/**
******************************************************************************
* @file stm32f7xx_hal_tim.c
* @author MCD Application Team
* @version V1.1.1
* @date 01-July-2016
* @brief TIM HAL module driver.
* This file provides firmware functions to manage the following
* functionalities of the Timer (TIM) peripheral:
* + Time Base Initialization
* + Time Base Start
* + Time Base Start Interruption
* + Time Base Start DMA
* + Time Output Compare/PWM Initialization
* + Time Output Compare/PWM Channel Configuration
* + Time Output Compare/PWM Start
* + Time Output Compare/PWM Start Interruption
* + Time Output Compare/PWM Start DMA
* + Time Input Capture Initialization
* + Time Input Capture Channel Configuration
* + Time Input Capture Start
* + Time Input Capture Start Interruption
* + Time Input Capture Start DMA
* + Time One Pulse Initialization
* + Time One Pulse Channel Configuration
* + Time One Pulse Start
* + Time Encoder Interface Initialization
* + Time Encoder Interface Start
* + Time Encoder Interface Start Interruption
* + Time Encoder Interface Start DMA
* + Commutation Event configuration with Interruption and DMA
* + Time OCRef clear configuration
* + Time External Clock configuration
@verbatim
==============================================================================
##### TIMER Generic features #####
==============================================================================
[..] The Timer features include:
(#) 16-bit up, down, up/down auto-reload counter.
(#) 16-bit programmable prescaler allowing dividing (also on the fly) the
counter clock frequency either by any factor between 1 and 65536.
(#) Up to 4 independent channels for:
(++) Input Capture
(++) Output Compare
(++) PWM generation (Edge and Center-aligned Mode)
(++) One-pulse mode output
##### How to use this driver #####
==============================================================================
[..]
(#) Initialize the TIM low level resources by implementing the following functions
depending from feature used :
(++) Time Base : HAL_TIM_Base_MspInit()
(++) Input Capture : HAL_TIM_IC_MspInit()
(++) Output Compare : HAL_TIM_OC_MspInit()
(++) PWM generation : HAL_TIM_PWM_MspInit()
(++) One-pulse mode output : HAL_TIM_OnePulse_MspInit()
(++) Encoder mode output : HAL_TIM_Encoder_MspInit()
(#) Initialize the TIM low level resources :
(##) Enable the TIM interface clock using __HAL_RCC_TIMx_CLK_ENABLE();
(##) TIM pins configuration
(+++) Enable the clock for the TIM GPIOs using the following function:
__HAL_RCC_GPIOx_CLK_ENABLE();
(+++) Configure these TIM pins in Alternate function mode using HAL_GPIO_Init();
(#) The external Clock can be configured, if needed (the default clock is the
internal clock from the APBx), using the following function:
HAL_TIM_ConfigClockSource, the clock configuration should be done before
any start function.
(#) Configure the TIM in the desired functioning mode using one of the
initialization function of this driver:
(++) HAL_TIM_Base_Init: to use the Timer to generate a simple time base
(++) HAL_TIM_OC_Init and HAL_TIM_OC_ConfigChannel: to use the Timer to generate an
Output Compare signal.
(++) HAL_TIM_PWM_Init and HAL_TIM_PWM_ConfigChannel: to use the Timer to generate a
PWM signal.
(++) HAL_TIM_IC_Init and HAL_TIM_IC_ConfigChannel: to use the Timer to measure an
external signal.
(++) HAL_TIM_OnePulse_Init and HAL_TIM_OnePulse_ConfigChannel: to use the Timer
in One Pulse Mode.
(++) HAL_TIM_Encoder_Init: to use the Timer Encoder Interface.
(#) Activate the TIM peripheral using one of the start functions depending from the feature used:
(++) Time Base : HAL_TIM_Base_Start(), HAL_TIM_Base_Start_DMA(), HAL_TIM_Base_Start_IT()
(++) Input Capture : HAL_TIM_IC_Start(), HAL_TIM_IC_Start_DMA(), HAL_TIM_IC_Start_IT()
(++) Output Compare : HAL_TIM_OC_Start(), HAL_TIM_OC_Start_DMA(), HAL_TIM_OC_Start_IT()
(++) PWM generation : HAL_TIM_PWM_Start(), HAL_TIM_PWM_Start_DMA(), HAL_TIM_PWM_Start_IT()
(++) One-pulse mode output : HAL_TIM_OnePulse_Start(), HAL_TIM_OnePulse_Start_IT()
(++) Encoder mode output : HAL_TIM_Encoder_Start(), HAL_TIM_Encoder_Start_DMA(), HAL_TIM_Encoder_Start_IT().
