import java.io.IOException;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import com.change_vision.jude.api.inf.exception.LicenseNotFoundException;
import com.change_vision.jude.api.inf.exception.NonCompatibleException;
import com.change_vision.jude.api.inf.exception.ProjectNotFoundException;
import com.change_vision.jude.api.inf.exception.ProjectLockedException;
import com.change_vision.jude.api.inf.model.IAttribute;
import com.change_vision.jude.api.inf.model.IClass;
import com.change_vision.jude.api.inf.model.IConstraint;
import com.change_vision.jude.api.inf.model.IElement;
import com.change_vision.jude.api.inf.model.IGeneralization;
import com.change_vision.jude.api.inf.model.IModel;
import com.change_vision.jude.api.inf.model.INamedElement;
import com.change_vision.jude.api.inf.model.IOperation;
import com.change_vision.jude.api.inf.model.IPackage;
import com.change_vision.jude.api.inf.model.IParameter;
import com.change_vision.jude.api.inf.model.IRealization;
import com.change_vision.jude.api.inf.project.ProjectAccessor;
import com.change_vision.jude.api.inf.project.ProjectAccessorFactory;
/**
* 指定されたプロジェクトから、クラス情報構築するクラス。
* Class to build class definition from selected project.
*/
public class ClassDefinitionBuilder {
private static final String EMPTY_COLUMN = "";
private String inputFile;
/**
* @param inputFile
* 入力するプロジェクト
* File to input
*/
public ClassDefinitionBuilder(String inputFile) {
this.inputFile = inputFile;
}
/**
* クラス情報を取得する。
* Get class information.
*
* @return クラス情報(Listに格納されたStringのList)
* Class information (String List stored in the List)
* @throws LicenseNotFoundException
* ライセンスが見つかりません
* License cannot be found
* @throws ProjectNotFoundException
* プロジェクトが見つかりません
* Project cannot be found
* @throws NonCompatibleException
* モデルバージョンが古い(プロジェクトを最後に編集したJUDEよりもAPIのバージョンが古い)です
* Old Model Version (The version of API is older than the version of JUDE that the project has been last edited with)
* @throws ClassNotFoundException
* 読み込めないモデルがあります
* Cannot read some models
* @throws IOException
* 入出力エラーです
* Input/Output error
* @throws ProjectLockedException
* プロジェクトファイルがロックされています
* Project file has been locked
*/
public List getContents() throws LicenseNotFoundException, ProjectNotFoundException,
NonCompatibleException, IOException, ClassNotFoundException, ProjectLockedException, Throwable {
// プロジェクトを開いて、起点となるモデルを取得する
ProjectAccessor prjAccessor = ProjectAccessorFactory.getProjectAccessor();
prjAccessor.open(inputFile);
IModel iModel = prjAccessor.getProject();
List contents = new ArrayList();
contents.add(getHeader());
// プロジェクトに含まれる全てのパッケージを取得する
List iPackages = getAllPackages(iModel);
// パッケージ毎に情報を構築する
for (Iterator iter = iPackages.iterator(); iter.hasNext();) {
IPackage iPackage = (IPackage)iter.next();
contents.addAll(getClassInfos(iPackage));
}
// プロジェクトを閉じる
prjAccessor.close();
return contents;
}
/**
* ヘッダ情報を取得する。
* Get header information.
* @return ヘッダ情報(StringのList)
* Header information (String List)
*/
private List getHeader() {
List header = new ArrayList();
header.add("Class");
header.add("Attribute/Operation");
header.add("Definition");
header.add("Generalization");
header.add("Realization");
return header;
}
/**
* プロジェクト配下の全てのパッケージを取得する。
* Get all packages in project.
