/**
* @license
* Video.js 7.8.4 <http://videojs.com/>
* Copyright Brightcove, Inc. <https://www.brightcove.com/>
* Available under Apache License Version 2.0
* <https://github.com/videojs/video.js/blob/master/LICENSE>
*
* Includes vtt.js <https://github.com/mozilla/vtt.js>
* Available under Apache License Version 2.0
* <https://github.com/mozilla/vtt.js/blob/master/LICENSE>
*/
(function (global, factory) {
typeof exports === 'object' && typeof module !== 'undefined' ? module.exports = factory(require('global/window'), require('global/document')) :
typeof define === 'function' && define.amd ? define(['global/window', 'global/document'], factory) :
(global = global || self, global.videojs = factory(global.window, global.document));
}(this, (function (window$3, document) { 'use strict';
window$3 = window$3 && Object.prototype.hasOwnProperty.call(window$3, 'default') ? window$3['default'] : window$3;
document = document && Object.prototype.hasOwnProperty.call(document, 'default') ? document['default'] : document;
var version = "7.8.4";
/**
* @file create-logger.js
* @module create-logger
*/
var history = [];
/**
* Log messages to the console and history based on the type of message
*
* @private
* @param {string} type
* The name of the console method to use.
*
* @param {Array} args
* The arguments to be passed to the matching console method.
*/
var LogByTypeFactory = function LogByTypeFactory(name, log) {
return function (type, level, args) {
var lvl = log.levels[level];
var lvlRegExp = new RegExp("^(" + lvl + ")$");
if (type !== 'log') {
// Add the type to the front of the message when it's not "log".
args.unshift(type.toUpperCase() + ':');
} // Add console prefix after adding to history.
args.unshift(name + ':'); // Add a clone of the args at this point to history.
if (history) {
history.push([].concat(args)); // only store 1000 history entries
var splice = history.length - 1000;
history.splice(0, splice > 0 ? splice : 0);
} // If there's no console then don't try to output messages, but they will
// still be stored in history.
if (!window$3.console) {
return;
} // Was setting these once outside of this function, but containing them
// in the function makes it easier to test cases where console doesn't exist
// when the module is executed.
var fn = window$3.console[type];
if (!fn && type === 'debug') {
// Certain browsers don't have support for console.debug. For those, we
// should default to the closest comparable log.
fn = window$3.console.info || window$3.console.log;
} // Bail out if there's no console or if this type is not allowed by the
// current logging level.
if (!fn || !lvl || !lvlRegExp.test(type)) {
return;
}
fn[Array.isArray(args) ? 'apply' : 'call'](window$3.console, args);
};
};
function createLogger(name) {
// This is the private tracking variable for logging level.
var level = 'info'; // the curried logByType bound to the specific log and history
var logByType;
/**
* Logs plain debug messages. Similar to `console.log`.
*
* Due to [limitations](https://github.com/jsdoc3/jsdoc/issues/955#issuecomment-313829149)
* of our JSDoc template, we cannot properly document this as both a function
* and a namespace, so its function signature is documented here.
*
* #### Arguments
* ##### *args
* Mixed[]
*
* Any combination of values that could be passed to `console.log()`.
*
* #### Return Value
*
* `undefined`
*
* @namespace
* @param {Mixed[]} args
* One or more messages or objects that should be logged.
*/
var log = function log() {
for (var _len = arguments.length, args = new Array(_len), _key = 0; _key < _len; _key++) {
args[_key] = arguments[_key];
}
logByType('log', level, args);
}; // This is the logByType helper that the logging methods below use
logByType = LogByTypeFactory(name, log);
/**
* Create a new sublogger which chains the old name to the new name.
*
* For example, doing `videojs.log.createLogger('player')` and then using that logger will log the following:
* ```js
* mylogger('foo');
* // > VIDEOJS: player: foo
* ```
*
* @param {string} name
* The name to add call the new logger
* @return {Object}
*/
log.createLogger = function (subname) {
return createLogger(name + ': ' + subname);
};
/**
* Enumeration of available logging levels, where the keys are the level names
* and the values are `|`-separated strings containing logging methods allowed
* in that logging level. These strings are used to create a regular expression
* matching the function name being called.
*
* Levels provided by Video.js are:
*
* - `off`: Matches no calls. Any value that can be cast to `false` will have
* this effect. The most restrictive.
