#include<iostream>
#include<cstring>
#include<fstream>
using namespace std;
class database
{
int accno, ssno;
char name[25];
long long int phone;
float totalbal;
public:
void accept();
void acceptedit();
void display();
char* getname();
long long int getnumber();
int getaccno();
int getssno();
void edit_rec();
void delete_rec();
};
void database :: accept()
{
cout<<"\nEnter Account Number: ";
cin>>accno;
cout<<"Enter Name : ";
cin>>name;
cout<<"Enter Phone number : ";
cin>>phone;
cout<<"Enter Balance: ";
cin>>totalbal;
cout<<"Enter Social Security Number : ";
cin>>ssno;
cout<<endl;
}
void database :: acceptedit()
{
cout<<"\nEnter Name : ";
cin>>name;
cout<<"Enter Phone number : ";
cin>>phone;
cout<<"Enter Balance: ";
cin>>totalbal;
cout<<endl;
}
void database :: display()
{
cout<<"\nAccount Number: "<<accno<<endl;
cout<<"Name : "<<name<<endl;
cout<<"Phone : "<<phone<<endl;
cout<<"Current Balance: $"<<totalbal<<endl;
cout<<"-------------------------------"<<endl;
}
char* database :: getname()
{
return name;
}
long long int database :: getnumber()
{
return phone;
}
int database :: getaccno()
{
return accno;
}
int database :: getssno()
{
return ssno;
}
void create_file()
{
int n;
database d1;
cout<<"\nEnter no. of Customers: ";
cin>>n;
ofstream file;
file.open("record.DAT");
for(int i=0; i<n; ++i)
{
d1.accept();
file.write((char*)&d1 , sizeof(d1));
}
file.close();
}
void display_file()
{
ifstream file;
database d1;
file.open("record.DAT");
cout<<"\nEntered Details are : ";
while(file)
{
file.read((char*)&d1,sizeof(d1));
if(file.eof())
break;
d1.display();
}
file.close();
}
void addnewrec()
{
ofstream file;
database d1;
file.open("record.DAT" , ios::app);
cout<<"\nEnter Data to add New Record at the end of Previous\n";
d1.accept();
file.write((char*)&d1,sizeof(d1));
file.close();
}
void searchonName()
{
ifstream file;
database d1;
char name[25];
bool flag = 0;
cout<<"\nEnter Name To Be Searched ";
cin>>name;
file.open("record.DAT" , ios::in);
while(file)
{
file.read((char*)&d1,sizeof(d1));
if (file.eof()!=0)
break;
if (strcmp(d1.getname(),name)==0)
{
cout<<"\nData Found ";
d1.display();
flag = 1;
break;
}
}
file.close();
if (flag == 0)
{
cout<<"\nRecord Not Found ";
}
}
void searchonNumber()
{
ifstream file;
database d1;
long long int phone;
bool flag = 0;
cout<<"\nEnter Number To Be Searched ";
cin>>phone;
file.open("record.DAT" , ios::in);
while(file)
{
file.read((char*)&d1,sizeof(d1));
