
java的一套GUI库,eclipse就是用这个写的。 用来做一些界面还是很不错的。 这套例子是swt官网上的小型例子,每个文件介绍一个简单的功能。 官方网址 http://www.eclipse.org/swt/snippets/ 需要的界面效果可以再官方网址上找到说明,再查看对应的java文件即可
资源推荐
资源详情
资源评论
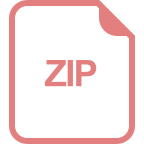
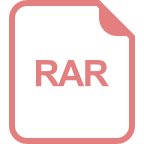
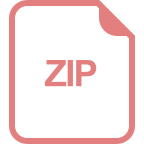
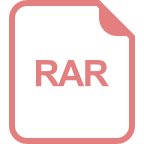
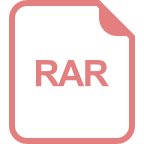
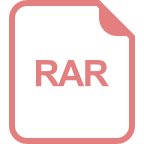
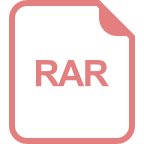
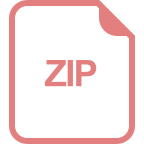
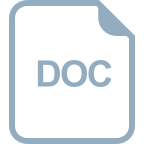
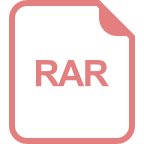
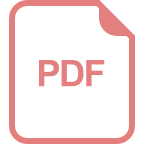
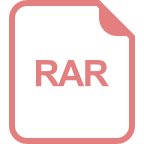
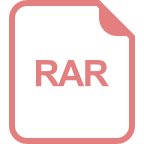
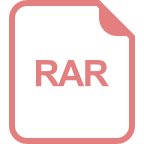
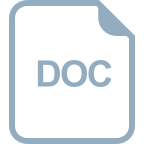
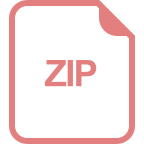
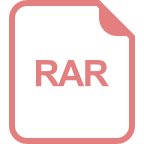
收起资源包目录

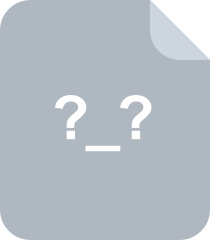
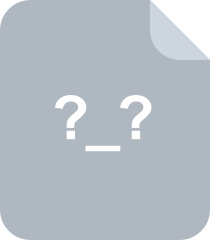
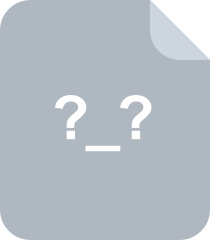
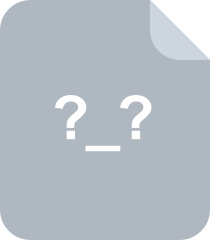
