没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
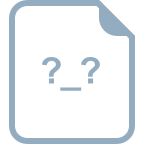
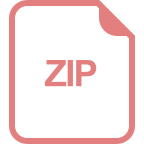
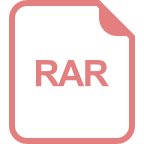
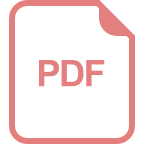
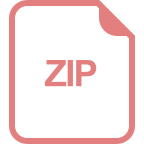
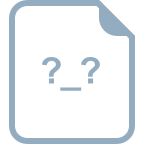
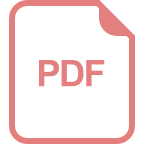
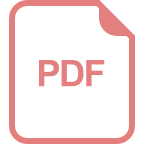
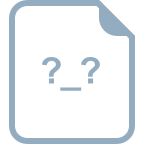
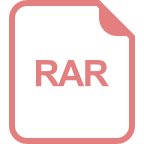
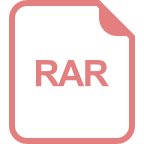
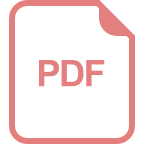
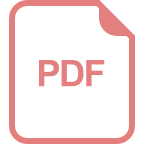
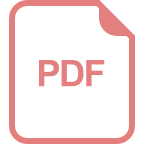
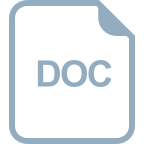
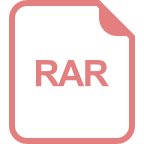
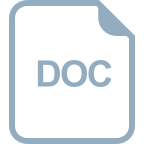
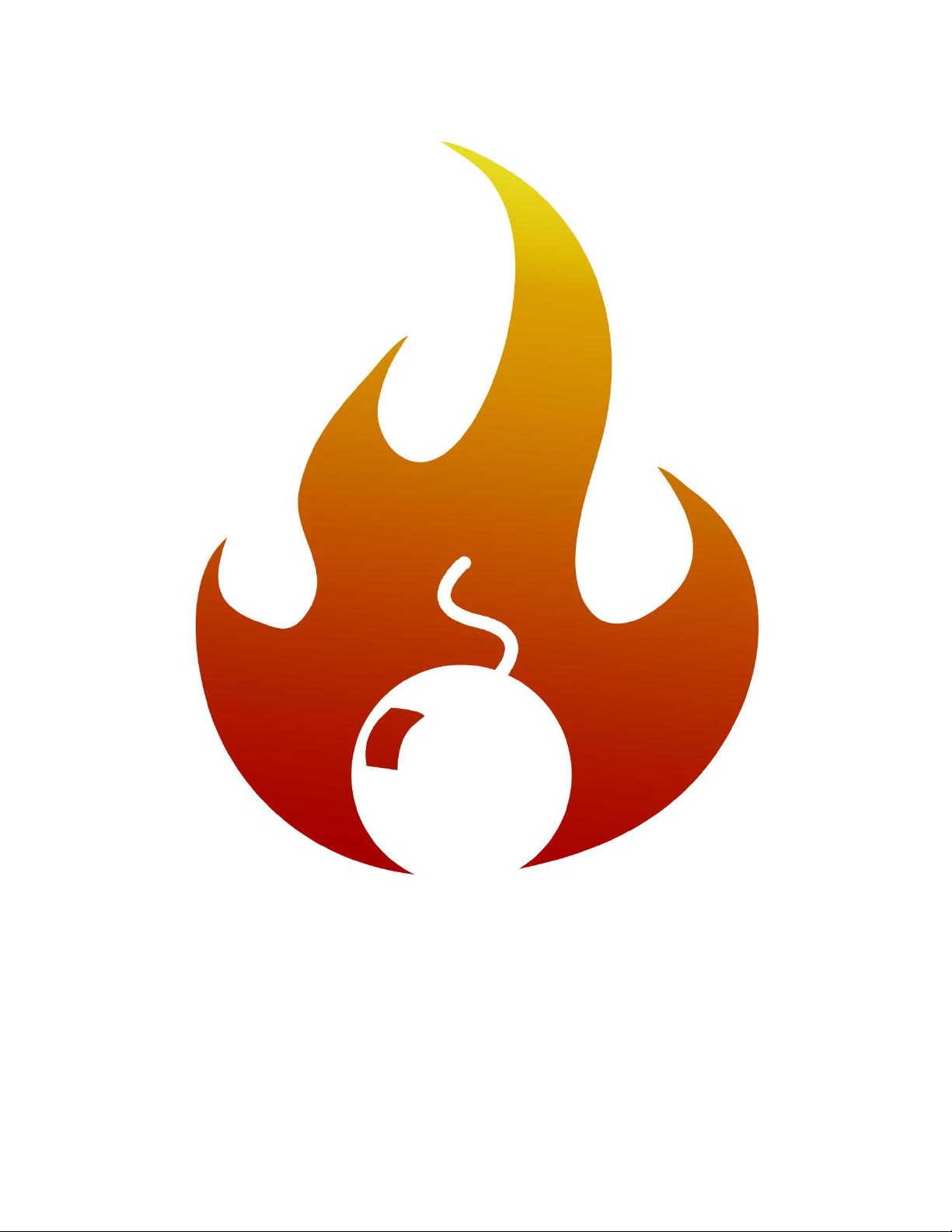
[THE CODING SURVIVAL GUIDE]
Habits and Pitfalls
Written by Martyr2 – Version 1.1
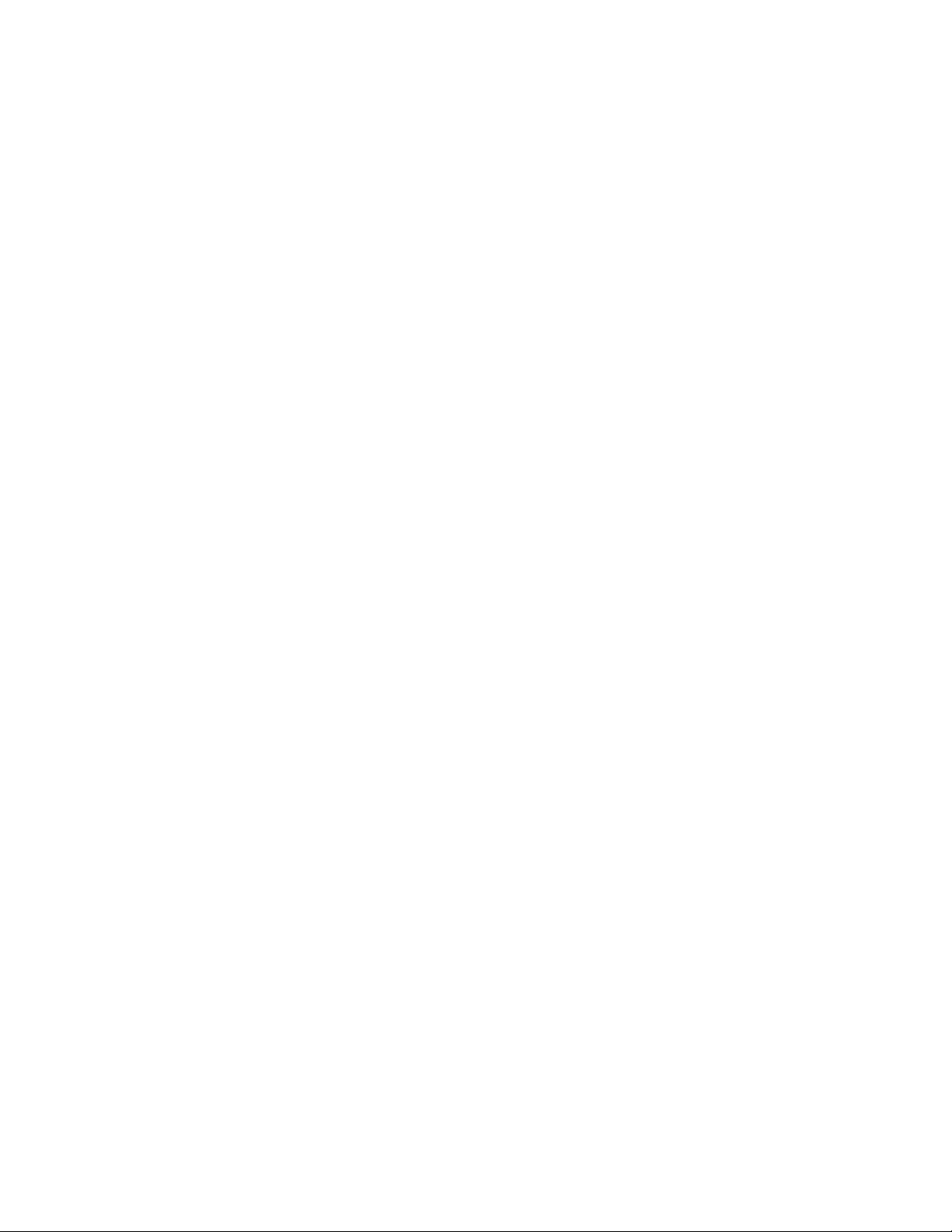
Table of Contents
Introduction ............................................................................................................ 1
Habits You Should Embrace ................................................................................. 1
Stubbing ............................................................................................................ 1
