// Specification of the External Interface for a VRML applet browser.
// FreeWRL Viewer Interface - bypass netscape and go directly
// to the viewer.
package vrml.external;
import java.util.*;
import java.applet.*;
//JAS import java.awt.*;
import java.net.*;
import java.io.*;
//JAS import java.lang.reflect.*;
import vrml.external.Node;
//JAS import vrml.external.field.EventOut;
import vrml.external.field.EventOutObserver;
import vrml.external.field.EventInMFNode;
import vrml.external.FreeWRLEAI.EAIoutThread;
import vrml.external.FreeWRLEAI.EAIinThread;
import vrml.external.FreeWRLEAI.EAIAsyncThread;
import vrml.external.exception.InvalidNodeException;
import vrml.external.exception.InvalidVrmlException;
import vrml.external.BrowserGlobals;
import java.lang.System;
//JAS - no longer using Netscape import netscape.security.*;
public class Browser implements BrowserInterface
{
//====================================================================
// Threads:
// Replies from FreeWRL.
static Thread FreeWRLThread; // of type EAIinThread
// Send commands to FreeWRL.
static EAIoutThread EAIoutSender;
// Handle Async communications from FreeWRL (eg, Regisered Listeners)
static EAIAsyncThread RL_Async;
//====================================================================
// Communication Paths:
// FreeWRLThread to Browser - responses from commands to FreeWRL.
PrintWriter EAIinThreadtoBrowser;
PipedWriter EAIinThreadtoBrowserPipe = null;
//
static BufferedReader BrowserfromEAI = null;
PipedReader BrowserfromEAIPipe = null;
// The following are used to send to/from the FreeWRL Browser by:
// - EAIinThread
Socket EAISocket;
Socket sock;
static PrintWriter EAIout;
// The following pipe listens for replies to events sent to
// the FreeWRL VRML viewer via the EAI port.
private String reply = "";
// Query Number as sent to the FreeWRL Browser.
static int queryno = 1;
// Sending to FreeWRL needs to synchronize on an object;
static Object FreeWRLToken = new Object();
// Interface methods.
public int get_Browser_EVtype (int event)
{
//System.out.println ("get_Browser_EVtype is returning " +
// BrowserGlobals.EVtype[event] +
// " for event " + event);
return BrowserGlobals.EVtype[event];
}
public EventOutObserver get_Browser_EVObserver (int eventno)
{
// System.out.println ("get_Browser_EVObserver is returning " +
// BrowserGlobals.EVObserver[eventno]);
return BrowserGlobals.EVObserver[eventno];
}
public void Browser_RL_Async_send (String EVentreply, int eventno)
{
int EVcounter;
for (EVcounter=0; EVcounter<BrowserGlobals.EVno; EVcounter++) {
if (BrowserGlobals.EVarray[EVcounter] == eventno) {
break;
}
}
//System.out.println ("Browser_RL_Async_send sending " + EVentreply + " to number " + EVcounter);
RL_Async.send(EVentreply, EVcounter);
}
// Associates this instance with the first embedded plugin in the current frame.
public Browser(Applet pApplet) {
int counter;
// Create a socket here for an EAI server on localhost
int incrport = -1;
EAISocket = null;
//System.out.println ("trying to open socket on 9877");
//enable privleges
//JAS try {
//JAS PrivilegeManager.enablePrivilege ("UniversalConnect");
//JAS } catch (Throwable e) {
//JAS System.out.println("EAI: not using Netscape");
//JAS }
counter = 1;
while (EAISocket == null) {
try {
EAISocket = new Socket("localhost",9877);
} catch (IOException e) {
// wait up to 30 seconds for FreeWRL to answer.
counter = counter + 1;
if (counter == 60) {
System.out.println ("EAI: Java code timed out finding FreeWRL");
System.exit(1);
}
try {Thread.sleep (500);} catch (InterruptedException f) { }
}
}
sock = EAISocket; //JAS - these are the same now...
//===================================================================
// create the EAIinThread to Browser.
