% Copyright �2010. The Regents of the University of California (Regents).
% All Rights Reserved. Contact The Office of Technology Licensing,
% UC Berkeley, 2150 Shattuck Avenue, Suite 510, Berkeley, CA 94720-1620,
% (510) 643-7201, for commercial licensing opportunities.
% Authors: Arvind Ganesh, Allen Y. Yang, Zihan Zhou.
% Contact: Allen Y. Yang, Department of EECS, University of California,
% Berkeley. <yang@eecs.berkeley.edu>
% IN NO EVENT SHALL REGENTS BE LIABLE TO ANY PARTY FOR DIRECT, INDIRECT,
% SPECIAL, INCIDENTAL, OR CONSEQUENTIAL DAMAGES, INCLUDING LOST PROFITS,
% ARISING OUT OF THE USE OF THIS SOFTWARE AND ITS DOCUMENTATION, EVEN IF
% REGENTS HAS BEEN ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
% REGENTS SPECIFICALLY DISCLAIMS ANY WARRANTIES, INCLUDING, BUT NOT LIMITED
% TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A
% PARTICULAR PURPOSE. THE SOFTWARE AND ACCOMPANYING DOCUMENTATION, IF ANY,
% PROVIDED HEREUNDER IS PROVIDED "AS IS". REGENTS HAS NO OBLIGATION TO
% PROVIDE MAINTENANCE, SUPPORT, UPDATES, ENHANCEMENTS, OR MODIFICATIONS.
%% This function is modified from Matlab Package SpaRSA
function [x,iter]= SolveSpaRSA(A,y,varargin)
% SpaRSA version 2.0, December 31, 2007
%
% This function solves the convex problem
%
% arg min_x = 0.5*|| y - A x ||_2^2 + lambda phi(x)
%
% using the SpaRSA algorithm, which is described in "Sparse Reconstruction
% by Separable Approximation" by S. Wright, R. Nowak, M. Figueiredo,
% IEEE Transactions on Signal Processing, 2009 (to appear).
%
% The algorithm is related GPSR (Figueiredo, Nowak, Wright) but does not
% rely on the conversion to QP form of the l1 norm, because it is not
% limited to being used with l1 regularization. Instead it forms a
% separable
% approximation to the first term of the objective, which has the form
%
% d'*A'*(A x - y) + 0.5*alpha*d'*d
%
% where alpha is obtained from a BB formula. In a monotone variant, alpha is
% increased until we see a decreasein the original objective function over
% this step.
%
% -----------------------------------------------------------------------
% Copyright (2007): Mario Figueiredo, Robert Nowak, Stephen Wright
%
% GPSR is distributed under the terms
% of the GNU General Public License 2.0.
%
% Permission to use, copy, modify, and distribute this software for
% any purpose without fee is hereby granted, provided that this entire
% notice is included in all copies of any software which is or includes
% a copy or modification of this software and in all copies of the
% supporting documentation for such software.
% This software is being provided "as is", without any express or
% implied warranty. In particular, the authors do not make any
% representation or warranty of any kind concerning the merchantability
% of this software or its fitness for any particular purpose."
% ----------------------------------------------------------------------
%
% Please check for the latest version of the code and paper at
% www.lx.it.pt/~mtf/SpaRSA
%
% ===== Required inputs =============
%
% y: 1D vector or 2D array (image) of observations
%
% A: if y and x are both 1D vectors, A can be a
% k*n (where k is the size of y and n the size of x)
% matrix or a handle to a function that computes
% products of the form A*v, for some vector v.
% In any other case (if y and/or x are 2D arrays),
% A has to be passed as a handle to a function which computes
% products of the form A*x; another handle to a function
% AT which computes products of the form A'*x is also required
% in this case. The size of x is determined as the size
% of the result of applying AT.
%
% lambda: regularization parameter (scalar)
%
% ===== Optional inputs =============
%
%
% 'AT' = function handle for the function that implements
