!function(t,e){for(var n in e)t[n]=e[n]}(exports,function(t){var e={};function n(r){if(e[r])return e[r].exports;var i=e[r]={i:r,l:!1,exports:{}};return t[r].call(i.exports,i,i.exports,n),i.l=!0,i.exports}return n.m=t,n.c=e,n.d=function(t,e,r){n.o(t,e)||Object.defineProperty(t,e,{enumerable:!0,get:r})},n.r=function(t){"undefined"!=typeof Symbol&&Symbol.toStringTag&&Object.defineProperty(t,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(t,"__esModule",{value:!0})},n.t=function(t,e){if(1&e&&(t=n(t)),8&e)return t;if(4&e&&"object"==typeof t&&t&&t.__esModule)return t;var r=Object.create(null);if(n.r(r),Object.defineProperty(r,"default",{enumerable:!0,value:t}),2&e&&"string"!=typeof t)for(var i in t)n.d(r,i,function(e){return t[e]}.bind(null,i));return r},n.n=function(t){var e=t&&t.__esModule?function(){return t.default}:function(){return t};return n.d(e,"a",e),e},n.o=function(t,e){return Object.prototype.hasOwnProperty.call(t,e)},n.p="",n(n.s=4)}([function(t,e,n){"use strict";const r=n(1),i=n(2);t.exports={atob:r,btoa:i}},function(t,e,n){"use strict";function r(t){return/[A-Z]/.test(t)?t.charCodeAt(0)-"A".charCodeAt(0):/[a-z]/.test(t)?t.charCodeAt(0)-"a".charCodeAt(0)+26:/[0-9]/.test(t)?t.charCodeAt(0)-"0".charCodeAt(0)+52:"+"===t?62:"/"===t?63:void 0}t.exports=function(t){if((t=(t=`${t}`).replace(/[ \t\n\f\r]/g,"")).length%4==0&&(t=t.replace(/==?$/,"")),t.length%4==1||/[^+/0-9A-Za-z]/.test(t))return null;let e="",n=0,i=0;for(let a=0;a<t.length;a++)n<<=6,n|=r(t[a]),24===(i+=6)&&(e+=String.fromCharCode((16711680&n)>>16),e+=String.fromCharCode((65280&n)>>8),e+=String.fromCharCode(255&n),n=i=0);return 12===i?(n>>=4,e+=String.fromCharCode(n)):18===i&&(n>>=2,e+=String.fromCharCode((65280&n)>>8),e+=String.fromCharCode(255&n)),e}},function(t,e,n){"use strict";function r(t){return t<26?String.fromCharCode(t+"A".charCodeAt(0)):t<52?String.fromCharCode(t-26+"a".charCodeAt(0)):t<62?String.fromCharCode(t-52+"0".charCodeAt(0)):62===t?"+":63===t?"/":void 0}t.exports=function(t){let e;for(t=`${t}`,e=0;e<t.length;e++)if(t.charCodeAt(e)>255)return null;let n="";for(e=0;e<t.length;e+=3){const i=[void 0,void 0,void 0,void 0];i[0]=t.charCodeAt(e)>>2,i[1]=(3&t.charCodeAt(e))<<4,t.length>e+1&&(i[1]|=t.charCodeAt(e+1)>>4,i[2]=(15&t.charCodeAt(e+1))<<2),t.length>e+2&&(i[2]|=t.charCodeAt(e+2)>>6,i[3]=63&t.charCodeAt(e+2));for(let t=0;t<i.length;t++)void 0===i[t]?n+="=":n+=r(i[t])}return n}},function(t,e){(function(e){t.exports=e}).call(this,{})},function(t,e,n){"use strict";n.r(e);var r=n(0);function i(t,e){for(var n=0;n<e.length;n++){var r=e[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(t,r.key,r)}}var a=new WeakMap,o=function(){function t(){!function(t,e){if(!(t instanceof e))throw new TypeError("Cannot call a class as a function")}(this,t),a.set(this,{})}var e,n,r;return e=t,(n=[{key:"addEventListener",value:function(t,e){var n=arguments.length>2&&void 0!