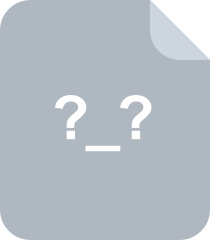
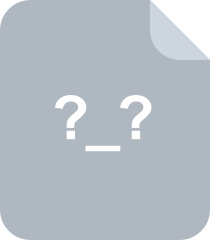
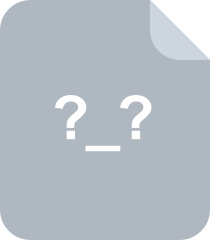
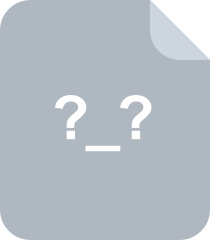
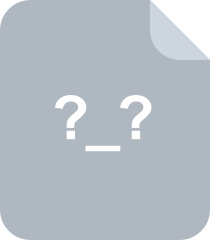
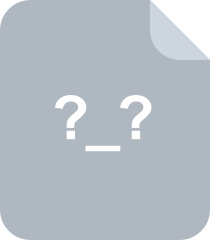
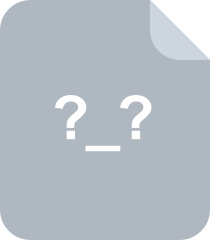
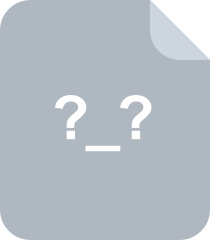
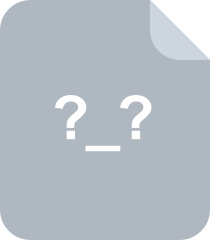
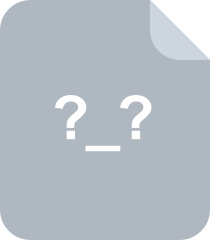
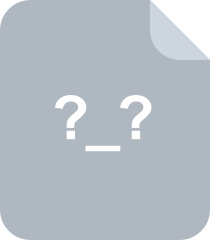
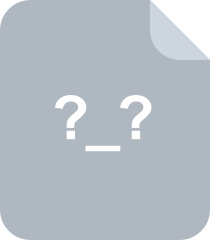
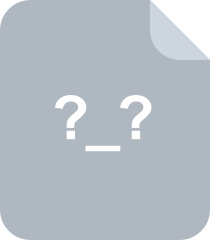
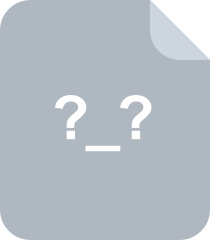
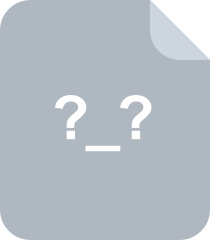
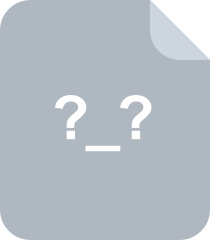
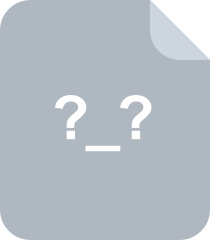
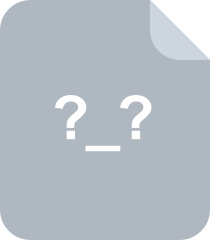
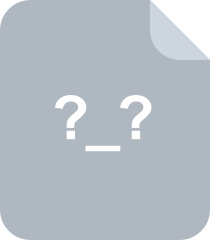
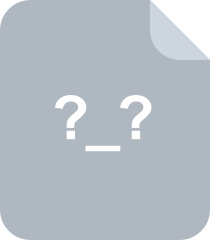
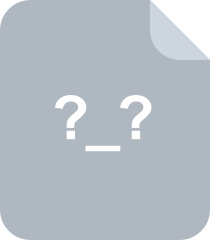
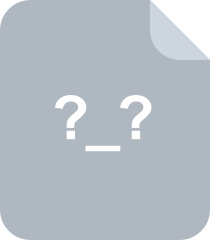
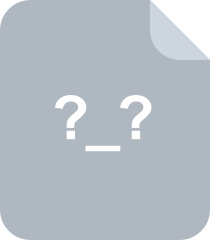
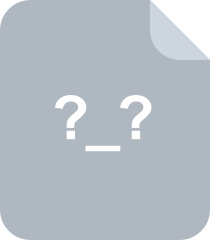
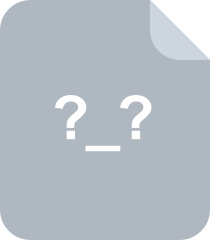
