// TEST3Dlg.cpp : implementation file
//
#include "stdafx.h"
#include "TEST3.h"
#include "TEST3Dlg.h"
#include "math.h"
#include "time.h"
#include "memory.h"
#ifdef _DEBUG
#define new DEBUG_NEW
#undef THIS_FILE
static char THIS_FILE[] = __FILE__;
#endif
/////////////////////////////////////////////////////////////////////////////
// CAboutDlg dialog used for App About
typedef struct Side{// 路径中的节点
int point; // 节点编号
struct Side *next;
}DefSide;
typedef struct Result{// 最短路径属性
int flag; // 标记
int start; // 路径的起始点,(除源点)
int totalValue; // 整条路径的总权值
DefSide *thead; // 路径经过的各个节点
}DefResult;
class CAboutDlg : public CDialog
{
public:
CAboutDlg();
// Dialog Data
//{{AFX_DATA(CAboutDlg)
enum { IDD = IDD_ABOUTBOX };
//}}AFX_DATA
// ClassWizard generated virtual function overrides
//{{AFX_VIRTUAL(CAboutDlg)
protected:
virtual void DoDataExchange(CDataExchange* pDX); // DDX/DDV support
//}}AFX_VIRTUAL
// Implementation
protected:
//{{AFX_MSG(CAboutDlg)
//}}AFX_MSG
DECLARE_MESSAGE_MAP()
};
CAboutDlg::CAboutDlg() : CDialog(CAboutDlg::IDD)
{
//{{AFX_DATA_INIT(CAboutDlg)
//}}AFX_DATA_INIT
}
void CAboutDlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CAboutDlg)
//}}AFX_DATA_MAP
}
BEGIN_MESSAGE_MAP(CAboutDlg, CDialog)
//{{AFX_MSG_MAP(CAboutDlg)
// No message handlers
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CTEST3Dlg dialog
CTEST3Dlg::CTEST3Dlg(CWnd* pParent /*=NULL*/)
: CDialog(CTEST3Dlg::IDD, pParent)
{
//{{AFX_DATA_INIT(CTEST3Dlg)
// NOTE: the ClassWizard will add member initialization here
//}}AFX_DATA_INIT
// Note that LoadIcon does not require a subsequent DestroyIcon in Win32
m_hIcon = AfxGetApp()->LoadIcon(IDR_MAINFRAME);
}
void CTEST3Dlg::DoDataExchange(CDataExchange* pDX)
{
CDialog::DoDataExchange(pDX);
//{{AFX_DATA_MAP(CTEST3Dlg)
// NOTE: the ClassWizard will add DDX and DDV calls here
//}}AFX_DATA_MAP
}
BEGIN_MESSAGE_MAP(CTEST3Dlg, CDialog)
//{{AFX_MSG_MAP(CTEST3Dlg)
ON_WM_SYSCOMMAND()
ON_WM_PAINT()
ON_WM_QUERYDRAGICON()
ON_BN_CLICKED(IDC_BUTTON1, OnButton1)
ON_BN_CLICKED(IDC_BUTTON2, Onlength)
//}}AFX_MSG_MAP
END_MESSAGE_MAP()
/////////////////////////////////////////////////////////////////////////////
// CTEST3Dlg message handlers
BOOL CTEST3Dlg::OnInitDialog()
{
CDialog::OnInitDialog();
// Add "About..." menu item to system menu.
// IDM_ABOUTBOX must be in the system command range.
ASSERT((IDM_ABOUTBOX & 0xFFF0) == IDM_ABOUTBOX);
ASSERT(IDM_ABOUTBOX < 0xF000);
CMenu* pSysMenu = GetSystemMenu(FALSE);
if (pSysMenu != NULL)
{
CString strAboutMenu;
strAboutMenu.LoadString(IDS_ABOUTBOX);
if (!strAboutMenu.IsEmpty())
{
pSysMenu->AppendMenu(MF_SEPARATOR);
pSysMenu->AppendMenu(MF_STRING, IDM_ABOUTBOX, strAboutMenu);
}
}
// Set the icon for this dialog. The framework does this automatically
// when the application's main window is not a dialog
SetIcon(m_hIcon, TRUE); // Set big icon
SetIcon(m_hIcon, FALSE); // Set small icon
// TODO: Add extra initialization here
return TRUE; // return TRUE unless you set the focus to a control
}
void CTEST3Dlg::OnSysCommand(UINT nID, LPARAM lParam)
{
if ((nID & 0xFFF0) == IDM_ABOUTBOX)
{
CAboutDlg dlgAbout;
dlgAbout.DoModal();
}
else
{
CDialog::OnSysCommand(nID, lParam);
}
}
// If you add a minimize button to your dialog, you will need the code below
// to draw the icon. For MFC applications using the document/view model,
// this is automatically done for you by the framework.
void CTEST3Dlg::OnPaint()
{
if (IsIconic())
{
CPaintDC dc(this); // device context for painting
SendMessage(WM_ICONERASEBKGND, (WPARAM) dc.GetSafeHdc(), 0);
// Center icon in client rectangle
int cxIcon = GetSystemMetrics(SM_CXICON);
int cyIcon = GetSystemMetrics(SM_CYICON);
CRect rect;
GetClientRect(&rect);
int x = (rect.Width() - cxIcon + 1) / 2;
int y = (rect.Height() - cyIcon + 1) / 2;
// Draw the icon
dc.DrawIcon(x, y, m_hIcon);
}
else
{
CDialog::OnPaint();
}
}
// The system calls this to obtain the cursor to display while the user drags
// the minimized window.
