/**
* Copyright (C) 2007 Sly Technologies, Inc. This library is free software; you
* can redistribute it and/or modify it under the terms of the GNU Lesser
* General Public License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version. This
* library is distributed in the hope that it will be useful, but WITHOUT ANY
* WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR
* A PARTICULAR PURPOSE. See the GNU Lesser General Public License for more
* details. You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*/
package org.jnetpcap;
import java.io.File;
import java.io.IOException;
import java.net.SocketException;
import java.nio.ByteBuffer;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.atomic.AtomicInteger;
import junit.framework.TestCase;
import junit.textui.TestRunner;
import org.jnetpcap.nio.JBuffer;
import org.jnetpcap.nio.JMemory;
import org.jnetpcap.nio.JNumber;
import org.jnetpcap.nio.JNumber.Type;
/**
* @author Mark Bednarczyk
* @author Sly Technologies, Inc.
*/
@SuppressWarnings("deprecation")
public class TestPcapJNI
extends TestCase {
// private final static String device =
// "\\Device\\NPF_{BC81C4FC-242F-4F1C-9DAD-EA9523CC992D}";
private final static String win =
"\\Device\\NPF_{BC81C4FC-242F-4F1C-9DAD-EA9523CC992D}";
private final static String linux = "any";
private final static boolean isWindows =
"Windows XP".equals(System.getProperty("os.name"));
private final static String device = (isWindows) ? win : linux;
private final static String fname = "tests/test-l2tp.pcap";
private static final int OK = 0;
private static final int snaplen = 64 * 1024;
private static final int promisc = 1;
private static final int oneSecond = 1000;
/**
* Will generate HTTP traffic to a website. Use start() to start in a test
* method, and always put stop() in tearDown. Safe to call stop even when
* never started.
*/
private static final HttpTrafficGenerator gen = new HttpTrafficGenerator();
private static File tmpFile;
static {
try {
tmpFile = File.createTempFile("temp-", "-TestPcapJNI");
} catch (IOException e) {
tmpFile = null;
System.err.println("Unable to initialize a temporary file");
}
}
/**
* Command line launcher to run the jUnit tests cases in this test class.
*
* @param args
* -h for help
*/
public static void main(String[] args) {
if (args.length == 1 && "-h".equals(args[0])) {
System.out
.println("Usage: java -jar jnetpcap.jar [-h]\n"
+ " -h This help message\n"
+ " (No other command line options are supported.)\n"
+ "----------------------------------------------------------------\n\n"
+ "The 'main' method invoked here, runs several dozen jUnit tests\n"
+ "which test the functionality of this jNetPcap library.\n"
+ "The tests are actual excersizes using native libpcap\n"
+ "library linked with 'jnetpcap.dll' or 'libjnetpcap.so' on\n"
+ "unix systems.\n\n"
+ "If you are having trouble linking the native library and get\n"
+ "'UnsatisfiedLinkError', which means java is not finding the\n"
+ "library, here are a few pointers:\n\n"
+ "Java's native library loader DOES NOT USE CLASSPATH variable\n"
+ "to locate native libraries. Each operating system uses different\n"
+ "algorithm to locate files, as described below. You can always\n"
+ "force java to look for native library with Java VM command\n"
+ "line option 'java -Djava.library.path=lib' where lib is\n"
+ "a directory where 'jnetpcap.dll' or 'libjnetpcap.so' resides\n"
+ "relative to the installation directory of jNetStream package.\n"
+ "Or replace lib with the directory where you have installed the\n"
+ "library.\n\n"
+ "On Win32 systems:\n"
+ " Windows systems use /windows and /windows/system32 folder\n"
+ " to search for jnetpcap.dll. Also the 'PATH' variable, the same\n"
+ " one used to specify executable commands, is used as well.\n\n"
+ "On Unix systems:\n"
+ " All unix systems use the standard 'LD_LIBRARY_PATH' variable.\n\n"
+ "Of course as mentioned earlier, to override this behaviour use\n"
+ "the '-Djava.library.path=' directory, to force java to look in\n"
+ "that particular directory. Do not set the path which includes the\n"
+ "name of the library itself, just the directory to search in.\n\n"
+ "Final note, native librariers can not be loaded from jar files.\n"
+ "You have to extract it to a physical directory if you want java to\n"
+ "load it. This was done purposely by Sun for security reasons.");
return;
}
TestRunner.main(new String[] { "org.jnetpcap.TestPcapJNI" });
}
private StringBuilder errbuf = new StringBuilder();
private final PcapHandler<?> doNothingHandler = new PcapHandler<Object>() {
public void nextPacket(Object userObject, long seconds, int useconds,
int caplen, int len, ByteBuffer buffer) {
// Do nothing handler
}
};
/**
* @throws java.lang.Exception
*/
protected void setUp() throws Exception {
errbuf = new StringBuilder();
if (tmpFile.exists()) {
assertTrue(tmpFile.delete());
}
}
/**
* Test disabled, as it requires live packets to capture. To enable the test
* just rename the method, by removing the prefix SKIP. Then make sure there
* are live packets to be captured.