* @param project
* プロジェクト
* Project
* @return パッケージリスト
* Package list
*/
private List getAllPackages(IModel project) {
List packages = new ArrayList();
packages.add(project);
return getPackages(project, packages);
}
/**
* 指定パッケージ配下のパッケージを、再帰的に全て取得する。
* How to get packages under Package recursively
* @param iPackage
* 指定パッケージ
* Selected package
* @param iPackages
* パッケージ一覧を格納するリスト
* List of all stored packages
* @return パッケージ一覧を格納したリスト
* List of all stored packages
*/
private List getPackages(IPackage iPackage, List iPackages) {
INamedElement[] iNamedElements = iPackage.getOwnedElements();
for (int i = 0; i < iNamedElements.length; i++) {
INamedElement iNamedElement = iNamedElements[i];
if (iNamedElement instanceof IPackage) {
iPackages.add(iNamedElement);
getPackages((IPackage)iNamedElement, iPackages);
}
}
return iPackages;
}
/**
* 指定パッケージ配下のクラス情報を取得する。
* Get class information in selected package.
* @param iPackage
* 指定パッケージ
* Selected package
* @return クラス情報(Listに格納されたStringのList)
* Class information (String List stored in the list)
*/
private List getClassInfos(IPackage iPackage) {
List classInfos = new ArrayList();
List classes = getIClasses(iPackage);
for (Iterator iter = classes.iterator(); iter.hasNext();) {
IClass iClass = (IClass)iter.next();
