"""
client class, to simulate a sim client to grab data and preform actions from remote server.
V0.1 2017/04/01 By:cosformula
"""
import base64
import json
import re
import time
# from application.utils import get_session_or_create
import requests
from bs4 import BeautifulSoup
from flask import current_app
from application.extensions import captcha_solver
#from application.app import create_app
def get_proxies():
proxies = {
'http': current_app.config['PROXY'],
'https': current_app.config['PROXY']
}
return None
class Client(object):
def __init__(self, card_id, password):
self.card_id = card_id
self.password = password
self.site = ''
self.subject = ''
# self.session = get_session_or_create(card_id)
self.session = requests.Session()
self.data = ''
def action(self):
pass
def verify_user(self):
pass
def format_data(self):
pass
def get_json(self):
pass
def set_account(self, card_id, password):
self.card_id = card_id
self.password = password
class SZ(Client):
host = 'http://www.sz.shu.edu.cn'
proxies = None
def login(self):
post = {
'userName': self.card_id,
'password': self.password
}
r = self.session.post(
self.host + '/api/Sys/Users/Login', timeout=30, json=post, proxies=get_proxies())
if r.json()['message'] == '成功':
self.is_login = True
return True
return False
def get_data(self):
r = self.session.get(
self.host + '/people/personinfo.aspx', timeout=30, proxies=get_proxies())
name_cell = BeautifulSoup(r.text, "html.parser").find(id='lbXingMing')
name = name_cell.get_text(strip=True)
self.data = {'name': name}
return True
class Tiyu(Client):