// Open the pipe for EAI replies to be sent to us...
try {
EAIinThreadtoBrowserPipe = new PipedWriter();
BrowserfromEAIPipe = new PipedReader(EAIinThreadtoBrowserPipe);
} catch (IOException ie) {
System.out.println ("EAI: caught error in new PipedReader: " + ie);
}
EAIinThreadtoBrowser = new PrintWriter(EAIinThreadtoBrowserPipe);
BrowserfromEAI = new BufferedReader (BrowserfromEAIPipe);
// Start the readfrom FREEWRL thread...
FreeWRLThread = new Thread ( new EAIinThread(sock, pApplet,
EAIinThreadtoBrowser, this));
FreeWRLThread.start();
//====================================================================
// Start the thread that allows Registered Listenered
// updates to come in.
RL_Async = new EAIAsyncThread();
RL_Async.start();
//====================================================================
// create the EAIoutThread - send data to FreeWRL.
try {
EAIout = new PrintWriter (sock.getOutputStream());
} catch (IOException e) {
System.out.print ("EAI: Problem in handshaking with Browser");
}
// Start the SendTo FREEWRL thread...
EAIoutSender = new EAIoutThread(EAIout);
EAIoutSender.start();
//====================================================================
// Browser is "gotten", and is started.
return;
}
// construct an instance of the Browser class
// If frameName is NULL, current frame is assumed.
public Browser(Applet pApplet, String frameName, int index) {
System.out.println ("Browser2 Not Implemented");
