### Direct Memory Access (DMA) in Linux In the context of embedded systems and real-time applications, Direct Memory Access (DMA) is a crucial technique that allows hardware devices to directly access physical memory without involving the CPU. This method significantly reduces the CPU’s workload and improves overall system performance, especially in scenarios requiring high-speed data transfer. #### Key Concepts and Terminology Before diving into the details, let’s define some important terms: - **Physical Memory**: Refers to the actual RAM chips on the motherboard. - **Virtual Memory**: The abstraction layer provided by the operating system to manage memory, often mapped to physical addresses but not always directly. - **DMA**: A mechanism that enables hardware devices to directly access physical memory without going through the CPU. - **Kernel Module**: A piece of code that can be loaded or unloaded into the kernel at runtime, providing additional functionality. #### Understanding the Challenge The original poster is working on an embedded Linux project where they need to access physical memory directly for implementing a high-performance logging solution. The goal is to allocate a specific block of memory (1.5 MB worth) and ensure that this memory is not allocated to any other process by the kernel. The challenge lies in consistently accessing the same physical memory space and preventing the kernel from using it for other purposes. #### Exploring Solutions To address these requirements, several approaches can be considered: 1. **Using `/dev/mem`**: The simplest method is to use the `/dev/mem` device file, which provides access to the physical memory. However, this approach has limitations, as the memory accessed in this way can easily be allocated to any process. The provided code snippet demonstrates how to open `/dev/mem`, allocate memory, and map it using `mmap()`. 2. **Limiting Kernel Visibility**: The poster tried limiting the amount of memory visible to the kernel by booting with `mem=XXXm` and setting `BASE_ADDRESS` above the visible memory range. However, this did not provide consistent results. This is likely due to the complex interactions between the kernel’s memory management and the physical memory layout. 3. **Kernel Modules and DMA Functions**: - **ioremap()**: This function maps a physical memory range into the kernel’s virtual address space. It is commonly used for DMA operations. By using `ioremap()`, you can allocate a specific block of physical memory and map it into the kernel’s virtual address space, ensuring that it is not allocated to user processes. - **remap_pfn_range()**: This function maps a physical page frame number (PFN) range into the kernel’s virtual address space. It is useful when you need to map a continuous physical memory region into the kernel’s virtual address space. Unlike `ioremap()`, `remap_pfn_range()` does not require a specific address and can be more flexible in terms of addressing. 4. **Creating a Kernel Module**: - **Module Initialization**: When initializing the module, use `ioremap()` or `remap_pfn_range()` to map the desired physical memory region into the kernel’s virtual address space. Ensure that the memory range is not already mapped or used by the kernel. - **Module Cleanup**: On module removal, unmap the memory using `iounmap()` or `munmap()` to free up the resources. #### Detailed Implementation Example Here is a simplified example of how you might implement a kernel module to achieve the desired behavior: ```c #include <linux/module.h> #include <linux/kernel.h> #include <linux/init.h> #include <linux/ioremap.h> #define MEMORY_SIZE (1.5 * 1024 * 1024) // 1.5 MB #define BASE_ADDRESS 0x12345678 // Physical base address static void __iomem *mapped_memory; static int __init my_dma_module_init(void) { mapped_memory = ioremap(BASE_ADDRESS, MEMORY_SIZE); if (!mapped_memory) { printk(KERN_ERR "Failed to map memory\n"); return -ENOMEM; } printk(KERN_INFO "Memory mapped successfully\n"); return 0; } static void __exit my_dma_module_exit(void) { iounmap(mapped_memory); printk(KERN_INFO "Memory unmapped successfully\n"); } module_init(my_dma_module_init); module_exit(my_dma_module_exit); MODULE_LICENSE("GPL"); MODULE_AUTHOR("Your Name"); MODULE_DESCRIPTION("A simple DMA module for accessing physical memory in Linux."); ``` This example demonstrates how to create a basic kernel module that maps a specific physical memory region (`1.5 MB`) starting from a given address (`0x12345678`). The module uses `ioremap()` to map the memory into the kernel’s virtual address space. On module initialization, the memory is mapped, and on module removal, it is unmapped. #### Considerations and Best Practices - **Performance Optimization**: When implementing DMA, consider optimizing your code for performance. Use appropriate data types and avoid unnecessary data copies. - **Error Handling**: Always include proper error handling to handle cases where memory mapping fails. - **Testing**: Thoroughly test your implementation on different hardware configurations to ensure reliability and compatibility. - **Documentation**: Document your code and the logic behind your design decisions. This will make it easier for others (or yourself in the future) to understand and maintain the code. #### Conclusion Direct Memory Access (DMA) in Linux is a powerful technique that can significantly improve the performance of embedded systems and real-time applications. By understanding the limitations of traditional methods like `/dev/mem` and leveraging kernel modules and DMA functions such as `ioremap()` and `remap_pfn_range()`, developers can allocate and access specific blocks of physical memory efficiently and reliably. With careful planning and implementation, it is possible to create robust and performant solutions for high-speed data transfer and logging in Linux-based embedded systems.
