#include "huaray_camera.h"
#include <QImage>
#include <QLabel>
#include <QMetaMethod>
#include <QPixmap>
huarayspc::huarayInterface huaray_camera::huarayctrl;
huaray_camera::huaray_camera(QObject *parent)
:abstractCamera(parent)
{
g_hEventBusy=CreateEvent(nullptr,true,false,nullptr);
g_hEventImageReady=CreateEvent(nullptr,true,false,nullptr);
g_hEventSnap=CreateEvent(nullptr,true,false,nullptr);
InitializeCriticalSection(&m_triggleMutex);
InitializeCriticalSection(&m_shwMutex);
grab=new grabThread_Huaray(this);
grab->setcamera(this);
//connect(this,&huaray_camera::setStreamWnd,qobject_cast<grabThread_Huaray*>(grab),&grabThread_Huaray::setstreamwnd);
}
huaray_camera::~huaray_camera()
{
closeCamera();
if(g_hEventImageReady)
CloseHandle(g_hEventImageReady);
if(g_hEventBusy)
CloseHandle(g_hEventBusy);
if(g_hEventSnap)
CloseHandle(g_hEventSnap);
DeleteCriticalSection(&m_triggleMutex);
DeleteCriticalSection(&m_shwMutex);
}
bool huaray_camera::openCamera(unsigned int index)
{
if(!huarayctrl.ErrMessage().isEmpty())
{
setErrMessage(huarayctrl.ErrMessage());
return false;
}
auto devicelist=huarayctrl.getDeviceList();
if(devicelist.nDevNum<=0)
{
setErrMessage("camera open fail:there is no camera founded");
return false;
}
else if(index==0||index>devicelist.nDevNum)
{
setErrMessage(QString("camera open fail:index out of range [1,%1]").arg(devicelist.nDevNum));
return false;
}
if(isopened())return true;
int no=index-1;
auto rslt=huarayctrl.CreateHandle(&handle,modeByIndex,(void*)&no);
if(IMV_OK!=rslt)
{
setErrMessage(QString("Create devHandle failed! ErrorCode[%1]").arg(rslt));
return false;
}
