package com.miaotian;
import java.io.BufferedReader;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.Iterator;
import java.util.TreeMap;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.Intent;
import android.database.Cursor;
import android.media.MediaPlayer;
import android.media.MediaPlayer.OnCompletionListener;
import android.media.MediaPlayer.OnPreparedListener;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.os.Handler;
import android.os.Message;
import android.provider.MediaStore;
import android.util.Log;
import android.view.KeyEvent;
import android.view.View;
import android.view.View.OnClickListener;
import android.view.ViewGroup.LayoutParams;
import android.widget.ImageButton;
import android.widget.ProgressBar;
import android.widget.SeekBar;
import android.widget.TextView;
import android.widget.Toast;
import android.widget.SeekBar.OnSeekBarChangeListener;
public class Music_Play extends Activity implements OnClickListener, Runnable {
// private TextView tv_lyrics;
private ViewlrcKLOK lyrics;
private TextView tv_has_played;
private TextView tv_duration;
private TextView tv_title;
private TextView tv_singer;
private SeekBar seekbar;
private ImageButton btn_setpbackward;
private ImageButton btn_play_pause;
private ImageButton btn_setpforward;
private MediaPlayer myMediaPlayer;
private int curPosition;
private int position;
private int[] ids;
private Uri uri;
private boolean isPlaying;
private Handler myHandler;
private Cursor myCur;
private OnPreparedListener mediaPreparedListener;
private OnCompletionListener mediaCompletionListner;
private OnSeekBarChangeListener seekbarChangeListener;
private String URL_str = "http://i01.c.aliimg.com/club/upload/2006/3/30/10369560_368f8262e.mp3";
