function _extends() {
_extends = Object.assign || function (target) {
for (let i = 1; i < arguments.length; i++) {
const source = arguments[i]
for (const key in source) {
if (Object.prototype.hasOwnProperty.call(source, key)) {
target[key] = source[key]
}
}
}
return target
}
return _extends.apply(this, arguments)
}
/* eslint no-console:0 */
const formatRegExp = /%[sdj%]/g
let warning = function warning() {} // don't print warning message when in production env or node runtime
if (typeof process !== 'undefined' && process.env && process.env.NODE_ENV !== 'production' && typeof window
!== 'undefined' && typeof document !== 'undefined') {
warning = function warning(type, errors) {
if (typeof console !== 'undefined' && console.warn) {
if (errors.every((e) => typeof e === 'string')) {
console.warn(type, errors)
}
}
}
}
function convertFieldsError(errors) {
if (!errors || !errors.length) return null
const fields = {}
errors.forEach((error) => {
const { field } = error
fields[field] = fields[field] || []
fields[field].push(error)
})
return fields
}
function format() {
for (var _len = arguments.length, args = new Array(_len), _key = 0; _key < _len; _key++) {
args[_key] = arguments[_key]
}
let i = 1
const f = args[0]
const len = args.length
if (typeof f === 'function') {
return f.apply(null, args.slice(1))
}
if (typeof f === 'string') {
let str = String(f).replace(formatRegExp, (x) => {
if (x === '%%') {
return '%'
}
if (i >= len) {
return x
}
switch (x) {
case '%s':
return String(args[i++])
case '%d':
return Number(args[i++])
case '%j':
try {
return JSON.stringify(args[i++])
} catch (_) {
return '[Circular]'
}
break
default:
return x
}
})
for (let arg = args[i]; i < len; arg = args[++i]) {
str += ` ${arg}`
}
return str
}
return f
}
function isNativeStringType(type) {
return type === 'string' || type === 'url' || type === 'hex' || type === 'email' || type === 'pattern'
}
function isEmptyValue(value, type) {
if (value === undefined || value === null) {
return true
}
if (type === 'array' && Array.isArray(value) && !value.length) {
return true
}
if (isNativeStringType(type) && typeof value === 'string' && !value) {
return true
}
return false
}
function asyncParallelArray(arr, func, callback) {
const results = []
let total = 0
const arrLength = arr.length
function count(errors) {
results.push.apply(results, errors)
total++
if (total === arrLength) {
callback(results)
}
}
arr.forEach((a) => {
func(a, count)
})
}
function asyncSerialArray(arr, func, callback) {
let index = 0
const arrLength = arr.length
function next(errors) {
if (errors && errors.length) {
callback(errors)
return
}
const original = index
index += 1
if (original < arrLength) {
func(arr[original], next)
} else {
callback([])
}
}
next([])
}
function flattenObjArr(objArr) {
const ret = []
Object.keys(objArr).forEach((k) => {
ret.push.apply(ret, objArr[k])
})
return ret
}
function asyncMap(objArr, option, func, callback) {
if (option.first) {
const _pending = new Promise((resolve, reject) => {
const next = function next(errors) {
callback(errors)
return errors.length ? reject({
errors,
fields: convertFieldsError(errors)
}) : resolve()
}
const flattenArr = flattenObjArr(objArr)
asyncSerialArray(flattenArr, func, next)
})
_pending.catch((e) => e)
return _pending
}
let firstFields = option.firstFields || []
if (firstFields === true) {
firstFields = Object.keys(objArr)
}
const objArrKeys = Object.keys(objArr)
const objArrLength = objArrKeys.length
let total = 0
const results = []
const pending = new Promise((resolve, reject) => {
const next = function next(errors) {
results.push.apply(results, errors)
total++
if (total === objArrLength) {
callback(results)
return results.length ? reject({
errors: results,
fields: convertFieldsError(results)
}) : resolve()
}
}
if (!objArrKeys.length) {
callback(results)
resolve()
}
objArrKeys.forEach((key) => {
const arr = objArr[key]
if (firstFields.indexOf(key) !== -1) {
asyncSerialArray(arr, func, next)
} else {
asyncParallelArray(arr, func, next)
}
})
})
pending.catch((e) => e)
return pending
}
function complementError(rule) {
return function (oe) {
if (oe && oe.message) {
oe.field = oe.field || rule.fullField
return oe
}
return {
message: typeof oe === 'function' ? oe() : oe,
field: oe.field || rule.fullField
}
}
}
function deepMerge(target, source) {
if (source) {
for (const s in source) {
if (source.hasOwnProperty(s)) {
const value = source[s]
if (typeof value === 'object' && typeof target[s] === 'object') {
target[s] = { ...target[s], ...value }
} else {
target[s] = value
}
}
}
}
return target
}
/**
* Rule for validating required fields.
