#include <stdio.h>
#include <stdlib.h>
enum return_result {EMPTY_OK = 100, EMPTY_NO, PUSH_OK, PUSH_NO, POP_OK, POP_NO};
typedef struct node
{
int data;
struct node * next;
}Node, *Link;//链结构
typedef struct stk
{
Link space;
}Stack;//栈结构
/*malloc是否正确执行*/
void is_malloc_ok(void * node)
{
if (node == NULL)
{
printf("malloc error!\n");
exit(-1);
}
}
/*创建一个栈*/
void create_stack(Stack ** stack)
{
*stack = (Stack *)malloc(sizeof(Stack));
is_malloc_ok(*stack);
(*stack)->space = (Link)malloc(sizeof(Node));
is_malloc_ok((*stack)->space);
}
/*初始化栈*/
void init_stack(Stack *stack)
{
stack->space->next = NULL;
}
/*创建一个压栈元素*/
void create_node(Link * new_node)
{
*new_node = (Link)malloc(sizeof(Node));
is_malloc_ok(*new_node);
}
/*压栈*/
void push_stack(Stack *stack, Link new_node)
{
new_node->next = stack->space->next;
stack->space->next = new_node;
}
/*判断栈空*/
int is_stack_empty(Stack *stack)
{
Link p = NULL;
p = stack->space->next;
if (p == NULL)
{
return EMPTY_OK;
}
return EMPTY_NO;
}
/*出栈*/
int pop_stack(Stack *stack)
{
Link p = NULL;
int data;
if (stack == NULL)
{//栈不存在
printf("stack is not exist!\n");
exit(-1);
}
if (EMPTY_OK == is_stack_empty(stack))
{
printf("stack is empty!\n");
return POP_NO;
}
p = stack->space->next;
stack->space->next = p->next;
data = p->data;
free(p);//释放栈顶
return data;
}
/**/
void release_stack(Stack **stack)
{
Link p = NULL;
if (EMPTY_NO == is_stack_empty(*stack))
{//栈不空
p = (*stack)->space->next;
while ((*stack)->space->next != NULL)
{
(*stack)->space->next = p->next;
free(p);
p = (*stack)->space->next;
}
}
free((*stack)->space);//链头结点
free(*stack);//释放栈
*stack = NULL;
}
int main()
{
int i;
int ret;
Stack *stack = NULL;
Link new_node = NULL;//入栈新元素
create_stack(&stack);
init_stack(stack);
for (i = 0; i < 10; i++)
{//入栈
create_node(&new_node);
new_node->data = i + 1;
push_stack(stack, new_node);//相当于链表的头插
}
for (i = 0; i < 5; i++)
{//出栈
ret = pop_stack(stack);
if (ret == POP_NO)
{
break;
}
printf("%d\n", ret);
}
release_stack(&stack);
pop_stack(stack);
return 0;
}

枫蜜柚子茶
- 粉丝: 9003
- 资源: 5351
最新资源
- 基于and-design-vue的vue后台管理系统模板.zip
- 基于canvas Fabric.js库创建的vue Fabric组件,定制画板,图片关联较差.zip
- 基于eggjs、vuejs的设备管理系统,有帮助的话麻烦给个star谢谢.zip
- 基于element-ui的数据驱动表单组件.zip
- 基于ElementUI 2.x的扩展组件(已废弃 已被废弃).zip
- 基于RageFrame2的一款免费开源基础商城销售功能的开源微商城 .zip
- 基于vue+vant搭建h5通用框架子(包含cli3,cli4,typescript版本).zip
- 基于vue-cli(vue2.X,webpack1.X,es6,sass环境)多页面开发.zip
- 基于Vue.js的百万级数据表格组件,支持编辑、筛选、过滤、粘贴、拖动调整列宽等多种功能.zip
- 基于vue2.0生态的后台管理系统模板(spa) 一个基于vue2.0 + vue-router + vuex + element-ui +ES6+ webpack + npm 的vue管理系.zip
- 基于vue2.0的活动倒计时组件.zip
- 基于vue2和iview2的后台管理系统.zip
- 基于Vue3+Element Plus+FastAPI开发的一个通用中后台管理框架(若依的FastAPI版本).zip
- 基于Vue3+TypeScript+ Vue-Cli4.0 ,构建手机端模板脚手架.zip
- 基于Vue3+Vite+TypeScript+compositionApi+naiveui构建的精致的支持夜间模式网易云音乐流媒体网站webApp.zip
- 基于Vue3.0 Composition Api 快速构建实战项目.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


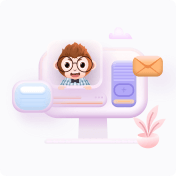