package service
import (
"encoding/json"
"errors"
"ferry/global/orm"
"ferry/models/base"
"ferry/models/process"
"ferry/models/system"
"ferry/pkg/notify"
"ferry/tools"
"fmt"
"reflect"
"time"
"github.com/jinzhu/gorm"
"github.com/gin-gonic/gin"
)
/*
@Author : lanyulei
@Desc : 处理工单
*/
/*
-- 节点 --
start: 开始节点
userTask: 审批节点
receiveTask: 处理节点
scriptTask: 任务节点
end: 结束节点
-- 网关 --
exclusiveGateway: 排他网关
parallelGateway: 并行网关
inclusiveGateway: 包容网关
*/
type Handle struct {
cirHistoryList []process.CirculationHistory
workOrderId int
updateValue map[string]interface{}
stateValue map[string]interface{}
targetStateValue map[string]interface{}
WorkOrderData [][]byte
workOrderDetails process.WorkOrderInfo
endHistory bool
flowProperties int
circulationValue string
processState ProcessState
tx *gorm.DB
}
// 会签
func (h *Handle) Countersign(c *gin.Context) (err error) {
var (
stateList []map[string]interface{}
stateIdMap map[string]interface{}
currentState map[string]interface{}
cirHistoryCount int
userInfoList []system.SysUser
circulationStatus bool
)
err = json.Unmarshal(h.workOrderDetails.State, &stateList)
if err != nil {
return
}
stateIdMap = make(map[string]interface{})
for _, v := range stateList {
stateIdMap[v["id"].(string)] = v["label"]
if v["id"].(string) == h.stateValue["id"].(string) {
currentState = v
}
}
userStatusCount := 0
circulationStatus = false
for _, cirHistoryValue := range h.cirHistoryList {
if len(currentState["processor"].([]interface{})) > 1 {
if _, ok := stateIdMap[cirHistoryValue.Source]; !ok {
break
}
}
if currentState["process_method"].(string) == "person" {
// 用户会签
for _, processor := range currentState["processor"].([]interface{}) {
if cirHistoryValue.ProcessorId != tools.GetUserId(c) &&
cirHistoryValue.Source == currentState["id"].(string) &&
cirHistoryValue.ProcessorId == int(processor.(float64)) {
cirHistoryCount += 1
}
}
if cirHistoryCount == len(currentState["processor"].([]interface{}))-1 {
circulationStatus = true
break
}
} else if currentState["process_method"].(string) == "role" || currentState["process_method"].(string) == "department" {
// 全员处理
var tmpUserList []system.SysUser
if h.stateValue["fullHandle"].(bool) {
db := orm.Eloquent.Model(&system.SysUser{})
if currentState["process_method"].(string) == "role" {
db = db.Where("role_id in (?)", currentState["processor"].([]interface{}))
} else if currentState["process_method"].(string) == "department" {
db = db.Where("dept_id in (?)", currentState["processor"].([]interface{}))
}
err = db.Find(&userInfoList).Error
if err != nil {
return
}
temp := map[string]struct{}{}
for _, user := range userInfoList {
if _, ok := temp[user.Username]; !ok {
temp[user.Username] = struct{}{}
tmpUserList = append(tmpUserList, user)
}
}
for _, user := range tmpUserList {
if cirHistoryValue.Source == currentState["id"].(string) &&
cirHistoryValue.ProcessorId != tools.GetUserId(c) &&
cirHistoryValue.ProcessorId == user.UserId {
userStatusCount += 1
break
}
}
} else {
// 普通会签
for _, processor := range currentState["processor"].([]interface{}) {
db := orm.Eloquent.Model(&system.SysUser{})
if currentState["process_method"].(string) == "role" {
db = db.Where("role_id = ?", processor)
} else if currentState["process_method"].(string) == "department" {
db = db.Where("dept_id = ?", processor)
}
err = db.Find(&userInfoList).Error
if err != nil {
return
}
for _, user := range userInfoList {
if user.UserId != tools.GetUserId(c) &&
cirHistoryValue.Source == currentState["id"].(string) &&
cirHistoryValue.ProcessorId == user.UserId {
userStatusCount += 1
break
}
}
}
}
if h.stateValue["fullHandle"].(bool) {
if userStatusCount == len(tmpUserList)-1 {
circulationStatus = true
}
} else {
if userStatusCount == len(currentState["processor"].([]interface{}))-1 {
circulationStatus = true
}
}
}
}
if circulationStatus {
h.endHistory = true
err = h.circulation()
if err != nil {
return
}
}
return
}
// 工单跳转
func (h *Handle) circulation() (err error) {
var (
stateValue []byte
)
stateList := make([]interface{}, 0)
for _, v := range h.updateValue["state"].([]map[string]interface{}) {
stateList = append(stateList, v)
}
err = GetVariableValue(stateList, h.workOrderDetails.Creator)
if err != nil {
return
}
stateValue, err = json.Marshal(h.updateValue["state"])
if err != nil {
return
}
err = h.tx.Model(&process.WorkOrderInfo{}).