rslt=huarayctrl.Open(handle);
if(IMV_OK!=rslt)
{
setErrMessage(QString("Open camera failed! ErrorCode[%1]").arg(rslt));
return false;
}
QString aserial=devicelist.pDevInfo[no].serialNumber;
setSerialno(aserial);
setIsopened(true);
getcamWidth();
getcamHeight();
getcamExporseTime();
getcamTriggermode();
return true;
}
bool huaray_camera::openCamera(QString serial)
{
if(!huarayctrl.ErrMessage().isEmpty())
{
setErrMessage(huarayctrl.ErrMessage());
return false;
}
auto devicelist=huarayctrl.getDeviceList();
if(devicelist.nDevNum<=0)
{
setErrMessage("open camera failed:there is no camera founded");
return false;
}
unsigned index=0;
for(unsigned int n=0;n<devicelist.nDevNum;n++)
{
QString aserial=devicelist.pDevInfo[n].serialNumber;
if(aserial.compare(serial)==0)
{
index=n+1;
break;
}
}
if(index>0)
return openCamera(index);
else
{
setErrMessage(QString("open camera failed as no camera named %1 is founded").arg(serial));
return false;
}
return true;
}
bool huaray_camera::closeCamera()
{
if(grab)
{
if(grab->isRunning())
{
grab->setM_quit(true);
grab->wait();
}
}
if(isopened())
{
if(isGrabing())closegrab();
auto vl=huarayctrl.Close(handle);
if(IMV_OK!=vl)
{
setErrMessage(QString("close camera failed! ErrorCode[%1]").arg(vl));
return false;
}
setIsopened(false);
huarayctrl.DestroyHandle(handle);
handle=nullptr;
return true;
}
return true;
}
bool huaray_camera::setcamExporseTime(QString vl)
{
bool blok;
auto floatvl=vl.toDouble(&blok);
if(false==blok)
{
setErrMessage(QString("setcamExporseTime failed as ExposureTime=%1 is Invalid ").arg(vl));
return false;
}
if(isopened()==false)
{