private int num = 1;
private File download_file;
private boolean isOnline;
private int total_read = 0;
private int readLength = 0;
private int oldLength = 0;
private int video_length = 0;
private boolean flag;
private boolean canNext;
private AlertDialog dia;
private TreeMap<Integer, lrcObject> lrc_map = new TreeMap<Integer, lrcObject>();
private int lrc_timeend = 0;
public void init() {
myHandler = new Handler() {
@Override
public void handleMessage(Message msg) {
switch (msg.what) {
case 1:// 开始播放
if (myMediaPlayer != null) {
if (isOnline) {
if (myMediaPlayer.getCurrentPosition() != 0) {
curPosition = myMediaPlayer
.getCurrentPosition();
}
if (!myMediaPlayer.isPlaying()) {
if (dia.isShowing()) {
break;
} else {
dia.show();
}
} else {
dia.dismiss();
}
} else {
curPosition = myMediaPlayer.getCurrentPosition();
if (curPosition > lrc_timeend && isPlaying) {
// 查找歌词
Iterator<Integer> iterator = lrc_map.keySet()
.iterator();
while (iterator.hasNext()) {
Object ob = iterator.next();
lrcObject val = (lrcObject) lrc_map.get(ob);
if (curPosition > val.begintime) {
lrcObject val_1 = new lrcObject();
val_1.begintime = 0;
val_1.lrc = "...";
val_1.timeline = 0;
if (iterator.hasNext()) {
Object ob_1 = iterator.next();
val_1 = (lrcObject) lrc_map
.get(ob_1);
}
lrc_timeend = val_1.begintime
+ val_1.timeline;
lyrics.SetlrcContent(val.lrc,
val.timeline, val_1.begintime
- val.begintime
- val.timeline,
val_1.lrc, val_1.timeline);
lyrics.invalidate();
}
}
}
}
tv_has_played.setText(toTime(curPosition));
seekbar.setProgress(curPosition);
myHandler.sendEmptyMessage(1);
}
break;
case 2:
setPath(download_file.getAbsolutePath());
break;
}
super.handleMessage(msg);
}
};
mediaPreparedListener = new OnPreparedListener() {
@Override