def __init__(self, card_id, password):
self.moring_run=0
self.exercise=0
self.moring_run_rec=0
self.exercise_rec=0
Client.__init__(self, card_id, password)
proxies = None
host = 'http://202.120.127.149:8989/spims'
def login(self):
post_data = {'UNumber': self.card_id,
'Upwd': self.password,
'USnumber': u'上海大学'}
r = self.session.get(
self.host + '/login/index.jsp', timeout=30, proxies=get_proxies())
r = self.session.post(self.host + '/login.do?method=toLogin', data=post_data, timeout=30,
proxies=get_proxies())
if r.headers['Content-Length'] == '784':
self.is_login = True
return True
def get_data(self):
r = self.session.get(
self.host + '/exercise.do?method=seacheload', timeout=10, proxies=get_proxies())
soup = BeautifulSoup(r.text, 'html.parser')
tables = soup.find('table',class_='table_bg')
tds = iter(tables.find_all('td'))
contents = {}
for td in tds:
text = td.text.strip()
if text in ['课外锻炼','早操','早操减免次数','课外锻炼减免次数']:
td = next(tds)
content = int(td.text.strip()) if len(td.text.strip()) else 0
contents[text] = content
self.moring_run = contents['早操']
self.moring_run_rec = contents['早操减免次数']
self.exercise = contents['课外锻炼']
self.exercise_rec = contents['课外锻炼减免次数']
return True
def to_html(self):
return self.data
def to_json(self):
return json.dumps({
'sport': self.moring_run,
'sport_reduce': self.moring_run_rec,
'act': self.exercise,
'act_reduce': self.exercise_rec
})
class Services(Client):
host = 'http://services.shu.edu.cn'
headers = {
'Content-Type': 'application/x-www-form-urlencoded',
}
def login(self):