Where("id = ?", h.workOrderId).
Updates(map[string]interface{}{
"state": stateValue,
"is_denied": h.flowProperties,
"related_person": h.updateValue["related_person"],
}).Error
if err != nil {
h.tx.Rollback()
return
}
// 如果是跳转到结束节点,则需要修改节点状态
if h.targetStateValue["clazz"] == "end" {
err = h.tx.Model(&process.WorkOrderInfo{}).
Where("id = ?", h.workOrderId).
Update("is_end", 1).Error
if err != nil {
h.tx.Rollback()
return
}
}
return
}
// 条件判断
func (h *Handle) ConditionalJudgment(condExpr map[string]interface{}) (result bool, err error) {
var (
condExprOk bool
condExprValue interface{}
)
defer func() {
if r := recover(); r != nil {
switch e := r.(type) {
case string:
err = errors.New(e)
case error:
err = e
default:
err = errors.New("未知错误")
}
return
}
}()
for _, data := range h.WorkOrderData {
var formData map[string]interface{}
err = json.Unmarshal(data, &formData)
if err != nil {
return
}
if condExprValue, condExprOk = formData[condExpr["key"].(string)]; condExprOk {
break
}
}
if condExprValue == nil {
err = errors.New("未查询到对应的表单数据。")
return
}
// todo 待优化
switch reflect.TypeOf(condExprValue).String() {
case "string":
switch condExpr["sign"] {
case "==":
if condExprValue.(string) == condExpr["value"].(string) {
result = true
}
case "!=":
if condExprValue.(string) != condExpr["value"].(string) {