public void onPrepared(MediaPlayer mp) {
if (!isOnline) {
tv_duration.setText(toTime(myMediaPlayer.getDuration()));
seekbar.setMax(myMediaPlayer.getDuration());
}
isPlaying = true;
btn_play_pause
.setImageResource(R.drawable.media_player_pause_button_selecor);
myHandler.sendEmptyMessage(1);
myMediaPlayer.start();
lyrics.Star();
myMediaPlayer.seekTo(curPosition);
Log.d("test", "--------------" + curPosition);
}
};
mediaCompletionListner = new OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mp) {
if (isOnline) {
if (canNext) {
if (++position > ids.length - 1) {
position = 0;
}
setPath(ids[position]);
refreshView();
canNext = false;
isOnline = false;
}
} else {
if (++position > ids.length - 1) {
position = 0;
}
setPath(ids[position]);
refreshView();
}
}
};
seekbarChangeListener = new OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress,
boolean fromUser) {
if (fromUser) {
if (!isOnline) {
myMediaPlayer.seekTo(progress);
}
}
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
// TODO Auto-generated method stub
}
};
// tv_lyrics = (TextView) findViewById(R.id.lyrics);
lyrics = (ViewlrcKLOK) findViewById(R.id.lyrics);
lyrics.SetlrcContent("没有找到歌词", 0, 0, "…………", 0);
tv_has_played = (TextView) findViewById(R.id.has_played);
tv_duration = (TextView) findViewById(R.id.duration);
tv_title = (TextView) findViewById(R.id.title);
tv_title.setSelected(true);
tv_singer = (TextView) findViewById(R.id.singer);
seekbar = (SeekBar) findViewById(R.id.seekbar);
btn_setpbackward = (ImageButton) findViewById(R.id.btn_stepbackward);
btn_setpbackward.setOnClickListener(this);
btn_play_pause = (ImageButton) findViewById(R.id.btn_play_pause);
btn_play_pause.setOnClickListener(this);
