package com.lyq.action.product;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.util.HashMap;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import org.apache.struts2.ServletActionContext;
import org.springframework.context.annotation.Scope;
import org.springframework.stereotype.Controller;
import com.lyq.action.BaseAction;
import com.lyq.model.PageModel;
import com.lyq.model.product.ProductCategory;
import com.lyq.model.product.ProductInfo;
import com.lyq.model.product.UploadFile;
import com.lyq.util.StringUitl;
import com.opensymphony.xwork2.ModelDriven;
/**
* 商品Action
* @author Li Yongqiang
*/
@Scope("prototype")
@Controller("productAction")
public class ProductAction extends BaseAction implements ModelDriven<ProductInfo>{
private static final long serialVersionUID = 1L;
/**
* 根据id查看商品信息(查看后更新人气点击次数)
* @return String
* @throws Exception
*/
public String select() throws Exception {
if(product.getId() != null && product.getId() > 0){
product = productDao.get(product.getId());
product.setClickcount(product.getClickcount() + 1);
productDao.update(product);
}
return SELECT;
}
/**
* 根据类别id查询所有商品信息
* @return String
* @throws Exception
*/
public String getByCategoryId() throws Exception{
if(product.getCategory().getId() != null){
String where = "where category.id = ?";
Object[] queryParams = {product.getCategory().getId()};
pageModel = productDao.find(pageNo, pageSize, where, queryParams);
}
return LIST;
}
/**
* 新品上市
* @return
* @throws Exception
*/
public String findNewProduct() throws Exception{
Map<String, String> orderby = new HashMap<String, String>();//定义Map集合
orderby.put("createTime", "desc");//为Map集合赋值
pageModel = productDao.find(1, 5, orderby );//执行查找方法