result = true
}
case ">":
if condExprValue.(string) > condExpr["value"].(string) {
result = true
}
case ">=":
if condExprValue.(string) >= condExpr["value"].(string) {
result = true
}
case "<":
if condExprValue.(string) < condExpr["value"].(string) {
result = true
}
case "<=":
if condExprValue.(string) <= condExpr["value"].(string) {
result = true
}
default:
err = errors.New("目前仅支持6种常规判断类型,包括(等于、不等于、大于、大于等于、小于、小于等于)")
}
case "float64":
switch condExpr["sign"] {
case "==":
if condExprValue.(float64) == condExpr["value"].(float64) {
result = true
}
case "!=":
if condExprValue.(float64) != condExpr["value"].(float64) {
result = true
}
case ">":
if condExprValue.(float64) > condExpr["value"].(float64) {
result = true
}
case ">=":
if condExprValue.(float64) >= condExpr["value"].(float64) {
result = true
}
case "<":
if condExprValue.(float64) < condExpr["value"].(float64) {
result = true
}
case "<=":
if condExprValue.(float64) <= condExpr["value"].(float64) {
result = true
}
default:
err = errors.New("目前仅支持6种常规判断类型,包括(等于、不等于、大于、大于等于、小于、小于等于)")
}
default:
err = errors.New("条件判断目前仅支持字符串、整型。")
}
没有合适的资源?快使用搜索试试~ 我知道了~
基于JavaScript、Go、CSS、Shell、HTML、Python的集成式开源工单系统设计源码
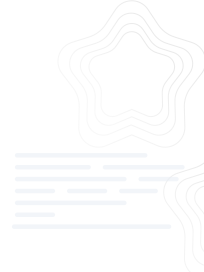
共633个文件
js:438个
go:117个
svg:19个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 66 浏览量
2024-09-26