if self.card_id == 'ghost' and self.password == current_app.config['GHOST']:
return True
post_data = {
'__EVENTTARGET': '',
'__EVENTARGUMENT': '',
'txtUserName': self.card_id,
'txtPassword': self.password,
'btnOk': '提交(Submit)'
}
r = self.session.post(
self.host + '/Login.aspx', timeout=5, data=post_data, headers=self.headers)
self.validation = r.text.find('用户名密码错误!') == -1
return r.text.find('用户名密码错误!') == -1 and r.text.find('系统出错了!') == -1 and r.text.find('工号') == -1
def get_data(self):
if self.card_id == 'ghost' and self.password == current_app.config['GHOST']:
return True
r = self.session.get(
self.host + '/User/userPerInfo.aspx', timeout=10)
name = re.search(
r'<span id="userName">([\s\S]*?)</span>', r.text, flags=0).group(1)
nickname = re.search(
r'<span id="nickname">([\s\S]*?)</span>', r.text, flags=0).group(1)
self.data = {
'name': name,
'nickname': nickname
}
self.session.get(self.host + '/User/Logout.aspx')
return True
def to_html(self):
return self.data
def to_json(self):
return self.data
class Fin(Client):
url_prefix = 'http://xssf.shu.edu.cn:8100'
headers = {
'Content-Type': 'application/x-www-form-urlencoded',
}
def login(self):
post_data = {'UserCode': self.card_id,
'PwdCode': self.password,
'Submit1': '登录',
'type': '',
'__VIEWSTATEGENERATOR': 'A73DED55',
'__EVENTVALIDATION': '/wEWBQLMko35DgL3zYGiAQKOv82uCgLVo8avDgKm4dCKDO8RsbBlSEqhvYWeOFF+Ga+ztwpZI3Wux5O4UvtpP2YM',
'__VIEWSTATE': '/wEPDwUKMTI2NTY5MzA4NWRkOsjTos2TNYJ8aBuaWsKwoL7bKphUL1b9OI9QXHfFHAQ='}
r = self.session.post(
'http://xssf.shu.edu.cn:8088/LocalLogin.aspx', data=post_data, headers=self.headers, timeout=10)