% the multiplication by the conjugate of A, when A
% is a function handle. If A is an array, AT is ignored.
%
% 'Psi' = handle to the denoising function, that is, to a function
% that computes the solution of the densoing probelm
% corresponding to the desired regularizer. That is,
% Psi(y,lambda) = arg min_x (1/2)*(x - y)^2 + lambda phi(x).
% Default: in the absence of any Phi given by the user,
% it is assumed that phi(x) = ||x||_1 thus
% Psi(y,lambda) = soft(y,lambda)
% Important: if Psi is given, phi must also be given,
% so that the algorithm may also compute
% the objective function.
%
% 'StopCriterion' = type of stopping criterion to use
% 0 = algorithm stops when the relative
% change in the number of non-zero
% components of the estimate falls
% below 'tolerance'
% 1 = stop when the relative
% change in the objective function
% falls below 'tolerance'
% 2 = stop when relative duality gap
% falls below 'tolerance'
% 3 = stop when relative noise magnitude
% falls below 'tolerance'
% 4 = stop when the objective function
% becomes equal or less than toleranceA.
% Default = 3
%
% 'Tolerance' = stopping threshold; Default = 0.01
%
% 'Debias' = debiasing option: 1 = yes, 0 = no.
% Default = 0.
%
% 'ToleranceD' = stopping threshold for the debiasing phase:
% Default = 0.0001.
% If no debiasing takes place, this parameter,
% if present, is ignored.
%
% 'MaxiterA' = maximum number of iterations allowed in the
% main phase of the algorithm.
% Default = 1000
%
% 'MiniterA' = minimum number of iterations performed in the
% main phase of the algorithm.
% Default = 5
%
% 'MaxiterD' = maximum number of iterations allowed in the
% debising phase of the algorithm.
% Default = 200
%
% 'MiniterD' = minimum number of iterations to perform in the
% debiasing phase of the algorithm.
% Default = 5
%
% 'Initialization' must be one of {0,1,2,array}
% 0 -> Initialization at zero.
% 1 -> Random initialization.
% 2 -> initialization with A'*y.
% array -> initialization provided by the user.
% Default = 0;
%
% 'BB_variant' specifies which variant of Barzila-Borwein to use, or not.
% 0 -> don't use a BB rule - instead pick the starting alpha
% based on the successful value at the previous iteration
% 1 -> standard BB choice s'r/s's
% 2 -> inverse BB variant r'r/r's
% Default = 1
%
% 'BB_cycle' specifies the cycle length - the number of iterations between
% recalculation of alpha. Requires integer value at least
% 1. Relevant only if a **nonmonotone BB rule** is used
% (BB_variant = 1 or 2 and Monotone=0).
% Default = 1
%
% 'Monotone' = enforce monotonic decrease in f, or not?
% any nonzero -> enforce monotonicity (overrides 'Safeguard')
% 0 -> don't enforce monotonicity.
% Default = 0;
%
% 'Safeguard' = enforce a "sufficient decrease" over the largest
% objective value of the past M iterations.
% any nonzero -> safeguard
% 0 -> don't safeguard
% Default = 0.
%
% 'M' = number of steps to look back in the safeguarding process.
% Ignored if Safeguard=0 or if Monotone is nonzero.
% (positive integer. Default = 5)
%
% 'sigma' = sigma value used in Safeguarding test for sufficient
% decrease. Ignored unless 'Safeguard' is nonzero. Must be
% in (0,1). Drfault: .01
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
【达摩老生出品,必属精品,亲测校正,质量保证】 资源名:稀疏表示人脸识别核心问题L1范数源代码_稀疏表示_人脸识别_L1范数_matlab 资源类型:matlab项目全套源码 源码说明: 全部项目源码都是经过测试校正后百分百成功运行的,如果您下载后不能运行可联系我进行指导或者更换。 适合人群:新手及有一定经验的开发人员
资源推荐
资源详情
资源评论
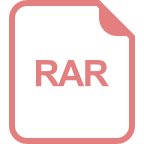
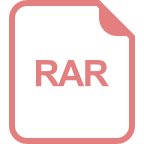
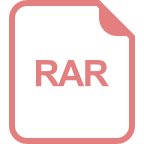
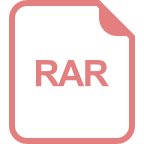
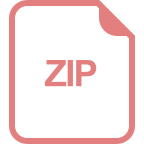
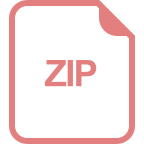
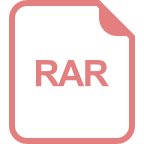
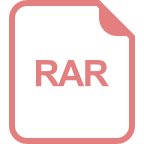
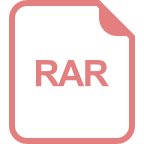
收起资源包目录