(#) The DMA Burst is managed with the two following functions:
HAL_TIM_DMABurst_WriteStart()
HAL_TIM_DMABurst_ReadStart()
@endverbatim
******************************************************************************
* @attention
*
* <h2><center>© COPYRIGHT(c) 2016 STMicroelectronics</center></h2>
*
* Redistribution and use in source and binary forms, with or without modification,
* are permitted provided that the following conditions are met:
* 1. Redistributions of source code must retain the above copyright notice,
* this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright notice,
* this list of conditions and the following disclaimer in the documentation
* and/or other materials provided with the distribution.
* 3. Neither the name of STMicroelectronics nor the names of its contributors
* may be used to endorse or promote products derived from this software
* without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
* AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE
* IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
* CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
* OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*
******************************************************************************
*/
/* Includes ------------------------------------------------------------------*/
#include "stm32f7xx_hal.h"
/** @addtogroup STM32F7xx_HAL_Driver
* @{
*/
/** @defgroup TIM TIM
* @brief TIM HAL module driver
* @{
*/
#ifdef HAL_TIM_MODULE_ENABLED
/* Private typedef -----------------------------------------------------------*/
/* Private define ------------------------------------------------------------*/
/* Private macro -------------------------------------------------------------*/
/* Private variables ---------------------------------------------------------*/
/** @addtogroup TIM_Private_Functions
* @{
*/
/* Private function prototypes -----------------------------------------------*/
static void TIM_TI1_ConfigInputStage(TIM_TypeDef *TIMx, uint32_t TIM_ICPolarity, uint32_t TIM_ICFi
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
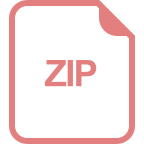
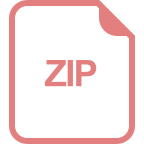
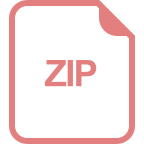
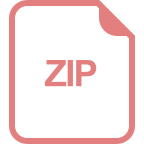
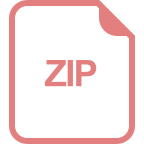
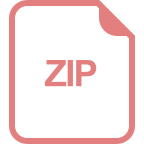
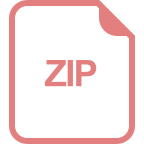
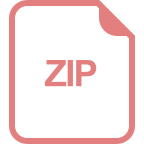
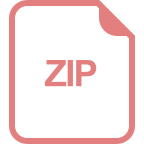
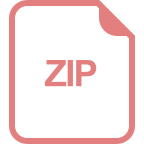
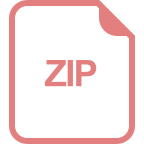
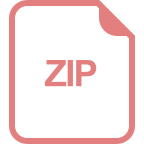