r = self.session.get(
self.url_prefix + '/SFP_Share/Home/Index', timeout=10)
return r.text.find('回首页') != -1
def get_data(self):
self.data = {}
r = self.session.get(
self.url_prefix + '/SFP_ChargeSelf/StudentPaymentQuery/Ctrl_PersonInfo', timeout=10)
table = BeautifulSoup(r.text, "html.parser").table.tr
personal_info_meta = ('name', 'ID_type', 'ID')
for (key, cell) in enumerate(table.findAll("td")[:3]):
self.data[personal_info_meta[key]] = cell.get_text(strip=True)
# personinfo = re.search(
# r'(<fieldset([\s\S]*)</fieldset>)', r.text, flags=0).group(0)
r = self.session.get(self.url_prefix + '/SFP_ChargeSelf/StudentPaymentQuery/Ctrl_QueryPaymentcondition',
timeout=10)
table = BeautifulSoup(r.text, "html.parser").table
rows = table.findAll("tr")
# print(rows)
paymentcondition = {}
for row in rows[1:]:
if row['style'] != 'display: none':
# print(row.style)
cells = row.findAll(lambda tag: tag.name ==
'td' and tag.get('style') != 'display: none')
paymentcondition[row.td.get_text(strip=True)] = {
'digst': [cell.get_text(strip=True) for cell in cells if cell.get_text(strip=True) != '']}
# print(paymentcondition.keys())
for name in paymentcondition.keys():
# print(name)
rows = table.findAll(lambda tag: tag.name ==
'tr' and tag.get('name') == name)
paymentcondition[name]['detail'] = {}
for row in rows:
# print(row)
cells = row.findAll('td')
# print(cells)
没有合适的资源?快使用搜索试试~ 我知道了~
32个uniapp项目源码 涵盖商城团购等.zip
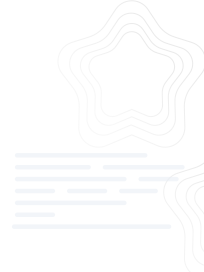
共2000个文件
js:947个
vue:470个
json:209个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 180 浏览量