*/
public void SKIPtestOpenLiveAndDispatch() {
Pcap pcap = Pcap.openLive(device, 10000, 1, 60 * 1000, errbuf);
assertNotNull(errbuf.toString(), pcap);
PcapHandler<String> handler = new PcapHandler<String>() {
public void nextPacket(String user, long seconds, int useconds,
int caplen, int len, ByteBuffer buffer) {
// System.out.printf("%s, ts=%s caplen=%d len=%d capacity=%d\n", user
// .toString(), new Date(seconds * 1000).toString(), caplen, len,
// buffer.capacity());
}
};
pcap.dispatch(10, handler, "Hello");
pcap.close();
}
/**
* @throws java.lang.Exception
*/
protected void tearDown() throws Exception {
errbuf = null;
/*
* Stop the traffic generator, even when not running need to call to make
* sure its not running.
*/
gen.stop();
if (tmpFile != null && tmpFile.exists()) {
tmpFile.delete();
}
}
public void testCompileNoPcapNullPtrHandling() {
try {
Pcap.compileNoPcap(1, 1, null, null, 1, 1);
fail("Expected a NULL pointer exception.");
} catch (NullPointerException e) {
// OK
}
}
public void testCompileNullPtrHandling() {
Pcap pcap = Pcap.openOffline(fname, errbuf);
try {
pcap.compile(null, null, 1, 0);
fail("Expected a NULL pointer exception.");
} catch (NullPointerException e) {