setErrMessage("setcamExporseTime failed as camera is not opened");
return false;
}
auto ret=huarayctrl.SetDoubleFeatureValue(handle,(const char*)"ExposureTime",floatvl);
if(IMV_OK!=ret)
{
setErrMessage(QString("setcamExporseTime failed! ErrorCode[%1]").arg(ret));
return false;
}
getcamExporseTime();
return true;
}
bool huaray_camera::getcamExporseTime()
{
if(isopened()==false)
{
setErrMessage("getcamExporseTime failed as camera is not opened");
return false;
}
double flt=0.00;
auto ret=huarayctrl.GetDoubleFeatureValue(handle,(const char*)"ExposureTime",&flt);
if(IMV_OK!=ret)
{
setErrMessage(QString("getcamExporseTime failed! ErrorCode[%1]").arg(ret));
return false;
}
setExposureTime(QString::number(flt));
return true;
}
bool huaray_camera::setcamWidth(unsigned int width)
{
if(isopened()==false)
{
setErrMessage("setcamWidth failed as camera is not opened");
return false;
}
auto ret=huarayctrl.SetIntFeatureValue(handle,(const char*)"Width",width);
if(IMV_OK!=ret)
{
setErrMessage(QString("setcamWidth failed! ErrorCode[%1]").arg(ret));
return false;
}
getcamWidth();
return true;
}
bool huaray_camera::getcamWidth()
{
if(isopened()==false)
{
setErrMessage("getcamWidth failed as camera is not opened");
return false;
}
int64_t val=0;
auto ret=huarayctrl.GetIntFeatureValue(handle,(const char*)"Width",&val);
if(IMV_OK!=ret)
{
setErrMessage(QString("getcamWidth failed! ErrorCode[%1]").arg(ret));
return false;
}
setWidth(val);
return true;
}
bool huaray_camera::setcamHeight(unsigned int height)
{
if(isopened()==false)
{
setErrMessage("setcamheight failed as camera is not opened");
return false;
}