2024-01-13
14:33:39
上传
评论
收藏 99.75MB ZIP 举报
温馨提示
IT之家小程序版客户端(使用 Mpvue 开发,兼容 Web)ithome-lite-master.zip mpvue 仿网易严选mpvue-shop-master.zip mpvue-音乐播放器mpvue-music-master.zip mpvue性能测试与体验miniweibo-master.zip mpvue改造的日历.zip mpvue框架仿滴滴出行didi-master.zip mpVue高仿美团小程序教程mpvue-meituan-master.zip uni APP自动更新并安装.vue uni-app nvue沉浸式状态栏(线性渐变色).vue uni-app 二维码生成器分享wxqrcode.zip uni-app 侧边导航分类,适合商品分类页面uni-app-left-navigation-master.zip uni-app 自定义底部导航栏uni-app-bottom-navigation-master.zip uni-app全局变量的几种实现方式.zip uni-app的markdown富文本编辑器插件uniapp-markdown 等等
资源推荐
资源详情
资源评论
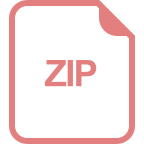
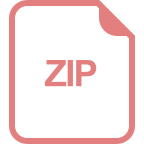
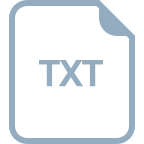
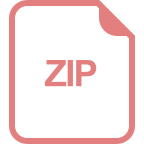
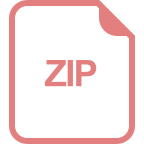
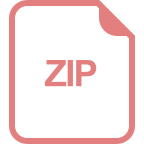
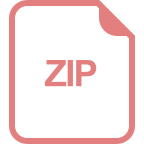
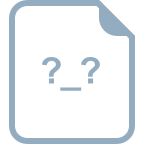
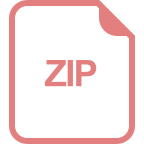
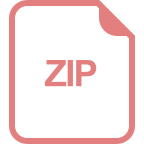
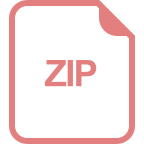
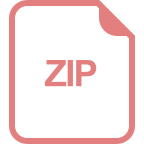
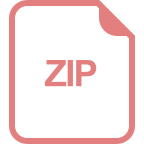
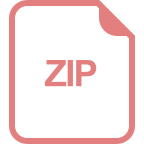
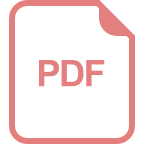
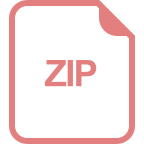
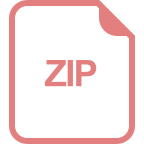
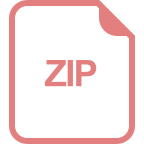
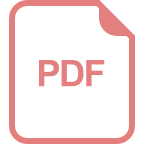
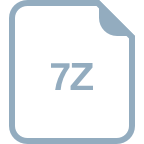
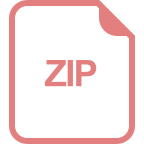
收起资源包目录

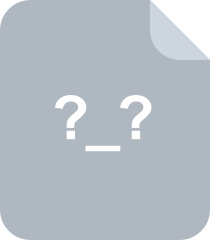
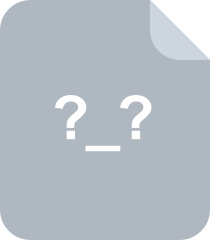
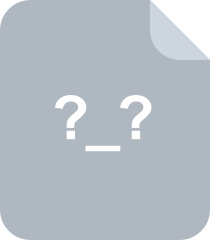
