<?php
/*~ class.phpmailer.php
.---------------------------------------------------------------------------.
| Software: PHPMailer - PHP email class |
| Version: 2.1 |
| Contact: via sourceforge.net support pages (also www.codeworxtech.com) |
| Info: http://phpmailer.sourceforge.net |
| Support: http://sourceforge.net/projects/phpmailer/ |
| ------------------------------------------------------------------------- |
| Author: Andy Prevost (project admininistrator) |
| Author: Brent R. Matzelle (original founder) |
| Copyright (c) 2004-2007, Andy Prevost. All Rights Reserved. |
| Copyright (c) 2001-2003, Brent R. Matzelle |
| ------------------------------------------------------------------------- |
| License: Distributed under the Lesser General Public License (LGPL) |
| http://www.gnu.org/copyleft/lesser.html |
| This program is distributed in the hope that it will be useful - WITHOUT |
| ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or |
| FITNESS FOR A PARTICULAR PURPOSE. |
| ------------------------------------------------------------------------- |
| We offer a number of paid services (www.codeworxtech.com): |
| - Web Hosting on highly optimized fast and secure servers |
| - Technology Consulting |
| - Oursourcing (highly qualified programmers and graphic designers) |
'---------------------------------------------------------------------------'
/**
* PHPMailer - PHP email transport class
* NOTE: Designed for use with PHP version 5 and up
* @package PHPMailer
* @author Andy Prevost
* @copyright 2004 - 2008 Andy Prevost
*/
class PHPMailer {
/////////////////////////////////////////////////
// PROPERTIES, PUBLIC
/////////////////////////////////////////////////
/**
* Email priority (1 = High, 3 = Normal, 5 = low).
* @var int
*/
public $Priority = 3;
/**
* Sets the CharSet of the message.
* @var string
*/
public $CharSet = 'iso-8859-1';
/**
* Sets the Content-type of the message.
* @var string
*/
public $ContentType = 'text/plain';
/**
* Sets the Encoding of the message. Options for this are "8bit",
* "7bit", "binary", "base64", and "quoted-printable".
* @var string
*/
public $Encoding = '8bit';
/**
* Holds the most recent mailer error message.
* @var string
*/
public $ErrorInfo = '';
/**
* Sets the From email address for the message.
* @var string
*/
public $From = 'root@localhost';
/**
* Sets the From name of the message.
* @var string
*/
public $FromName = 'Root User';
/**
* Sets the Sender email (Return-Path) of the message. If not empty,
* will be sent via -f to sendmail or as 'MAIL FROM' in smtp mode.
* @var string
*/
public $Sender = '';
/**
* Sets the Subject of the message.
* @var string
*/
public $Subject = '';
/**
* Sets the Body of the message. This can be either an HTML or text body.
* If HTML then run IsHTML(true).
* @var string
*/
public $Body = '';
/**
* Sets the text-only body of the message. This automatically sets the
* email to multipart/alternative. This body can be read by mail
* clients that do not have HTML email capability such as mutt. Clients
* that can read HTML will view the normal Body.
* @var string
*/
public $AltBody = '';
/**
* Sets word wrapping on the body of the message to a given number of
* characters.
* @var int
*/
public $WordWrap = 0;
/**
* Method to send mail: ("mail", "sendmail", or "smtp").
* @var string
*/
public $Mailer = 'mail';
/**
* Sets the path of the sendmail program.
* @var string
*/
public $Sendmail = '/usr/sbin/sendmail';
/**
* Path to PHPMailer plugins. This is now only useful if the SMTP class
* is in a different directory than the PHP include path.
