/********************************************************************/
/********************************************************************/
/***** *****/
/***** L A B C E N T E R E L E C T R O N I C S *****/
/***** *****/
/***** PROTEUS VSM TINY CHESS SAMPLE *****/
/***** *****/
/***** Move Validation Control *****/
/***** *****/
/********************************************************************/
/********************************************************************/
#include "chess.h"
/************************************************************************
***** Piece Validation Functions *****
**************************************/
VOID val_empty(LOC from,BOOL piececolour)
{// Do nothing...
from = from;
piececolour = piececolour;
}
VOID val_pawn(LOC from,BOOL piececolour)
// Find all Valid moves for a pawn.
{ COORD row = from[0],col = from[1];
BOOL piecefound = FALSE;
// Get the opponents king position.
if (piececolour == WHITE)
{ opp_king_pos[0] = bl_king_pos[0];
opp_king_pos[1] = bl_king_pos[1];
}
else
{ opp_king_pos[0] = wh_king_pos[0];
opp_king_pos[1] = wh_king_pos[1];
}
// Increment the row towards the opponents pieces.
if (piececolour == WHITE)
row++;
else
row--;
// We can move into an empty square directly in front of us.
if (board[row][col] == EMPTY)
{ validmovemask[row] |= (1<<col);
}
else
piecefound = TRUE;
// We can diagonally take an opponents piece.
if ((is_opp_piece(row,col-1,piececolour)) && (col > MIN_COL))
{ //left
validmovemask[row] |= (1<<(col - 1));
capturemask[row] |= (1<<(col - 1));
if (capturemask[opp_king_pos[0]] & (1 << opp_king_pos[1])) kingcapture = TRUE;
}
if ((is_opp_piece(row,col+1,piececolour)) && (col < MAX_COL))
{ //right
validmovemask[row] |= (1<<(col + 1));
capturemask[row] |= (1<<(col + 1));
if (capturemask[opp_king_pos[0]] & (1 << opp_king_pos[1])) kingcapture = TRUE;
}
// If a pawn is on it's base row it can move two squares.
if ((piececolour == BLACK && from[0] == BL_PAWN_BASEROW) || (piececolour == WHITE && from[0] == WH_PAWN_BASEROW))
{ if (piececolour == WHITE)
row++;
else
row--;
if ((board[row][col] == EMPTY) && (piecefound ==FALSE))
{ validmovemask[row] |= (1<<col);
}
}
}
VOID val_bishop(LOC from,BOOL piececolour)
// Find all valid moves for a bishop. We look at
// all 4 directions and travel until either the
// edge of the board or until a piece is encountered.
{
// Get the opponents king position.
if (piececolour == WHITE)
{ opp_king_pos[0] = bl_king_pos[0];
opp_king_pos[1] = bl_king_pos[1];
}
else
{ opp_king_pos[0] = wh_king_pos[0];
opp_king_pos[1] = wh_king_pos[1];
}
// Check Valid Moves.
val_northeast (from,piececolour,-1);
val_northwest (from,piececolour,-1);
val_southeast (from,piececolour,-1);
val_southwest (from,piececolour,-1);
}
VOID val_knight(LOC from,BOOL piececolour)
// Find all valid moves for a knight.We use a mask byte
// to flag available rows and columns.
// | 0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 |
// | +1r_up | +2r_up | +1r_dwn | +2r_dwn | +1col_lft| +2col_lft| +1col_rgt | +2col_rgt |
{ BYTE mask = 0,i = 0;
COORD row = 0,col = 0;
// Set up row and column offsets for the various knight moves. All moves are initialised to zero.
INT move[] = {0, 2, 1, 0, 2, -1, 0, 1, 2, 0, 1, -2, 0, -1, 2, 0, -1, -2, 0, -2, -1, 0, -2, 1};
// Get the opponents king position.
if (piececolour == WHITE)
{ opp_king_pos[0] = bl_king_pos[0];
opp_king_pos[1] = bl_king_pos[1];
}
else
{ opp_king_pos[0] = wh_king_pos[0];
opp_king_pos[1] = wh_king_pos[1];
}
// Set Bits to indicate how many rows we can move up or down.
switch (from[0])
{ case 0 : mask |= 0x03; break; // Two up - None down.
case 1 : mask |= 0x07; break; // Two up - One down.
case 6 : mask |= 0x0D; break; // One up - Two down.
case 7 : mask |= 0x0C; break; // None up - Two down.
case 2 :
case 3 :
case 4 :
case 5 : mask |= 0x0F; break; // Two up - Two down.
}
// Set Bits to indicate how many columns we can move left or right.
switch (from[1])
{ case 0 : mask |= 0xC0; break; // None left - Two right.
case 1 : mask |= 0xD0; break; // One left - Two right.
case 6 : mask |= 0x70; break; // Two left - One right.
case 7 : mask |= 0x30; break; // Two left - None right.
case 2 :
case 3 :
case 4 :
case 5 : mask |= 0xF0; break; // Two left - Two right.