image.put("url", "01.gif");//设置副标题图片
return "list";//返回商品列表页面
}
/**
* 热销商品
* @return
* @throws Exception
*/
public String findSellProduct() throws Exception{
Map<String, String> orderby = new HashMap<String, String>();//定义Map集合
orderby.put("sellCount", "desc");//为Map集合赋值
pageModel = productDao.find(1, 5, orderby );//执行查找方法
image.put("url", "03.gif");
return "list";//返回商品列表页面
}
/**
* 推荐商品
* @return
* @throws Exception
*/
public String findCommendProduct() throws Exception{
Map<String, String> orderby = new HashMap<String, String>();//定义Map集合
orderby.put("createTime", "desc");//为Map集合赋值
String where = "where commend = ?";//设置条件语句
Object[] queryParams = {true};//设置参数值
pageModel = productDao.find(where, queryParams, orderby, 1, 5);//执行查询方法
image.put("url", "06.gif");
return "list";//返回商品列表页面
}
/**
* 人气商品
* @return
* @throws Exception
*/
public String findEnjoyProduct() throws Exception{
Map<String, String> orderby = new HashMap<String, String>();//定义Map集合
orderby.put("clickcount", "desc");//为Map集合赋值
pageModel = productDao.find(1, 5, orderby );//执行查找方法
image.put("url", "07.gif");
return "list";//返回商品列表页面
}
/**
* 根据名称模糊查询
* @return String
* @throws Exception
*/
public String findByName() throws Exception {
if(product.getName() != null){
String where = "where name like ?";//查询的条件语句
Object[] queryParams = {"%" + product.getName() + "%"};//为参数赋值
pageModel = productDao.find(pageNo, pageSize, where, queryParams );//执行查询方法
}
image.put("url", "04.gif");
return LIST;//返回列表首页
}
/**
* 按人气查询
* @return String
* @throws Exception
*/
public String findByClick() throws Exception{
Map<String, String> orderby = new HashMap<String, String>();//定义Map集合