* - `all`: Matches only Video.js-provided functions (`debug`, `log`,
* `log.warn`, and `log.error`).
* - `debug`: Matches `log.debug`, `log`, `log.warn`, and `log.error` calls.
* - `info` (default): Matches `log`, `log.warn`, and `log.error` calls.
* - `warn`: Matches `log.warn` and `log.error` calls.
* - `error`: Matches only `log.error` calls.
*
* @type {Object}
*/
log.levels = {
all: 'debug|log|warn|error',
off: '',
debug: 'debug|log|warn|error',
info: 'log|warn|error',
warn: 'warn|error',
error: 'error',
DEFAULT: level
};
/**
* Get or set the current logging level.
*
* If a string matching a key from {@link module:log.levels} is provided, acts
* as a setter.
*
* @param {string} [lvl]
* Pass a valid level to set a new logging level.
*
* @return {string}
* The current logging level.
*/
log.level = function (lvl) {
if (typeof lvl === 'string') {
if (!log.levels.hasOwnProperty(lvl)) {
throw new Error("\"" + lvl + "\" in not a valid log level");
}
level = lvl;
}
return level;
};
/**
* Returns an array containing everything that has been logged to the history.
*
* This array is a shallow clone of the internal history record. However, its
* contents are _not_ cloned; so, mutating objects inside this array will
* mutate them in history.
*
* @return {Array}
*/
log.history = function () {
return history ? [].concat(history) : [];
};
/**
* Allows you to filter the history by the given logger name
*
* @param {string} fname
* The name to filter by
*
* @return {Array}
* The filtered list to return
*/
log.history.filter = function (fname) {
return (history || []).filter(function (historyItem) {
// if the first item in each historyItem includes `fname`, then it's a match
return new RegExp(".*" + fname + ".*").test(historyItem[0]);
});
};
/**
* Clears the internal history tracking, but does not prevent further history
* tracking.
*/
log.history.clear = function () {
if (history) {
history.length = 0;
}
};
/**
* Disable history tracking if it is currently enabled.
*/
log.history.disable = function () {
if (history !== null) {
history.length = 0;
history = null;
}
};
/**
* Enable history tracking if it is currently disabled.
*/
log.history.enable = function () {
if (history === null) {
history = [];
}

岛上程序猿
- 粉丝: 5817
- 资源: 4248
最新资源
- 理工大学本科毕业设计-基于深度学习的行人重识别系统python源码.zip
- 爱普生L301-L111-L211-L303-L351-L353-L358打印机清零工具
- 源码-相见恨晚的 Python 项目打包工具
- 现代社会最赚钱的十种方式-这也许是你跨越阶层的一次机会
- 惯导里程计GPS组合导航算法,matlab代码卡尔曼滤波
- asmarty3.1中文手册chm版最新版本
- LLM 友好的异步爬虫框架
- jjd.txt顶顶顶顶顶顶顶顶顶顶
- Matlab搭建双输入深度学习模型,双输入网络 相比普通的单输入网络,双输入网络能处理两种输入数据,在科研上也更具有优势和创新性 如何用Matlab搭建双输入网络也是困扰本人很长时间的一个问题,现
- VMD-SSA-BILSTM基于变分模态分解和麻雀算法优化的双向长短期记忆网络多维时间序列预测MATLAB代码(含BILSTM、VMD-BILSTM、VMD-SSA-BILSTM三个模型的对比)
- AndroidTooapk签名工具2025
- 离散化两电平逆变器并网仿真,图1对电路参数进行了说明,并网电压电流正常
- 永磁同步电机转速滑模控制Matlab simulink仿真模型,参数已设置好,可直接运行 属于PMSM转速电流双闭环矢量控制系统 电流内环采用PI控制器,转速外环采用滑模控制 波形完美,包含原理
- 数字图像信号处理综合应用系统matlab(MATLAB各类gui图像处理应用),可以实现对图像的读入与保存、鼠标截取需要的区域并对该区域进行各种几何变(包括添加高斯、椒盐、乘性噪声,进行时域的均值和中
- RMBG-2-Studio V2.0 抠图工具,支持批处理,毛发丝轻松扣.mp4
- Matching Anything by Segmenting Anything gits依赖
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


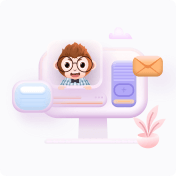