}
// Get the browser name
public static String getName() {
String retval;
synchronized (FreeWRLToken) {
EAIoutSender.send ("" + queryno + "K\n");
retval = getVRMLreply(queryno);
queryno += 1;
}
return retval;
}
public String getVersion() {
String retval;
synchronized (FreeWRLToken) {
EAIoutSender.send ("" + queryno + "L\n");
retval = getVRMLreply(queryno);
queryno += 1;
}
return retval;
}
// Get the current velocity of the bound viewpoint in meters/sec,
public float getCurrentSpeed() {
String retval;
synchronized (FreeWRLToken) {
EAIoutSender.send ("" + queryno + "M\n");
retval = getVRMLreply(queryno);
queryno += 1;
}
return Float.valueOf(retval).floatValue();
}
// Get the current frame rate of the browser, or 0.0 if not available
public float getCurrentFrameRate() {
String retval;
synchronized (FreeWRLToken) {
EAIoutSender.send ("" + queryno + "N\n");
retval = getVRMLreply(queryno);
queryno += 1;
}
return Float.valueOf(retval).floatValue();
}
// Get the URL for the root of the current world, or an empty string
// if not available
public String getWorldURL() {
String retval;
synchronized (FreeWRLToken) {
EAIoutSender.send ("" + queryno + "O\n");
retval = getVRMLreply(queryno);
queryno += 1;
}
return retval;
}
// Replace the current world with the passed array of nodes
public void replaceWorld(Node[] nodes)
throws IllegalArgumentException {
String SysString = "";
String retval;
int count;