orderby.put("clickcount", "desc");//为Map集合赋值
pageModel = productDao.find(1, 8, orderby );//执行查找方法
return "clickList";//返回product_click_list.jsp页面
}
/**
* 按推荐查询
* @return String
* @throws Exception
*/
public String findByCommend() throws Exception{
Map<String, String> orderby = new HashMap<String, String>();//定义Map集合
orderby.put("sellCount", "desc");//为Map集合赋值
String where = "where commend = ?";//设置条件语句
Object[] queryParams = {true};//设置参数值
pageModel = productDao.find(where, queryParams, orderby, pageNo, pageSize);//执行查询方法
return "findList";//返回product_find_list.jsp页面
}
/**
* 按销量查询
* @return String
* @throws Exception
*/
public String findBySellCount() throws Exception{
Map<String, String> orderby = new HashMap<String, String>();//定义Map集合
orderby.put("sellCount", "desc");//为Map集合赋值
pageModel = productDao.find(1, 6, orderby );//执行查询方法
return "findList";//返回热销商品列表
}
/**
* 添加商品
*/
@Override
public String add() throws Exception {
createCategoryTree();
return INPUT;
}
/**
* 保存商品
* @return
* @throws Exception
*/
public String save() throws Exception{
if(file != null ){//如果文件路径不为空
//获取服务器的绝对路径
String path = ServletActionContext.getServletContext().getRealPath("/upload");
File dir = new File(path);
if(!dir.exists()){//如果文件夹不存在
dir.mkdir();//创建文件夹
}
String fileName = StringUitl.getStringTime() + ".jpg";//自定义图片名称
FileInputStream fis = null;//输入流
FileOutputStream fos = null;//输出流
try {
fis = new FileInputStream(file);//根据上传文件创建InputStream实例
fos = new FileOutputStream(new File(dir,fileName)); //创建写入服务器地址的输出流对象
byte[] bs = new byte[1024 * 4]; //创建字节数组实例
int len = -1;
while((len = fis.read(bs)) != -1){//循环读取文件
fos.write(bs, 0, len);//向指定的文件夹中写数据
}
UploadFile uploadFile = new UploadFile();//实例化对象
