#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdarg.h>
#include <time.h>
#include <curses.h>
#define ENTER 10
#define key_F 70
#define key_f 102
#define key_BKSP 32
/* 日志级别 */
#define LOG_ERR 9, __FILE__, __LINE__
#define LOG_NML 6, __FILE__, __LINE__
#define LOG_DBG 3, __FILE__, __LINE__
int ARR_SIZE_X = 0;
int ARR_SIZE_Y = 0;
int MINE_NUM = 0;
int **glb_arr;
int **flg_arr;
WINDOW *w_frame = NULL;
WINDOW **w_grid;
int pos = 0;
int flag_num = 0;
int best_time[3];
int win_times[3];
int total_times[3];
/* 函数声明 */
int Log(int fmt, ...);
char *GetTime_HHMMSS( char * buff );
int main()
{
int key = 0;
char str[3] = {0};
int number = 0;
int exitflag = 0;
int isfirst = 0;
time_t start_time, end_time;
unsigned int elapsed_time;
char elapsed_time_str[7] = {0};
int flag = 0;
char scurrtime[14+1] = {0};
FILE *fp = NULL;
Log(LOG_DBG, "------------游戏开始-------------");
isfirst = 1;
init_game();
gen_grid();
disp_grid();
while(1)
{
sprintf(str, "剩余个数:%2d", MINE_NUM-flag_num);
mvwaddstr(w_frame, 11, 2, str);
wrefresh(w_frame);
wattron(w_grid[pos], COLOR_PAIR(1));
box(w_grid[pos], 0, 0);
wattroff(w_grid[pos], COLOR_PAIR(1));
wrefresh(w_grid[pos]);
key = wgetch(w_frame);
switch(key)
{
case KEY_RIGHT: press_right(); break;
case KEY_LEFT: press_left(); break;
case KEY_UP: press_up(); break;
case KEY_DOWN: press_down(); break;
case key_BKSP:
if(flag==0)
{
start_time = time(NULL);
GetTime_HHMMSS(scurrtime);
Log(LOG_DBG, "start-time : %s", scurrtime);
flag = 1;
if(ARR_SIZE_X==9 && ARR_SIZE_Y==9 && MINE_NUM==10) total_times[0]++;
else if(ARR_SIZE_X==16 && ARR_SIZE_Y==16 && MINE_NUM==40) total_times[1]++;
else if(ARR_SIZE_X==16 && ARR_SIZE_Y==30 && MINE_NUM==99) total_times[2]++;
}
while(1)
{
if(flg_arr[pos/ARR_SIZE_Y][pos%ARR_SIZE_Y]==2) break;
number = glb_arr[pos/ARR_SIZE_Y][pos%ARR_SIZE_Y];
if(number==9)
{
if(isfirst==1)
{
Log(LOG_DBG, "第一次踩雷,重新生成数组");
free_win();
gen_grid();
disp_grid();
continue;
}
wattron(w_grid[pos], COLOR_PAIR(2));
strcpy(str, "雷");
mvwaddstr(w_grid[pos], 1, 1, str);
wattroff(w_grid[pos], COLOR_PAIR(2));
wrefresh(w_grid[pos]);
flg_arr[pos/ARR_SIZE_Y][pos%ARR_SIZE_Y] = 1;
wattron(w_frame, COLOR_PAIR(1));
mvwaddstr(w_frame, 3, 2, "遗憾,你输了");
wattroff(w_frame, COLOR_PAIR(1));
game_over();
exitflag = 1;
}
else if(number==0)
{
Log(LOG_DBG, "计算要连带打开的格子");
compute_blank(pos/ARR_SIZE_Y, pos%ARR_SIZE_Y);
// Log(LOG_DBG, "------标志数组打印------");
// arr2log(flg_arr);
}
else
{
sprintf(str, "%d ", number);
mvwaddstr(w_grid[pos], 1, 1, str);
wrefresh(w_grid[pos]);