*
* @param rule The validation rule.
* @param value The value of the field on the source object.
* @param source The source object being validated.
* @param errors An array of errors that this rule may add
* validation errors to.
* @param options The validation options.
* @param options.messages The validation messages.
*/
function required(rule, value, source, errors, options, type) {
if (rule.required && (!source.hasOwnProperty(rule.field) || isEmptyValue(value, type || rule.type))) {
errors.push(format(options.messages.required, rule.fullField))
}
}
/**
* Rule for validating whitespace.
*
* @param rule The validation rule.
* @param value The value of the field on the source object.
* @param source The source object being validated.
* @param errors An array of errors that this rule may add
* validation errors to.
* @param options The validation options.
* @param options.messages The validation messages.
*/
function whitespace(rule, value, source, errors, options) {
if (/^\s+$/.test(value) || value === '') {
errors.push(format(options.messages.whitespace, rule.fullField))
}
}
/* eslint max-len:0 */
const pattern = {
// http://emailregex.com/
email: /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\
没有合适的资源?快使用搜索试试~ 我知道了~
基于uniapp和Vue的团购商城小程序设计源码
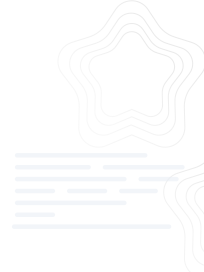
共421个文件
js:253个
vue:113个
scss:23个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 89 浏览量
2025-01-08
00:17:00
上传
评论
收藏 1.49MB ZIP 举报
温馨提示
本项目是一款基于uniapp和Vue框架开发的团购商城小程序源码,总计包含419个文件,其中JavaScript文件252个,Vue文件113个,SCSS文件20个,PNG图片16个,JSON文件5个,Markdown文件4个,WXS文件4个,Git忽略文件1个,Prettier配置文件1个,HTML文件1个。该系统专注于提供团购商城的便捷购物体验,适用于各类移动设备使用。
资源推荐
资源详情
资源评论
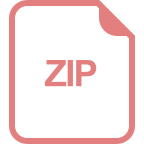
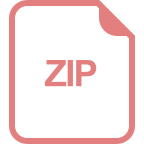
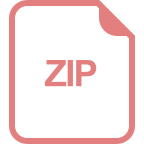
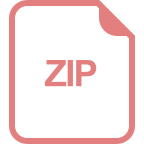
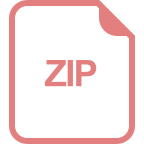
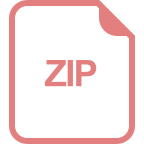
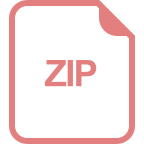
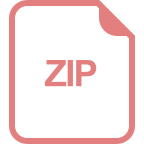
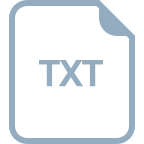
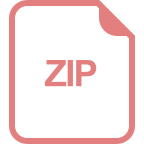
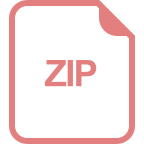
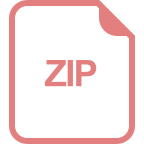
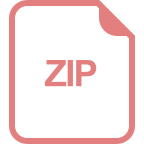
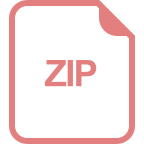
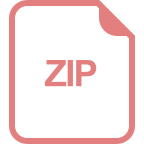
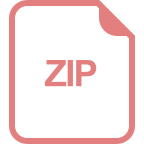
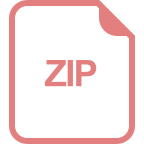
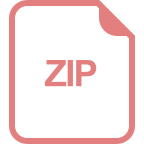
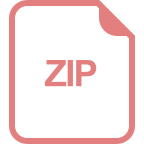
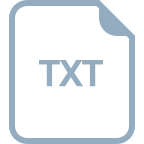
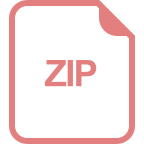
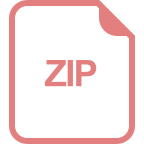