uploadFile.setPath(fileName);//设置文件名称
product.setUploadFile(uploadFile);//设置上传路径
} catch (Exception e) {
e.printStackTrace();
}finally{
fos.flush();
fos.close();
fis.close();
}
}
//如果商品类别和商品类别ID不为空,则保存商品类别信息
if(product.getCategory() != null && product.getCategory().getId() != null){
product.setCategory(categoryDao.load(product.getCategory().getId()));
}
//如果上传文件和上传文件ID不为空,则保存文件的上传路径信息
if(product.getUploadFile() != null && product.getUploadFile().getId() != null){
product.setUploadFile(uploadFileDao.load(product.getUploadFile().getId()));
}
productDao.saveOrUpdate(product);//保存商品信息
return list();
}
/**
* 查询商品
* @return
* @throws Exception
*/
public String list() throws Exception{
pageModel = productDao.find(pageNo, pageSize);//调用公共的查询方法
return LIST;//返回后台商品列表页面
}
/**
* 编辑商品
* @return String
* @throws Exception
*/
public String edit() throws Exception{
this.product = productDao.get(product.getId());//执行封装的查询方法
createCategoryTree();//生成商品的类别树
return EDIT;//返回商品信息编辑页面
}
/**
* 删除商品
* @return String
* @throws Exception
*/
public String del() throws Exception{
productDao.delete(product.getId());//执行删除操作
return list();//返回商品列表查找方法
}
/**
* 生成类别树
*/
private void createCategoryTree(){
String where = "where level=1";//查询一级节点
PageModel<ProductCategory> pageModel = categoryDao.find(-1, -1,where ,null);//执行查询方法
List<ProductCategory> allCategorys = pageModel.getList();
map = new LinkedHashMap<Integer, String>();//创建新的集合
for(ProductCategory category : allCategorys){//遍历所有的一级节点
setNodeMap(map,category,false);//将节点添加到Map集合中
}
}
/**
* 将节点设置到Map中
* @pa
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶网络商城(JavaWeb基于SSM框架的毕业设计)天下陶
资源推荐
资源详情
资源评论
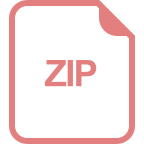