Use braces whenever possible .......................................................................... 2
Refactoring ........................................................................................................ 3
Limit parameters to functions ............................................................................. 4
Knowing when to use an abstract class versus an interface .............................. 5
Incessant testing ............................................................................................... 5
Picking a naming convention and be consistent with it ...................................... 6
Reduce the number of calls to a function if it always results in same answer ..... 6
Use flags where they make sense ..................................................................... 7
Ask yourself “What if this happened?” ............................................................... 8
Use constants .................................................................................................... 9
Be resourceful ................................................................................................... 9
Pitfalls to Avoid ................................................................................................... 10
Long methods, especially in main() ................................................................. 10
Not commenting enough, commenting too much or too vague of comments ... 10
Tight coupling .................................................................................................. 12
Variable letter abbreviations ............................................................................ 13
Too much error handling .................................................................................. 14
Complex conditionals ...................................................................................... 15
Conditionals that test the opposite of another conditional ................................ 16
Premature or incessant optimization ................................................................ 18
Reinventing the wheel ..................................................................................... 18
Using code you don’t understand .................................................................... 18
Accepting all the input a user gives “as is” ....................................................... 19
Thinking you are done when you are never really done ................................... 20
Conclusion ........................................................................................................... 20
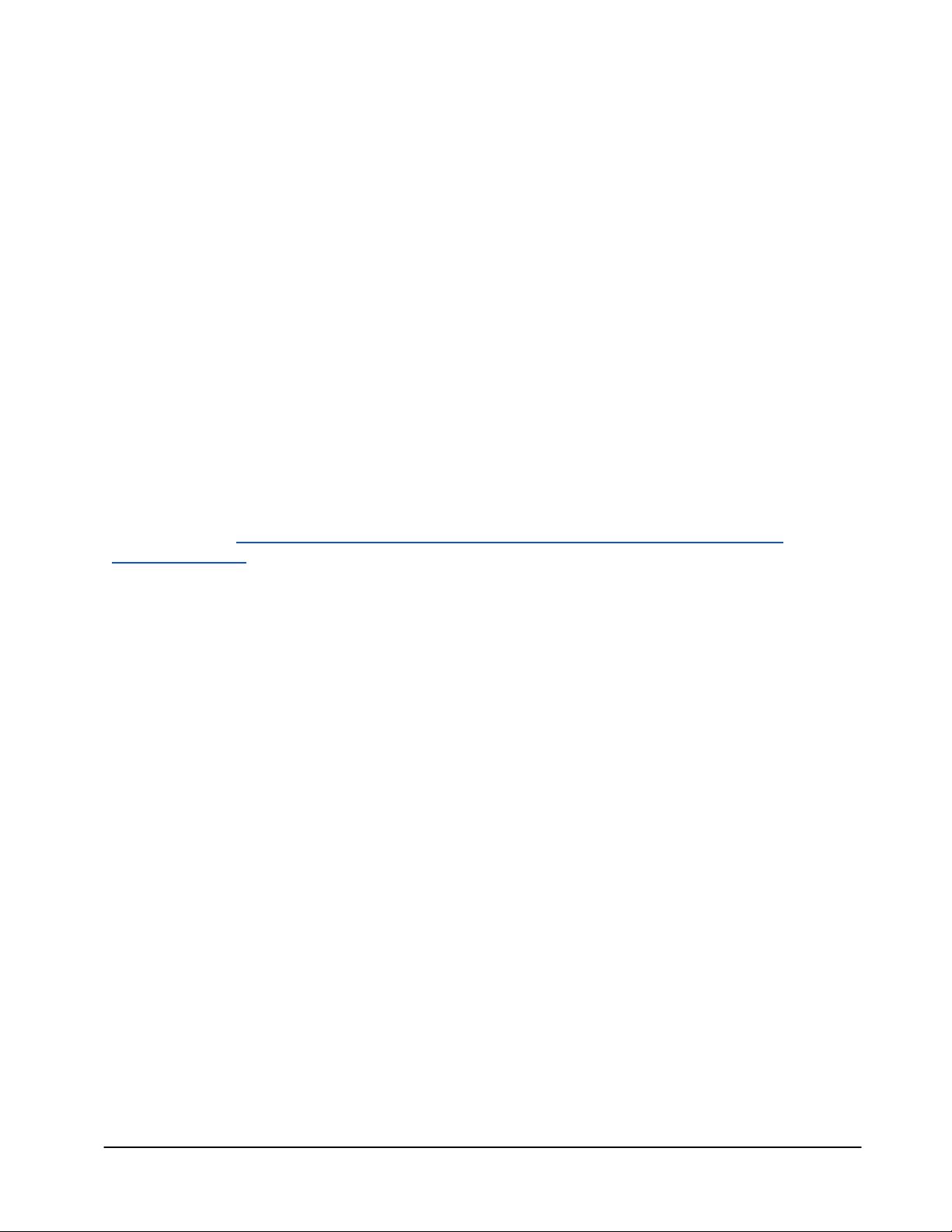
The Coding Survival Guide 1
Introduction
Learning how to code can be an enjoyable experience and a challenging one. You have to
be a person who is willing to sit on a problem for an hour, a day, a week or even a month
before the answer finally hits you. It is a profession where there are a lot of problems to
solve and you are expected to provide a lot of solutions. But those solutions will reward you
with a sense of accomplishment; a sense that you beat the problem and that can be a
euphoric experience.
Our only hope, as programmers, is that we learn enough tools and techniques to make the
problems we face seem less daunting. Maybe even allow us to get to the more difficult
problems which will change the world. This survival guide is meant to provide you with
some tips and tricks I have learned over the past 16 years of developing. By learning these
tricks I hope you will find programming a little easier and sharpen your programming skills.
In other words, I hope these tricks will help you stand on the shoulders of giants.