// OK
} finally {
pcap.close();
}
}
public void testDataLinkNameToValNullPtrHandling() {
try {
Pcap.datalinkNameToVal(null);
fail("Expected a NULL pointer exception.");
} catch (NullPointerException e) {
// OK
}
}
public void testPcapClosedExceptionHandling() {
Pcap pcap = Pcap.openOffline(fname, errbuf);
pcap.close();
try {
pcap.breakloop();
fail("Expected PcapClosedException");
} catch (PcapClosedException e) {
// Success
}
}
public void testDatalinkNameToValue() {
assertEquals(1, Pcap.datalinkNam
没有合适的资源?快使用搜索试试~ 我知道了~
JnetPcap源码
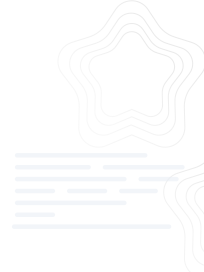
共230个文件
class:150个
java:48个
dep2:10个


温馨提示
jNetPcap是libpcap的一个Java完整封装。jNetPcap使用与libpcap相同风格的API。libpcap是unix/linux平台下的网络数据包捕获函数库,大多数网络监控软件都以它为基础。 Libpcap可以在绝大多数类unix平台下工作。Libpcap提供了系统独立的用户级别网络数据包捕获接口,并充分考虑到应用程序的可移植性。
资源推荐
资源详情
资源评论
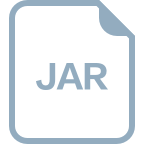
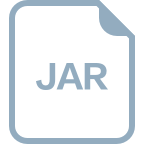
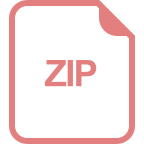
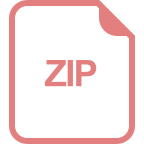
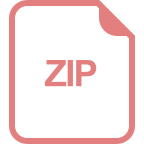
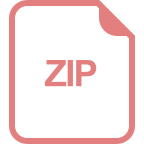
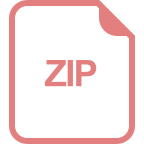
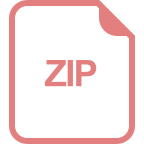
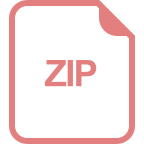