}
// Define Boolean tests for each possible knight move.
#define up2_rgt1 ((mask & 0x40) && (mask & 0x02))
#define up2_lft1 ((mask & 0x10) && (mask & 0x02))
#define up1_rgt2 ((mask & 0x80) && (mask & 0x01))
#define up1_lft2 ((mask & 0x20) && (mask & 0x01))
#define dwn1_rgt2 ((mask & 0x80) && (mask & 0x04))
#define dwn1_lft2 ((mask & 0x20) && (mask & 0x04))
#define dwn2_lft1 ((mask & 0x10) && (mask & 0x08))
#define dwn2_rgt1 ((mask & 0x40) && (mask & 0x08))
// Set Valid moves in our array.
move[0] = up2_rgt1;
move[3] = up2_lft1;
move[6] = up1_rgt2;
move[9] = up1_lft2;
move[12] = dwn1_rgt2;
move[15] = dwn1_lft2;
move[18] = dwn2_lft1;
move[21] = dwn2_rgt1;
// Deal with all the possibles.
for (i = 0; i <= 21; i += 3)
{ if (move[i])
{ // Valid Move.
row = (from[0] + move[i+1]);
col = (from[1] + move[i+2]);
if (board[row][col] == EMPTY)
{ // Empty Square.
validmovemask[row] |= (1<<col);
}
else if (is_opp_piece(row,col,piececolour))
{ // Opponents Piece.
validmovemask[row] |= (1<<col);
capturemask[row] |= (1<<col);
if (capturemask[opp_king_pos[0]] & (1 << opp_king_pos[1])) kingcapture = TRUE;
}
}
}
}
VOID val_rook(LOC from,BOOL piececolour)
// Get all valid moves for a rook. We look
// at all 4 possible directions and extend until
// a piece is encountered.
{ // Get the opponents king position.
if (piececolour == WHITE)
{ opp_king_pos[0] = bl_king_pos[0];
opp_king_pos[1] = bl_king_pos[1];
}
else
{ opp_king_pos[0] = wh_king_pos[0];
opp_king_pos[1] = wh_king_pos[1];
}
// Get all valid Moves.
val_north (from,piececolour,-1);
val_south (from,piececolour,-1);
val_east (from,piececolour,-1);
val_west (from,piececolour,-1);
}
VOID val_queen(LOC from,BOOL piececolour)
// Calculate all valid moves for a queen.
// The Queen is in effect a rook and a bishop
// combined so all that is necessary here is to
// call all the directional validation functions.
{
// Get the opponents king position.
if (piececolour == WHITE)
{ opp_king_pos[0] = bl_king_pos[0];
opp_king_pos[1] = bl_king_pos[1];
}
else
{ opp_king_pos[0] = wh_king_pos[0];
opp_king_pos[1] = wh_king_pos[1];
}
// Get all Valid Moves.
val_north (from,piececolour,-1);
val_south (from,piececolour,-1);
val_east (from,piececolour,-1);
val_west (from,piececolour,-1);
val_northeast (from,piececolour,-1);
val_northwest (from,piece
没有合适的资源?快使用搜索试试~ 我知道了~
ATMEGA103+74LS138+74LS373+LCD+其它外围电路设计的游戏系统电路proteus仿真工程包含原理图+源代...