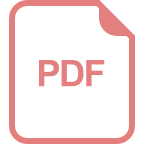
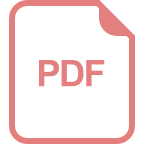
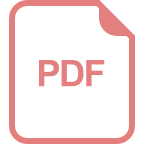
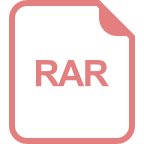
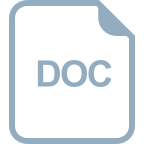
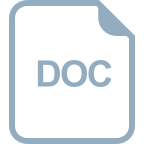
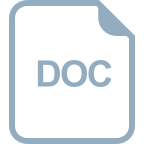
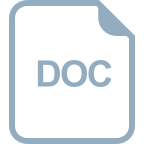
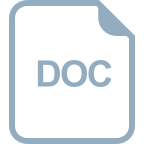
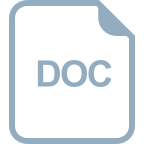
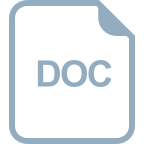
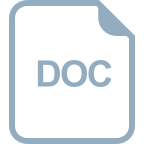
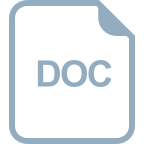
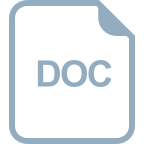
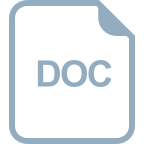
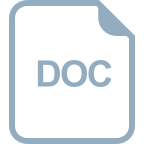
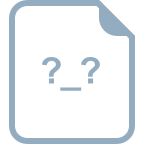
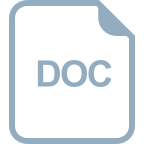
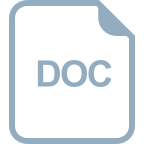
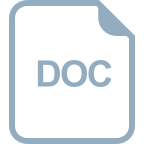