classInfos.addAll(getClassInfo(iClass));
}
return classInfos;
}
/**
* 指定パッケージ配下のクラスを取得する。
* Get classes in selected package.
* @param iPackage
* 指定パッケージ
* Selected package
* @return クラス一覧を格納したリスト
* List of all stored classes
*/
private List getIClasses(IPackage iPackage) {
List iClasses = new ArrayList();
INamedElement[] iNamedElements = iPackage.getOwnedElements();
for (int i = 0; i < iNamedElements.length; i++) {
INamedElement iNamedElement = iNamedElements[i];
if (iNamedElement instanceof IClass) {
iClasses.add(iNamedElement);
}
}
return iClasses;
}
/**
* 指定クラスの情報を取得する。
* Get information of selected class.
* @param iClass
* 指定クラス
* Selected class
* @return クラス情報(Listに格納されたStringのList)
* Class information (Strings list stored in the list)
*/
private List getClassInfo(IClass iClass) {
List lines = new ArrayList();
lines.add(getClassNameLine(iClass));
lines.addAll(getAttributeLines(iClass));
lines.addAll(getOperationLines(iClass));
return lines;
}
/**
* クラス名の行の情報を取得する。
* Get class name line.
* @param iClass
* クラス
* Class
* @return クラス名の行の情報(StringのList)
* Information of Class name lines (String list)
*/
private List getClassNameLine(IClass iClass) {
List line = new ArrayList();
line.add(getFullName(iClass
没有合适的资源?快使用搜索试试~ 我知道了~
JUDE-Community 5.5.2