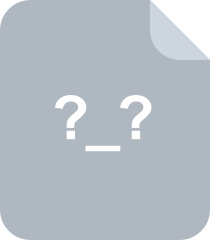
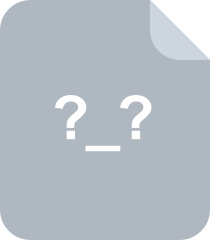
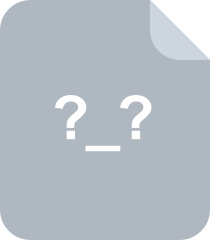
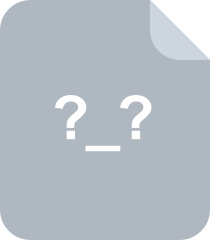
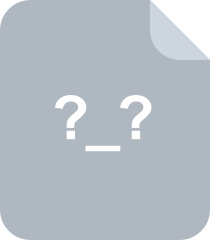
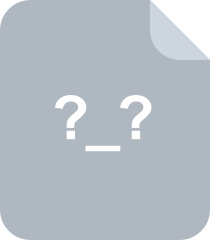
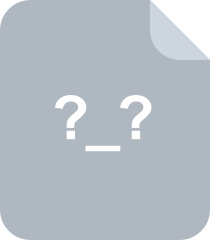
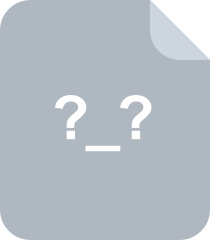
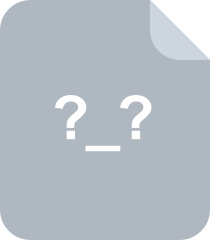
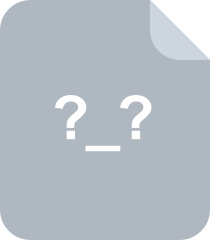
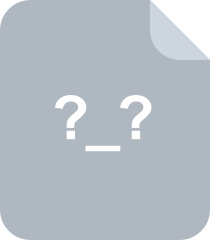
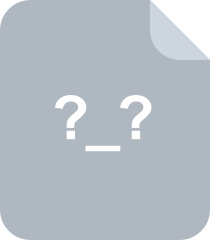
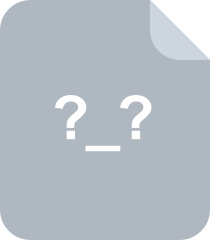
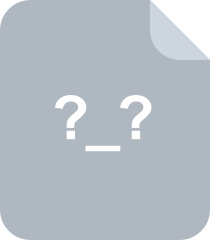
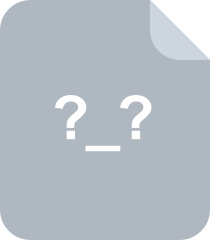
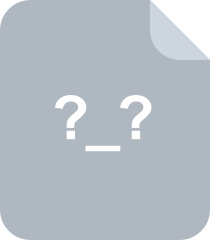
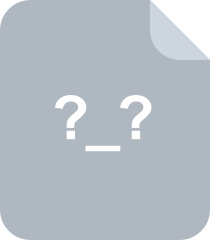
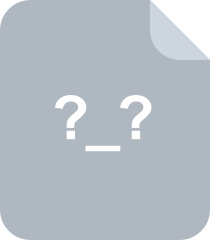
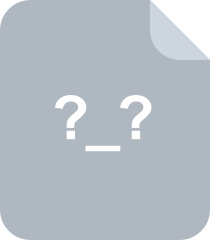
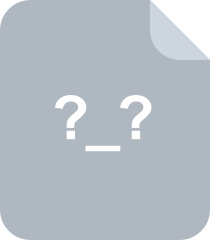
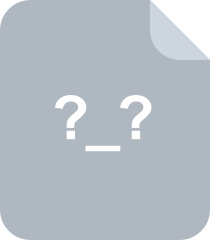
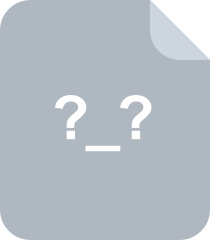
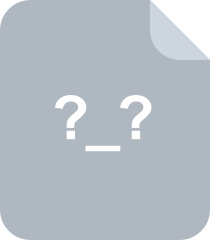
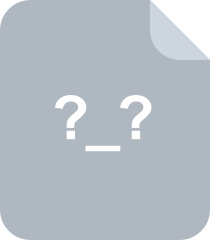
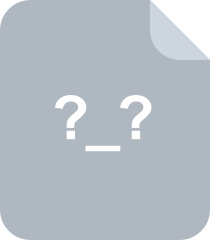
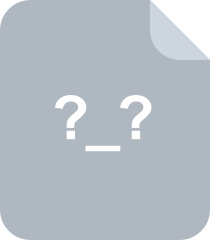