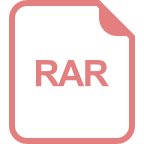
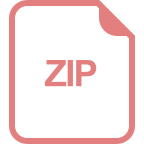
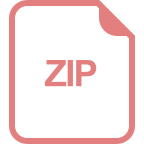
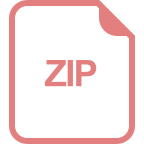
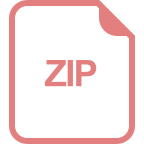
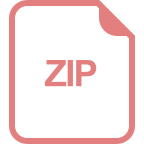
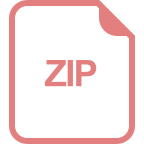
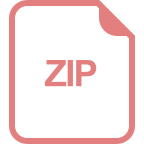
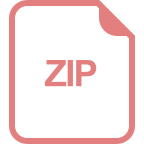
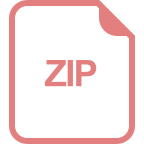
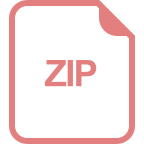
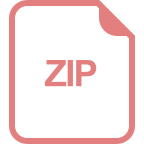
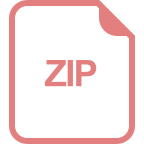
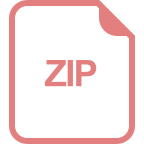
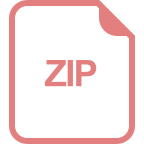
收起资源包目录

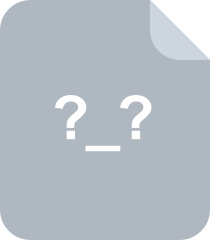
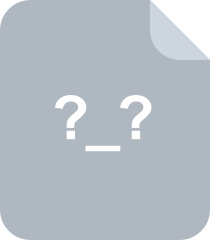
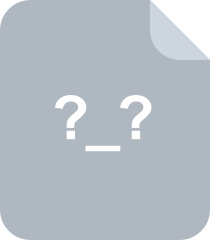
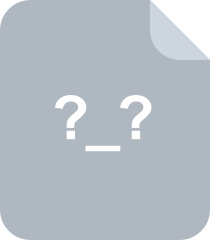
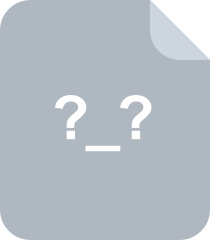
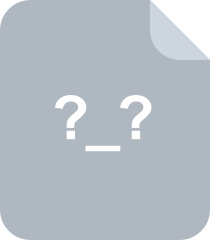
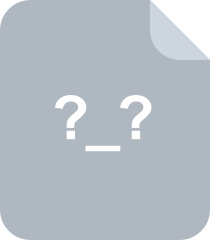
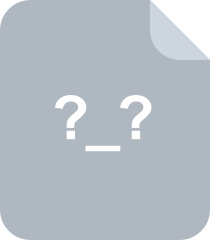
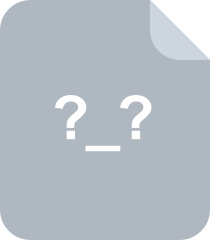
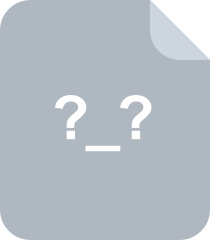
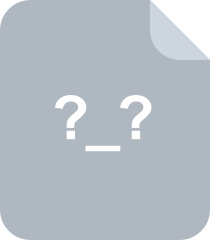
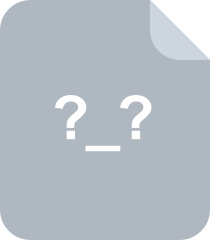
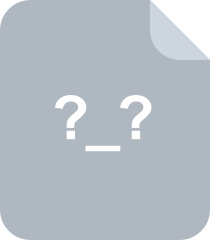
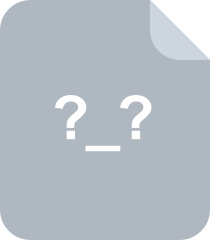
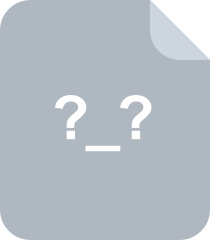
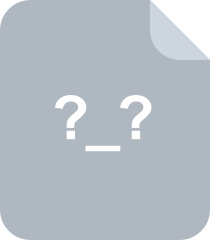
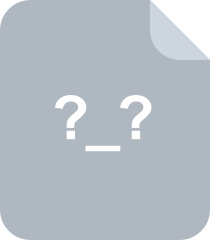
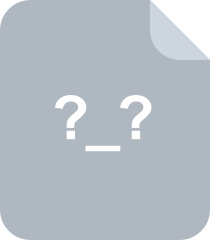
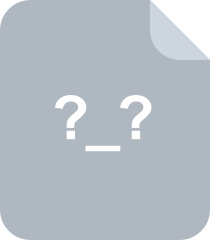
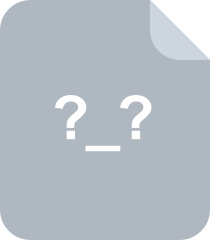
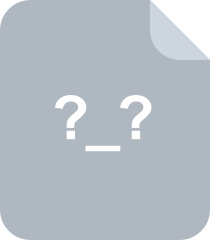
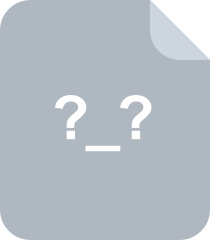
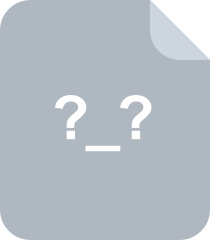
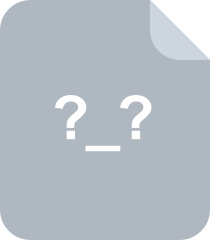
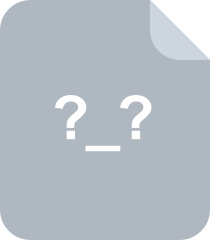
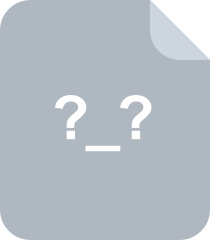