for (count=0; count<nodes.length; count++) {
SysString = SysString + " " + nodes[count].nodeptr;
}
synchronized (FreeWRLToken) {
EAIoutSender.send ("" + queryno + "P" + SysString);
retval = getVRMLreply(queryno);
queryno += 1;
}
}
// Load the given URL with the passed parameters (as described
// in the Anchor node)
public void loadURL(String[] url, String[] parameter) {
System.out.println ("EAI: loadURL Not Implemented");
return
没有合适的资源?快使用搜索试试~ 我知道了~
FreeWRL-opengl编程
需积分: 10 3 下载量 167 浏览量
2009-09-14
14:34:02
上传
评论
收藏 3.81MB GZ 举报
温馨提示
利用opengl实现的一些比较悬的效果,可用于初学者学习
资源推荐
资源详情
资源评论
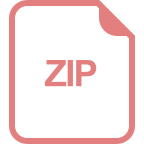
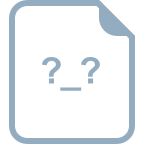
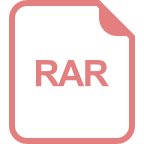
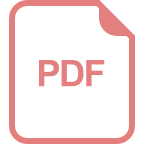
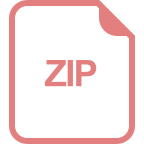
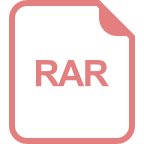
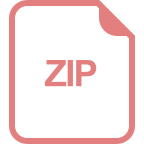
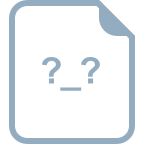
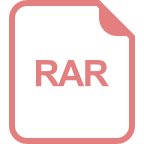
收起资源包目录

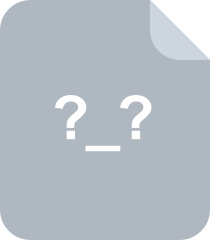
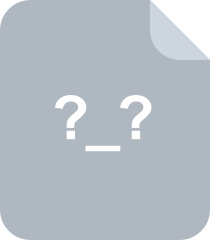
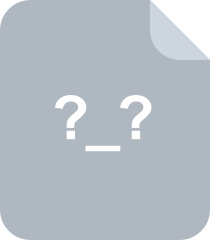
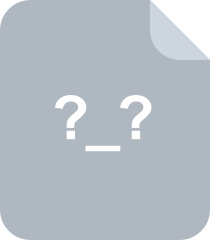
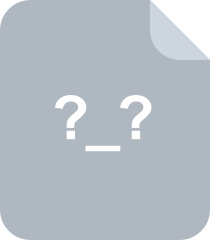
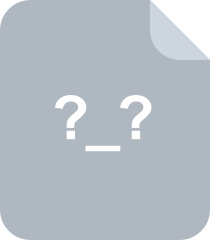
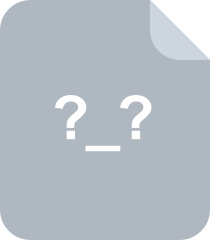
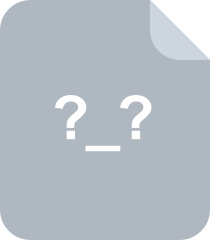
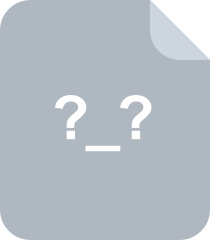
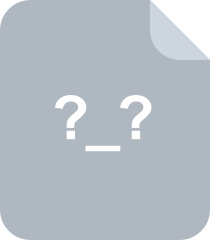
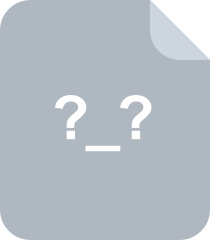
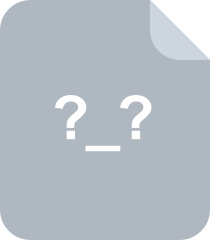