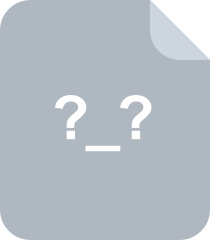

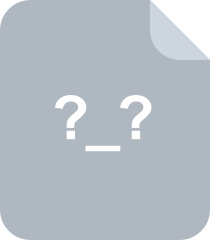
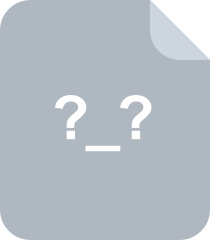
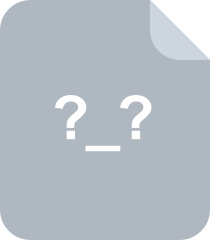

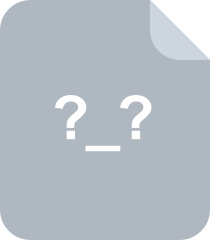
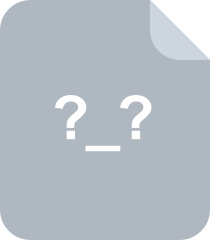
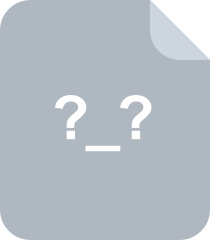
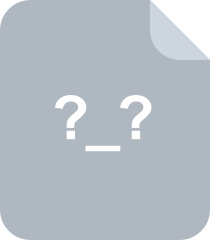
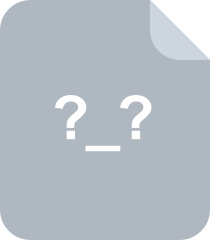
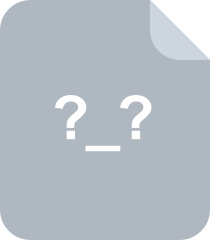
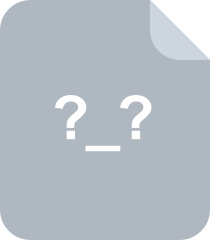
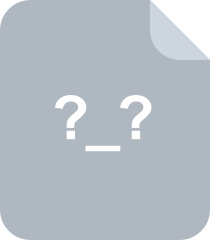
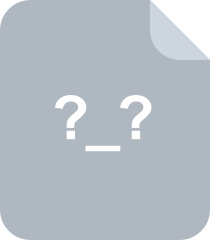
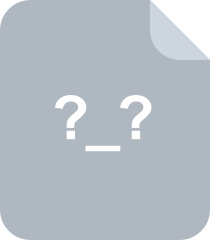
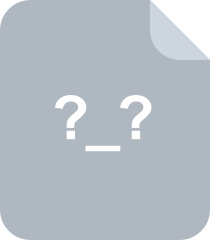
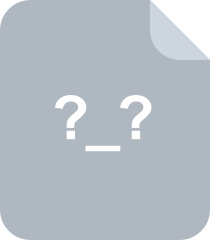


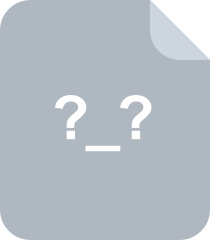
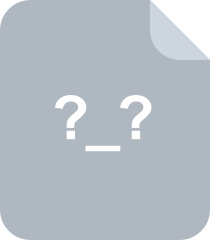

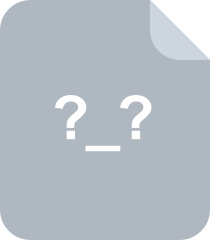
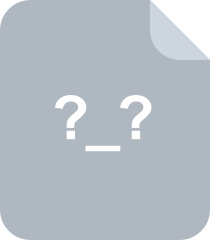
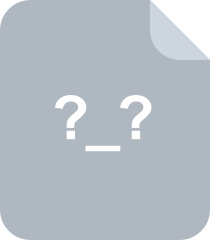
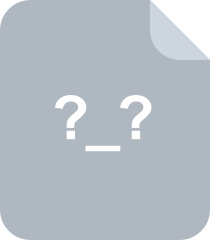
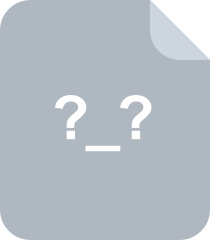
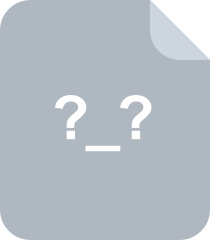
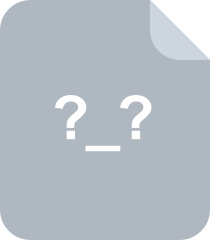
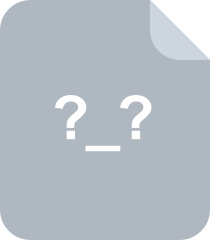
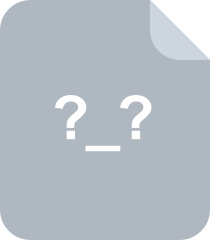
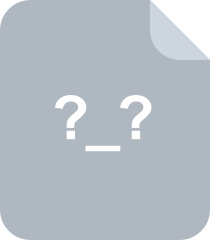
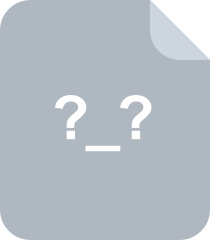
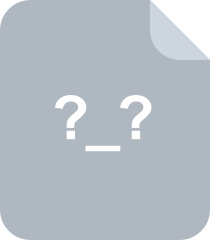

