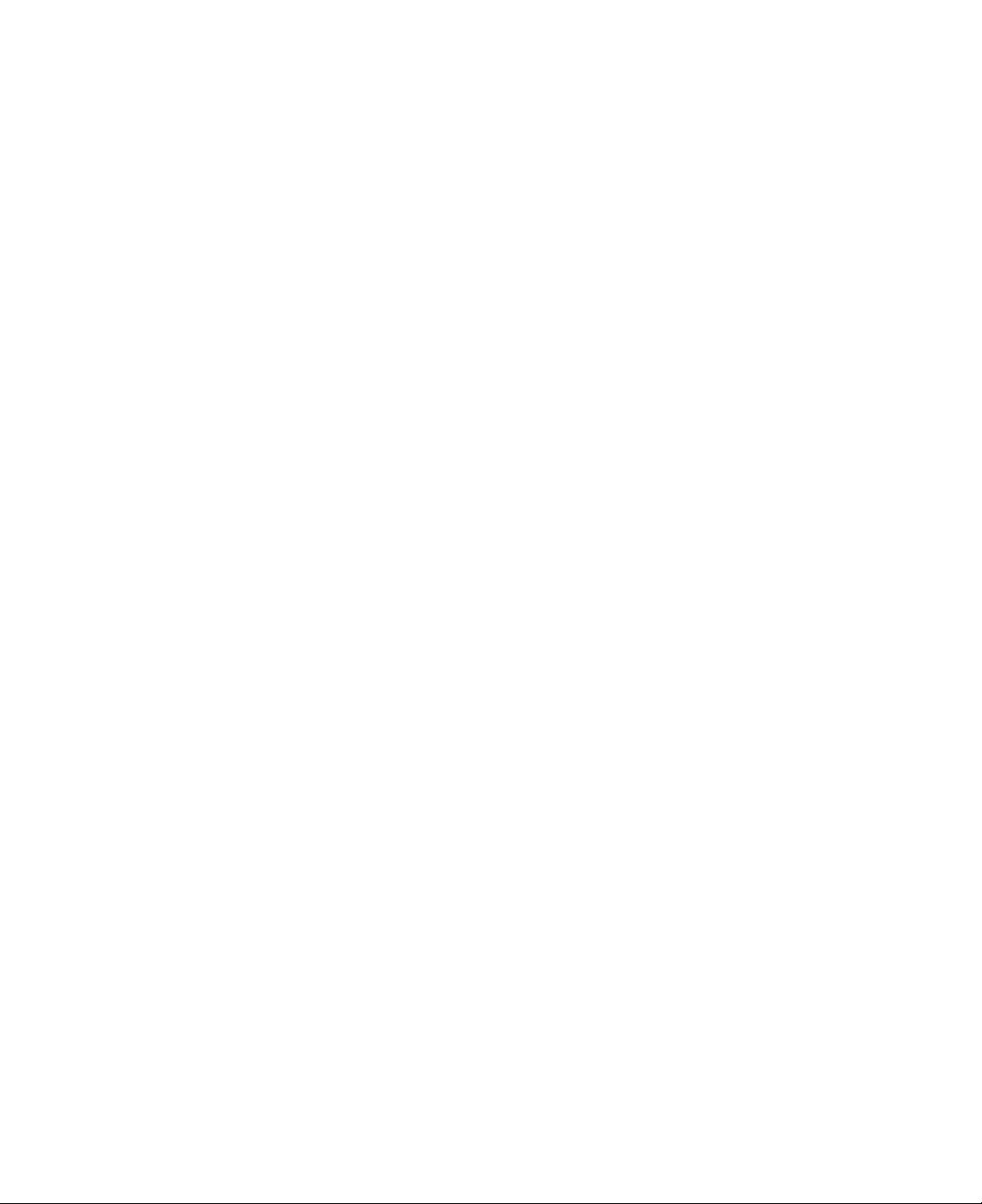
Local Variables......................................................................................................................................................................... 153
The self Keyword...................................................................................................................................................................... 156
Allocating and Returning Objects from Methods................................................................................................................... 157
Exercises.................................................................................................................................................................................. 163
Chapter 8. Inheritance................................................................................................................................................................ 164
It All Begins at the Root........................................................................................................................................................... 164
Extension Through Inheritance: Adding New Methods......................................................................................................... 169
Overriding Methods
................................................................................................................................................................. 182
Extension Through Inheritance: Adding New Instance Variables......................................................................................... 188
Abstract Classes....................................................................................................................................................................... 190
Exercises................................................................................................................................................................................... 191
Chapter 9. Polymorphism, Dynamic Typing, and Dynamic Binding......................................................................................... 194
Polymorphism: Same Name, Different Class.......................................................................................................................... 194
Dynamic Binding and the id Type........................................................................................................................................... 198
Compile Time Versus Runtime Checking............................................................................................................................... 200
The id Data Type and Static Typing........................................................................................................................................ 201
Asking Questions About Classes............................................................................................................................................. 202
Exception Handling Using @try............................................................................................................................................. 207
Exercises.................................................................................................................................................................................. 210
Chapter 10. More on Variables and Data Types......................................................................................................................... 212
Initializing Classes................................................................................................................................................................... 212
Scope Revisited........................................................................................................................................................................ 214
Storage Class Specifiers.......................................................................................................................................................... 220
Enumerated Data Types.......................................................................................................................................................... 222
The typedef Statement............................................................................................................................................................ 225
Data Type Conversions............................................................................................................................................................ 227
Exercises.................................................................................................................................................................................. 229
Chapter 11. Categories and Protocols......................................................................................................................................... 232
Categories................................................................................................................................................................................ 232
Protocols.................................................................................................................................................................................. 238
Composite Objects................................................................................................................................................................... 242
Exercises.................................................................................................................................................................................. 243
Chapter 12. The Preprocessor..................................................................................................................................................... 246
The #define Statement............................................................................................................................................................ 246
The #import Statement........................................................................................................................................................... 254
Conditional Compilation......................................................................................................................................................... 257
Exercises.................................................................................................................................................................................. 260
Chapter 13. Underlying C Language Features............................................................................................................................ 262
Arrays...................................................................................................................................................................................... 263
Functions................................................................................................................................................................................. 269
Structures................................................................................................................................................................................ 278
Pointers................................................................................................................................................................................... 290
Unions..................................................................................................................................................................................... 309
They’re Not Objects!................................................................................................................................................................ 312
Miscellaneous Language Features........................................................................................................................................... 312
How Things Work.................................................................................................................................................................... 317
Exercises.................................................................................................................................................................................. 319
Part II: The Foundation Framework........................................................ 322
Chapter 14. Introduction to the Foundation Framework.......................................................................................................... 324
Foundation Documentation.................................................................................................................................................... 324
Chapter 15. Numbers, Strings, and Collections.......................................................................................................................... 328
Number Objects....................................................................................................................................................................... 329
String Objects.......................................................................................................................................................................... 333
Array Objects........................................................................................................................................................................... 348
Synthesized AddressCard Methods........................................................................................................................................ 356
Dictionary Objects................................................................................................................................................................... 374
Set Objects............................................................................................................................................................................... 377
Exercises.................................................................................................................................................................................. 382
Chapter 16. Working with Files.................................................................................................................................................. 384
Managing Files and Directories: NSFileManager.................................................................................................................. 385