根据给定文件的信息,我们可以总结出以下几个主要的知识点: ### 1. 数据排序与输出 在函数 `print_descending` 中,实现了一个简单的算法来对三个整数进行降序排列并输出。 ```c void print_descending(int x, int y, int z) { scanf("%d,%d,%d", &x, &y, &z); // 输入三个整数 if (x < y) swap(x, y); // 如果 x 小于 y,则交换它们 if (y < z) swap(y, z); // 如果 y 小于 z,则交换它们 if (x < y) swap(x, y); // 再次检查 x 和 y 的顺序 printf("%d%d%d", x, y, z); // 输出降序排列后的结果 } ``` 其中,`swap` 函数用于交换两个变量的值。这个函数通过比较输入的三个整数,确保它们按照从大到小的顺序输出。 ### 2. 斐波那契数列变种 函数 `fib` 实现了一个斐波那契数列的变种计算方法。 ```c Status fib(int k, int m, int& f) { int tempd; if (k < 2 || m < 0) return ERROR; // 验证输入的有效性 if (m == 0 || m == 1) f = 1; // 初始化条件 else { int temp[m]; // 创建一个动态数组存储中间结果 temp[0] = 0; temp[1] = 1; for (int i = 2; i <= m; i++) { // 计算斐波那契数列的第 m 项 temp[i] = temp[i - 1] + temp[i - 2]; if (i >= k) temp[i] += temp[i - k]; // 加入额外的计算规则 } f = temp[m]; // 返回计算结果 } return OK; } ``` 该函数接收两个整数参数 `k` 和 `m`,计算斐波那契数列的一个变种版本的第 `m` 项,其中每一项不仅依赖于前两项,还额外依赖于 `k` 步之前的项。此算法的时间复杂度为 O(m),空间复杂度为 O(m)。 ### 3. 体育比赛成绩统计 函数 `summary` 实现了对多个体育比赛的结果进行统计的功能。 ```c typedef struct { char *sport; // 比赛项目 enum {male, female} gender; // 性别 char schoolname; // 学校名称 char *result; // 比赛结果 int score; // 得分 } resulttype; typedef struct { int malescore; // 男生总分 int femalescore; // 女生总分 int totalscore; // 总分 } scoretype; void summary(resulttype result[]) { scoretype score[MAXSIZE]; int i = 0; while (result[i].sport != NULL) { // 遍历所有结果 switch (result[i].schoolname) { case 'A': case 'B': // 更新对应学校的成绩统计 score[0].totalscore += result[i].score; if (result[i].gender == male) score[0].malescore += result[i].score; else score[0].femalescore += result[i].score; break; } i++; } // 打印每个学校的得分情况 for (int i = 0; i < 5; i++) { printf("School %d:\n", i); printf("Total score of male: %d\n", score[i].malescore); printf("Total score of female: %d\n", score[i].femalescore); printf("Total score of all: %d\n\n", score[i].totalscore); } } ``` 该函数通过遍历传入的 `result` 数组,并根据每个参赛者的学校、性别以及得分更新对应的统计数据。打印出每个学校男生、女生及总分的情况。 ### 4. 数组运算与异常处理 函数 `algo119` 展示了一种数组运算的方法,并处理了可能出现的溢出异常。 ```c Status algo119(int a[ARRSIZE]) { int last = 1; for (int i = 1; i <= ARRSIZE; i++) { a[i - 1] = last * 2 * i; if ((a[i - 1] / last) != (2 * i)) return OVERFLOW; // 检查是否溢出 last = a[i - 1]; } return OK; } ``` 该函数接收一个整型数组作为参数,并通过循环填充数组中的元素。为了避免整数溢出,通过判断 `a[i - 1] / last` 是否等于 `2 * i` 来检测是否发生溢出。如果出现溢出,则返回 `OVERFLOW`;否则返回 `OK`。 ### 5. 多项式求值 函数 `polyvalue` 实现了多项式的求值功能。 ```c void polyvalue() { float temp; float *p = a; printf("Input number of terms:"); scanf("%d", &n); printf("Input value of x:"); scanf("%f", &x); printf("Input the %d coefficients from a0 to a%d:\n", n + 1, n); p = a; xp = 1; sum = 0; for (int i = 0; i <= n; i++) { scanf("%f", &temp); sum += xp * (temp); xp *= x; } printf("Value is: %f", sum); } ``` 该函数首先获取多项式的项数、变量 `x` 的值以及每一项的系数。然后通过循环计算多项式的值并输出。此函数提供了一种简单有效的方法来计算任意多项式的值。 ### 6. 删除数组指定位置的元素 最后一个片段展示了如何删除数组中指定位置的元素。 ```c Status DeleteK(SqList &a, int i, int k) { // 删除 SqList 类型数组 a 中从位置 i 开始的 k 个元素 } ``` 这个函数的具体实现没有给出,但可以推测其功能是删除数组 `a` 中从位置 `i` 开始的 `k` 个元素。这通常可以通过将后续元素向前移动的方式实现,从而覆盖被删除的元素。
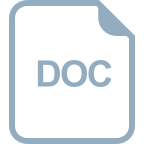
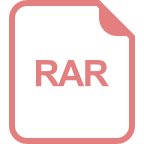
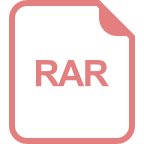
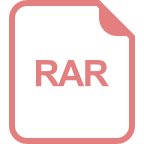
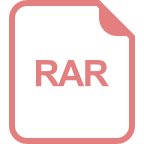
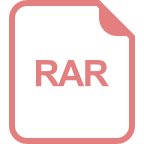
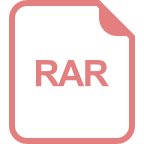
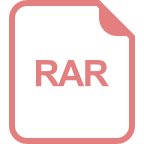
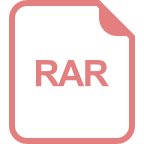
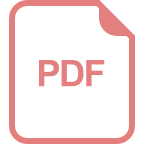
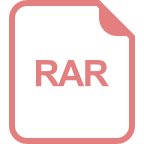
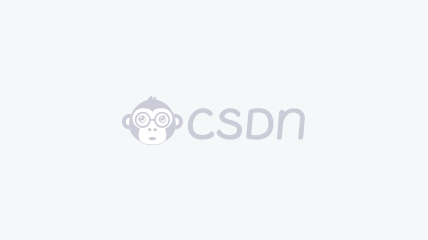

- 粉丝: 35
- 资源: 37
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

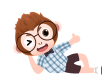