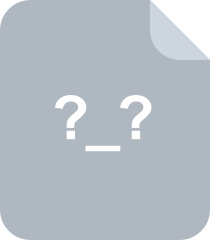
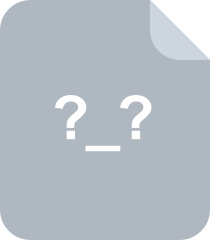
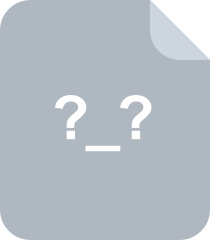
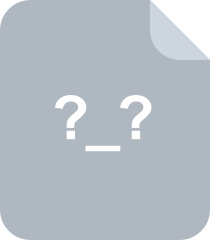
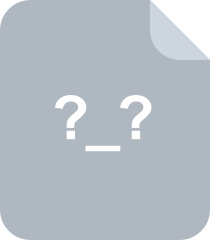
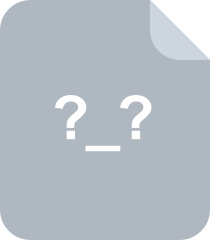
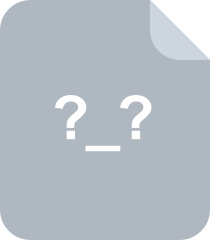
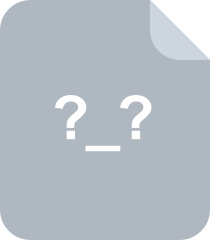
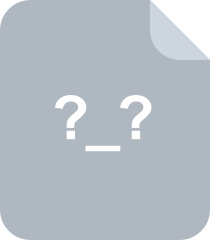
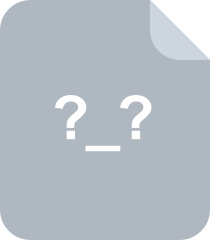
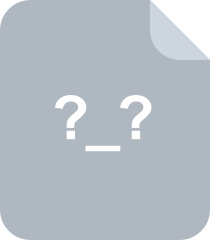
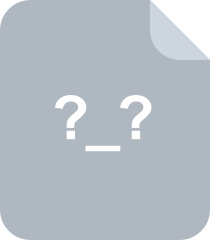
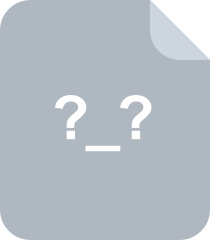
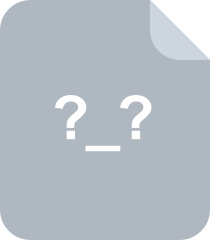
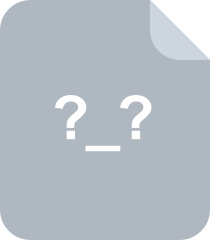
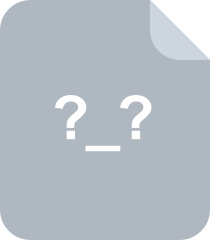
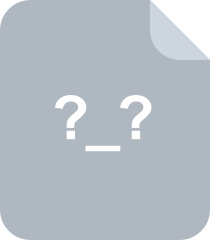
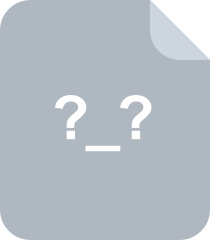
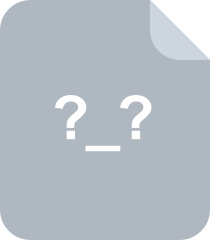
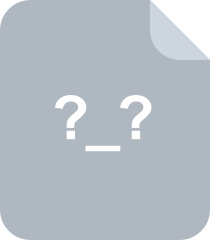
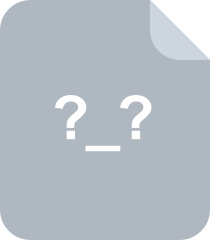
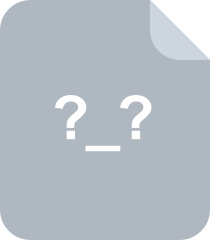
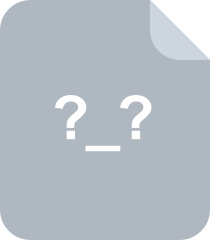
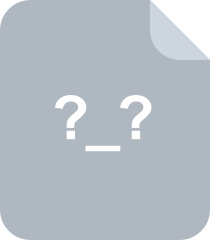
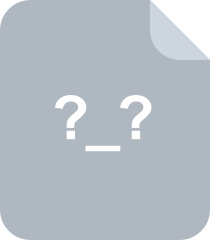
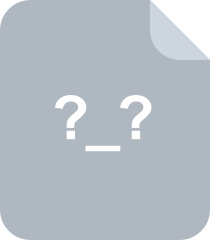
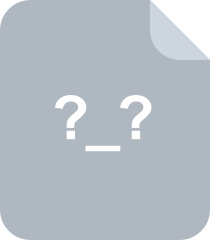
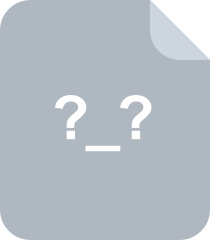
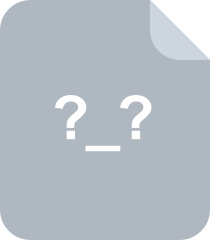
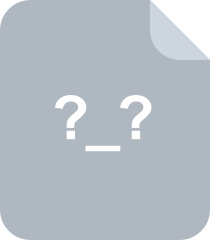
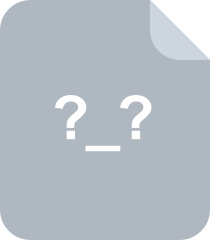
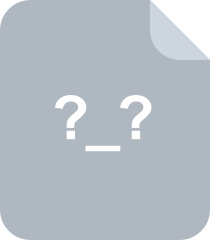
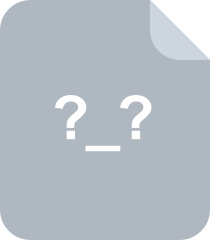
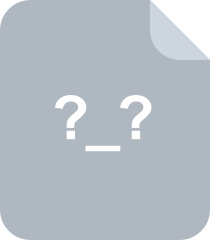
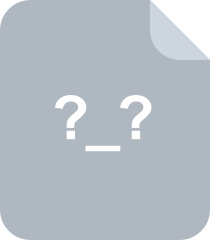