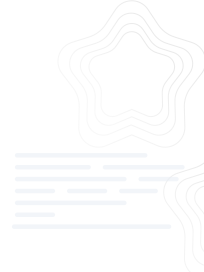
共626个文件
html:532个
png:35个
jude:11个


温馨提示
JUDE-Community 5.5.2 典藏绿色汉化版 Jude改名为astah后很多功能被限制使用 此版本为完整功能典藏版,增加了罕见的汉化补丁:) 无须安装,解压后。 1)Windows, 双击 jude.bat,点击yes 2)Linux,在终端中,当前路径下,执行命令 ./jude & ,点击确定
资源推荐
资源详情
资源评论
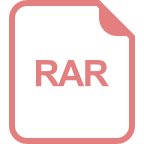
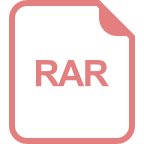
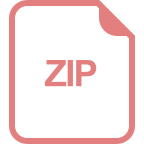
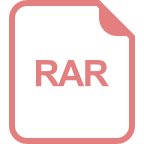
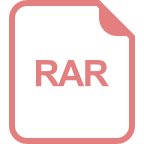
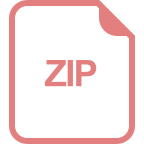
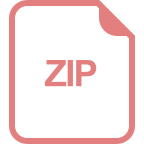
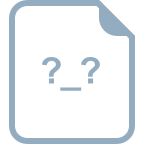
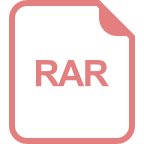
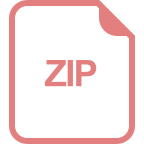
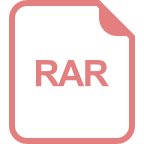
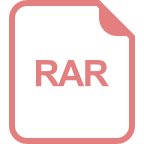
收起资源包目录

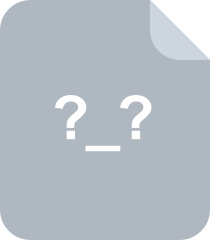
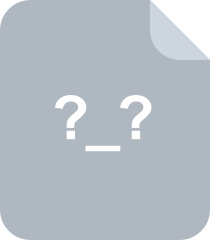
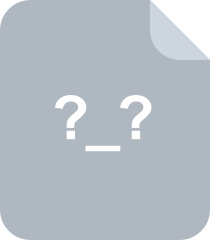
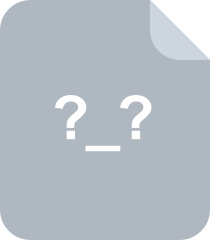
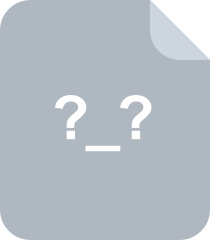
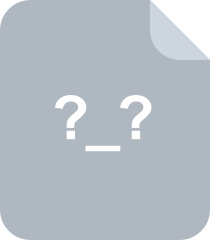
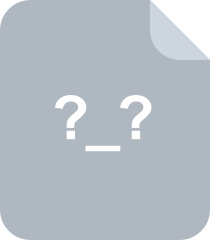
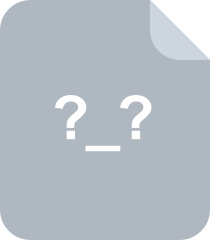
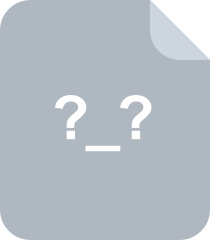
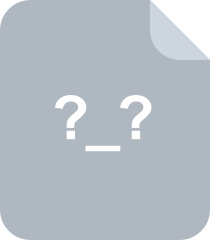
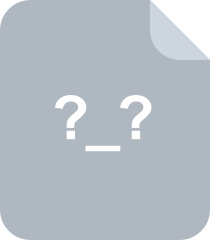
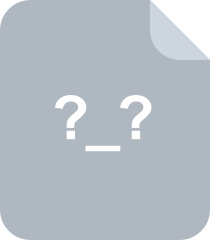
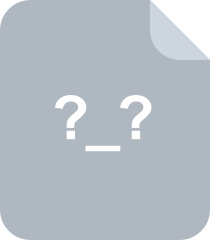