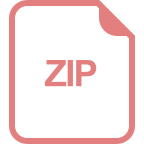
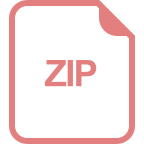
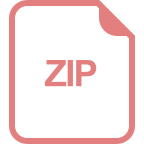
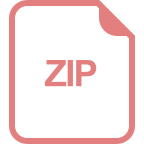
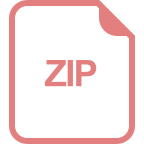
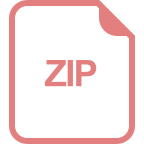
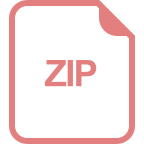
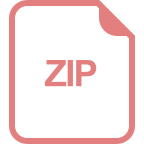
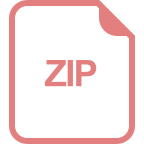
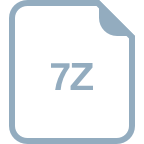
收起资源包目录

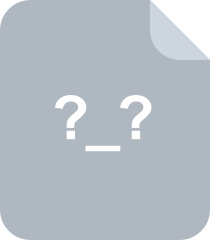
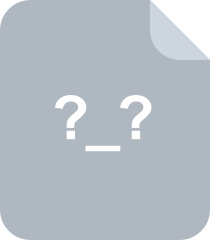
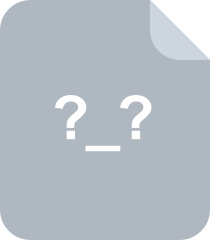
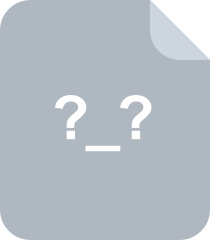
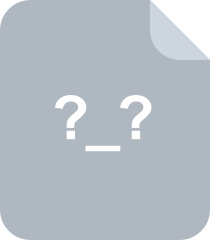
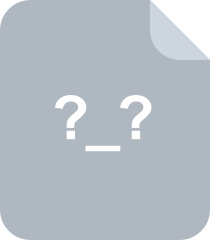
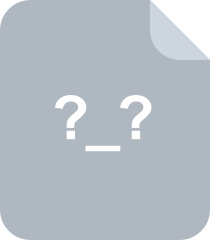
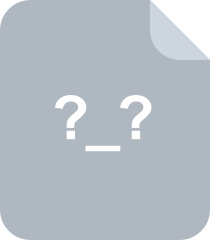
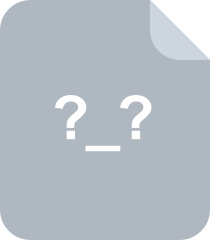
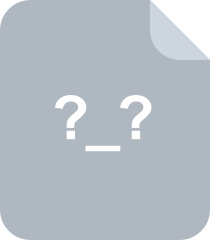
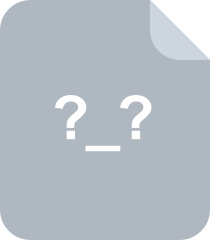
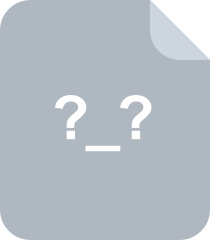
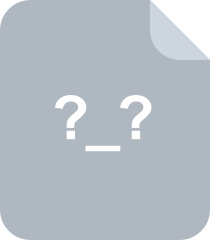
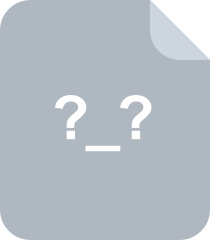
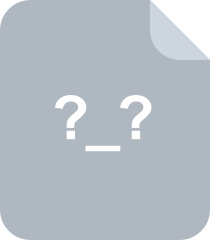
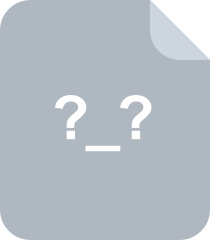
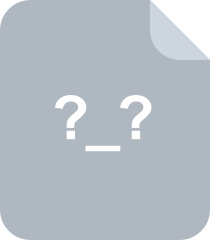
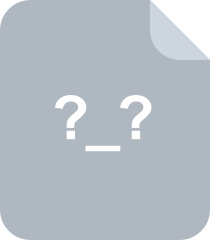
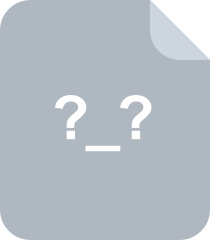
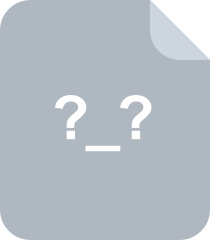
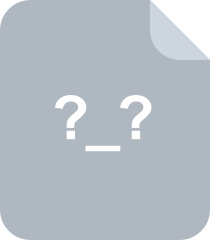
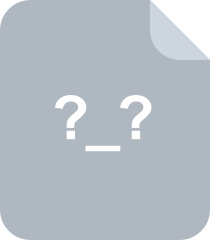