03:24:44
上传
评论
收藏 26.8MB ZIP 举报
温馨提示
该项目是一个集成式开源工单系统,采用JavaScript作为主要开发语言,并融合了Go、CSS、Shell、HTML、Python等多种编程语言,总计包含633个文件。其中,JavaScript文件438个,Go文件117个,SVG文件19个,CSS文件9个,HTML模板文件7个,字体文件(woff、ttf、eot)共15个,YML和配置文件共8个,Shell脚本文件3个。该系统集工单统计、任务钩子、权限管理、灵活配置流程与模板等功能于一体,旨在提升工作效率、工作质量,减少沟通成本和人为错误,是理想的跨部门协作和工作流引擎解决方案。
资源推荐
资源详情
资源评论
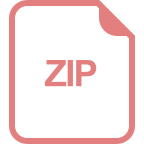
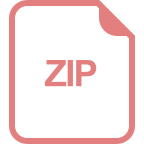
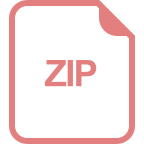
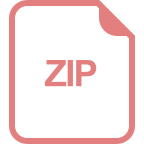
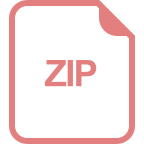
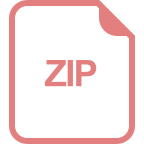
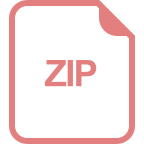
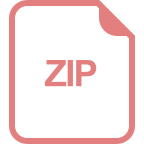
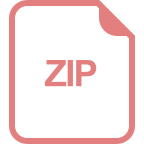
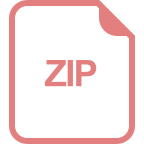
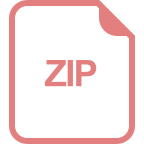
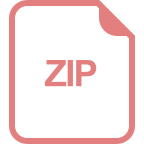
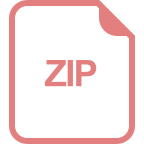
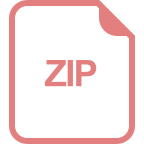
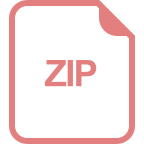
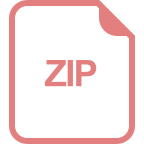
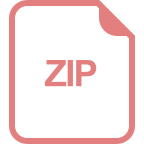
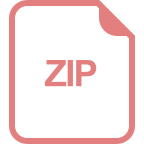
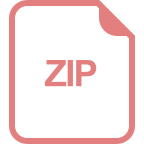
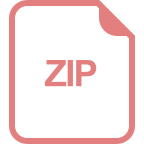
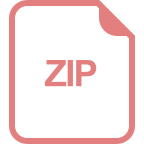
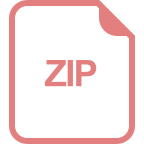
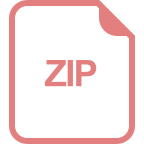
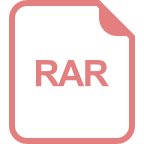