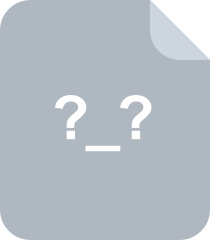
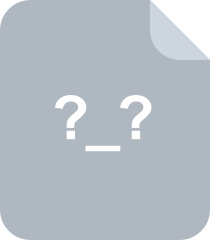
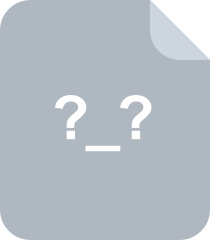
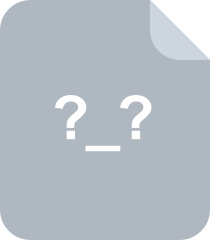
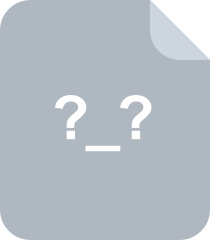
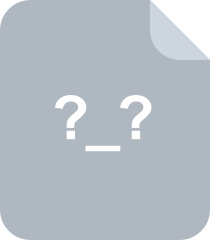
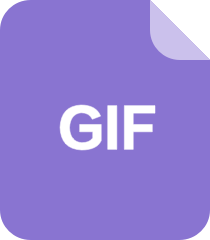
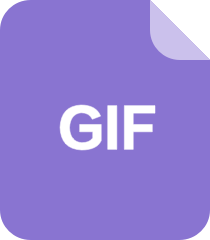
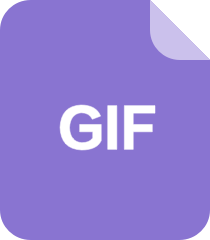
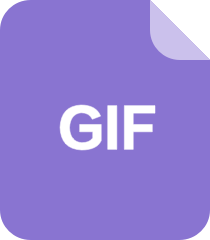
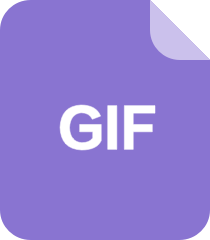
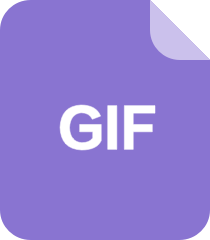
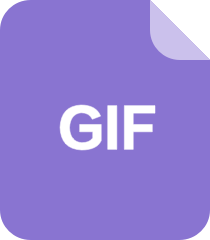
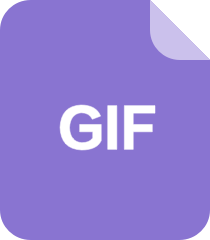
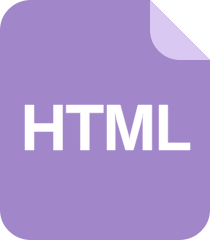
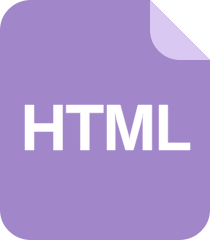
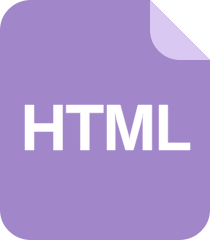
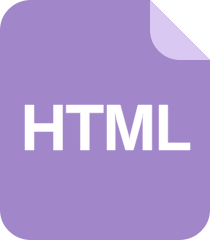
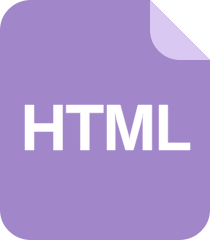
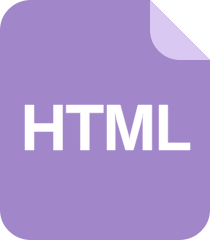
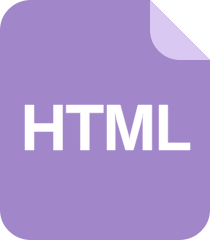
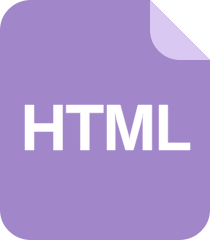
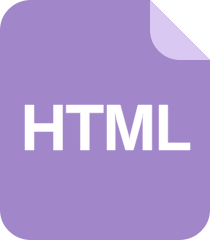
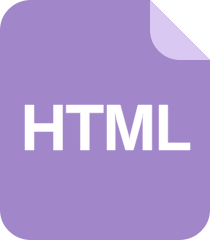
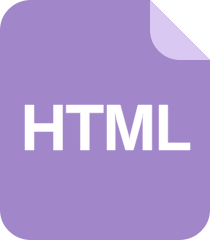
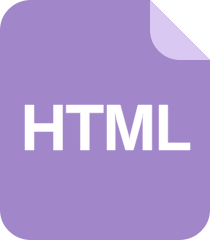
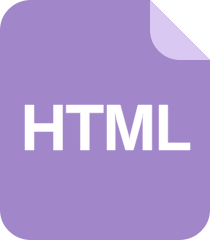
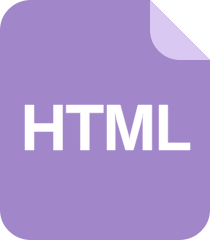
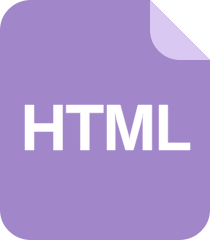
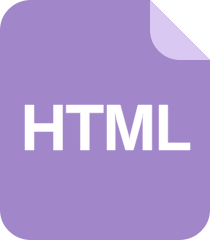
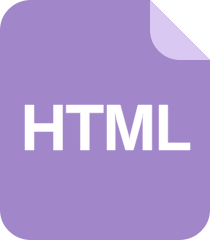