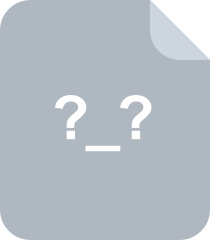
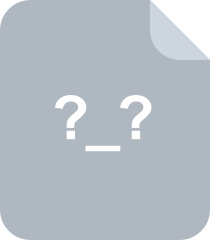
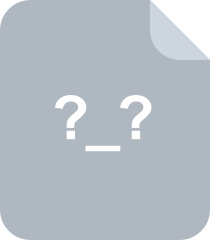
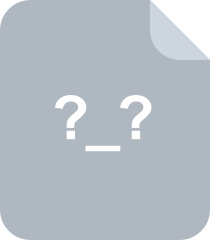
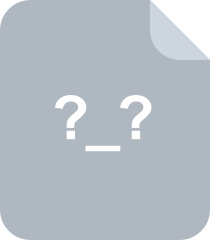
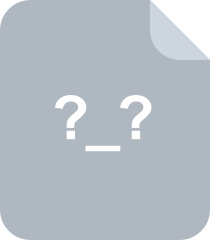
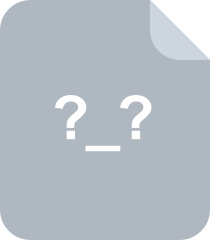
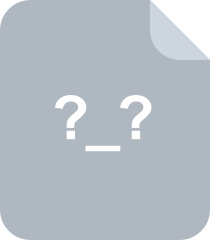
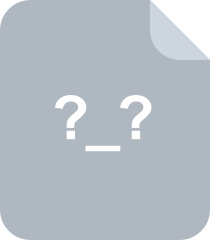
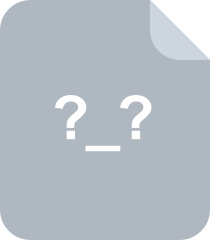
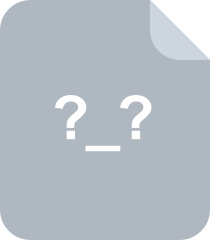
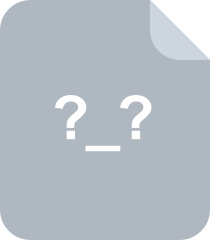
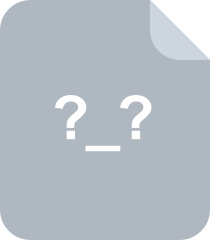
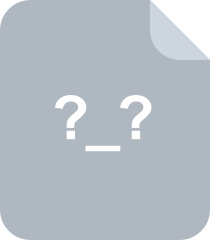
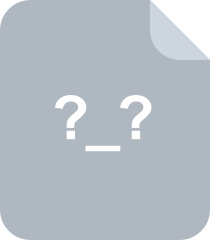
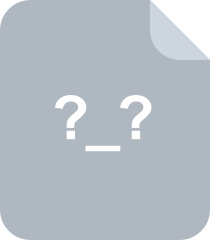
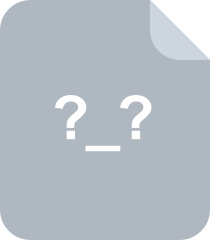
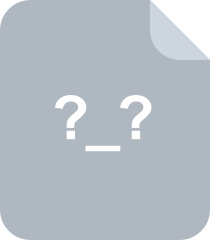
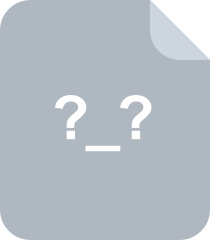
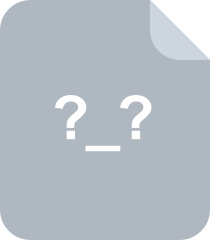
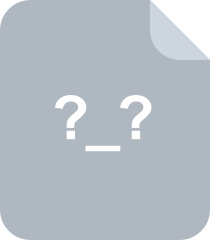
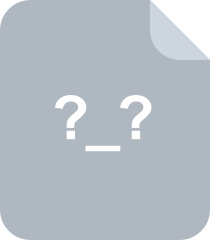
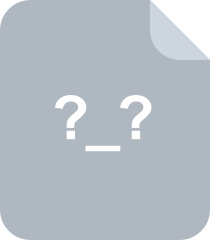
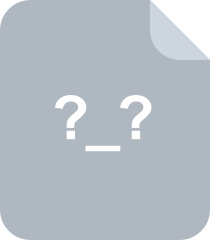
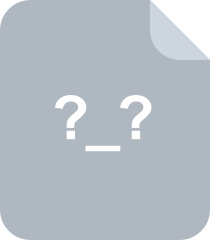
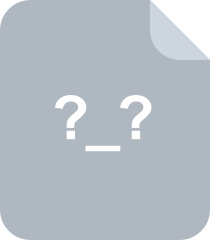
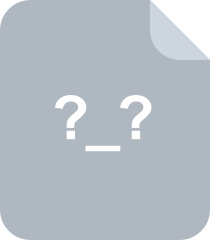
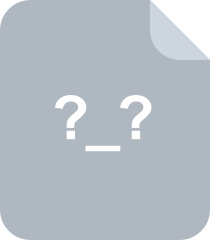
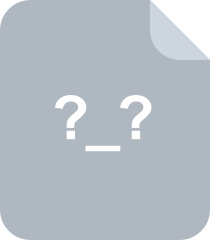
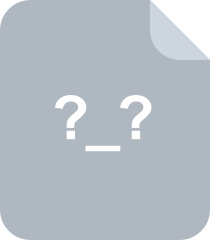
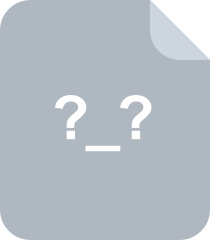
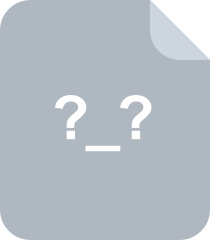
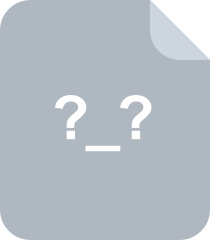
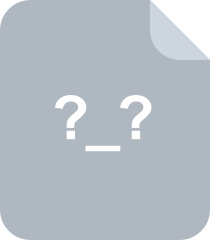
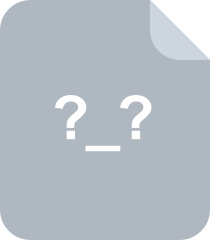
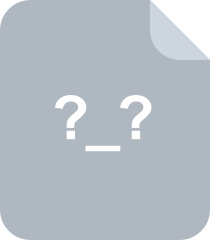
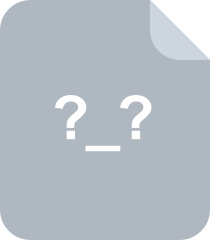
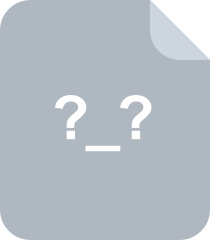