btn_setpforward = (ImageButton) findViewById(R.id.btn_stepforward);
btn_setpforward.setOnClickListener(this);
dia = new AlertDialog.Builder(this).create();
View view = getLayoutInflater().inflate(R.layout.download, null);
dia.setView(view);
position = getIntent().getIntExtra("position", 0);
ids = getIntent().getIntArrayExtra("ids");
setPath(ids[position]);
}
public String toTime(int times) {
times /= 1000;
int minutes = times / 60;
int hour = minutes / 60;
int seconds = times % 60;
minutes %= 60;
return String.format("%02d:%02d", minutes, seconds);
}
public void setPath(int position) {
uri = Uri.withAppendedPath(MediaStore.Audio.Media.EXTERNAL_CONTENT_URI,
"" + position);
if (myMediaPlayer != null) {
myMediaPlayer.reset();
myMediaPlayer.release();
myMediaPlayer = null;
}
try {
myMediaPlayer = new MediaPlayer();
myMediaPlayer.setOnPreparedListener(mediaPreparedListener);
myMediaPlayer.setOnCompletionListener(mediaCompletionListner);
myMediaPlayer.setDataSource(this, uri);
myMediaPlayer.prepare();
} catch (Exception e) {
// TODO: handle exception
}
}
public void setPath(String uriString) {
uri = Uri.parse(uriString);
if (myMediaPlayer != null) {
myMediaPlayer.reset();
myMediaPlayer.release();
myMediaPlayer =
android 整理代码笔记
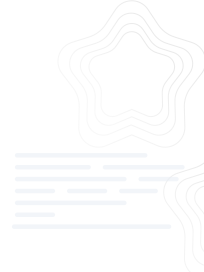


在Android开发领域,掌握核心知识点是提升编程技能的关键。这篇笔记主要涵盖了多个Android开发的实践模块,包括数据存储、用户界面、网络通信等基础且重要的主题。以下是对这些主题的详细解析: 1. **Fuxi**: 可能是指一个项目的基础框架或者组件,Fuxi在汉语中没有特定的编程含义,但在这里可能是指开发者自定义的一个框架或系统,用于提高代码复用性和项目结构的合理性。 2. **ListView**: ListView是Android中常用的控件,用于显示大量可滚动的数据列表。开发者通常需要自定义适配器(Adapter)来绑定数据,并处理item点击事件和滑动刷新等交互功能。 3. **Client**: 在Android中,Client通常指的是客户端应用,可能是实现与服务器进行数据交换的应用部分。这涉及到网络请求、JSON解析、HTTPS安全通信等技术,例如使用OkHttp或Retrofit库进行网络请求。 4. **Music_Test**: 这个文件名暗示了一个关于音乐播放的功能测试。