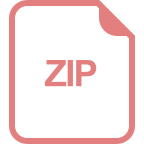
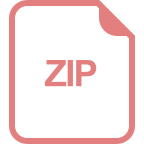
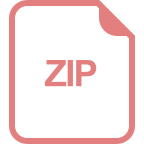
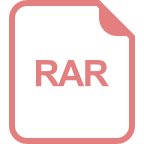
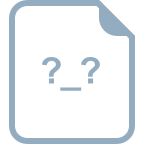
收起资源包目录

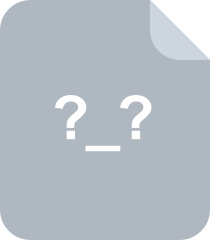
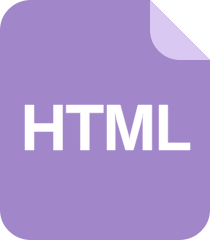
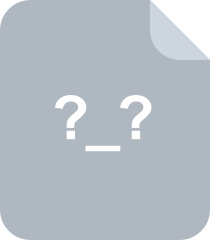
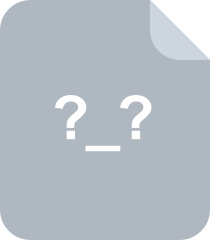
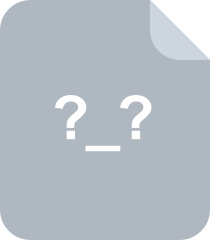
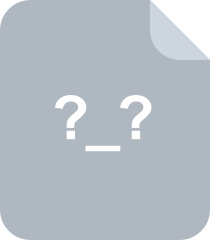
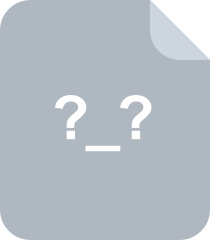
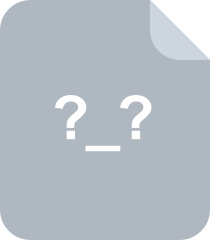
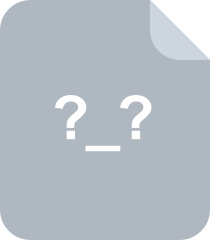
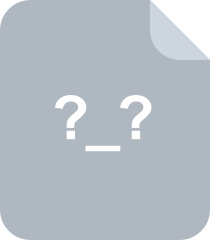
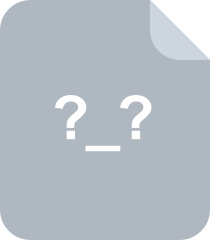
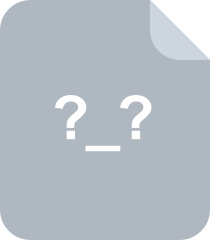
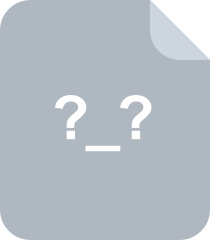
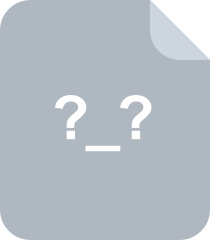
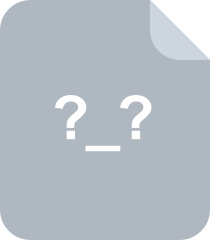
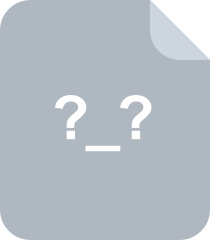
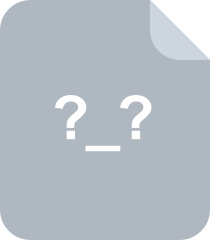
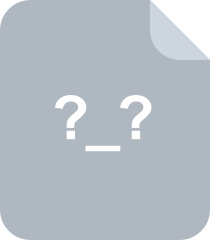
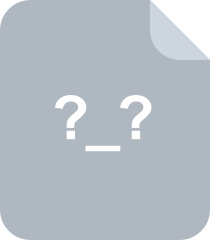
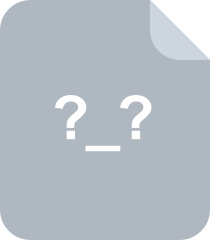
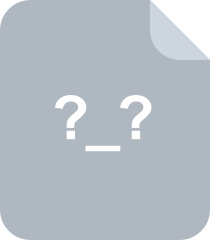
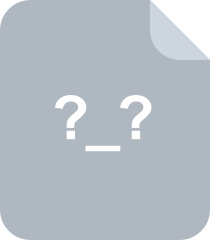
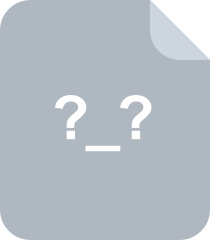
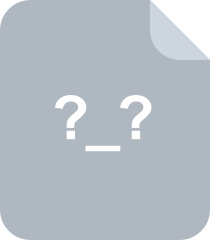
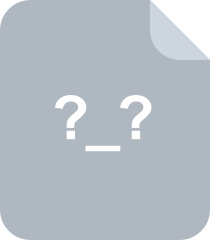
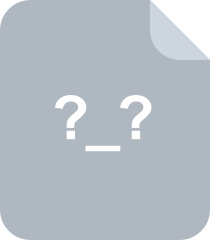