if (file.eof()!=0)
break;
if (d1.getnumber() == phone)
{
cout<<"\nData Found ";
d1.display();
flag = 1;
break;
}
}
file.close();
if (flag == 0)
{
cout<<"\nRecord Not Found ";
}
}
void searchonAccNumber()
{
ifstream file;
database d1;
int accno;
bool flag = 0;
cout<<"\nEnter Account Number To Be Searched ";
cin>>accno;
file.open("record.DAT" , ios::in);
while(file)
{
file.read((char*)&d1,sizeof(d1));
if (file.eof()!=0)
break;
if (accno == d1.getaccno())
{
cout<<"\nData Found ";
d1.display();
flag = 1;
break;
}
}
file.close();
if (flag == 0)
{
cout<<"\nRecord Not Found ";
}
}
void modifyonname()
{
fstream file;
database d1;
char name[25];
int ssno;
bool flag = 0;
cout<<"\nEnter Name To Be Searched : ";
cin>>name;
file.open("record.DAT" , ios::in | ios::out | ios::binary);
while(file)
{
file.read((char*)&d1,sizeof(d1));
if (file.eof()!=0)
break;
if (strcmp(d1.getname(),name)==0)
{
cout<<"\nAccount Found ";
cout<<"\nEnter Your Social Security Number : ";
cin>>ssno;
if(ssno == d1.getssno())
{
cout<<"\nEnter New Details : ---->>>";
d1.acceptedit();
file.seekp(-sizeof(d1),ios::cur);
file.write((char*)&d1,sizeof(d1));
flag = 1;
break;
}
else
cout<<"\nGod Is Watching Don't Cheat!!!\n";
}
}
file.close();
if (flag == 0)
{
cout<<"\nRecord Not Found!!!! ";
}
}
void modifyonaccno()
{
fstream file;
database d1;
int accno;
int ssno;
bool flag = 0;
cout<<"\nEnter Account Number To Be Searched : ";
cin>>accno;
file.open("record.DAT" , ios::in | ios::out | ios::binary);
while(file)
{
file.read((char*)&d1,sizeof(d1));
if (file.eof()!=0)
break;
if (d1.getaccno() == accno)
{
cout<<"\nAccount Found ";
cout<<"\nEnter Your Social Security Number : ";
cin>>ssno;
if(ssno == d1.getssno())
{
cout<<"\nEnter New Details : --->>>";
d1.acceptedit();
file.seekp(-sizeof(d1),ios::cur);
file.write((char*)&d1,sizeof(d1));
flag = 1;
break;
}
else
cout<<"\nGod Is Watching Don't Cheat!!!\n";
}
}
file.close();
if (flag == 0)
{
cout<<"\nRecord Not Found ";
}
}
int main()
{
int ch, password, pass;
int i=0;
password = 12345;
do
{
cout<<"\n======== BANK ACCOUNT MANAGEMENT SYSTEM ========";
cout<<"\nAdmin Enter Your Password : ";
cin>>pass;
if (password == pass)
{
cout<<"\n***** WELCOME *****\n";
do
{
cout<<"\n1. Create Database";
cout<<"\n2. Add Record";
cout<<"\n3. Search Customer By Name ";
cout<<"\n4. Search Customer By Phone Number";
cout<<"\n5. Search Customer By Account Number";
cout<<"\n6. Update Customer Data By Name ";