在Android中,可以使用MediaPlayer类来播放音频文件,同时还需要处理媒体控制(如播放、暂停、停止)、音频焦点管理等问题。 5. **MyService**: Android中的服务(Service)是一种在后台长时间运行的组件,即使用户离开应用程序也可以继续执行任务。MyService可能是一个自定义的服务,用于执行后台音乐播放、定时任务或其他持久性操作。 6. **MyClient**: 类似于Client,MyClient可能是开发者创建的特定功能的客户端,比如与服务器进行特定协议的通信,或者实现特定的业务逻辑。 7. **SharedPreferences**: SharedPreferences是Android中轻量级的数据存储方式,用于保存简单的键值对数据,如用户的设置或应用状态,适合小量非敏感数据的持久化。 8. **Custom1**: 这可能代表一个自定义视图或组件。在Android中,为了满足个性化需求,开发者常会根据需求扩展标准UI组件,如自定义布局、按钮样式等。 9. **SQLite**: SQLite是Android内置的关系型数据库,适用于存储大量结构化数据。开发者需要掌握SQL语句、ContentProvider以及Cursor的概念,以便在应用中创建、查询、更新和删除数据。 10. **MyCustomer**: 这可能是表示一个自定义的用户类或者客户类,用于处理与用户相关的业务逻辑,例如用户登录、注册、权限验证等。 通过这些笔记,开发者可以深入了解Android开发的多个方面,包括UI设计、数据存储、网络通信以及服务管理等,这些都是构建完整Android应用不可或缺的知识点。学习并熟练运用这些技能,将有助于提升开发者在Android平台上的编程能力。



























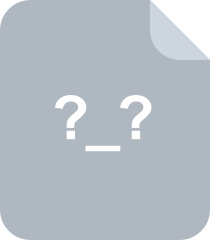
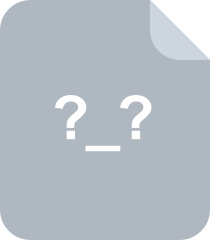
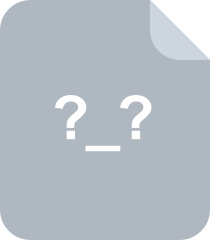
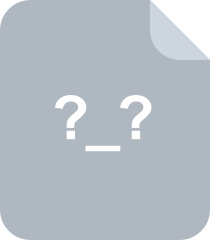
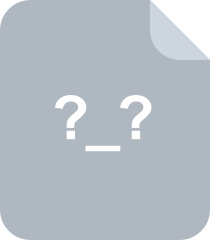
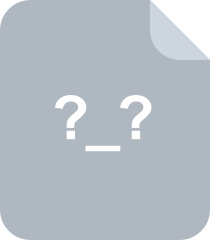
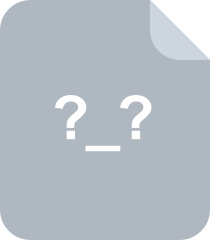
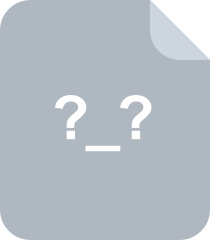
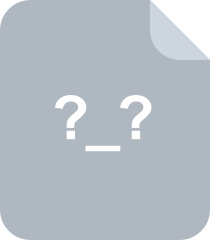
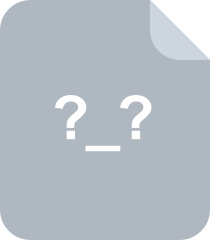
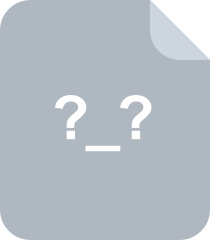
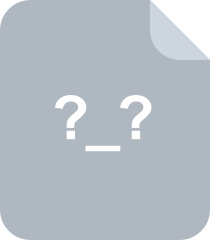
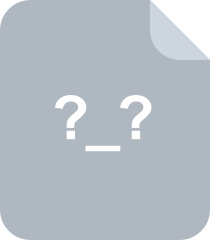
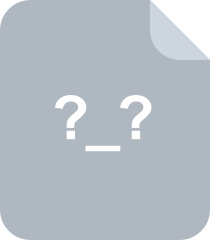
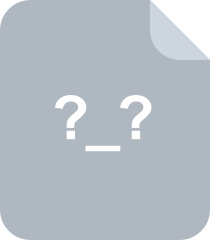
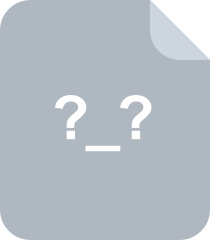
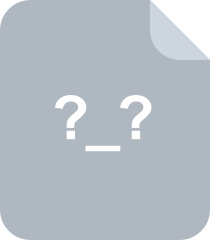
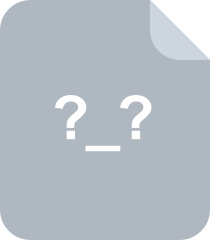
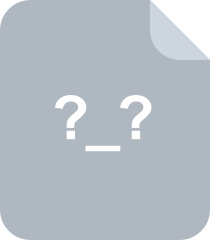
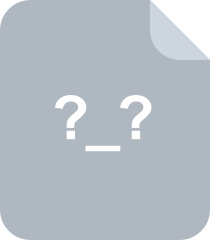
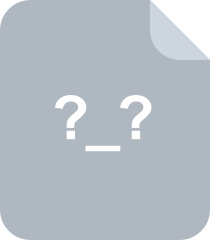
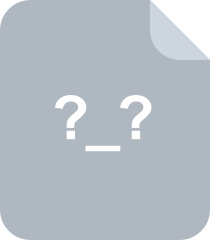
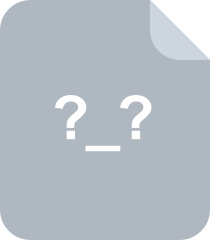
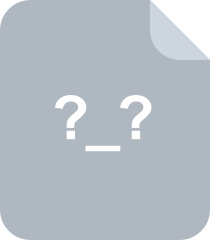