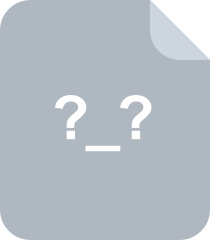
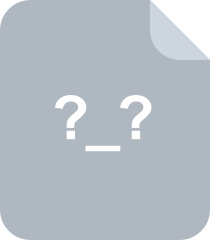
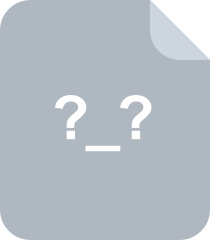
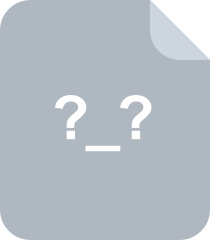
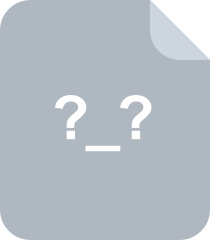
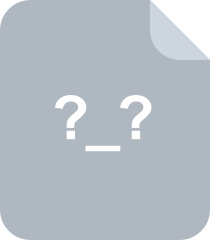
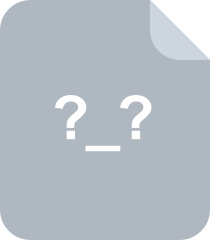
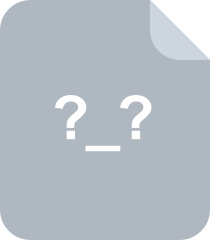
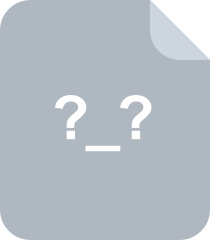
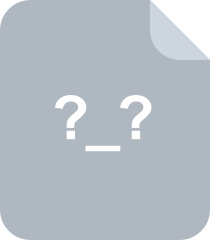
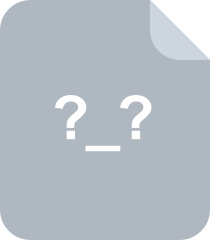
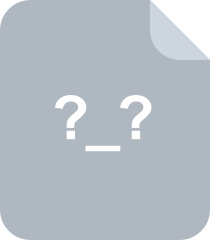
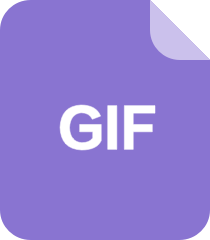
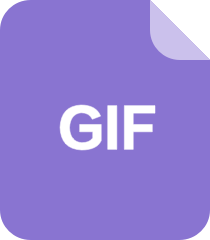
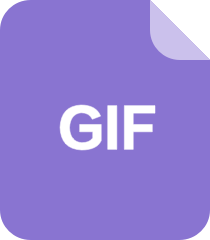
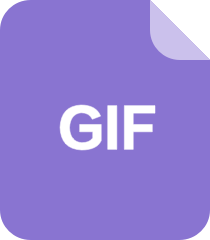
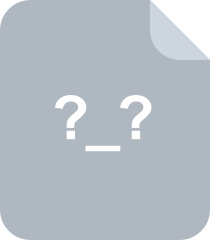
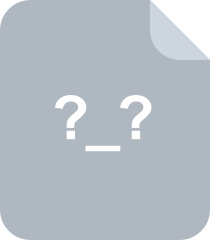
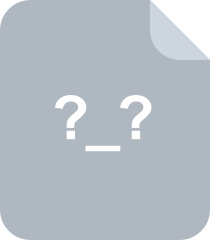
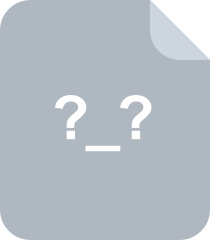
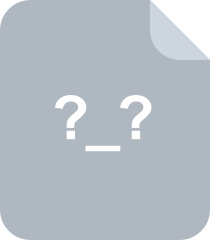
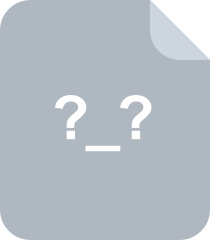
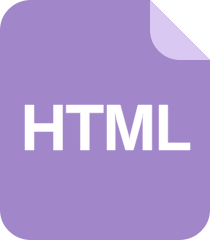
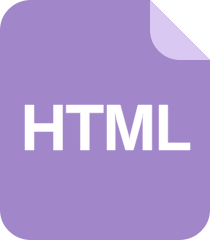
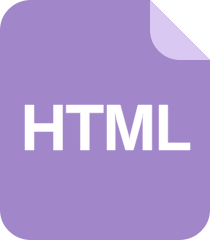
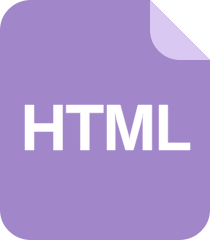
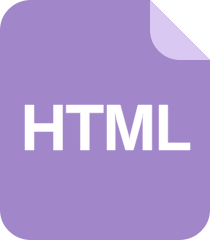
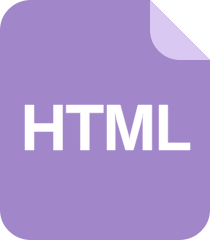
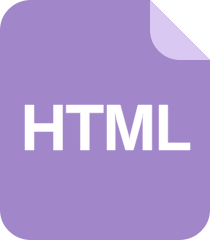
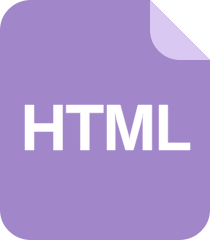
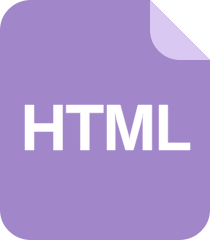
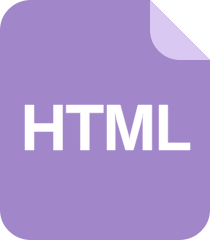
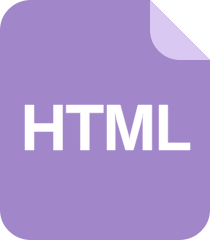
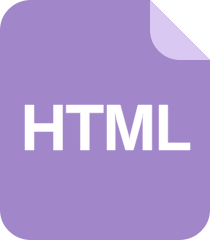
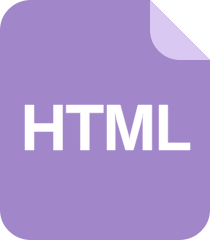
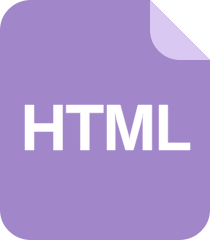
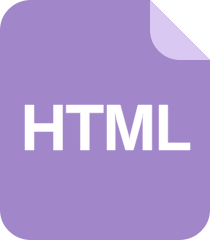
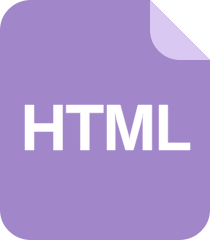