HCURSOR CTEST3Dlg::OnQueryDragIcon()
{
return (HCURSOR) m_hIcon;
}
int m_Link1[600000]={0}; //存放连接边的起点
int m_Link2[600000]={0}; //存放连接边的终边
int *m_Node;
int *m_OutDegree; //存放节点的出度、入度
int *m_InDegree;
int *m_OutWeight; //存放节点的出权、入权
int *m_InWeight;
int *m_NodeWeight; //存放点权
int *m_InWeightVsNum,*m_OutWeightVsNum,*m_EdgeWeightVsNum,*m_NodeWeightVsNum;
int *m_DegreeVsNum,*m_OutDegreeVsNum,*m_InDegreeVsNum; //存放网络所有的边
int *m_Edge;
int *m_Degree; //存放网络节点的度
int m_EdgeWeight[17][17]; //表示两节点之间边的权重,如m_EdgeWeight[i][j]表示 i和j之间的边的权重值
long int m_Nodeptr=1; //存放网络所有节点的当前存储位置指针
float m_ClusterCo,m_MeanLean;
void CTEST3Dlg::OnButton1()
{
// TODO: Add your control notification handler code here
FILE *fp,*fp1,*Node,*fp_outmeasure,*fp_out;
long int m_TotalDegree=0; //存放网络所有节点的总度
long int m_TotalEdgeWeight=0; //存放网络所有边的总权重
long int m_Degreeptr=0; //存放网络节点度的当前存储位置指针
long int m_Edgeptr=0; //存放网络所有边的当前存储位置指针
long int m_OutDegreeptr=0; //存放网络节点出度的当前存储位置指针
long int m_InDegreeptr=0; //存放网络节点入度的当前存储位置指针
//屏蔽点的代码是用来计算度分布的
long int m_MaxDegree,m_MaxOutDegree,m_MaxInDegree,m_MaxInWeight,m_MaxOutWeight,m_MaxEdgeWeight,m_MaxNodeWeight;
m_MaxDegree=m_MaxOutDegree=m_MaxInDegree=m_MaxInWeight=m_MaxOutWeight=m_MaxEdgeWeight=m_MaxNodeWeight=0;
long int i,j;
char line[256],line2[256],line3[256];
int m_Temp;
m_Node=(int *)calloc(1,sizeof(int)*17);
m_Degree=(int *)calloc(1,sizeof(int)*17);
m_OutDegree=(int *)calloc(1,sizeof(int)*17);
m_InDegree=(int *)calloc(1,sizeof(int)*17);
m_NodeWeight=(int *)calloc(1,sizeof(int)*17);
m_OutWeight=(int *)calloc(1,sizeof(int)*17);
m_InWeight=(int *)calloc(1,sizeof(int)*17);
m_Edge=(int *)calloc(1,sizeof(int)*1200);
for(i=0;i<17433;i++)
{ m_Node[i]=0; //为网络节点存储区清零
m_Degree[i]=0; //为网络节点度存储区清零
m_OutDegree[i]=0; //为网络节点出度存储区清零
m_InDegree[i]=0; //为网络节点入度存储区清零
m_OutWeight[i]=0; //为网络节点出度存储区清零
m_InWeight[i]=0; //为网络节点入度存储区清零
}
for(i=0;i<1200000;i++)
m_Edge[i]=0; //为网络边存储区清零
for(i=0;i<17433;i++)
{ for(j=0;j<17433;j++)
m_EdgeWeight[i][j]=0; //为网络边标志存储区清零
}
fp=fopen("authorid.txt","r"); //打开文本文件authorid
if(fp==NULL)
{
printf("cannot open this file\n");
exit(0);
}
fp1=fopen("p_authorid.txt","r"); //打开文本文件p_authorid
if(fp==NULL)
{
printf("cannot open this file\n");
exit(0);
}
Node=fopen("node.txt","r"); //打开文本文件node
if(fp==NULL)
{
printf("cannot open this file\n");
exit(0);
}
//获取authorid文本文件的数据,即连接的起点
i=0;
j=1;
fgets(line,256,fp);
while (!feof(fp))
{
m_Temp=atoi(line);
m_Link1[i]=m_Temp;
i++;
j++;
fgets(line,256,fp);
}
//获取p_authorid文本文件的数据,即�

limaolin1981611
- 粉丝: 0
- 资源: 5
最新资源
- 开关电源工程师-应具备的理论知识,实践技能和工程素质
- 技术资料分享CC2530中文数据手册完全版非常好的技术资料.zip
- 技术资料分享CC2530非常好的技术资料.zip
- 技术资料分享AU9254A21非常好的技术资料.zip
- 技术资料分享AT070TN92非常好的技术资料.zip
- 技术资料分享ADV7123非常好的技术资料.zip
- TestBank.java
- js-leetcode题解之146-lru-cache.js
- js-leetcode题解之145-binary-tree-postorder-traversal.js
- js-leetcode题解之144-binary-tree-preorder-traversal.js
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


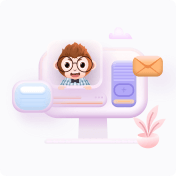
- 1
- 2
- 3
- 4
- 5
- 6
前往页