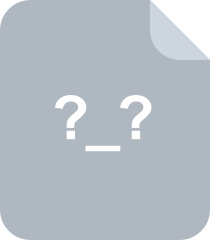
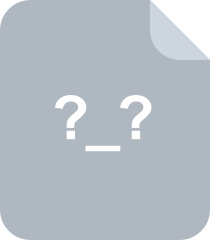
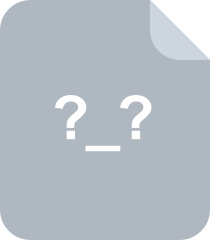
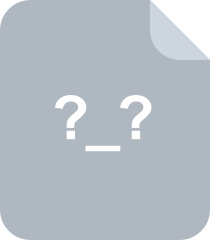
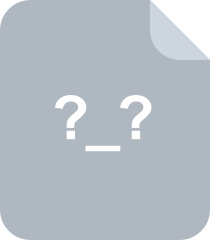
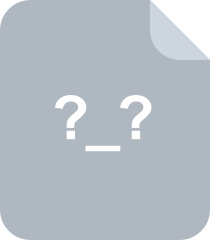
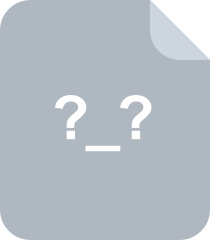
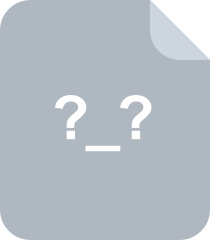
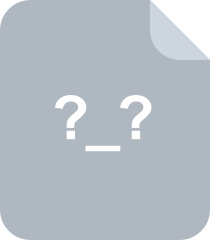
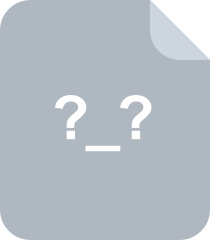
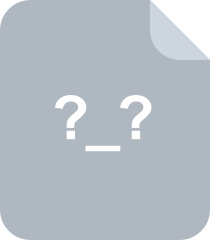
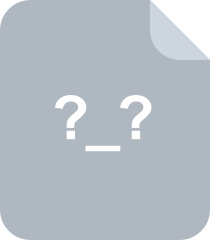
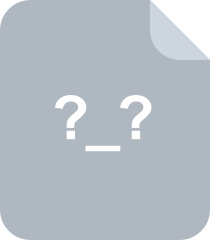
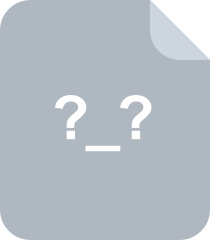
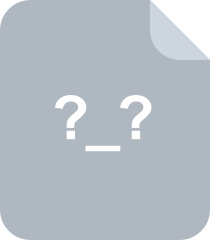
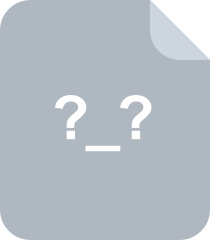
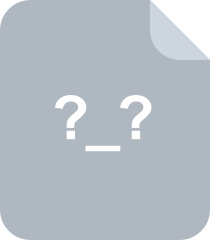
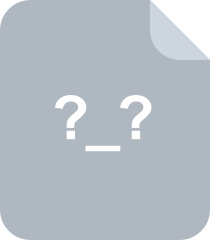
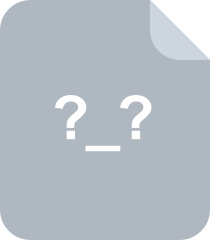
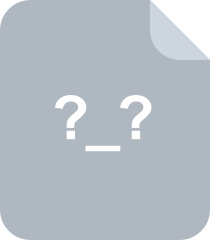
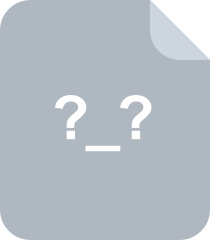
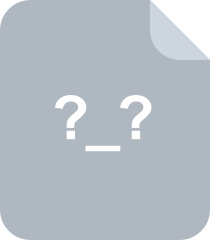
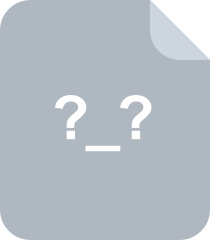
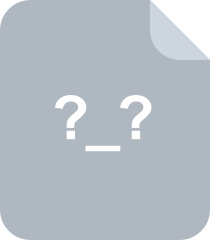
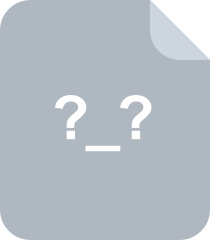
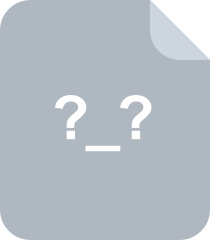
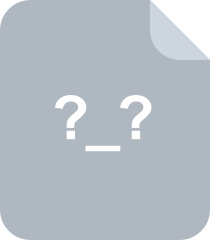
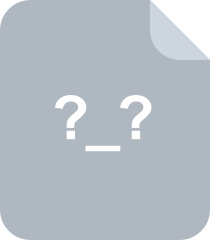
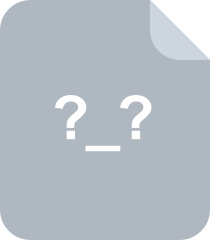
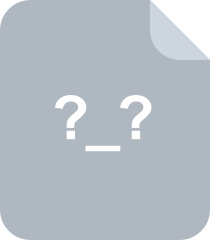
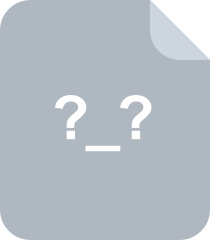
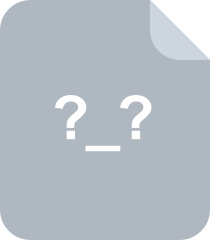