This guide will be your ticket to learning about the great habits you should embrace and the
pitfalls many programmers face during their careers. I have bundled this guide with the
popular ebook “The Programmers Idea Book: 200 Software Project Ideas and Tips to
Developing them” to provide you some direction while you code your next project. If you find
yourself stuck, review these tips and tricks and see if perhaps one will help you with your
current situation. Enjoy!
Habits You Should Embrace
Stubbing - Stubbing is the process by which you first identify the functions, methods,
classes, structures and files you will need to help solve the problem. You then code the
framework of those pieces to fill in later with detail. For example, if you were building a
calculator you might need functions to handle each of the arithmetic operations... add,
subtract, multiply, divide and maybe a function to print a sum of values. Below are three
stubs for add, subtract and a third to print the sum of values in an array. Notice that we have
only written the “signature” of these functions and have not actually written their function
bodies.
int add(int num1, int num2) {
}
int subtract(int num1, int num2) {
}
void printSum(int nums[]) {
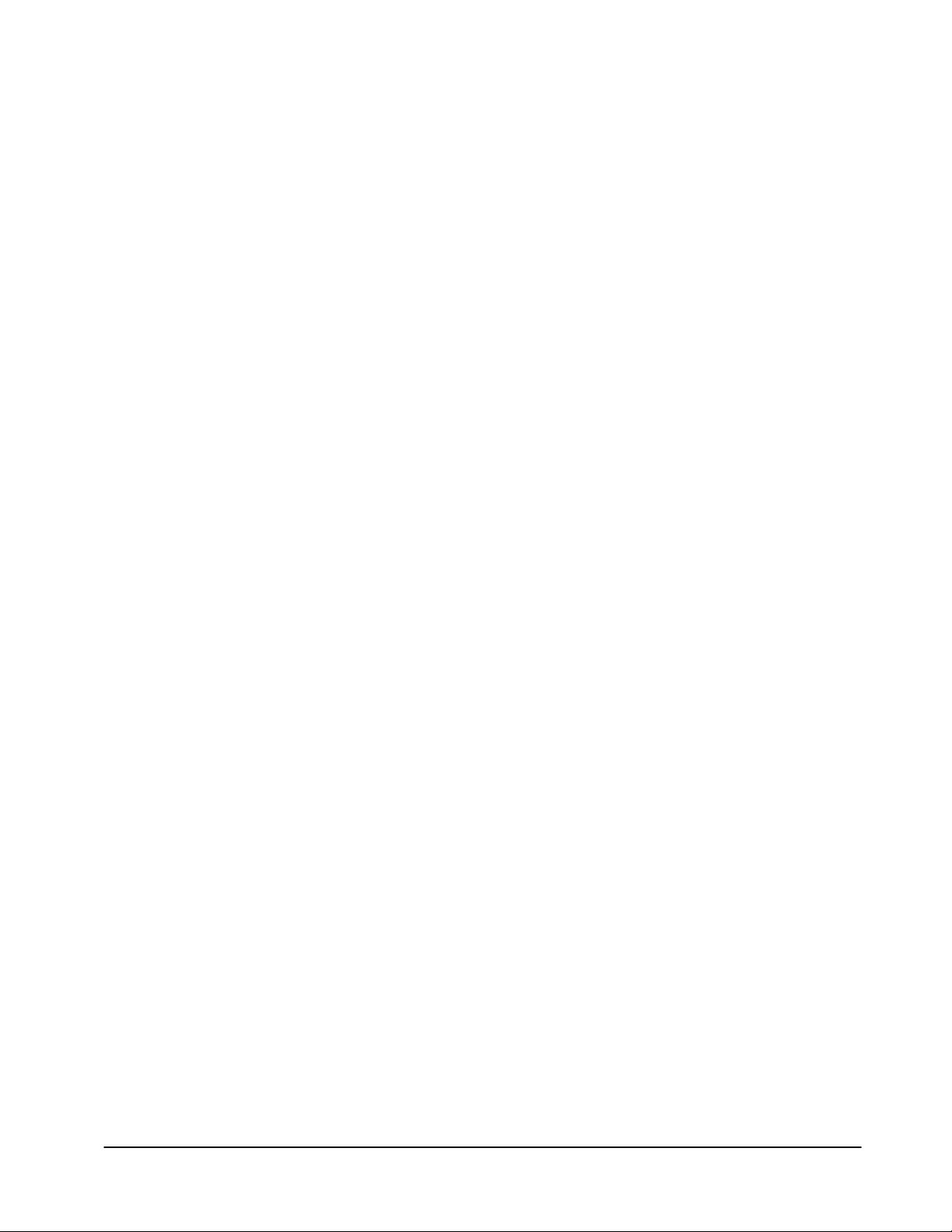
The Coding Survival Guide 2
}
You would stub out each function that you need for your calculator, and once you discover
all the functions and defined their headers, you will have a “code by number” setup where
you can easily code the body of each function. This would help you stay focused on each
function and reduce the complexity of having to keep track of multiple things going on at the
same time.