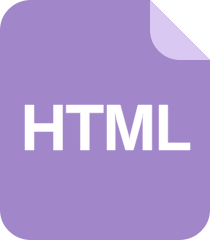
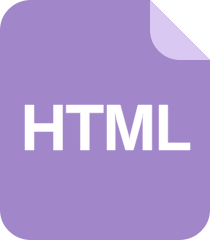
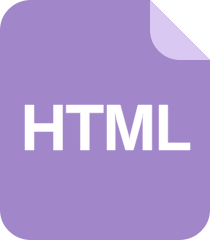
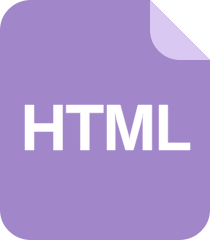
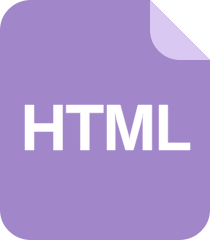
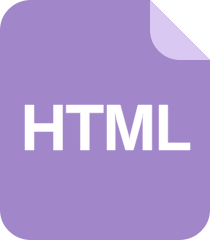
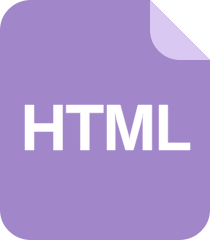
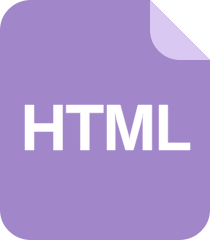
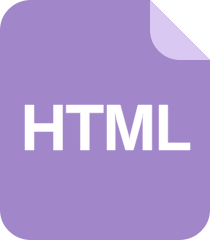
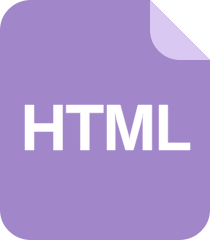
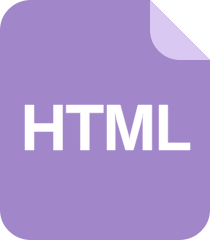
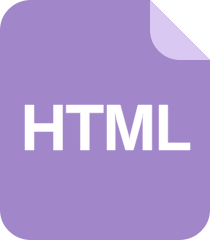
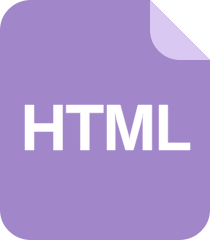
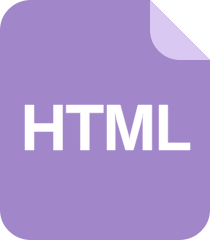
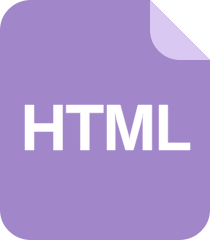
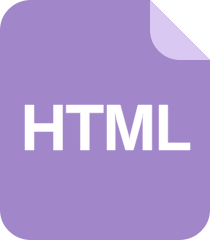
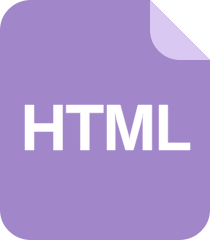
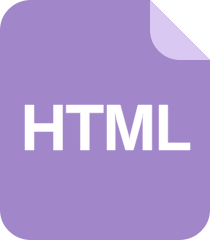
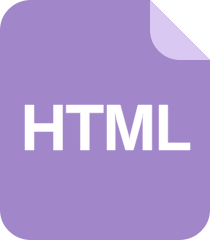
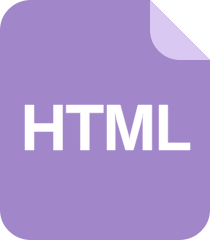
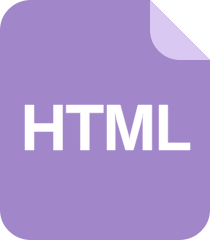
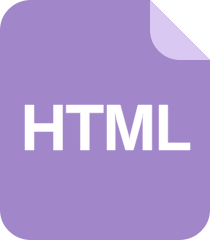
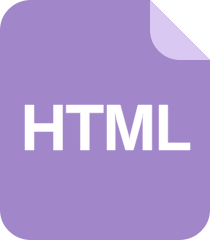
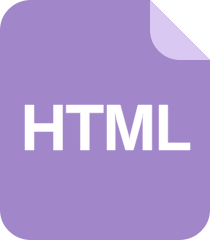
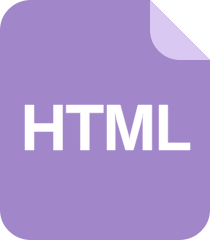
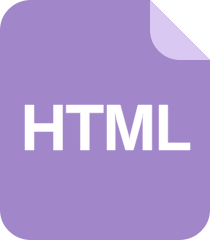
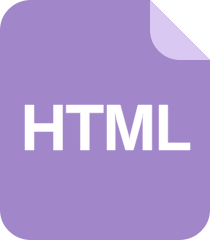
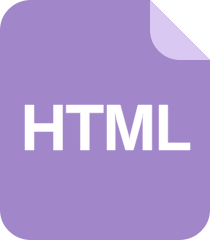
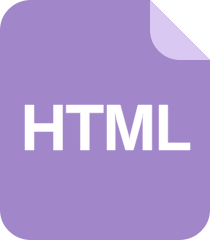
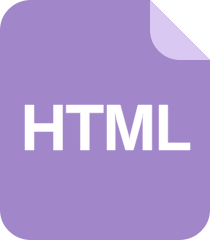
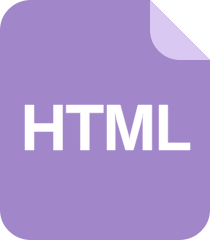