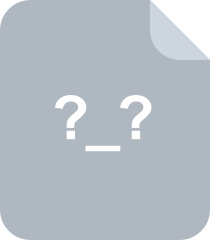
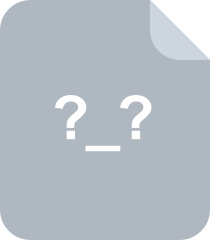
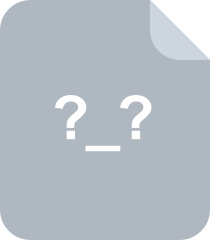
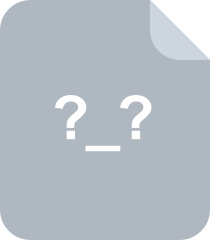
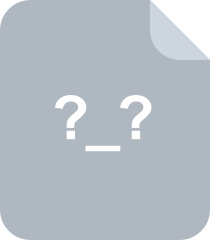
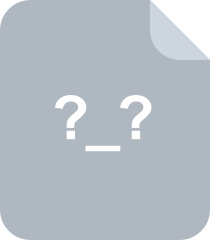
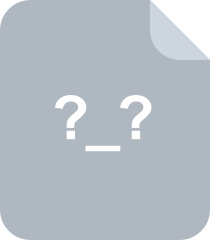
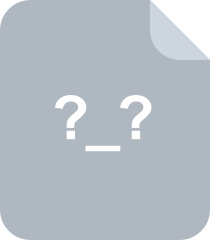
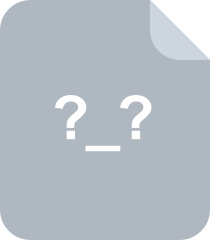
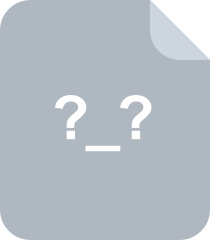
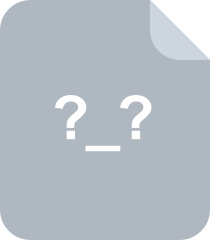
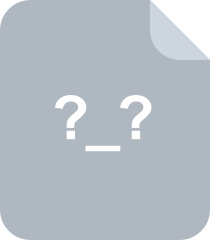
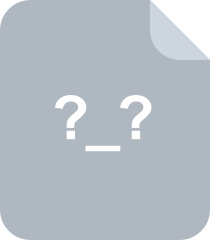
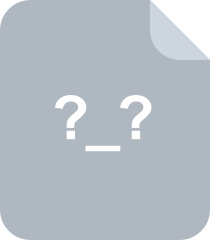
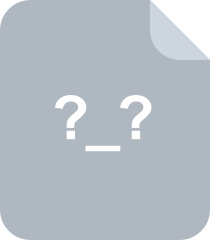
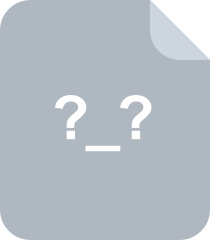
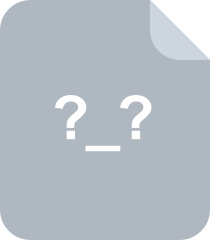
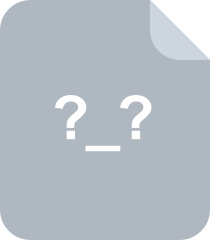
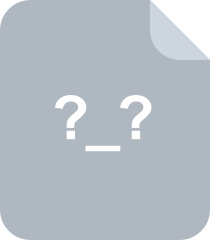
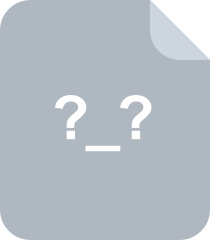
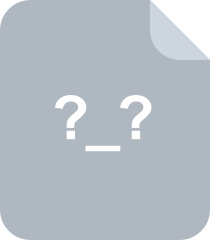
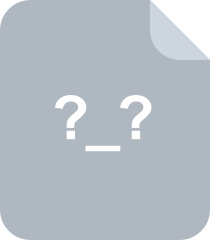
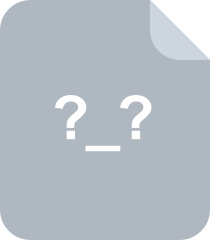
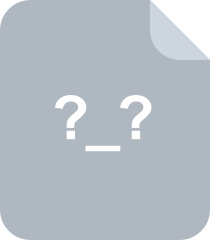
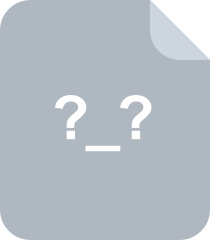
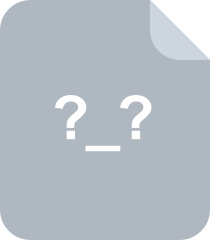
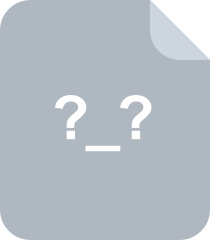
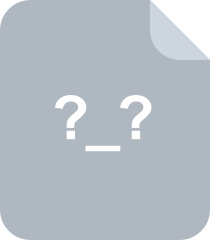
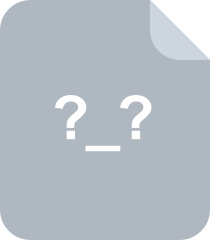
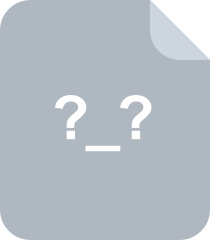
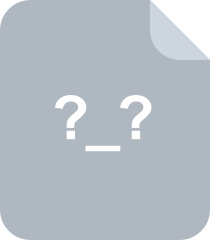
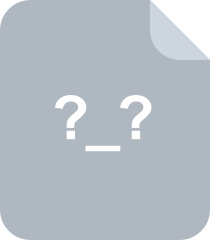
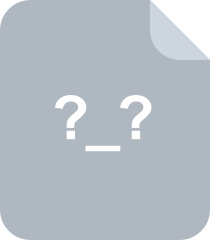
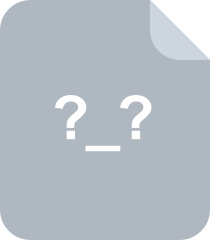
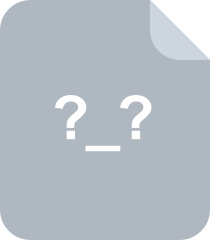
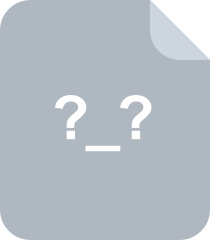
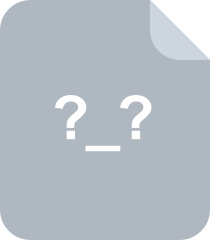
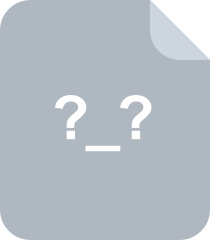
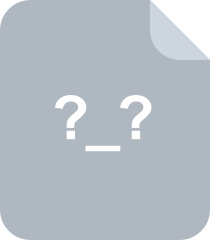
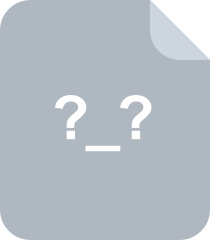
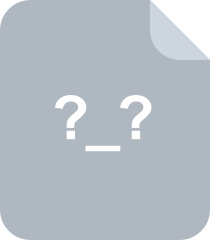