auto ret=huarayctrl.SetIntFeatureValue(handle,(const char*)"Height",height);
if(IMV_OK!=ret)
{
setErrMessage(QString("setcamheight failed! ErrorCode[%1]").arg(ret));
return false;
}
getcamHeight();
return true;
}
bool huaray_camera::getcamHeight()
{
if(isopened()==false)
{
setErrMessage("getcamheight failed as camera is not opened");
return false;
}
int64_t val=0;
auto ret=huarayctrl.GetIntFeatureValue(handle,(const char*)"Height",&val);
if(IMV_OK!=ret)
{
setErrMessage(QString("getcamheight failed! ErrorCode[%1]").arg(ret));
return false;
}
setHeight(val);
return true;
}
bool huaray_camera::setcamTriggermode(bool modetriggerOn)
{
if(isopened()==false)
{
setErrMessage("setcamTriggermode failed as camera is not opened");
return false;
}
auto ret=huarayctrl.SetEnumFeatureValue(handle,"TriggerMode",modetriggerOn?1:0);
if(IMV_OK!=ret)
{
setErrMessage(QString("setcamTriggermode failed! ErrorCode[%1]").arg(ret));
return false;
}
getcamTriggermode();
return true;
}
bool huaray_camera::getcamTriggermode()
{
if(isopened()==false)
{
setErrMessage("getcamTriggermode failed as camera is not opened");
return false;
}
uint64_t vl;
auto ret=huarayctrl.GetEnumFeatureValue(handle,"TriggerMode", &vl);
if(IMV_OK!=vl)
{
setErrMessage(QString("getcamTriggermode failed! ErrorCode[%1]").arg(ret));
return false;
}
setIstriggerModeON(vl);
return true;
}
bool huaray_camera::setcamTriggersource(TRIGGERSOURCE sc)
{
if(isopened()==false)
{
setErrMessage("setcamTriggersource failed as camera is not opened");
return false;
}
int m_nTriggerSource=0;
int nRet = IMV_OK;
if(sc==HARDWAR
没有合适的资源?快使用搜索试试~ 我知道了~
海康大华相机SDK封装Qt版
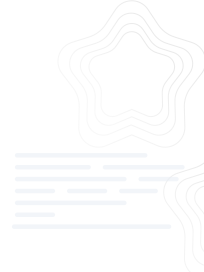