cout<<"\n7. Update Customer Data By Account Number";
cout<<"\n8. View Your Database";
cout<<"\n\nEnter Your Choice : ";
cin>>ch;
switch(ch)
{
case 1 : create_file(); break;
case 2 : addnewrec(); break;
case 3 : searchonName(); break;
case 4 : searchonNumber(); break;
case 5 : searchonAccNumber(); break;
case 6 : modifyonname(); break;
case 7 : modifyonaccno(); break;
case 8 : display_file(); break;
}
}while(ch>0 && ch<9);
}
else
{
cout<<"\nYou Are No Admin\n";
i++;
cout<<'\n'<<5-i<<" Trie(s) More Left";
}
}while(i>0 && i<5);
return 0;
}
没有合适的资源?快使用搜索试试~ 我知道了~
C++源代码银行帐户管理系统
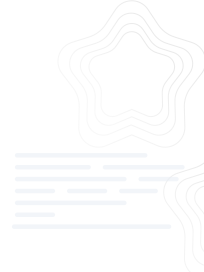
共1个文件
cpp:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 37 浏览量
2024-02-28
18:18:10
上传
评论 1
收藏 2KB ZIP 举报
温馨提示
银行账户管理系统仅使用C++编程语言创建。该系统是一种用户友好的系统,可以轻松修改。该系统为您提供了记录客户银行详细信息的最快方法。系统受用户登录信息保护,您需要输入正确的密码才能访问全部功能。所有输入的数据都存储为 dat 文件扩展名。 C++源代码银行帐户管理系统特征 创建数据库 添加记录 按名称搜索客户 按电话号码搜索客户 按帐号搜索客户 按名称更新客户数据 按帐号更新客户数据 查看数据库
资源推荐
资源详情
资源评论
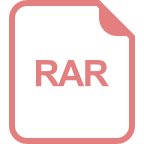
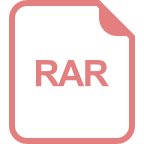
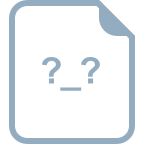
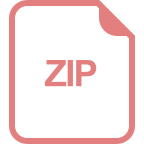
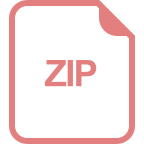
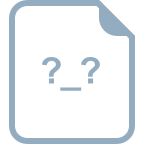
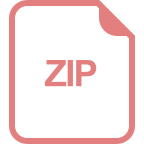
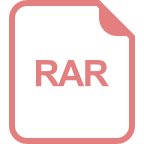
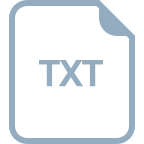
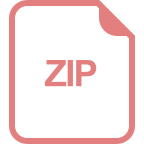
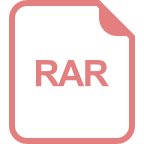
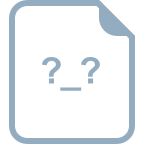
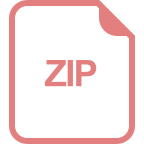
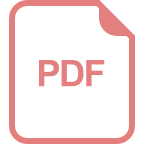
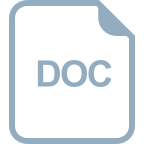
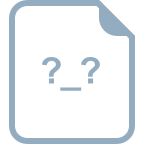
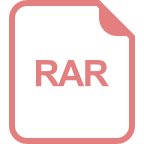
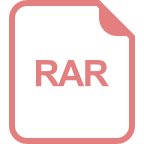
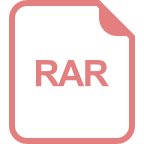
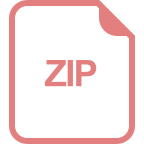
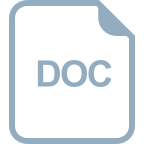
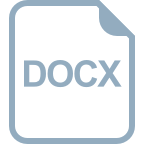
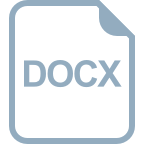
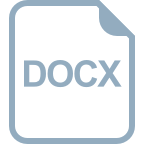
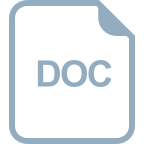
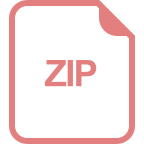
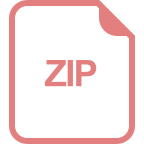
收起资源包目录


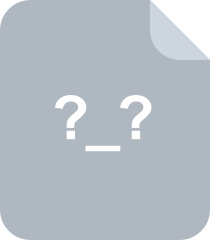
共 1 条
- 1
资源评论
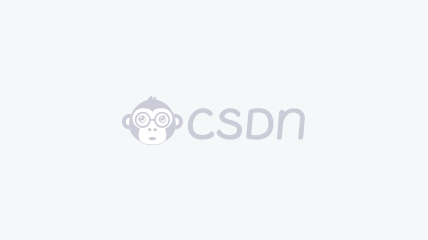


新华
- 粉丝: 1w+
- 资源: 628
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

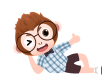
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


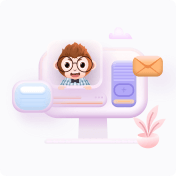
安全验证
文档复制为VIP权益,开通VIP直接复制