* @var string
*/
public $PluginDir = '';
/**
* Holds PHPMailer version.
* @var string
*/
public $Version = "2.1";
/**
* Sets the email address that a reading confirmation will be sent.
* @var string
*/
public $ConfirmReadingTo = '';
/**
* Sets the hostname to use in Message-Id and Received headers
* and as default HELO string. If empty, the value returned
* by SERVER_NAME is used or 'localhost.localdomain'.
* @var string
*/
public $Hostname = '';
/**
* Sets the message ID to be used in the Message-Id header.
* If empty, a unique id will be generated.
* @var string
*/
public $MessageID = '';
/////////////////////////////////////////////////
// PROPERTIES FOR SMTP
/////////////////////////////////////////////////
/**
* Sets the SMTP hosts. All hosts must be separated by a
* semicolon. You can also specify a different port
* for each host by using this format: [hostname:port]
* (e.g. "smtp1.example.com:25;smtp2.example.com").
* Hosts will be tried in order.
* @var string
*/
public $Host = 'localhost';
/**
* Sets the default SMTP server port.
* @var int
*/
public $Port = 25;
/**
* Sets the SMTP HELO of the message (Default is $Hostname).
* @var string
*/
public $Helo = '';
/**
* Sets connection prefix.
* Options are "", "ssl" or "tls"
* @var string
*/
public $SMTPSecure = "";
/**
* Sets SMTP authentication. Utilizes the Username and Password variables.
* @var bool
*/
public $SMTPAuth = false;
/**
* Sets SMTP username.
* @var string
*/
public $Username = '';
/**
* Sets SMTP password.
* @var string
*/
public $Password = '';
/**
* Sets the SMTP server timeout in seconds. This function will not
* work with the win32 version.
* @var int
*/
public $Timeout = 100;
/**
* Sets SMTP class debugging on or off.
* @var bool
*/
public $SMTPDebug = false;
/**
* Prevents the SMTP connection from being closed after each mail
* sending. If this is set to true then to close the connection
* requires an explicit call to SmtpClose().
* @var bool
*/
public $SMTPKeepAlive = false;
/**
* Provides the ability to have the TO field process individual
* emails, instead of sending to entire TO addresses
* @var bool
*/
public $SingleTo = false;
/////////////////////////////////////////////////
// PROPERTIES, PRIVATE
/////////////////////////////////////////////////
private $smtp = NULL;
private $to = array();
private $cc = array();
private $bcc = array();
private $ReplyTo = array();
private $attachment = array();
private $CustomHeader = array();
private $message_type = '';
private $boundary = array();
private $language = array();
private $error_count = 0;
private $LE = "\n";
private $sign_key_file = "";
private $sign_key_pass = "";
/////////////////////////////////////////////////
// METHODS, VARIABLES
/////////////////////////////////////////////////
/**
* Sets message type to HTML.
* @param bool $bool
* @retu
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
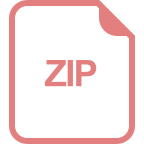
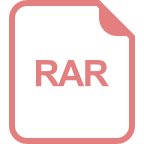
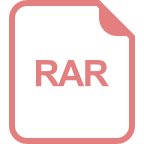
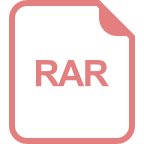
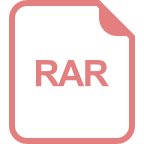
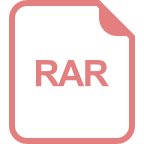
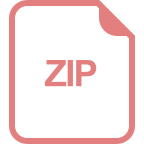
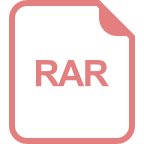
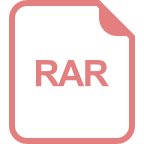
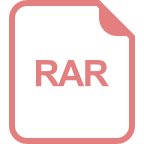
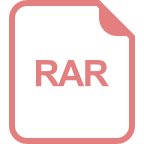
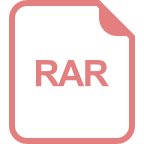
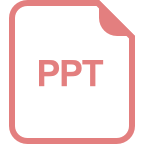
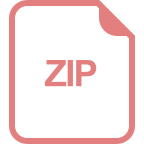
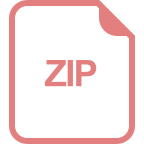
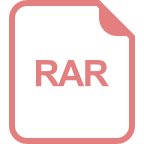
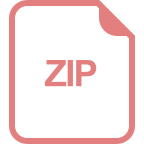
收起资源包目录