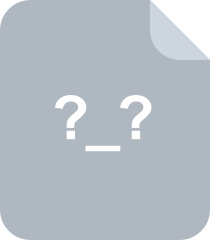
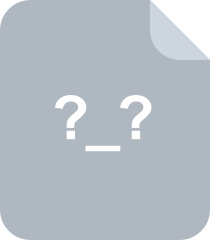
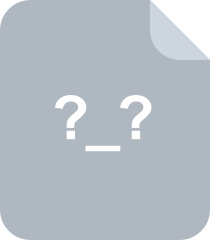
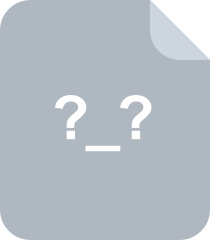
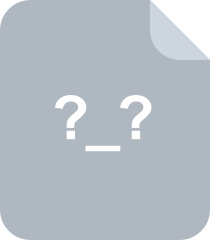
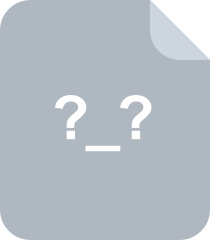
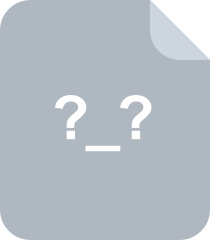
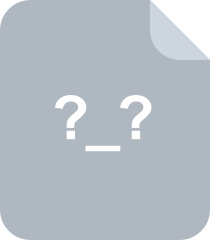
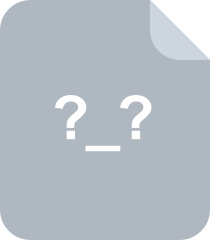
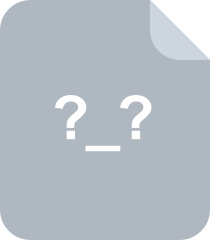
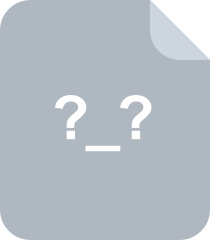
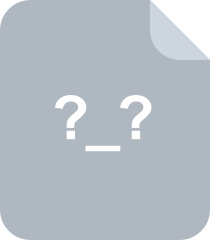
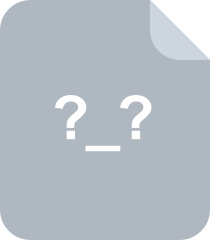
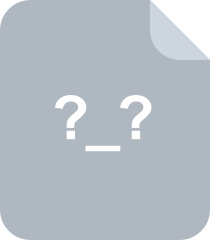
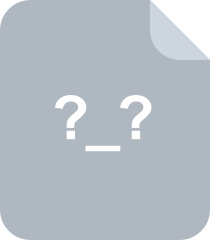
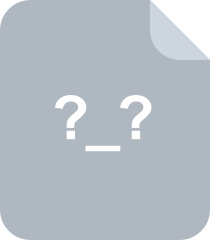
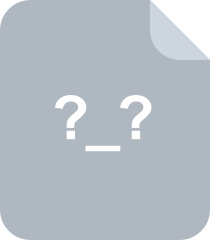
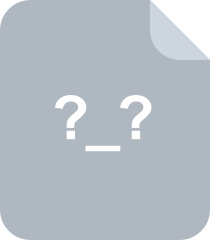
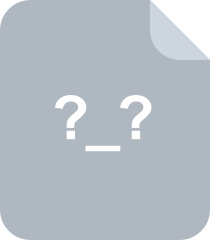
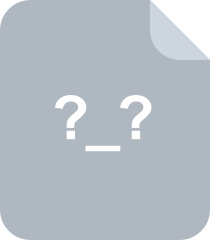
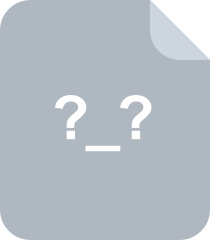
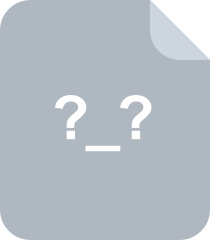
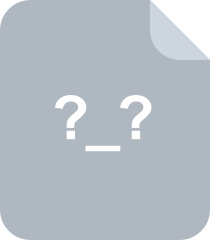
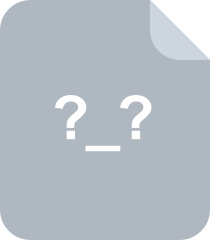
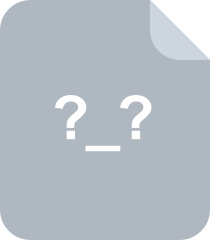
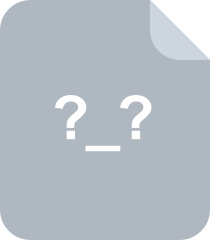
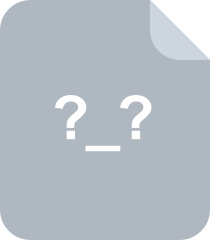
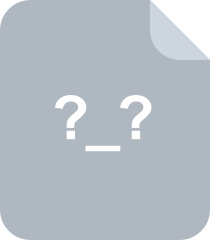
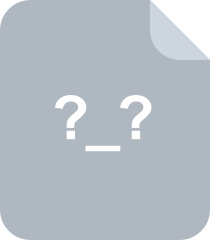
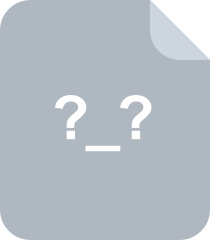
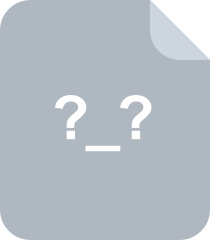
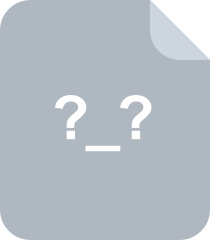
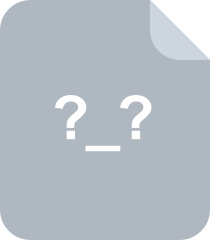
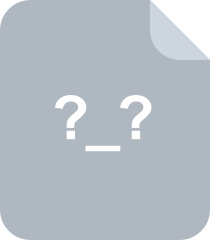
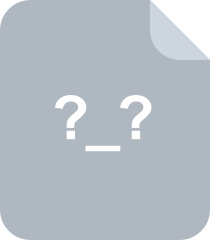
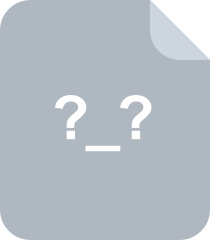
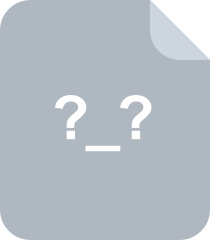
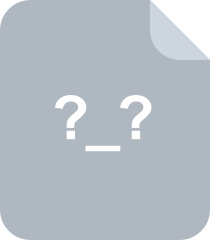
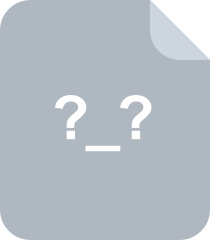
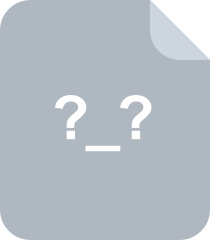
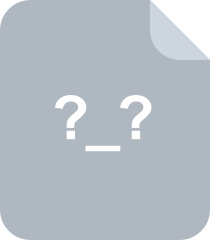
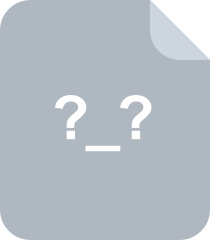