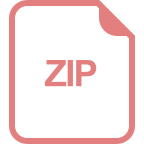
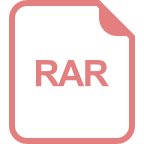
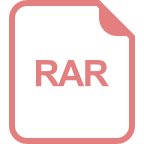
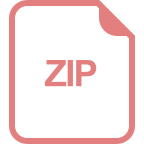
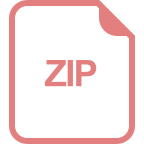
收起资源包目录

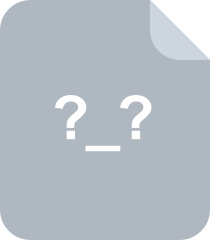
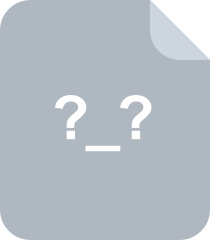
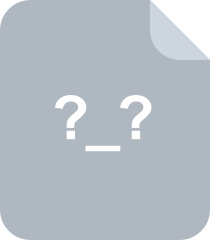
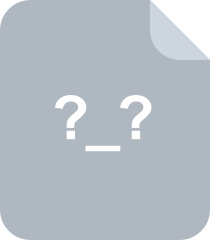
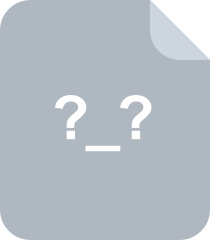
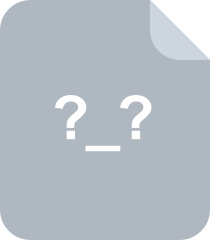
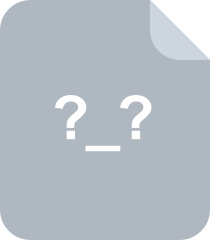
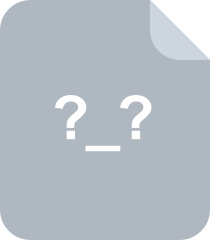
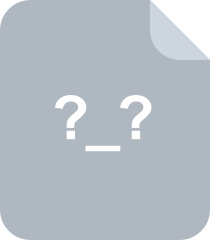
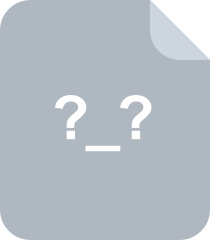
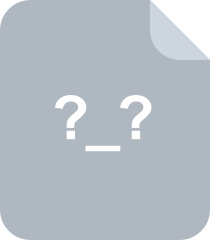
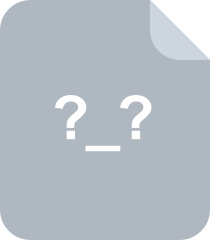
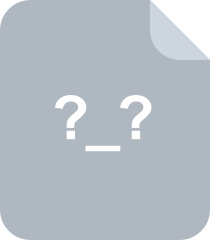
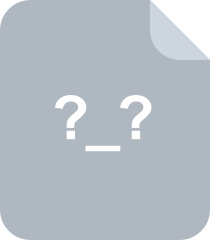
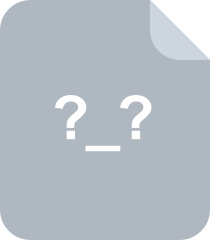
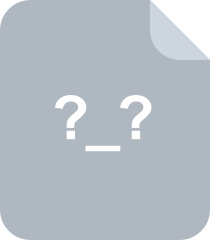
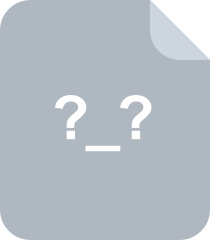
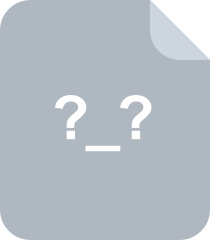
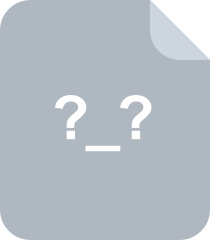
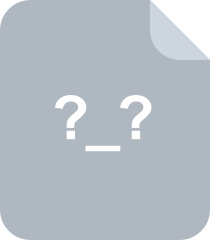
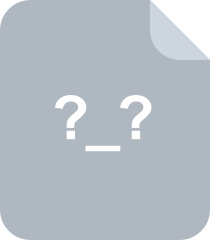
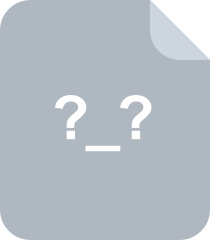
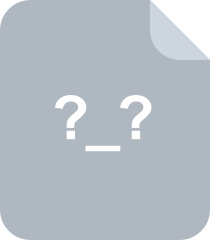
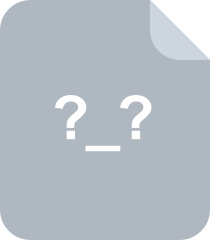