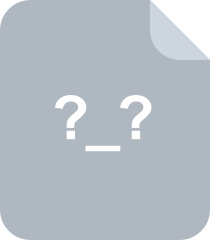
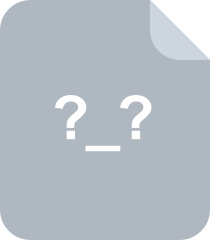


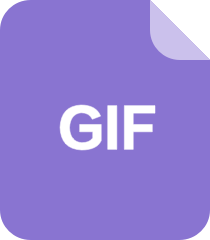
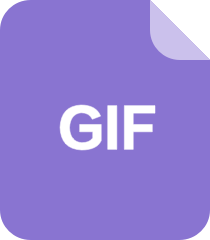
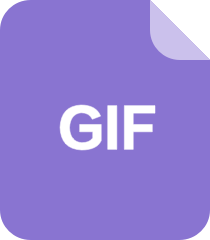
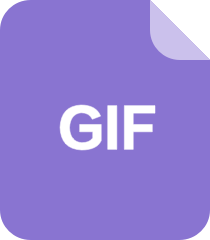
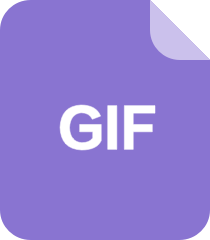
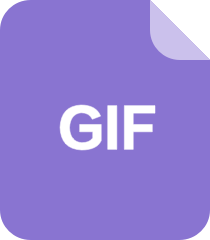
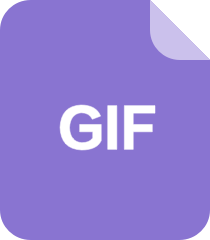
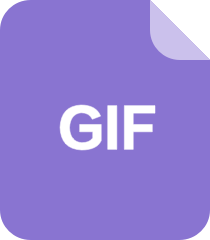
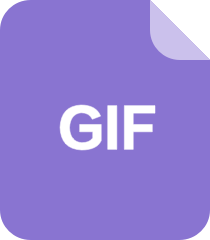
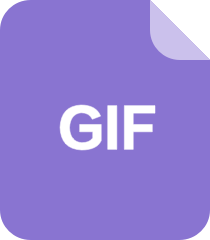
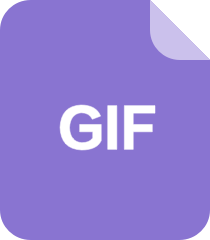
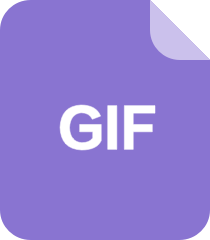
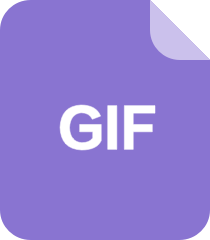
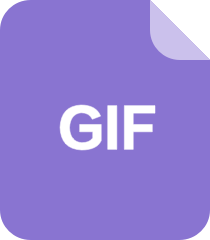
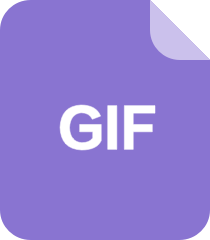
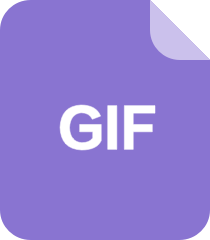
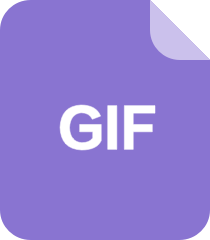
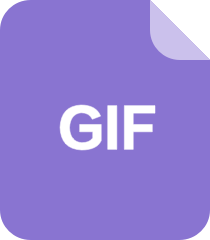
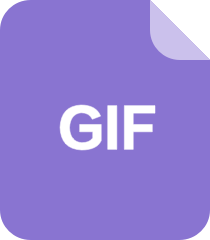
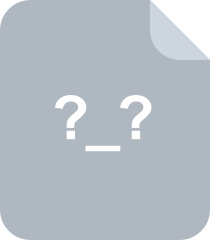
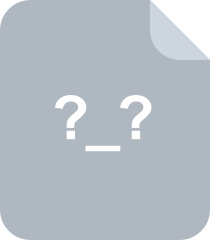
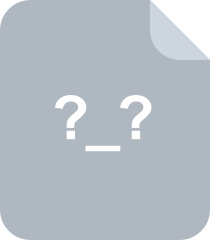
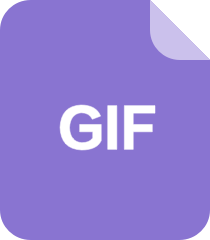

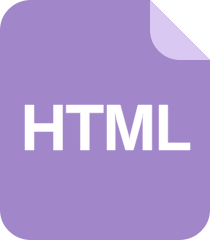
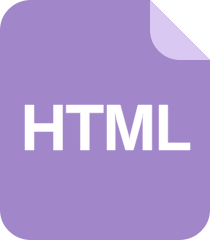
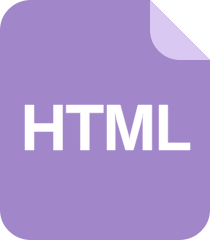
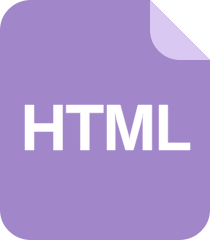
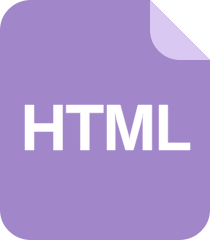
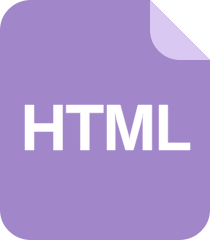