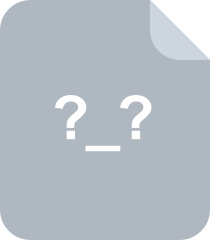
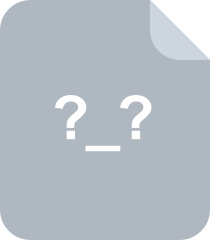
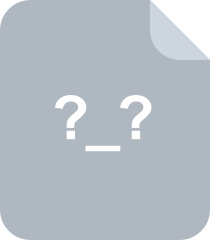
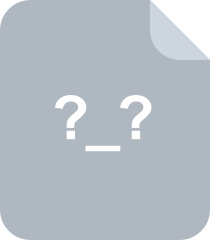
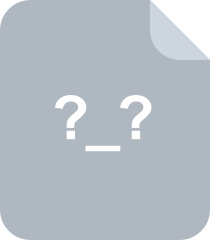
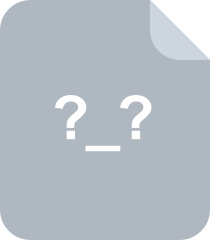
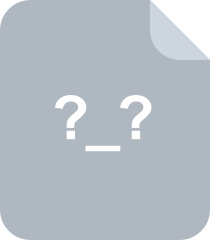
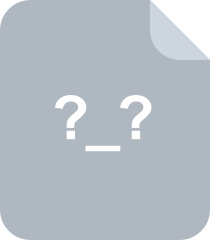
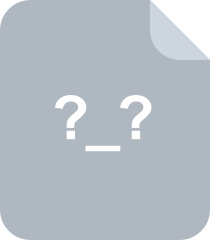
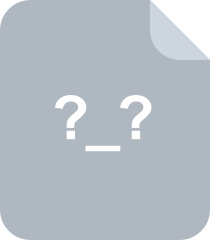
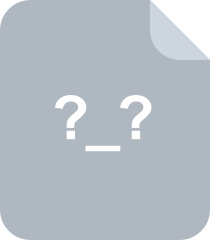
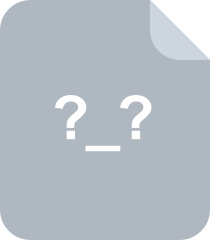
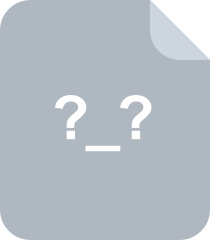
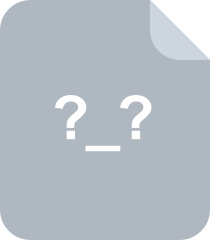
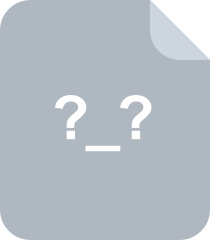
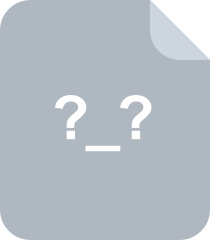
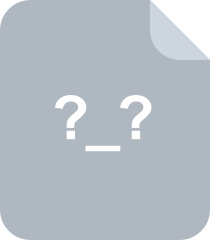
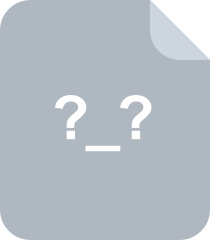
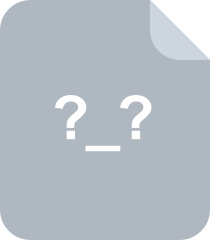
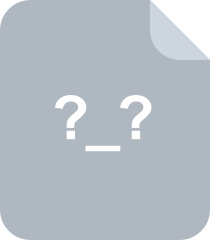
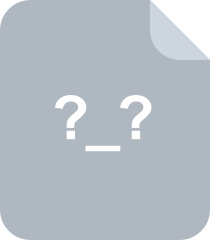
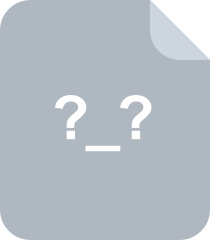
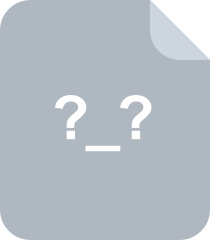
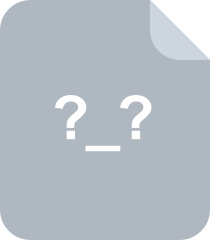
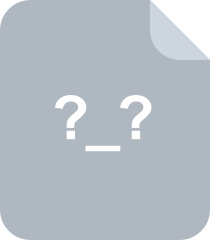
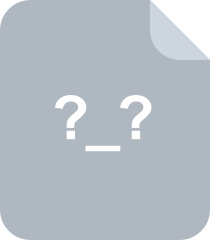
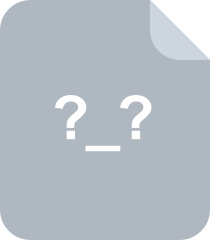
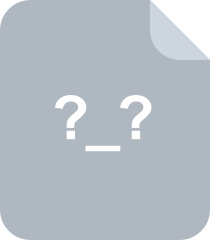
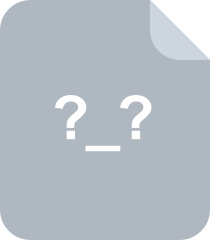
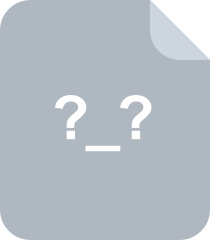
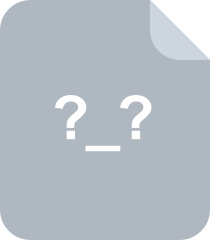
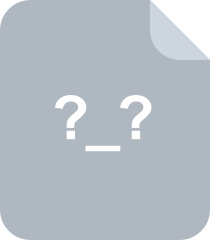
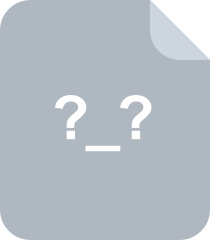
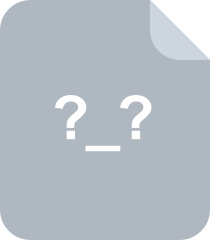
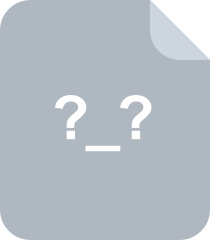