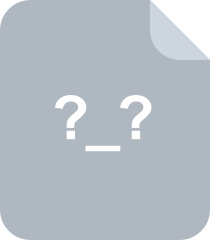
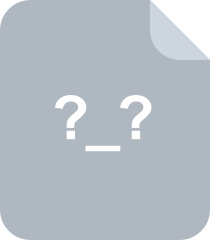
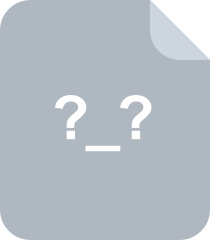
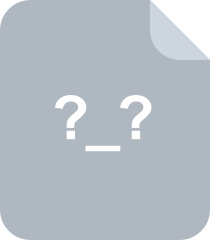
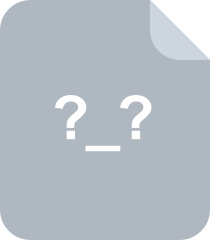
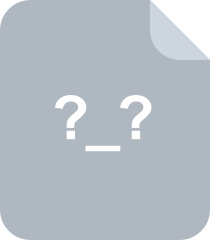
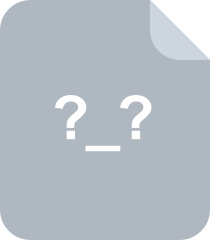
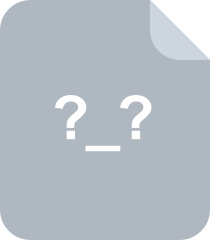
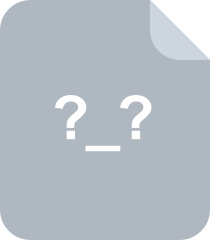
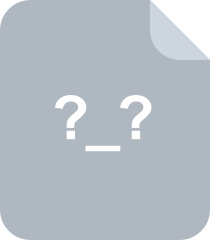
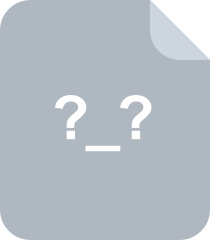
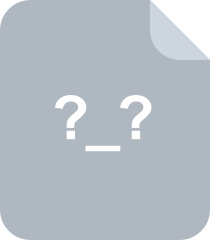
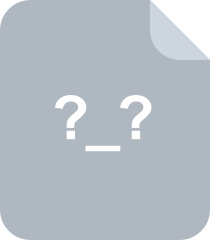
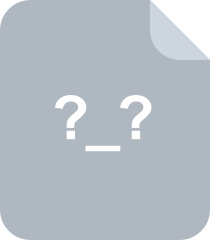
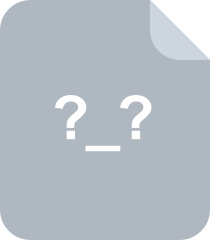
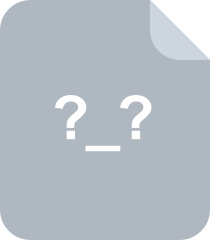
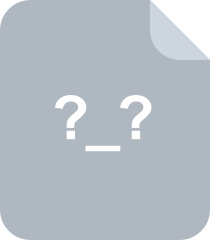
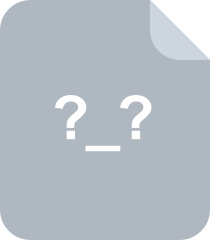
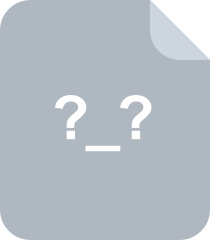
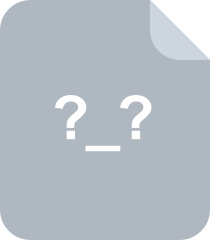
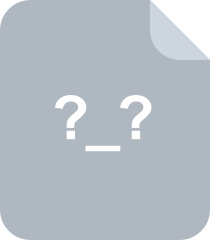
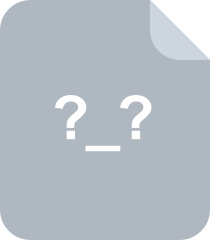
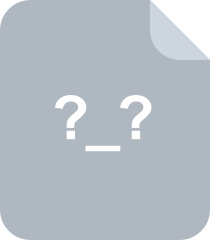
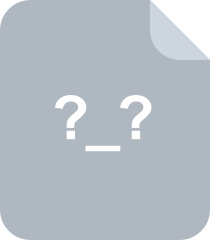
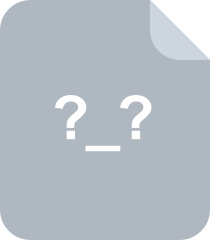
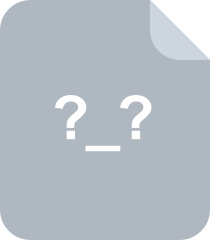
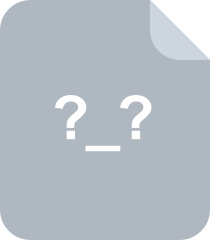
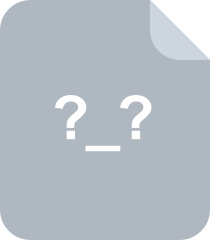
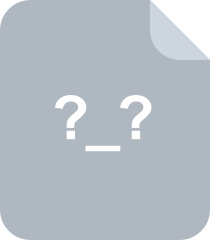