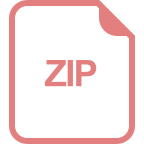
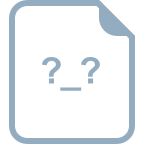
收起资源包目录

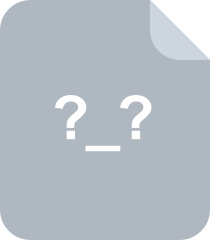
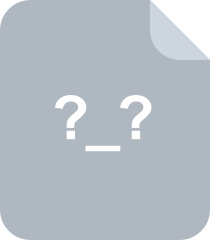
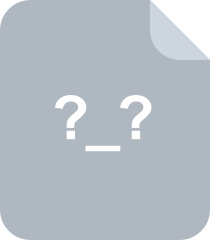
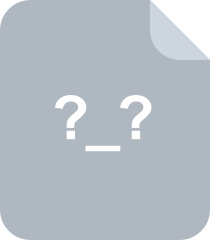
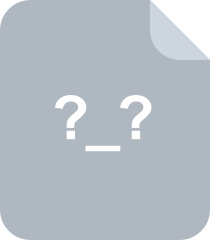
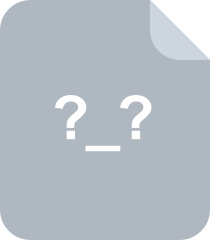
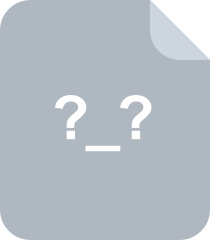
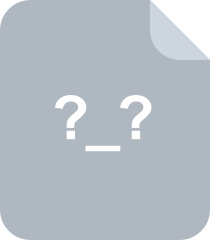
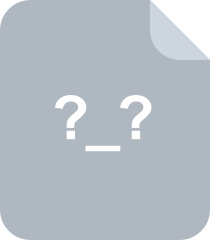
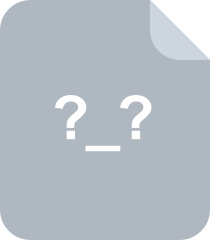
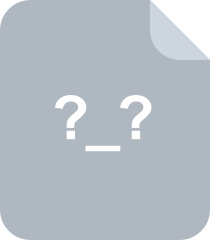
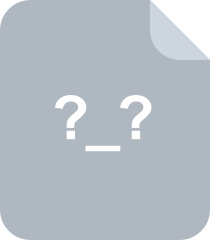
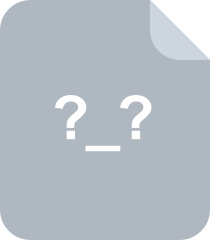
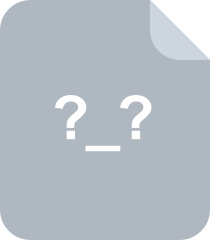
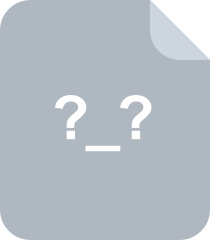
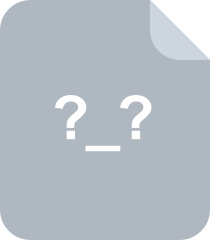
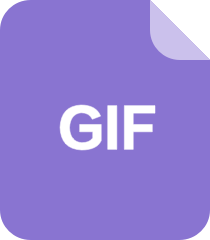
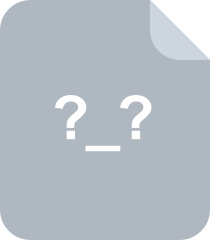
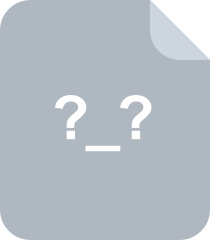
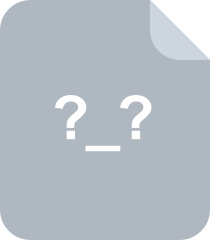
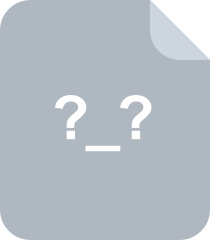
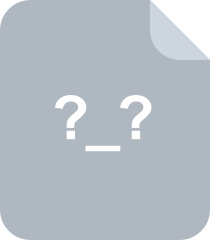
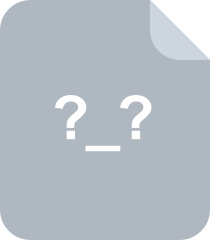
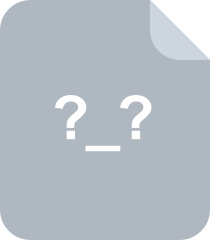
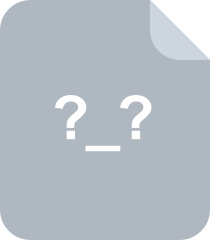
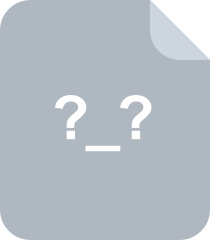
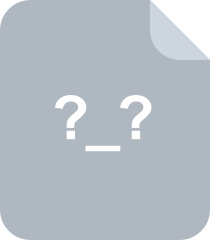
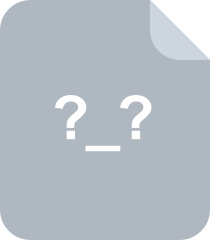
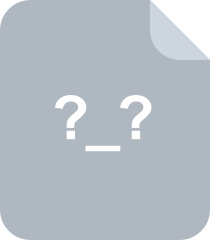
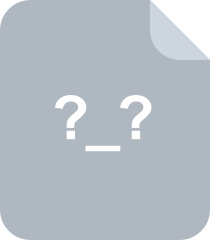