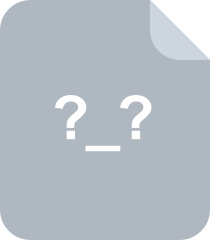
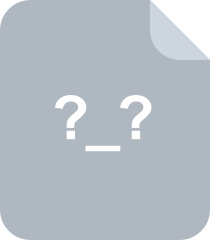
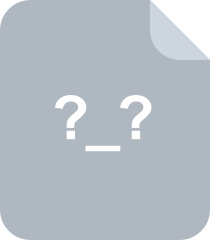
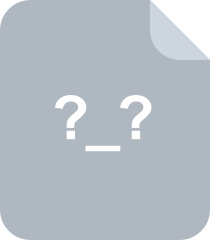
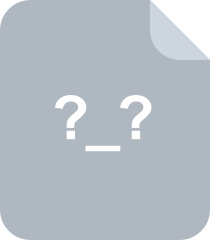
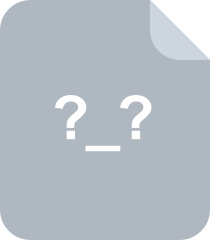
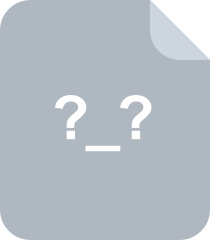
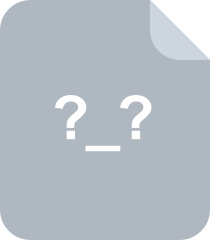
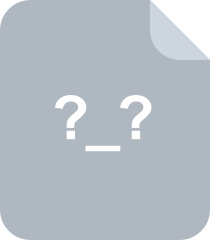
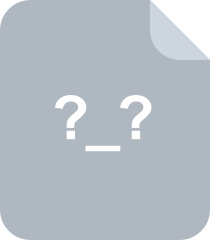
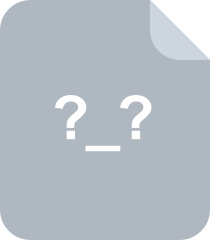
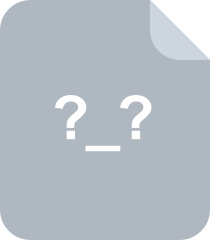
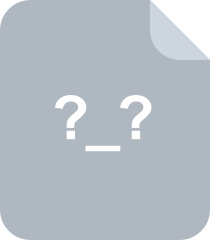
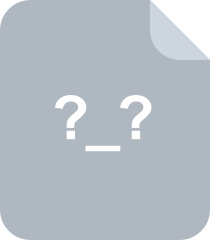
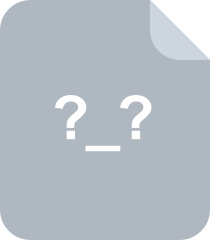
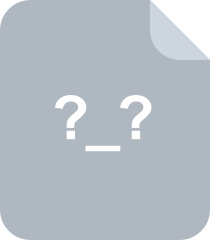
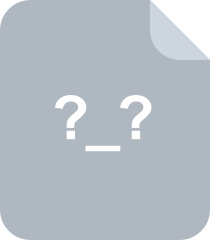
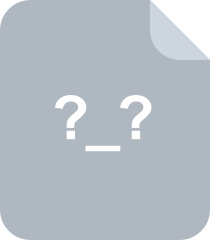
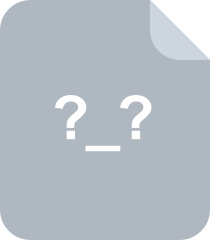
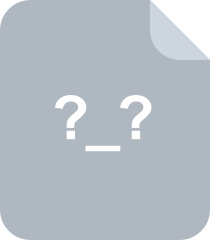
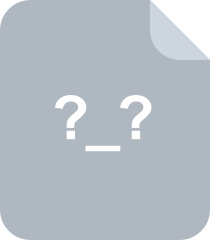
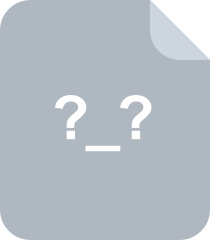
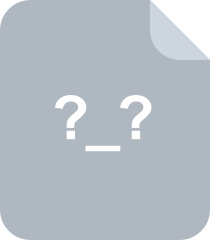
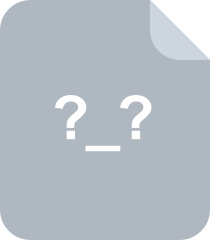
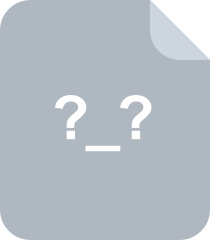
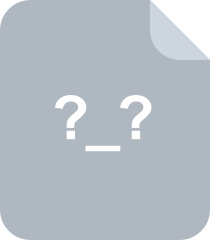
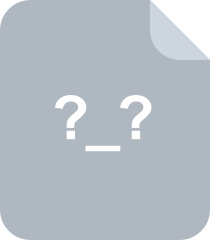
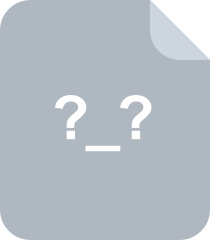
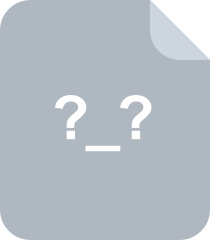
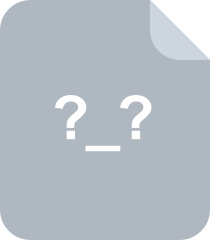
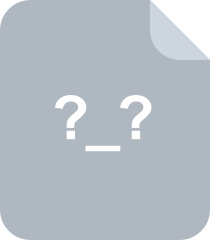