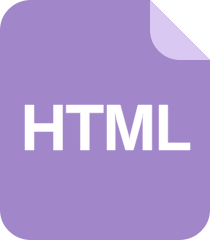
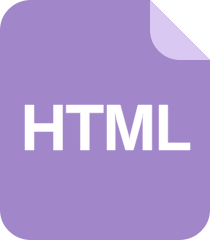
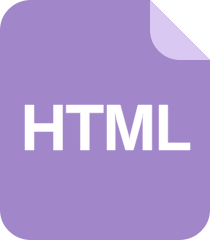
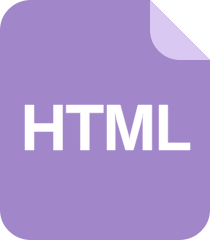
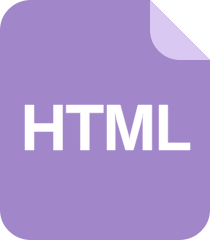
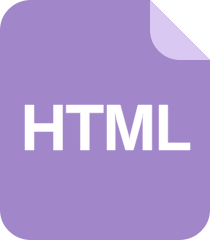
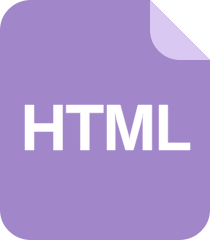
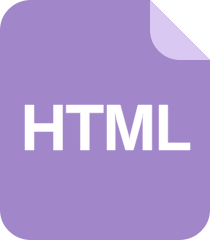
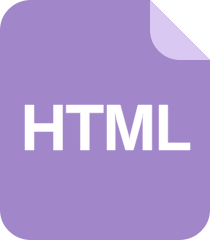
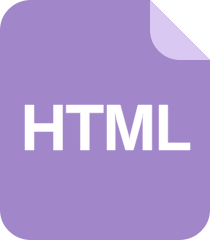
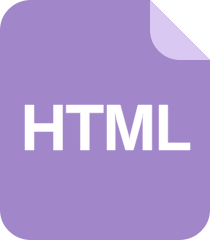
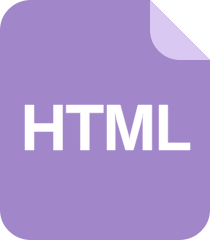
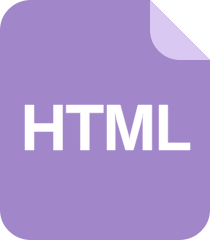
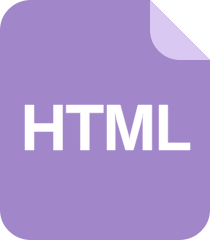
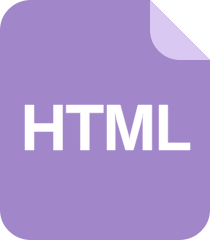
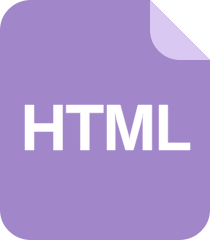
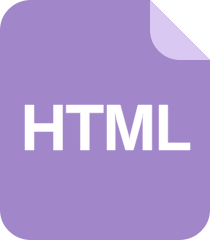
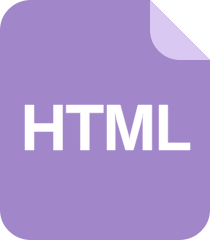
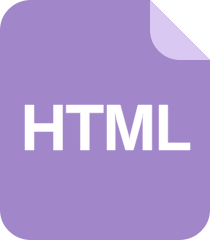
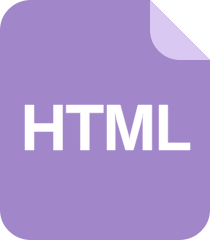
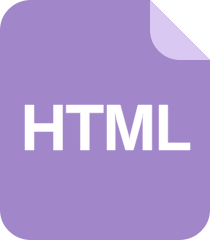
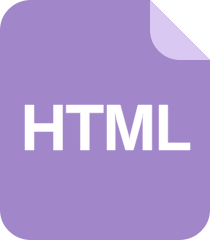
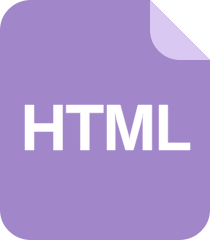
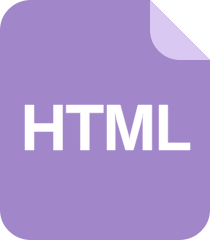
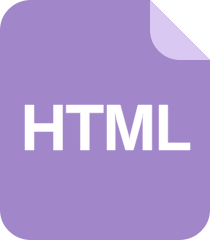
共 626 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7

longfeihufengyun
- 粉丝: 40
- 资源: 17
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

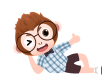
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页