==arguments[2]?arguments[2]:{},r=a.get(this);r||(r={},a.set(this,r)),r[t]||(r[t]=[]),r[t].push(e),n.capture,n.once,n.passive}},{key:"removeEventListener",value:function(t,e){var n=a.get(this);if(n){var r=n[t];if(r&&r.length>0)for(var i=r.length;i--;i>0)if(r[i]===e){r.splice(i,1);break}}}},{key:"dispatchEvent",value:function(){var t=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{},e=a.get(this)[t.type];if(e)for(var n=0;n<e.length;n++)e[n](t)}}])&&i(e.prototype,n),r&&i(e,r),t}();function s(t){return(s="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(t){return typeof t}:function(t){return t&&"function"==typeof Symbol&&t.constructor===Symbol&&t!==Symbol.prototype?"symbol":typeof t})(t)}function c(t,e){for(var n=0;n<e.length;n++){var r=e[n];r.enumerable=r.enumerable||!1,r.configurable=!0,"value"in r&&(r.writable=!0),Object.defineProperty(t,r.key,r)}}function l(t){return(l=Object.setPrototypeOf?Object.getPrototypeOf:function(t){return t.__proto__||Object.getPrototypeOf(t)})(t)}function h(t){if(void 0===t)throw new ReferenceError("this hasn't been initialised - super() hasn't been called");return t}function u(t,e){return(u=Object.setPrototypeOf||function(t,e){return t.__proto__=e,t})(t,e)}var p=new WeakMap,d=new WeakMap,f=new WeakMap;function m(t){var e=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{};e.target=e.target||this,"function"==typeof this["on".concat(t)]&&this["on".concat(t)].call(this,e)}function v(t){var e=arguments.length>1&&void 0!==arguments[1]?arguments[1]:{};this.readyState=t,e.readyState=t,m.call(this,"readystatechange",e)}function g(t){return!/^(http|https|ftp|wxfile):\/\/.*/i.test(t)}var y=function(t){function e(){var t;return function(t,e){if(!(t instanceof e))throw new TypeError("Cannot call a class as a function")}(this,e),(t=function(t,e){return!e||"object"!==s(e)&&"function"!=typeof e?h(t):e}(this,l(e).call(this))).onabort=null,t.onerror=null,t.onload=null,t.onloadstart=null,t.onprogress=null,t.ontimeout=null,t.onloadend=null,t.onreadystatechange=null,t.readyState=0,t.response=null,t.responseText=null,t.responseType="text",t.dataType="string",t.responseXML=null,t.status=0,t.statusText="",t.upload={},t.withCredentials=!1,p.set(h(t),{"content-type":"application/x-www-form-urlencoded"}),d.set(h(t),{}),t}var n,r,i;return function(t,e){if("function"!=typeof e&&null!==e)throw new TypeError("Super expression must either be null or a function");t.prototype=Object.create(e&&e.prototype,{constructor:{value:t,writable:!0,configurable:!0}}),e&&u(t,e)}(e,t),n=e,(r=[{key:"abort",value:function(){var t=f.get(this);t&&t.abort()}},{key:"getAllResponseHeaders",value:function(){var t=d.get(this);return Object.keys(t).map((function(e){return"".concat(e,": ").concat(t[e])})).join("\n")}},{key:"getResponseHeader",value:function(t){return d.get(this)[t]}},{key:"open",value:function(t,n){this._method=t,this._url=n,v.call(this,e.OPENED)}},{key:"overrideMimeType",value:function(){}},{key:"send",value:function(){var t=this,n=arguments.length>0&&void 0!