# ClauDB
ClauDB is a REDIS implementation in Java. At the moment is in development and only implements a small
subset of commands and features. The objetive is implement a full functional one-to-one replacement
for REDIS (2.8 branch).
You will probably wonder why I do this, the answer is I do it Just For Fun.
## Why ClauDB?
Initially I called this project TinyDB, but there's another project with the same name, so, I've
decided to chage to ClaudDB.
Clau is :key: in Valencià, a language spoken in eastern Spain, and ClauDB is a key-value database.
## Implemented commands
<details>
<summary>Server</summary>
- FLUSHDB
- INFO
- TIME
- SYNC
- SLAVEOF
- ROLE
</details>
<details>
<summary>Connection</summary>
- ECHO
- PING
- QUIT
- SELECT
</details>
<details>
<summary>Key</summary>
- DEL
- EXISTS
- KEYS
- RENAME
- TYPE
- EXPIRE
- PERSIST
- TTL
- PTTL
</details>
<details>
<summary>String</summary>
- APPEND
- DECRBY
- DECR
- GET
- GETSET
- INCRBY
- INCR
- MGET
- MSET
- MSETNX
- SET (with NX, PX, NX and XX options)
- SETEX
- SETNX
- STRLEN
</details>
<details>
<summary>Hash</summary>
- HDEL
- HEXISTS
- HGETALL
- HGET
- HKEYS
- HLEN
- HMGET
- HMSET
- HSET
- HVALS
</details>
<details>
<summary>List</summary>
- LPOP
- LPUSH
- LINDEX
- LLEN
- LRANGE
- LSET
- RPOP
- RPUSH
</details>
<details>
<summary>Set</summary>
- SADD
- SCARD
- SDIFF
- SINTER
- SISMEMBER
- SMEMBERS
- SPOP
- SRANDMEMBER
- SREM
- SUNION
</details>
<details>
<summary>Sorted Set</summary>
- ZADD
- ZCARD
- ZRANGEBYSCORE
- ZRANGE
- ZREM
- ZREVRANGE
- ZINCRBY
</details>
<details>
<summary>Pub/Sub</summary>
- SUBSCRIBE
- UNSUBSCRIBE
- PSUBSCRIBE
- PUNSUBSCRIBE
- PUBLISH
</details>
<details>
<summary>Transactions</summary>
- MULTI
- EXEC
- DISCARD
</details>
<details>
<summary>Scripting</summary>
- EVAL
- EVALSHA
- SCRIPT LOAD
- SCRIPT EXISTS
- SCRIPT FLUSH
</details>
## Design
ClauDB is implemented using Java8. Is single thread, like REDIS. It uses asynchronous IO
(netty) and reactive programing paradigm (rxjava).
Requests come from IO threads and enqueues to rxjava single thread scheduler. Then IO thread is free
to process another request. When request is done, the response is sended to client asyncronously. Then,
every request is managed one by one, in a single thread, so there's no concurrency issues to care
about.
## Features
Now only implements a subset of REDIS commands, but is usable.
~~ClauDB also supports persistence compatible with REDIS, RDB dumps and AOF journal. It can create
compatible RDB files you can load in a REDIS server.~~ Removed since 2.0 version
Now ClauDB support master/slave replication, a master can have multiple slaves, but at the moment
slaves can't have slaves.
Also implements partially the Pub/Sub subsystem.
## Changes in 2.0 version
The RDB file format support has been dropped and replaced with h2 [MVStore](http://www.h2database.com/html/mvstore.html).
This allows ClauDB to have file persistence and off heap memory support.
Another important change, now ClauDB has been splited in several subprojects:
- claudb-lib: implementation of the server and commands.
- claudb-app: command line application to run standalone server and client.
- claudb-junit4: implements a Junit4 compatible @Rule to use in junit4 based tests. [Example](https://github.com/tonivade/claudb/blob/master/junit4/src/test/java/com/github/tonivade/claudb/junit4/TestJunit4Rule.java)
- claudb-junit5: implements a Junit5 compatible extension to use in junit5 based tests. [Example](https://github.com/tonivade/claudb/blob/master/junit5/src/test/java/com/github/tonivade/claudb/junit5/TestJunit5Extension.java)
## Performance
Performance is quite good, not as good as REDIS, but it's good enough for Java.
This is ClauDB
$ redis-benchmark -t set,get -h localhost -p 7081 -n 100000 -q
SET: 82576.38 requests per second, p50=0.303 msec
GET: 93896.71 requests per second, p50=0.287 msec
And this is REDIS
$ redis-benchmark -t set,get -h localhost -p 6379 -n 100000 -q
SET: 148148.14 requests per second, p50=0.167 msec
GET: 147710.48 requests per second, p50=0.175 msec
In my laptop (Intel Core i7-1065G7, with 32G of RAM, running linux)
## BUILD
You need to clon the repo:
$ git clone https://github.com/tonivade/claudb.git
ClauDB uses Gradle as building tool, but you don't need Gradle installed, just type:
$ ./gradlew build
This scripts automatically download Gradle and then runs the tasks.
Or if you have Gradle installed, just type
$ gradle build
Create all-in-one jar
$ ./gradlew fatJar
## DOCKER
You can create your own docker images for ClauDB using the provided `Dockerfile`
$ docker build -t claudb .
And then run the image
$ docker run -p 7081:7081 claudb
## USAGE
You can start a new server listening in default port 7081.