共311个文件
hpp:197个
h:74个
dll:15个

需积分: 28 8 下载量 199 浏览量
2022-11-04
08:22:04
上传
评论 1
收藏 168.53MB ZIP 举报
温馨提示
海康大华相机的Qt平台开发接口封装,操作简单便捷。
资源推荐
资源详情
资源评论
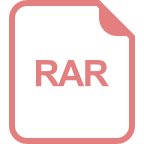
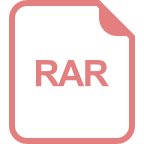
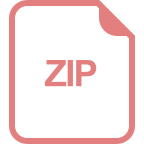
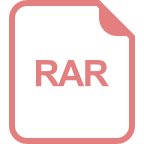
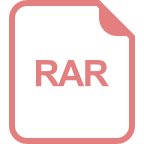
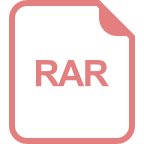
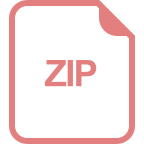
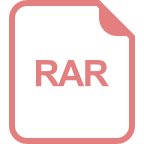
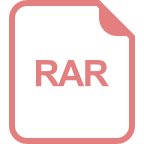
收起资源包目录

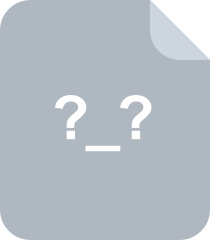
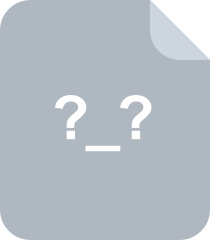
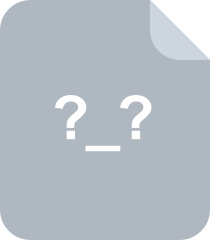
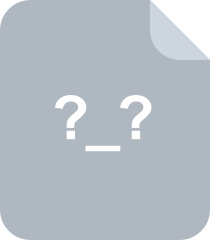
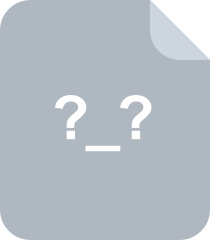
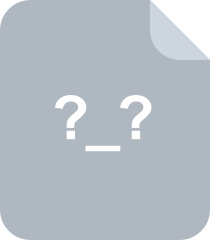
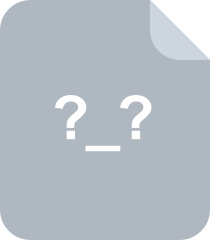
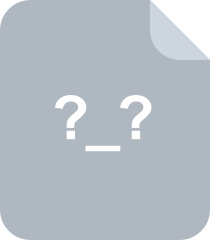
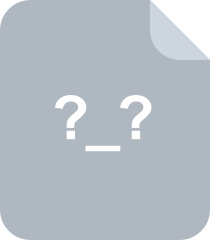
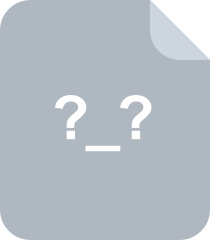
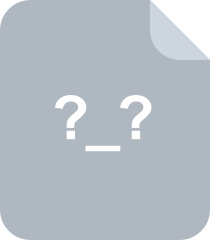
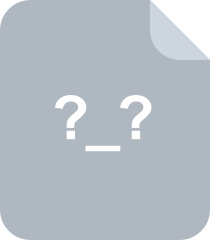
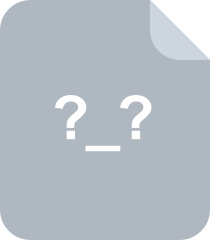
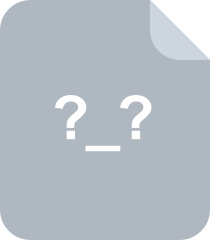
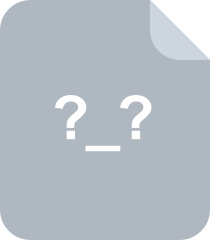
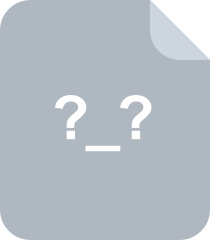
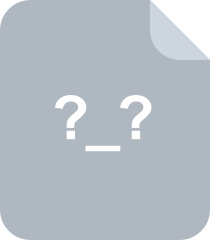
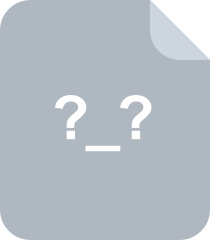
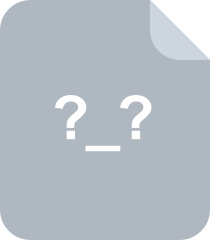
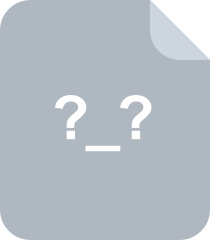
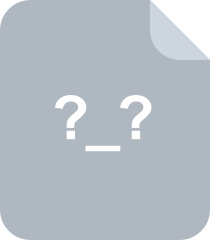
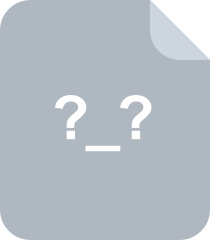
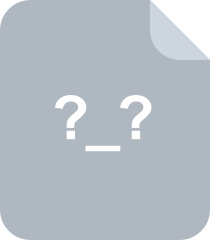
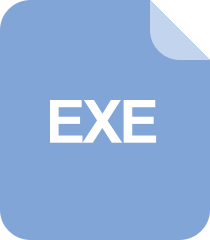
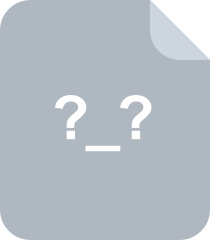
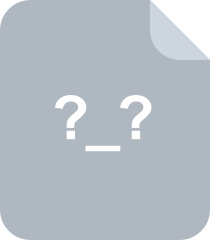