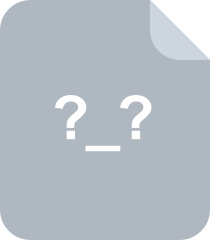
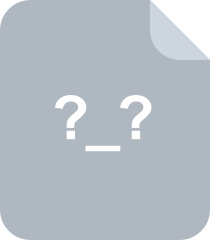
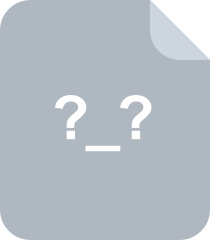
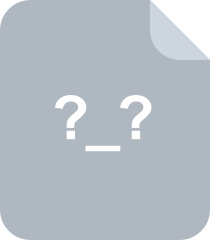
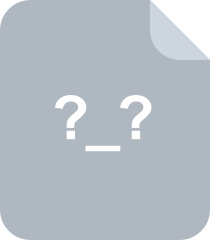
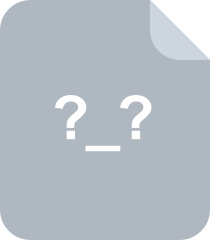
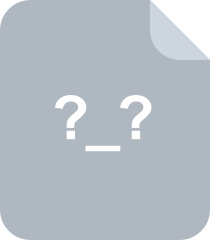
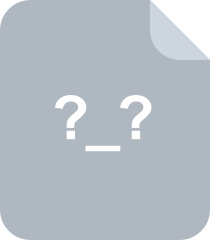
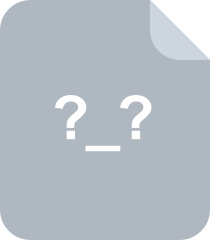
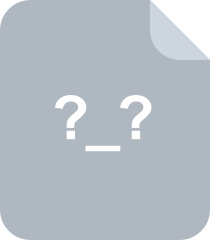
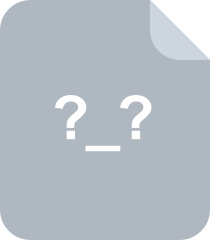
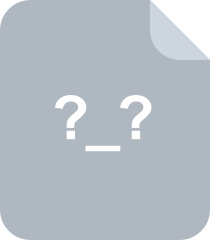
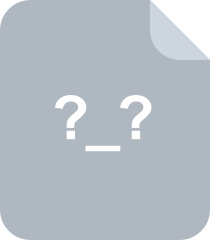
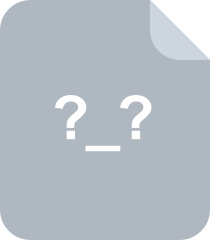
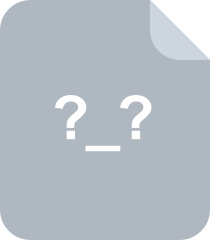
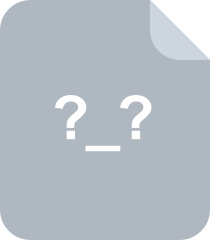
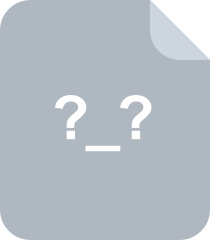
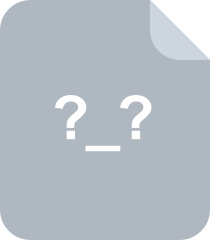
共 1026 条
- 1
- 2
- 3
- 4
- 5
- 6
- 11
资源评论
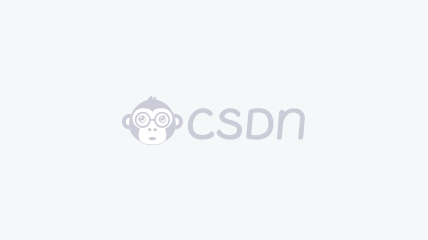

lixiaokai8990
- 粉丝: 6
- 资源: 11
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

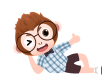
最新资源
- “人力资源+大数据+薪酬报告+涨薪调薪”
- PVE系统配置优化脚本
- “人力资源+大数据+薪酬报告+涨薪调薪”
- 含源码java Swing基于socket实现的五子棋含客户端和服务端
- 【java毕业设计】鹿幸公司员工在线餐饮管理系统的设计与实现源码(springboot+vue+mysql+LW).zip
- OpenCV C++第三方库
- 毕设分享:基于SpringBoot+Vue的礼服租聘系统-后端
- 复合铜箔:预计到2025年,这一数字将跃升至291.5亿元,新材料革命下的市场蓝海
- 【java毕业设计】流浪动物管理系统源码(springboot+vue+mysql+说明文档+LW).zip
- 【源码+数据库】采用纯原生的方式,基于mybatis框架实现增删改查
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


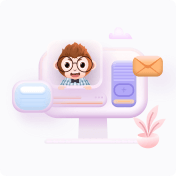
安全验证
文档复制为VIP权益,开通VIP直接复制