flg_arr[pos/ARR_SIZE_Y][pos%ARR_SIZE_Y] = 1;
}
break;
}
isfirst = 0;
if(exitflag!=1 && 1==iswin())
{
Log(LOG_DBG, "你赢了!");
wattron(w_frame, COLOR_PAIR(2));
mvwaddstr(w_frame, 3, 2, "恭喜,你赢了");
wattroff(w_frame, COLOR_PAIR(2));
mvwaddstr(w_frame, 11, 2, "剩余个数: 0");
wrefresh(w_frame);
game_over();
exitflag = 2;
if(ARR_SIZE_X==9 && ARR_SIZE_Y==9 && MINE_NUM==10) win_times[0]++;
else if(ARR_SIZE_X==16 && ARR_SIZE_Y==16 && MINE_NUM==40) win_times[1]++;
else if(ARR_SIZE_X==16 && ARR_SIZE_Y==30 && MINE_NUM==99) win_times[2]++;
}
break;
case key_F:
case key_f:
if(flg_arr[pos/ARR_SIZE_Y][pos%ARR_SIZE_Y]==0)
{
wattron(w_grid[pos], A_REVERSE);
wattron(w_grid[pos], COLOR_PAIR(1));
mvwaddstr(w_grid[pos], 1, 1, " ");
wattroff(w_grid[pos], COLOR_PAIR(1));
wattroff(w_grid[pos], A_REVERSE);
wrefresh(w_grid[pos]);
flg_arr[pos/ARR_SIZE_Y][pos%ARR_SIZE_Y] = 2;
flag_num++;
}
else if(flg_arr[pos/ARR_SIZE_Y][pos%ARR_SIZE_Y]==2)
{
wattron(w_grid[pos], COLOR_PAIR(3));
mvwaddstr(w_grid[pos], 1, 1, " ");
wattroff(w_grid[pos], COLOR_PAIR(3));
wrefresh(w_grid[pos]);
flg_arr[pos/ARR_SIZE_Y][pos%ARR_SIZE_Y] = 0;
flag_num--;
}
break;
default:
Log(LOG_DBG, "press:%d pos:%d x=%d y=%d arr=%d", key, pos, pos/ARR_SIZE_Y, pos%ARR_SIZE_Y, glb_arr[pos/ARR_SIZE_Y][pos%ARR_SIZE_Y]);
break;
}
if(exitflag==1 || exitflag==2) break;
}
end_time = time(NULL);
GetTime_HHMMSS(scurrtime);
Log(LOG_DBG, "end-time : %s", scurrtime);
elapsed_time = difftime(start_time, end_time);
sprintf(elapsed_time_str, "用时:%04d秒", -elapsed_time);
wattron(w_frame, COLOR_PAIR(exitflag));
mvwaddstr(w_frame, 4, 2, elapsed_time_str);
wattroff(w_frame, COLOR_PAIR(exitflag));
if(exitflag==2)
{
if(ARR_SIZE_X==9 && ARR_SIZE_Y==9 && MINE_NUM==10 && -elapsed_time<best_time[0]) best_time[0] = -elapsed_time;
else if(ARR_SIZE_X==16 && ARR_SIZE_Y==16 && MINE_NUM==40 && -elapsed_time<best_time[1]) best_time[1] = -elapsed_time;
else if(ARR_SIZE_X==16 && ARR_SIZE_Y==30 && MINE_NUM==99 && -elapsed_time<best_time[2]) best_time[2] = -elapsed_time;
}
mvwaddstr(w_frame, 9, 2, "回车键:退出");
if((ARR_SIZE_X==9 && ARR_SIZE_Y==9 && MINE_NUM==10) \

lockyjay
- 粉丝: 0
- 资源: 11
最新资源
- (源码)基于C语言和汇编语言的简单操作系统内核.zip
- (源码)基于Spring Boot框架的AntOA后台管理系统.zip
- (源码)基于Arduino的红外遥控和灯光控制系统.zip
- (源码)基于STM32的简易音乐键盘系统.zip
- (源码)基于Spring Boot和Vue的管理系统.zip
- (源码)基于Spring Boot框架的报表管理系统.zip
- (源码)基于树莓派和TensorFlow Lite的智能厨具环境监测系统.zip
- (源码)基于OpenCV和Arduino的面部追踪系统.zip
- (源码)基于C++和ZeroMQ的分布式系统中间件.zip
- (源码)基于SSM框架的学生信息管理系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


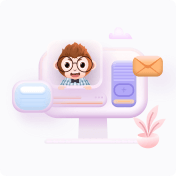