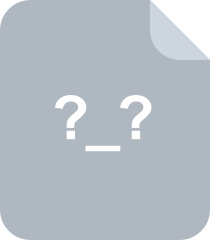
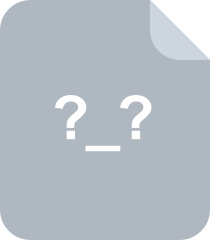
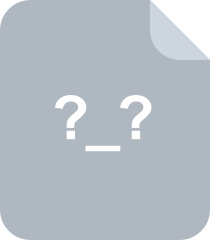
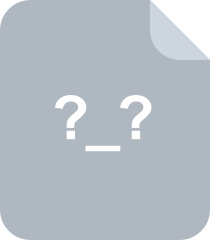
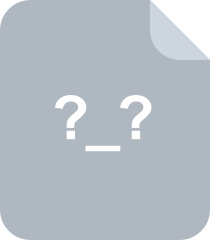
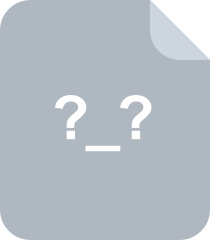
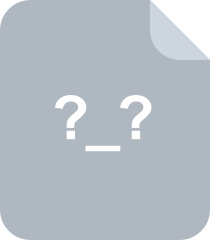
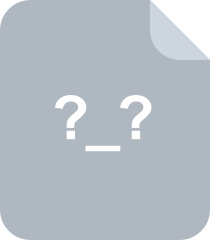
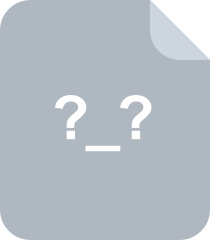
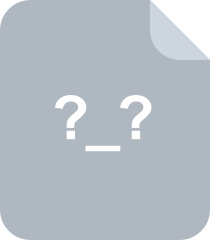
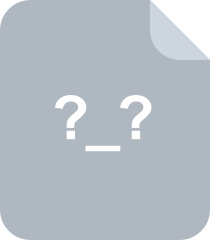
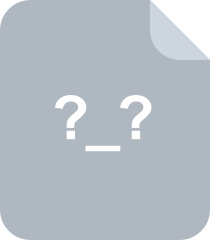
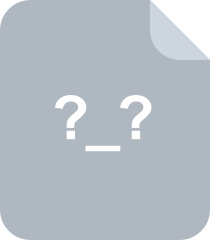
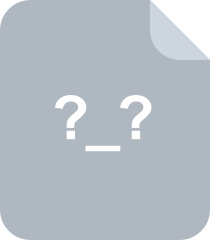
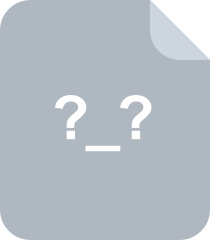
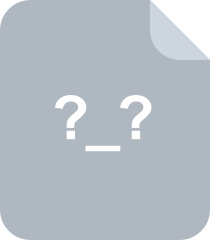
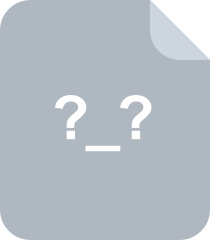
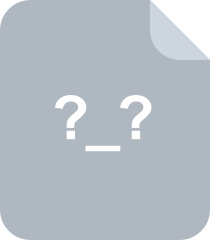
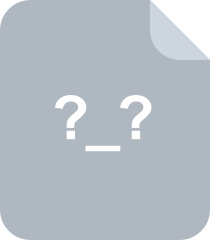
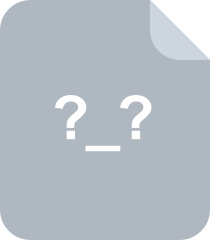
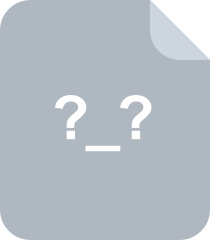
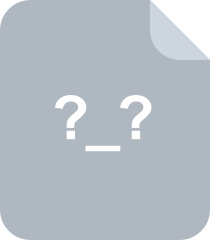
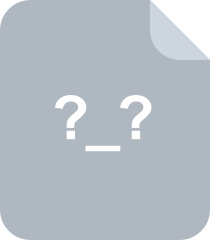
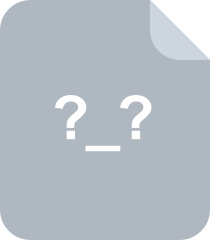
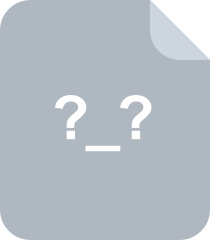
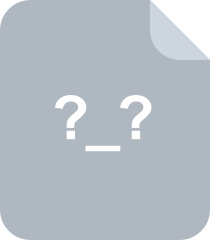
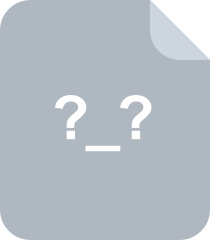
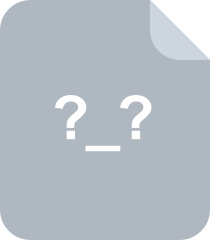
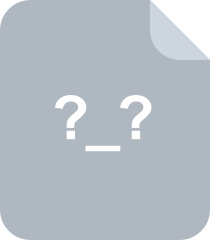
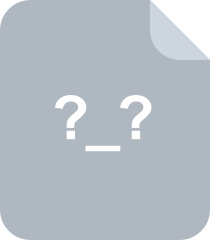
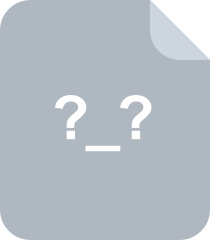
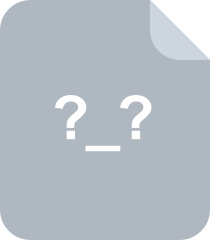
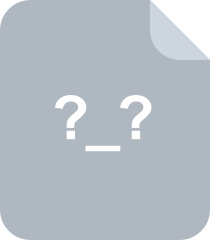
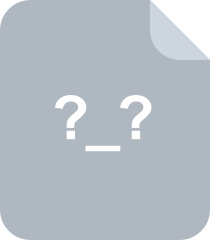
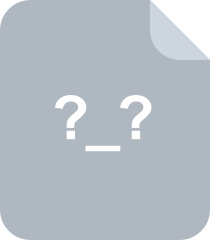
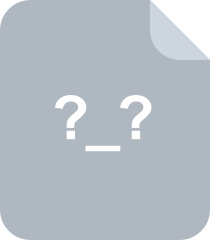
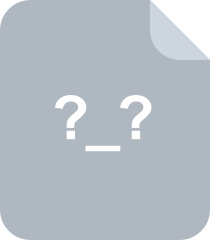
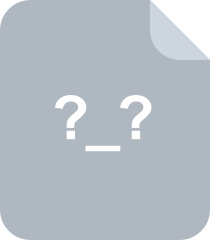
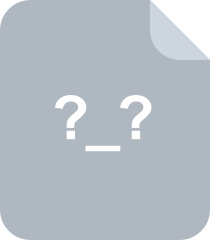
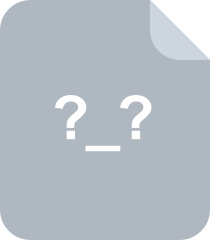