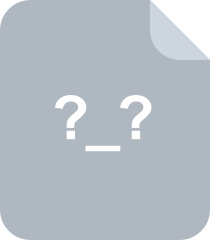
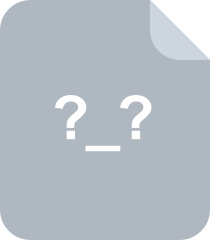
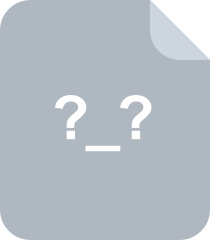
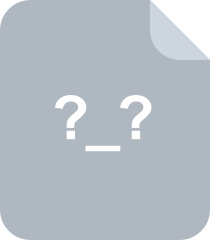
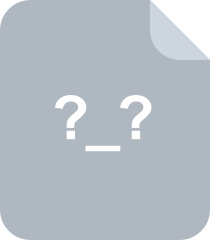
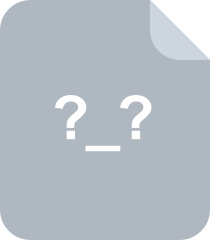
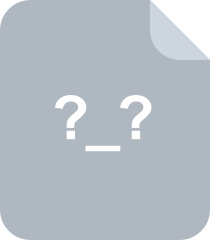
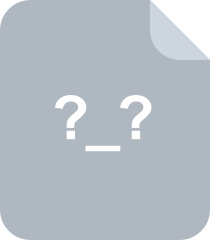
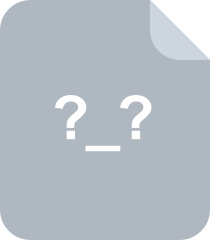
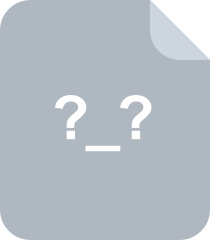
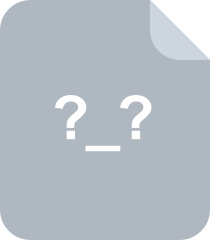
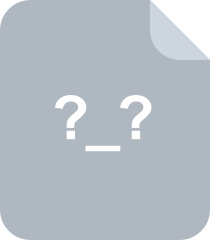
共 42 条
- 1
资源评论
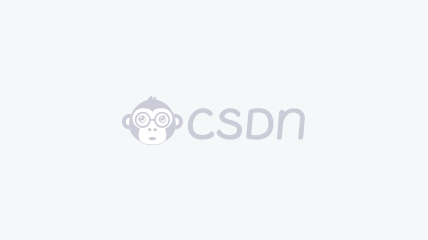
- you_ha1112022-12-11感谢大佬分享的资源,对我启发很大,给了我新的灵感。
- 武佳可2023-10-24资源内容总结的很到位,内容详实,很受用,学到了~
- SOZ1012023-01-20果断支持这个资源,资源解决了当前遇到的问题,给了新的灵感,感谢分享~
- ggbond_22023-11-17资源有很好的参考价值,总算找到了自己需要的资源啦。
- LonelyBoy11032023-06-01这个资源值得下载,资源内容详细全面,与描述一致,受益匪浅。


阿里matlab建模师
- 粉丝: 3731
- 资源: 2812

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

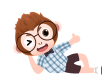
最新资源
- Facebook.apk
- 推荐一款JTools的call-this-method插件
- json的合法基色来自红包东i请各位
- 项目采用YOLO V4算法模型进行目标检测,使用Deep SORT目标跟踪算法 .zip
- 针对实时视频流和静态图像实现的对象检测和跟踪算法 .zip
- 部署 yolox 算法使用 deepstream.zip
- 基于webmagic、springboot和mybatis的MagicToe Java爬虫设计源码
- 通过实时流协议 (RTSP) 使用 Yolo、OpenCV 和 Python 进行深度学习的对象检测.zip
- 基于Python和HTML的tb商品列表查询分析设计源码
- 基于国民技术RT-THREAD的MULTInstrument多功能电子测量仪器设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


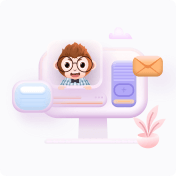
安全验证
文档复制为VIP权益,开通VIP直接复制