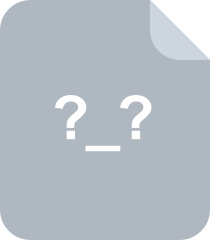
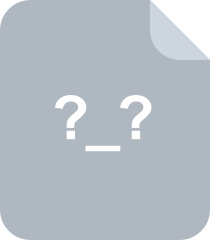
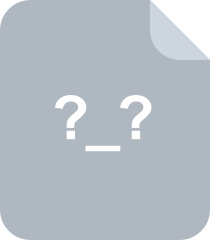
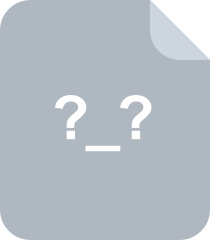
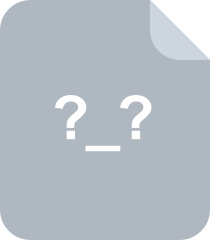
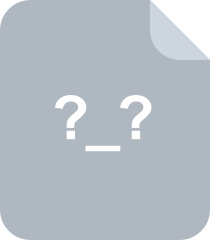
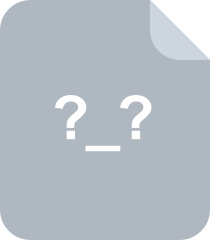
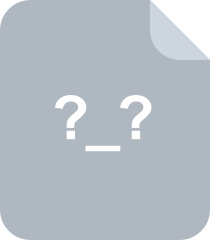
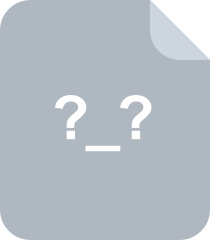
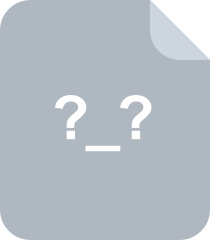
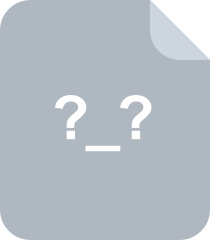
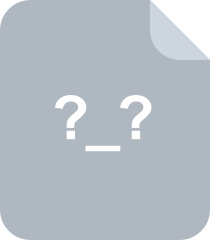
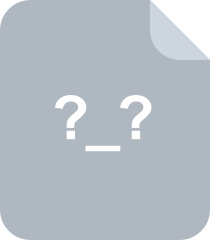
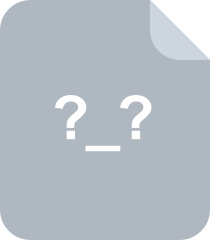
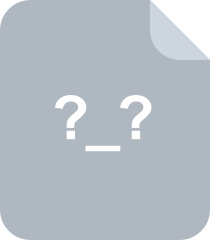
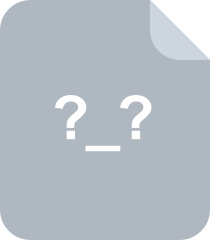
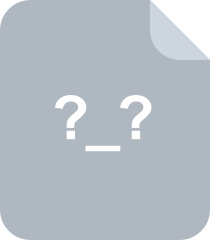
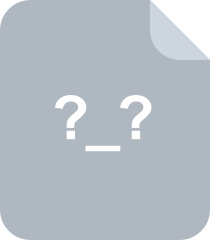
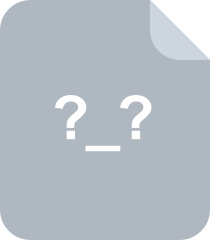
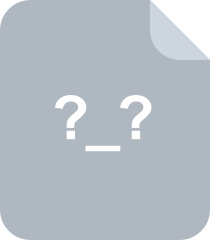
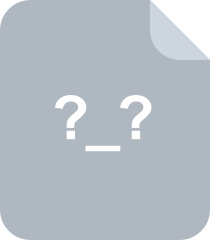
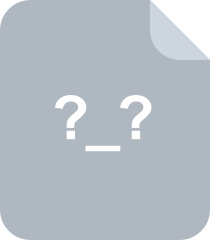
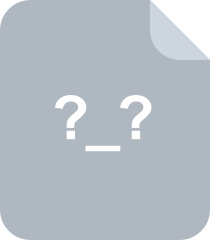
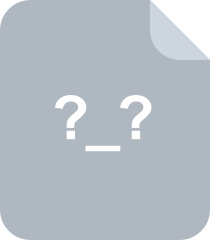
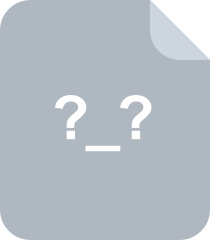