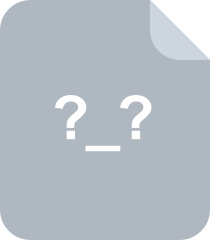
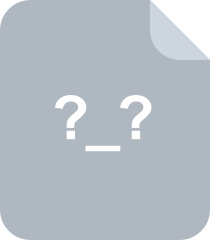
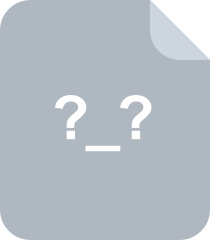
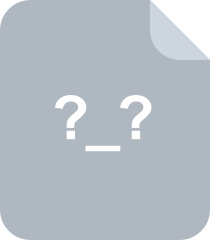
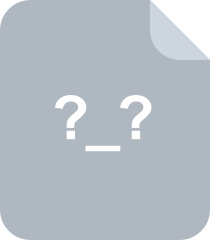
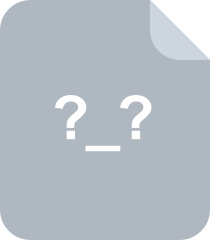
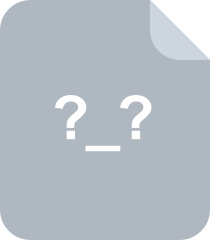
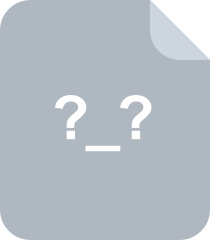
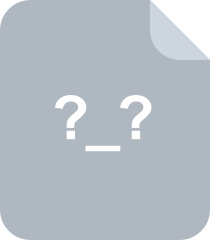
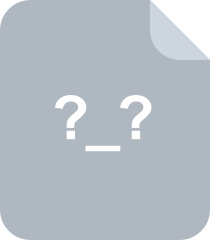
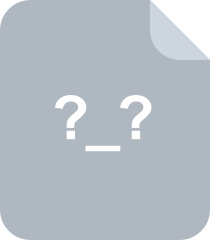
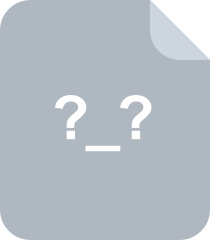
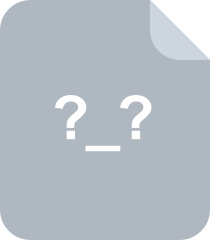
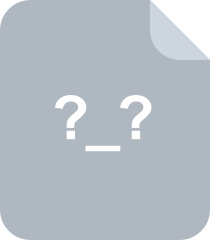
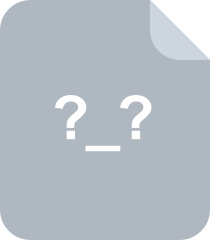
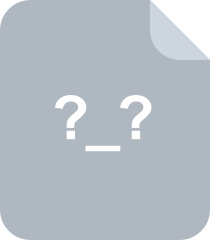
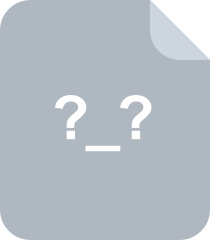
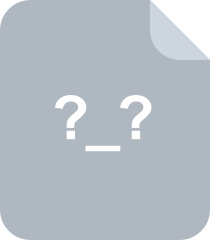
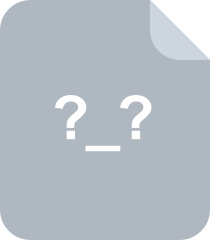
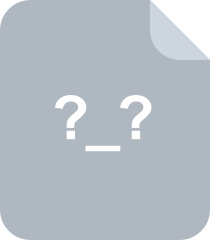
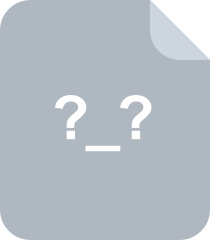
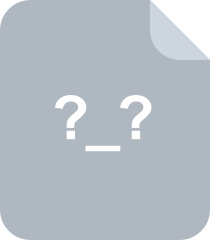
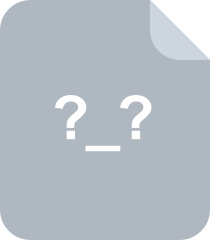
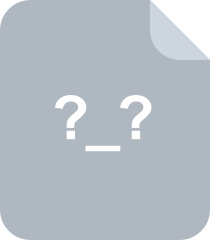
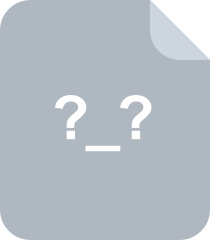
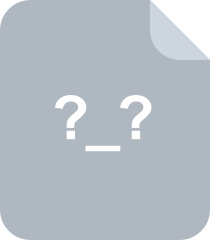
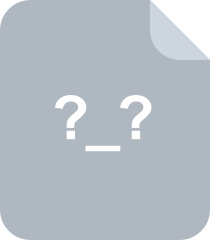
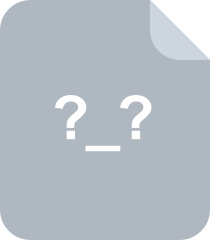
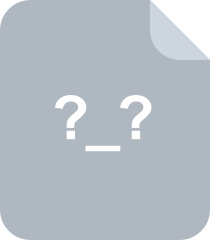
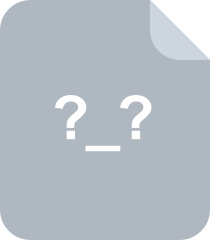
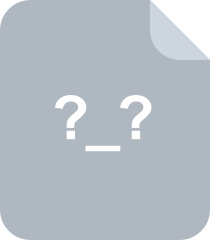