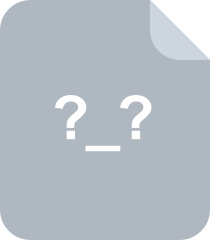
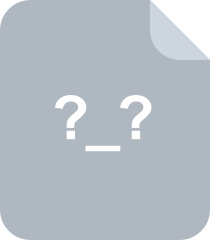
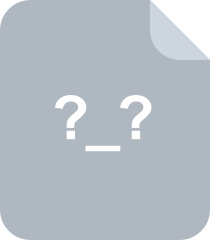
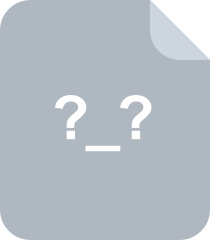
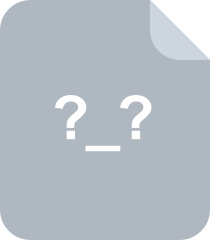
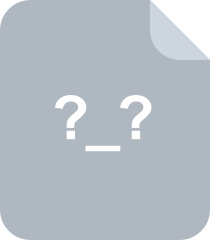
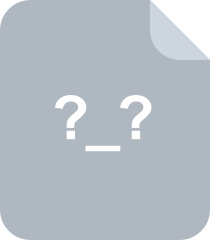
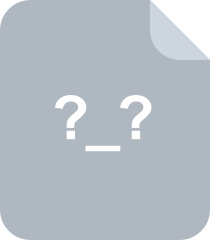
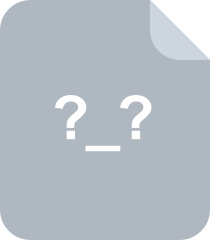
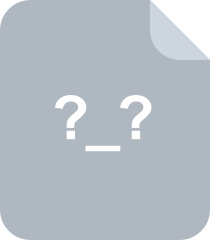
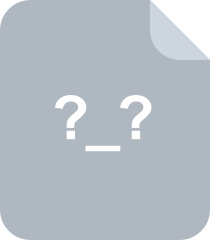
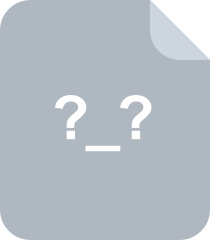
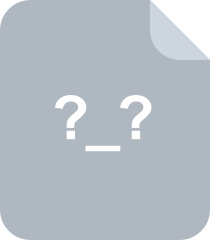
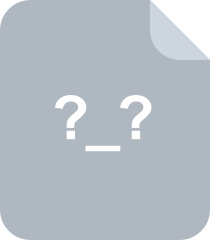
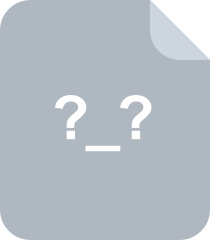
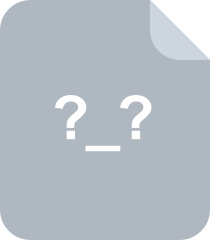
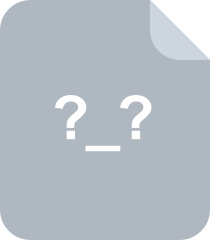
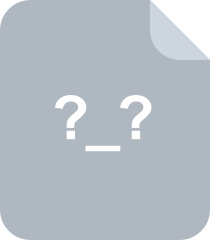
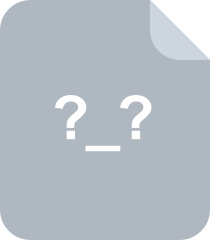
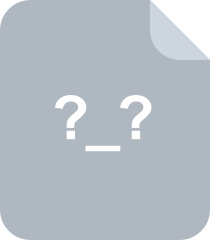
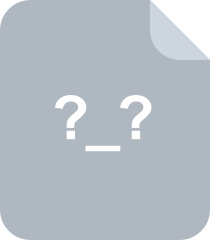
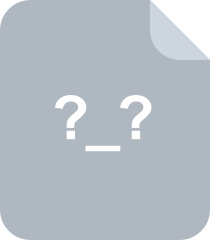
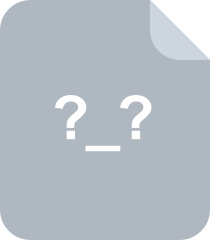
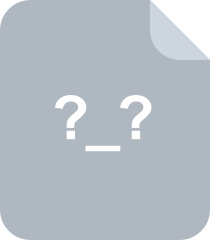
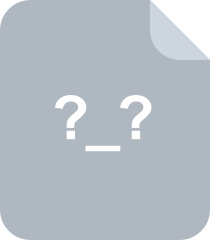
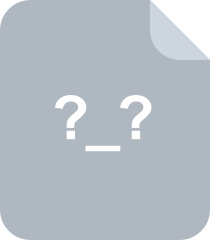
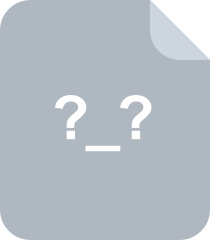
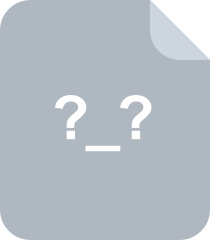
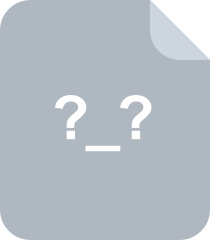