$ wget https://repo1.maven.org/maven2/com/github/tonivade/claudb-app/2.0.1/claudb-app-2.0.1-all.jar
$ java -jar claudb-2.0.1-all.jar
or using [jgo](https://github.com/scijava/jgo) utility
$ jgo com.github.tonivade:claudb-app:2.0.1:com.github.tonivade.claudb.Server
Parameters:
Option Description
------ -----------
-N enable keyspace notifications (experimental)
-O enable off heap memory (experimental)
-P [String: file name] enable persistence (experimental) (default: ./claudb.data)
-V verbose mode
-h <String: host> define listen host (default: localhost)
--help print help
-p <Integer: port> define listen port (default: 7081)
Also you can use inside your project using Maven
<dependency>
<groupId>com.github.tonivade</groupId>
<artifactId>claudb</artifactId>
<version>2.0.1</version>
</dependency>
Or gradle
compile 'com.github.tonivade:claudb:2.0.1'
Or embed in your source code
```java
RespServer server = ClauDB.builder().host("localhost").port(7081).build();
server.start();
```
## Native Image
Now is possible to generate a native image thanks to graalvm. You can generate one with this command:
```shell
$ ./gradlew clean nativeImage
```
Some features are not available like lua runtime and offheap memory.
## TODO
- Ziplist and Maplist encoding not implemented yet.
- Master/Slave replication improvements. Slave with Slaves
- Partitioning?
- Clustering?
- Geo Commands
## Continuous Integration
[](https://github.com/tonivade/claudb/actions/workflows/gradle.yml)
[](https://www.codacy.com/app/tonivade/claudb?utm_source=github.com&utm_medium=referral&utm_content=tonivade/claudb&utm_campaign=Badge_Grade)
[](https://www.codacy.com/app/tonivade/claudb?utm_source=github.com&utm_medium=referral&utm_content=tonivade/claudb&utm_campaign=Badge_Coverage)
[](https://maven-badges.herokuapp.com/maven-central/com.github.tonivade/claudb)
[](https://gitter.im/tonivade/claudb?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
## Stargazers over time
[](https://starchart.cc/tonivade/claudb)
## LICENSE
ClauDB is released under MIT License

徐浪老师
- 粉丝: 8527
- 资源: 1万+
最新资源
- 9-顶刊复现基于球形向量改进的粒子群算法PSO的无人机3D路径规划,spherical vector based particle swarm optimization,MATLAB编写,包含参考文献
- 半桥LLC谐振变器滞环控制仿真,与变频控制作对比 启动过程输出电压更平滑,切载过程滞环控制响应速度更快 管子软开关特性仍能保持 仿真0.1s处为切载过程 第二、三幅图分别为启动和切载时输出电压
- matlab仿真,simulink仿真,以及非线性磁链观测器+PLL 文档推导算法pdf介绍 大名鼎鼎的VESC里面的观测器 对学习非线性观磁链测器有很大帮助,图一为观测位置角度与真实角度波形
- SIEMENS 西门子SIEMENS 西门子西门子水处理程序 包含:1200Plc程序,通讯点表,CAD原理图,操作说明 触摸屏包含:组态画面,操作画面,参数设置画面,报警记录等 程序结构严谨
- DEM数据全国DEM数据 ,仅供学习练习使用,有需要自取,500m分辨率
- 光伏控制器,光伏三相并网仿真 带说明文件,参考文献 模型内容: 1.光伏+MPPT控制+两级式并网逆变器(boost+三相桥式逆变) 2.坐标变+锁相环+dq功率控制+解耦控制+电流内环电压外环控
- 2500线磁编码器方案 电机反馈系统 ,用于交直流伺服和步进闭环,替原来光编方案 原理图和PCB全套文件 支持电机驱动器:迈信EP100、广数DA98、数控SD200A等 增量ABZ差分输出
- 双机并联下垂控制仿真 两台单相全桥逆变器并联,采用下垂控制,可实现调节下垂系数控制功率的分配 波形符合理论值,可用于快速入门学习~
- 16bit高精度逐次逼近型SAR ADC电路设计成品 单端结构原理清晰,加上目前写过的最详细的设计与仿真报告,用来入门学习不成问题 而且各方面性能都很好,不像另外几个单端sar只能学习没有实用性,这
- 四旋翼无人机位置姿态控制 四旋翼无人机仿真 四旋翼无人机动力学模型 包含力方程组与力矩方程组 级联PID控制器 内环姿态环,外环位置环 有参考文献,很详细,简洁易懂 有报告文档直接用
- 热器蒸发器冷凝器管翅式热器三维可编辑模型 这是应用于家用空调系统的热器 格式为.sldprt,需要solidworks2020及以上版本才能打开 各部分零件图也可单独使用,可用于工业设计和仿真
- 基于粒子群算法的综合能源系统优化调度 参考文档:自己整理的模型 matlab 主要内容:综合能源系统中设备包含:风力发电、光伏发电、燃气轮机、蓄电池、燃气锅炉,目标函数已系统运行成本最低,通过粒子群算
- YL-335B自动生产线供料单元MCGS7.7仿真模拟程序T150,带运行效果视频
- dsp 28377d pwm波,adc程序代码模板,已配置3路epwm,4路adc,定时器中断,回调已写好,
- 机械臂仿真,RRT避障算法,六自由度机械臂 机械臂matlab仿真,RRT避障算法,六自由度机械臂避障算法,RRT避障算法,避障仿真,无机械臂关节碰撞机械臂 机器人 DH参数 运动学 正逆解 urd
- 基于变预测时域的MPC自适应轨迹跟踪控制,针对轮胎刚度时变的特点造成控制模型精度降低,基于最小递归二乘法(RLS)估算的轮胎侧偏刚度,提升了模型的控制精度和鲁棒性,通过carsim与simulink联
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


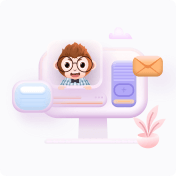