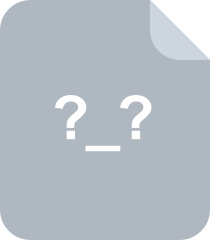
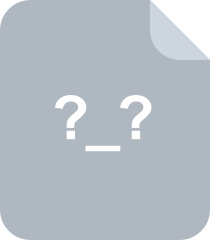
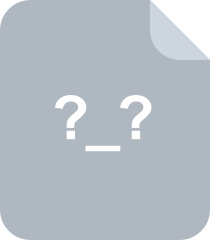
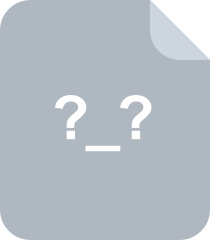
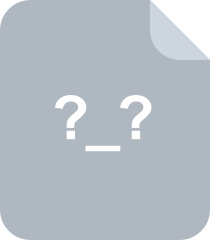
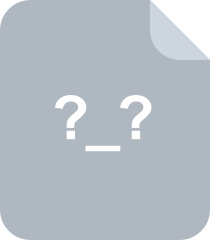
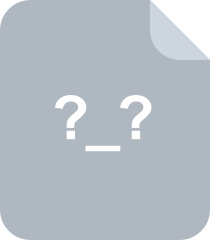
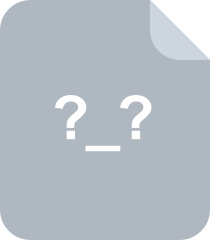
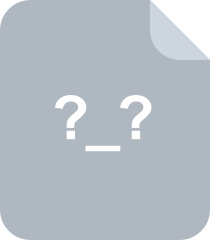
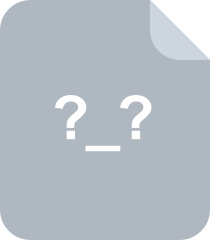
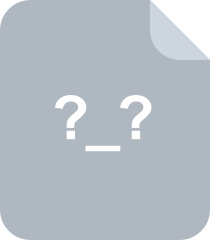
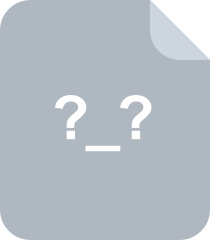
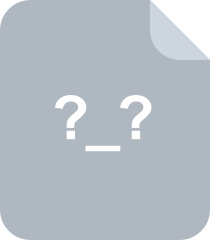
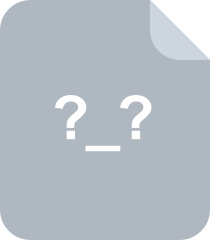
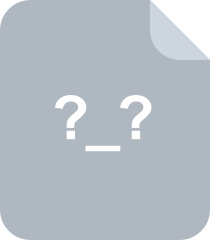
共 227 条
- 1
- 2
- 3
资源评论
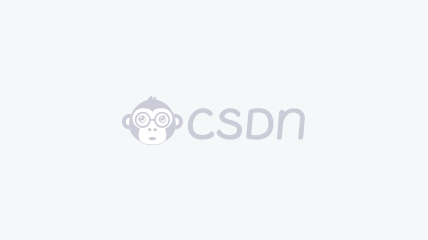
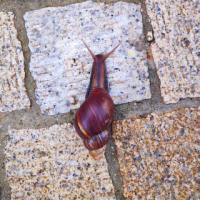

不脱发的程序猿
- 粉丝: 24w+
- 资源: 5757
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

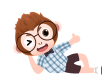
最新资源
- APT漏洞利用利器工具
- 关于哈法亚侏罗系深层探井地质工程设计方案汇报的请示.pdf
- 购物网站html+css+js 源码+报告
- 对AD采集的IQ数据进行FFT计算
- 基于FreeRTOS、STM32F103C8、LCD1602、GP2Y0A700K0F 的红外测距应用proteus仿真
- 8-Channel 12-Bit ADC for Raspberry Pi (STM32F030)-原理图
- Java+编程+阿里+开发+提升
- JavaScript 事件处理 下拉列表和可选项 示例代码
- 海信智能电视刷机数据 LED48K681X3DU(0000) 生产用软件数据 务必确认机编一致 强制刷机 整机USB升级程序
- 2024最新支行联行号信息
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


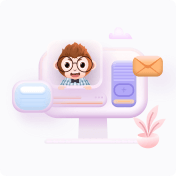
安全验证
文档复制为VIP权益,开通VIP直接复制