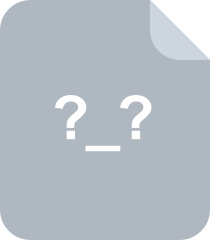
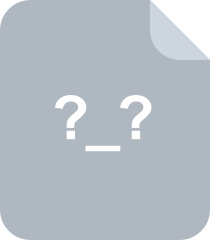
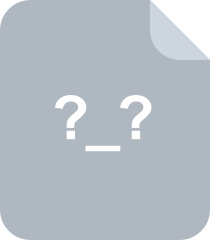
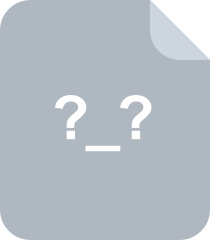
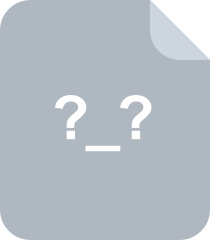
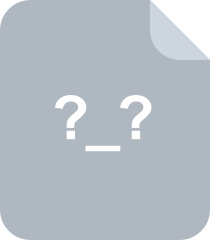
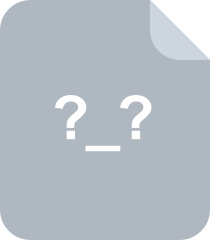
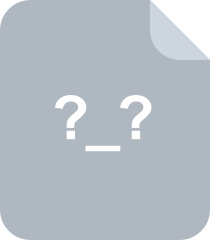
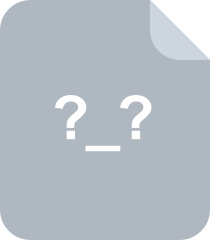
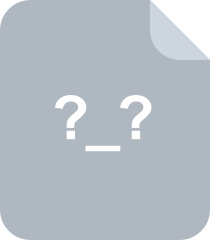
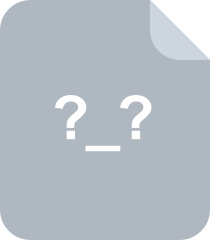
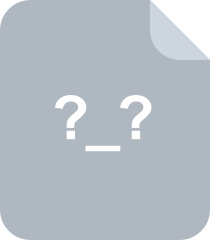
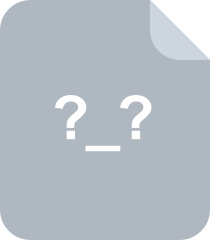
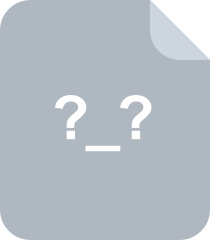
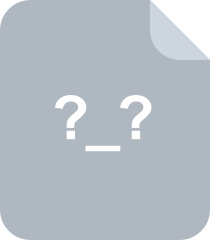
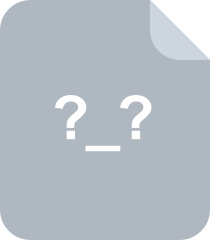
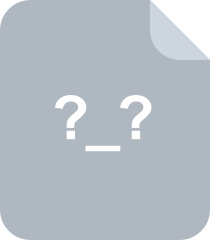
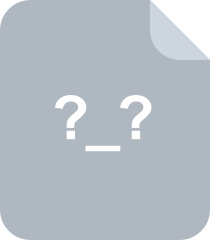
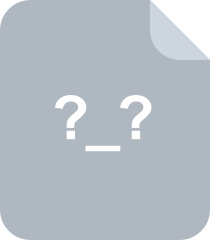
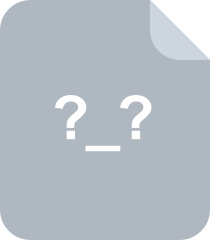
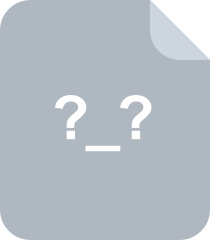
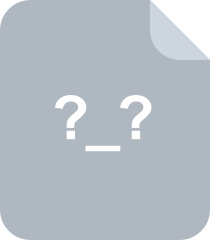
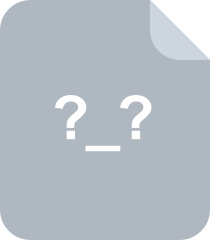
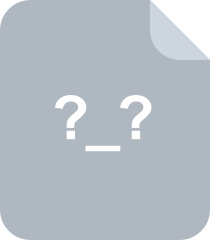
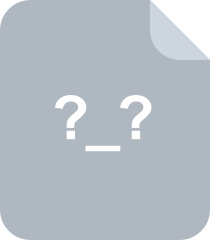
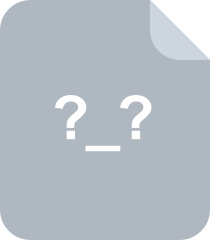
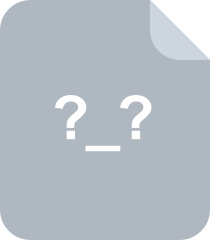
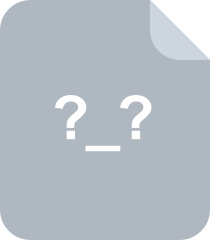
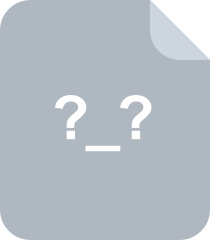
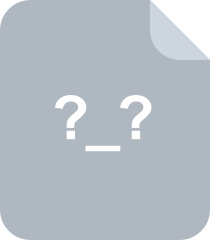
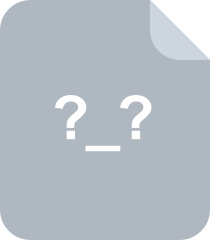
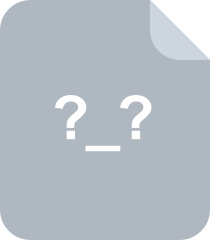
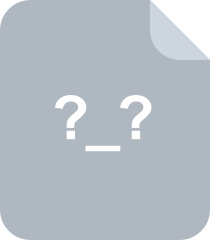