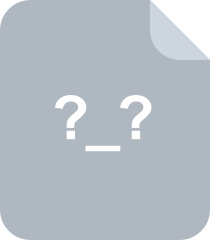
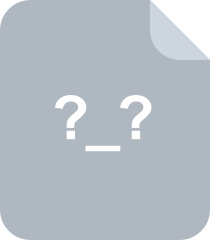
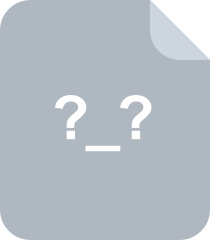
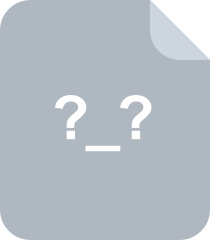
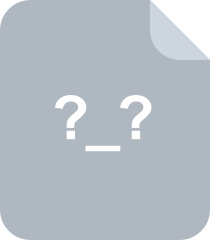
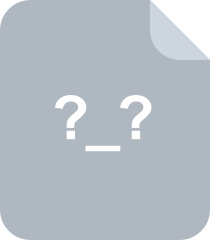
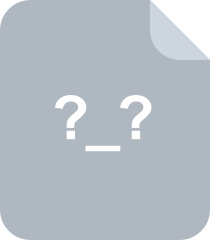
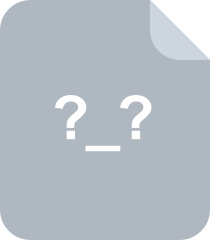
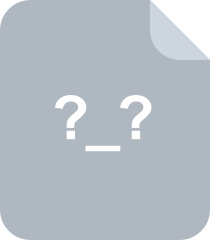
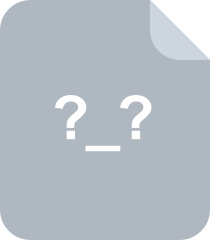
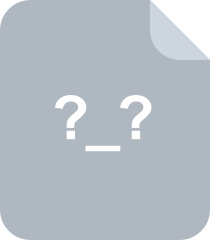
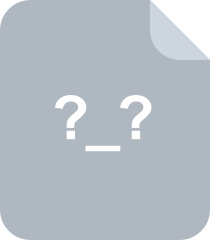
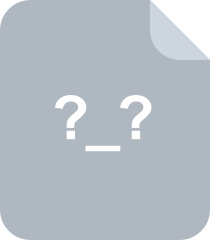
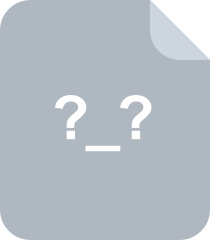
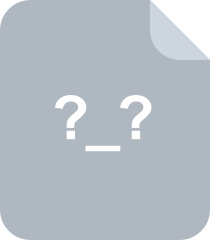
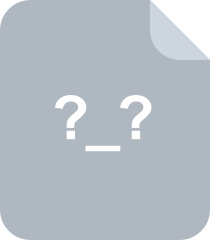
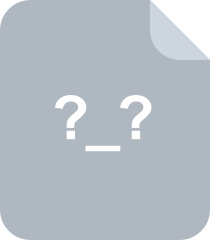
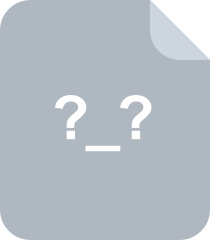
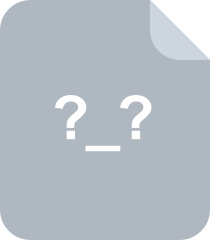
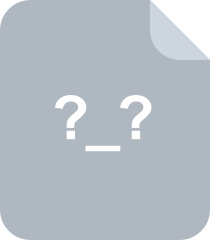
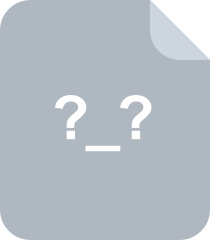
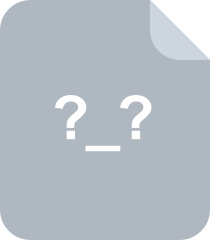
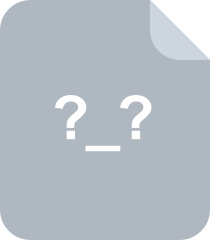
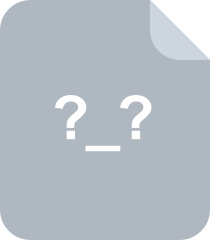
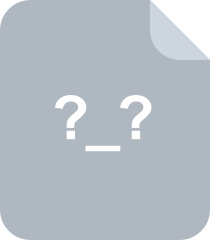
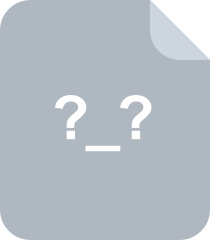
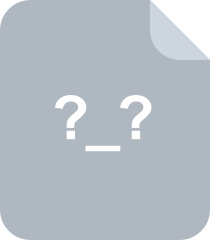
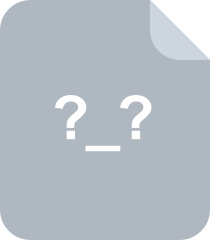
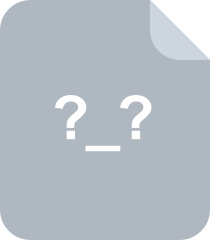
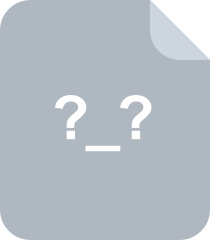
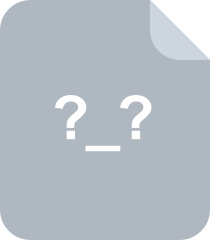
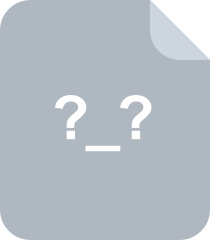
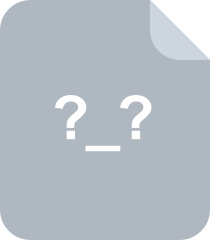
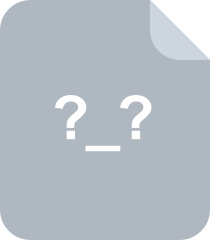
共 311 条
- 1
- 2
- 3
- 4
资源评论
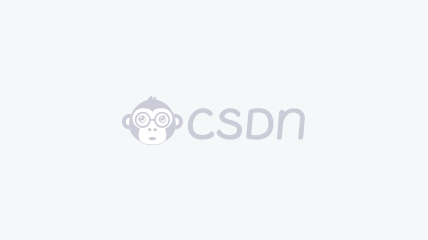

luckyfone
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

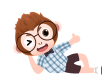
安全验证
文档复制为VIP权益,开通VIP直接复制