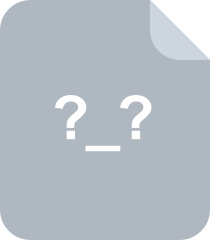
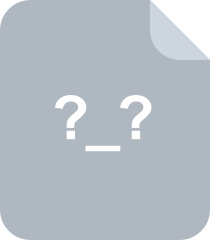
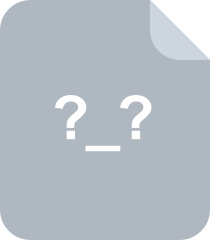
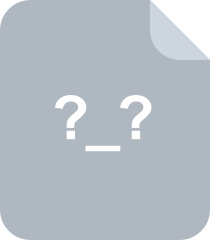
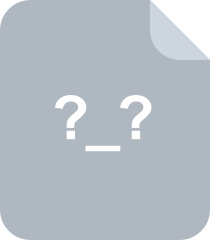
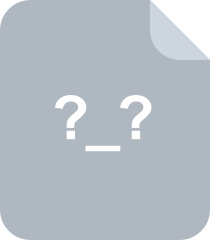


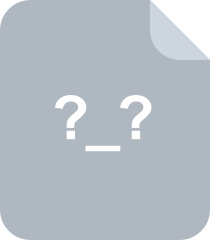
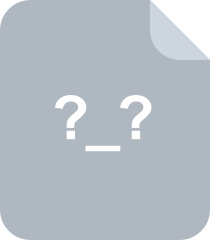
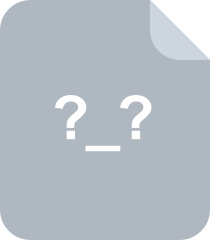
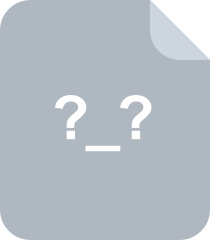
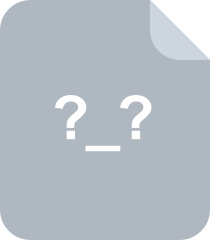
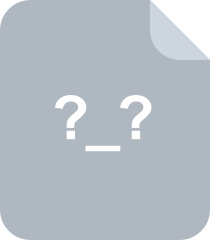
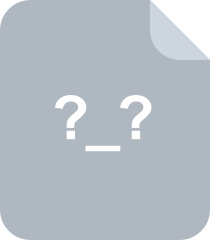
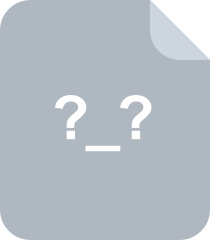
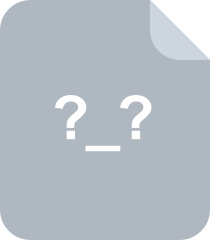
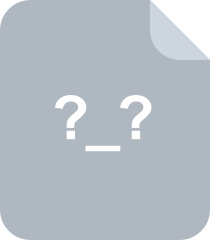
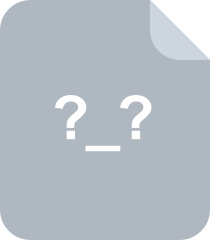
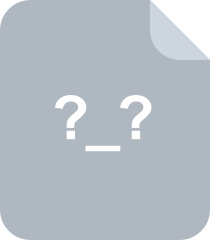
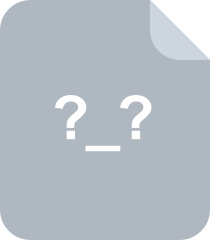
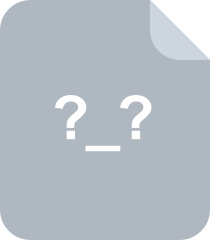
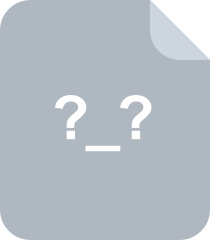
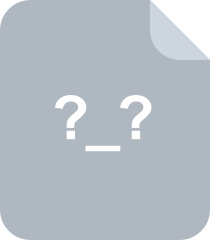
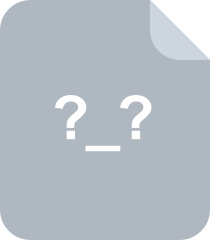
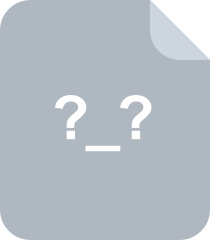
共 55 条
- 1
资源评论
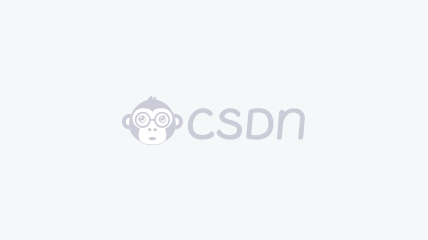
- liyuluyi2015-04-17还是不错的资源,只不过是php的
- jxp_9272011-09-29晕死 是PHP的!!!

木木小马
- 粉丝: 4
- 资源: 7
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

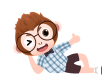
安全验证
文档复制为VIP权益,开通VIP直接复制