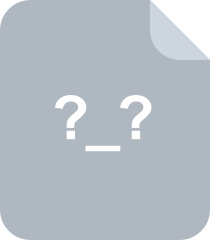
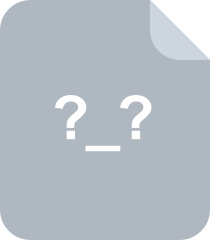
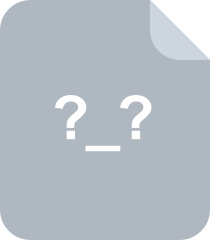
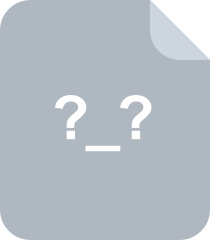
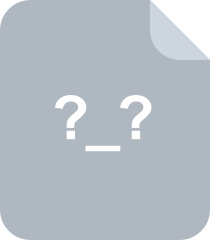
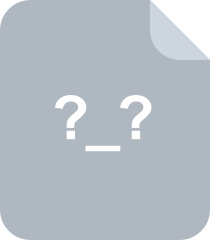
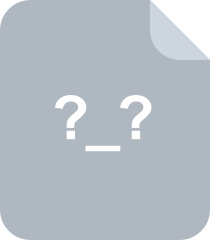
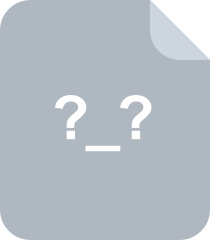
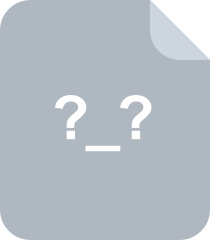
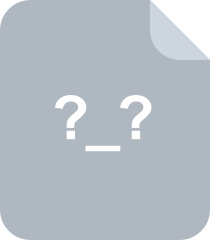
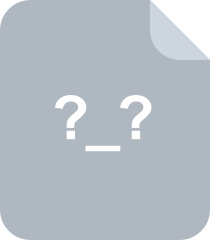
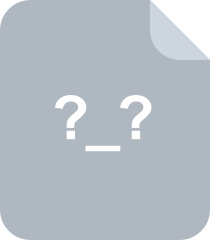
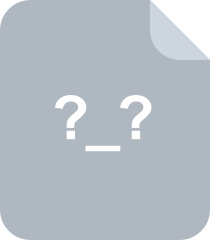
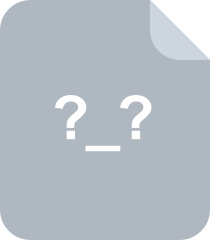
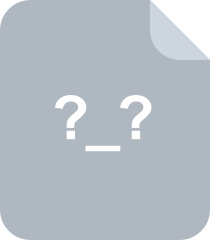
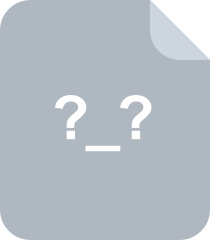
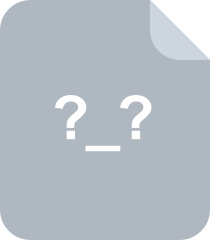
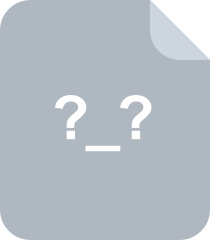
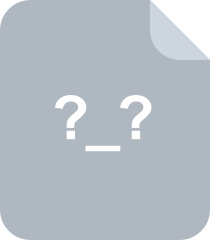
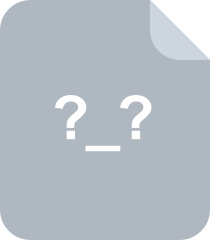
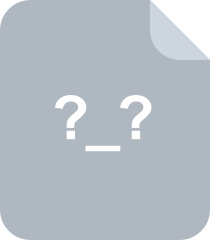
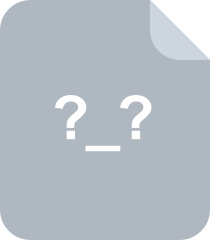
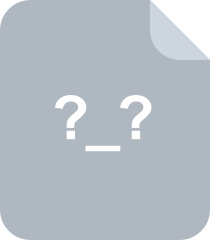
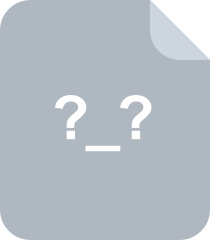
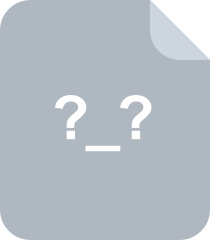
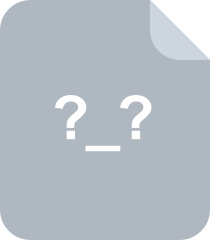
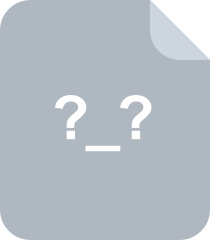
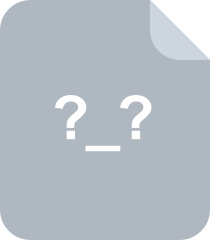
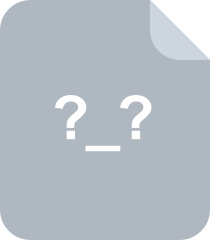
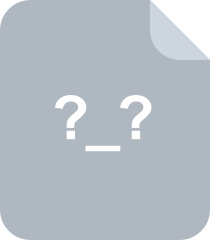
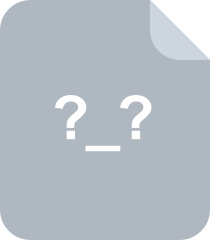
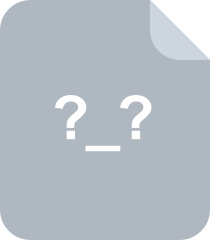