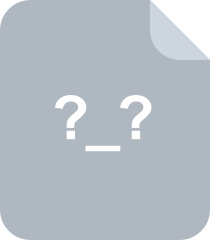
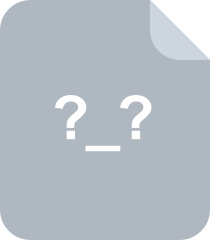
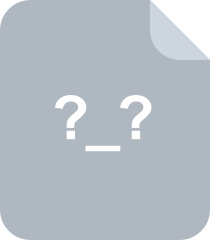
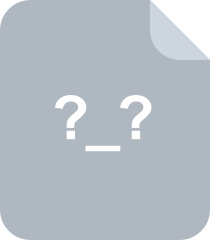
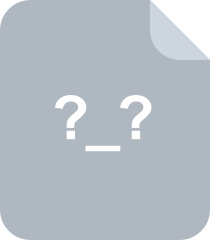
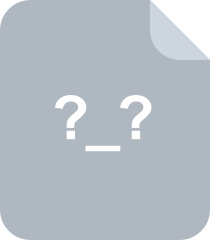
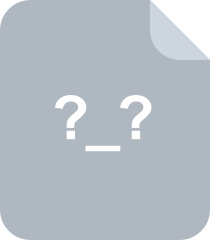
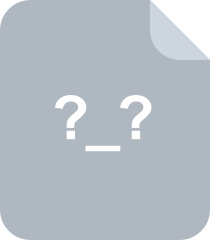
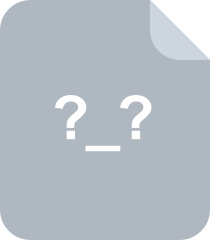
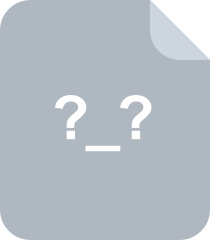
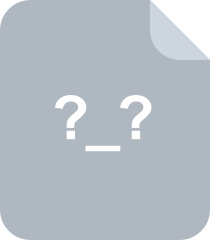
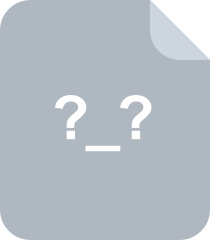
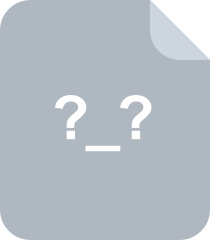
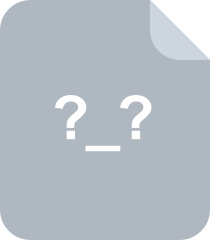
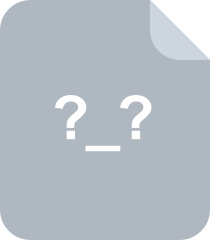
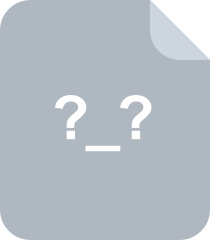
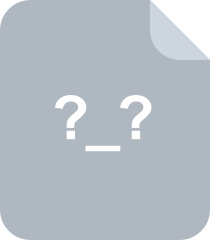
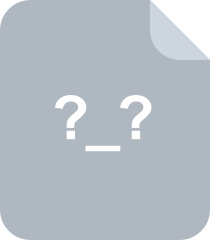
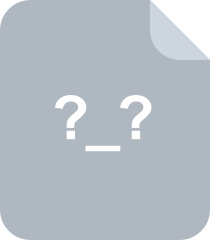
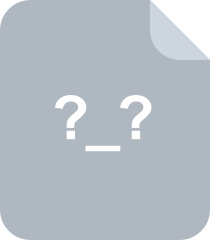
共 1172 条
- 1
- 2
- 3
- 4
- 5
- 6
- 12

sharkdoodoo
- 粉丝: 19
- 资源: 7
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

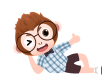
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页