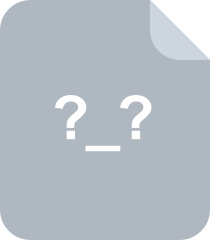
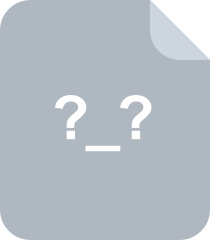
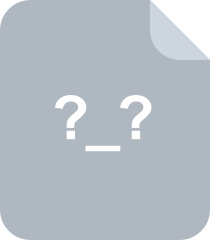
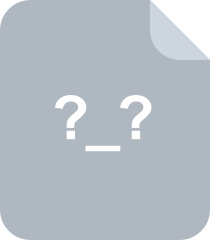
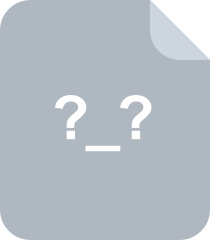
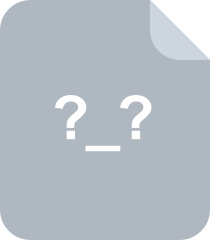
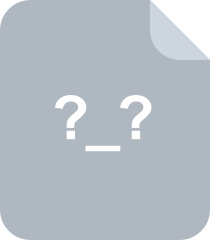
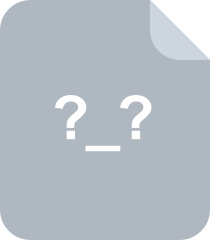
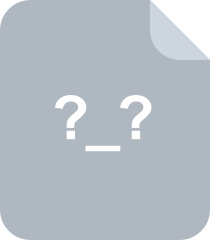
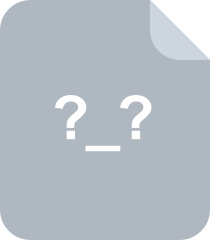
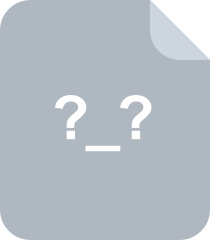
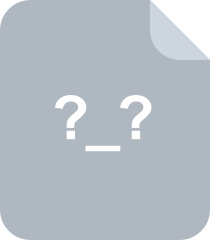
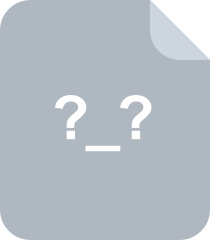
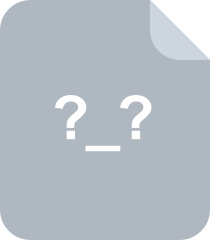
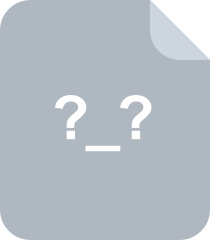
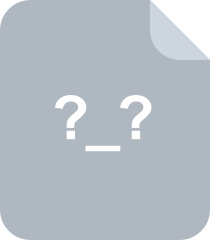
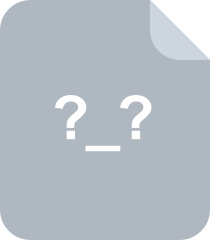
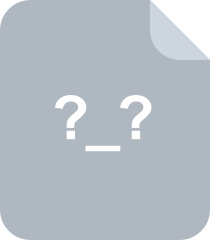
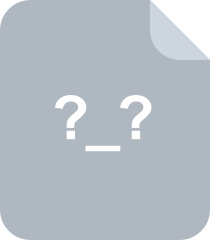
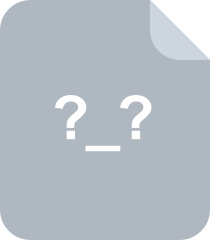
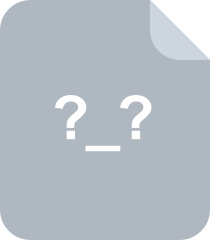
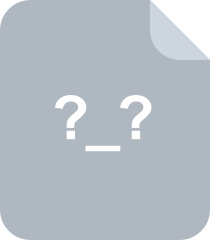
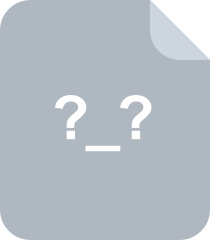
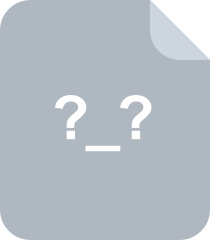
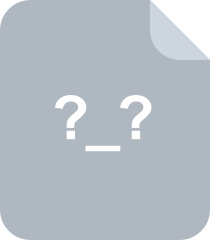
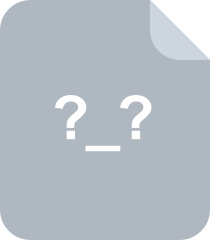
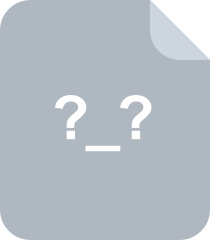
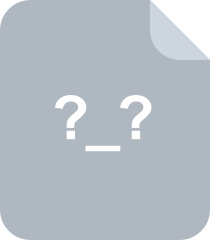
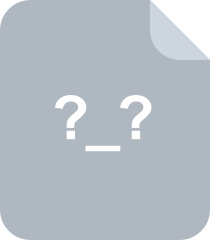
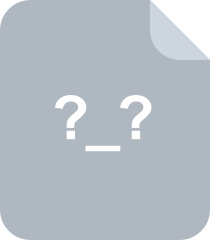
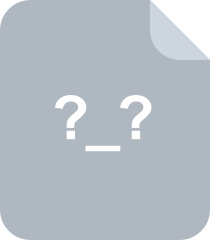