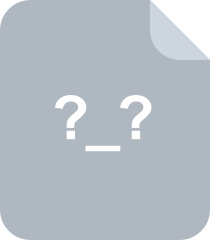
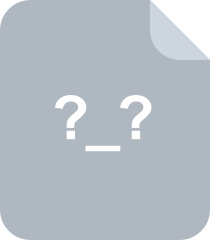
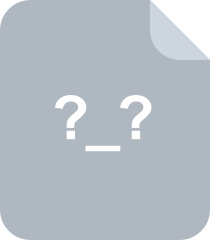
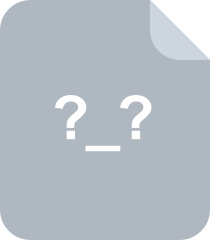
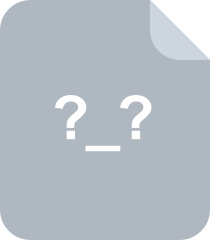
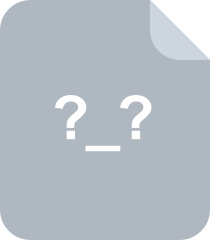
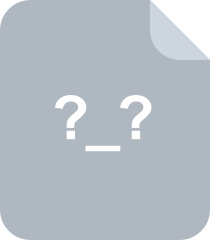
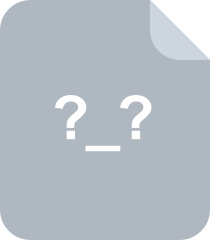
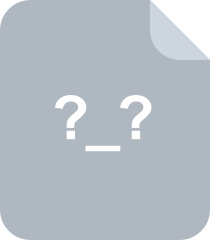
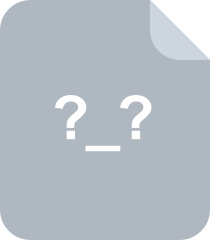
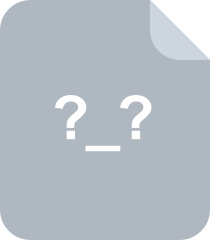
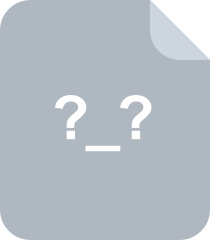
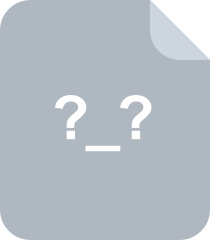
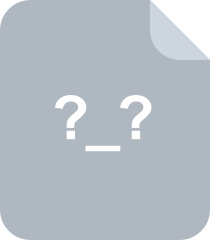
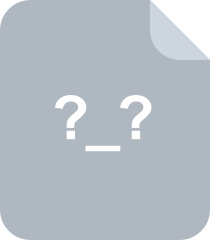
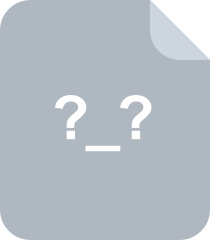
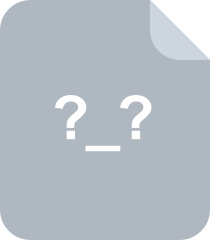
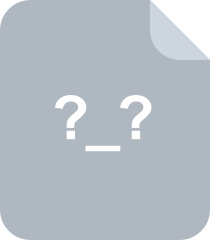
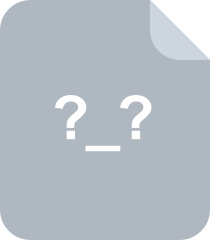
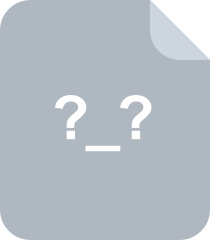
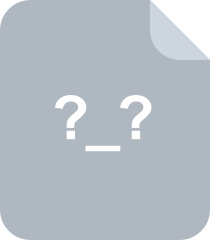
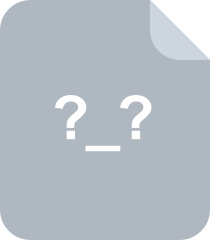
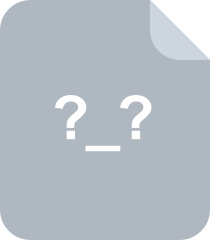
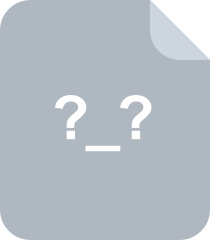
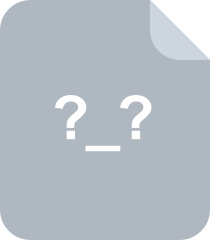
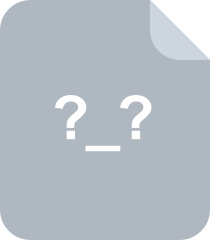
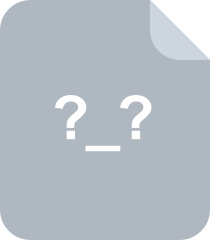
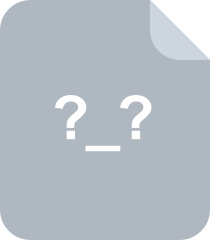
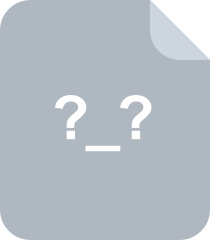
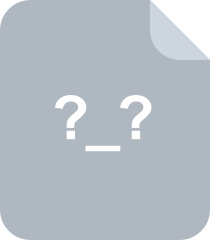
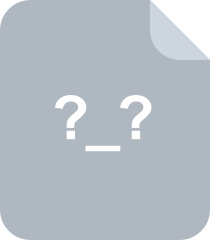
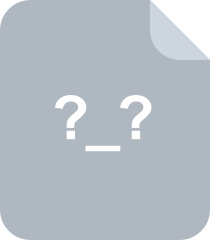
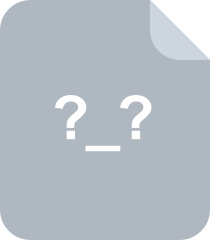
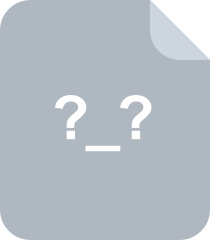
- 1
- 2
- 3
- 4
- 5
- 6
- 7
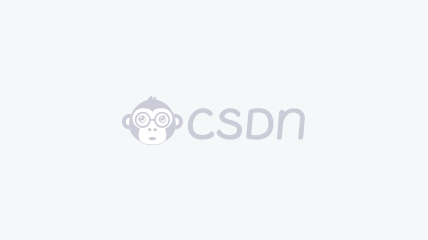
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整
- hbldw5212013-03-13看了资料,对自己进来研究android帮助很大

- 粉丝: 0
- 资源: 8
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

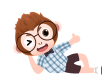
最新资源

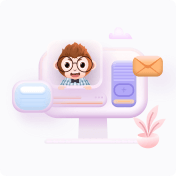