==arguments[0]?arguments[0]:"";if(this.readyState!==e.OPENED)throw new Error("Failed to execute 'send' on 'XMLHttpRequest': The object's state must be OPENED.");var r,i=this._url,a=p.get(this),o=this.responseType,s=this.dataType,c=g(i);"arraybuffer"===o||(r="utf8"),delete this.response,this.response=null;var l=function(n){var r=n.data,i=n.statusCode,a=n.header;if(i=void 0===i?200:i,"string"!=typeof r&&!(r instanceof ArrayBuffer))try{r=JSON.stringify(r)}catch(t){}t.status=i,a&&d.set(t,a),m.call(t,"loadstart"),v.call(t,e.HEADERS_RECEIVED),v.call(t,e.LOADING),t.response=r,r instanceof ArrayBuffer?Object.defineProperty(t,"responseText",{enumerable:!0,configurable:!0,get:function(){throw"InvalidStateError : responseType is "+this.responseType}}):t.responseText=r,v.call(t,e.DONE),m.call(t,"load"),m.call(t,"loadend")},h=function(e){var n=e.errMsg;-1!==n.indexOf("abort")?m.call(t,"abort"):m.call(t,"error",{message:n}),m.call(t,"loadend"),c&&console.warn(n)};if(c){var u=wx.getFileSystemManager(),f={filePath:i,success:l,fail:h};return r&&(f.encoding=r),void u.readFile(f)}wx.request({data:n,url:i,method:this._method,header:a,dataType:s,responseType:o,success:l,fail:h})}},{key:"setRequestHeader",value:function(t,e){var n=p.get(this);n[t]=e,p.set(this,n)}},{key:"addEventListener",value:function(t,e){var n=this;"function"==typeof e&&(this["on"+t]=function(){var t=arguments.length>0&&void 0!==arguments[0]?arguments[0]:{};t.target=t.target||n,e.call(n,t)})}},{key:"removeEventListener",value:function(t,e){this["on"+t]===e&&(this["on"+t]=null)}}])&&c(n.prototype,r),i&&c(n,i),e}(o);function x(t){return(x="function"==typeof Symbol&&"symbol"==typeof Symbol.iterator?function(t){return typeof t}:function(t){return t&&"function"==typeof Symbol&&t.constructor===Symbol&&t!==Symbol.prototype?"symbol":typeof t})(t)}function b(e){e.style={width:e.width+"px",height:e.height+"px"},e.addEventListener=function(){},e.removeEventListener=function(){};var i,a,o={createElementNS:function(t,n){return"canvas"===n?e:"img"===

飞影铠甲
- 粉丝: 4847
- 资源: 219
最新资源
- 蚁群算法小程序-matlab
- 粒子群算法小程序-matlab
- 《新能源接入的电力市场主辅联合出清》 出清模型以考虑安全约束的机组组合模型(SCUC)和经济调度模型(SCED)组成 程序基于IEEE30节点编写,并接入风电机组参与电力市场,辅助服务市场为备用市场
- 个人创作原画作品,禁止盗用
- 遗传算法程序-matlab
- 游戏人物检测15-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- Windows 7安装NET补丁
- 高动态导航技术全套技术资料.zip
- cms测试练习项目(linux系统部署)
- 游戏人物检测15-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord数据集合集.rar
- 名城小区物业管理-JAVA-基于Spring boot的名城小区物业管理系统设计实现(毕业论文+开题)
- 多媒体素材库-JAVA-基于springboot的多媒体素材库的开发与应用(毕业论文)
- 大学生心理健康管理-JAVA-基于springBoot大学生心理健康管理系统的设计与实现(毕业论文)
- 论坛系统-JAVA-基于SpringBoot的论坛系统设计与实现(毕业论文+开题+PPT)
- 游戏人物检测17-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 大学生智能消费记账-JAVA-springboot205大学生智能消费记账系统的设计与实现(毕业论文)
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


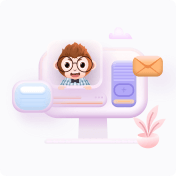