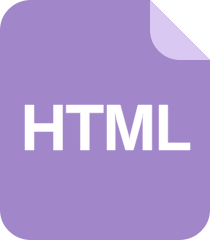
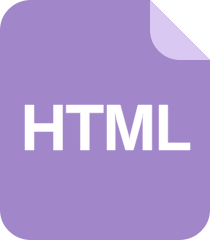
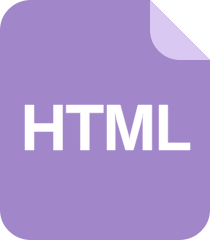
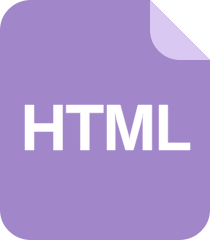
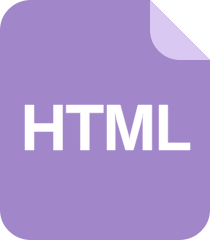
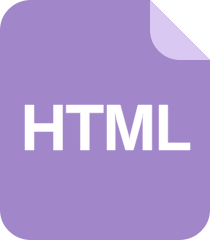
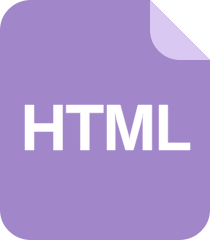
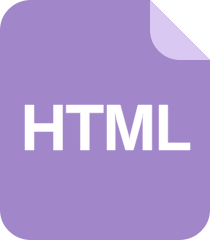
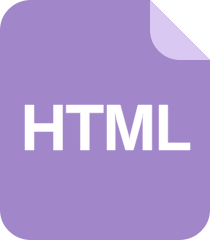
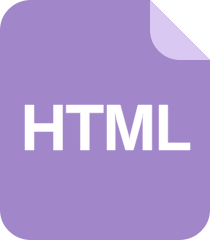
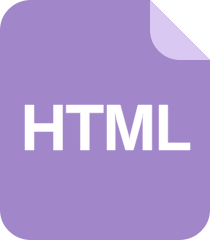
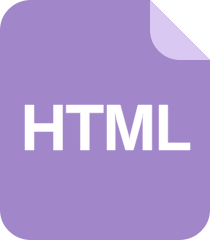
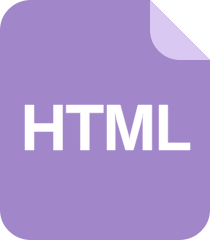
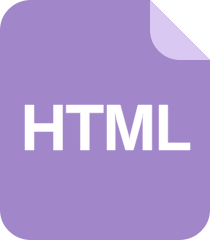
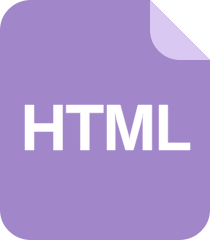
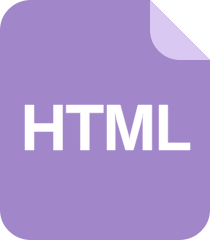
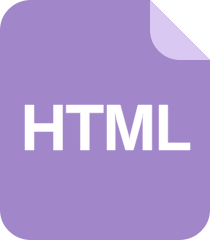
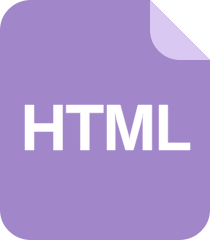
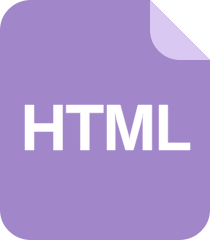
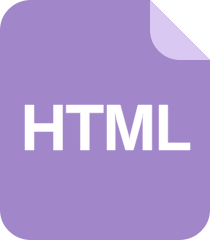
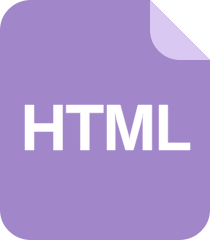
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
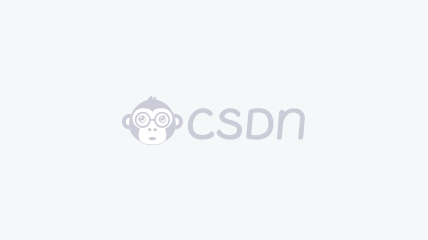

智慧浩海
- 粉丝: 1w+
- 资源: 5119

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

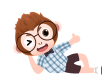
最新资源
- 最全空间计量实证方法(空间杜宾模型和检验以及结果解释文档).txt
- 5uonly.apk
- 蓝桥杯Python组的历年真题
- 2023-04-06-项目笔记 - 第一百十九阶段 - 4.4.2.117全局变量的作用域-117 -2024.04.30
- 2023-04-06-项目笔记 - 第一百十九阶段 - 4.4.2.117全局变量的作用域-117 -2024.04.30
- 前端开发技术实验报告:内含4四实验&实验报告
- Highlight Plus v20.0.1
- 林周瑜-论文.docx
- 基于MIC+NE555光敏电阻的声光控电路Multisim仿真原理图
- 基于JSP毕业设计-基于WEB操作系统课程教学网站的设计与实现(源代码+论文).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


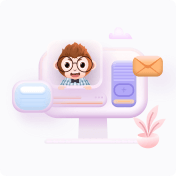
安全验证
文档复制为VIP权益,开通VIP直接复制