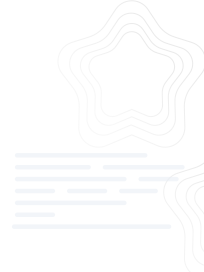
共7个文件
c:3个
pdsprj:1个
bin:1个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 141 浏览量
2024-12-14
09:41:41
上传
评论
收藏 55KB ZIP 举报
温馨提示
ATMEGA103+74LS138+74LS373+LCD+其它外围电路设计的游戏系统电路proteus仿真工程包含原理图+源代码100%可以仿真跑起来.zip
资源推荐
资源详情
资源评论
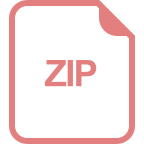
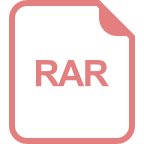
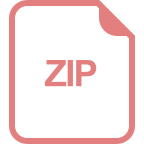
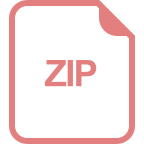
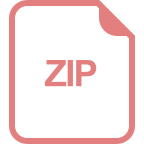
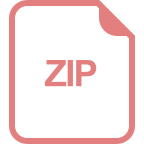
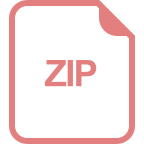
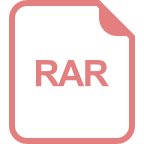
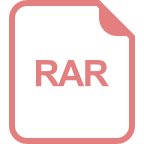
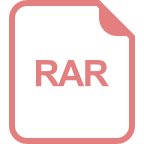
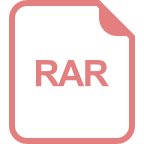
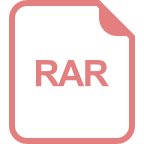
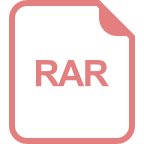
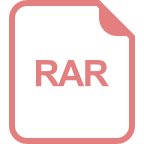
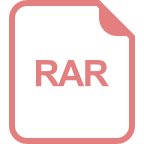
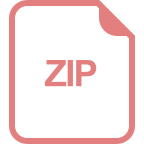
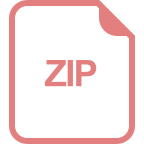
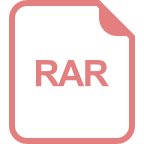
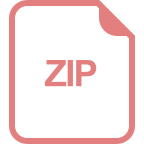
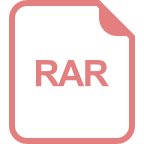
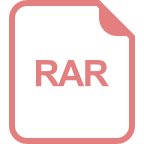
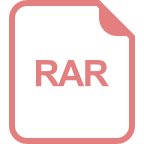
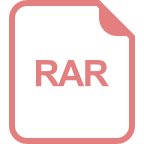
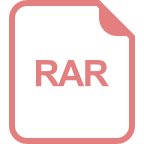
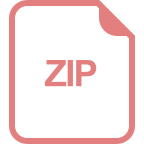
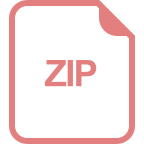
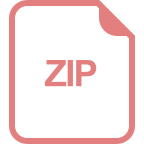
收起资源包目录


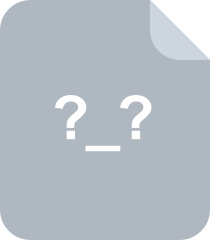
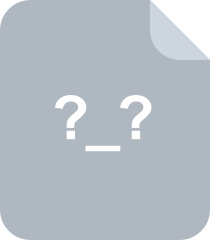
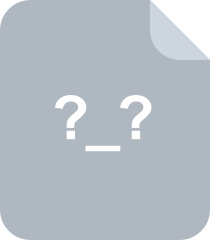
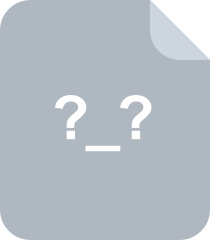
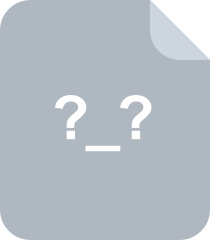
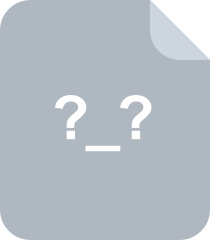
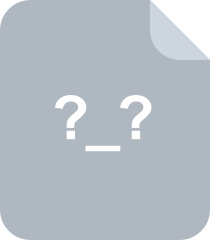
共 7 条
- 1
资源评论
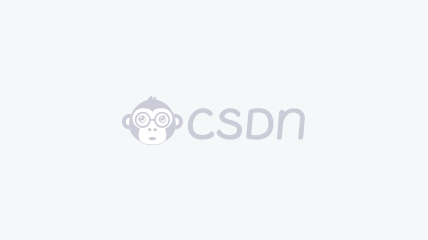
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

m0_70960708
- 粉丝: 612
- 资源: 2636
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

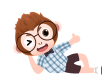
最新资源
- WPS、Word、PDF文档汇编工具
- 右键菜单-打开cmd.reg
- 牡蛎检测49-YOLO(v5至v9)、COCO、CreateML、Darknet、VOC数据集合集.rar
- 医学分割数据集白内障严重程度分割数据集labelme格式719张3类别.zip
- 非常好的单片机+74HC595+多路LED灯设计的流水灯电路proteus仿真工程100%好用.zip
- ruby-线程生命周期及其状态
- 基于java+ssm+mysq的计算机科学与技术学习网站的开题报告.docx
- 基于java+ssm+mysq的化妆品配方及工艺管理系统的开题报告.docx
- excel文件数据的读取
- 基于java+ssm+mysq的户管理系统的开题报告.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


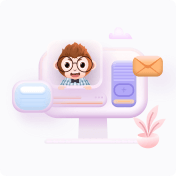
安全验证
文档复制为VIP权益,开通VIP直接复制