You could use stubbing to “stub out” whole classes, subsystems or often a whole program.
After stubbing out everything you will have a better picture of how each of the pieces might
work together. This will give you a better idea of where you might need that extra function or
class to complete the project.
Use braces whenever possible - One style I always recommend you do is putting in the
braces (also known as braces or brackets) characters for those languages that use them. In
languages like C++, C# or Java you can define one line loop bodies, and control flow
statements, that don’t require braces. When the compiler encounters this type of code it
looks for the braces to denote the body of the loop or statement. If these braces are not
found, the compiler will only execute the first line following the condition of the loop or
statement. Below is an example...
for (int i = 0; i < 10; i++)
cout << “Hello World”;
This for loop has a one line body which prints out the statement “Hello World” ten times. If
we were to add another line after the cout statement, the compiler would see that line as an
independent line, not part of the loop. For the compiler to see multiple lines in a loop, we
would have to add the braces...
for (int i = 0; i < 10; i++) {
cout << “Hello World”;
cout << “How are you doing?”;
}
This tip recommends that you just add the braces even if the body has one line. Why do
that? Well, there might be a chance (a pretty good chance in fact) that you or another
person may need to add additional commands to the body later. At a future time you would
have to add the braces anyways. So you might as well do it now and make it easier for
others later. This future person may not be you and they could forget the braces, causing a
hidden issue. So add them as a matter of kindness and increased readability. The braces
剩余21页未读,继续阅读
资源评论
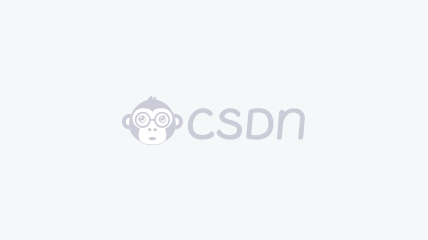

冰水比水冰
- 粉丝: 195
- 资源: 11
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

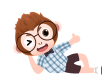
最新资源
- 鼎微R16中控升级包R16-4.5.10-20170221及强制升级方法
- 鼎微R16中控升级包公版UI 2015及强制升级方法,救砖包
- 基于CSS与JavaScript的积分系统设计源码
- 生物化学作业_1_生物化学作业资料.pdf
- 基于libgdx引擎的Java开发连连看游戏设计源码
- 基于MobileNetV3的SSD目标检测算法PyTorch实现设计源码
- 基于Java JDK的全面框架设计源码学习项目
- 基于Python黑魔法原理的Python编程技巧设计源码
- 基于Python的EducationCRM管理系统前端设计源码
- 基于Django4.0+Python3.10的在线学习系统Scss设计源码
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


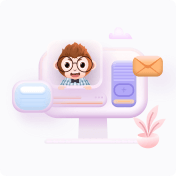
安全验证
文档复制为VIP权益,开通VIP直接复制