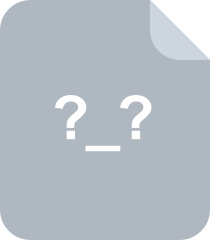
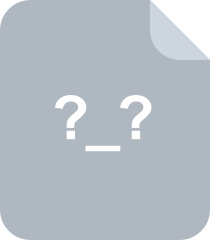
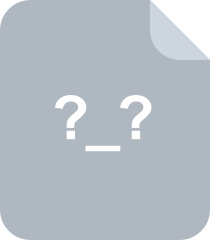
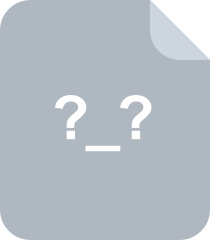
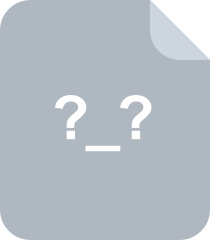
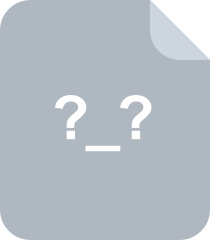
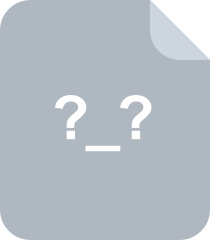
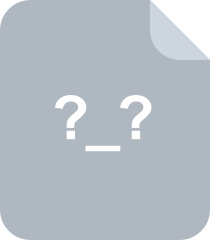
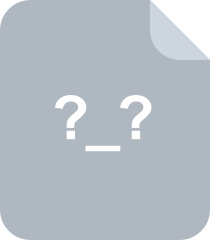
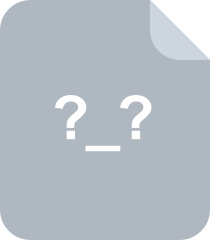
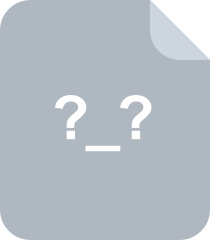
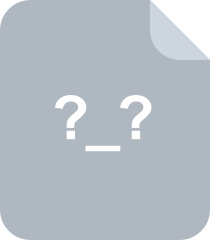
共 421 条
- 1
- 2
- 3
- 4
- 5
资源评论
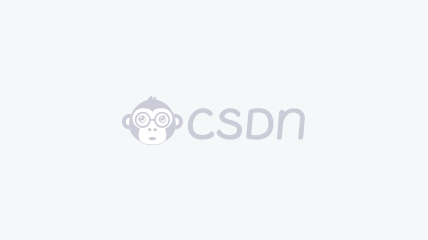

lly202406
- 粉丝: 3172
- 资源: 5551
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

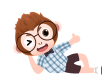
最新资源
- 英雄联盟(League of Legends, LOL)数据集,包含:英雄联盟比赛数据,英雄联盟选手数据(KDA,伤害数,承伤数,补刀等),英雄联盟对战情况数据
- Python爬虫开发与实战-从入门到精通
- Spring项目集成FastDFS文件服务器代码
- 江科大STM32学习笔记(上)-最终版本
- 2024 Java offer 收割指南.pdf
- 12万字 java 面经总结.pdf
- SpringMVC面试题.pdf
- JAVA核心面试知识整理.pdf
- SpringCloud面试题.pdf
- SpringBoot面试题.pdf
- Spring面试专题.pdf
- 并发编程基础知识.pdf
- 代码随想录知识星球精华(最强八股文)第五版(Go篇).pdf
- 代码随想录知识星球精华(最强八股文)第五版(概述).pdf
- 代码随想录知识星球精华(最强八股文)第五版(面经篇).pdf
- 代码随想录知识星球精华(最强八股文)第五版(星球资源篇).pdf
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


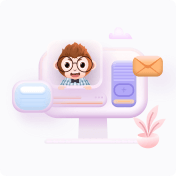
安全验证
文档复制为VIP权益,开通VIP直接复制