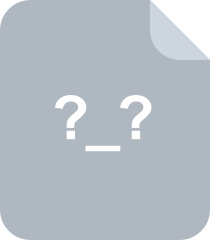
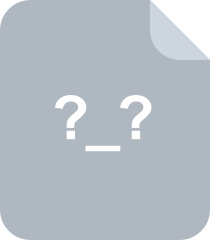
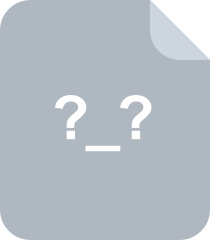
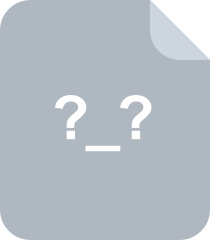
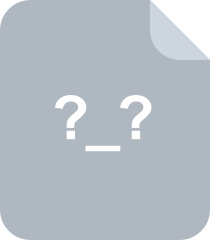
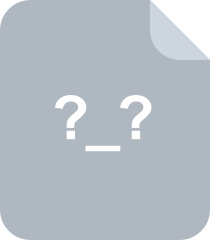
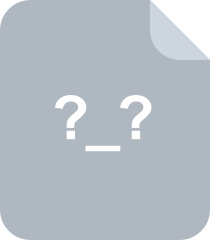
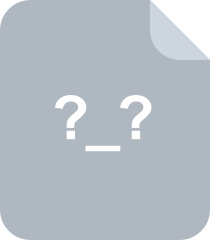
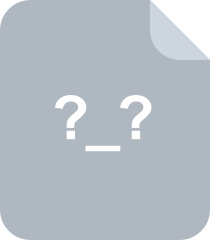
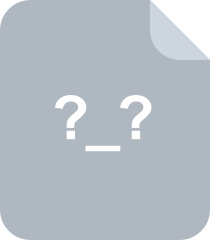
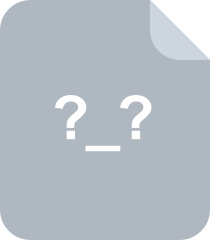
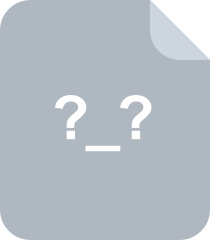
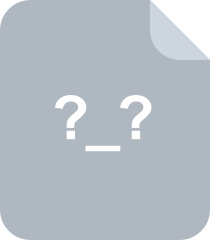
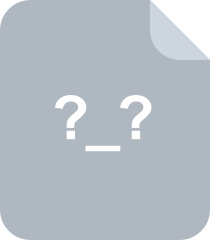
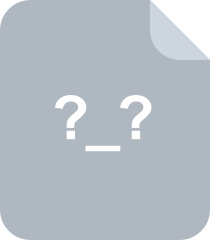
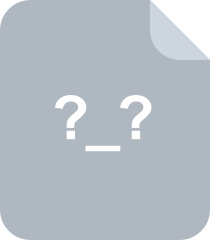
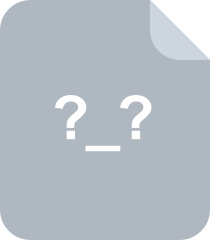
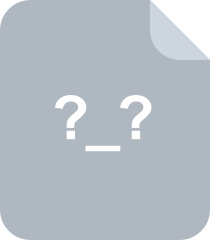
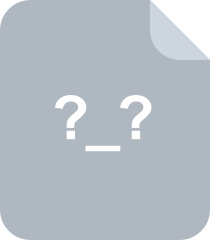
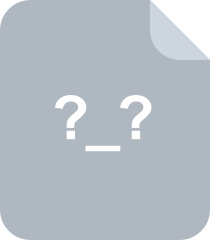
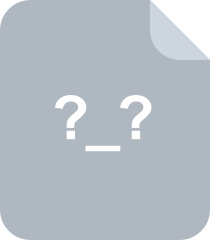
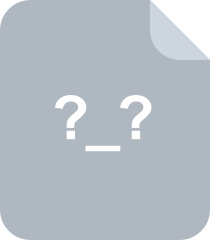
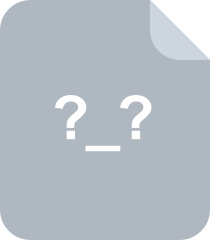
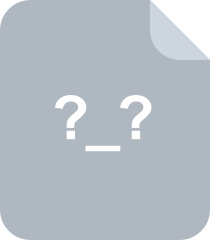
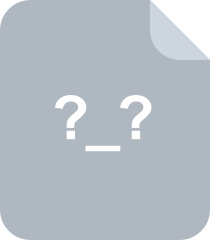
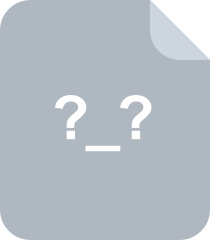
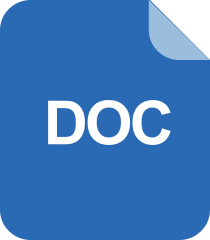
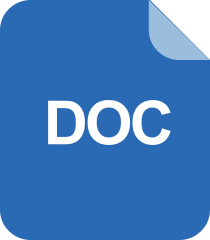
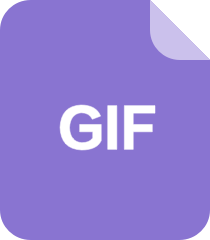
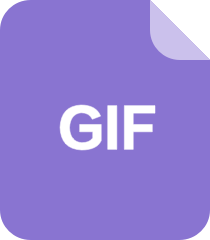
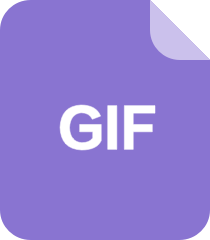
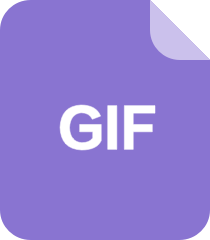
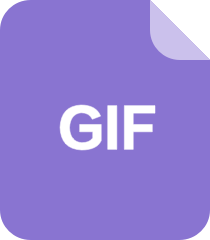
共 477 条
- 1
- 2
- 3
- 4
- 5
资源评论
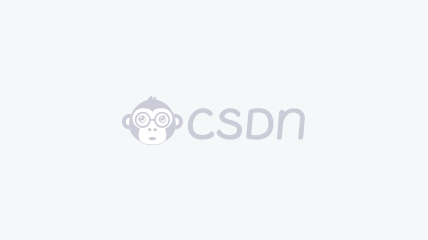

岛上程序猿
- 粉丝: 5282
- 资源: 4179
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

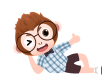
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


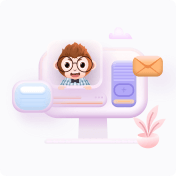
安全验证
文档复制为VIP权益,开通VIP直接复制