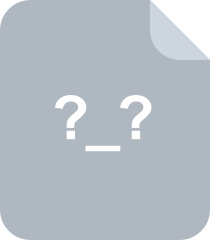
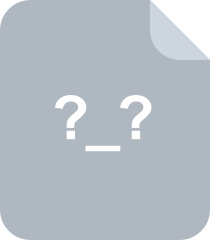
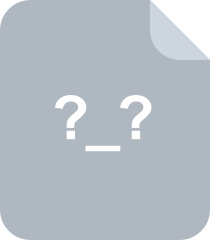
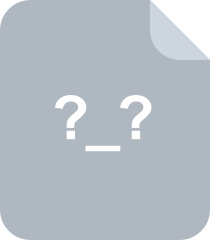
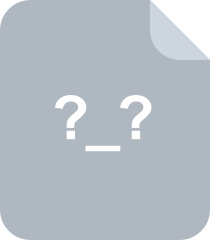
共 633 条
- 1
- 2
- 3
- 4
- 5
- 6
- 7
资源评论
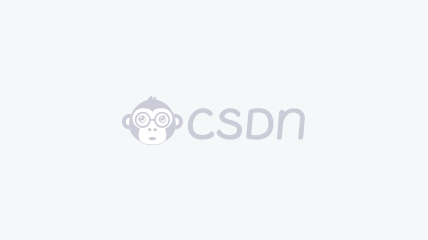

lly202406
- 粉丝: 3013
- 资源: 5522
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

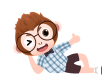
最新资源
- 《济南的冬天》教学设计与反思.docx
- 基于java+springboot+vue+mysql的古典舞在线交流平台 源码+数据库+论文(高分毕业设计).zip
- 形状检测32-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma数据集合集.rar
- 百度智能云千帆大模型平台推进企业多模态生成式AI应用
- 互联网金融发展指数 (第二期,2014年1月-2015年12月).zip
- 社区团购网站:技术驱动下的电子商务新模式
- 2025年人形机器人产业发展蓝皮书-量产及商业化关键挑战
- C# 面试题 100 问:从基础到进阶,全面解析与实战.docx
- 基于java+springboot+vue+mysql的读书笔记共享平台 源码+数据库+论文(高分毕业设计).zip
- Python+Django+Mysql个性化图书推荐系统 图书在线推荐系统 基于用户、项目、内容的协同过滤推荐算法 帮远程安装部署 一、项目简介 1、开发工具和实现技术 Python3.8,Djan
- 基于Java的环境保护与宣传网站的设计与实现毕业论文.doc
- 基于java+springboot+vue+mysql的海滨体育馆管理系统 源码+数据库+论文(高分毕业设计).zip
- 2025年 UiPath AI和自动化趋势:代理型AI的崛起及企业影响
- 基于java+springboot+vue+mysql的网上超市系统 源码+数据库+论文(高分毕业设计).zip
- 电力系统静态稳定性仿真simulink仿真 用simulink搭建搭建单机无穷大系统,对其静态稳定性进行仿真分析
- 柑橘多种疾病类型图像分类数据集【已标注,约1,000张数据】
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


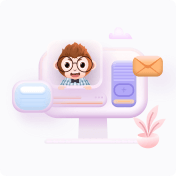
安全验证
文档复制为VIP权益,开通VIP直接复制