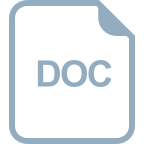
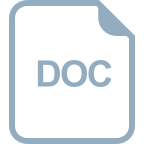
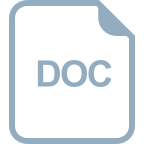
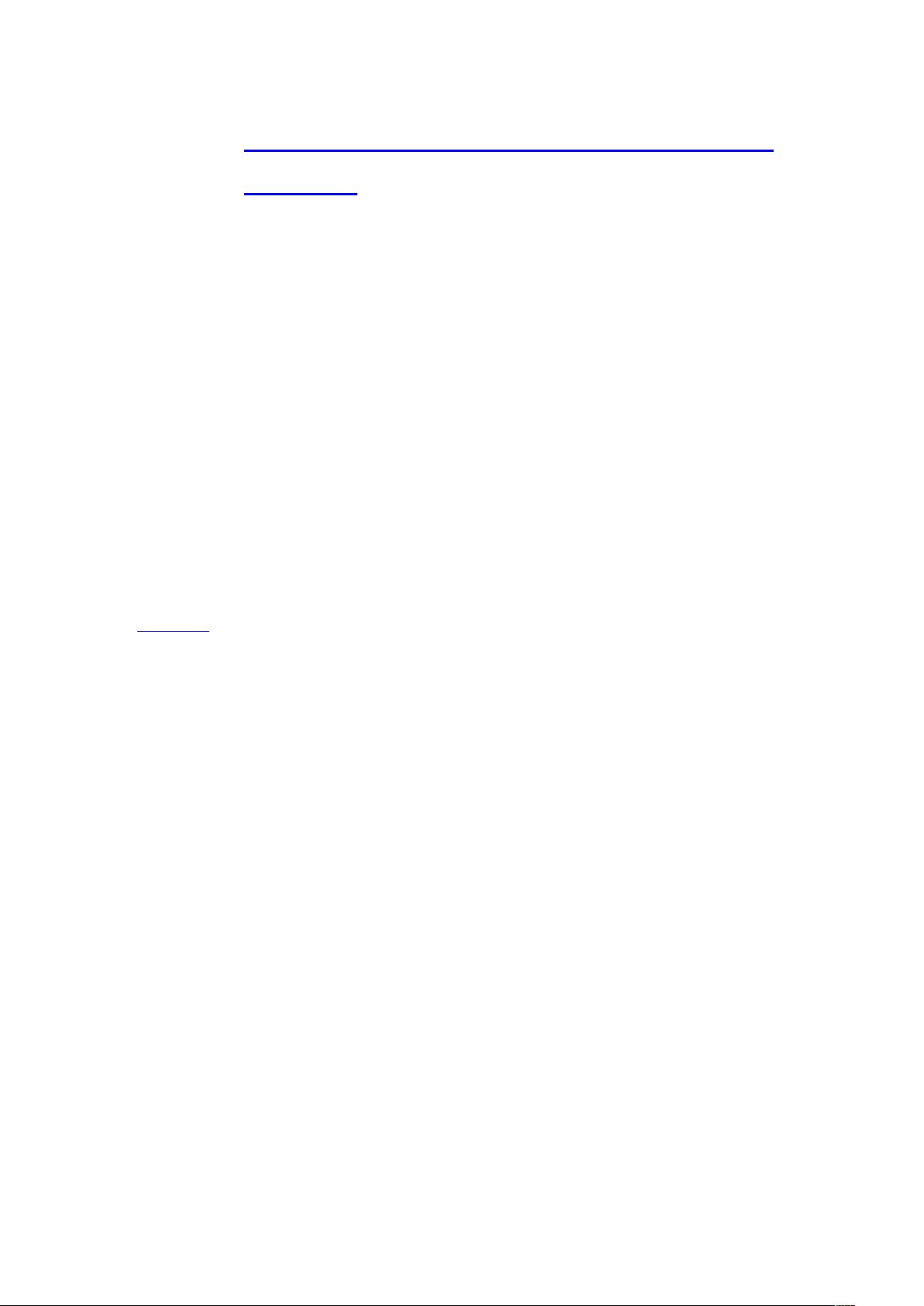
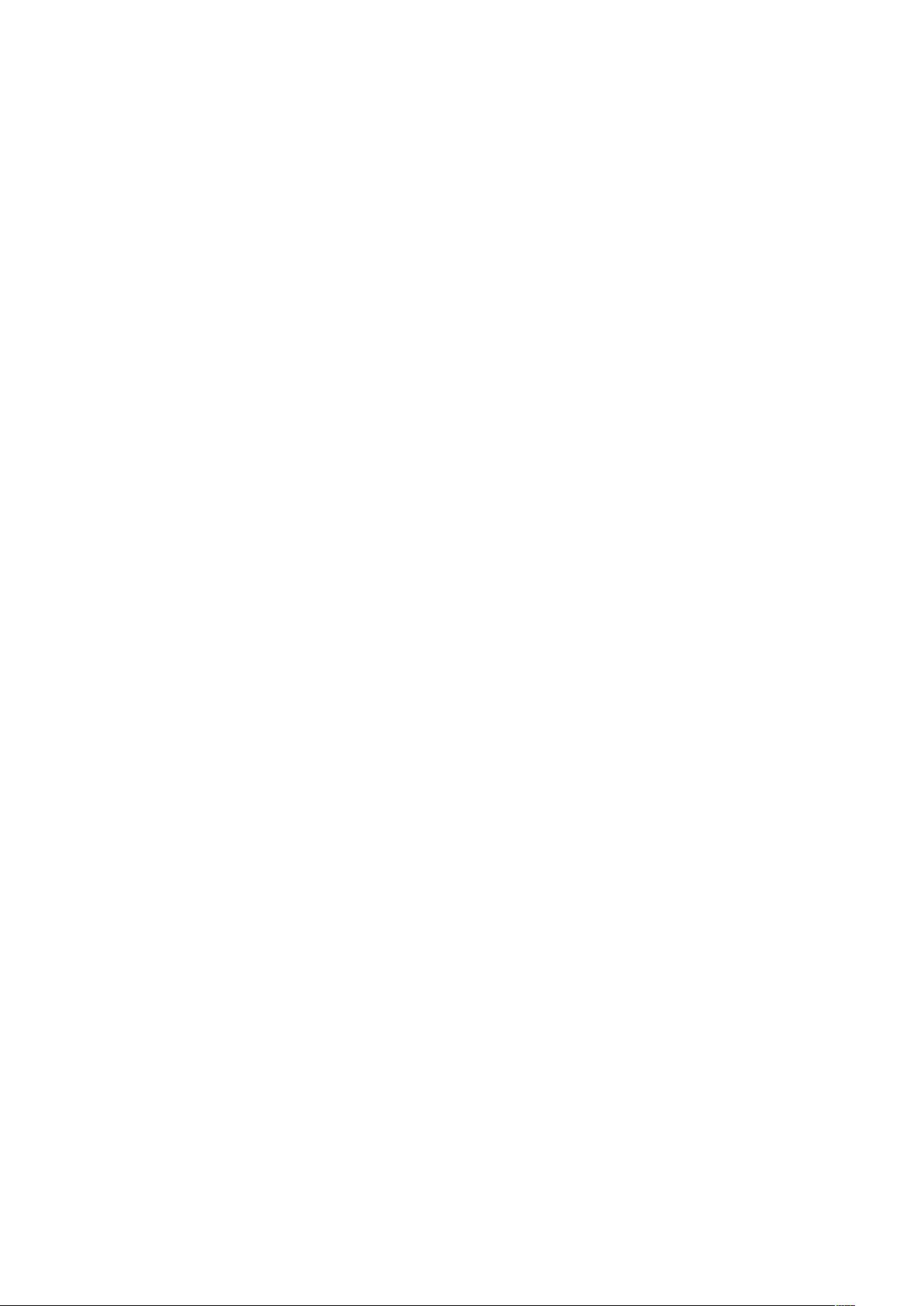
剩余9页未读,继续阅读
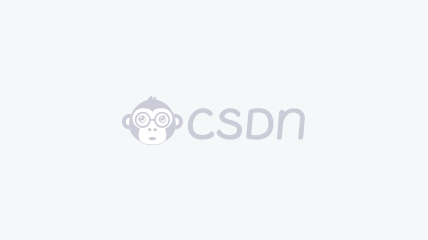

- 粉丝: 398
- 资源: 60
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

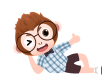
最新资源

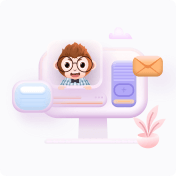