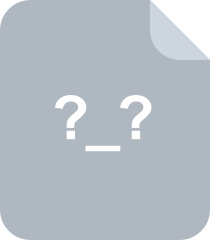
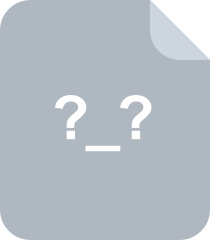
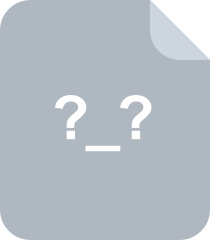
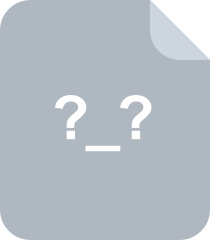
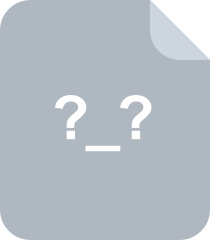
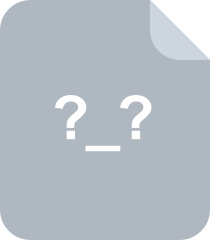
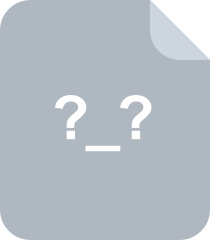
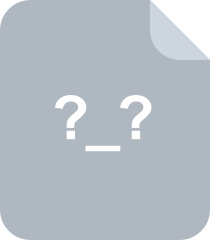
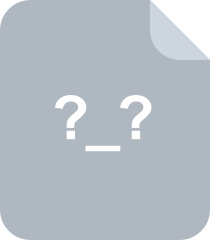
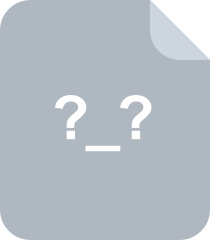
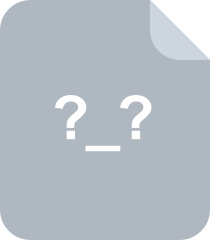
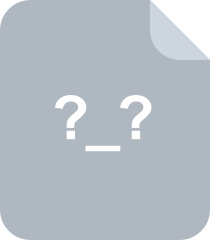
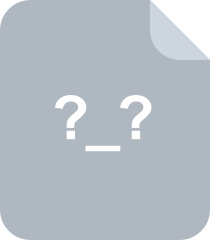
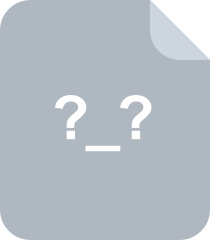
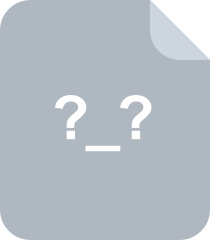
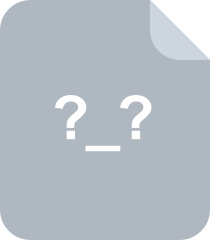
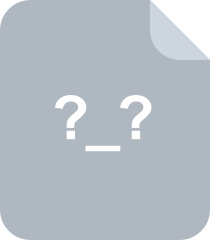
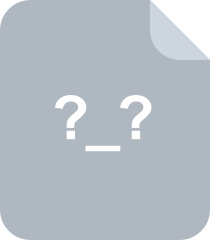
共 230 条
- 1
- 2
- 3
资源评论
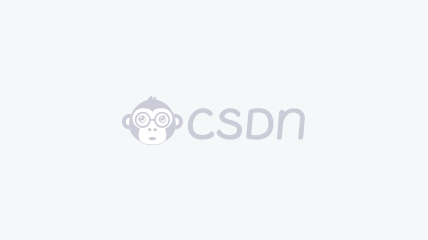
- tabuaiwo5212012-08-28楼主可以有详细的操作指导说明附送就最好了,多谢
- ryf123012012-04-11现在正在用它来做自己的毕业设计,因为要用java来编写捕获和分析数据包,但是java并不支持底层协议的处理,所以用此开发包可以解决这个问题。
- 右_手2013-05-03没有例子看不懂啊 郁闷了

myjch
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

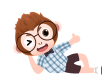
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


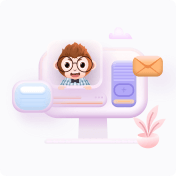
安全验证
文档复制为VIP权益,开通VIP直接复制
